Sinon: Test React component method is calling function pass as a prop (Mocha + Chai + Sinon is being used for unit test)
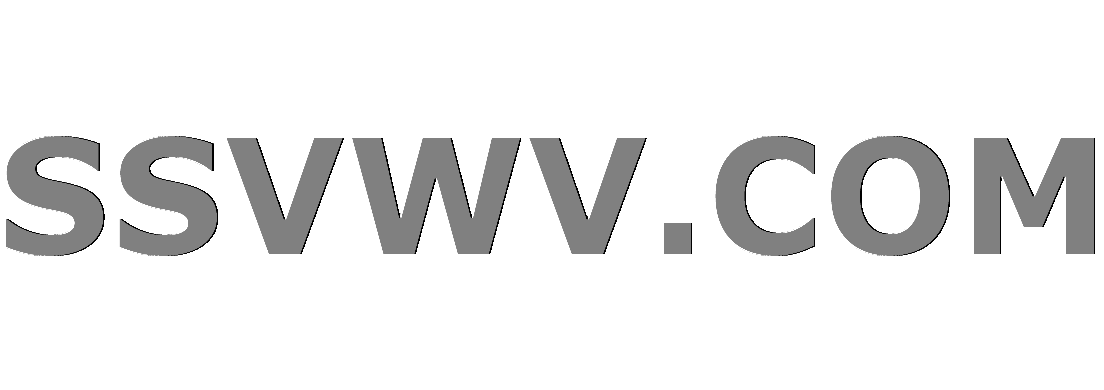
Multi tool use
I have a react component which has a method
export class Hit extends React.Component
constructor(props)
super(props);
this.triggerClick= this.triggerClick.bind(this);
triggerClick(event)
this.props.triggerClick(event.target.click);
render()
return (
....................................
<input ........ onChange=this.triggerClick />
.............................................
);
I want to check if triggerClick(event) method is being called or not. Hence, I am pasting the code I have related to this. Though if you see in the logs of the hitComponent something like below,
onChange: [Function: bound triggerClick]
log of spy says it is not being called. Not sure where I am going wrong.
let mockFunction = sinon.fake();
let hitComponent = renderShallow(<Hit ........ triggerClick= mockFunction/>)
let spy = sinon.spy(Hit.prototype, 'triggerClick');
console.log(hitComponent)
console.log(spy)
o/p:
hitComponent:
[ '$$typeof': Symbol(react.element),
type: 'input',
key: null,
ref: null,
props:
type: '.....',
checked: true,
onChange: [Function: bound triggerClick] ,
.....]
o/p:
spy:
[Function: proxy]
isSinonProxy: true,
called: false,
notCalled: true,
calledOnce: false,
calledTwice: false,
calledThrice: false,
callCount: 0,
firstCall: null,
secondCall: null,
thirdCall: null,
lastCall: null,
args: ,
returnValues: ,
thisValues: ,
exceptions: ,
callIds: ,
errorsWithCallStack: ,
displayName: 'triggerClick',
toString: [Function: toString],
instantiateFake: [Function: create],
id: 'spy#1',
stackTraceError:
Error: Stack Trace for original
at wrapMethod ..........
restore: [Function] sinon: true ,
wrappedMethod: [Function: triggerClick]
There is a helper class to use react-test-renderer
import ShallowRenderer from 'react-test-renderer/shallow';
function renderShallow(component)
const shallowRenderer = new ShallowRenderer();
shallowRenderer.render(component);
return shallowRenderer.getRenderOutput();;
export renderShallow
reactjs unit-testing sinon chai react-test-renderer
add a comment |
I have a react component which has a method
export class Hit extends React.Component
constructor(props)
super(props);
this.triggerClick= this.triggerClick.bind(this);
triggerClick(event)
this.props.triggerClick(event.target.click);
render()
return (
....................................
<input ........ onChange=this.triggerClick />
.............................................
);
I want to check if triggerClick(event) method is being called or not. Hence, I am pasting the code I have related to this. Though if you see in the logs of the hitComponent something like below,
onChange: [Function: bound triggerClick]
log of spy says it is not being called. Not sure where I am going wrong.
let mockFunction = sinon.fake();
let hitComponent = renderShallow(<Hit ........ triggerClick= mockFunction/>)
let spy = sinon.spy(Hit.prototype, 'triggerClick');
console.log(hitComponent)
console.log(spy)
o/p:
hitComponent:
[ '$$typeof': Symbol(react.element),
type: 'input',
key: null,
ref: null,
props:
type: '.....',
checked: true,
onChange: [Function: bound triggerClick] ,
.....]
