Can't find the proper output in this code
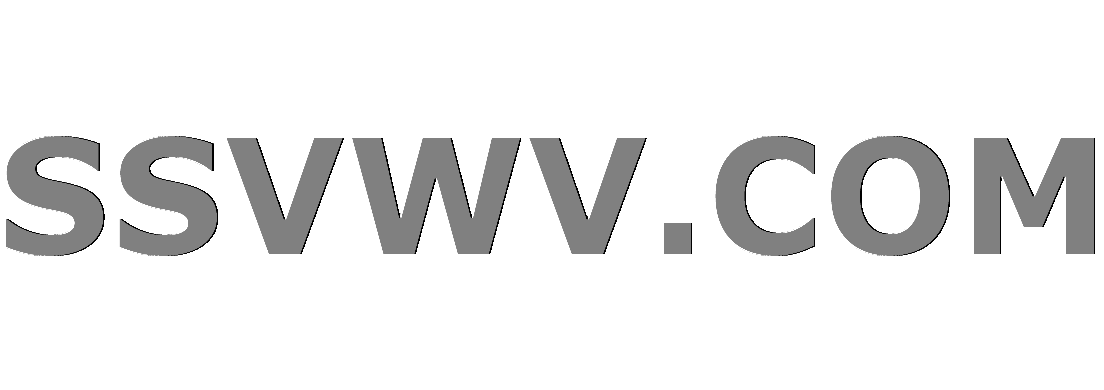
Multi tool use
up vote
-2
down vote
favorite
I was asked to write a program about a traveling man.
The direction of the program:
Suppose a man (say, A) stands at (0, 0) and waits for the user to give him the direction and distance to go.
The user may enter N E W S for north, east, west, south, and any value for the distance.
When the user enters 0 as direction, stop and print out the location where the man stopped
My Code:
#include <stdio.h>
int main()
float x=0,y=0;
char dir;
float mile;
while(1)
printf("Enter input direction as N,S,E,W (0 to exit): ");
scanf("%c",&dir);
if(dir == '0')
break;
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
printf("Invalid Direction, re-enter n");
continue;
printf("Input mile in %c dir: ",dir);
scanf("%f",&mile);
if(dir == 'N')
y+=mile;
else if(dir == 'S')
y-=mile;
else if(dir == 'E')
x+=mile;
else if(dir == 'W')
x-=mile;
printf("nCurrent position of A: (%4.2f,%4.2f)n",x,y);
return 0;
But when I run the program after the first two inputs, it prints invalid outputs in the valid inputs.
output:
c loops if-statement scanf
add a comment |
up vote
-2
down vote
favorite
I was asked to write a program about a traveling man.
The direction of the program:
Suppose a man (say, A) stands at (0, 0) and waits for the user to give him the direction and distance to go.
The user may enter N E W S for north, east, west, south, and any value for the distance.
When the user enters 0 as direction, stop and print out the location where the man stopped
My Code:
#include <stdio.h>
int main()
float x=0,y=0;
char dir;
float mile;
while(1)
printf("Enter input direction as N,S,E,W (0 to exit): ");
scanf("%c",&dir);
if(dir == '0')
break;
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
printf("Invalid Direction, re-enter n");
continue;
printf("Input mile in %c dir: ",dir);
scanf("%f",&mile);
if(dir == 'N')
y+=mile;
else if(dir == 'S')
y-=mile;
else if(dir == 'E')
x+=mile;
else if(dir == 'W')
x-=mile;
printf("nCurrent position of A: (%4.2f,%4.2f)n",x,y);
return 0;
But when I run the program after the first two inputs, it prints invalid outputs in the valid inputs.
output:
c loops if-statement scanf
Show the output (edit the question)
– Michael Butscher
Nov 10 at 13:38
1
Don't post an image of text output. Also, if that is your current output: that is different code than the one in your question.
– usr2564301
Nov 10 at 13:40
@singlepiece .. Its a classic mistake of OR vs AND. It should beif(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
. For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S'..
– pOrinG
Nov 10 at 13:41
I missed the last line. But the code is not working.
– singlepiece000
Nov 10 at 13:43
Add a space before yourscanf
format strings. You are processing the blank Return.
– usr2564301
Nov 10 at 13:51
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I was asked to write a program about a traveling man.
