How to create a C# generic method
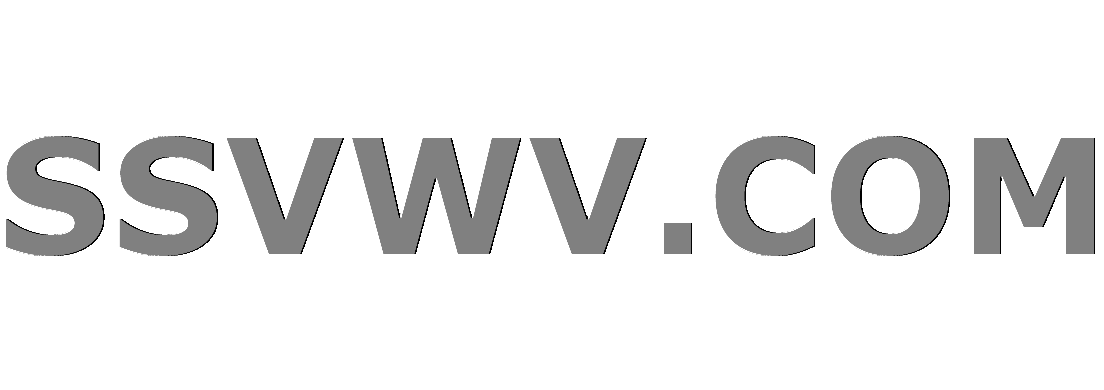
Multi tool use
up vote
0
down vote
favorite
I have a method that I copy paste into many classes. The method hydrates a model into an IdNameViewModel. How do I create a class with this method that I can pass in the repository model and get back a list of IdNames for that model?
Note: ProjectValue is a model/class that I'm passing in for example but it really could be any model/class that that contains an id and name property.
private IdNameVM HydrateEntityToVM(ProjectValue projectValue)
if (projectValue == null) return null;
return new IdNameVM()
Id = projectValue.Id,
Name = projectValue.Name
;
Here is what I came up with based on the answers below:
public class GenericHydrateIdName<TModel> where TModel : INameId
public IdNameVM HydrateEntityToVM(TModel model)
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
}
Just a note if anyone is following, the class above caused way too much work and violates the Clean Code Principle. I used the extension method below.
c# generics
add a comment |
up vote
0
down vote
favorite
I have a method that I copy paste into many classes. The method hydrates a model into an IdNameViewModel. How do I create a class with this method that I can pass in the repository model and get back a list of IdNames for that model?
Note: ProjectValue is a model/class that I'm passing in for example but it really could be any model/class that that contains an id and name property.
private IdNameVM HydrateEntityToVM(ProjectValue projectValue)
if (projectValue == null) return null;
return new IdNameVM()
Id = projectValue.Id,
Name = projectValue.Name
;
Here is what I came up with based on the answers below:
public class GenericHydrateIdName<TModel> where TModel : INameId
public IdNameVM HydrateEntityToVM(TModel model)
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
}
Just a note if anyone is following, the class above caused way too much work and violates the Clean Code Principle. I used the extension method below.
c# generics
I didn't get the point of the class. It makes you enter your type each time too
– Neville Nazerane
Nov 10 at 18:27
@NevilleNazerane I agree, this morning I realized that an extension method would be better and less code (Clean Code). Thanks! I will update my post when I'm done.
– Jim Kiely
Nov 11 at 16:14
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a method that I copy paste into many classes. The method hydrates a model into an IdNameViewModel. How do I create a class with this method that I can pass in the repository model and get back a list of IdNames for that model?
Note: ProjectValue is a model/class that I'm passing in for example but it really could be any model/class that that contains an id and name property.
private IdNameVM HydrateEntityToVM(ProjectValue projectValue)
if (projectValue == null) return null;
return new IdNameVM()
Id = projectValue.Id,
Name = projectValue.Name
;
Here is what I came up with based on the answers below:
public class GenericHydrateIdName<TModel> where TModel : INameId
public IdNameVM HydrateEntityToVM(TModel model)
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
}
Just a note if anyone is following, the class above caused way too much work and violates the Clean Code Principle. I used the extension method below.
c# generics
I have a method that I copy paste into many classes. The method hydrates a model into an IdNameViewModel. How do I create a class with this method that I can pass in the repository model and get back a list of IdNames for that model?
