Spring Tool Suite- referencing projects
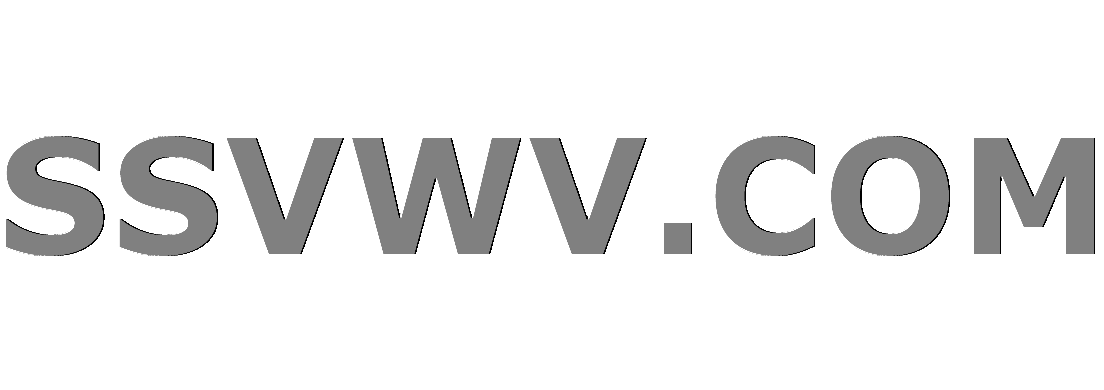
Multi tool use
I come from a C#Visual Studio background and can't wrap my head around how to reference project B from project A such that when I do a Maven build, it actually builds. Using Spring Tool Suite:
- In Project A, Properties> Java Build Path > Projects, I've added Project B.
- Project B builds via Maven just fine as a package.
- Lovely, intellisense works and I'm happily coding along.
- I go to do a Maven build on project A and get a whole bunch of
[ERROR]: ...package [project B] does not exist.
I get that somehow i need to make Maven aware of project B's jar...but I don't understand how this works. I don't think i want to force the jar into my local Maven repo (if i do, then what happens when i use the company's maven repo?), i really just want to have maven pick up the .class files from project B's build and be on my merry way. What am I missing?
EDIT: adding poms:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.firm</groupId>
<artifactId>projectA</artifactId>
<packaging>jar</packaging>
<version>0.0.2-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
</parent>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<executable>true</executable>
</configuration>
</plugin>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20090211</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.13</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
POM 2:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.firm</groupId>
<artifactId>projectB</artifactId>
<packaging>jar</packaging>
<version>0.0.1-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
</parent>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20090211</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.ldap</groupId>
<artifactId>spring-ldap-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
java spring maven
add a comment |
I come from a C#Visual Studio background and can't wrap my head around how to reference project B from project A such that when I do a Maven build, it actually builds. Using Spring Tool Suite:
- In Project A, Properties> Java Build Path > Projects, I've added Project B.
- Project B builds via Maven just fine as a package.
- Lovely, intellisense works and I'm happily coding along.
- I go to do a Maven build on project A and get a whole bunch of
[ERROR]: ...package [project B] does not exist.
I get that somehow i need to make Maven aware of project B's jar...but I don't understand how this works. I don't think i want to force the jar into my local Maven repo (if i do, then what happens when i use the company's maven repo?), i really just want to have maven pick up the .class files from project B's build and be on my merry way. What am I missing?
EDIT: adding poms:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.firm</groupId>
<artifactId>projectA</artifactId>
<packaging>jar</packaging>
<version>0.0.2-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
</parent>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<executable>true</executable>
</configuration>
</plugin>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20090211</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.13</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
POM 2:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.firm</groupId>
<artifactId>projectB</artifactId>
<packaging>jar</packaging>
<version>0.0.1-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
</parent>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20090211</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.ldap</groupId>
<artifactId>spring-ldap-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
java spring maven
add a comment |
I come from a C#Visual Studio background and can't wrap my head around how to reference project B from project A such that when I do a Maven build, it actually builds. Using Spring Tool Suite:
- In Project A, Properties> Java Build Path > Projects, I've added Project B.
- Project B builds via Maven just fine as a package.
- Lovely, intellisense works and I'm happily coding along.
- I go to do a Maven build on project A and get a whole bunch of
[ERROR]: ...package [project B] does not exist.
I get that somehow i need to make Maven aware of project B's jar...but I don't understand how this works. I don't think i want to force the jar into my local Maven repo (if i do, then what happens when i use the company's maven repo?), i really just want to have maven pick up the .class files from project B's build and be on my merry way. What am I missing?
