Getting selected value from Picker in Xamarin
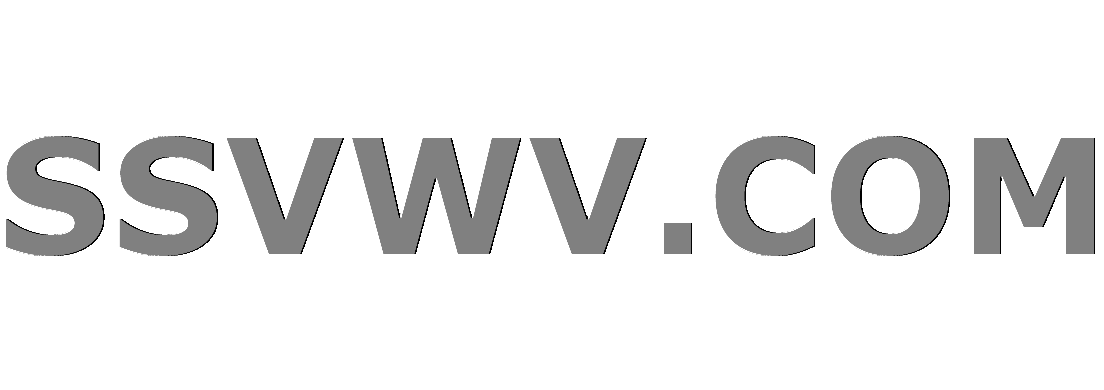
Multi tool use
up vote
0
down vote
favorite
I am getting data from a web service and I am loading it in Picker. Now I want to call a new web service to get some data related to selected item. But I am not getting that selected item.
I am using below class model to get data from web service and loading it in Picker.
public class ModelGetEmployeeList
public string ServiceStatus get; set;
public List<EmployeeList> EmpList get; set;
public class EmployeeList
public string uid get; set;
public string fname get; set;
public string lname get; set;
This is how I loaded data in Picker
:
if (response.IsSuccessStatusCode)
var content = await response.Content.ReadAsStringAsync ();
var Items = JsonConvert.DeserializeObject <ModelGetEmployeeList> (content);
foreach(EmployeeList emp in Items.EmpList)
pickerEmployee.Items.Add(emp.uid.ToString()+"-"+emp.fname.ToString()+" "+emp.lname.ToString());
Now I am implementing SelectedIndexChanged
event like this:
public void PickerEmployee_SelectedIndexChanged(object sender, SelectedItemChangedEventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var item = sender as EmployeeList;
var selectedItem = item.uid;
DisplayAlert (selectedItem.ToString (), "OK", "OK");
But its giving me an error that above method has wrong signature.
xamarin.forms picker
add a comment |
up vote
0
down vote
favorite
I am getting data from a web service and I am loading it in Picker. Now I want to call a new web service to get some data related to selected item. But I am not getting that selected item.
I am using below class model to get data from web service and loading it in Picker.
public class ModelGetEmployeeList
public string ServiceStatus get; set;
public List<EmployeeList> EmpList get; set;
public class EmployeeList
public string uid get; set;
public string fname get; set;
public string lname get; set;
This is how I loaded data in Picker
:
if (response.IsSuccessStatusCode)
var content = await response.Content.ReadAsStringAsync ();
var Items = JsonConvert.DeserializeObject <ModelGetEmployeeList> (content);
foreach(EmployeeList emp in Items.EmpList)
pickerEmployee.Items.Add(emp.uid.ToString()+"-"+emp.fname.ToString()+" "+emp.lname.ToString());
Now I am implementing SelectedIndexChanged
event like this:
public void PickerEmployee_SelectedIndexChanged(object sender, SelectedItemChangedEventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var item = sender as EmployeeList;
var selectedItem = item.uid;
DisplayAlert (selectedItem.ToString (), "OK", "OK");
But its giving me an error that above method has wrong signature.
xamarin.forms picker
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am getting data from a web service and I am loading it in Picker. Now I want to call a new web service to get some data related to selected item. But I am not getting that selected item.
I am using below class model to get data from web service and loading it in Picker.
public class ModelGetEmployeeList
public string ServiceStatus get; set;
public List<EmployeeList> EmpList get; set;
public class EmployeeList
public string uid get; set;
public string fname get; set;
public string lname get; set;
This is how I loaded data in Picker
:
if (response.IsSuccessStatusCode)
var content = await response.Content.ReadAsStringAsync ();
var Items = JsonConvert.DeserializeObject <ModelGetEmployeeList> (content);
foreach(EmployeeList emp in Items.EmpList)
pickerEmployee.Items.Add(emp.uid.ToString()+"-"+emp.fname.ToString()+" "+emp.lname.ToString());
Now I am implementing SelectedIndexChanged
event like this:
public void PickerEmployee_SelectedIndexChanged(object sender, SelectedItemChangedEventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var item = sender as EmployeeList;
var selectedItem = item.uid;
DisplayAlert (selectedItem.ToString (), "OK", "OK");
But its giving me an error that above method has wrong signature.
xamarin.forms picker
I am getting data from a web service and I am loading it in Picker. Now I want to call a new web service to get some data related to selected item. But I am not getting that selected item.