Note: ProjectValue is a model/class that I'm passing in for example but it really could be any model/class that that contains an id and name property.
private IdNameVM HydrateEntityToVM(ProjectValue projectValue)
if (projectValue == null) return null;
return new IdNameVM()
Id = projectValue.Id,
Name = projectValue.Name
;
Here is what I came up with based on the answers below:
public class GenericHydrateIdName<TModel> where TModel : INameId
public IdNameVM HydrateEntityToVM(TModel model)
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
}
Just a note if anyone is following, the class above caused way too much work and violates the Clean Code Principle. I used the extension method below.
c# generics
c# generics
edited Nov 11 at 16:24
asked Nov 10 at 17:08
Jim Kiely
81116
81116
I didn't get the point of the class. It makes you enter your type each time too
– Neville Nazerane
Nov 10 at 18:27
@NevilleNazerane I agree, this morning I realized that an extension method would be better and less code (Clean Code). Thanks! I will update my post when I'm done.
– Jim Kiely
Nov 11 at 16:14
add a comment |
I didn't get the point of the class. It makes you enter your type each time too
– Neville Nazerane
Nov 10 at 18:27
@NevilleNazerane I agree, this morning I realized that an extension method would be better and less code (Clean Code). Thanks! I will update my post when I'm done.
– Jim Kiely
Nov 11 at 16:14
I didn't get the point of the class. It makes you enter your type each time too
– Neville Nazerane
Nov 10 at 18:27
I didn't get the point of the class. It makes you enter your type each time too
– Neville Nazerane
Nov 10 at 18:27
@NevilleNazerane I agree, this morning I realized that an extension method would be better and less code (Clean Code). Thanks! I will update my post when I'm done.
– Jim Kiely
Nov 11 at 16:14
@NevilleNazerane I agree, this morning I realized that an extension method would be better and less code (Clean Code). Thanks! I will update my post when I'm done.
– Jim Kiely
Nov 11 at 16:14
add a comment |
4 Answers
4
active
oldest
votes
up vote
2
down vote
accepted
Basically, the best type-safe way I can see is having an interface.
interface INameId
int Id get; set;
string Name get; set;
You can have this interface in every class you would like to use it in. Based on your current usage, I would suggest an extension function
static class VMExtensions
public static IdNameVM HydrateEntityToVM<TModel>(this TModel model)
where TModel : INameId
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
// update: without generics as Marcus Höglund pointed out
public static IdNameVM HydrateEntityToVM2(this INameId model)
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
static void Test()
var model = new ProjectValue();
var model2 = new AnotherModel();
var viewModel = model2.HydrateEntityToVM();
var viewModel2 = model2.HydrateEntityToVM();
If you don't want to add the interface to all your models, you can use reflection (but note that it would be really slow).
Update
As Marcus pointed out, In this case, there is no real point of using generics in the function. You can use the HydrateEntityToVM2
implementation that directly uses the interface. However, in a future point if you want to use something like IdNameVM<TModel>
so you can still access the original model if required, HydrateEntityToVM
function with generics will be helpful.
What about passing an anonymous object as new Id = 1, Name = "abc"
– Hassaan
Nov 10 at 17:55
I like this method and I think it is a lot closer to the answer I was looking for.
– Jim Kiely
Nov 10 at 17:56
@Hassaan OP already has a classIdNameVM
which I am guessing he is using in his views
– Neville Nazerane
Nov 10 at 17:59
1
+1 for pointing out the type-safe and the extension. But, IMO it makes more sence to just extend the interface instead of putting a type constraint, HydrateEntityToVM(this INameId model)
– Marcus Höglund
Nov 10 at 18:14
1
@MarcusHöglund this is not the first time I have done something very similar and stupid without realizing I can just use the interface. Thanks
– Neville Nazerane
Nov 10 at 18:17
|
show 2 more comments
up vote
0
down vote
For creating a Generic Method, you have to keep in mind the few rules
- What Types you are going to support
- What will be the resultant
- There must be singularity between the solutions
Let's take the above mentioned example: You need a generic method of name HydrateEntityToVM
. The very first step is the declaration syntax of the method along and object casting from T type of Strong Type.
