Bezier curve surface
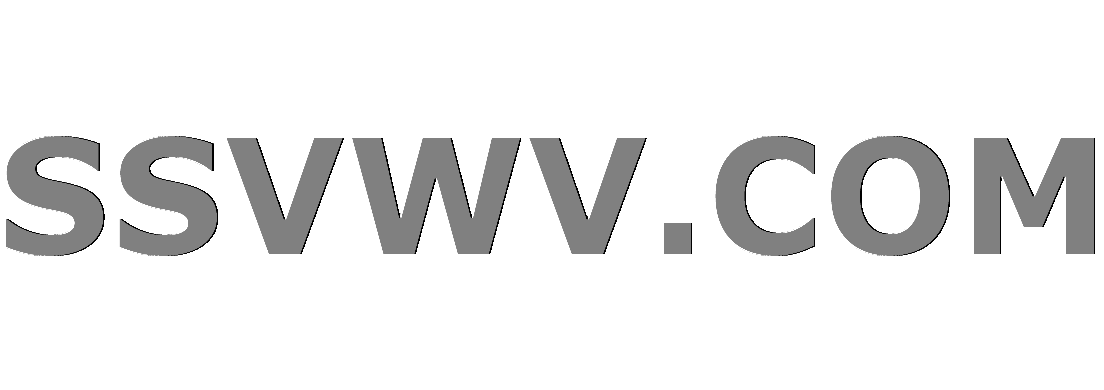
Multi tool use
I have Bezier curve and I need to make surface of revolution based on this curve. Bezier curve should be rotating around an axis of rotation. How it can be done? Maybe some examples? Dots can be fixed, but only 3
import matplotlib as mpl
import numpy as np
from scipy.misc import comb
from matplotlib import pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import math
import pylab
def bernstein_poly(i, n, t):
return comb(n, i) * (t**(n - i)) * (1 - t)**i
def bezier_curve(points, nTimes=1000):
nPoints = len(points)
xPoints = np.array([p[0] for p in points])
yPoints = np.array([p[1] for p in points])
zPoints = np.array([p[2] for p in points])
t = np.linspace(0.0, 1.0, nTimes)
polynomial_array = np.array(
[bernstein_poly(i, nPoints - 1, t) for i in range(0, nPoints)])
xvals = np.dot(xPoints, polynomial_array)
yvals = np.dot(yPoints, polynomial_array)
zvals = np.dot(zPoints, polynomial_array)
return xvals, yvals, zvals
from math import pi ,sin, cos
def R(theta, u):
return [[cos(theta) + u[0]**2 * (1-cos(theta)),
u[0] * u[1] * (1-cos(theta)) - u[2] * sin(theta),
u[0] * u[2] * (1 - cos(theta)) + u[1] * sin(theta)],
[u[0] * u[1] * (1-cos(theta)) + u[2] * sin(theta),
cos(theta) + u[1]**2 * (1-cos(theta)),
u[1] * u[2] * (1 - cos(theta)) - u[0] * sin(theta)],
[u[0] * u[2] * (1-cos(theta)) - u[1] * sin(theta),
u[1] * u[2] * (1-cos(theta)) + u[0] * sin(theta),
cos(theta) + u[2]**2 * (1-cos(theta))]]
def Rotate(pointToRotate, point1, point2, theta):
u=
squaredSum = 0
for i,f in zip(point1, point2):
u.append(f-i)
squaredSum += (f-i) **2
u = [i/squaredSum for i in u]
r = R(theta, u)
rotated =
for i in range(3):
rotated.append(round(sum([r[j][i] * pointToRotate[j] for j in range(3)])))
return rotated
And main part
if __name__ == "__main__":
nPoints = 3
points = [[0,0,0],[0,1,2],[0,2,1]]#3 points of curve
xvals, yvals, zvals = bezier_curve(points, nTimes=1000)
fig = plt.figure()
ax = fig.gca(projection='3d')
ax.plot(xvals, yvals, zvals, label='bezier')
p1=[0,0,0]#axis of rotation
p2=[0,0,1]#axis of rotation
angle = pi/12
while angle <= 2*pi:
pp1 = Rotate(points[0], p1, p2, angle)
pp2 = Rotate(points[1], p1, p2, angle)
pp3 = Rotate(points[2], p1, p2, angle)
npoints=[pp1,pp2,pp3]
xnvals, ynvals, znvals = bezier_curve(npoints, nTimes=1000)
ax.plot(xnvals, ynvals, znvals, label='bezier')
angle= angle + pi/24
plt.show()
upd: I do something on this task. But this is not exactly what I need.Now its many lines, not surface. I tried to make a surface, but failed
Upd2:
This is my attempt to make surface of revolution, but its failed too
x = np.arange (0, 0.1, 0.005)
