Finding a reversed words in a given string using C program
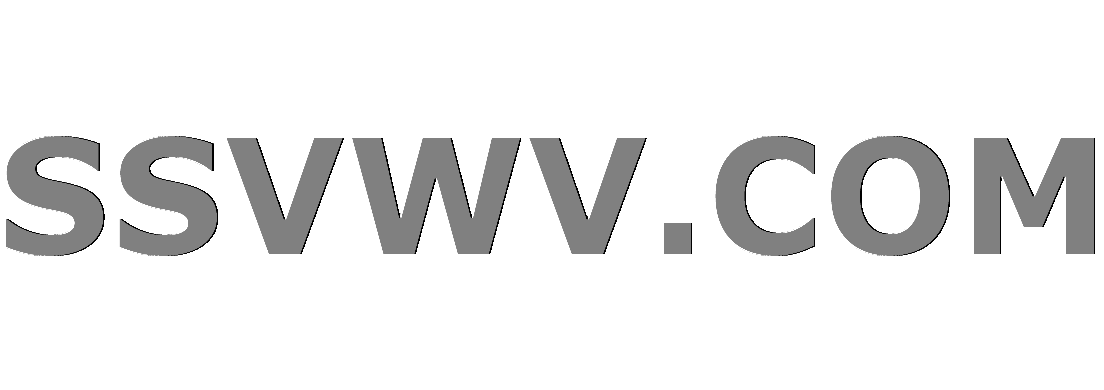
Multi tool use
Actually I have been searching for more than 1 week to find a solution for finding a reversed words in a given string using C. My question is, I have been given a string like this "bakelovekac". Here I have a reversed word of "ake" as "eka" in a string. Now I need to find out this reversed word in a given string and print it. How can it be done? Thanks in advance!
c
|
show 4 more comments
Actually I have been searching for more than 1 week to find a solution for finding a reversed words in a given string using C. My question is, I have been given a string like this "bakelovekac". Here I have a reversed word of "ake" as "eka" in a string. Now I need to find out this reversed word in a given string and print it. How can it be done? Thanks in advance!
c
Seems an ACM or OI problem... What's the range of string length? What's the time limit? If there's no restriction on time, you may just use a stupid brute force; otherwise you need some clever hash trick or dynamic programming I guess.
– Hoblovski
Nov 15 '18 at 1:41
There is no time limit
– Dhans
Nov 15 '18 at 2:10
is ake a word? is it just any series of characters or does it have to be a real word?
– Bwebb
Nov 15 '18 at 2:18
@Bwebb No it doesn't need to be a real word. Just being a series of characters is enough.
– Dhans
Nov 15 '18 at 2:22
any limit on the size of the original word?
– Bwebb
Nov 15 '18 at 2:40
|
show 4 more comments
Actually I have been searching for more than 1 week to find a solution for finding a reversed words in a given string using C. My question is, I have been given a string like this "bakelovekac". Here I have a reversed word of "ake" as "eka" in a string. Now I need to find out this reversed word in a given string and print it. How can it be done? Thanks in advance!
c
Actually I have been searching for more than 1 week to find a solution for finding a reversed words in a given string using C. My question is, I have been given a string like this "bakelovekac". Here I have a reversed word of "ake" as "eka" in a string. Now I need to find out this reversed word in a given string and print it. How can it be done? Thanks in advance!
c
c
asked Nov 15 '18 at 1:36


DhansDhans
1067
1067
Seems an ACM or OI problem... What's the range of string length? What's the time limit? If there's no restriction on time, you may just use a stupid brute force; otherwise you need some clever hash trick or dynamic programming I guess.
– Hoblovski
Nov 15 '18 at 1:41
There is no time limit
– Dhans
Nov 15 '18 at 2:10
is ake a word? is it just any series of characters or does it have to be a real word?
– Bwebb
Nov 15 '18 at 2:18
@Bwebb No it doesn't need to be a real word. Just being a series of characters is enough.
– Dhans
Nov 15 '18 at 2:22
any limit on the size of the original word?
– Bwebb
Nov 15 '18 at 2:40
|
show 4 more comments
Seems an ACM or OI problem... What's the range of string length? What's the time limit? If there's no restriction on time, you may just use a stupid brute force; otherwise you need some clever hash trick or dynamic programming I guess.
– Hoblovski
Nov 15 '18 at 1:41
There is no time limit
– Dhans
Nov 15 '18 at 2:10
is ake a word? is it just any series of characters or does it have to be a real word?
– Bwebb
Nov 15 '18 at 2:18
@Bwebb No it doesn't need to be a real word. Just being a series of characters is enough.
– Dhans
Nov 15 '18 at 2:22
any limit on the size of the original word?
