initialValues prefills data only when hardcoded but not with dynamic data
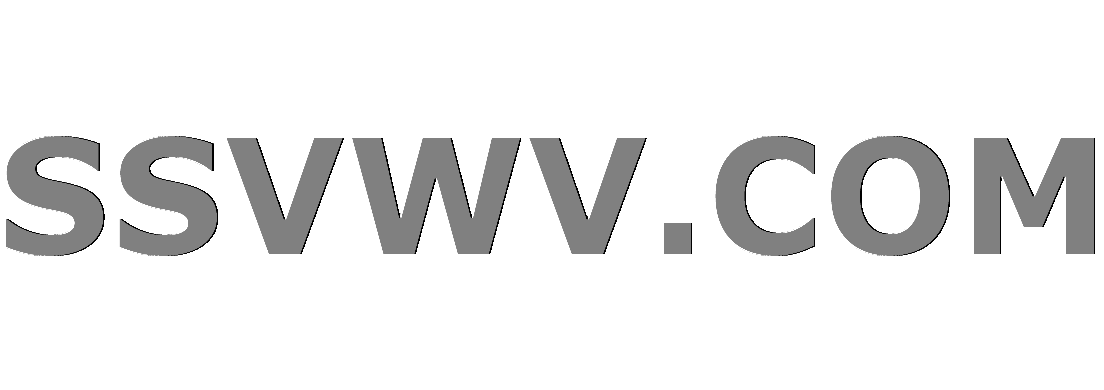
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty height:90px;width:728px;box-sizing:border-box;
I am trying to populate a form field inside a dialog and I am using redux form
Parent Component Code
constructor(props)
super(props);
this.state =
user: ,
student: ,
url: false,
permissions: 'canEdit',
answers:,
questions:,
applications:,
isAddNoteDialogOpen: false,
application_note: 'Hey'
this.toggleDialog = this.toggleDialog.bind(this);
componentDidMount()
console.log('parent');
if(this.props.match.params.id)
let axiosConfig = ;
axiosConfig.headers =
Authorization: 'Bearer ' + this.props.auth.token
;
const url = `/application/internship/$this.props.match.params.id`
axios
.get(url, axiosConfig)
.then((response) =>
this.setState(
student: response.data.student,
user: response.data.student.user,
url: true,
permissions: response.data.permissions,
applications: response.data.application[0],
answers: response.data.application[0].application_answers,
application_note: response.data.application[0].application_note,
questions: response.data.questions
);
);
child component being called from parent
<AddNoteDialog
initialValues=application_note: this.state.application_note
application_note=this.state.application_note
application=applications.id
isOpen=isChangePasswordDialogOpen
setOpen=status =>
this.toggleDialog(status, 'isChangePasswordDialogOpen')
/>
AddNoteDialogComponent
render()
const isOpen, setOpen, submitting, handleSubmit = this.props;
return (
<Dialog
fullWidth
open=isOpen
onClose=() => setOpen(false)
aria-labelledby="add-note-title"
>
<DialogTitle id="add-note-title">Enter Note</DialogTitle>
<DialogContent>
/* <DialogContentText>Some Text</DialogContentText> */
<form
id="add-note-details"
onSubmit=handleSubmit(this.addNoteSubmit.bind(this))
>
<Grid
container
justify='center'
direction='column'
spacing=24
>
<Grid item xs=12>
<Field
name="application_note"
component=renderTextField
label="Note"
type="Text"
required
placeholder="Enter Note"
/>
</Grid>
</Grid>
</form>
</DialogContent>
<DialogActions>
<LoadingButton
variant="contained"
color="secondary"
type="submit"
form='add-note-details'
submitting=submitting
>
UPDATE
</LoadingButton>
<Button
id="closeModal"
color="primary"
onClick=() => setOpen(false)
>
Cancel
</Button>
</DialogActions>
</Dialog>
);
}
export default reduxForm(
form: 'add-note-details-form',
validate
)(AddNoteDialogComponent);
Now when I hardcode initialValues=application_note: "Hey"
or if I set a default state then it works properly but with data coming from axios it does not show any data
Please Note :- this.state.application_note is updating and I have only pasted code which I thought was relevant but if you need anything else then do lemme know
reactjs redux redux-form
add a comment |
I am trying to populate a form field inside a dialog and I am using redux form
Parent Component Code
constructor(props)
super(props);
this.state =
user: ,
student: ,
url: false,
permissions: 'canEdit',
answers:,
questions:,
applications:,
isAddNoteDialogOpen: false,
application_note: 'Hey'
this.toggleDialog = this.toggleDialog.bind(this);
componentDidMount()
console.log('parent');
if(this.props.match.params.id)
let axiosConfig = ;
axiosConfig.headers =
Authorization: 'Bearer ' + this.props.auth.token
;
const url = `/application/internship/$this.props.match.params.id`
axios
.get(url, axiosConfig)
.then((response) =>
this.setState(
student: response.data.student,
user: response.data.student.user,
url: true,
permissions: response.data.permissions,
applications: response.data.application[0],
answers: response.data.application[0].application_answers,
application_note: response.data.application[0].application_note,
questions: response.data.questions
);
);
child component being called from parent
<AddNoteDialog
initialValues=application_note: this.state.application_note
application_note=this.state.application_note
application=applications.id
isOpen=isChangePasswordDialogOpen
setOpen=status =>
this.toggleDialog(status, 'isChangePasswordDialogOpen')
/>
AddNoteDialogComponent
render()
const isOpen, setOpen, submitting, handleSubmit = this.props;
return (
<Dialog
fullWidth
open=isOpen
