reproduce OutOfMemoryError with -Xss
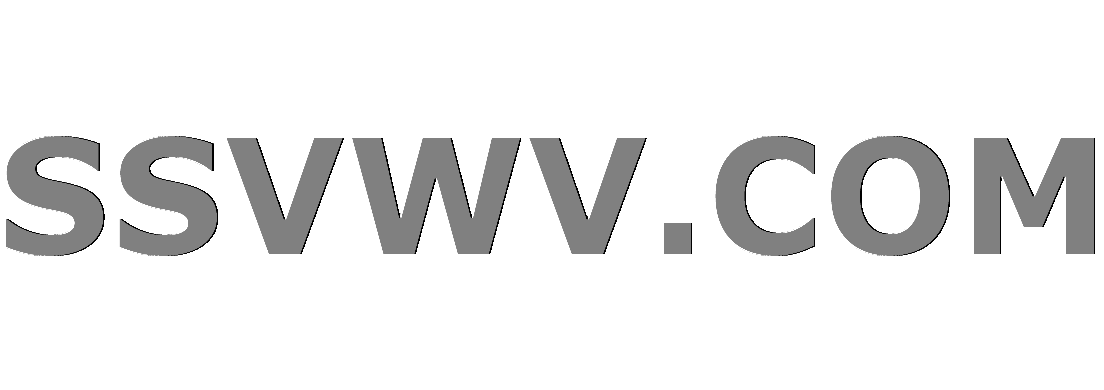
Multi tool use
up vote
1
down vote
favorite
I'm trying to reproduce java.lang.OutOfMemoryError: unable to create new native thread
But with -Xss VM argument.
I'm guessing that if we have a large number of threads, and every thread takes X stack space, I will have the exception if threads*X > total stack size.
But nothing happened.
my tester:
`
public static void main(String args) throws Exception
ThreadPoolExecutor executor = new ThreadPoolExecutor(1000, 15000, 0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
int i = 0;
try
Thread.sleep(100);
for (; i <= 10; i++)
Runnable t = new Runnable()
List<Object> objects = new LinkedList<>();
public void run()
while (true)
objects.add(new Object());
;
executor.submit(t);
catch (Throwable e)
System.err.println("stop with " + i + " threads, " + e);
System.err.println("task count " + executor.getTaskCount());
System.out.println("get active thread count " + executor.getActiveCount());
executor.shutdownNow();
`
And my VM args are
-Xms512m -Xmx512m -Xss1g
Any Idea why I'm not having the exception? and how do I repudce it?
thanks
java exception jvm out-of-memory
add a comment |
up vote
1
down vote
favorite
I'm trying to reproduce java.lang.OutOfMemoryError: unable to create new native thread
But with -Xss VM argument.
I'm guessing that if we have a large number of threads, and every thread takes X stack space, I will have the exception if threads*X > total stack size.
But nothing happened.
my tester:
`
public static void main(String args) throws Exception
ThreadPoolExecutor executor = new ThreadPoolExecutor(1000, 15000, 0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
int i = 0;
try
Thread.sleep(100);
for (; i <= 10; i++)
Runnable t = new Runnable()
List<Object> objects = new LinkedList<>();
public void run()
while (true)
objects.add(new Object());
;
executor.submit(t);
catch (Throwable e)
System.err.println("stop with " + i + " threads, " + e);
System.err.println("task count " + executor.getTaskCount());
System.out.println("get active thread count " + executor.getActiveCount());
executor.shutdownNow();
`
And my VM args are
-Xms512m -Xmx512m -Xss1g
Any Idea why I'm not having the exception? and how do I repudce it?
thanks
java exception jvm out-of-memory
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to reproduce java.lang.OutOfMemoryError: unable to create new native thread
But with -Xss VM argument.
I'm guessing that if we have a large number of threads, and every thread takes X stack space, I will have the exception if threads*X > total stack size.
