Assembly MIPS: Converting a nested for loop to MIPS
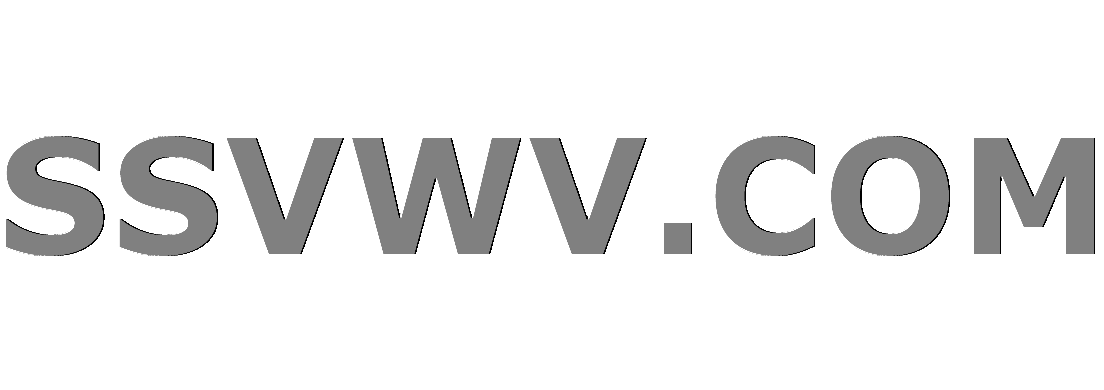
Multi tool use
my_data = [4, 8, 15, 16, 23, 42]
index = 0
for item in my_data:
k = 0
for i in range(2, item+1):
if (i % 2 == 0):
k += 1
my_data[index] = k
index += 1
print my_data
The above written code, takes a list of numbers and divides them by 2, and I am trying to convert it into MIPS, however I haven't got the grasp of it completely. If anyone could clarify it for me I would appreciate it.
.data
my_data: .word 4, 8, 15, 16, 23, 42
.text
la $s0, my_data # data of the list
addi $s1, $zero, 6
# $s1 is set to the size of the list
add $t0, $zero, $zero
#index=0
add $t4, $zero, $zero
#for the items
loop1:
beq $t4, $s1,Exit
add $t5, $t0, $t0
add $t5, $t5, $t5
add $t5, $s0, $t5
addi $t6, $zero, 2
#k=0
add $t1, $zero, $zero
lw $t6, 0($t5)
Exit:
j loop1
loop2:
addi $t2, $zero, 2
addi $t3, $t6, 1
sub $t3, $t3, $t2
j loop2
beq $t2, $t3, Exit
#if
rem $t7, $t2, $t6
beq $t2, $zero, TRUE
TRUE: addi $t1, $t1, 1
#if
addi $t2, $t2, 1
j loop2
syscall
#inner loop
sw $t1, 0($t5)
addi $t0, $t0, 1
addi $t4, $t4, 1
j loop1
I am Currently on this situation there is an issue with running a code now. The program has been accepted, but it does nothing.
python assembly pycharm mips
|
show 1 more comment
my_data = [4, 8, 15, 16, 23, 42]
index = 0
for item in my_data:
k = 0
for i in range(2, item+1):
if (i % 2 == 0):
k += 1
my_data[index] = k
index += 1
print my_data
The above written code, takes a list of numbers and divides them by 2, and I am trying to convert it into MIPS, however I haven't got the grasp of it completely. If anyone could clarify it for me I would appreciate it.
.data
my_data: .word 4, 8, 15, 16, 23, 42
.text
la $s0, my_data # data of the list
addi $s1, $zero, 6
# $s1 is set to the size of the list
add $t0, $zero, $zero
#index=0
add $t4, $zero, $zero
#for the items
loop1:
beq $t4, $s1,Exit
add $t5, $t0, $t0
add $t5, $t5, $t5
add $t5, $s0, $t5
addi $t6, $zero, 2
#k=0
add $t1, $zero, $zero
lw $t6, 0($t5)
Exit:
j loop1
loop2:
addi $t2, $zero, 2
addi $t3, $t6, 1
sub $t3, $t3, $t2
j loop2
beq $t2, $t3, Exit
#if
rem $t7, $t2, $t6
beq $t2, $zero, TRUE
TRUE: addi $t1, $t1, 1
#if
addi $t2, $t2, 1
j loop2
syscall
#inner loop
sw $t1, 0($t5)
addi $t0, $t0, 1
addi $t4, $t4, 1
j loop1
I am Currently on this situation there is an issue with running a code now. The program has been accepted, but it does nothing.
python assembly pycharm mips
Please don't add unrelated tags to your question.
