How do I turn this array of objects into a string using ES6 methods?
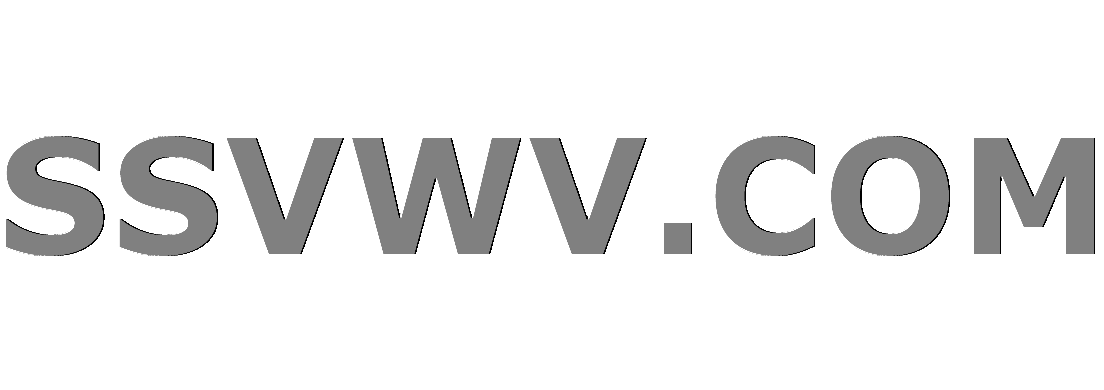
Multi tool use
up vote
0
down vote
favorite
I have the following array of objects:
const pagesByBook = [
bookName: "An old tome", pages: 123,
bookName: "Really ancient stuff", pages: 432,
bookName: "Yup, another old book", pages: 218
]
And I would like to get the following string output:
const output= "['An old tome', 123, 'old', null],
['Really ancient stuff', 432, 'old', null],
['Yup, another old book', 218, 'old', null]"
How can I do this using in a few lines using ES6 methods such as map?
javascript arrays ecmascript-6
|
show 1 more comment
up vote
0
down vote
favorite
I have the following array of objects:
const pagesByBook = [
bookName: "An old tome", pages: 123,
bookName: "Really ancient stuff", pages: 432,
bookName: "Yup, another old book", pages: 218
]
And I would like to get the following string output:
const output= "['An old tome', 123, 'old', null],
['Really ancient stuff', 432, 'old', null],
['Yup, another old book', 218, 'old', null]"
How can I do this using in a few lines using ES6 methods such as map?
javascript arrays ecmascript-6
Maybe. Have you tried anything? DId you get stuck somewhere?
– George Jempty
Nov 11 at 18:37
You already mentioned the best method...did you try it?
– charlietfl
Nov 11 at 18:37
Why that weird string format? Can't you just use JSON?
– Bergi
Nov 11 at 18:39
Where does that'old'
come from, is that a constant?
– Bergi
Nov 11 at 18:40
@Bergi: I guess 'old' could be a constant. Regarding the weird string format, I don't know, because I need to output like a string. I don't know how I could do that with JSON. If it works the same then I'm ok with that possibility.
– nicokruk
Nov 11 at 18:46
|
show 1 more comment
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have the following array of objects:
const pagesByBook = [
bookName: "An old tome", pages: 123,
bookName: "Really ancient stuff", pages: 432,
bookName: "Yup, another old book", pages: 218
]
And I would like to get the following string output:
const output= "['An old tome', 123, 'old', null],
['Really ancient stuff', 432, 'old', null],
['Yup, another old book', 218, 'old', null]"
How can I do this using in a few lines using ES6 methods such as map?
javascript arrays ecmascript-6
I have the following array of objects:
const pagesByBook = [
bookName: "An old tome", pages: 123,
bookName: "Really ancient stuff", pages: 432,
bookName: "Yup, another old book", pages: 218
]
And I would like to get the following string output:
const output= "['An old tome', 123, 'old', null],
['Really ancient stuff', 432, 'old', null],
['Yup, another old book', 218, 'old', null]"
How can I do this using in a few lines using ES6 methods such as map?
javascript arrays ecmascript-6
javascript arrays ecmascript-6
asked Nov 11 at 18:33
nicokruk
244
244
Maybe. Have you tried anything? DId you get stuck somewhere?
– George Jempty
Nov 11 at 18:37
You already mentioned the best method...did you try it?