o/p:
spy:
[Function: proxy]
isSinonProxy: true,
called: false,
notCalled: true,
calledOnce: false,
calledTwice: false,
calledThrice: false,
callCount: 0,
firstCall: null,
secondCall: null,
thirdCall: null,
lastCall: null,
args: ,
returnValues: ,
thisValues: ,
exceptions: ,
callIds: ,
errorsWithCallStack: ,
displayName: 'triggerClick',
toString: [Function: toString],
instantiateFake: [Function: create],
id: 'spy#1',
stackTraceError:
Error: Stack Trace for original
at wrapMethod ..........
restore: [Function] sinon: true ,
wrappedMethod: [Function: triggerClick]
There is a helper class to use react-test-renderer
import ShallowRenderer from 'react-test-renderer/shallow';
function renderShallow(component)
const shallowRenderer = new ShallowRenderer();
shallowRenderer.render(component);
return shallowRenderer.getRenderOutput();;
export renderShallow
reactjs unit-testing sinon chai react-test-renderer
add a comment |
I have a react component which has a method
export class Hit extends React.Component
constructor(props)
super(props);
this.triggerClick= this.triggerClick.bind(this);
triggerClick(event)
this.props.triggerClick(event.target.click);
render()
return (
....................................
<input ........ onChange=this.triggerClick />
.............................................
);
I want to check if triggerClick(event) method is being called or not. Hence, I am pasting the code I have related to this. Though if you see in the logs of the hitComponent something like below,
onChange: [Function: bound triggerClick]
log of spy says it is not being called. Not sure where I am going wrong.
let mockFunction = sinon.fake();
let hitComponent = renderShallow(<Hit ........ triggerClick= mockFunction/>)
let spy = sinon.spy(Hit.prototype, 'triggerClick');
console.log(hitComponent)
console.log(spy)
o/p:
hitComponent:
[ '$$typeof': Symbol(react.element),
type: 'input',
key: null,
ref: null,
props:
type: '.....',
checked: true,
onChange: [Function: bound triggerClick] ,
.....]
o/p:
spy:
[Function: proxy]
isSinonProxy: true,
called: false,
notCalled: true,
calledOnce: false,
calledTwice: false,
calledThrice: false,
callCount: 0,
firstCall: null,
secondCall: null,
thirdCall: null,
lastCall: null,
args: ,
returnValues: ,
thisValues: ,
exceptions: ,
callIds: ,
errorsWithCallStack: ,
displayName: 'triggerClick',
toString: [Function: toString],
instantiateFake: [Function: create],
id: 'spy#1',
stackTraceError:
Error: Stack Trace for original
at wrapMethod ..........
restore: [Function] sinon: true ,
wrappedMethod: [Function: triggerClick]
There is a helper class to use react-test-renderer
import ShallowRenderer from 'react-test-renderer/shallow';
function renderShallow(component)
const shallowRenderer = new ShallowRenderer();
shallowRenderer.render(component);
return shallowRenderer.getRenderOutput();;
export renderShallow
reactjs unit-testing sinon chai react-test-renderer
I have a react component which has a method
export class Hit extends React.Component
constructor(props)
super(props);
this.triggerClick= this.triggerClick.bind(this);
triggerClick(event)
this.props.triggerClick(event.target.click);
render()
return (
....................................
<input ........ onChange=this.triggerClick />
.............................................
);
I want to check if triggerClick(event) method is being called or not. Hence, I am pasting the code I have related to this. Though if you see in the logs of the hitComponent something like below,
onChange: [Function: bound triggerClick]
log of spy says it is not being called. Not sure where I am going wrong.
let mockFunction = sinon.fake();
let hitComponent = renderShallow(<Hit ........ triggerClick= mockFunction/>)
let spy = sinon.spy(Hit.prototype, 'triggerClick');
console.log(hitComponent)
console.log(spy)
o/p:
hitComponent:
[ '$$typeof': Symbol(react.element),
type: 'input',
key: null,
ref: null,
props:
type: '.....',
checked: true,
onChange: [Function: bound triggerClick] ,
.....]
o/p:
spy:
[Function: proxy]
isSinonProxy: true,
called: false,
notCalled: true,
calledOnce: false,
calledTwice: false,
calledThrice: false,
callCount: 0,
firstCall: null,
secondCall: null,
thirdCall: null,
lastCall: null,
args: ,
returnValues: ,
thisValues: ,
exceptions: ,
callIds: ,
errorsWithCallStack: ,
displayName: 'triggerClick',
toString: [Function: toString],
instantiateFake: [Function: create],
id: 'spy#1',
stackTraceError:
Error: Stack Trace for original
at wrapMethod ..........
