Checkbox input always return false value
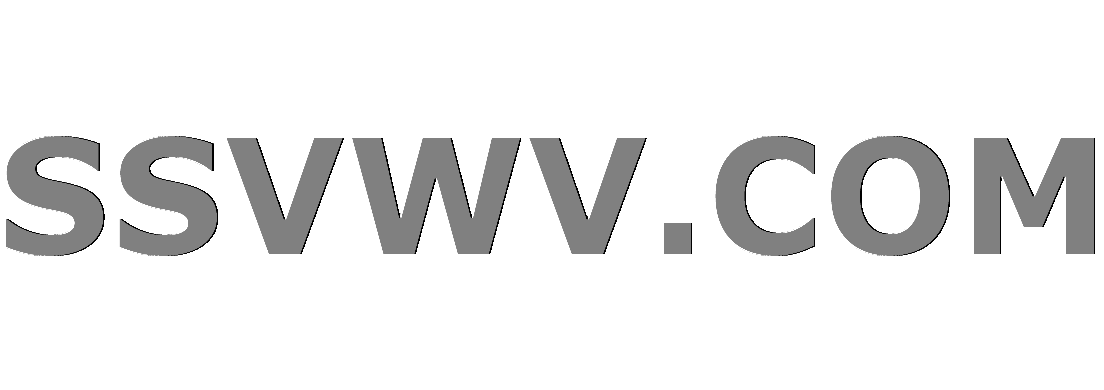
Multi tool use
I'm using asp.net core 2.1 and I have a simple view with form like:
@model Security.WebUi.Pages.AssignClaimToUserModel
<form method="post">
<div>
<label>User: </label>
<select asp-for="UserId" asp-items="@Model.UserList">
<option>Please select one</option>
</select>
</div>
<div>
<label>Role?</label>
<input type="checkbox" name="IsRole" id="isRole" />
</div>
<div>
<label>Claim Type</label>
<input type="text" name="ClaimType" id="claimType" />
</div>
<div>
<label>Claim Value</label>
<input type="text" name="ClaimValue" />
</div>
<button type="submit">Submit</button>
</form>
As you can see I have a checkbox with IsRole
property
So in my model I have a boolean:
public class ClaimToUserdModel
public string ClaimType get; set;
public string ClaimValue get; set;
public Guid UserId get; set;
public bool IsRole get; set;
then in method I call as:
public async Task<IActionResult> OnPost(ClaimToUserdModel model)
....
But it always throw false, it does not care about checked or not. What am I doing wrong?
c# asp.net asp.net-core asp.net-core-2.1
add a comment |
I'm using asp.net core 2.1 and I have a simple view with form like:
@model Security.WebUi.Pages.AssignClaimToUserModel
<form method="post">
<div>
<label>User: </label>
<select asp-for="UserId" asp-items="@Model.UserList">
<option>Please select one</option>
</select>
</div>
<div>
<label>Role?</label>
<input type="checkbox" name="IsRole" id="isRole" />
</div>
<div>
<label>Claim Type</label>
<input type="text" name="ClaimType" id="claimType" />
</div>
<div>
<label>Claim Value</label>
<input type="text" name="ClaimValue" />
</div>
<button type="submit">Submit</button>
</form>
As you can see I have a checkbox with IsRole
property
So in my model I have a boolean:
public class ClaimToUserdModel
public string ClaimType get; set;
public string ClaimValue get; set;
public Guid UserId get; set;
public bool IsRole get; set;
then in method I call as:
public async Task<IActionResult> OnPost(ClaimToUserdModel model)
....
But it always throw false, it does not care about checked or not. What am I doing wrong?
c# asp.net asp.net-core asp.net-core-2.1
2
By default a bool is false unless you set it to true somewhere in the code.
– Casperonian
Nov 15 '18 at 23:39
add a comment |
I'm using asp.net core 2.1 and I have a simple view with form like:
@model Security.WebUi.Pages.AssignClaimToUserModel
<form method="post">
<div>
<label>User: </label>
<select asp-for="UserId" asp-items="@Model.UserList">
<option>Please select one</option>
</select>
</div>
<div>
<label>Role?</label>
<input type="checkbox" name="IsRole" id="isRole" />
</div>
<div>
<label>Claim Type</label>
<input type="text" name="ClaimType" id="claimType" />
</div>
<div>
<label>Claim Value</label>
<input type="text" name="ClaimValue" />
</div>
<button type="submit">Submit</button>
</form>
As you can see I have a checkbox with IsRole
property
So in my model I have a boolean:
public class ClaimToUserdModel
public string ClaimType get; set;
public string ClaimValue get; set;
public Guid UserId get; set;
public bool IsRole get; set;
then in method I call as:
public async Task<IActionResult> OnPost(ClaimToUserdModel model)
....
