Find index of nth occurence of 1 in binary vector (matlab)
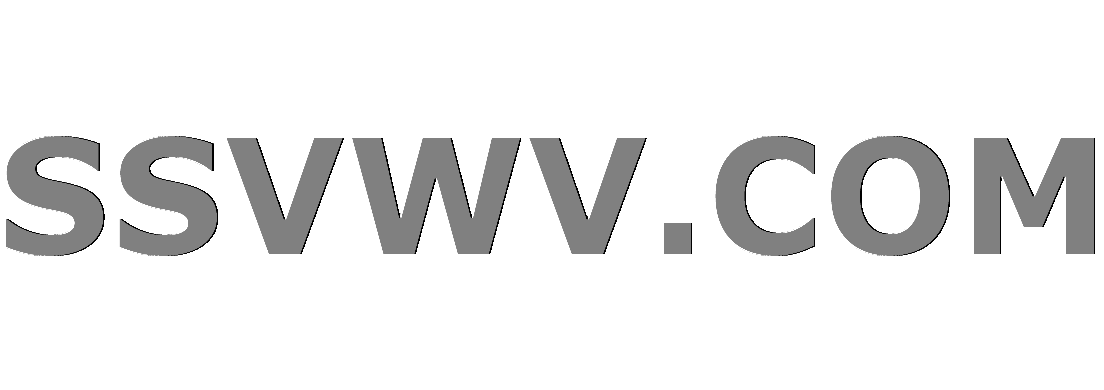
Multi tool use
up vote
0
down vote
favorite
I have a binary vector like this x = [0 0 1 1 1 1 1 1 1]
. I want to find the index of lets say the 7th 1, which is 9.
I know I can do this:
y = find(x);
index = y(7);
But what if the vector is huge and I want to conserve memory usage? Wouldn't y = find(x)
use alot of memory? If so, is there any way around this?
I am using this as an alternate way of storing indexes for nonbasis and basis elements in a linear programming problem. So I would like to avoid storing the indices as numerical values.
Would the following be a good solution?
basis = [0 0 1 1 1 1 1 1 1];
basisIndex = 7;
correctIndex = getIndex(basisIndex, basis); % should be 9 when basisIndex = 7
function ret = getIndex(basisIndex, basis)
counter = 1;
for value = find(basis) % iterate through [3, 4, 5, 6, 7, 8, 9]
if counter == basisIndex
ret = value;
break;
end
counter = counter + 1;
end
end
matlab vector indexing binary
add a comment |
up vote
0
down vote
favorite
I have a binary vector like this x = [0 0 1 1 1 1 1 1 1]
. I want to find the index of lets say the 7th 1, which is 9.
I know I can do this:
y = find(x);
index = y(7);
But what if the vector is huge and I want to conserve memory usage? Wouldn't y = find(x)
use alot of memory? If so, is there any way around this?
I am using this as an alternate way of storing indexes for nonbasis and basis elements in a linear programming problem. So I would like to avoid storing the indices as numerical values.
Would the following be a good solution?
basis = [0 0 1 1 1 1 1 1 1];
basisIndex = 7;
correctIndex = getIndex(basisIndex, basis); % should be 9 when basisIndex = 7
function ret = getIndex(basisIndex, basis)
counter = 1;
for value = find(basis) % iterate through [3, 4, 5, 6, 7, 8, 9]
if counter == basisIndex
ret = value;
break;
end
counter = counter + 1;
end
end
matlab vector indexing binary
I added some information at the end of the question. I was thinking that saving the values returned by find would take away the advantage of storing the indices in a binary vector.
– StraightUpBusta
2 days ago
@SardarUsama I was thinking that the function I added would conserve memory?
– StraightUpBusta
2 days ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a binary vector like this x = [0 0 1 1 1 1 1 1 1]
. I want to find the index of lets say the 7th 1, which is 9.