onClose=() => setOpen(false)
aria-labelledby="add-note-title"
>
<DialogTitle id="add-note-title">Enter Note</DialogTitle>
<DialogContent>
/* <DialogContentText>Some Text</DialogContentText> */
<form
id="add-note-details"
onSubmit=handleSubmit(this.addNoteSubmit.bind(this))
>
<Grid
container
justify='center'
direction='column'
spacing=24
>
<Grid item xs=12>
<Field
name="application_note"
component=renderTextField
label="Note"
type="Text"
required
placeholder="Enter Note"
/>
</Grid>
</Grid>
</form>
</DialogContent>
<DialogActions>
<LoadingButton
variant="contained"
color="secondary"
type="submit"
form='add-note-details'
submitting=submitting
>
UPDATE
</LoadingButton>
<Button
id="closeModal"
color="primary"
onClick=() => setOpen(false)
>
Cancel
</Button>
</DialogActions>
</Dialog>
);
}
export default reduxForm(
form: 'add-note-details-form',
validate
)(AddNoteDialogComponent);
Now when I hardcode initialValues=application_note: "Hey"
or if I set a default state then it works properly but with data coming from axios it does not show any data
Please Note :- this.state.application_note is updating and I have only pasted code which I thought was relevant but if you need anything else then do lemme know
reactjs redux redux-form
add a comment |
I am trying to populate a form field inside a dialog and I am using redux form
Parent Component Code
constructor(props)
super(props);
this.state =
user: ,
student: ,
url: false,
permissions: 'canEdit',
answers:,
questions:,
applications:,
isAddNoteDialogOpen: false,
application_note: 'Hey'
this.toggleDialog = this.toggleDialog.bind(this);
componentDidMount()
console.log('parent');
if(this.props.match.params.id)
let axiosConfig = ;
axiosConfig.headers =
Authorization: 'Bearer ' + this.props.auth.token
;
const url = `/application/internship/$this.props.match.params.id`
axios
.get(url, axiosConfig)
.then((response) =>
this.setState(
student: response.data.student,
user: response.data.student.user,
url: true,
permissions: response.data.permissions,
applications: response.data.application[0],
answers: response.data.application[0].application_answers,
application_note: response.data.application[0].application_note,
questions: response.data.questions
);
);
child component being called from parent
<AddNoteDialog
initialValues=application_note: this.state.application_note
application_note=this.state.application_note
application=applications.id
isOpen=isChangePasswordDialogOpen
setOpen=status =>
this.toggleDialog(status, 'isChangePasswordDialogOpen')
/>
AddNoteDialogComponent
render()
const isOpen, setOpen, submitting, handleSubmit = this.props;
return (
<Dialog
fullWidth
open=isOpen
onClose=() => setOpen(false)
aria-labelledby="add-note-title"
>
<DialogTitle id="add-note-title">Enter Note</DialogTitle>
<DialogContent>
/* <DialogContentText>Some Text</DialogContentText> */
<form
id="add-note-details"
onSubmit=handleSubmit(this.addNoteSubmit.bind(this))
>
<Grid
container
justify='center'
direction='column'
spacing=24
>
<Grid item xs=12>
<Field
name="application_note"
component=renderTextField
label="Note"
type="Text"
required
placeholder="Enter Note"
/>
</Grid>
</Grid>
</form>
</DialogContent>
<DialogActions>
<LoadingButton
variant="contained"
color="secondary"
type="submit"
form='add-note-details'
submitting=submitting
>
UPDATE
</LoadingButton>
<Button
id="closeModal"
color="primary"
onClick=() => setOpen(false)
>
Cancel
</Button>
</DialogActions>
</Dialog>
);
}
export default reduxForm(
form: 'add-note-details-form',
validate
)(AddNoteDialogComponent);
Now when I hardcode initialValues=application_note: "Hey"
or if I set a default state then it works properly but with data coming from axios it does not show any data
Please Note :- this.state.application_note is updating and I have only pasted code which I thought was relevant but if you need anything else then do lemme know
reactjs redux redux-form
I am trying to populate a form field inside a dialog and I am using redux form
Parent Component Code
constructor(props)
super(props);
this.state =
user: ,
student: ,
url: false,
permissions: 'canEdit',
answers:,
questions:,
applications:,
isAddNoteDialogOpen: false,
application_note: 'Hey'
this.toggleDialog = this.toggleDialog.bind(this);
componentDidMount()
