Getting a map() to return a list in Python 3.x
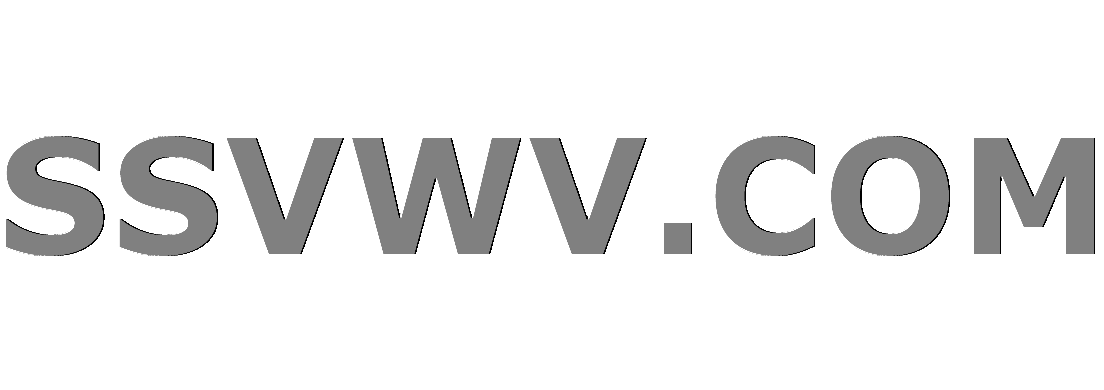
Multi tool use
up vote
386
down vote
favorite
I'm trying to map a list into hex, and then use the list elsewhere. In python 2.6, this was easy:
A: Python 2.6:
>>> map(chr, [66, 53, 0, 94])
['B', '5', 'x00', '^']
However, in Python 3.1, the above returns a map object.
B: Python 3.1:
>>> map(chr, [66, 53, 0, 94])
<map object at 0x00AF5570>
How do I retrieve the mapped list (as in A above) on Python 3.x?
Alternatively, is there a better way of doing this? My initial list object has around 45 items and id like to convert them to hex.
python list python-3.x map-function
add a comment |
up vote
386
down vote
favorite
I'm trying to map a list into hex, and then use the list elsewhere. In python 2.6, this was easy:
A: Python 2.6:
>>> map(chr, [66, 53, 0, 94])
['B', '5', 'x00', '^']
However, in Python 3.1, the above returns a map object.
B: Python 3.1:
>>> map(chr, [66, 53, 0, 94])
<map object at 0x00AF5570>
How do I retrieve the mapped list (as in A above) on Python 3.x?
Alternatively, is there a better way of doing this? My initial list object has around 45 items and id like to convert them to hex.
python list python-3.x map-function
add a comment |
up vote
386
down vote
favorite
up vote
386
down vote
favorite
I'm trying to map a list into hex, and then use the list elsewhere. In python 2.6, this was easy:
A: Python 2.6:
>>> map(chr, [66, 53, 0, 94])
['B', '5', 'x00', '^']
However, in Python 3.1, the above returns a map object.
B: Python 3.1:
>>> map(chr, [66, 53, 0, 94])
<map object at 0x00AF5570>
How do I retrieve the mapped list (as in A above) on Python 3.x?
Alternatively, is there a better way of doing this? My initial list object has around 45 items and id like to convert them to hex.
python list python-3.x map-function
I'm trying to map a list into hex, and then use the list elsewhere. In python 2.6, this was easy:
A: Python 2.6:
>>> map(chr, [66, 53, 0, 94])
['B', '5', 'x00', '^']
However, in Python 3.1, the above returns a map object.
B: Python 3.1:
>>> map(chr, [66, 53, 0, 94])
<map object at 0x00AF5570>
How do I retrieve the mapped list (as in A above) on Python 3.x?
Alternatively, is there a better way of doing this? My initial list object has around 45 items and id like to convert them to hex.
python list python-3.x map-function
python list python-3.x map-function
edited Oct 13 at 20:37
Simeon Leyzerzon
13k42746
13k42746
asked Aug 20 '09 at 0:27
mozami
2,84131619
2,84131619
add a comment |
add a comment |
9 Answers
9
active
oldest
votes
up vote
588
down vote
accepted
Do this:
list(map(chr,[66,53,0,94]))
In Python 3+, many processes that iterate over iterables return iterators themselves. In most cases, this ends up saving memory, and should make things go faster.
If all you're going to do is iterate over this list eventually, there's no need to even convert it to a list, because you can still iterate over the map
object like so:
# Prints "ABCD"
for ch in map(chr,[65,66,67,68]):
print(ch)
1
Thank you for the great explanation!!
– mozami
Aug 20 '09 at 0:35
13
Of course, you can iterate over this, too: (chr(x) for x in [65,66,67,68]). It doesn't even need map.
– hughdbrown
Aug 20 '09 at 0:40
@hughdbrown The argument for using 3.1'smap
would be lazy evaluation when iterating on a complex function, large data sets, or streams.
– Andrew Keeton
Aug 20 '09 at 0:45
16
@Andrew actually Hugh is uing a generator comprehension which would do the same thing. Note the parentheses rather than square brackets.
– Triptych
Aug 20 '09 at 0:49
5
Alternate solution (faster for large inputs too) when the values are known to be ASCII/latin-1 is to do bulk conversions at the C layer:bytes(sequence_of_ints_in_range_0_to_256).decode('latin-1')
which makes astr
faster by avoiding Python function calls for each element in favor of a bulk conversion of all elements using only C level function calls. You can wrap the above inlist
if you really need alist
of the individual characters, but sincestr
is already an iterable of its own characters, the only reason you'd do so is if you need mutability.
– ShadowRanger
Jul 1 '16 at 1:52
|
show 4 more comments
up vote
82
down vote
Why aren't you doing this:
[chr(x) for x in [66,53,0,94]]
It's called a list comprehension. You can find plenty of information on Google, but here's the link to the Python (2.6) documentation on list comprehensions. You might be more interested in the Python 3 documenation, though.
8
Yes to list comprehensions.
– hughdbrown
Aug 20 '09 at 0:29
4
Hmmmm. Maybe there needs to be a general posting on list comprehensions, generators, map(), zip(), and a lot of other speedy iteration goodness in python.
– hughdbrown
Aug 20 '09 at 0:55
33
I guess because it's more verbose, you have to write an extra variable (twice)... If the operation is more complex and you end up writing a lambda, or you need also to drop some elements, I think a comprehension is definitively better than a map+filter, but if you already have the function you want to apply, map is more succinct.
– fortran
Jun 25 '10 at 9:43
1
+1: Easier to read & allows you to use functions with many parameters
– Le Droid
Mar 6 '14 at 21:55
map(chr, [66,53,0,94])
is definitely more concise than[chr(x) for x in [66,53,0,94]]
.