y = np.arange (0, 0.1, 0.005)
xgrid, ygrid = np.meshgrid(x, y)
points = [[0,0,0],[0,1,2],[0,2,1]] #Bezier curve points
p1=[0,0,0]#axis of rotation
p2=[0,0,1]#axis of rotation
angle = pi/12
xval, yval, zval = bezier_curve(points, nTimes=20)
x=xgrid
y=ygrid
z=zval
fig = pylab.figure()
axes = Axes3D(fig)
axes.plot_surface(x, y, z)
while angle <= 2*pi:
pp1 = Rotate(points[0], p1, p2, angle)
pp2 = Rotate(points[1], p1, p2, angle)
pp3 = Rotate(points[2], p1, p2, angle)
npoints=[pp1,pp2,pp3]
x = np.arange (0, 0.1, 0.005)
y = np.arange (0, 0.1, 0.005)
xgrid, ygrid = np.meshgrid(x, y)
xval, yval, zval = bezier_curve(npoints, nTimes=20)
zgrid= zval
x=xgrid
y=ygrid
z=zgrid
axes.plot_surface(x, y, z)
angle= angle + pi/24
python 3d bezier
|
show 8 more comments
I have Bezier curve and I need to make surface of revolution based on this curve. Bezier curve should be rotating around an axis of rotation. How it can be done? Maybe some examples? Dots can be fixed, but only 3
import matplotlib as mpl
import numpy as np
from scipy.misc import comb
from matplotlib import pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import math
import pylab
def bernstein_poly(i, n, t):
return comb(n, i) * (t**(n - i)) * (1 - t)**i
def bezier_curve(points, nTimes=1000):
nPoints = len(points)
xPoints = np.array([p[0] for p in points])
yPoints = np.array([p[1] for p in points])
zPoints = np.array([p[2] for p in points])
t = np.linspace(0.0, 1.0, nTimes)
polynomial_array = np.array(
[bernstein_poly(i, nPoints - 1, t) for i in range(0, nPoints)])
xvals = np.dot(xPoints, polynomial_array)
yvals = np.dot(yPoints, polynomial_array)
zvals = np.dot(zPoints, polynomial_array)
return xvals, yvals, zvals
from math import pi ,sin, cos
def R(theta, u):
return [[cos(theta) + u[0]**2 * (1-cos(theta)),
u[0] * u[1] * (1-cos(theta)) - u[2] * sin(theta),
u[0] * u[2] * (1 - cos(theta)) + u[1] * sin(theta)],
[u[0] * u[1] * (1-cos(theta)) + u[2] * sin(theta),
cos(theta) + u[1]**2 * (1-cos(theta)),
u[1] * u[2] * (1 - cos(theta)) - u[0] * sin(theta)],
[u[0] * u[2] * (1-cos(theta)) - u[1] * sin(theta),
u[1] * u[2] * (1-cos(theta)) + u[0] * sin(theta),
cos(theta) + u[2]**2 * (1-cos(theta))]]
def Rotate(pointToRotate, point1, point2, theta):
u=
squaredSum = 0
for i,f in zip(point1, point2):
u.append(f-i)
squaredSum += (f-i) **2
u = [i/squaredSum for i in u]
r = R(theta, u)
rotated =
for i in range(3):
rotated.append(round(sum([r[j][i] * pointToRotate[j] for j in range(3)])))
return rotated
And main part
if __name__ == "__main__":
nPoints = 3
points = [[0,0,0],[0,1,2],[0,2,1]]#3 points of curve
xvals, yvals, zvals = bezier_curve(points, nTimes=1000)