I know I can do this:
y = find(x);
index = y(7);
But what if the vector is huge and I want to conserve memory usage? Wouldn't y = find(x)
use alot of memory? If so, is there any way around this?
I am using this as an alternate way of storing indexes for nonbasis and basis elements in a linear programming problem. So I would like to avoid storing the indices as numerical values.
Would the following be a good solution?
basis = [0 0 1 1 1 1 1 1 1];
basisIndex = 7;
correctIndex = getIndex(basisIndex, basis); % should be 9 when basisIndex = 7
function ret = getIndex(basisIndex, basis)
counter = 1;
for value = find(basis) % iterate through [3, 4, 5, 6, 7, 8, 9]
if counter == basisIndex
ret = value;
break;
end
counter = counter + 1;
end
end
matlab vector indexing binary
I have a binary vector like this x = [0 0 1 1 1 1 1 1 1]
. I want to find the index of lets say the 7th 1, which is 9.
I know I can do this:
y = find(x);
index = y(7);
But what if the vector is huge and I want to conserve memory usage? Wouldn't y = find(x)
use alot of memory? If so, is there any way around this?
I am using this as an alternate way of storing indexes for nonbasis and basis elements in a linear programming problem. So I would like to avoid storing the indices as numerical values.
Would the following be a good solution?
basis = [0 0 1 1 1 1 1 1 1];
basisIndex = 7;
correctIndex = getIndex(basisIndex, basis); % should be 9 when basisIndex = 7
function ret = getIndex(basisIndex, basis)
counter = 1;
for value = find(basis) % iterate through [3, 4, 5, 6, 7, 8, 9]
if counter == basisIndex
ret = value;
break;
end
counter = counter + 1;
end
end
matlab vector indexing binary
matlab vector indexing binary
edited 2 days ago
asked 2 days ago


StraightUpBusta
346
346
I added some information at the end of the question. I was thinking that saving the values returned by find would take away the advantage of storing the indices in a binary vector.
– StraightUpBusta
2 days ago
@SardarUsama I was thinking that the function I added would conserve memory?
– StraightUpBusta
2 days ago
add a comment |
I added some information at the end of the question. I was thinking that saving the values returned by find would take away the advantage of storing the indices in a binary vector.
– StraightUpBusta
2 days ago
@SardarUsama I was thinking that the function I added would conserve memory?
– StraightUpBusta
2 days ago
I added some information at the end of the question. I was thinking that saving the values returned by find would take away the advantage of storing the indices in a binary vector.
– StraightUpBusta
2 days ago
I added some information at the end of the question. I was thinking that saving the values returned by find would take away the advantage of storing the indices in a binary vector.
– StraightUpBusta
2 days ago
@SardarUsama I was thinking that the function I added would conserve memory?
– StraightUpBusta
2 days ago
@SardarUsama I was thinking that the function I added would conserve memory?
– StraightUpBusta
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
Just iterate through x
. First, it will not create a new vector y=find(x)
(save memory). Second, if basisIndex
is small, it will be more efficient.
Suppose x
is a 1e8 by 1 vector. Let's compare find
with just iteration.
basis = randi(2,1e8,1) - 1;
basisIndex = 7;
tic % your first method
y = find(basis);
index = y(basisIndex);
toc
tic % iterate through base
index = 1;
match = 0;
while true
if basis(index)
match = match + 1;
if match == basisIndex
break
end
end
index = index + 1;
end
toc
Output
Elapsed time is 1.214597 seconds.
Elapsed time is 0.000061 seconds.
Even if the basisIndex
is large
basisIndex = 5e7;
The result from iteration is still more efficient
Elapsed time is 1.250430 seconds. % use find
Elapsed time is 0.757767 seconds. % use iteration
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Just iterate through x
. First, it will not create a new vector y=find(x)
(save memory). Second, if basisIndex
is small, it will be more efficient.