– Giorgio
Oct 26 at 20:01
add a comment |
up vote
63
down vote
New and neat in Python 3.5:
[*map(chr, [66, 53, 0, 94])]
Thanks to Additional Unpacking Generalizations
UPDATE
Always seeking for shorter ways, I discovered this one also works:
*map(chr, [66, 53, 0, 94]),
Unpacking works in tuples too. Note the comma at the end. This makes it a tuple of 1 element. That is, it's equivalent to (*map(chr, [66, 53, 0, 94]),)
It's shorter by only one char from the version with the list-brackets, but, in my opinion, better to write, because you start right ahead with the asterisk - the expansion syntax, so I feel it's softer on the mind. :)
10
What's wrong withlist()
?
– Quelklef
Aug 12 '17 at 12:56
8
@Quelkleflist()
doesn't look as neat
– Arijoon
Sep 28 '17 at 13:15
3
@Quelklef: Also, the unpacking approach is trivially faster thanks to not needing to look up thelist
constructor and invoke general function call machinery. For a long input, it won't matter; for a short one, it can make a big difference. Using the above code with the input as atuple
so it's not repeatedly reconstructed,ipython
microbenchmarks show thelist()
wrapping approach takes about 20% longer than unpacking. Mind you, in absolute terms, we're talking about 150 ns, which is trivial, but you get the idea.
– ShadowRanger
Nov 8 '17 at 4:41
What was wrong with the oldmap
? Maybe with a new name (lmap
?) if the new default is to return an iterator?
– Giorgio
Oct 26 at 20:04
add a comment |
up vote
20
down vote
List-returning map function has the advantage of saving typing, especially during interactive sessions. You can define lmap
function (on the analogy of python2's imap
) that returns list:
lmap = lambda func, *iterable: list(map(func, *iterable))
Then calling lmap
instead of map
will do the job: lmap(str, x)
is shorter by 5 characters (30% in this case) than list(map(str, x))
and is certainly shorter than [str(v) for v in x]
. You may create similar functions for filter
too.
There was a comment to the original question:
I would suggest a rename to Getting map() to return a list in Python 3.* as it applies to all Python3 versions. Is there a way to do this? – meawoppl Jan 24 at 17:58
It is possible to do that, but it is a very bad idea. Just for fun, here's how you may (but should not) do it:
__global_map = map #keep reference to the original map
lmap = lambda func, *iterable: list(__global_map(func, *iterable)) # using "map" here will cause infinite recursion
map = lmap
x = [1, 2, 3]
map(str, x) #test
map = __global_map #restore the original map and don't do that again
map(str, x) #iterator
add a comment |
up vote
11
down vote
I'm not familiar with Python 3.1, but will this work?
[chr(x) for x in [66, 53, 0, 94]]
1
works perfectly.
– ExceptionSlayer
Apr 18 '16 at 9:52
add a comment |
up vote
3
down vote
Converting my old comment for better visibility: For a "better way to do this" without map
entirely, if your inputs are known to be ASCII ordinals, it's generally much faster to convert to bytes
and decode, a la bytes(list_of_ordinals).decode('ascii')
. That gets you a str
of the values, but if you need a list
for mutability or the like, you can just convert it (and it's still faster). For example, in ipython
microbenchmarks converting 45 inputs:
>>> %%timeit -r5 ordinals = list(range(45))
... list(map(chr, ordinals))
...
3.91 µs ± 60.2 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*map(chr, ordinals)]
...
3.84 µs ± 219 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*bytes(ordinals).decode('ascii')]
...
1.43 µs ± 49.7 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... bytes(ordinals).decode('ascii')
...
781 ns ± 15.9 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
If you leave it as a str
, it takes ~20% of the time of the fastest map
solutions; even converting back to list it's still less than 40% of the fastest map
solution. Bulk convert via bytes
and bytes.decode
then bulk converting back to list
saves a lot of work, but as noted, only works if all your inputs are ASCII ordinals (or ordinals in some one byte per character locale specific encoding, e.g. latin-1
).
add a comment |
up vote
0
down vote
list(map(chr, [66, 53, 0, 94]))
map(func, *iterables) --> map object
Make an iterator that computes the function using arguments from
each of the iterables. Stops when the shortest iterable is exhausted.
"Make an iterator"
means it will return an iterator.
"that computes the function using arguments from each of the iterables"
means that the next() function of the iterator will take one value of each iterables and pass each of them to one positional parameter of the function.
So you get an iterator from the map() funtion and jsut pass it to the list() builtin function or use list comprehensions.
add a comment |
up vote
0
down vote
In addition to above answers in Python 3
, we may simply create a list
of result values from a map
as
li =
for x in map(chr,[66,53,0,94]):
li.append(x)
print (li)
>>>['B', '5', 'x00', '^']
We may generalize by another example where I was struck, operations on map can also be handled in similar fashion like in regex
problem, we can write function to obtain list
of items to map and get result set at the same time. Ex.
b = 'Strings: 1,072, Another String: 474 '
li =
for x in map(int,map(int, re.findall('d+', b))):
li.append(x)
print (li)
>>>[1, 72, 474]
@miradulo I supposed in Python 2, a list was returned, but in Python 3, only type is returned and I just tried to give in same format. If you think its needless, there maybe people like me who can find it useful and thats why I added.
– Harry_pb
Jun 15 at 17:32
1
When there is already a list comprehension, a list function, and an unpacking answer, an explicit for loop doesn’t add much IMHO.
– miradulo
Jun 15 at 20:04
add a comment |
up vote
0
down vote
Using list comprehension in python and basic map function utility, one can do this also:
chi = [x for x in map(chr,[66,53,0,94])]
chi list will be containing, the ASIC value of the given elements.
– darshan k s
Nov 11 at 17:01
add a comment |
9 Answers
9
active
oldest
votes
9 Answers
9
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
588
down vote
accepted
Do this:
list(map(chr,[66,53,0,94]))
In Python 3+, many processes that iterate over iterables return iterators themselves. In most cases, this ends up saving memory, and should make things go faster.
If all you're going to do is iterate over this list eventually, there's no need to even convert it to a list, because you can still iterate over the map
object like so:
# Prints "ABCD"
for ch in map(chr,[65,66,67,68]):
print(ch)
1
Thank you for the great explanation!!
– mozami
Aug 20 '09 at 0:35
13
Of course, you can iterate over this, too: (chr(x) for x in [65,66,67,68]). It doesn't even need map.
– hughdbrown
Aug 20 '09 at 0:40
@hughdbrown The argument for using 3.1'smap
would be lazy evaluation when iterating on a complex function, large data sets, or streams.
– Andrew Keeton
Aug 20 '09 at 0:45
16
@Andrew actually Hugh is uing a generator comprehension which would do the same thing. Note the parentheses rather than square brackets.