Point to be noted here is you are passing a T type and want a resultant to be always IdNameVM class then your implementation should be as:
//The Method declaration of T type
private IdNameVM HydrateEntityToVM<T>(T projectValue)
//Checking whether the object exists or not
//if null than return the default value of IdNameVM
if(projectValue == null) return default(IdNameVM);
//Checking if we are supporting the implementation or not for that class
//In your case YourStrongTypeClass is ProjectValue Class
if(projectValue is YourStrongTypeClass)
//Casting the T object to strong type object
var obj = projectValue as YourStrongTypeClass;
return new IdNameVM()
Id = obj.Id,
Name = obj.Name
;
else
//The else statement is for if you want to handle some other type of T implementation
throw new NotImplementedException($"The type typeof(T) is not implemented");
you can useprojectValue is YourStrongTypeClass obj
directly and you will not need the next line if it is the latest c#. Visual studio would suggest this as well
– Neville Nazerane
Nov 10 at 17:34
just to keep in mind not everyone is aware of c# 7.0. We have to carry the basics too
– Hassaan
Nov 10 at 17:35
ok i am not editing the answer in that case, although i don't think we can keep using older syntax considering how long c# 7.0 has been around
– Neville Nazerane
Nov 10 at 17:38
your code doesn't really make much use of the generic by the way. You can get the type directly from the projectValue. It looks like generics is just forced into it.object projectValue
can do the same
– Neville Nazerane
Nov 10 at 17:40
If I do not know what the class/model that is being passed in how do I know what to cast the object as?
– Jim Kiely
Nov 10 at 17:44
|
show 3 more comments
up vote
0
down vote
these are the basics of C#. take a look at this link
class MyClass<T> where T : ProjectValue
private IdNameVM HydrateEntityToVM(T projectValue)
if (projectValue == null) return null;
return new IdNameVM()
Id = projectValue.Id,
Name = projectValue.Name
;
in case if you don't know the abstraction of model (but be careful , this method can consume any object containing id and name public properties) , then try the following:
public void SomeGenericMethod<T>(T model) where T : class
propName == null)
return;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
1
anyone wants to post the reason for the downvote?
– Neville Nazerane
Nov 10 at 17:27
yeah , it because a gave example of generic method declared with reference parameters
– Z.R.T.
Nov 10 at 17:29
use reflection if you don't know the basic class of your models
– Z.R.T.
Nov 10 at 18:02
add a comment |
up vote
0
down vote
I like Neville Nazerane's answer because removes the need for reflection. However, if you did want to do this using reflection then below is a workable way that constructs the result even if it cannot find matching Id or Name properties.
Please note that in this sample I have not added any checking on whether the types for the Id and Name properties match between T and TResult.
public static TResult HydrateEntityToVM<T, TResult>(T inputValue, TResult resultValue) where T : class
if (inputValue == null
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Basically, the best type-safe way I can see is having an interface.
interface INameId
int Id get; set;
string Name get; set;
You can have this interface in every class you would like to use it in. Based on your current usage, I would suggest an extension function
static class VMExtensions
public static IdNameVM HydrateEntityToVM<TModel>(this TModel model)
where TModel : INameId
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
// update: without generics as Marcus Höglund pointed out
public static IdNameVM HydrateEntityToVM2(this INameId model)
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
static void Test()
var model = new ProjectValue();
var model2 = new AnotherModel();
var viewModel = model2.HydrateEntityToVM();
var viewModel2 = model2.HydrateEntityToVM();
If you don't want to add the interface to all your models, you can use reflection (but note that it would be really slow).
Update
As Marcus pointed out, In this case, there is no real point of using generics in the function. You can use the HydrateEntityToVM2
implementation that directly uses the interface. However, in a future point if you want to use something like IdNameVM<TModel>
so you can still access the original model if required, HydrateEntityToVM
function with generics will be helpful.
What about passing an anonymous object as new Id = 1, Name = "abc"
– Hassaan
Nov 10 at 17:55
I like this method and I think it is a lot closer to the answer I was looking for.
– Jim Kiely
Nov 10 at 17:56
@Hassaan OP already has a classIdNameVM
which I am guessing he is using in his views
– Neville Nazerane
Nov 10 at 17:59
1
+1 for pointing out the type-safe and the extension. But, IMO it makes more sence to just extend the interface instead of putting a type constraint, HydrateEntityToVM(this INameId model)
– Marcus Höglund
Nov 10 at 18:14
1
@MarcusHöglund this is not the first time I have done something very similar and stupid without realizing I can just use the interface. Thanks
– Neville Nazerane
Nov 10 at 18:17
|
show 2 more comments
up vote
2
down vote
accepted
Basically, the best type-safe way I can see is having an interface.
interface INameId
int Id get; set;
string Name get; set;
You can have this interface in every class you would like to use it in. Based on your current usage, I would suggest an extension function
static class VMExtensions
public static IdNameVM HydrateEntityToVM<TModel>(this TModel model)
where TModel : INameId
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
// update: without generics as Marcus Höglund pointed out
public static IdNameVM HydrateEntityToVM2(this INameId model)
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
static void Test()
var model = new ProjectValue();
var model2 = new AnotherModel();
var viewModel = model2.HydrateEntityToVM();
var viewModel2 = model2.HydrateEntityToVM();
If you don't want to add the interface to all your models, you can use reflection (but note that it would be really slow).