restore: [Function] sinon: true ,
wrappedMethod: [Function: triggerClick]
There is a helper class to use react-test-renderer
import ShallowRenderer from 'react-test-renderer/shallow';
function renderShallow(component)
const shallowRenderer = new ShallowRenderer();
shallowRenderer.render(component);
return shallowRenderer.getRenderOutput();;
export renderShallow
reactjs unit-testing sinon chai react-test-renderer
reactjs unit-testing sinon chai react-test-renderer
edited Nov 15 '18 at 23:40
Vicky
asked Nov 15 '18 at 5:27
VickyVicky
138113
138113
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
triggerClick
is bound when a class is instantiated. It cannot be spied or mocked on Hit.prototype
after that.
It should be either:
let spy = sinon.spy(Hit.prototype, 'triggerClick');
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
Or:
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
sinon.spy(hitComponent.instance(), 'triggerClick');
Both seems to be not working. 1st option is having no change. 2nd option is throwing "TypeError: hitComponent.instance is not a function"
– Vicky
Nov 15 '18 at 14:34
I expect the first option to work, this is how a method should be spied. Since the question doesn't contain stackoverflow.com/help/mcve but just several snippets, I'm unable to tell why it doesn't work.onChange
event isn't triggered in the code you posted, for starters. If it isn't triggered really, that's the reason fortriggerClick
to not be called.
– estus
Nov 15 '18 at 14:41
It's also unclear whatrenderShallow
is. I assumed that this is Enzyme'sshallow
, and in this case there would be such function, airbnb.io/enzyme/docs/api/ShallowWrapper/instance.html . In case Enzyme isn't used, consider updating the question with relevant info.
– estus
Nov 15 '18 at 14:41
Sorry I missed to add the code for renderShallow which I did now. It is a helper component to use shallow stuff from the react. Also, if you see the output of hitComponent, it shows the below for onchange, onChange: [Function: bound triggerClick] } which I thought is triggering the method.
– Vicky
Nov 15 '18 at 15:44
1
There's nothing in the question that suggests that you trigger onchange event or call triggerClick in any other way. So it's not called and there's nothing to spy on. I don't use react-test-renderer myself and cannot suggest a good way to trigger an event with it, but it's possiblyhitComponent.props.onChange()
and then assert that a spy was called. Notice that even the documentation reactjs.org/docs/shallow-renderer.html suggests to use Enzyme's shallow renderer because react-test-renderer is lacking. I'd suggest to do this.
– estus
Nov 15 '18 at 16:27
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312969%2fsinon-test-react-component-method-is-calling-function-pass-as-a-prop-mocha-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
triggerClick
is bound when a class is instantiated. It cannot be spied or mocked on Hit.prototype
after that.
It should be either:
let spy = sinon.spy(Hit.prototype, 'triggerClick');
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
Or:
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
sinon.spy(hitComponent.instance(), 'triggerClick');
Both seems to be not working. 1st option is having no change. 2nd option is throwing "TypeError: hitComponent.instance is not a function"
– Vicky
Nov 15 '18 at 14:34
I expect the first option to work, this is how a method should be spied. Since the question doesn't contain stackoverflow.com/help/mcve but just several snippets, I'm unable to tell why it doesn't work.onChange
event isn't triggered in the code you posted, for starters. If it isn't triggered really, that's the reason fortriggerClick
to not be called.
– estus
Nov 15 '18 at 14:41
It's also unclear whatrenderShallow
is. I assumed that this is Enzyme'sshallow
, and in this case there would be such function, airbnb.io/enzyme/docs/api/ShallowWrapper/instance.html . In case Enzyme isn't used, consider updating the question with relevant info.
– estus
Nov 15 '18 at 14:41
Sorry I missed to add the code for renderShallow which I did now. It is a helper component to use shallow stuff from the react. Also, if you see the output of hitComponent, it shows the below for onchange, onChange: [Function: bound triggerClick] } which I thought is triggering the method.
– Vicky
Nov 15 '18 at 15:44
1
There's nothing in the question that suggests that you trigger onchange event or call triggerClick in any other way. So it's not called and there's nothing to spy on. I don't use react-test-renderer myself and cannot suggest a good way to trigger an event with it, but it's possiblyhitComponent.props.onChange()
and then assert that a spy was called. Notice that even the documentation reactjs.org/docs/shallow-renderer.html suggests to use Enzyme's shallow renderer because react-test-renderer is lacking. I'd suggest to do this.
– estus
Nov 15 '18 at 16:27
|
show 1 more comment
triggerClick
is bound when a class is instantiated. It cannot be spied or mocked on Hit.prototype
after that.