But nothing happened.
my tester:
`
public static void main(String args) throws Exception
ThreadPoolExecutor executor = new ThreadPoolExecutor(1000, 15000, 0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
int i = 0;
try
Thread.sleep(100);
for (; i <= 10; i++)
Runnable t = new Runnable()
List<Object> objects = new LinkedList<>();
public void run()
while (true)
objects.add(new Object());
;
executor.submit(t);
catch (Throwable e)
System.err.println("stop with " + i + " threads, " + e);
System.err.println("task count " + executor.getTaskCount());
System.out.println("get active thread count " + executor.getActiveCount());
executor.shutdownNow();
`
And my VM args are
-Xms512m -Xmx512m -Xss1g
Any Idea why I'm not having the exception? and how do I repudce it?
thanks
java exception jvm out-of-memory
I'm trying to reproduce java.lang.OutOfMemoryError: unable to create new native thread
But with -Xss VM argument.
I'm guessing that if we have a large number of threads, and every thread takes X stack space, I will have the exception if threads*X > total stack size.
But nothing happened.
my tester:
`
public static void main(String args) throws Exception
ThreadPoolExecutor executor = new ThreadPoolExecutor(1000, 15000, 0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
int i = 0;
try
Thread.sleep(100);
for (; i <= 10; i++)
Runnable t = new Runnable()
List<Object> objects = new LinkedList<>();
public void run()
while (true)
objects.add(new Object());
;
executor.submit(t);
catch (Throwable e)
System.err.println("stop with " + i + " threads, " + e);
System.err.println("task count " + executor.getTaskCount());
System.out.println("get active thread count " + executor.getActiveCount());
executor.shutdownNow();
`
And my VM args are
-Xms512m -Xmx512m -Xss1g
Any Idea why I'm not having the exception? and how do I repudce it?
thanks
java exception jvm out-of-memory
java exception jvm out-of-memory
asked Nov 11 at 15:51
user2302639
4716
4716
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
3
down vote
On most OSEes, the stack is allocated lazily, ie only the pages you actually use turn into real memory. Your process is limited to 128 to 256 TB of virtual memory per process, depending on the OS you are using, so at 1 GB per thread, you need at least 128k threads. I would try a much larger stack. E.g. 256g
EDIT: Trying this myself, it looks like it ignores stack sizes of 4g and above. The largest size is -Xss4000m on windows.
Trying to reproduce this on Windows, and it appears to overload the machine before any exception is thrown.
This is what I tried. Run with -Xss4000m
, it got to over 20 threads (total 80g before my windows laptop stopped working)
You might find in Linux, it will reach a ulimit
before overloading the machine.
import java.util.concurrent.*;
class A
public static void main(String args) throws InterruptedException
ThreadPoolExecutor pool = new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<>());
try
for (int i = 0; i < 100; i++)
System.out.println(i);
pool.submit(() ->
try
System.out.println(recurse() + " size " + pool.getPoolSize());
catch (Throwable t)
t.printStackTrace();
return null;
);
Thread.sleep(1000);
finally
pool.shutdown();
static long recurse()
try
return 1 + recurse();
catch (Error e)
try
Thread.sleep(10000);
catch (InterruptedException e1)
e1.printStackTrace();
return 1;
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
On most OSEes, the stack is allocated lazily, ie only the pages you actually use turn into real memory. Your process is limited to 128 to 256 TB of virtual memory per process, depending on the OS you are using, so at 1 GB per thread, you need at least 128k threads. I would try a much larger stack. E.g. 256g
EDIT: Trying this myself, it looks like it ignores stack sizes of 4g and above. The largest size is -Xss4000m on windows.
Trying to reproduce this on Windows, and it appears to overload the machine before any exception is thrown.
This is what I tried. Run with -Xss4000m
, it got to over 20 threads (total 80g before my windows laptop stopped working)
You might find in Linux, it will reach a ulimit
before overloading the machine.
import java.util.concurrent.*;
class A
public static void main(String args) throws InterruptedException
ThreadPoolExecutor pool = new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<>());
try
for (int i = 0; i < 100; i++)
System.out.println(i);
pool.submit(() ->
try
System.out.println(recurse() + " size " + pool.getPoolSize());
catch (Throwable t)
t.printStackTrace();
return null;
);
Thread.sleep(1000);
finally
pool.shutdown();
static long recurse()
try
return 1 + recurse();
catch (Error e)
try
Thread.sleep(10000);
catch (InterruptedException e1)
e1.printStackTrace();
return 1;
add a comment |
up vote
3
down vote
On most OSEes, the stack is allocated lazily, ie only the pages you actually use turn into real memory. Your process is limited to 128 to 256 TB of virtual memory per process, depending on the OS you are using, so at 1 GB per thread, you need at least 128k threads. I would try a much larger stack. E.g. 256g
EDIT: Trying this myself, it looks like it ignores stack sizes of 4g and above. The largest size is -Xss4000m on windows.