Update
As Marcus pointed out, In this case, there is no real point of using generics in the function. You can use the HydrateEntityToVM2
implementation that directly uses the interface. However, in a future point if you want to use something like IdNameVM<TModel>
so you can still access the original model if required, HydrateEntityToVM
function with generics will be helpful.
What about passing an anonymous object as new Id = 1, Name = "abc"
– Hassaan
Nov 10 at 17:55
I like this method and I think it is a lot closer to the answer I was looking for.
– Jim Kiely
Nov 10 at 17:56
@Hassaan OP already has a classIdNameVM
which I am guessing he is using in his views
– Neville Nazerane
Nov 10 at 17:59
1
+1 for pointing out the type-safe and the extension. But, IMO it makes more sence to just extend the interface instead of putting a type constraint, HydrateEntityToVM(this INameId model)
– Marcus Höglund
Nov 10 at 18:14
1
@MarcusHöglund this is not the first time I have done something very similar and stupid without realizing I can just use the interface. Thanks
– Neville Nazerane
Nov 10 at 18:17
|
show 2 more comments
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Basically, the best type-safe way I can see is having an interface.
interface INameId
int Id get; set;
string Name get; set;
You can have this interface in every class you would like to use it in. Based on your current usage, I would suggest an extension function
static class VMExtensions
public static IdNameVM HydrateEntityToVM<TModel>(this TModel model)
where TModel : INameId
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
// update: without generics as Marcus Höglund pointed out
public static IdNameVM HydrateEntityToVM2(this INameId model)
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
static void Test()
var model = new ProjectValue();
var model2 = new AnotherModel();
var viewModel = model2.HydrateEntityToVM();
var viewModel2 = model2.HydrateEntityToVM();
If you don't want to add the interface to all your models, you can use reflection (but note that it would be really slow).
Update
As Marcus pointed out, In this case, there is no real point of using generics in the function. You can use the HydrateEntityToVM2
implementation that directly uses the interface. However, in a future point if you want to use something like IdNameVM<TModel>
so you can still access the original model if required, HydrateEntityToVM
function with generics will be helpful.
Basically, the best type-safe way I can see is having an interface.
interface INameId
int Id get; set;
string Name get; set;
You can have this interface in every class you would like to use it in. Based on your current usage, I would suggest an extension function
static class VMExtensions
public static IdNameVM HydrateEntityToVM<TModel>(this TModel model)
where TModel : INameId
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
// update: without generics as Marcus Höglund pointed out
public static IdNameVM HydrateEntityToVM2(this INameId model)
if (model == null) return null;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
static void Test()
var model = new ProjectValue();
var model2 = new AnotherModel();
var viewModel = model2.HydrateEntityToVM();
var viewModel2 = model2.HydrateEntityToVM();
If you don't want to add the interface to all your models, you can use reflection (but note that it would be really slow).
Update
As Marcus pointed out, In this case, there is no real point of using generics in the function. You can use the HydrateEntityToVM2
implementation that directly uses the interface. However, in a future point if you want to use something like IdNameVM<TModel>
so you can still access the original model if required, HydrateEntityToVM
function with generics will be helpful.
edited Nov 10 at 18:24
answered Nov 10 at 17:52
Neville Nazerane
2,25111433
2,25111433
What about passing an anonymous object as new Id = 1, Name = "abc"
– Hassaan
Nov 10 at 17:55
I like this method and I think it is a lot closer to the answer I was looking for.
– Jim Kiely
Nov 10 at 17:56
@Hassaan OP already has a classIdNameVM
which I am guessing he is using in his views
– Neville Nazerane
Nov 10 at 17:59
1
+1 for pointing out the type-safe and the extension. But, IMO it makes more sence to just extend the interface instead of putting a type constraint, HydrateEntityToVM(this INameId model)
– Marcus Höglund
Nov 10 at 18:14
1
@MarcusHöglund this is not the first time I have done something very similar and stupid without realizing I can just use the interface. Thanks
– Neville Nazerane
Nov 10 at 18:17
|
show 2 more comments
What about passing an anonymous object as new Id = 1, Name = "abc"
– Hassaan
Nov 10 at 17:55
I like this method and I think it is a lot closer to the answer I was looking for.