– Felix Kling
Nov 13 '18 at 17:21
appreciate the thought, i am new in this website, learning takes time.
– WOlf Veizcum
Nov 13 '18 at 17:59
1
you have basic syntax errors, likeli $t1,$zero,$s0
, where even the assembler is trying to help you with desired syntax by "Expected: li $t1,-100" ... not sure what you need more, syntax has to be learnt and obeyed, especially with SPIM/MARS kind of simulator, which have not very precise assemblers, so they often will compile also invalid syntax into something different than you originally wrote, so learning basic syntax is vital there, or checking against some cheat sheet. BTW your "nested loop" is basicallymy_data[index++] = (item+1)/2;
single loop.
– Ped7g
Nov 13 '18 at 19:01
Thank you, i was working now on it and i updated the code, could you suggest anything at this point? There is an issue with running now. @Ped7g
– WOlf Veizcum
Nov 13 '18 at 19:38
there is infinite loop in firstloop1
, both byt4
staying same and by every code path ending onj loop1
either way, even ift4
would count. BTW, you don't need separateindex
, keep just pointer tomy_data
and advance that (as both read ofitem
and write of result access the same index, so one pointer sequentially advancing +4 every outer loop is enough and simplest way of writing it). Do you MARS or SPIM? They have built-in debugger.. learn to use it, it will make "assembly" much easier
– Ped7g
Nov 14 '18 at 0:06
|
show 1 more comment
my_data = [4, 8, 15, 16, 23, 42]
index = 0
for item in my_data:
k = 0
for i in range(2, item+1):
if (i % 2 == 0):
k += 1
my_data[index] = k
index += 1
print my_data
The above written code, takes a list of numbers and divides them by 2, and I am trying to convert it into MIPS, however I haven't got the grasp of it completely. If anyone could clarify it for me I would appreciate it.
.data
my_data: .word 4, 8, 15, 16, 23, 42
.text
la $s0, my_data # data of the list
addi $s1, $zero, 6
# $s1 is set to the size of the list
add $t0, $zero, $zero
#index=0
add $t4, $zero, $zero
#for the items
loop1:
beq $t4, $s1,Exit
add $t5, $t0, $t0
add $t5, $t5, $t5
add $t5, $s0, $t5
addi $t6, $zero, 2
#k=0
add $t1, $zero, $zero
lw $t6, 0($t5)
Exit:
j loop1
loop2:
addi $t2, $zero, 2
addi $t3, $t6, 1
sub $t3, $t3, $t2
j loop2
beq $t2, $t3, Exit
#if
rem $t7, $t2, $t6
beq $t2, $zero, TRUE
TRUE: addi $t1, $t1, 1
#if
addi $t2, $t2, 1
j loop2
syscall
#inner loop
sw $t1, 0($t5)
addi $t0, $t0, 1
addi $t4, $t4, 1
j loop1
I am Currently on this situation there is an issue with running a code now. The program has been accepted, but it does nothing.
python assembly pycharm mips
my_data = [4, 8, 15, 16, 23, 42]
index = 0
for item in my_data:
k = 0
for i in range(2, item+1):
if (i % 2 == 0):
k += 1
my_data[index] = k
index += 1
print my_data
The above written code, takes a list of numbers and divides them by 2, and I am trying to convert it into MIPS, however I haven't got the grasp of it completely. If anyone could clarify it for me I would appreciate it.