EDIT: adding poms:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.firm</groupId>
<artifactId>projectA</artifactId>
<packaging>jar</packaging>
<version>0.0.2-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
</parent>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<executable>true</executable>
</configuration>
</plugin>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20090211</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.13</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
POM 2:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.firm</groupId>
<artifactId>projectB</artifactId>
<packaging>jar</packaging>
<version>0.0.1-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
</parent>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20090211</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.ldap</groupId>
<artifactId>spring-ldap-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
java spring maven
I come from a C#Visual Studio background and can't wrap my head around how to reference project B from project A such that when I do a Maven build, it actually builds. Using Spring Tool Suite:
- In Project A, Properties> Java Build Path > Projects, I've added Project B.
- Project B builds via Maven just fine as a package.
- Lovely, intellisense works and I'm happily coding along.
- I go to do a Maven build on project A and get a whole bunch of
[ERROR]: ...package [project B] does not exist.
I get that somehow i need to make Maven aware of project B's jar...but I don't understand how this works. I don't think i want to force the jar into my local Maven repo (if i do, then what happens when i use the company's maven repo?), i really just want to have maven pick up the .class files from project B's build and be on my merry way. What am I missing?
EDIT: adding poms:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.firm</groupId>
<artifactId>projectA</artifactId>
<packaging>jar</packaging>
<version>0.0.2-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
</parent>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<executable>true</executable>
</configuration>
</plugin>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20090211</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.13</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
POM 2:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.firm</groupId>
<artifactId>projectB</artifactId>
<packaging>jar</packaging>
<version>0.0.1-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
</parent>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20090211</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.ldap</groupId>
<artifactId>spring-ldap-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
java spring maven
java spring maven
edited Nov 13 '18 at 15:38
forTheLoveOfFish
asked Nov 13 '18 at 13:52
forTheLoveOfFishforTheLoveOfFish
83
83
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Once you build a project via maven it automatically being added to maven local repository and you can reference it from any maven project inside pom.xml.
Please attach pom files for both project.
Edit:
add this dependency to projectA pom.xml file:
<dependency>
<groupId>com.firm</groupId>
<artifactId>projectB</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
I added the poms - any help you can provide would be greatly appreciated!! Thanks
– forTheLoveOfFish
Nov 13 '18 at 17:17
You have to add projectB as dependency in projectA pom.xml. add this pom file: <dependency> <groupId>com.firm</groupId> <artifactId>projectB</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency>
– Muhammad Alsaied
Nov 13 '18 at 18:14
i've never had this issue before, but maven is complaining that it can't download the maven-install-plugin because it "Failed to authenticate with proxy", although all other dependencies and plugins load fine? I doubt anyone can help, but thought i'd ask! I'm almost there.
– forTheLoveOfFish
Nov 14 '18 at 13:18
I figured it out - refreshed https credentials on my local proxy server, and everything flowed in. Thanks!
– forTheLoveOfFish
Nov 14 '18 at 13:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282562%2fspring-tool-suite-referencing-projects%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Once you build a project via maven it automatically being added to maven local repository and you can reference it from any maven project inside pom.xml.
Please attach pom files for both project.
Edit:
add this dependency to projectA pom.xml file:
<dependency>
<groupId>com.firm</groupId>
<artifactId>projectB</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
I added the poms - any help you can provide would be greatly appreciated!! Thanks
– forTheLoveOfFish
Nov 13 '18 at 17:17
You have to add projectB as dependency in projectA pom.xml. add this pom file: <dependency> <groupId>com.firm</groupId> <artifactId>projectB</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency>
– Muhammad Alsaied
Nov 13 '18 at 18:14
i've never had this issue before, but maven is complaining that it can't download the maven-install-plugin because it "Failed to authenticate with proxy", although all other dependencies and plugins load fine? I doubt anyone can help, but thought i'd ask! I'm almost there.
– forTheLoveOfFish
Nov 14 '18 at 13:18
I figured it out - refreshed https credentials on my local proxy server, and everything flowed in. Thanks!
– forTheLoveOfFish
Nov 14 '18 at 13:26
add a comment |
Once you build a project via maven it automatically being added to maven local repository and you can reference it from any maven project inside pom.xml.
Please attach pom files for both project.
Edit:
add this dependency to projectA pom.xml file:
<dependency>
<groupId>com.firm</groupId>
<artifactId>projectB</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
I added the poms - any help you can provide would be greatly appreciated!! Thanks
– forTheLoveOfFish
Nov 13 '18 at 17:17
You have to add projectB as dependency in projectA pom.xml. add this pom file: <dependency> <groupId>com.firm</groupId> <artifactId>projectB</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency>
– Muhammad Alsaied
Nov 13 '18 at 18:14
i've never had this issue before, but maven is complaining that it can't download the maven-install-plugin because it "Failed to authenticate with proxy", although all other dependencies and plugins load fine? I doubt anyone can help, but thought i'd ask! I'm almost there.