– Jim Kiely
Nov 10 at 17:56
@Hassaan OP already has a classIdNameVM
which I am guessing he is using in his views
– Neville Nazerane
Nov 10 at 17:59
1
+1 for pointing out the type-safe and the extension. But, IMO it makes more sence to just extend the interface instead of putting a type constraint, HydrateEntityToVM(this INameId model)
– Marcus Höglund
Nov 10 at 18:14
1
@MarcusHöglund this is not the first time I have done something very similar and stupid without realizing I can just use the interface. Thanks
– Neville Nazerane
Nov 10 at 18:17
What about passing an anonymous object as new Id = 1, Name = "abc"
– Hassaan
Nov 10 at 17:55
What about passing an anonymous object as new Id = 1, Name = "abc"
– Hassaan
Nov 10 at 17:55
I like this method and I think it is a lot closer to the answer I was looking for.
– Jim Kiely
Nov 10 at 17:56
I like this method and I think it is a lot closer to the answer I was looking for.
– Jim Kiely
Nov 10 at 17:56
@Hassaan OP already has a class
IdNameVM
which I am guessing he is using in his views– Neville Nazerane
Nov 10 at 17:59
@Hassaan OP already has a class
IdNameVM
which I am guessing he is using in his views– Neville Nazerane
Nov 10 at 17:59
1
1
+1 for pointing out the type-safe and the extension. But, IMO it makes more sence to just extend the interface instead of putting a type constraint, HydrateEntityToVM(this INameId model)
– Marcus Höglund
Nov 10 at 18:14
+1 for pointing out the type-safe and the extension. But, IMO it makes more sence to just extend the interface instead of putting a type constraint, HydrateEntityToVM(this INameId model)
– Marcus Höglund
Nov 10 at 18:14
1
1
@MarcusHöglund this is not the first time I have done something very similar and stupid without realizing I can just use the interface. Thanks
– Neville Nazerane
Nov 10 at 18:17
@MarcusHöglund this is not the first time I have done something very similar and stupid without realizing I can just use the interface. Thanks
– Neville Nazerane
Nov 10 at 18:17
|
show 2 more comments
up vote
0
down vote
For creating a Generic Method, you have to keep in mind the few rules
- What Types you are going to support
- What will be the resultant
- There must be singularity between the solutions
Let's take the above mentioned example: You need a generic method of name HydrateEntityToVM
. The very first step is the declaration syntax of the method along and object casting from T type of Strong Type.
Point to be noted here is you are passing a T type and want a resultant to be always IdNameVM class then your implementation should be as:
//The Method declaration of T type
private IdNameVM HydrateEntityToVM<T>(T projectValue)
//Checking whether the object exists or not
//if null than return the default value of IdNameVM
if(projectValue == null) return default(IdNameVM);
//Checking if we are supporting the implementation or not for that class
//In your case YourStrongTypeClass is ProjectValue Class
if(projectValue is YourStrongTypeClass)
//Casting the T object to strong type object
var obj = projectValue as YourStrongTypeClass;
return new IdNameVM()
Id = obj.Id,
Name = obj.Name
;
else
//The else statement is for if you want to handle some other type of T implementation
throw new NotImplementedException($"The type typeof(T) is not implemented");
you can useprojectValue is YourStrongTypeClass obj
directly and you will not need the next line if it is the latest c#. Visual studio would suggest this as well
– Neville Nazerane
Nov 10 at 17:34
just to keep in mind not everyone is aware of c# 7.0. We have to carry the basics too
– Hassaan
Nov 10 at 17:35
ok i am not editing the answer in that case, although i don't think we can keep using older syntax considering how long c# 7.0 has been around
– Neville Nazerane
Nov 10 at 17:38
your code doesn't really make much use of the generic by the way. You can get the type directly from the projectValue. It looks like generics is just forced into it.object projectValue
can do the same
– Neville Nazerane
Nov 10 at 17:40
If I do not know what the class/model that is being passed in how do I know what to cast the object as?
– Jim Kiely
Nov 10 at 17:44
|
show 3 more comments
up vote
0
down vote
For creating a Generic Method, you have to keep in mind the few rules
- What Types you are going to support
- What will be the resultant
- There must be singularity between the solutions
Let's take the above mentioned example: You need a generic method of name HydrateEntityToVM
. The very first step is the declaration syntax of the method along and object casting from T type of Strong Type.
