Binary Search Tree within BST
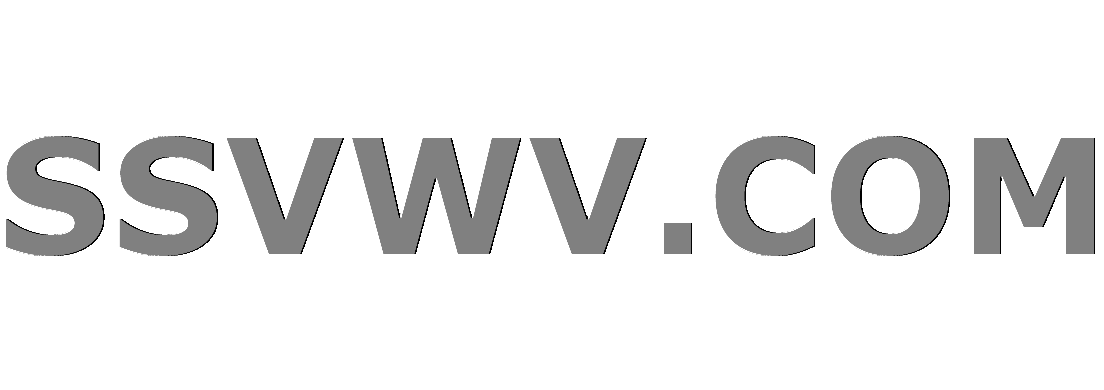
Multi tool use
up vote
0
down vote
favorite
I need to make a BST. However, each node is essentially the root for a binary search tree that can be added to that. The example is:
You have several shoe shops stored in a BST but each shop carries different shoes
Once the shop is made, I don't understand how to use that shop root (node) and make a new tree under each shop that will hold the shoe name and a left and right pointer connecting other shoes. Would I need to make another insert function? I know I'll have to free memory, but for now I am trying to figure out the insertion of the other nodes.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct mainNode
char* name;// Shop Name
struct mainNode* left;
struct mainNode* right;
struct regNode* root; // Pointer To Sub Node
MainTreeNode;
typedef struct regNode
char * name;// Shoe Name
struct regNode *left;
struct regNode *right;
regNode;
MainTreeNode* insert(MainTreeNode* root, char* name);
void inOrder(MainTreeNode* root);
int main()
// Create tree
MainTreeNode* MainTreeRoot = NULL;
MainTreeRoot = insert(MainTreeRoot ,"nike");
MainTreeRoot = insert(MainTreeRoot, "adidas");
MainTreeRoot = insert(MainTreeRoot, "underArmour");
MainTreeRoot = insert(MainTreeRoot, "rebbok");
inOrder(MainTreeRoot);
printf("n");
return 0;
// Inserts a new node into the tree rooted at root with data set to value.
// and returns a pointer to the root of the resulting tree.
MainTreeNode* insert(MainTreeNode* root,char* name)
// Inserting into an empty tree.
if (root == NULL)
MainTreeNode* temp = malloc(sizeof(MainTreeNode));
temp->name = name;
temp->left = NULL;
temp->right = NULL;
temp->root = NULL;
return temp;
// Go left
if (strcmp(name,root->name) < 0)
root->left = insert(root->left,name);
// Go right
else
root->right = insert(root->right, name);
// Must return the root of this tree
return root;
void inOrder(MainTreeNode* root)
if (root != NULL)
inOrder(root->left);
printf("%s n", root->name);
inOrder(root->right);
c
add a comment |
up vote
0
down vote
favorite
I need to make a BST. However, each node is essentially the root for a binary search tree that can be added to that. The example is:
You have several shoe shops stored in a BST but each shop carries different shoes
Once the shop is made, I don't understand how to use that shop root (node) and make a new tree under each shop that will hold the shoe name and a left and right pointer connecting other shoes. Would I need to make another insert function? I know I'll have to free memory, but for now I am trying to figure out the insertion of the other nodes.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct mainNode
char* name;// Shop Name
struct mainNode* left;
struct mainNode* right;
struct regNode* root; // Pointer To Sub Node
MainTreeNode;
typedef struct regNode
char * name;// Shoe Name
struct regNode *left;
struct regNode *right;
regNode;
MainTreeNode* insert(MainTreeNode* root, char* name);
void inOrder(MainTreeNode* root);
int main()
// Create tree
MainTreeNode* MainTreeRoot = NULL;
MainTreeRoot = insert(MainTreeRoot ,"nike");
MainTreeRoot = insert(MainTreeRoot, "adidas");
MainTreeRoot = insert(MainTreeRoot, "underArmour");
MainTreeRoot = insert(MainTreeRoot, "rebbok");
inOrder(MainTreeRoot);
printf("n");
return 0;
// Inserts a new node into the tree rooted at root with data set to value.