It should be either:
let spy = sinon.spy(Hit.prototype, 'triggerClick');
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
Or:
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
sinon.spy(hitComponent.instance(), 'triggerClick');
Both seems to be not working. 1st option is having no change. 2nd option is throwing "TypeError: hitComponent.instance is not a function"
– Vicky
Nov 15 '18 at 14:34
I expect the first option to work, this is how a method should be spied. Since the question doesn't contain stackoverflow.com/help/mcve but just several snippets, I'm unable to tell why it doesn't work.onChange
event isn't triggered in the code you posted, for starters. If it isn't triggered really, that's the reason fortriggerClick
to not be called.
– estus
Nov 15 '18 at 14:41
It's also unclear whatrenderShallow
is. I assumed that this is Enzyme'sshallow
, and in this case there would be such function, airbnb.io/enzyme/docs/api/ShallowWrapper/instance.html . In case Enzyme isn't used, consider updating the question with relevant info.
– estus
Nov 15 '18 at 14:41
Sorry I missed to add the code for renderShallow which I did now. It is a helper component to use shallow stuff from the react. Also, if you see the output of hitComponent, it shows the below for onchange, onChange: [Function: bound triggerClick] } which I thought is triggering the method.
– Vicky
Nov 15 '18 at 15:44
1
There's nothing in the question that suggests that you trigger onchange event or call triggerClick in any other way. So it's not called and there's nothing to spy on. I don't use react-test-renderer myself and cannot suggest a good way to trigger an event with it, but it's possiblyhitComponent.props.onChange()
and then assert that a spy was called. Notice that even the documentation reactjs.org/docs/shallow-renderer.html suggests to use Enzyme's shallow renderer because react-test-renderer is lacking. I'd suggest to do this.
– estus
Nov 15 '18 at 16:27
|
show 1 more comment
triggerClick
is bound when a class is instantiated. It cannot be spied or mocked on Hit.prototype
after that.
It should be either:
let spy = sinon.spy(Hit.prototype, 'triggerClick');
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
Or:
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
sinon.spy(hitComponent.instance(), 'triggerClick');
triggerClick
is bound when a class is instantiated. It cannot be spied or mocked on Hit.prototype
after that.
It should be either:
let spy = sinon.spy(Hit.prototype, 'triggerClick');
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
Or:
let hitComponent = renderShallow(<Hit triggerClick= mockFunction/>)
sinon.spy(hitComponent.instance(), 'triggerClick');
answered Nov 15 '18 at 7:29
estusestus
76.2k22112232
76.2k22112232
Both seems to be not working. 1st option is having no change. 2nd option is throwing "TypeError: hitComponent.instance is not a function"
– Vicky
Nov 15 '18 at 14:34
I expect the first option to work, this is how a method should be spied. Since the question doesn't contain stackoverflow.com/help/mcve but just several snippets, I'm unable to tell why it doesn't work.onChange
event isn't triggered in the code you posted, for starters. If it isn't triggered really, that's the reason fortriggerClick
to not be called.
– estus
Nov 15 '18 at 14:41
It's also unclear whatrenderShallow
is. I assumed that this is Enzyme'sshallow
, and in this case there would be such function, airbnb.io/enzyme/docs/api/ShallowWrapper/instance.html . In case Enzyme isn't used, consider updating the question with relevant info.
– estus
Nov 15 '18 at 14:41
Sorry I missed to add the code for renderShallow which I did now. It is a helper component to use shallow stuff from the react. Also, if you see the output of hitComponent, it shows the below for onchange, onChange: [Function: bound triggerClick] } which I thought is triggering the method.
– Vicky
Nov 15 '18 at 15:44
1
There's nothing in the question that suggests that you trigger onchange event or call triggerClick in any other way. So it's not called and there's nothing to spy on. I don't use react-test-renderer myself and cannot suggest a good way to trigger an event with it, but it's possiblyhitComponent.props.onChange()
and then assert that a spy was called. Notice that even the documentation reactjs.org/docs/shallow-renderer.html suggests to use Enzyme's shallow renderer because react-test-renderer is lacking. I'd suggest to do this.
– estus
Nov 15 '18 at 16:27
|
show 1 more comment
Both seems to be not working. 1st option is having no change. 2nd option is throwing "TypeError: hitComponent.instance is not a function"
– Vicky
Nov 15 '18 at 14:34
I expect the first option to work, this is how a method should be spied. Since the question doesn't contain stackoverflow.com/help/mcve but just several snippets, I'm unable to tell why it doesn't work.onChange
event isn't triggered in the code you posted, for starters. If it isn't triggered really, that's the reason fortriggerClick
to not be called.