Point to be noted here is you are passing a T type and want a resultant to be always IdNameVM class then your implementation should be as:
//The Method declaration of T type
private IdNameVM HydrateEntityToVM<T>(T projectValue)
//Checking whether the object exists or not
//if null than return the default value of IdNameVM
if(projectValue == null) return default(IdNameVM);
//Checking if we are supporting the implementation or not for that class
//In your case YourStrongTypeClass is ProjectValue Class
if(projectValue is YourStrongTypeClass)
//Casting the T object to strong type object
var obj = projectValue as YourStrongTypeClass;
return new IdNameVM()
Id = obj.Id,
Name = obj.Name
;
else
//The else statement is for if you want to handle some other type of T implementation
throw new NotImplementedException($"The type typeof(T) is not implemented");
you can useprojectValue is YourStrongTypeClass obj
directly and you will not need the next line if it is the latest c#. Visual studio would suggest this as well
– Neville Nazerane
Nov 10 at 17:34
just to keep in mind not everyone is aware of c# 7.0. We have to carry the basics too
– Hassaan
Nov 10 at 17:35
ok i am not editing the answer in that case, although i don't think we can keep using older syntax considering how long c# 7.0 has been around
– Neville Nazerane
Nov 10 at 17:38
your code doesn't really make much use of the generic by the way. You can get the type directly from the projectValue. It looks like generics is just forced into it.object projectValue
can do the same
– Neville Nazerane
Nov 10 at 17:40
If I do not know what the class/model that is being passed in how do I know what to cast the object as?
– Jim Kiely
Nov 10 at 17:44
|
show 3 more comments
up vote
0
down vote
up vote
0
down vote
For creating a Generic Method, you have to keep in mind the few rules
- What Types you are going to support
- What will be the resultant
- There must be singularity between the solutions
Let's take the above mentioned example: You need a generic method of name HydrateEntityToVM
. The very first step is the declaration syntax of the method along and object casting from T type of Strong Type.
Point to be noted here is you are passing a T type and want a resultant to be always IdNameVM class then your implementation should be as:
//The Method declaration of T type
private IdNameVM HydrateEntityToVM<T>(T projectValue)
//Checking whether the object exists or not
//if null than return the default value of IdNameVM
if(projectValue == null) return default(IdNameVM);
//Checking if we are supporting the implementation or not for that class
//In your case YourStrongTypeClass is ProjectValue Class
if(projectValue is YourStrongTypeClass)
//Casting the T object to strong type object
var obj = projectValue as YourStrongTypeClass;
return new IdNameVM()
Id = obj.Id,
Name = obj.Name
;
else
//The else statement is for if you want to handle some other type of T implementation
throw new NotImplementedException($"The type typeof(T) is not implemented");
For creating a Generic Method, you have to keep in mind the few rules
- What Types you are going to support
- What will be the resultant
- There must be singularity between the solutions
Let's take the above mentioned example: You need a generic method of name HydrateEntityToVM
. The very first step is the declaration syntax of the method along and object casting from T type of Strong Type.
Point to be noted here is you are passing a T type and want a resultant to be always IdNameVM class then your implementation should be as:
//The Method declaration of T type
private IdNameVM HydrateEntityToVM<T>(T projectValue)
//Checking whether the object exists or not
//if null than return the default value of IdNameVM
if(projectValue == null) return default(IdNameVM);
//Checking if we are supporting the implementation or not for that class
//In your case YourStrongTypeClass is ProjectValue Class
if(projectValue is YourStrongTypeClass)
//Casting the T object to strong type object
var obj = projectValue as YourStrongTypeClass;
return new IdNameVM()
Id = obj.Id,
Name = obj.Name
;
else
//The else statement is for if you want to handle some other type of T implementation
throw new NotImplementedException($"The type typeof(T) is not implemented");
answered Nov 10 at 17:33


Hassaan
1,75082149
1,75082149
you can useprojectValue is YourStrongTypeClass obj
directly and you will not need the next line if it is the latest c#. Visual studio would suggest this as well
– Neville Nazerane
Nov 10 at 17:34
just to keep in mind not everyone is aware of c# 7.0. We have to carry the basics too
– Hassaan
Nov 10 at 17:35
ok i am not editing the answer in that case, although i don't think we can keep using older syntax considering how long c# 7.0 has been around
– Neville Nazerane
Nov 10 at 17:38
your code doesn't really make much use of the generic by the way. You can get the type directly from the projectValue. It looks like generics is just forced into it.object projectValue
can do the same
– Neville Nazerane
Nov 10 at 17:40
If I do not know what the class/model that is being passed in how do I know what to cast the object as?