// and returns a pointer to the root of the resulting tree.
MainTreeNode* insert(MainTreeNode* root,char* name)
// Inserting into an empty tree.
if (root == NULL)
MainTreeNode* temp = malloc(sizeof(MainTreeNode));
temp->name = name;
temp->left = NULL;
temp->right = NULL;
temp->root = NULL;
return temp;
// Go left
if (strcmp(name,root->name) < 0)
root->left = insert(root->left,name);
// Go right
else
root->right = insert(root->right, name);
// Must return the root of this tree
return root;
void inOrder(MainTreeNode* root)
if (root != NULL)
inOrder(root->left);
printf("%s n", root->name);
inOrder(root->right);
c
Welcome to StackOverflow...but posting code and going "I don't know what to do, just in general" isn't how it works. Please read through the How to Ask section. Basically you have to edit down to a very specific focused question. It may be that getting whatever you want done involves asking several of these focused questions, but the responsibility is on you to do the work of being specific and pointing to the exact roadblock or line of code that you want that question to be about.
– HostileFork
Nov 11 at 4:04
How is the data presented? At the moment, you have shops callednike
,adidas
,underArmour
andreebok
(except you have too many b's and not enough e's). So, if you're presented with anike
shoe calledairJordan
, what are you going to do? Yes, you need a different insert function. What will you do if you're presented with atarget
shoe calledePluribusUnum
?
– Jonathan Leffler
Nov 11 at 5:27
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I need to make a BST. However, each node is essentially the root for a binary search tree that can be added to that. The example is:
You have several shoe shops stored in a BST but each shop carries different shoes
Once the shop is made, I don't understand how to use that shop root (node) and make a new tree under each shop that will hold the shoe name and a left and right pointer connecting other shoes. Would I need to make another insert function? I know I'll have to free memory, but for now I am trying to figure out the insertion of the other nodes.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct mainNode
char* name;// Shop Name
struct mainNode* left;
struct mainNode* right;
struct regNode* root; // Pointer To Sub Node
MainTreeNode;
typedef struct regNode
char * name;// Shoe Name
struct regNode *left;
struct regNode *right;
regNode;
MainTreeNode* insert(MainTreeNode* root, char* name);
void inOrder(MainTreeNode* root);
int main()
// Create tree
MainTreeNode* MainTreeRoot = NULL;
MainTreeRoot = insert(MainTreeRoot ,"nike");
MainTreeRoot = insert(MainTreeRoot, "adidas");
MainTreeRoot = insert(MainTreeRoot, "underArmour");
MainTreeRoot = insert(MainTreeRoot, "rebbok");
inOrder(MainTreeRoot);
printf("n");
return 0;
// Inserts a new node into the tree rooted at root with data set to value.
// and returns a pointer to the root of the resulting tree.
MainTreeNode* insert(MainTreeNode* root,char* name)
// Inserting into an empty tree.
if (root == NULL)
MainTreeNode* temp = malloc(sizeof(MainTreeNode));
temp->name = name;
temp->left = NULL;
temp->right = NULL;
temp->root = NULL;
return temp;
// Go left
if (strcmp(name,root->name) < 0)
root->left = insert(root->left,name);
// Go right
else
root->right = insert(root->right, name);
// Must return the root of this tree
return root;
void inOrder(MainTreeNode* root)
if (root != NULL)
inOrder(root->left);
printf("%s n", root->name);
inOrder(root->right);
c
I need to make a BST. However, each node is essentially the root for a binary search tree that can be added to that. The example is:
You have several shoe shops stored in a BST but each shop carries different shoes
Once the shop is made, I don't understand how to use that shop root (node) and make a new tree under each shop that will hold the shoe name and a left and right pointer connecting other shoes. Would I need to make another insert function? I know I'll have to free memory, but for now I am trying to figure out the insertion of the other nodes.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct mainNode
char* name;// Shop Name
struct mainNode* left;
struct mainNode* right;
struct regNode* root; // Pointer To Sub Node
MainTreeNode;
typedef struct regNode
char * name;// Shoe Name
struct regNode *left;
struct regNode *right;
regNode;
MainTreeNode* insert(MainTreeNode* root, char* name);
void inOrder(MainTreeNode* root);
int main()
// Create tree
MainTreeNode* MainTreeRoot = NULL;
MainTreeRoot = insert(MainTreeRoot ,"nike");
MainTreeRoot = insert(MainTreeRoot, "adidas");
MainTreeRoot = insert(MainTreeRoot, "underArmour");
MainTreeRoot = insert(MainTreeRoot, "rebbok");
inOrder(MainTreeRoot);
printf("n");
return 0;
// Inserts a new node into the tree rooted at root with data set to value.
// and returns a pointer to the root of the resulting tree.