– Triptych
Aug 20 '09 at 0:49
5
Alternate solution (faster for large inputs too) when the values are known to be ASCII/latin-1 is to do bulk conversions at the C layer:bytes(sequence_of_ints_in_range_0_to_256).decode('latin-1')
which makes astr
faster by avoiding Python function calls for each element in favor of a bulk conversion of all elements using only C level function calls. You can wrap the above inlist
if you really need alist
of the individual characters, but sincestr
is already an iterable of its own characters, the only reason you'd do so is if you need mutability.
– ShadowRanger
Jul 1 '16 at 1:52
|
show 4 more comments
up vote
588
down vote
accepted
Do this:
list(map(chr,[66,53,0,94]))
In Python 3+, many processes that iterate over iterables return iterators themselves. In most cases, this ends up saving memory, and should make things go faster.
If all you're going to do is iterate over this list eventually, there's no need to even convert it to a list, because you can still iterate over the map
object like so:
# Prints "ABCD"
for ch in map(chr,[65,66,67,68]):
print(ch)
1
Thank you for the great explanation!!
– mozami
Aug 20 '09 at 0:35
13
Of course, you can iterate over this, too: (chr(x) for x in [65,66,67,68]). It doesn't even need map.
– hughdbrown
Aug 20 '09 at 0:40
@hughdbrown The argument for using 3.1'smap
would be lazy evaluation when iterating on a complex function, large data sets, or streams.
– Andrew Keeton
Aug 20 '09 at 0:45
16
@Andrew actually Hugh is uing a generator comprehension which would do the same thing. Note the parentheses rather than square brackets.
– Triptych
Aug 20 '09 at 0:49
5
Alternate solution (faster for large inputs too) when the values are known to be ASCII/latin-1 is to do bulk conversions at the C layer:bytes(sequence_of_ints_in_range_0_to_256).decode('latin-1')
which makes astr
faster by avoiding Python function calls for each element in favor of a bulk conversion of all elements using only C level function calls. You can wrap the above inlist
if you really need alist
of the individual characters, but sincestr
is already an iterable of its own characters, the only reason you'd do so is if you need mutability.
– ShadowRanger
Jul 1 '16 at 1:52
|
show 4 more comments
up vote
588
down vote
accepted
up vote
588
down vote
accepted
Do this:
list(map(chr,[66,53,0,94]))
In Python 3+, many processes that iterate over iterables return iterators themselves. In most cases, this ends up saving memory, and should make things go faster.
If all you're going to do is iterate over this list eventually, there's no need to even convert it to a list, because you can still iterate over the map
object like so:
# Prints "ABCD"
for ch in map(chr,[65,66,67,68]):
print(ch)
Do this:
list(map(chr,[66,53,0,94]))
In Python 3+, many processes that iterate over iterables return iterators themselves. In most cases, this ends up saving memory, and should make things go faster.
If all you're going to do is iterate over this list eventually, there's no need to even convert it to a list, because you can still iterate over the map
object like so:
# Prints "ABCD"
for ch in map(chr,[65,66,67,68]):
print(ch)
edited Aug 20 '09 at 0:42
answered Aug 20 '09 at 0:28
Triptych
152k29134165
152k29134165
1
Thank you for the great explanation!!
– mozami
Aug 20 '09 at 0:35
13
Of course, you can iterate over this, too: (chr(x) for x in [65,66,67,68]). It doesn't even need map.
– hughdbrown
Aug 20 '09 at 0:40
@hughdbrown The argument for using 3.1'smap
would be lazy evaluation when iterating on a complex function, large data sets, or streams.
– Andrew Keeton
Aug 20 '09 at 0:45
16
@Andrew actually Hugh is uing a generator comprehension which would do the same thing. Note the parentheses rather than square brackets.
– Triptych
Aug 20 '09 at 0:49
5
Alternate solution (faster for large inputs too) when the values are known to be ASCII/latin-1 is to do bulk conversions at the C layer:bytes(sequence_of_ints_in_range_0_to_256).decode('latin-1')
which makes astr
faster by avoiding Python function calls for each element in favor of a bulk conversion of all elements using only C level function calls. You can wrap the above inlist
if you really need alist
of the individual characters, but sincestr
is already an iterable of its own characters, the only reason you'd do so is if you need mutability.
– ShadowRanger
Jul 1 '16 at 1:52
|
show 4 more comments
1
Thank you for the great explanation!!
– mozami
Aug 20 '09 at 0:35
13
Of course, you can iterate over this, too: (chr(x) for x in [65,66,67,68]). It doesn't even need map.
– hughdbrown
Aug 20 '09 at 0:40
@hughdbrown The argument for using 3.1'smap
would be lazy evaluation when iterating on a complex function, large data sets, or streams.
– Andrew Keeton
Aug 20 '09 at 0:45
16
@Andrew actually Hugh is uing a generator comprehension which would do the same thing. Note the parentheses rather than square brackets.
– Triptych
Aug 20 '09 at 0:49
5
Alternate solution (faster for large inputs too) when the values are known to be ASCII/latin-1 is to do bulk conversions at the C layer:bytes(sequence_of_ints_in_range_0_to_256).decode('latin-1')
which makes astr
faster by avoiding Python function calls for each element in favor of a bulk conversion of all elements using only C level function calls. You can wrap the above inlist
if you really need alist
of the individual characters, but sincestr
is already an iterable of its own characters, the only reason you'd do so is if you need mutability.
– ShadowRanger
Jul 1 '16 at 1:52
1
1
Thank you for the great explanation!!
– mozami
Aug 20 '09 at 0:35
Thank you for the great explanation!!
– mozami
Aug 20 '09 at 0:35
13
13
Of course, you can iterate over this, too: (chr(x) for x in [65,66,67,68]). It doesn't even need map.
– hughdbrown
Aug 20 '09 at 0:40
Of course, you can iterate over this, too: (chr(x) for x in [65,66,67,68]). It doesn't even need map.
– hughdbrown
Aug 20 '09 at 0:40
@hughdbrown The argument for using 3.1's
map
would be lazy evaluation when iterating on a complex function, large data sets, or streams.– Andrew Keeton
Aug 20 '09 at 0:45
@hughdbrown The argument for using 3.1's
map
would be lazy evaluation when iterating on a complex function, large data sets, or streams.– Andrew Keeton
Aug 20 '09 at 0:45
16
16
@Andrew actually Hugh is uing a generator comprehension which would do the same thing. Note the parentheses rather than square brackets.
– Triptych
Aug 20 '09 at 0:49
@Andrew actually Hugh is uing a generator comprehension which would do the same thing. Note the parentheses rather than square brackets.