The direction of the program:
Suppose a man (say, A) stands at (0, 0) and waits for the user to give him the direction and distance to go.
The user may enter N E W S for north, east, west, south, and any value for the distance.
When the user enters 0 as direction, stop and print out the location where the man stopped
My Code:
#include <stdio.h>
int main()
float x=0,y=0;
char dir;
float mile;
while(1)
printf("Enter input direction as N,S,E,W (0 to exit): ");
scanf("%c",&dir);
if(dir == '0')
break;
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
printf("Invalid Direction, re-enter n");
continue;
printf("Input mile in %c dir: ",dir);
scanf("%f",&mile);
if(dir == 'N')
y+=mile;
else if(dir == 'S')
y-=mile;
else if(dir == 'E')
x+=mile;
else if(dir == 'W')
x-=mile;
printf("nCurrent position of A: (%4.2f,%4.2f)n",x,y);
return 0;
But when I run the program after the first two inputs, it prints invalid outputs in the valid inputs.
output:
c loops if-statement scanf
I was asked to write a program about a traveling man.
The direction of the program:
Suppose a man (say, A) stands at (0, 0) and waits for the user to give him the direction and distance to go.
The user may enter N E W S for north, east, west, south, and any value for the distance.
When the user enters 0 as direction, stop and print out the location where the man stopped
My Code:
#include <stdio.h>
int main()
float x=0,y=0;
char dir;
float mile;
while(1)
printf("Enter input direction as N,S,E,W (0 to exit): ");
scanf("%c",&dir);
if(dir == '0')
break;
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
printf("Invalid Direction, re-enter n");
continue;
printf("Input mile in %c dir: ",dir);
scanf("%f",&mile);
if(dir == 'N')
y+=mile;
else if(dir == 'S')
y-=mile;
else if(dir == 'E')
x+=mile;
else if(dir == 'W')
x-=mile;
printf("nCurrent position of A: (%4.2f,%4.2f)n",x,y);
return 0;
But when I run the program after the first two inputs, it prints invalid outputs in the valid inputs.
output:
c loops if-statement scanf
c loops if-statement scanf
edited Nov 10 at 13:51
melpomene
55.6k54387
55.6k54387
asked Nov 10 at 13:35
singlepiece000
125
125
Show the output (edit the question)
– Michael Butscher
Nov 10 at 13:38
1
Don't post an image of text output. Also, if that is your current output: that is different code than the one in your question.
– usr2564301
Nov 10 at 13:40
@singlepiece .. Its a classic mistake of OR vs AND. It should beif(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
. For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S'..
– pOrinG
Nov 10 at 13:41
I missed the last line. But the code is not working.
– singlepiece000
Nov 10 at 13:43
Add a space before yourscanf
format strings. You are processing the blank Return.
– usr2564301
Nov 10 at 13:51
add a comment |
Show the output (edit the question)
– Michael Butscher
Nov 10 at 13:38
1
Don't post an image of text output. Also, if that is your current output: that is different code than the one in your question.
– usr2564301
Nov 10 at 13:40
@singlepiece .. Its a classic mistake of OR vs AND. It should beif(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
. For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S'..
– pOrinG
Nov 10 at 13:41
I missed the last line. But the code is not working.
– singlepiece000
Nov 10 at 13:43
Add a space before yourscanf
format strings. You are processing the blank Return.
– usr2564301
Nov 10 at 13:51
Show the output (edit the question)
– Michael Butscher
Nov 10 at 13:38
Show the output (edit the question)
– Michael Butscher
Nov 10 at 13:38
1
1
Don't post an image of text output. Also, if that is your current output: that is different code than the one in your question.
– usr2564301
Nov 10 at 13:40
Don't post an image of text output. Also, if that is your current output: that is different code than the one in your question.
– usr2564301
Nov 10 at 13:40
@singlepiece .. Its a classic mistake of OR vs AND. It should be
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
. For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S'..– pOrinG
Nov 10 at 13:41
@singlepiece .. Its a classic mistake of OR vs AND. It should be
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
. For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S'..– pOrinG
Nov 10 at 13:41
I missed the last line. But the code is not working.
– singlepiece000
Nov 10 at 13:43
I missed the last line. But the code is not working.