– forTheLoveOfFish
Nov 14 '18 at 13:18
I figured it out - refreshed https credentials on my local proxy server, and everything flowed in. Thanks!
– forTheLoveOfFish
Nov 14 '18 at 13:26
add a comment |
Once you build a project via maven it automatically being added to maven local repository and you can reference it from any maven project inside pom.xml.
Please attach pom files for both project.
Edit:
add this dependency to projectA pom.xml file:
<dependency>
<groupId>com.firm</groupId>
<artifactId>projectB</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
Once you build a project via maven it automatically being added to maven local repository and you can reference it from any maven project inside pom.xml.
Please attach pom files for both project.
Edit:
add this dependency to projectA pom.xml file:
<dependency>
<groupId>com.firm</groupId>
<artifactId>projectB</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
edited Nov 13 '18 at 18:16
answered Nov 13 '18 at 15:05


Muhammad AlsaiedMuhammad Alsaied
2813
2813
I added the poms - any help you can provide would be greatly appreciated!! Thanks
– forTheLoveOfFish
Nov 13 '18 at 17:17
You have to add projectB as dependency in projectA pom.xml. add this pom file: <dependency> <groupId>com.firm</groupId> <artifactId>projectB</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency>
– Muhammad Alsaied
Nov 13 '18 at 18:14
i've never had this issue before, but maven is complaining that it can't download the maven-install-plugin because it "Failed to authenticate with proxy", although all other dependencies and plugins load fine? I doubt anyone can help, but thought i'd ask! I'm almost there.
– forTheLoveOfFish
Nov 14 '18 at 13:18
I figured it out - refreshed https credentials on my local proxy server, and everything flowed in. Thanks!
– forTheLoveOfFish
Nov 14 '18 at 13:26
add a comment |
I added the poms - any help you can provide would be greatly appreciated!! Thanks
– forTheLoveOfFish
Nov 13 '18 at 17:17
You have to add projectB as dependency in projectA pom.xml. add this pom file: <dependency> <groupId>com.firm</groupId> <artifactId>projectB</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency>
– Muhammad Alsaied
Nov 13 '18 at 18:14
i've never had this issue before, but maven is complaining that it can't download the maven-install-plugin because it "Failed to authenticate with proxy", although all other dependencies and plugins load fine? I doubt anyone can help, but thought i'd ask! I'm almost there.
– forTheLoveOfFish
Nov 14 '18 at 13:18
I figured it out - refreshed https credentials on my local proxy server, and everything flowed in. Thanks!
– forTheLoveOfFish
Nov 14 '18 at 13:26
I added the poms - any help you can provide would be greatly appreciated!! Thanks
– forTheLoveOfFish
Nov 13 '18 at 17:17
I added the poms - any help you can provide would be greatly appreciated!! Thanks
– forTheLoveOfFish
Nov 13 '18 at 17:17
You have to add projectB as dependency in projectA pom.xml. add this pom file: <dependency> <groupId>com.firm</groupId> <artifactId>projectB</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency>
– Muhammad Alsaied
Nov 13 '18 at 18:14
You have to add projectB as dependency in projectA pom.xml. add this pom file: <dependency> <groupId>com.firm</groupId> <artifactId>projectB</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency>
– Muhammad Alsaied
Nov 13 '18 at 18:14
i've never had this issue before, but maven is complaining that it can't download the maven-install-plugin because it "Failed to authenticate with proxy", although all other dependencies and plugins load fine? I doubt anyone can help, but thought i'd ask! I'm almost there.
– forTheLoveOfFish
Nov 14 '18 at 13:18
i've never had this issue before, but maven is complaining that it can't download the maven-install-plugin because it "Failed to authenticate with proxy", although all other dependencies and plugins load fine? I doubt anyone can help, but thought i'd ask! I'm almost there.
– forTheLoveOfFish
Nov 14 '18 at 13:18
I figured it out - refreshed https credentials on my local proxy server, and everything flowed in. Thanks!
– forTheLoveOfFish
Nov 14 '18 at 13:26
I figured it out - refreshed https credentials on my local proxy server, and everything flowed in. Thanks!
– forTheLoveOfFish
Nov 14 '18 at 13:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282562%2fspring-tool-suite-referencing-projects%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
G W2,j06 3Advtin1 3 Y,NrVHUwc i kjwzDMOKpXhv Ss0GNyEVV0FGa,ZhfeQ3cJj5kKTx,n,UAH