– Triptych
Aug 20 '09 at 0:49
5
5
Alternate solution (faster for large inputs too) when the values are known to be ASCII/latin-1 is to do bulk conversions at the C layer:
bytes(sequence_of_ints_in_range_0_to_256).decode('latin-1')
which makes a str
faster by avoiding Python function calls for each element in favor of a bulk conversion of all elements using only C level function calls. You can wrap the above in list
if you really need a list
of the individual characters, but since str
is already an iterable of its own characters, the only reason you'd do so is if you need mutability.– ShadowRanger
Jul 1 '16 at 1:52
Alternate solution (faster for large inputs too) when the values are known to be ASCII/latin-1 is to do bulk conversions at the C layer:
bytes(sequence_of_ints_in_range_0_to_256).decode('latin-1')
which makes a str
faster by avoiding Python function calls for each element in favor of a bulk conversion of all elements using only C level function calls. You can wrap the above in list
if you really need a list
of the individual characters, but since str
is already an iterable of its own characters, the only reason you'd do so is if you need mutability.– ShadowRanger
Jul 1 '16 at 1:52
|
show 4 more comments
up vote
82
down vote
Why aren't you doing this:
[chr(x) for x in [66,53,0,94]]
It's called a list comprehension. You can find plenty of information on Google, but here's the link to the Python (2.6) documentation on list comprehensions. You might be more interested in the Python 3 documenation, though.
8
Yes to list comprehensions.
– hughdbrown
Aug 20 '09 at 0:29
4
Hmmmm. Maybe there needs to be a general posting on list comprehensions, generators, map(), zip(), and a lot of other speedy iteration goodness in python.
– hughdbrown
Aug 20 '09 at 0:55
33
I guess because it's more verbose, you have to write an extra variable (twice)... If the operation is more complex and you end up writing a lambda, or you need also to drop some elements, I think a comprehension is definitively better than a map+filter, but if you already have the function you want to apply, map is more succinct.
– fortran
Jun 25 '10 at 9:43
1
+1: Easier to read & allows you to use functions with many parameters
– Le Droid
Mar 6 '14 at 21:55
map(chr, [66,53,0,94])
is definitely more concise than[chr(x) for x in [66,53,0,94]]
.
– Giorgio
Oct 26 at 20:01
add a comment |
up vote
82
down vote
Why aren't you doing this:
[chr(x) for x in [66,53,0,94]]
It's called a list comprehension. You can find plenty of information on Google, but here's the link to the Python (2.6) documentation on list comprehensions. You might be more interested in the Python 3 documenation, though.
8
Yes to list comprehensions.
– hughdbrown
Aug 20 '09 at 0:29
4
Hmmmm. Maybe there needs to be a general posting on list comprehensions, generators, map(), zip(), and a lot of other speedy iteration goodness in python.
– hughdbrown
Aug 20 '09 at 0:55
33
I guess because it's more verbose, you have to write an extra variable (twice)... If the operation is more complex and you end up writing a lambda, or you need also to drop some elements, I think a comprehension is definitively better than a map+filter, but if you already have the function you want to apply, map is more succinct.
– fortran
Jun 25 '10 at 9:43
1
+1: Easier to read & allows you to use functions with many parameters
– Le Droid
Mar 6 '14 at 21:55
map(chr, [66,53,0,94])
is definitely more concise than[chr(x) for x in [66,53,0,94]]
.
– Giorgio
Oct 26 at 20:01
add a comment |
up vote
82
down vote
up vote
82
down vote
Why aren't you doing this:
[chr(x) for x in [66,53,0,94]]
It's called a list comprehension. You can find plenty of information on Google, but here's the link to the Python (2.6) documentation on list comprehensions. You might be more interested in the Python 3 documenation, though.
Why aren't you doing this:
[chr(x) for x in [66,53,0,94]]
It's called a list comprehension. You can find plenty of information on Google, but here's the link to the Python (2.6) documentation on list comprehensions. You might be more interested in the Python 3 documenation, though.
edited Aug 20 '09 at 0:36
answered Aug 20 '09 at 0:28


Mark Rushakoff
179k29359370
179k29359370
8
Yes to list comprehensions.
– hughdbrown
Aug 20 '09 at 0:29
4
Hmmmm. Maybe there needs to be a general posting on list comprehensions, generators, map(), zip(), and a lot of other speedy iteration goodness in python.
– hughdbrown
Aug 20 '09 at 0:55
33
I guess because it's more verbose, you have to write an extra variable (twice)... If the operation is more complex and you end up writing a lambda, or you need also to drop some elements, I think a comprehension is definitively better than a map+filter, but if you already have the function you want to apply, map is more succinct.
– fortran
Jun 25 '10 at 9:43
1
+1: Easier to read & allows you to use functions with many parameters
– Le Droid
Mar 6 '14 at 21:55
map(chr, [66,53,0,94])
is definitely more concise than[chr(x) for x in [66,53,0,94]]
.
– Giorgio
Oct 26 at 20:01
add a comment |
8
Yes to list comprehensions.
– hughdbrown
Aug 20 '09 at 0:29
4
Hmmmm. Maybe there needs to be a general posting on list comprehensions, generators, map(), zip(), and a lot of other speedy iteration goodness in python.
– hughdbrown
Aug 20 '09 at 0:55
33
I guess because it's more verbose, you have to write an extra variable (twice)... If the operation is more complex and you end up writing a lambda, or you need also to drop some elements, I think a comprehension is definitively better than a map+filter, but if you already have the function you want to apply, map is more succinct.
– fortran
Jun 25 '10 at 9:43
1
+1: Easier to read & allows you to use functions with many parameters
– Le Droid
Mar 6 '14 at 21:55
map(chr, [66,53,0,94])
is definitely more concise than[chr(x) for x in [66,53,0,94]]
.
– Giorgio
Oct 26 at 20:01
8
8
Yes to list comprehensions.
– hughdbrown
Aug 20 '09 at 0:29
Yes to list comprehensions.
– hughdbrown
Aug 20 '09 at 0:29
4
4
Hmmmm. Maybe there needs to be a general posting on list comprehensions, generators, map(), zip(), and a lot of other speedy iteration goodness in python.
– hughdbrown
Aug 20 '09 at 0:55
Hmmmm. Maybe there needs to be a general posting on list comprehensions, generators, map(), zip(), and a lot of other speedy iteration goodness in python.
– hughdbrown
Aug 20 '09 at 0:55
33
33
I guess because it's more verbose, you have to write an extra variable (twice)... If the operation is more complex and you end up writing a lambda, or you need also to drop some elements, I think a comprehension is definitively better than a map+filter, but if you already have the function you want to apply, map is more succinct.
– fortran
Jun 25 '10 at 9:43
I guess because it's more verbose, you have to write an extra variable (twice)... If the operation is more complex and you end up writing a lambda, or you need also to drop some elements, I think a comprehension is definitively better than a map+filter, but if you already have the function you want to apply, map is more succinct.