– singlepiece000
Nov 10 at 13:43
Add a space before your
scanf
format strings. You are processing the blank Return.– usr2564301
Nov 10 at 13:51
Add a space before your
scanf
format strings. You are processing the blank Return.– usr2564301
Nov 10 at 13:51
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
So, I found your problem: When you were pressing "Enter" after you entered the "miles", that "Enter" got registered as input, as a 'n' character. I have a solution here for you, but if you don't prefer it, just make a special case in which you ignore the 'n' character. Something like else if (dir == 'n') continue;
. I suspected this might be the problem but I confirmed it once I printed that "invalid character".
int main()
float x = 0, y = 0;
char dir;
float mile;
do
printf("Enter input direction as N,S,E,W (0 to exit): ");
scanf("%c", &dir);
switch (dir)
case 'N':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y += mile;
break;
case 'S':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y -= mile;
break;
case 'E':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x += mile;
break;
case 'W':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x -= mile;
break;
case 'n':
break;
case '0':
break;
default:
printf("Invalid Direction, re-enter; You entered: %c n", dir);
break;
while (dir != '0');
printf("nCurrent position of A: (%4.2f, %4.2f)n", x, y);
return 0;
New contributor
Rakirnd is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
Its a classic mistake of OR vs AND.
It should be
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W') .
For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S' and the if condition has a OR.
Edit: (Following @usr2564301 's advice.)
Add a space before scanf input %c
.
scanf(" %c",&dir);
Check link for more explanation.
I code is just like you said.
– singlepiece000
Nov 10 at 13:45
Hmm.. You just edited your post to remove || and added && ... Now I look like a fool.. lol
– pOrinG
Nov 10 at 13:46
The revisions show it has been that all the time. But you could remove that from your answer so only my advice remains.
– usr2564301
Nov 10 at 14:06
1
@usr2564301 God yeah!! Anyway when he put the question, I had copied his source code into my editor to check. There was an OR in it.. I even double checked before commenting on the post and then later answering it ..
– pOrinG
Nov 10 at 14:09
@singlepiece000 Kindly do let me know if I am delusional. You started with a code which had || (OR) operator in if condition instead of && (AND) right ?
– pOrinG
Nov 10 at 14:11
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
So, I found your problem: When you were pressing "Enter" after you entered the "miles", that "Enter" got registered as input, as a 'n' character. I have a solution here for you, but if you don't prefer it, just make a special case in which you ignore the 'n' character. Something like else if (dir == 'n') continue;
. I suspected this might be the problem but I confirmed it once I printed that "invalid character".
int main()
float x = 0, y = 0;
char dir;
float mile;
do
printf("Enter input direction as N,S,E,W (0 to exit): ");
scanf("%c", &dir);
switch (dir)
case 'N':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y += mile;
break;
case 'S':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y -= mile;
break;
case 'E':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x += mile;
break;
case 'W':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x -= mile;
break;
case 'n':
break;
case '0':
break;
default:
printf("Invalid Direction, re-enter; You entered: %c n", dir);
break;
while (dir != '0');
printf("nCurrent position of A: (%4.2f, %4.2f)n", x, y);
return 0;
New contributor
Rakirnd is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
accepted
So, I found your problem: When you were pressing "Enter" after you entered the "miles", that "Enter" got registered as input, as a 'n' character. I have a solution here for you, but if you don't prefer it, just make a special case in which you ignore the 'n' character. Something like else if (dir == 'n') continue;
. I suspected this might be the problem but I confirmed it once I printed that "invalid character".
int main()
float x = 0, y = 0;
char dir;
float mile;
do
printf("Enter input direction as N,S,E,W (0 to exit): ");
scanf("%c", &dir);
switch (dir)
case 'N':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y += mile;
break;
case 'S':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y -= mile;
break;
case 'E':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x += mile;
break;
case 'W':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x -= mile;
break;
case 'n':
break;
case '0':
break;
default:
printf("Invalid Direction, re-enter; You entered: %c n", dir);
break;
while (dir != '0');
printf("nCurrent position of A: (%4.2f, %4.2f)n", x, y);
return 0;
New contributor
Rakirnd is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
So, I found your problem: When you were pressing "Enter" after you entered the "miles", that "Enter" got registered as input, as a 'n' character. I have a solution here for you, but if you don't prefer it, just make a special case in which you ignore the 'n' character. Something like else if (dir == 'n') continue;
. I suspected this might be the problem but I confirmed it once I printed that "invalid character".