– charlietfl
Nov 11 at 18:37
Why that weird string format? Can't you just use JSON?
– Bergi
Nov 11 at 18:39
Where does that'old'
come from, is that a constant?
– Bergi
Nov 11 at 18:40
@Bergi: I guess 'old' could be a constant. Regarding the weird string format, I don't know, because I need to output like a string. I don't know how I could do that with JSON. If it works the same then I'm ok with that possibility.
– nicokruk
Nov 11 at 18:46
|
show 1 more comment
Maybe. Have you tried anything? DId you get stuck somewhere?
– George Jempty
Nov 11 at 18:37
You already mentioned the best method...did you try it?
– charlietfl
Nov 11 at 18:37
Why that weird string format? Can't you just use JSON?
– Bergi
Nov 11 at 18:39
Where does that'old'
come from, is that a constant?
– Bergi
Nov 11 at 18:40
@Bergi: I guess 'old' could be a constant. Regarding the weird string format, I don't know, because I need to output like a string. I don't know how I could do that with JSON. If it works the same then I'm ok with that possibility.
– nicokruk
Nov 11 at 18:46
Maybe. Have you tried anything? DId you get stuck somewhere?
– George Jempty
Nov 11 at 18:37
Maybe. Have you tried anything? DId you get stuck somewhere?
– George Jempty
Nov 11 at 18:37
You already mentioned the best method...did you try it?
– charlietfl
Nov 11 at 18:37
You already mentioned the best method...did you try it?
– charlietfl
Nov 11 at 18:37
Why that weird string format? Can't you just use JSON?
– Bergi
Nov 11 at 18:39
Why that weird string format? Can't you just use JSON?
– Bergi
Nov 11 at 18:39
Where does that
'old'
come from, is that a constant?– Bergi
Nov 11 at 18:40
Where does that
'old'
come from, is that a constant?– Bergi
Nov 11 at 18:40
@Bergi: I guess 'old' could be a constant. Regarding the weird string format, I don't know, because I need to output like a string. I don't know how I could do that with JSON. If it works the same then I'm ok with that possibility.
– nicokruk
Nov 11 at 18:46
@Bergi: I guess 'old' could be a constant. Regarding the weird string format, I don't know, because I need to output like a string. I don't know how I could do that with JSON. If it works the same then I'm ok with that possibility.
– nicokruk
Nov 11 at 18:46
|
show 1 more comment
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
Use JSON.stringify, Array#map, Object.values, Array#slice and Array#concat to get your required result.
const pagesByBook = [
bookName: "An old tome",
pages: 123
,
bookName: "Really ancient stuff",
pages: 432
,
bookName: "Yup, another old book",
pages: 218
]
const res = JSON.stringify(pagesByBook.map(item => Object.values(item).concat("old", null))).slice(1, -1);
console.log(res)
add a comment |
up vote
1
down vote
This seems to me to be a simple map
with ES6 destructuring. Assuming that the last 2 elements in the arrays are constants (old
, null
):
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => [bookName, pages, 'old', null])
console.log(JSON.stringify(result))
If a string representation of this is needed you could change it to:
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => JSON.stringify([bookName, pages, 'old', null]))
console.log(result.join(','))
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Use JSON.stringify, Array#map, Object.values, Array#slice and Array#concat to get your required result.
const pagesByBook = [
bookName: "An old tome",
pages: 123
,
bookName: "Really ancient stuff",
pages: 432
,
bookName: "Yup, another old book",
pages: 218
]
const res = JSON.stringify(pagesByBook.map(item => Object.values(item).concat("old", null))).slice(1, -1);
console.log(res)
add a comment |
up vote
0
down vote
accepted
Use JSON.stringify, Array#map, Object.values, Array#slice and Array#concat to get your required result.
const pagesByBook = [
bookName: "An old tome",
pages: 123
,
bookName: "Really ancient stuff",
pages: 432
,
bookName: "Yup, another old book",
pages: 218
]
const res = JSON.stringify(pagesByBook.map(item => Object.values(item).concat("old", null))).slice(1, -1);
console.log(res)
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Use JSON.stringify, Array#map, Object.values, Array#slice and Array#concat to get your required result.