– fortran
Jun 25 '10 at 9:43
1
1
+1: Easier to read & allows you to use functions with many parameters
– Le Droid
Mar 6 '14 at 21:55
+1: Easier to read & allows you to use functions with many parameters
– Le Droid
Mar 6 '14 at 21:55
map(chr, [66,53,0,94])
is definitely more concise than [chr(x) for x in [66,53,0,94]]
.– Giorgio
Oct 26 at 20:01
map(chr, [66,53,0,94])
is definitely more concise than [chr(x) for x in [66,53,0,94]]
.– Giorgio
Oct 26 at 20:01
add a comment |
up vote
63
down vote
New and neat in Python 3.5:
[*map(chr, [66, 53, 0, 94])]
Thanks to Additional Unpacking Generalizations
UPDATE
Always seeking for shorter ways, I discovered this one also works:
*map(chr, [66, 53, 0, 94]),
Unpacking works in tuples too. Note the comma at the end. This makes it a tuple of 1 element. That is, it's equivalent to (*map(chr, [66, 53, 0, 94]),)
It's shorter by only one char from the version with the list-brackets, but, in my opinion, better to write, because you start right ahead with the asterisk - the expansion syntax, so I feel it's softer on the mind. :)
10
What's wrong withlist()
?
– Quelklef
Aug 12 '17 at 12:56
8
@Quelkleflist()
doesn't look as neat
– Arijoon
Sep 28 '17 at 13:15
3
@Quelklef: Also, the unpacking approach is trivially faster thanks to not needing to look up thelist
constructor and invoke general function call machinery. For a long input, it won't matter; for a short one, it can make a big difference. Using the above code with the input as atuple
so it's not repeatedly reconstructed,ipython
microbenchmarks show thelist()
wrapping approach takes about 20% longer than unpacking. Mind you, in absolute terms, we're talking about 150 ns, which is trivial, but you get the idea.
– ShadowRanger
Nov 8 '17 at 4:41
What was wrong with the oldmap
? Maybe with a new name (lmap
?) if the new default is to return an iterator?
– Giorgio
Oct 26 at 20:04
add a comment |
up vote
63
down vote
New and neat in Python 3.5:
[*map(chr, [66, 53, 0, 94])]
Thanks to Additional Unpacking Generalizations
UPDATE
Always seeking for shorter ways, I discovered this one also works:
*map(chr, [66, 53, 0, 94]),
Unpacking works in tuples too. Note the comma at the end. This makes it a tuple of 1 element. That is, it's equivalent to (*map(chr, [66, 53, 0, 94]),)
It's shorter by only one char from the version with the list-brackets, but, in my opinion, better to write, because you start right ahead with the asterisk - the expansion syntax, so I feel it's softer on the mind. :)
10
What's wrong withlist()
?
– Quelklef
Aug 12 '17 at 12:56
8
@Quelkleflist()
doesn't look as neat
– Arijoon
Sep 28 '17 at 13:15
3
@Quelklef: Also, the unpacking approach is trivially faster thanks to not needing to look up thelist
constructor and invoke general function call machinery. For a long input, it won't matter; for a short one, it can make a big difference. Using the above code with the input as atuple
so it's not repeatedly reconstructed,ipython
microbenchmarks show thelist()
wrapping approach takes about 20% longer than unpacking. Mind you, in absolute terms, we're talking about 150 ns, which is trivial, but you get the idea.
– ShadowRanger
Nov 8 '17 at 4:41
What was wrong with the oldmap
? Maybe with a new name (lmap
?) if the new default is to return an iterator?
– Giorgio
Oct 26 at 20:04
add a comment |
up vote
63
down vote
up vote
63
down vote
New and neat in Python 3.5:
[*map(chr, [66, 53, 0, 94])]
Thanks to Additional Unpacking Generalizations
UPDATE
Always seeking for shorter ways, I discovered this one also works:
*map(chr, [66, 53, 0, 94]),
Unpacking works in tuples too. Note the comma at the end. This makes it a tuple of 1 element. That is, it's equivalent to (*map(chr, [66, 53, 0, 94]),)
It's shorter by only one char from the version with the list-brackets, but, in my opinion, better to write, because you start right ahead with the asterisk - the expansion syntax, so I feel it's softer on the mind. :)
New and neat in Python 3.5:
[*map(chr, [66, 53, 0, 94])]
Thanks to Additional Unpacking Generalizations
UPDATE
Always seeking for shorter ways, I discovered this one also works:
*map(chr, [66, 53, 0, 94]),
Unpacking works in tuples too. Note the comma at the end. This makes it a tuple of 1 element. That is, it's equivalent to (*map(chr, [66, 53, 0, 94]),)
It's shorter by only one char from the version with the list-brackets, but, in my opinion, better to write, because you start right ahead with the asterisk - the expansion syntax, so I feel it's softer on the mind. :)
edited Oct 28 at 11:25
answered Aug 1 '16 at 15:18


Israel Unterman
8,49211829
8,49211829
10
What's wrong withlist()
?
– Quelklef
Aug 12 '17 at 12:56
8
@Quelkleflist()
doesn't look as neat
– Arijoon
Sep 28 '17 at 13:15
3
@Quelklef: Also, the unpacking approach is trivially faster thanks to not needing to look up thelist
constructor and invoke general function call machinery. For a long input, it won't matter; for a short one, it can make a big difference. Using the above code with the input as atuple
so it's not repeatedly reconstructed,ipython
microbenchmarks show thelist()
wrapping approach takes about 20% longer than unpacking. Mind you, in absolute terms, we're talking about 150 ns, which is trivial, but you get the idea.
– ShadowRanger
Nov 8 '17 at 4:41
What was wrong with the oldmap
? Maybe with a new name (lmap
?) if the new default is to return an iterator?
– Giorgio
Oct 26 at 20:04
add a comment |
10
What's wrong withlist()
?
– Quelklef
Aug 12 '17 at 12:56
8
@Quelkleflist()
doesn't look as neat
– Arijoon
Sep 28 '17 at 13:15
3
@Quelklef: Also, the unpacking approach is trivially faster thanks to not needing to look up thelist
constructor and invoke general function call machinery. For a long input, it won't matter; for a short one, it can make a big difference. Using the above code with the input as atuple
so it's not repeatedly reconstructed,ipython
microbenchmarks show thelist()
wrapping approach takes about 20% longer than unpacking. Mind you, in absolute terms, we're talking about 150 ns, which is trivial, but you get the idea.
– ShadowRanger
Nov 8 '17 at 4:41
What was wrong with the oldmap
? Maybe with a new name (lmap
?) if the new default is to return an iterator?