int main()
float x = 0, y = 0;
char dir;
float mile;
do
printf("Enter input direction as N,S,E,W (0 to exit): ");
scanf("%c", &dir);
switch (dir)
case 'N':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y += mile;
break;
case 'S':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y -= mile;
break;
case 'E':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x += mile;
break;
case 'W':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x -= mile;
break;
case 'n':
break;
case '0':
break;
default:
printf("Invalid Direction, re-enter; You entered: %c n", dir);
break;
while (dir != '0');
printf("nCurrent position of A: (%4.2f, %4.2f)n", x, y);
return 0;
New contributor
Rakirnd is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
So, I found your problem: When you were pressing "Enter" after you entered the "miles", that "Enter" got registered as input, as a 'n' character. I have a solution here for you, but if you don't prefer it, just make a special case in which you ignore the 'n' character. Something like else if (dir == 'n') continue;
. I suspected this might be the problem but I confirmed it once I printed that "invalid character".
int main()
float x = 0, y = 0;
char dir;
float mile;
do
printf("Enter input direction as N,S,E,W (0 to exit): ");
scanf("%c", &dir);
switch (dir)
case 'N':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y += mile;
break;
case 'S':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
y -= mile;
break;
case 'E':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x += mile;
break;
case 'W':
printf("Input mile in %c dir: ", dir);
scanf("%f", &mile);
x -= mile;
break;
case 'n':
break;
case '0':
break;
default:
printf("Invalid Direction, re-enter; You entered: %c n", dir);
break;
while (dir != '0');
printf("nCurrent position of A: (%4.2f, %4.2f)n", x, y);
return 0;
New contributor
Rakirnd is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Rakirnd is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered Nov 10 at 14:09


Rakirnd
713
713
New contributor
Rakirnd is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Rakirnd is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Rakirnd is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
up vote
0
down vote
Its a classic mistake of OR vs AND.
It should be
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W') .
For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S' and the if condition has a OR.
Edit: (Following @usr2564301 's advice.)
Add a space before scanf input %c
.
scanf(" %c",&dir);
Check link for more explanation.
I code is just like you said.
– singlepiece000
Nov 10 at 13:45
Hmm.. You just edited your post to remove || and added && ... Now I look like a fool.. lol
– pOrinG
Nov 10 at 13:46
The revisions show it has been that all the time. But you could remove that from your answer so only my advice remains.
– usr2564301
Nov 10 at 14:06
1
@usr2564301 God yeah!! Anyway when he put the question, I had copied his source code into my editor to check. There was an OR in it.. I even double checked before commenting on the post and then later answering it ..
– pOrinG
Nov 10 at 14:09
@singlepiece000 Kindly do let me know if I am delusional. You started with a code which had || (OR) operator in if condition instead of && (AND) right ?
– pOrinG
Nov 10 at 14:11
add a comment |
up vote
0
down vote
Its a classic mistake of OR vs AND.
It should be
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W') .
For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S' and the if condition has a OR.
Edit: (Following @usr2564301 's advice.)
Add a space before scanf input %c
.
scanf(" %c",&dir);
Check link for more explanation.
I code is just like you said.
– singlepiece000
Nov 10 at 13:45
Hmm.. You just edited your post to remove || and added && ... Now I look like a fool.. lol
– pOrinG
Nov 10 at 13:46
The revisions show it has been that all the time. But you could remove that from your answer so only my advice remains.
– usr2564301
Nov 10 at 14:06
1
@usr2564301 God yeah!! Anyway when he put the question, I had copied his source code into my editor to check. There was an OR in it.. I even double checked before commenting on the post and then later answering it ..
– pOrinG
Nov 10 at 14:09
@singlepiece000 Kindly do let me know if I am delusional. You started with a code which had || (OR) operator in if condition instead of && (AND) right ?
– pOrinG
Nov 10 at 14:11
add a comment |
up vote
0
down vote
up vote
0
down vote
Its a classic mistake of OR vs AND.
It should be
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W') .
For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S' and the if condition has a OR.
Edit: (Following @usr2564301 's advice.)