console.log('parent');
if(this.props.match.params.id)
let axiosConfig = ;
axiosConfig.headers =
Authorization: 'Bearer ' + this.props.auth.token
;
const url = `/application/internship/$this.props.match.params.id`
axios
.get(url, axiosConfig)
.then((response) =>
this.setState(
student: response.data.student,
user: response.data.student.user,
url: true,
permissions: response.data.permissions,
applications: response.data.application[0],
answers: response.data.application[0].application_answers,
application_note: response.data.application[0].application_note,
questions: response.data.questions
);
);
child component being called from parent
<AddNoteDialog
initialValues=application_note: this.state.application_note
application_note=this.state.application_note
application=applications.id
isOpen=isChangePasswordDialogOpen
setOpen=status =>
this.toggleDialog(status, 'isChangePasswordDialogOpen')
/>
AddNoteDialogComponent
render()
const isOpen, setOpen, submitting, handleSubmit = this.props;
return (
<Dialog
fullWidth
open=isOpen
onClose=() => setOpen(false)
aria-labelledby="add-note-title"
>
<DialogTitle id="add-note-title">Enter Note</DialogTitle>
<DialogContent>
/* <DialogContentText>Some Text</DialogContentText> */
<form
id="add-note-details"
onSubmit=handleSubmit(this.addNoteSubmit.bind(this))
>
<Grid
container
justify='center'
direction='column'
spacing=24
>
<Grid item xs=12>
<Field
name="application_note"
component=renderTextField
label="Note"
type="Text"
required
placeholder="Enter Note"
/>
</Grid>
</Grid>
</form>
</DialogContent>
<DialogActions>
<LoadingButton
variant="contained"
color="secondary"
type="submit"
form='add-note-details'
submitting=submitting
>
UPDATE
</LoadingButton>
<Button
id="closeModal"
color="primary"
onClick=() => setOpen(false)
>
Cancel
</Button>
</DialogActions>
</Dialog>
);
}
export default reduxForm(
form: 'add-note-details-form',
validate
)(AddNoteDialogComponent);
Now when I hardcode initialValues=application_note: "Hey"
or if I set a default state then it works properly but with data coming from axios it does not show any data
Please Note :- this.state.application_note is updating and I have only pasted code which I thought was relevant but if you need anything else then do lemme know
reactjs redux redux-form
reactjs redux redux-form
asked Nov 16 '18 at 11:46
Dhaval ChhedaDhaval Chheda
2,50811225
2,50811225
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The axios is call is asynchronous so in this line:
initialValues=application_note: this.state.application_note
this.state.application_note
is probably undefined
.
You have to wait for the axios request to finish and then you need to update your state and then you need to render the form.
Something like this:
componentDidMount()
...
axios.get(url, axiosConfig)
.then((response) =>
this.setState(
student: response.data.student,
user: response.data.student.user,
url: true,
permissions: response.data.permissions,
applications: response.data.application[0],
answers: response.data.application[0].application_answers,
application_note: response.data.application[0].application_note,
questions: response.data.questions
);
);
...
render()
// If we don't have the application_note then we don't want to show the form.
// We want this data so that when we do show the form initialValues will contain
// the data we want
if (!this.state.application_note)
return 'Loading...';
// If we do have the data then we can return the form component.
return (<YourFormComponent />);
add a comment |
The solution that worked for me was to add
enableReinitialize: true in the reduxForm() export in the Dialog component like below
export default reduxForm(
form: 'add-note-details-form',
validate,
enableReinitialize: true
)(AddNoteDialogComponent);
Hope this helps somebody
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53337251%2finitialvalues-prefills-data-only-when-hardcoded-but-not-with-dynamic-data%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The axios is call is asynchronous so in this line:
initialValues=application_note: this.state.application_note
this.state.application_note
is probably undefined
.
You have to wait for the axios request to finish and then you need to update your state and then you need to render the form.