– Giorgio
Oct 26 at 20:04
10
10
What's wrong with
list()
?– Quelklef
Aug 12 '17 at 12:56
What's wrong with
list()
?– Quelklef
Aug 12 '17 at 12:56
8
8
@Quelklef
list()
doesn't look as neat– Arijoon
Sep 28 '17 at 13:15
@Quelklef
list()
doesn't look as neat– Arijoon
Sep 28 '17 at 13:15
3
3
@Quelklef: Also, the unpacking approach is trivially faster thanks to not needing to look up the
list
constructor and invoke general function call machinery. For a long input, it won't matter; for a short one, it can make a big difference. Using the above code with the input as a tuple
so it's not repeatedly reconstructed, ipython
microbenchmarks show the list()
wrapping approach takes about 20% longer than unpacking. Mind you, in absolute terms, we're talking about 150 ns, which is trivial, but you get the idea.– ShadowRanger
Nov 8 '17 at 4:41
@Quelklef: Also, the unpacking approach is trivially faster thanks to not needing to look up the
list
constructor and invoke general function call machinery. For a long input, it won't matter; for a short one, it can make a big difference. Using the above code with the input as a tuple
so it's not repeatedly reconstructed, ipython
microbenchmarks show the list()
wrapping approach takes about 20% longer than unpacking. Mind you, in absolute terms, we're talking about 150 ns, which is trivial, but you get the idea.– ShadowRanger
Nov 8 '17 at 4:41
What was wrong with the old
map
? Maybe with a new name (lmap
?) if the new default is to return an iterator?– Giorgio
Oct 26 at 20:04
What was wrong with the old
map
? Maybe with a new name (lmap
?) if the new default is to return an iterator?– Giorgio
Oct 26 at 20:04
add a comment |
up vote
20
down vote
List-returning map function has the advantage of saving typing, especially during interactive sessions. You can define lmap
function (on the analogy of python2's imap
) that returns list:
lmap = lambda func, *iterable: list(map(func, *iterable))
Then calling lmap
instead of map
will do the job: lmap(str, x)
is shorter by 5 characters (30% in this case) than list(map(str, x))
and is certainly shorter than [str(v) for v in x]
. You may create similar functions for filter
too.
There was a comment to the original question:
I would suggest a rename to Getting map() to return a list in Python 3.* as it applies to all Python3 versions. Is there a way to do this? – meawoppl Jan 24 at 17:58
It is possible to do that, but it is a very bad idea. Just for fun, here's how you may (but should not) do it:
__global_map = map #keep reference to the original map
lmap = lambda func, *iterable: list(__global_map(func, *iterable)) # using "map" here will cause infinite recursion
map = lmap
x = [1, 2, 3]
map(str, x) #test
map = __global_map #restore the original map and don't do that again
map(str, x) #iterator
add a comment |
up vote
20
down vote
List-returning map function has the advantage of saving typing, especially during interactive sessions. You can define lmap
function (on the analogy of python2's imap
) that returns list:
lmap = lambda func, *iterable: list(map(func, *iterable))
Then calling lmap
instead of map
will do the job: lmap(str, x)
is shorter by 5 characters (30% in this case) than list(map(str, x))
and is certainly shorter than [str(v) for v in x]
. You may create similar functions for filter
too.
There was a comment to the original question:
I would suggest a rename to Getting map() to return a list in Python 3.* as it applies to all Python3 versions. Is there a way to do this? – meawoppl Jan 24 at 17:58
It is possible to do that, but it is a very bad idea. Just for fun, here's how you may (but should not) do it:
__global_map = map #keep reference to the original map
lmap = lambda func, *iterable: list(__global_map(func, *iterable)) # using "map" here will cause infinite recursion
map = lmap
x = [1, 2, 3]
map(str, x) #test
map = __global_map #restore the original map and don't do that again
map(str, x) #iterator
add a comment |
up vote
20
down vote
up vote
20
down vote
List-returning map function has the advantage of saving typing, especially during interactive sessions. You can define lmap
function (on the analogy of python2's imap
) that returns list:
lmap = lambda func, *iterable: list(map(func, *iterable))
Then calling lmap
instead of map
will do the job: lmap(str, x)
is shorter by 5 characters (30% in this case) than list(map(str, x))
and is certainly shorter than [str(v) for v in x]
. You may create similar functions for filter
too.
There was a comment to the original question:
I would suggest a rename to Getting map() to return a list in Python 3.* as it applies to all Python3 versions. Is there a way to do this? – meawoppl Jan 24 at 17:58
It is possible to do that, but it is a very bad idea. Just for fun, here's how you may (but should not) do it:
__global_map = map #keep reference to the original map
lmap = lambda func, *iterable: list(__global_map(func, *iterable)) # using "map" here will cause infinite recursion
map = lmap
x = [1, 2, 3]
map(str, x) #test
map = __global_map #restore the original map and don't do that again
map(str, x) #iterator
List-returning map function has the advantage of saving typing, especially during interactive sessions. You can define lmap
function (on the analogy of python2's imap
) that returns list:
lmap = lambda func, *iterable: list(map(func, *iterable))
Then calling lmap
instead of map
will do the job: lmap(str, x)
is shorter by 5 characters (30% in this case) than list(map(str, x))
and is certainly shorter than [str(v) for v in x]
. You may create similar functions for filter
too.
There was a comment to the original question:
I would suggest a rename to Getting map() to return a list in Python 3.* as it applies to all Python3 versions. Is there a way to do this? – meawoppl Jan 24 at 17:58
It is possible to do that, but it is a very bad idea. Just for fun, here's how you may (but should not) do it:
__global_map = map #keep reference to the original map
lmap = lambda func, *iterable: list(__global_map(func, *iterable)) # using "map" here will cause infinite recursion
map = lmap
x = [1, 2, 3]
map(str, x) #test
map = __global_map #restore the original map and don't do that again
map(str, x) #iterator
edited May 25 at 18:01
Michael Goldshteyn
49.7k1499160
49.7k1499160
answered Jul 1 '14 at 9:41
Boris Gorelik
11.3k2296143
11.3k2296143
add a comment |
add a comment |
up vote
11
down vote
I'm not familiar with Python 3.1, but will this work?
[chr(x) for x in [66, 53, 0, 94]]
1
works perfectly.
– ExceptionSlayer
Apr 18 '16 at 9:52
add a comment |
up vote
11
down vote
I'm not familiar with Python 3.1, but will this work?
[chr(x) for x in [66, 53, 0, 94]]
1
works perfectly.
– ExceptionSlayer
Apr 18 '16 at 9:52
add a comment |
up vote
11
down vote
up vote
11
down vote
I'm not familiar with Python 3.1, but will this work?
[chr(x) for x in [66, 53, 0, 94]]
I'm not familiar with Python 3.1, but will this work?
[chr(x) for x in [66, 53, 0, 94]]
answered Aug 20 '09 at 0:28
Andrew Keeton
14.2k53464
14.2k53464
1
works perfectly.
– ExceptionSlayer
Apr 18 '16 at 9:52
add a comment |
1
works perfectly.