const pagesByBook = [
bookName: "An old tome",
pages: 123
,
bookName: "Really ancient stuff",
pages: 432
,
bookName: "Yup, another old book",
pages: 218
]
const res = JSON.stringify(pagesByBook.map(item => Object.values(item).concat("old", null))).slice(1, -1);
console.log(res)
Use JSON.stringify, Array#map, Object.values, Array#slice and Array#concat to get your required result.
const pagesByBook = [
bookName: "An old tome",
pages: 123
,
bookName: "Really ancient stuff",
pages: 432
,
bookName: "Yup, another old book",
pages: 218
]
const res = JSON.stringify(pagesByBook.map(item => Object.values(item).concat("old", null))).slice(1, -1);
console.log(res)
const pagesByBook = [
bookName: "An old tome",
pages: 123
,
bookName: "Really ancient stuff",
pages: 432
,
bookName: "Yup, another old book",
pages: 218
]
const res = JSON.stringify(pagesByBook.map(item => Object.values(item).concat("old", null))).slice(1, -1);
console.log(res)
const pagesByBook = [
bookName: "An old tome",
pages: 123
,
bookName: "Really ancient stuff",
pages: 432
,
bookName: "Yup, another old book",
pages: 218
]
const res = JSON.stringify(pagesByBook.map(item => Object.values(item).concat("old", null))).slice(1, -1);
console.log(res)
answered Nov 11 at 18:45


kemicofa
8,04543478
8,04543478
add a comment |
add a comment |
up vote
1
down vote
This seems to me to be a simple map
with ES6 destructuring. Assuming that the last 2 elements in the arrays are constants (old
, null
):
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => [bookName, pages, 'old', null])
console.log(JSON.stringify(result))
If a string representation of this is needed you could change it to:
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => JSON.stringify([bookName, pages, 'old', null]))
console.log(result.join(','))
add a comment |
up vote
1
down vote
This seems to me to be a simple map
with ES6 destructuring. Assuming that the last 2 elements in the arrays are constants (old
, null
):
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => [bookName, pages, 'old', null])
console.log(JSON.stringify(result))
If a string representation of this is needed you could change it to:
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => JSON.stringify([bookName, pages, 'old', null]))
console.log(result.join(','))
add a comment |
up vote
1
down vote
up vote
1
down vote
This seems to me to be a simple map
with ES6 destructuring. Assuming that the last 2 elements in the arrays are constants (old
, null
):
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => [bookName, pages, 'old', null])
console.log(JSON.stringify(result))
If a string representation of this is needed you could change it to:
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => JSON.stringify([bookName, pages, 'old', null]))
console.log(result.join(','))
This seems to me to be a simple map
with ES6 destructuring. Assuming that the last 2 elements in the arrays are constants (old
, null
):
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => [bookName, pages, 'old', null])
console.log(JSON.stringify(result))
If a string representation of this is needed you could change it to:
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => JSON.stringify([bookName, pages, 'old', null]))
console.log(result.join(','))
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => [bookName, pages, 'old', null])
console.log(JSON.stringify(result))
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => [bookName, pages, 'old', null])
console.log(JSON.stringify(result))
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => JSON.stringify([bookName, pages, 'old', null]))
console.log(result.join(','))
const data = [ bookName: "An old tome", pages: 123, bookName: "Really ancient stuff", pages: 432, bookName: "Yup, another old book", pages: 218 ]
const result = data.map((bookName, pages) => JSON.stringify([bookName, pages, 'old', null]))
console.log(result.join(','))
edited Nov 11 at 22:38
answered Nov 11 at 22:31


Akrion
9,03411223
9,03411223
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53251878%2fhow-do-i-turn-this-array-of-objects-into-a-string-using-es6-methods%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fh8rSrZEs4uqztZu d,KvaNal0KHMIqPS7HJ
Maybe. Have you tried anything? DId you get stuck somewhere?
– George Jempty
Nov 11 at 18:37
You already mentioned the best method...did you try it?
– charlietfl
Nov 11 at 18:37
Why that weird string format? Can't you just use JSON?
– Bergi
Nov 11 at 18:39
Where does that
'old'
come from, is that a constant?– Bergi
Nov 11 at 18:40
@Bergi: I guess 'old' could be a constant. Regarding the weird string format, I don't know, because I need to output like a string. I don't know how I could do that with JSON. If it works the same then I'm ok with that possibility.
– nicokruk
Nov 11 at 18:46