.data
my_data: .word 4, 8, 15, 16, 23, 42
.text
la $s0, my_data # data of the list
addi $s1, $zero, 6
# $s1 is set to the size of the list
add $t0, $zero, $zero
#index=0
add $t4, $zero, $zero
#for the items
loop1:
beq $t4, $s1,Exit
add $t5, $t0, $t0
add $t5, $t5, $t5
add $t5, $s0, $t5
addi $t6, $zero, 2
#k=0
add $t1, $zero, $zero
lw $t6, 0($t5)
Exit:
j loop1
loop2:
addi $t2, $zero, 2
addi $t3, $t6, 1
sub $t3, $t3, $t2
j loop2
beq $t2, $t3, Exit
#if
rem $t7, $t2, $t6
beq $t2, $zero, TRUE
TRUE: addi $t1, $t1, 1
#if
addi $t2, $t2, 1
j loop2
syscall
#inner loop
sw $t1, 0($t5)
addi $t0, $t0, 1
addi $t4, $t4, 1
j loop1
I am Currently on this situation there is an issue with running a code now. The program has been accepted, but it does nothing.
python assembly pycharm mips
python assembly pycharm mips
edited Nov 13 '18 at 19:32
WOlf Veizcum
asked Nov 13 '18 at 13:38


WOlf VeizcumWOlf Veizcum
11
11
Please don't add unrelated tags to your question.
– Felix Kling
Nov 13 '18 at 17:21
appreciate the thought, i am new in this website, learning takes time.
– WOlf Veizcum
Nov 13 '18 at 17:59
1
you have basic syntax errors, likeli $t1,$zero,$s0
, where even the assembler is trying to help you with desired syntax by "Expected: li $t1,-100" ... not sure what you need more, syntax has to be learnt and obeyed, especially with SPIM/MARS kind of simulator, which have not very precise assemblers, so they often will compile also invalid syntax into something different than you originally wrote, so learning basic syntax is vital there, or checking against some cheat sheet. BTW your "nested loop" is basicallymy_data[index++] = (item+1)/2;
single loop.
– Ped7g
Nov 13 '18 at 19:01
Thank you, i was working now on it and i updated the code, could you suggest anything at this point? There is an issue with running now. @Ped7g
– WOlf Veizcum
Nov 13 '18 at 19:38
there is infinite loop in firstloop1
, both byt4
staying same and by every code path ending onj loop1
either way, even ift4
would count. BTW, you don't need separateindex
, keep just pointer tomy_data
and advance that (as both read ofitem
and write of result access the same index, so one pointer sequentially advancing +4 every outer loop is enough and simplest way of writing it). Do you MARS or SPIM? They have built-in debugger.. learn to use it, it will make "assembly" much easier
– Ped7g
Nov 14 '18 at 0:06
|
show 1 more comment
Please don't add unrelated tags to your question.
– Felix Kling
Nov 13 '18 at 17:21
appreciate the thought, i am new in this website, learning takes time.
– WOlf Veizcum
Nov 13 '18 at 17:59
1
you have basic syntax errors, likeli $t1,$zero,$s0
, where even the assembler is trying to help you with desired syntax by "Expected: li $t1,-100" ... not sure what you need more, syntax has to be learnt and obeyed, especially with SPIM/MARS kind of simulator, which have not very precise assemblers, so they often will compile also invalid syntax into something different than you originally wrote, so learning basic syntax is vital there, or checking against some cheat sheet. BTW your "nested loop" is basicallymy_data[index++] = (item+1)/2;
single loop.
– Ped7g
Nov 13 '18 at 19:01
Thank you, i was working now on it and i updated the code, could you suggest anything at this point? There is an issue with running now. @Ped7g
– WOlf Veizcum
Nov 13 '18 at 19:38
there is infinite loop in firstloop1
, both byt4
staying same and by every code path ending onj loop1
either way, even ift4
would count. BTW, you don't need separateindex
, keep just pointer tomy_data
and advance that (as both read ofitem
and write of result access the same index, so one pointer sequentially advancing +4 every outer loop is enough and simplest way of writing it). Do you MARS or SPIM? They have built-in debugger.. learn to use it, it will make "assembly" much easier
– Ped7g
Nov 14 '18 at 0:06
Please don't add unrelated tags to your question.
– Felix Kling
Nov 13 '18 at 17:21
Please don't add unrelated tags to your question.
– Felix Kling
Nov 13 '18 at 17:21
appreciate the thought, i am new in this website, learning takes time.
– WOlf Veizcum
Nov 13 '18 at 17:59
appreciate the thought, i am new in this website, learning takes time.