– ExceptionSlayer
Apr 18 '16 at 9:52
1
1
works perfectly.
– ExceptionSlayer
Apr 18 '16 at 9:52
works perfectly.
– ExceptionSlayer
Apr 18 '16 at 9:52
add a comment |
up vote
3
down vote
Converting my old comment for better visibility: For a "better way to do this" without map
entirely, if your inputs are known to be ASCII ordinals, it's generally much faster to convert to bytes
and decode, a la bytes(list_of_ordinals).decode('ascii')
. That gets you a str
of the values, but if you need a list
for mutability or the like, you can just convert it (and it's still faster). For example, in ipython
microbenchmarks converting 45 inputs:
>>> %%timeit -r5 ordinals = list(range(45))
... list(map(chr, ordinals))
...
3.91 µs ± 60.2 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*map(chr, ordinals)]
...
3.84 µs ± 219 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*bytes(ordinals).decode('ascii')]
...
1.43 µs ± 49.7 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... bytes(ordinals).decode('ascii')
...
781 ns ± 15.9 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
If you leave it as a str
, it takes ~20% of the time of the fastest map
solutions; even converting back to list it's still less than 40% of the fastest map
solution. Bulk convert via bytes
and bytes.decode
then bulk converting back to list
saves a lot of work, but as noted, only works if all your inputs are ASCII ordinals (or ordinals in some one byte per character locale specific encoding, e.g. latin-1
).
add a comment |
up vote
3
down vote
Converting my old comment for better visibility: For a "better way to do this" without map
entirely, if your inputs are known to be ASCII ordinals, it's generally much faster to convert to bytes
and decode, a la bytes(list_of_ordinals).decode('ascii')
. That gets you a str
of the values, but if you need a list
for mutability or the like, you can just convert it (and it's still faster). For example, in ipython
microbenchmarks converting 45 inputs:
>>> %%timeit -r5 ordinals = list(range(45))
... list(map(chr, ordinals))
...
3.91 µs ± 60.2 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*map(chr, ordinals)]
...
3.84 µs ± 219 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*bytes(ordinals).decode('ascii')]
...
1.43 µs ± 49.7 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... bytes(ordinals).decode('ascii')
...
781 ns ± 15.9 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
If you leave it as a str
, it takes ~20% of the time of the fastest map
solutions; even converting back to list it's still less than 40% of the fastest map
solution. Bulk convert via bytes
and bytes.decode
then bulk converting back to list
saves a lot of work, but as noted, only works if all your inputs are ASCII ordinals (or ordinals in some one byte per character locale specific encoding, e.g. latin-1
).
add a comment |
up vote
3
down vote
up vote
3
down vote
Converting my old comment for better visibility: For a "better way to do this" without map
entirely, if your inputs are known to be ASCII ordinals, it's generally much faster to convert to bytes
and decode, a la bytes(list_of_ordinals).decode('ascii')
. That gets you a str
of the values, but if you need a list
for mutability or the like, you can just convert it (and it's still faster). For example, in ipython
microbenchmarks converting 45 inputs:
>>> %%timeit -r5 ordinals = list(range(45))
... list(map(chr, ordinals))
...
3.91 µs ± 60.2 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*map(chr, ordinals)]
...
3.84 µs ± 219 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*bytes(ordinals).decode('ascii')]
...
1.43 µs ± 49.7 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... bytes(ordinals).decode('ascii')
...
781 ns ± 15.9 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
If you leave it as a str
, it takes ~20% of the time of the fastest map
solutions; even converting back to list it's still less than 40% of the fastest map
solution. Bulk convert via bytes
and bytes.decode
then bulk converting back to list
saves a lot of work, but as noted, only works if all your inputs are ASCII ordinals (or ordinals in some one byte per character locale specific encoding, e.g. latin-1
).
Converting my old comment for better visibility: For a "better way to do this" without map
entirely, if your inputs are known to be ASCII ordinals, it's generally much faster to convert to bytes
and decode, a la bytes(list_of_ordinals).decode('ascii')
. That gets you a str
of the values, but if you need a list
for mutability or the like, you can just convert it (and it's still faster). For example, in ipython
microbenchmarks converting 45 inputs:
>>> %%timeit -r5 ordinals = list(range(45))
... list(map(chr, ordinals))
...
3.91 µs ± 60.2 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*map(chr, ordinals)]
...
3.84 µs ± 219 ns per loop (mean ± std. dev. of 5 runs, 100000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... [*bytes(ordinals).decode('ascii')]
...
1.43 µs ± 49.7 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
>>> %%timeit -r5 ordinals = list(range(45))
... bytes(ordinals).decode('ascii')
...
781 ns ± 15.9 ns per loop (mean ± std. dev. of 5 runs, 1000000 loops each)
If you leave it as a str
, it takes ~20% of the time of the fastest map
solutions; even converting back to list it's still less than 40% of the fastest map
solution. Bulk convert via bytes
and bytes.decode
then bulk converting back to list
saves a lot of work, but as noted, only works if all your inputs are ASCII ordinals (or ordinals in some one byte per character locale specific encoding, e.g. latin-1
).
answered Nov 8 '17 at 4:52


ShadowRanger
56.2k44992
56.2k44992
add a comment |
add a comment |
up vote
0
down vote
list(map(chr, [66, 53, 0, 94]))
map(func, *iterables) --> map object
Make an iterator that computes the function using arguments from
each of the iterables. Stops when the shortest iterable is exhausted.
"Make an iterator"
means it will return an iterator.
"that computes the function using arguments from each of the iterables"
means that the next() function of the iterator will take one value of each iterables and pass each of them to one positional parameter of the function.
So you get an iterator from the map() funtion and jsut pass it to the list() builtin function or use list comprehensions.
add a comment |
up vote
0
down vote
list(map(chr, [66, 53, 0, 94]))
map(func, *iterables) --> map object
Make an iterator that computes the function using arguments from
each of the iterables. Stops when the shortest iterable is exhausted.
"Make an iterator"
means it will return an iterator.
"that computes the function using arguments from each of the iterables"
means that the next() function of the iterator will take one value of each iterables and pass each of them to one positional parameter of the function.
So you get an iterator from the map() funtion and jsut pass it to the list() builtin function or use list comprehensions.
add a comment |
up vote
0
down vote
up vote
0
down vote
list(map(chr, [66, 53, 0, 94]))
map(func, *iterables) --> map object
Make an iterator that computes the function using arguments from
each of the iterables. Stops when the shortest iterable is exhausted.
"Make an iterator"
means it will return an iterator.
"that computes the function using arguments from each of the iterables"
means that the next() function of the iterator will take one value of each iterables and pass each of them to one positional parameter of the function.
So you get an iterator from the map() funtion and jsut pass it to the list() builtin function or use list comprehensions.
list(map(chr, [66, 53, 0, 94]))
map(func, *iterables) --> map object
Make an iterator that computes the function using arguments from
each of the iterables. Stops when the shortest iterable is exhausted.