fig = plt.figure()
ax = fig.gca(projection='3d')
ax.plot(xvals, yvals, zvals, label='bezier')
p1=[0,0,0]#axis of rotation
p2=[0,0,1]#axis of rotation
angle = pi/12
while angle <= 2*pi:
pp1 = Rotate(points[0], p1, p2, angle)
pp2 = Rotate(points[1], p1, p2, angle)
pp3 = Rotate(points[2], p1, p2, angle)
npoints=[pp1,pp2,pp3]
xnvals, ynvals, znvals = bezier_curve(npoints, nTimes=1000)
ax.plot(xnvals, ynvals, znvals, label='bezier')
angle= angle + pi/24
plt.show()
upd: I do something on this task. But this is not exactly what I need.Now its many lines, not surface. I tried to make a surface, but failed
Upd2:
This is my attempt to make surface of revolution, but its failed too
x = np.arange (0, 0.1, 0.005)
y = np.arange (0, 0.1, 0.005)
xgrid, ygrid = np.meshgrid(x, y)
points = [[0,0,0],[0,1,2],[0,2,1]] #Bezier curve points
p1=[0,0,0]#axis of rotation
p2=[0,0,1]#axis of rotation
angle = pi/12
xval, yval, zval = bezier_curve(points, nTimes=20)
x=xgrid
y=ygrid
z=zval
fig = pylab.figure()
axes = Axes3D(fig)
axes.plot_surface(x, y, z)
while angle <= 2*pi:
pp1 = Rotate(points[0], p1, p2, angle)
pp2 = Rotate(points[1], p1, p2, angle)
pp3 = Rotate(points[2], p1, p2, angle)
npoints=[pp1,pp2,pp3]
x = np.arange (0, 0.1, 0.005)
y = np.arange (0, 0.1, 0.005)
xgrid, ygrid = np.meshgrid(x, y)
xval, yval, zval = bezier_curve(npoints, nTimes=20)
zgrid= zval
x=xgrid
y=ygrid
z=zgrid
axes.plot_surface(x, y, z)
angle= angle + pi/24
python 3d bezier
Copy-rotate your coordinates about whatever axis vector you want, and then construct your surface as a quad surface using the vertices that generates?
– Mike 'Pomax' Kamermans
Nov 20 '18 at 18:19
@mike-pomax-kamermans, yeah)) I know it too, but i cant do it
– Ильшат Мурзурбеков
Nov 25 '18 at 12:13
1
What do you mean you know it but you can't do it? Either you know it, and then you know how to do it (or you know how to look up how to do it, there's even wikipedia articles about 3D rotations), or you don't know it, in which case step one is look up how to do it and then as last resort when you absolutely can't find anything, ask on SO. If you have no idea how to get started, then read through en.wikipedia.org/wiki/… and the article around it, but finding a python package to do this for you is probably less work.
– Mike 'Pomax' Kamermans
Nov 25 '18 at 16:25
@mike-pomax-kamermans I updated my post. I have some problems with surface based on this curve
– Ильшат Мурзурбеков
Nov 25 '18 at 17:41
If you have all the coordinates, surely SO always has the answer for you over on stackoverflow.com/a/38946791/740553, which you can easily find by searching for something like "python define 3d bezier surface"?