MainTreeNode* insert(MainTreeNode* root,char* name)
// Inserting into an empty tree.
if (root == NULL)
MainTreeNode* temp = malloc(sizeof(MainTreeNode));
temp->name = name;
temp->left = NULL;
temp->right = NULL;
temp->root = NULL;
return temp;
// Go left
if (strcmp(name,root->name) < 0)
root->left = insert(root->left,name);
// Go right
else
root->right = insert(root->right, name);
// Must return the root of this tree
return root;
void inOrder(MainTreeNode* root)
if (root != NULL)
inOrder(root->left);
printf("%s n", root->name);
inOrder(root->right);
c
c
edited Nov 11 at 4:26


Jonathan Leffler
555k886621014
555k886621014
asked Nov 11 at 3:59
Los
1
1
Welcome to StackOverflow...but posting code and going "I don't know what to do, just in general" isn't how it works. Please read through the How to Ask section. Basically you have to edit down to a very specific focused question. It may be that getting whatever you want done involves asking several of these focused questions, but the responsibility is on you to do the work of being specific and pointing to the exact roadblock or line of code that you want that question to be about.
– HostileFork
Nov 11 at 4:04
How is the data presented? At the moment, you have shops callednike
,adidas
,underArmour
andreebok
(except you have too many b's and not enough e's). So, if you're presented with anike
shoe calledairJordan
, what are you going to do? Yes, you need a different insert function. What will you do if you're presented with atarget
shoe calledePluribusUnum
?
– Jonathan Leffler
Nov 11 at 5:27
add a comment |
Welcome to StackOverflow...but posting code and going "I don't know what to do, just in general" isn't how it works. Please read through the How to Ask section. Basically you have to edit down to a very specific focused question. It may be that getting whatever you want done involves asking several of these focused questions, but the responsibility is on you to do the work of being specific and pointing to the exact roadblock or line of code that you want that question to be about.
– HostileFork
Nov 11 at 4:04
How is the data presented? At the moment, you have shops callednike
,adidas
,underArmour
andreebok
(except you have too many b's and not enough e's). So, if you're presented with anike
shoe calledairJordan
, what are you going to do? Yes, you need a different insert function. What will you do if you're presented with atarget
shoe calledePluribusUnum
?
– Jonathan Leffler
Nov 11 at 5:27
Welcome to StackOverflow...but posting code and going "I don't know what to do, just in general" isn't how it works. Please read through the How to Ask section. Basically you have to edit down to a very specific focused question. It may be that getting whatever you want done involves asking several of these focused questions, but the responsibility is on you to do the work of being specific and pointing to the exact roadblock or line of code that you want that question to be about.
– HostileFork
Nov 11 at 4:04
Welcome to StackOverflow...but posting code and going "I don't know what to do, just in general" isn't how it works. Please read through the How to Ask section. Basically you have to edit down to a very specific focused question. It may be that getting whatever you want done involves asking several of these focused questions, but the responsibility is on you to do the work of being specific and pointing to the exact roadblock or line of code that you want that question to be about.
– HostileFork
Nov 11 at 4:04
How is the data presented? At the moment, you have shops called
nike
, adidas
, underArmour
and reebok
(except you have too many b's and not enough e's). So, if you're presented with a nike
shoe called airJordan
, what are you going to do? Yes, you need a different insert function. What will you do if you're presented with a target
shoe called ePluribusUnum
?– Jonathan Leffler
Nov 11 at 5:27
How is the data presented? At the moment, you have shops called
nike
, adidas
, underArmour
and reebok
(except you have too many b's and not enough e's). So, if you're presented with a nike
shoe called airJordan
, what are you going to do? Yes, you need a different insert function. What will you do if you're presented with a target
shoe called ePluribusUnum
?– Jonathan Leffler
Nov 11 at 5:27
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245723%2fbinary-search-tree-within-bst%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7FpjRM UZ,2qeXCExjMmA,Ep0,89t6M9ja,NDW Exbvis,5YLO DH MHxNKLlp,bnomwJjFneO4TnS9Nfg0FB
Welcome to StackOverflow...but posting code and going "I don't know what to do, just in general" isn't how it works. Please read through the How to Ask section. Basically you have to edit down to a very specific focused question. It may be that getting whatever you want done involves asking several of these focused questions, but the responsibility is on you to do the work of being specific and pointing to the exact roadblock or line of code that you want that question to be about.
– HostileFork
Nov 11 at 4:04
How is the data presented? At the moment, you have shops called
nike
,adidas
,underArmour
andreebok
(except you have too many b's and not enough e's). So, if you're presented with anike
shoe calledairJordan
, what are you going to do? Yes, you need a different insert function. What will you do if you're presented with atarget
shoe calledePluribusUnum
?– Jonathan Leffler
Nov 11 at 5:27