– WOlf Veizcum
Nov 13 '18 at 17:59
1
1
you have basic syntax errors, like
li $t1,$zero,$s0
, where even the assembler is trying to help you with desired syntax by "Expected: li $t1,-100" ... not sure what you need more, syntax has to be learnt and obeyed, especially with SPIM/MARS kind of simulator, which have not very precise assemblers, so they often will compile also invalid syntax into something different than you originally wrote, so learning basic syntax is vital there, or checking against some cheat sheet. BTW your "nested loop" is basically my_data[index++] = (item+1)/2;
single loop.– Ped7g
Nov 13 '18 at 19:01
you have basic syntax errors, like
li $t1,$zero,$s0
, where even the assembler is trying to help you with desired syntax by "Expected: li $t1,-100" ... not sure what you need more, syntax has to be learnt and obeyed, especially with SPIM/MARS kind of simulator, which have not very precise assemblers, so they often will compile also invalid syntax into something different than you originally wrote, so learning basic syntax is vital there, or checking against some cheat sheet. BTW your "nested loop" is basically my_data[index++] = (item+1)/2;
single loop.– Ped7g
Nov 13 '18 at 19:01
Thank you, i was working now on it and i updated the code, could you suggest anything at this point? There is an issue with running now. @Ped7g
– WOlf Veizcum
Nov 13 '18 at 19:38
Thank you, i was working now on it and i updated the code, could you suggest anything at this point? There is an issue with running now. @Ped7g
– WOlf Veizcum
Nov 13 '18 at 19:38
there is infinite loop in first
loop1
, both by t4
staying same and by every code path ending on j loop1
either way, even if t4
would count. BTW, you don't need separate index
, keep just pointer to my_data
and advance that (as both read of item
and write of result access the same index, so one pointer sequentially advancing +4 every outer loop is enough and simplest way of writing it). Do you MARS or SPIM? They have built-in debugger.. learn to use it, it will make "assembly" much easier– Ped7g
Nov 14 '18 at 0:06
there is infinite loop in first
loop1
, both by t4
staying same and by every code path ending on j loop1
either way, even if t4
would count. BTW, you don't need separate index
, keep just pointer to my_data
and advance that (as both read of item
and write of result access the same index, so one pointer sequentially advancing +4 every outer loop is enough and simplest way of writing it). Do you MARS or SPIM? They have built-in debugger.. learn to use it, it will make "assembly" much easier– Ped7g
Nov 14 '18 at 0:06
|
show 1 more comment
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282286%2fassembly-mips-converting-a-nested-for-loop-to-mips%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282286%2fassembly-mips-converting-a-nested-for-loop-to-mips%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qJCrK,I Uzf,8GgwVDwTW2c f hlh
Please don't add unrelated tags to your question.
– Felix Kling
Nov 13 '18 at 17:21
appreciate the thought, i am new in this website, learning takes time.
– WOlf Veizcum
Nov 13 '18 at 17:59
1
you have basic syntax errors, like
li $t1,$zero,$s0
, where even the assembler is trying to help you with desired syntax by "Expected: li $t1,-100" ... not sure what you need more, syntax has to be learnt and obeyed, especially with SPIM/MARS kind of simulator, which have not very precise assemblers, so they often will compile also invalid syntax into something different than you originally wrote, so learning basic syntax is vital there, or checking against some cheat sheet. BTW your "nested loop" is basicallymy_data[index++] = (item+1)/2;
single loop.– Ped7g
Nov 13 '18 at 19:01
Thank you, i was working now on it and i updated the code, could you suggest anything at this point? There is an issue with running now. @Ped7g
– WOlf Veizcum
Nov 13 '18 at 19:38
there is infinite loop in first
loop1
, both byt4
staying same and by every code path ending onj loop1
either way, even ift4
would count. BTW, you don't need separateindex
, keep just pointer tomy_data
and advance that (as both read ofitem
and write of result access the same index, so one pointer sequentially advancing +4 every outer loop is enough and simplest way of writing it). Do you MARS or SPIM? They have built-in debugger.. learn to use it, it will make "assembly" much easier– Ped7g
Nov 14 '18 at 0:06