– Mike 'Pomax' Kamermans
Nov 25 '18 at 17:58
|
show 8 more comments
I have Bezier curve and I need to make surface of revolution based on this curve. Bezier curve should be rotating around an axis of rotation. How it can be done? Maybe some examples? Dots can be fixed, but only 3
import matplotlib as mpl
import numpy as np
from scipy.misc import comb
from matplotlib import pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import math
import pylab
def bernstein_poly(i, n, t):
return comb(n, i) * (t**(n - i)) * (1 - t)**i
def bezier_curve(points, nTimes=1000):
nPoints = len(points)
xPoints = np.array([p[0] for p in points])
yPoints = np.array([p[1] for p in points])
zPoints = np.array([p[2] for p in points])
t = np.linspace(0.0, 1.0, nTimes)
polynomial_array = np.array(
[bernstein_poly(i, nPoints - 1, t) for i in range(0, nPoints)])
xvals = np.dot(xPoints, polynomial_array)
yvals = np.dot(yPoints, polynomial_array)
zvals = np.dot(zPoints, polynomial_array)
return xvals, yvals, zvals
from math import pi ,sin, cos
def R(theta, u):
return [[cos(theta) + u[0]**2 * (1-cos(theta)),
u[0] * u[1] * (1-cos(theta)) - u[2] * sin(theta),
u[0] * u[2] * (1 - cos(theta)) + u[1] * sin(theta)],
[u[0] * u[1] * (1-cos(theta)) + u[2] * sin(theta),
cos(theta) + u[1]**2 * (1-cos(theta)),
u[1] * u[2] * (1 - cos(theta)) - u[0] * sin(theta)],
[u[0] * u[2] * (1-cos(theta)) - u[1] * sin(theta),
u[1] * u[2] * (1-cos(theta)) + u[0] * sin(theta),
cos(theta) + u[2]**2 * (1-cos(theta))]]
def Rotate(pointToRotate, point1, point2, theta):
u=
squaredSum = 0
for i,f in zip(point1, point2):
u.append(f-i)
squaredSum += (f-i) **2
u = [i/squaredSum for i in u]
r = R(theta, u)
rotated =
for i in range(3):
rotated.append(round(sum([r[j][i] * pointToRotate[j] for j in range(3)])))
return rotated
And main part
if __name__ == "__main__":
nPoints = 3
points = [[0,0,0],[0,1,2],[0,2,1]]#3 points of curve
xvals, yvals, zvals = bezier_curve(points, nTimes=1000)
fig = plt.figure()
ax = fig.gca(projection='3d')
ax.plot(xvals, yvals, zvals, label='bezier')
p1=[0,0,0]#axis of rotation
p2=[0,0,1]#axis of rotation
angle = pi/12
while angle <= 2*pi:
pp1 = Rotate(points[0], p1, p2, angle)
pp2 = Rotate(points[1], p1, p2, angle)
pp3 = Rotate(points[2], p1, p2, angle)
npoints=[pp1,pp2,pp3]
xnvals, ynvals, znvals = bezier_curve(npoints, nTimes=1000)
ax.plot(xnvals, ynvals, znvals, label='bezier')
angle= angle + pi/24
plt.show()
upd: I do something on this task. But this is not exactly what I need.Now its many lines, not surface. I tried to make a surface, but failed
Upd2:
This is my attempt to make surface of revolution, but its failed too
x = np.arange (0, 0.1, 0.005)
y = np.arange (0, 0.1, 0.005)
xgrid, ygrid = np.meshgrid(x, y)
points = [[0,0,0],[0,1,2],[0,2,1]] #Bezier curve points
p1=[0,0,0]#axis of rotation
p2=[0,0,1]#axis of rotation
angle = pi/12
xval, yval, zval = bezier_curve(points, nTimes=20)
x=xgrid
y=ygrid
z=zval
fig = pylab.figure()
axes = Axes3D(fig)
axes.plot_surface(x, y, z)
while angle <= 2*pi:
pp1 = Rotate(points[0], p1, p2, angle)
pp2 = Rotate(points[1], p1, p2, angle)
pp3 = Rotate(points[2], p1, p2, angle)
npoints=[pp1,pp2,pp3]
x = np.arange (0, 0.1, 0.005)