– Bwebb
Nov 15 '18 at 2:40
Seems an ACM or OI problem... What's the range of string length? What's the time limit? If there's no restriction on time, you may just use a stupid brute force; otherwise you need some clever hash trick or dynamic programming I guess.
– Hoblovski
Nov 15 '18 at 1:41
Seems an ACM or OI problem... What's the range of string length? What's the time limit? If there's no restriction on time, you may just use a stupid brute force; otherwise you need some clever hash trick or dynamic programming I guess.
– Hoblovski
Nov 15 '18 at 1:41
There is no time limit
– Dhans
Nov 15 '18 at 2:10
There is no time limit
– Dhans
Nov 15 '18 at 2:10
is ake a word? is it just any series of characters or does it have to be a real word?
– Bwebb
Nov 15 '18 at 2:18
is ake a word? is it just any series of characters or does it have to be a real word?
– Bwebb
Nov 15 '18 at 2:18
@Bwebb No it doesn't need to be a real word. Just being a series of characters is enough.
– Dhans
Nov 15 '18 at 2:22
@Bwebb No it doesn't need to be a real word. Just being a series of characters is enough.
– Dhans
Nov 15 '18 at 2:22
any limit on the size of the original word?
– Bwebb
Nov 15 '18 at 2:40
any limit on the size of the original word?
– Bwebb
Nov 15 '18 at 2:40
|
show 4 more comments
2 Answers
2
active
oldest
votes
A basic approach would be to iterate over all the characters of the string and for each character check if it is being repeated, if yes then check for the presence of a possible reverse string.
A crude code for above approach would look something like this:
#include <stdio.h>
void checkForRevWord(char *str, char *rev)
int length = 0;
while(1)
if(str >= rev)
break;
if(*str != *rev)
break;
length++;
str++;
rev--;
if(length > 1)
while(length--)
printf("%c", *(rev+length+1));
printf("n");
return;
int main()
char *inputStr = "bakelovekac";
char *cur = inputStr;
char *tmp;
while(*cur != '')
tmp = cur+1;
/* find if current char gets repeated in the input string*/
while(*tmp != '')
if(*tmp == *cur)
checkForRevWord(cur, tmp);
tmp++;
cur++;
add a comment |
Go through this program
#include <stdio.h>
#include <string.h>
int main()
char text[50]; //this character array to store string
int len,i;
printf("Enter a textn");
scanf("%[^n]s",text);//getting the user input with spaces until the end of the line
len=strlen(text);//getting the length of the array and assigning it the len variable
for(i=len-1;i>=0;i--)
printf("%c",text[i]); //printing the text from backwards
return 0;
thank you.
This seems to not address the OPs question about finding a sub string inside the string. I will let him decide though.
– Bwebb
Nov 15 '18 at 20:19
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311240%2ffinding-a-reversed-words-in-a-given-string-using-c-program%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
A basic approach would be to iterate over all the characters of the string and for each character check if it is being repeated, if yes then check for the presence of a possible reverse string.
A crude code for above approach would look something like this:
#include <stdio.h>
void checkForRevWord(char *str, char *rev)
int length = 0;
while(1)
if(str >= rev)
break;
if(*str != *rev)
break;
length++;
str++;
rev--;
if(length > 1)
while(length--)
printf("%c", *(rev+length+1));
printf("n");
return;
int main()
char *inputStr = "bakelovekac";
char *cur = inputStr;
char *tmp;
while(*cur != '')
tmp = cur+1;
/* find if current char gets repeated in the input string*/
while(*tmp != '')
if(*tmp == *cur)
checkForRevWord(cur, tmp);
tmp++;
cur++;
add a comment |
A basic approach would be to iterate over all the characters of the string and for each character check if it is being repeated, if yes then check for the presence of a possible reverse string.
A crude code for above approach would look something like this:
#include <stdio.h>
void checkForRevWord(char *str, char *rev)
int length = 0;
while(1)
if(str >= rev)
break;
if(*str != *rev)
break;
length++;
str++;
rev--;
if(length > 1)
while(length--)
printf("%c", *(rev+length+1));
printf("n");
return;
int main()
char *inputStr = "bakelovekac";
char *cur = inputStr;
char *tmp;
while(*cur != '')
tmp = cur+1;
/* find if current char gets repeated in the input string*/
while(*tmp != '')
if(*tmp == *cur)
checkForRevWord(cur, tmp);
tmp++;
cur++;
add a comment |
A basic approach would be to iterate over all the characters of the string and for each character check if it is being repeated, if yes then check for the presence of a possible reverse string.