But it always throw false, it does not care about checked or not. What am I doing wrong?
c# asp.net asp.net-core asp.net-core-2.1
I'm using asp.net core 2.1 and I have a simple view with form like:
@model Security.WebUi.Pages.AssignClaimToUserModel
<form method="post">
<div>
<label>User: </label>
<select asp-for="UserId" asp-items="@Model.UserList">
<option>Please select one</option>
</select>
</div>
<div>
<label>Role?</label>
<input type="checkbox" name="IsRole" id="isRole" />
</div>
<div>
<label>Claim Type</label>
<input type="text" name="ClaimType" id="claimType" />
</div>
<div>
<label>Claim Value</label>
<input type="text" name="ClaimValue" />
</div>
<button type="submit">Submit</button>
</form>
As you can see I have a checkbox with IsRole
property
So in my model I have a boolean:
public class ClaimToUserdModel
public string ClaimType get; set;
public string ClaimValue get; set;
public Guid UserId get; set;
public bool IsRole get; set;
then in method I call as:
public async Task<IActionResult> OnPost(ClaimToUserdModel model)
....
But it always throw false, it does not care about checked or not. What am I doing wrong?
c# asp.net asp.net-core asp.net-core-2.1
c# asp.net asp.net-core asp.net-core-2.1
asked Nov 15 '18 at 22:55
JonathanJonathan
26011
26011
2
By default a bool is false unless you set it to true somewhere in the code.
– Casperonian
Nov 15 '18 at 23:39
add a comment |
2
By default a bool is false unless you set it to true somewhere in the code.
– Casperonian
Nov 15 '18 at 23:39
2
2
By default a bool is false unless you set it to true somewhere in the code.
– Casperonian
Nov 15 '18 at 23:39
By default a bool is false unless you set it to true somewhere in the code.
– Casperonian
Nov 15 '18 at 23:39
add a comment |
2 Answers
2
active
oldest
votes
I got the same issue, I fixed it by writing html checkbox tag, giving it the name same as property name, and value = true, if the checkbox is not checked no need to worry as it won't be submitted anyway, in your case this will be it
<input type="checkbox" name="Remember" value="true" checked="@Model.YourmodelPropertyname"/>
also you set checkbox checked property value using jquery.
add a comment |
You can use try :
<input type="checkbox" name="IsRole" id="isRole" value="true" />
It seems you are using ASP.NET Core Razor Page ,you can also refer to below sample :
@page
@model razorpages.Pages.AssignClaimToUserModel
@
ViewData["Title"] = "AssignClaimToUser";
<form method="post">
<div>
<label>Role?</label>
<input asp-for="ClaimToUserdModel.IsRole" name="IsRole">
</div>
<button type="submit">Submit</button>
Code behind :
public class AssignClaimToUserModel : PageModel
public ClaimToUserdModel ClaimToUserdModel;
public void OnGet()
public async Task<IActionResult> OnPost(ClaimToUserdModel model)
return null;
public class ClaimToUserdModel
public string ClaimType get; set;
public string ClaimValue get; set;
public Guid UserId get; set;
public bool IsRole get; set;
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53329036%2fcheckbox-input-always-return-false-value%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I got the same issue, I fixed it by writing html checkbox tag, giving it the name same as property name, and value = true, if the checkbox is not checked no need to worry as it won't be submitted anyway, in your case this will be it
<input type="checkbox" name="Remember" value="true" checked="@Model.YourmodelPropertyname"/>
also you set checkbox checked property value using jquery.
add a comment |
I got the same issue, I fixed it by writing html checkbox tag, giving it the name same as property name, and value = true, if the checkbox is not checked no need to worry as it won't be submitted anyway, in your case this will be it
<input type="checkbox" name="Remember" value="true" checked="@Model.YourmodelPropertyname"/>
also you set checkbox checked property value using jquery.
add a comment |
I got the same issue, I fixed it by writing html checkbox tag, giving it the name same as property name, and value = true, if the checkbox is not checked no need to worry as it won't be submitted anyway, in your case this will be it
<input type="checkbox" name="Remember" value="true" checked="@Model.YourmodelPropertyname"/>
also you set checkbox checked property value using jquery.