y = np.arange (0, 0.1, 0.005)
xgrid, ygrid = np.meshgrid(x, y)
xval, yval, zval = bezier_curve(npoints, nTimes=20)
zgrid= zval
x=xgrid
y=ygrid
z=zgrid
axes.plot_surface(x, y, z)
angle= angle + pi/24
python 3d bezier
I have Bezier curve and I need to make surface of revolution based on this curve. Bezier curve should be rotating around an axis of rotation. How it can be done? Maybe some examples? Dots can be fixed, but only 3
import matplotlib as mpl
import numpy as np
from scipy.misc import comb
from matplotlib import pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import math
import pylab
def bernstein_poly(i, n, t):
return comb(n, i) * (t**(n - i)) * (1 - t)**i
def bezier_curve(points, nTimes=1000):
nPoints = len(points)
xPoints = np.array([p[0] for p in points])
yPoints = np.array([p[1] for p in points])
zPoints = np.array([p[2] for p in points])
t = np.linspace(0.0, 1.0, nTimes)
polynomial_array = np.array(
[bernstein_poly(i, nPoints - 1, t) for i in range(0, nPoints)])
xvals = np.dot(xPoints, polynomial_array)
yvals = np.dot(yPoints, polynomial_array)
zvals = np.dot(zPoints, polynomial_array)
return xvals, yvals, zvals
from math import pi ,sin, cos
def R(theta, u):
return [[cos(theta) + u[0]**2 * (1-cos(theta)),
u[0] * u[1] * (1-cos(theta)) - u[2] * sin(theta),
u[0] * u[2] * (1 - cos(theta)) + u[1] * sin(theta)],
[u[0] * u[1] * (1-cos(theta)) + u[2] * sin(theta),
cos(theta) + u[1]**2 * (1-cos(theta)),
u[1] * u[2] * (1 - cos(theta)) - u[0] * sin(theta)],
[u[0] * u[2] * (1-cos(theta)) - u[1] * sin(theta),
u[1] * u[2] * (1-cos(theta)) + u[0] * sin(theta),
cos(theta) + u[2]**2 * (1-cos(theta))]]
def Rotate(pointToRotate, point1, point2, theta):
u=
squaredSum = 0
for i,f in zip(point1, point2):
u.append(f-i)
squaredSum += (f-i) **2
u = [i/squaredSum for i in u]
r = R(theta, u)
rotated =
for i in range(3):
rotated.append(round(sum([r[j][i] * pointToRotate[j] for j in range(3)])))
return rotated
And main part
if __name__ == "__main__":
nPoints = 3
points = [[0,0,0],[0,1,2],[0,2,1]]#3 points of curve
xvals, yvals, zvals = bezier_curve(points, nTimes=1000)
fig = plt.figure()
ax = fig.gca(projection='3d')
ax.plot(xvals, yvals, zvals, label='bezier')
p1=[0,0,0]#axis of rotation
p2=[0,0,1]#axis of rotation
angle = pi/12
while angle <= 2*pi:
pp1 = Rotate(points[0], p1, p2, angle)
pp2 = Rotate(points[1], p1, p2, angle)
pp3 = Rotate(points[2], p1, p2, angle)
npoints=[pp1,pp2,pp3]
xnvals, ynvals, znvals = bezier_curve(npoints, nTimes=1000)
ax.plot(xnvals, ynvals, znvals, label='bezier')
angle= angle + pi/24
plt.show()
upd: I do something on this task. But this is not exactly what I need.Now its many lines, not surface. I tried to make a surface, but failed
Upd2:
This is my attempt to make surface of revolution, but its failed too
x = np.arange (0, 0.1, 0.005)
y = np.arange (0, 0.1, 0.005)
xgrid, ygrid = np.meshgrid(x, y)
points = [[0,0,0],[0,1,2],[0,2,1]] #Bezier curve points
p1=[0,0,0]#axis of rotation
p2=[0,0,1]#axis of rotation
angle = pi/12
xval, yval, zval = bezier_curve(points, nTimes=20)
x=xgrid
y=ygrid
z=zval
fig = pylab.figure()
axes = Axes3D(fig)
axes.plot_surface(x, y, z)
while angle <= 2*pi:
pp1 = Rotate(points[0], p1, p2, angle)