– Jim Kiely
Nov 10 at 17:44
|
show 3 more comments
you can useprojectValue is YourStrongTypeClass obj
directly and you will not need the next line if it is the latest c#. Visual studio would suggest this as well
– Neville Nazerane
Nov 10 at 17:34
just to keep in mind not everyone is aware of c# 7.0. We have to carry the basics too
– Hassaan
Nov 10 at 17:35
ok i am not editing the answer in that case, although i don't think we can keep using older syntax considering how long c# 7.0 has been around
– Neville Nazerane
Nov 10 at 17:38
your code doesn't really make much use of the generic by the way. You can get the type directly from the projectValue. It looks like generics is just forced into it.object projectValue
can do the same
– Neville Nazerane
Nov 10 at 17:40
If I do not know what the class/model that is being passed in how do I know what to cast the object as?
– Jim Kiely
Nov 10 at 17:44
you can use
projectValue is YourStrongTypeClass obj
directly and you will not need the next line if it is the latest c#. Visual studio would suggest this as well– Neville Nazerane
Nov 10 at 17:34
you can use
projectValue is YourStrongTypeClass obj
directly and you will not need the next line if it is the latest c#. Visual studio would suggest this as well– Neville Nazerane
Nov 10 at 17:34
just to keep in mind not everyone is aware of c# 7.0. We have to carry the basics too
– Hassaan
Nov 10 at 17:35
just to keep in mind not everyone is aware of c# 7.0. We have to carry the basics too
– Hassaan
Nov 10 at 17:35
ok i am not editing the answer in that case, although i don't think we can keep using older syntax considering how long c# 7.0 has been around
– Neville Nazerane
Nov 10 at 17:38
ok i am not editing the answer in that case, although i don't think we can keep using older syntax considering how long c# 7.0 has been around
– Neville Nazerane
Nov 10 at 17:38
your code doesn't really make much use of the generic by the way. You can get the type directly from the projectValue. It looks like generics is just forced into it.
object projectValue
can do the same– Neville Nazerane
Nov 10 at 17:40
your code doesn't really make much use of the generic by the way. You can get the type directly from the projectValue. It looks like generics is just forced into it.
object projectValue
can do the same– Neville Nazerane
Nov 10 at 17:40
If I do not know what the class/model that is being passed in how do I know what to cast the object as?
– Jim Kiely
Nov 10 at 17:44
If I do not know what the class/model that is being passed in how do I know what to cast the object as?
– Jim Kiely
Nov 10 at 17:44
|
show 3 more comments
up vote
0
down vote
these are the basics of C#. take a look at this link
class MyClass<T> where T : ProjectValue
private IdNameVM HydrateEntityToVM(T projectValue)
if (projectValue == null) return null;
return new IdNameVM()
Id = projectValue.Id,
Name = projectValue.Name
;
in case if you don't know the abstraction of model (but be careful , this method can consume any object containing id and name public properties) , then try the following:
public void SomeGenericMethod<T>(T model) where T : class
propName == null)
return;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
1
anyone wants to post the reason for the downvote?
– Neville Nazerane
Nov 10 at 17:27
yeah , it because a gave example of generic method declared with reference parameters
– Z.R.T.
Nov 10 at 17:29
use reflection if you don't know the basic class of your models
– Z.R.T.
Nov 10 at 18:02
add a comment |
up vote
0
down vote
these are the basics of C#. take a look at this link
class MyClass<T> where T : ProjectValue
private IdNameVM HydrateEntityToVM(T projectValue)
if (projectValue == null) return null;
return new IdNameVM()
Id = projectValue.Id,
Name = projectValue.Name
;
in case if you don't know the abstraction of model (but be careful , this method can consume any object containing id and name public properties) , then try the following:
public void SomeGenericMethod<T>(T model) where T : class
propName == null)
return;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
1
anyone wants to post the reason for the downvote?
– Neville Nazerane
Nov 10 at 17:27
yeah , it because a gave example of generic method declared with reference parameters
– Z.R.T.
Nov 10 at 17:29
use reflection if you don't know the basic class of your models
– Z.R.T.