– estus
Nov 15 '18 at 14:41
It's also unclear whatrenderShallow
is. I assumed that this is Enzyme'sshallow
, and in this case there would be such function, airbnb.io/enzyme/docs/api/ShallowWrapper/instance.html . In case Enzyme isn't used, consider updating the question with relevant info.
– estus
Nov 15 '18 at 14:41
Sorry I missed to add the code for renderShallow which I did now. It is a helper component to use shallow stuff from the react. Also, if you see the output of hitComponent, it shows the below for onchange, onChange: [Function: bound triggerClick] } which I thought is triggering the method.
– Vicky
Nov 15 '18 at 15:44
1
There's nothing in the question that suggests that you trigger onchange event or call triggerClick in any other way. So it's not called and there's nothing to spy on. I don't use react-test-renderer myself and cannot suggest a good way to trigger an event with it, but it's possiblyhitComponent.props.onChange()
and then assert that a spy was called. Notice that even the documentation reactjs.org/docs/shallow-renderer.html suggests to use Enzyme's shallow renderer because react-test-renderer is lacking. I'd suggest to do this.
– estus
Nov 15 '18 at 16:27
Both seems to be not working. 1st option is having no change. 2nd option is throwing "TypeError: hitComponent.instance is not a function"
– Vicky
Nov 15 '18 at 14:34
Both seems to be not working. 1st option is having no change. 2nd option is throwing "TypeError: hitComponent.instance is not a function"
– Vicky
Nov 15 '18 at 14:34
I expect the first option to work, this is how a method should be spied. Since the question doesn't contain stackoverflow.com/help/mcve but just several snippets, I'm unable to tell why it doesn't work.
onChange
event isn't triggered in the code you posted, for starters. If it isn't triggered really, that's the reason for triggerClick
to not be called.– estus
Nov 15 '18 at 14:41
I expect the first option to work, this is how a method should be spied. Since the question doesn't contain stackoverflow.com/help/mcve but just several snippets, I'm unable to tell why it doesn't work.
onChange
event isn't triggered in the code you posted, for starters. If it isn't triggered really, that's the reason for triggerClick
to not be called.– estus
Nov 15 '18 at 14:41
It's also unclear what
renderShallow
is. I assumed that this is Enzyme's shallow
, and in this case there would be such function, airbnb.io/enzyme/docs/api/ShallowWrapper/instance.html . In case Enzyme isn't used, consider updating the question with relevant info.– estus
Nov 15 '18 at 14:41
It's also unclear what
renderShallow
is. I assumed that this is Enzyme's shallow
, and in this case there would be such function, airbnb.io/enzyme/docs/api/ShallowWrapper/instance.html . In case Enzyme isn't used, consider updating the question with relevant info.– estus
Nov 15 '18 at 14:41
Sorry I missed to add the code for renderShallow which I did now. It is a helper component to use shallow stuff from the react. Also, if you see the output of hitComponent, it shows the below for onchange, onChange: [Function: bound triggerClick] } which I thought is triggering the method.
– Vicky
Nov 15 '18 at 15:44
Sorry I missed to add the code for renderShallow which I did now. It is a helper component to use shallow stuff from the react. Also, if you see the output of hitComponent, it shows the below for onchange, onChange: [Function: bound triggerClick] } which I thought is triggering the method.
– Vicky
Nov 15 '18 at 15:44
1
1
There's nothing in the question that suggests that you trigger onchange event or call triggerClick in any other way. So it's not called and there's nothing to spy on. I don't use react-test-renderer myself and cannot suggest a good way to trigger an event with it, but it's possibly
hitComponent.props.onChange()
and then assert that a spy was called. Notice that even the documentation reactjs.org/docs/shallow-renderer.html suggests to use Enzyme's shallow renderer because react-test-renderer is lacking. I'd suggest to do this.– estus
Nov 15 '18 at 16:27
There's nothing in the question that suggests that you trigger onchange event or call triggerClick in any other way. So it's not called and there's nothing to spy on. I don't use react-test-renderer myself and cannot suggest a good way to trigger an event with it, but it's possibly
hitComponent.props.onChange()
and then assert that a spy was called. Notice that even the documentation reactjs.org/docs/shallow-renderer.html suggests to use Enzyme's shallow renderer because react-test-renderer is lacking. I'd suggest to do this.– estus
Nov 15 '18 at 16:27
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312969%2fsinon-test-react-component-method-is-calling-function-pass-as-a-prop-mocha-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Vr4IyfFCtm5b