"Make an iterator"
means it will return an iterator.
"that computes the function using arguments from each of the iterables"
means that the next() function of the iterator will take one value of each iterables and pass each of them to one positional parameter of the function.
So you get an iterator from the map() funtion and jsut pass it to the list() builtin function or use list comprehensions.
answered Nov 7 '17 at 9:04
Ini
216315
216315
add a comment |
add a comment |
up vote
0
down vote
In addition to above answers in Python 3
, we may simply create a list
of result values from a map
as
li =
for x in map(chr,[66,53,0,94]):
li.append(x)
print (li)
>>>['B', '5', 'x00', '^']
We may generalize by another example where I was struck, operations on map can also be handled in similar fashion like in regex
problem, we can write function to obtain list
of items to map and get result set at the same time. Ex.
b = 'Strings: 1,072, Another String: 474 '
li =
for x in map(int,map(int, re.findall('d+', b))):
li.append(x)
print (li)
>>>[1, 72, 474]
@miradulo I supposed in Python 2, a list was returned, but in Python 3, only type is returned and I just tried to give in same format. If you think its needless, there maybe people like me who can find it useful and thats why I added.
– Harry_pb
Jun 15 at 17:32
1
When there is already a list comprehension, a list function, and an unpacking answer, an explicit for loop doesn’t add much IMHO.
– miradulo
Jun 15 at 20:04
add a comment |
up vote
0
down vote
In addition to above answers in Python 3
, we may simply create a list
of result values from a map
as
li =
for x in map(chr,[66,53,0,94]):
li.append(x)
print (li)
>>>['B', '5', 'x00', '^']
We may generalize by another example where I was struck, operations on map can also be handled in similar fashion like in regex
problem, we can write function to obtain list
of items to map and get result set at the same time. Ex.
b = 'Strings: 1,072, Another String: 474 '
li =
for x in map(int,map(int, re.findall('d+', b))):
li.append(x)
print (li)
>>>[1, 72, 474]
@miradulo I supposed in Python 2, a list was returned, but in Python 3, only type is returned and I just tried to give in same format. If you think its needless, there maybe people like me who can find it useful and thats why I added.
– Harry_pb
Jun 15 at 17:32
1
When there is already a list comprehension, a list function, and an unpacking answer, an explicit for loop doesn’t add much IMHO.
– miradulo
Jun 15 at 20:04
add a comment |
up vote
0
down vote
up vote
0
down vote
In addition to above answers in Python 3
, we may simply create a list
of result values from a map
as
li =
for x in map(chr,[66,53,0,94]):
li.append(x)
print (li)
>>>['B', '5', 'x00', '^']
We may generalize by another example where I was struck, operations on map can also be handled in similar fashion like in regex
problem, we can write function to obtain list
of items to map and get result set at the same time. Ex.
b = 'Strings: 1,072, Another String: 474 '
li =
for x in map(int,map(int, re.findall('d+', b))):
li.append(x)
print (li)
>>>[1, 72, 474]
In addition to above answers in Python 3
, we may simply create a list
of result values from a map
as
li =
for x in map(chr,[66,53,0,94]):
li.append(x)
print (li)
>>>['B', '5', 'x00', '^']
We may generalize by another example where I was struck, operations on map can also be handled in similar fashion like in regex
problem, we can write function to obtain list
of items to map and get result set at the same time. Ex.
b = 'Strings: 1,072, Another String: 474 '
li =
for x in map(int,map(int, re.findall('d+', b))):
li.append(x)
print (li)
>>>[1, 72, 474]
answered Jun 15 at 16:35
Harry_pb
1,61511125
1,61511125
@miradulo I supposed in Python 2, a list was returned, but in Python 3, only type is returned and I just tried to give in same format. If you think its needless, there maybe people like me who can find it useful and thats why I added.
– Harry_pb
Jun 15 at 17:32
1
When there is already a list comprehension, a list function, and an unpacking answer, an explicit for loop doesn’t add much IMHO.
– miradulo
Jun 15 at 20:04
add a comment |
@miradulo I supposed in Python 2, a list was returned, but in Python 3, only type is returned and I just tried to give in same format. If you think its needless, there maybe people like me who can find it useful and thats why I added.
– Harry_pb
Jun 15 at 17:32
1
When there is already a list comprehension, a list function, and an unpacking answer, an explicit for loop doesn’t add much IMHO.
– miradulo
Jun 15 at 20:04
@miradulo I supposed in Python 2, a list was returned, but in Python 3, only type is returned and I just tried to give in same format. If you think its needless, there maybe people like me who can find it useful and thats why I added.
– Harry_pb
Jun 15 at 17:32
@miradulo I supposed in Python 2, a list was returned, but in Python 3, only type is returned and I just tried to give in same format. If you think its needless, there maybe people like me who can find it useful and thats why I added.
– Harry_pb
Jun 15 at 17:32
1
1
When there is already a list comprehension, a list function, and an unpacking answer, an explicit for loop doesn’t add much IMHO.
– miradulo
Jun 15 at 20:04
When there is already a list comprehension, a list function, and an unpacking answer, an explicit for loop doesn’t add much IMHO.
– miradulo
Jun 15 at 20:04
add a comment |
up vote
0
down vote
Using list comprehension in python and basic map function utility, one can do this also:
chi = [x for x in map(chr,[66,53,0,94])]
chi list will be containing, the ASIC value of the given elements.
– darshan k s
Nov 11 at 17:01
add a comment |
up vote
0
down vote
Using list comprehension in python and basic map function utility, one can do this also:
chi = [x for x in map(chr,[66,53,0,94])]
chi list will be containing, the ASIC value of the given elements.
– darshan k s
Nov 11 at 17:01
add a comment |
up vote
0
down vote
up vote
0
down vote
Using list comprehension in python and basic map function utility, one can do this also:
chi = [x for x in map(chr,[66,53,0,94])]
Using list comprehension in python and basic map function utility, one can do this also:
chi = [x for x in map(chr,[66,53,0,94])]
edited Nov 11 at 17:29
rassar
2,20811029
2,20811029
answered Nov 11 at 16:57
darshan k s
1
1
chi list will be containing, the ASIC value of the given elements.
– darshan k s
Nov 11 at 17:01
add a comment |
chi list will be containing, the ASIC value of the given elements.
– darshan k s
Nov 11 at 17:01
chi list will be containing, the ASIC value of the given elements.
– darshan k s
Nov 11 at 17:01
chi list will be containing, the ASIC value of the given elements.
– darshan k s
Nov 11 at 17:01
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f1303347%2fgetting-a-map-to-return-a-list-in-python-3-x%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
47hjjaEmAYWRLluC0,vQ5Z mb3vq