Something like this:
componentDidMount()
...
axios.get(url, axiosConfig)
.then((response) =>
this.setState(
student: response.data.student,
user: response.data.student.user,
url: true,
permissions: response.data.permissions,
applications: response.data.application[0],
answers: response.data.application[0].application_answers,
application_note: response.data.application[0].application_note,
questions: response.data.questions
);
);
...
render()
// If we don't have the application_note then we don't want to show the form.
// We want this data so that when we do show the form initialValues will contain
// the data we want
if (!this.state.application_note)
return 'Loading...';
// If we do have the data then we can return the form component.
return (<YourFormComponent />);
add a comment |
The axios is call is asynchronous so in this line:
initialValues=application_note: this.state.application_note
this.state.application_note
is probably undefined
.
You have to wait for the axios request to finish and then you need to update your state and then you need to render the form.
Something like this:
componentDidMount()
...
axios.get(url, axiosConfig)
.then((response) =>
this.setState(
student: response.data.student,
user: response.data.student.user,
url: true,
permissions: response.data.permissions,
applications: response.data.application[0],
answers: response.data.application[0].application_answers,
application_note: response.data.application[0].application_note,
questions: response.data.questions
);
);
...
render()
// If we don't have the application_note then we don't want to show the form.
// We want this data so that when we do show the form initialValues will contain
// the data we want
if (!this.state.application_note)
return 'Loading...';
// If we do have the data then we can return the form component.
return (<YourFormComponent />);
add a comment |
The axios is call is asynchronous so in this line:
initialValues=application_note: this.state.application_note
this.state.application_note
is probably undefined
.
You have to wait for the axios request to finish and then you need to update your state and then you need to render the form.
Something like this:
componentDidMount()
...
axios.get(url, axiosConfig)
.then((response) =>
this.setState(
student: response.data.student,
user: response.data.student.user,
url: true,
permissions: response.data.permissions,
applications: response.data.application[0],
answers: response.data.application[0].application_answers,
application_note: response.data.application[0].application_note,
questions: response.data.questions
);
);
...
render()
// If we don't have the application_note then we don't want to show the form.
// We want this data so that when we do show the form initialValues will contain
// the data we want
if (!this.state.application_note)
return 'Loading...';
// If we do have the data then we can return the form component.
return (<YourFormComponent />);
The axios is call is asynchronous so in this line:
initialValues=application_note: this.state.application_note
this.state.application_note
is probably undefined
.
You have to wait for the axios request to finish and then you need to update your state and then you need to render the form.
Something like this:
componentDidMount()
...
axios.get(url, axiosConfig)
.then((response) =>
this.setState(
student: response.data.student,
user: response.data.student.user,
url: true,
permissions: response.data.permissions,
applications: response.data.application[0],
answers: response.data.application[0].application_answers,
application_note: response.data.application[0].application_note,
questions: response.data.questions
);
);
...
render()
// If we don't have the application_note then we don't want to show the form.
// We want this data so that when we do show the form initialValues will contain
// the data we want
if (!this.state.application_note)
return 'Loading...';
// If we do have the data then we can return the form component.
return (<YourFormComponent />);
answered Nov 16 '18 at 12:37


RupertRupert
1,066620
1,066620
add a comment |
add a comment |
The solution that worked for me was to add
enableReinitialize: true in the reduxForm() export in the Dialog component like below
export default reduxForm(
form: 'add-note-details-form',
validate,
enableReinitialize: true
)(AddNoteDialogComponent);
Hope this helps somebody
add a comment |
The solution that worked for me was to add
enableReinitialize: true in the reduxForm() export in the Dialog component like below
export default reduxForm(
form: 'add-note-details-form',
validate,
enableReinitialize: true
)(AddNoteDialogComponent);
Hope this helps somebody
add a comment |
The solution that worked for me was to add
enableReinitialize: true in the reduxForm() export in the Dialog component like below
export default reduxForm(
form: 'add-note-details-form',
validate,
enableReinitialize: true
)(AddNoteDialogComponent);
Hope this helps somebody
The solution that worked for me was to add
enableReinitialize: true in the reduxForm() export in the Dialog component like below
export default reduxForm(
form: 'add-note-details-form',
validate,
enableReinitialize: true
)(AddNoteDialogComponent);
Hope this helps somebody
answered Nov 16 '18 at 16:09
Dhaval ChhedaDhaval Chheda
2,50811225
2,50811225
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53337251%2finitialvalues-prefills-data-only-when-hardcoded-but-not-with-dynamic-data%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
H2VbSjn3,Oz,FGrhC7r9dDOhe43Phk9ZpXZoUhWBBfIyjhtRAK1j