A crude code for above approach would look something like this:
#include <stdio.h>
void checkForRevWord(char *str, char *rev)
int length = 0;
while(1)
if(str >= rev)
break;
if(*str != *rev)
break;
length++;
str++;
rev--;
if(length > 1)
while(length--)
printf("%c", *(rev+length+1));
printf("n");
return;
int main()
char *inputStr = "bakelovekac";
char *cur = inputStr;
char *tmp;
while(*cur != '')
tmp = cur+1;
/* find if current char gets repeated in the input string*/
while(*tmp != '')
if(*tmp == *cur)
checkForRevWord(cur, tmp);
tmp++;
cur++;
A basic approach would be to iterate over all the characters of the string and for each character check if it is being repeated, if yes then check for the presence of a possible reverse string.
A crude code for above approach would look something like this:
#include <stdio.h>
void checkForRevWord(char *str, char *rev)
int length = 0;
while(1)
if(str >= rev)
break;
if(*str != *rev)
break;
length++;
str++;
rev--;
if(length > 1)
while(length--)
printf("%c", *(rev+length+1));
printf("n");
return;
int main()
char *inputStr = "bakelovekac";
char *cur = inputStr;
char *tmp;
while(*cur != '')
tmp = cur+1;
/* find if current char gets repeated in the input string*/
while(*tmp != '')
if(*tmp == *cur)
checkForRevWord(cur, tmp);
tmp++;
cur++;
answered Nov 15 '18 at 4:44
SandeepSandeep
647311
647311
add a comment |
add a comment |
Go through this program
#include <stdio.h>
#include <string.h>
int main()
char text[50]; //this character array to store string
int len,i;
printf("Enter a textn");
scanf("%[^n]s",text);//getting the user input with spaces until the end of the line
len=strlen(text);//getting the length of the array and assigning it the len variable
for(i=len-1;i>=0;i--)
printf("%c",text[i]); //printing the text from backwards
return 0;
thank you.
This seems to not address the OPs question about finding a sub string inside the string. I will let him decide though.
– Bwebb
Nov 15 '18 at 20:19
add a comment |
Go through this program
#include <stdio.h>
#include <string.h>
int main()
char text[50]; //this character array to store string
int len,i;
printf("Enter a textn");
scanf("%[^n]s",text);//getting the user input with spaces until the end of the line
len=strlen(text);//getting the length of the array and assigning it the len variable
for(i=len-1;i>=0;i--)
printf("%c",text[i]); //printing the text from backwards
return 0;
thank you.
This seems to not address the OPs question about finding a sub string inside the string. I will let him decide though.
– Bwebb
Nov 15 '18 at 20:19
add a comment |
Go through this program
#include <stdio.h>
#include <string.h>
int main()
char text[50]; //this character array to store string
int len,i;
printf("Enter a textn");
scanf("%[^n]s",text);//getting the user input with spaces until the end of the line
len=strlen(text);//getting the length of the array and assigning it the len variable
for(i=len-1;i>=0;i--)
printf("%c",text[i]); //printing the text from backwards
return 0;
thank you.
Go through this program
#include <stdio.h>
#include <string.h>
int main()
char text[50]; //this character array to store string
int len,i;
printf("Enter a textn");
scanf("%[^n]s",text);//getting the user input with spaces until the end of the line
len=strlen(text);//getting the length of the array and assigning it the len variable
for(i=len-1;i>=0;i--)
printf("%c",text[i]); //printing the text from backwards
return 0;
thank you.
answered Nov 15 '18 at 4:55
Jayaraj RohanJayaraj Rohan
164
164
This seems to not address the OPs question about finding a sub string inside the string. I will let him decide though.
– Bwebb
Nov 15 '18 at 20:19
add a comment |
This seems to not address the OPs question about finding a sub string inside the string. I will let him decide though.
– Bwebb
Nov 15 '18 at 20:19
This seems to not address the OPs question about finding a sub string inside the string. I will let him decide though.
– Bwebb
Nov 15 '18 at 20:19
This seems to not address the OPs question about finding a sub string inside the string. I will let him decide though.
– Bwebb
Nov 15 '18 at 20:19
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311240%2ffinding-a-reversed-words-in-a-given-string-using-c-program%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uuz,hj7FSjrbBGOzyAH03iOpBs3UKeNFS,Su9TW0Qq VdtfNaV9ZTU3sSkI
Seems an ACM or OI problem... What's the range of string length? What's the time limit? If there's no restriction on time, you may just use a stupid brute force; otherwise you need some clever hash trick or dynamic programming I guess.
– Hoblovski
Nov 15 '18 at 1:41
There is no time limit
– Dhans
Nov 15 '18 at 2:10
is ake a word? is it just any series of characters or does it have to be a real word?
– Bwebb
Nov 15 '18 at 2:18
@Bwebb No it doesn't need to be a real word. Just being a series of characters is enough.
– Dhans
Nov 15 '18 at 2:22
any limit on the size of the original word?
– Bwebb
Nov 15 '18 at 2:40