Nov 10 at 18:02
add a comment |
up vote
0
down vote
up vote
0
down vote
these are the basics of C#. take a look at this link
class MyClass<T> where T : ProjectValue
private IdNameVM HydrateEntityToVM(T projectValue)
if (projectValue == null) return null;
return new IdNameVM()
Id = projectValue.Id,
Name = projectValue.Name
;
in case if you don't know the abstraction of model (but be careful , this method can consume any object containing id and name public properties) , then try the following:
public void SomeGenericMethod<T>(T model) where T : class
propName == null)
return;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
these are the basics of C#. take a look at this link
class MyClass<T> where T : ProjectValue
private IdNameVM HydrateEntityToVM(T projectValue)
if (projectValue == null) return null;
return new IdNameVM()
Id = projectValue.Id,
Name = projectValue.Name
;
in case if you don't know the abstraction of model (but be careful , this method can consume any object containing id and name public properties) , then try the following:
public void SomeGenericMethod<T>(T model) where T : class
propName == null)
return;
return new IdNameVM()
Id = model.Id,
Name = model.Name
;
edited Nov 10 at 18:06
answered Nov 10 at 17:12
Z.R.T.
1,026511
1,026511
1
anyone wants to post the reason for the downvote?
– Neville Nazerane
Nov 10 at 17:27
yeah , it because a gave example of generic method declared with reference parameters
– Z.R.T.
Nov 10 at 17:29
use reflection if you don't know the basic class of your models
– Z.R.T.
Nov 10 at 18:02
add a comment |
1
anyone wants to post the reason for the downvote?
– Neville Nazerane
Nov 10 at 17:27
yeah , it because a gave example of generic method declared with reference parameters
– Z.R.T.
Nov 10 at 17:29
use reflection if you don't know the basic class of your models
– Z.R.T.
Nov 10 at 18:02
1
1
anyone wants to post the reason for the downvote?
– Neville Nazerane
Nov 10 at 17:27
anyone wants to post the reason for the downvote?
– Neville Nazerane
Nov 10 at 17:27
yeah , it because a gave example of generic method declared with reference parameters
– Z.R.T.
Nov 10 at 17:29
yeah , it because a gave example of generic method declared with reference parameters
– Z.R.T.
Nov 10 at 17:29
use reflection if you don't know the basic class of your models
– Z.R.T.
Nov 10 at 18:02
use reflection if you don't know the basic class of your models
– Z.R.T.
Nov 10 at 18:02
add a comment |
up vote
0
down vote
I like Neville Nazerane's answer because removes the need for reflection. However, if you did want to do this using reflection then below is a workable way that constructs the result even if it cannot find matching Id or Name properties.
Please note that in this sample I have not added any checking on whether the types for the Id and Name properties match between T and TResult.
public static TResult HydrateEntityToVM<T, TResult>(T inputValue, TResult resultValue) where T : class
if (inputValue == null
add a comment |
up vote
0
down vote
I like Neville Nazerane's answer because removes the need for reflection. However, if you did want to do this using reflection then below is a workable way that constructs the result even if it cannot find matching Id or Name properties.
Please note that in this sample I have not added any checking on whether the types for the Id and Name properties match between T and TResult.
public static TResult HydrateEntityToVM<T, TResult>(T inputValue, TResult resultValue) where T : class
if (inputValue == null
add a comment |
up vote
0
down vote
up vote
0
down vote
I like Neville Nazerane's answer because removes the need for reflection. However, if you did want to do this using reflection then below is a workable way that constructs the result even if it cannot find matching Id or Name properties.
Please note that in this sample I have not added any checking on whether the types for the Id and Name properties match between T and TResult.
public static TResult HydrateEntityToVM<T, TResult>(T inputValue, TResult resultValue) where T : class
if (inputValue == null
I like Neville Nazerane's answer because removes the need for reflection. However, if you did want to do this using reflection then below is a workable way that constructs the result even if it cannot find matching Id or Name properties.
Please note that in this sample I have not added any checking on whether the types for the Id and Name properties match between T and TResult.
public static TResult HydrateEntityToVM<T, TResult>(T inputValue, TResult resultValue) where T : class
if (inputValue == null
answered Nov 10 at 18:09
Sirgrowns
516
516
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241360%2fhow-to-create-a-c-sharp-generic-method%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
b4QoDavu,RtZ8F
I didn't get the point of the class. It makes you enter your type each time too
– Neville Nazerane
Nov 10 at 18:27
@NevilleNazerane I agree, this morning I realized that an extension method would be better and less code (Clean Code). Thanks! I will update my post when I'm done.
– Jim Kiely
Nov 11 at 16:14