I got the same issue, I fixed it by writing html checkbox tag, giving it the name same as property name, and value = true, if the checkbox is not checked no need to worry as it won't be submitted anyway, in your case this will be it
<input type="checkbox" name="Remember" value="true" checked="@Model.YourmodelPropertyname"/>
also you set checkbox checked property value using jquery.
answered Nov 16 '18 at 5:28
A.M. PatelA.M. Patel
689
689
add a comment |
add a comment |
You can use try :
<input type="checkbox" name="IsRole" id="isRole" value="true" />
It seems you are using ASP.NET Core Razor Page ,you can also refer to below sample :
@page
@model razorpages.Pages.AssignClaimToUserModel
@
ViewData["Title"] = "AssignClaimToUser";
<form method="post">
<div>
<label>Role?</label>
<input asp-for="ClaimToUserdModel.IsRole" name="IsRole">
</div>
<button type="submit">Submit</button>
Code behind :
public class AssignClaimToUserModel : PageModel
public ClaimToUserdModel ClaimToUserdModel;
public void OnGet()
public async Task<IActionResult> OnPost(ClaimToUserdModel model)
return null;
public class ClaimToUserdModel
public string ClaimType get; set;
public string ClaimValue get; set;
public Guid UserId get; set;
public bool IsRole get; set;
add a comment |
You can use try :
<input type="checkbox" name="IsRole" id="isRole" value="true" />
It seems you are using ASP.NET Core Razor Page ,you can also refer to below sample :
@page
@model razorpages.Pages.AssignClaimToUserModel
@
ViewData["Title"] = "AssignClaimToUser";
<form method="post">
<div>
<label>Role?</label>
<input asp-for="ClaimToUserdModel.IsRole" name="IsRole">
</div>
<button type="submit">Submit</button>
Code behind :
public class AssignClaimToUserModel : PageModel
public ClaimToUserdModel ClaimToUserdModel;
public void OnGet()
public async Task<IActionResult> OnPost(ClaimToUserdModel model)
return null;
public class ClaimToUserdModel
public string ClaimType get; set;
public string ClaimValue get; set;
public Guid UserId get; set;
public bool IsRole get; set;
add a comment |
You can use try :
<input type="checkbox" name="IsRole" id="isRole" value="true" />
It seems you are using ASP.NET Core Razor Page ,you can also refer to below sample :
@page
@model razorpages.Pages.AssignClaimToUserModel
@
ViewData["Title"] = "AssignClaimToUser";
<form method="post">
<div>
<label>Role?</label>
<input asp-for="ClaimToUserdModel.IsRole" name="IsRole">
</div>
<button type="submit">Submit</button>
Code behind :
public class AssignClaimToUserModel : PageModel
public ClaimToUserdModel ClaimToUserdModel;
public void OnGet()
public async Task<IActionResult> OnPost(ClaimToUserdModel model)
return null;
public class ClaimToUserdModel
public string ClaimType get; set;
public string ClaimValue get; set;
public Guid UserId get; set;
public bool IsRole get; set;
You can use try :
<input type="checkbox" name="IsRole" id="isRole" value="true" />
It seems you are using ASP.NET Core Razor Page ,you can also refer to below sample :
@page
@model razorpages.Pages.AssignClaimToUserModel
@
ViewData["Title"] = "AssignClaimToUser";
<form method="post">
<div>
<label>Role?</label>
<input asp-for="ClaimToUserdModel.IsRole" name="IsRole">
</div>
<button type="submit">Submit</button>
Code behind :
public class AssignClaimToUserModel : PageModel
public ClaimToUserdModel ClaimToUserdModel;
public void OnGet()
public async Task<IActionResult> OnPost(ClaimToUserdModel model)
return null;
public class ClaimToUserdModel
public string ClaimType get; set;
public string ClaimValue get; set;
public Guid UserId get; set;
public bool IsRole get; set;
answered Nov 16 '18 at 3:40


Nan YuNan Yu
7,1652761
7,1652761
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53329036%2fcheckbox-input-always-return-false-value%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
d9,tT7wZ
2
By default a bool is false unless you set it to true somewhere in the code.
– Casperonian
Nov 15 '18 at 23:39