pp2 = Rotate(points[1], p1, p2, angle)
pp3 = Rotate(points[2], p1, p2, angle)
npoints=[pp1,pp2,pp3]
x = np.arange (0, 0.1, 0.005)
y = np.arange (0, 0.1, 0.005)
xgrid, ygrid = np.meshgrid(x, y)
xval, yval, zval = bezier_curve(npoints, nTimes=20)
zgrid= zval
x=xgrid
y=ygrid
z=zgrid
axes.plot_surface(x, y, z)
angle= angle + pi/24
python 3d bezier
python 3d bezier
edited Nov 28 '18 at 15:38
Ильшат Мурзурбеков
asked Nov 16 '18 at 9:49
Ильшат МурзурбековИльшат Мурзурбеков
256
256
Copy-rotate your coordinates about whatever axis vector you want, and then construct your surface as a quad surface using the vertices that generates?
– Mike 'Pomax' Kamermans
Nov 20 '18 at 18:19
@mike-pomax-kamermans, yeah)) I know it too, but i cant do it
– Ильшат Мурзурбеков
Nov 25 '18 at 12:13
1
What do you mean you know it but you can't do it? Either you know it, and then you know how to do it (or you know how to look up how to do it, there's even wikipedia articles about 3D rotations), or you don't know it, in which case step one is look up how to do it and then as last resort when you absolutely can't find anything, ask on SO. If you have no idea how to get started, then read through en.wikipedia.org/wiki/… and the article around it, but finding a python package to do this for you is probably less work.
– Mike 'Pomax' Kamermans
Nov 25 '18 at 16:25
@mike-pomax-kamermans I updated my post. I have some problems with surface based on this curve
– Ильшат Мурзурбеков
Nov 25 '18 at 17:41
If you have all the coordinates, surely SO always has the answer for you over on stackoverflow.com/a/38946791/740553, which you can easily find by searching for something like "python define 3d bezier surface"?
– Mike 'Pomax' Kamermans
Nov 25 '18 at 17:58
|
show 8 more comments
Copy-rotate your coordinates about whatever axis vector you want, and then construct your surface as a quad surface using the vertices that generates?
– Mike 'Pomax' Kamermans
Nov 20 '18 at 18:19
@mike-pomax-kamermans, yeah)) I know it too, but i cant do it
– Ильшат Мурзурбеков
Nov 25 '18 at 12:13
1
What do you mean you know it but you can't do it? Either you know it, and then you know how to do it (or you know how to look up how to do it, there's even wikipedia articles about 3D rotations), or you don't know it, in which case step one is look up how to do it and then as last resort when you absolutely can't find anything, ask on SO. If you have no idea how to get started, then read through en.wikipedia.org/wiki/… and the article around it, but finding a python package to do this for you is probably less work.
– Mike 'Pomax' Kamermans
Nov 25 '18 at 16:25
@mike-pomax-kamermans I updated my post. I have some problems with surface based on this curve
– Ильшат Мурзурбеков
Nov 25 '18 at 17:41
If you have all the coordinates, surely SO always has the answer for you over on stackoverflow.com/a/38946791/740553, which you can easily find by searching for something like "python define 3d bezier surface"?
– Mike 'Pomax' Kamermans
Nov 25 '18 at 17:58
Copy-rotate your coordinates about whatever axis vector you want, and then construct your surface as a quad surface using the vertices that generates?