Trying to reproduce this on Windows, and it appears to overload the machine before any exception is thrown.
This is what I tried. Run with -Xss4000m
, it got to over 20 threads (total 80g before my windows laptop stopped working)
You might find in Linux, it will reach a ulimit
before overloading the machine.
import java.util.concurrent.*;
class A
public static void main(String args) throws InterruptedException
ThreadPoolExecutor pool = new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<>());
try
for (int i = 0; i < 100; i++)
System.out.println(i);
pool.submit(() ->
try
System.out.println(recurse() + " size " + pool.getPoolSize());
catch (Throwable t)
t.printStackTrace();
return null;
);
Thread.sleep(1000);
finally
pool.shutdown();
static long recurse()
try
return 1 + recurse();
catch (Error e)
try
Thread.sleep(10000);
catch (InterruptedException e1)
e1.printStackTrace();
return 1;
add a comment |
up vote
3
down vote
up vote
3
down vote
On most OSEes, the stack is allocated lazily, ie only the pages you actually use turn into real memory. Your process is limited to 128 to 256 TB of virtual memory per process, depending on the OS you are using, so at 1 GB per thread, you need at least 128k threads. I would try a much larger stack. E.g. 256g
EDIT: Trying this myself, it looks like it ignores stack sizes of 4g and above. The largest size is -Xss4000m on windows.
Trying to reproduce this on Windows, and it appears to overload the machine before any exception is thrown.
This is what I tried. Run with -Xss4000m
, it got to over 20 threads (total 80g before my windows laptop stopped working)
You might find in Linux, it will reach a ulimit
before overloading the machine.
import java.util.concurrent.*;
class A
public static void main(String args) throws InterruptedException
ThreadPoolExecutor pool = new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<>());
try
for (int i = 0; i < 100; i++)
System.out.println(i);
pool.submit(() ->
try
System.out.println(recurse() + " size " + pool.getPoolSize());
catch (Throwable t)
t.printStackTrace();
return null;
);
Thread.sleep(1000);
finally
pool.shutdown();
static long recurse()
try
return 1 + recurse();
catch (Error e)
try
Thread.sleep(10000);
catch (InterruptedException e1)
e1.printStackTrace();
return 1;
On most OSEes, the stack is allocated lazily, ie only the pages you actually use turn into real memory. Your process is limited to 128 to 256 TB of virtual memory per process, depending on the OS you are using, so at 1 GB per thread, you need at least 128k threads. I would try a much larger stack. E.g. 256g
EDIT: Trying this myself, it looks like it ignores stack sizes of 4g and above. The largest size is -Xss4000m on windows.
Trying to reproduce this on Windows, and it appears to overload the machine before any exception is thrown.
This is what I tried. Run with -Xss4000m
, it got to over 20 threads (total 80g before my windows laptop stopped working)
You might find in Linux, it will reach a ulimit
before overloading the machine.
import java.util.concurrent.*;
class A
public static void main(String args) throws InterruptedException
ThreadPoolExecutor pool = new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<>());
try
for (int i = 0; i < 100; i++)
System.out.println(i);
pool.submit(() ->
try
System.out.println(recurse() + " size " + pool.getPoolSize());
catch (Throwable t)
t.printStackTrace();
return null;
);
Thread.sleep(1000);
finally
pool.shutdown();
static long recurse()
try
return 1 + recurse();
catch (Error e)
try
Thread.sleep(10000);
catch (InterruptedException e1)
e1.printStackTrace();
return 1;
edited Nov 11 at 18:19
answered Nov 11 at 17:28
Peter Lawrey
438k55556953
438k55556953
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250441%2freproduce-outofmemoryerror-with-xss%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9Zjfq7DiVGumARRxcU,0kANFKZtY okHraScofvo