Add a space before scanf input %c
.
scanf(" %c",&dir);
Check link for more explanation.
Its a classic mistake of OR vs AND.
It should be
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W') .
For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S' and the if condition has a OR.
Edit: (Following @usr2564301 's advice.)
Add a space before scanf input %c
.
scanf(" %c",&dir);
Check link for more explanation.
edited Nov 10 at 14:19
answered Nov 10 at 13:43
pOrinG
505316
505316
I code is just like you said.
– singlepiece000
Nov 10 at 13:45
Hmm.. You just edited your post to remove || and added && ... Now I look like a fool.. lol
– pOrinG
Nov 10 at 13:46
The revisions show it has been that all the time. But you could remove that from your answer so only my advice remains.
– usr2564301
Nov 10 at 14:06
1
@usr2564301 God yeah!! Anyway when he put the question, I had copied his source code into my editor to check. There was an OR in it.. I even double checked before commenting on the post and then later answering it ..
– pOrinG
Nov 10 at 14:09
@singlepiece000 Kindly do let me know if I am delusional. You started with a code which had || (OR) operator in if condition instead of && (AND) right ?
– pOrinG
Nov 10 at 14:11
add a comment |
I code is just like you said.
– singlepiece000
Nov 10 at 13:45
Hmm.. You just edited your post to remove || and added && ... Now I look like a fool.. lol
– pOrinG
Nov 10 at 13:46
The revisions show it has been that all the time. But you could remove that from your answer so only my advice remains.
– usr2564301
Nov 10 at 14:06
1
@usr2564301 God yeah!! Anyway when he put the question, I had copied his source code into my editor to check. There was an OR in it.. I even double checked before commenting on the post and then later answering it ..
– pOrinG
Nov 10 at 14:09
@singlepiece000 Kindly do let me know if I am delusional. You started with a code which had || (OR) operator in if condition instead of && (AND) right ?
– pOrinG
Nov 10 at 14:11
I code is just like you said.
– singlepiece000
Nov 10 at 13:45
I code is just like you said.
– singlepiece000
Nov 10 at 13:45
Hmm.. You just edited your post to remove || and added && ... Now I look like a fool.. lol
– pOrinG
Nov 10 at 13:46
Hmm.. You just edited your post to remove || and added && ... Now I look like a fool.. lol
– pOrinG
Nov 10 at 13:46
The revisions show it has been that all the time. But you could remove that from your answer so only my advice remains.
– usr2564301
Nov 10 at 14:06
The revisions show it has been that all the time. But you could remove that from your answer so only my advice remains.
– usr2564301
Nov 10 at 14:06
1
1
@usr2564301 God yeah!! Anyway when he put the question, I had copied his source code into my editor to check. There was an OR in it.. I even double checked before commenting on the post and then later answering it ..
– pOrinG
Nov 10 at 14:09
@usr2564301 God yeah!! Anyway when he put the question, I had copied his source code into my editor to check. There was an OR in it.. I even double checked before commenting on the post and then later answering it ..
– pOrinG
Nov 10 at 14:09
@singlepiece000 Kindly do let me know if I am delusional. You started with a code which had || (OR) operator in if condition instead of && (AND) right ?
– pOrinG
Nov 10 at 14:11
@singlepiece000 Kindly do let me know if I am delusional. You started with a code which had || (OR) operator in if condition instead of && (AND) right ?
– pOrinG
Nov 10 at 14:11
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53239507%2fcant-find-the-proper-output-in-this-code%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
3pXxW,6v7g4U6cnYgE1D4gz AtgQez2OLXhkZrAGFAN9 luIG25Y,4rV
Show the output (edit the question)
– Michael Butscher
Nov 10 at 13:38
1
Don't post an image of text output. Also, if that is your current output: that is different code than the one in your question.
– usr2564301
Nov 10 at 13:40
@singlepiece .. Its a classic mistake of OR vs AND. It should be
if(dir != 'N' && dir != 'S' && dir != 'E' && dir != 'W')
. For eg if a user inputs 'N', in that case the if statement will still return success because its != 'S'..– pOrinG
Nov 10 at 13:41
I missed the last line. But the code is not working.
– singlepiece000
Nov 10 at 13:43
Add a space before your
scanf
format strings. You are processing the blank Return.– usr2564301
Nov 10 at 13:51