– Mike 'Pomax' Kamermans
Nov 20 '18 at 18:19
Copy-rotate your coordinates about whatever axis vector you want, and then construct your surface as a quad surface using the vertices that generates?
– Mike 'Pomax' Kamermans
Nov 20 '18 at 18:19
@mike-pomax-kamermans, yeah)) I know it too, but i cant do it
– Ильшат Мурзурбеков
Nov 25 '18 at 12:13
@mike-pomax-kamermans, yeah)) I know it too, but i cant do it
– Ильшат Мурзурбеков
Nov 25 '18 at 12:13
1
1
What do you mean you know it but you can't do it? Either you know it, and then you know how to do it (or you know how to look up how to do it, there's even wikipedia articles about 3D rotations), or you don't know it, in which case step one is look up how to do it and then as last resort when you absolutely can't find anything, ask on SO. If you have no idea how to get started, then read through en.wikipedia.org/wiki/… and the article around it, but finding a python package to do this for you is probably less work.
– Mike 'Pomax' Kamermans
Nov 25 '18 at 16:25
What do you mean you know it but you can't do it? Either you know it, and then you know how to do it (or you know how to look up how to do it, there's even wikipedia articles about 3D rotations), or you don't know it, in which case step one is look up how to do it and then as last resort when you absolutely can't find anything, ask on SO. If you have no idea how to get started, then read through en.wikipedia.org/wiki/… and the article around it, but finding a python package to do this for you is probably less work.
– Mike 'Pomax' Kamermans
Nov 25 '18 at 16:25
@mike-pomax-kamermans I updated my post. I have some problems with surface based on this curve
– Ильшат Мурзурбеков
Nov 25 '18 at 17:41
@mike-pomax-kamermans I updated my post. I have some problems with surface based on this curve
– Ильшат Мурзурбеков
Nov 25 '18 at 17:41
If you have all the coordinates, surely SO always has the answer for you over on stackoverflow.com/a/38946791/740553, which you can easily find by searching for something like "python define 3d bezier surface"?
– Mike 'Pomax' Kamermans
Nov 25 '18 at 17:58
If you have all the coordinates, surely SO always has the answer for you over on stackoverflow.com/a/38946791/740553, which you can easily find by searching for something like "python define 3d bezier surface"?
– Mike 'Pomax' Kamermans
Nov 25 '18 at 17:58
|
show 8 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53335240%2fbezier-curve-surface%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53335240%2fbezier-curve-surface%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MhbwFZ2i8WHH0MnUp372H0rCNz9wBtWj7GRrbTbVTVk7 Df8QLhay,vFz,sRiXk xhp0dL0e KjAvtFt,rspXywOvK64uCGUkl TKy
Copy-rotate your coordinates about whatever axis vector you want, and then construct your surface as a quad surface using the vertices that generates?
– Mike 'Pomax' Kamermans
Nov 20 '18 at 18:19
@mike-pomax-kamermans, yeah)) I know it too, but i cant do it
– Ильшат Мурзурбеков
Nov 25 '18 at 12:13
1
What do you mean you know it but you can't do it? Either you know it, and then you know how to do it (or you know how to look up how to do it, there's even wikipedia articles about 3D rotations), or you don't know it, in which case step one is look up how to do it and then as last resort when you absolutely can't find anything, ask on SO. If you have no idea how to get started, then read through en.wikipedia.org/wiki/… and the article around it, but finding a python package to do this for you is probably less work.
– Mike 'Pomax' Kamermans
Nov 25 '18 at 16:25
@mike-pomax-kamermans I updated my post. I have some problems with surface based on this curve
– Ильшат Мурзурбеков
Nov 25 '18 at 17:41
If you have all the coordinates, surely SO always has the answer for you over on stackoverflow.com/a/38946791/740553, which you can easily find by searching for something like "python define 3d bezier surface"?
– Mike 'Pomax' Kamermans
Nov 25 '18 at 17:58