Suppose x
is a 1e8 by 1 vector. Let's compare find
with just iteration.
basis = randi(2,1e8,1) - 1;
basisIndex = 7;
tic % your first method
y = find(basis);
index = y(basisIndex);
toc
tic % iterate through base
index = 1;
match = 0;
while true
if basis(index)
match = match + 1;
if match == basisIndex
break
end
end
index = index + 1;
end
toc
Output
Elapsed time is 1.214597 seconds.
Elapsed time is 0.000061 seconds.
Even if the basisIndex
is large
basisIndex = 5e7;
The result from iteration is still more efficient
Elapsed time is 1.250430 seconds. % use find
Elapsed time is 0.757767 seconds. % use iteration
add a comment |
up vote
2
down vote
accepted
Just iterate through x
. First, it will not create a new vector y=find(x)
(save memory). Second, if basisIndex
is small, it will be more efficient.
Suppose x
is a 1e8 by 1 vector. Let's compare find
with just iteration.
basis = randi(2,1e8,1) - 1;
basisIndex = 7;
tic % your first method
y = find(basis);
index = y(basisIndex);
toc
tic % iterate through base
index = 1;
match = 0;
while true
if basis(index)
match = match + 1;
if match == basisIndex
break
end
end
index = index + 1;
end
toc
Output
Elapsed time is 1.214597 seconds.
Elapsed time is 0.000061 seconds.
Even if the basisIndex
is large
basisIndex = 5e7;
The result from iteration is still more efficient
Elapsed time is 1.250430 seconds. % use find
Elapsed time is 0.757767 seconds. % use iteration
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Just iterate through x
. First, it will not create a new vector y=find(x)
(save memory). Second, if basisIndex
is small, it will be more efficient.
Suppose x
is a 1e8 by 1 vector. Let's compare find
with just iteration.
basis = randi(2,1e8,1) - 1;
basisIndex = 7;
tic % your first method
y = find(basis);
index = y(basisIndex);
toc
tic % iterate through base
index = 1;
match = 0;
while true
if basis(index)
match = match + 1;
if match == basisIndex
break
end
end
index = index + 1;
end
toc
Output
Elapsed time is 1.214597 seconds.
Elapsed time is 0.000061 seconds.
Even if the basisIndex
is large
basisIndex = 5e7;
The result from iteration is still more efficient
Elapsed time is 1.250430 seconds. % use find
Elapsed time is 0.757767 seconds. % use iteration
Just iterate through x
. First, it will not create a new vector y=find(x)
(save memory). Second, if basisIndex
is small, it will be more efficient.
Suppose x
is a 1e8 by 1 vector. Let's compare find
with just iteration.
basis = randi(2,1e8,1) - 1;
basisIndex = 7;
tic % your first method
y = find(basis);
index = y(basisIndex);
toc
tic % iterate through base
index = 1;
match = 0;
while true
if basis(index)
match = match + 1;
if match == basisIndex
break
end
end
index = index + 1;
end
toc
Output
Elapsed time is 1.214597 seconds.
Elapsed time is 0.000061 seconds.
Even if the basisIndex
is large
basisIndex = 5e7;
The result from iteration is still more efficient
Elapsed time is 1.250430 seconds. % use find
Elapsed time is 0.757767 seconds. % use iteration
answered 2 days ago


Banghua Zhao
696217
696217
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237630%2ffind-index-of-nth-occurence-of-1-in-binary-vector-matlab%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
0xM9x697dNS7vG8vuufQirCEcOjuTsqVKYtb,UnLRjS,8GJn9yqt7Fr2lWzVgto M uWdiRVvK8qr5AmbQh H GwrK7mxCu HAxdMr6Q64
I added some information at the end of the question. I was thinking that saving the values returned by find would take away the advantage of storing the indices in a binary vector.
– StraightUpBusta
2 days ago
@SardarUsama I was thinking that the function I added would conserve memory?
– StraightUpBusta
2 days ago