I am using below class model to get data from web service and loading it in Picker.
public class ModelGetEmployeeList
public string ServiceStatus get; set;
public List<EmployeeList> EmpList get; set;
public class EmployeeList
public string uid get; set;
public string fname get; set;
public string lname get; set;
This is how I loaded data in Picker
:
if (response.IsSuccessStatusCode)
var content = await response.Content.ReadAsStringAsync ();
var Items = JsonConvert.DeserializeObject <ModelGetEmployeeList> (content);
foreach(EmployeeList emp in Items.EmpList)
pickerEmployee.Items.Add(emp.uid.ToString()+"-"+emp.fname.ToString()+" "+emp.lname.ToString());
Now I am implementing SelectedIndexChanged
event like this:
public void PickerEmployee_SelectedIndexChanged(object sender, SelectedItemChangedEventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var item = sender as EmployeeList;
var selectedItem = item.uid;
DisplayAlert (selectedItem.ToString (), "OK", "OK");
But its giving me an error that above method has wrong signature.
xamarin.forms picker
xamarin.forms picker
edited Aug 4 '16 at 12:07


Joehl
2,52431538
2,52431538
asked Aug 4 '16 at 9:50
Dipak
3091827
3091827
add a comment |
add a comment |
6 Answers
6
active
oldest
votes
up vote
2
down vote
According to the Xamarin.Forms
Picker documentation SelectedIndexChanged
event is expecting delegate which matches EventHandler
delegate (EventHandler documentation)
So, you have to change signature of your method :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
...
Ohh.. Thanks. This problem is solve but it has occurred new error. Object reference is not set at var selectedItem = item.uid;
– Dipak
Aug 4 '16 at 10:31
add a comment |
up vote
1
down vote
Your signature is wrong.
Also the following code is wrong:
var item = sender as EmployeeList;
var selectedItem = item.uid;
Please find the corrected version below :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = PickerEmployee[SelectedIndex];
DisplayAlert (selectedItem, "OK", "OK");
The Xamarin Forms picker will get you only the string which was added to the list and not the object.
If you need the object either you can use the selectedIdex on your orginal lsit to get the object as :
var selectedEmp = Items.EmpList[SelectedIndex];
Or you can use a Bindable Picker.
Thank you sir. I need to get uid from the selected text. I separated uid and employee name with "dash" so I can get uid without accessing object.
– Dipak
Aug 4 '16 at 11:07
add a comment |
up vote
1
down vote
You can take sellected value with this:
string selectedEmployee = string.Empty;
selectedEmployee = pickerEmployee.Items[pickerEmployee.SelectedIndex];
add a comment |
up vote
0
down vote
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = (EmployeeList)PickerEmployee.SelectedItem;
DisplayAlert (selectedItem.fname, "OK", "OK");
add a comment |
up vote
0
down vote
The Items collection is a list of strings so you can get the currently selected value using SelectedIndex
var selectedValue = picker.Items [picker.SelectedIndex];
If you are using binding then yes, the exposed property is the SelectedIndex.
For more info click here
add a comment |
up vote
0
down vote
public void PickerEmployee_SelectedIndexChanged(object sender, SelectedItemChangedEventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var item = sender as Picker;
var selectedItem = item.SelectedItem as EmployeeList;
var uid =selectedItem.uid;
DisplayAlert (uid .ToString (), "OK", "OK");
New contributor
Mustafa Mohammed Eldkhri is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
According to the Xamarin.Forms
Picker documentation SelectedIndexChanged
event is expecting delegate which matches EventHandler
delegate (EventHandler documentation)
So, you have to change signature of your method :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
...
Ohh.. Thanks. This problem is solve but it has occurred new error. Object reference is not set at var selectedItem = item.uid;
– Dipak
Aug 4 '16 at 10:31
add a comment |
up vote
2
down vote
According to the Xamarin.Forms
Picker documentation SelectedIndexChanged
event is expecting delegate which matches EventHandler
delegate (EventHandler documentation)
So, you have to change signature of your method :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
...
Ohh.. Thanks. This problem is solve but it has occurred new error. Object reference is not set at var selectedItem = item.uid;
– Dipak
Aug 4 '16 at 10:31
add a comment |
up vote
2
down vote
up vote
2
down vote
According to the Xamarin.Forms
Picker documentation SelectedIndexChanged
event is expecting delegate which matches EventHandler
delegate (EventHandler documentation)
So, you have to change signature of your method :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
...
According to the Xamarin.Forms
Picker documentation SelectedIndexChanged
event is expecting delegate which matches EventHandler
delegate (EventHandler documentation)
So, you have to change signature of your method :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
...
answered Aug 4 '16 at 10:19
Arkadiusz K
1,48911416
1,48911416
Ohh.. Thanks. This problem is solve but it has occurred new error. Object reference is not set at var selectedItem = item.uid;
– Dipak
Aug 4 '16 at 10:31
add a comment |
Ohh.. Thanks. This problem is solve but it has occurred new error. Object reference is not set at var selectedItem = item.uid;
– Dipak
Aug 4 '16 at 10:31
Ohh.. Thanks. This problem is solve but it has occurred new error. Object reference is not set at var selectedItem = item.uid;
– Dipak
Aug 4 '16 at 10:31
Ohh.. Thanks. This problem is solve but it has occurred new error. Object reference is not set at var selectedItem = item.uid;
– Dipak
Aug 4 '16 at 10:31
add a comment |
up vote
1
down vote
Your signature is wrong.
Also the following code is wrong:
var item = sender as EmployeeList;
var selectedItem = item.uid;
Please find the corrected version below :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = PickerEmployee[SelectedIndex];
DisplayAlert (selectedItem, "OK", "OK");
The Xamarin Forms picker will get you only the string which was added to the list and not the object.
If you need the object either you can use the selectedIdex on your orginal lsit to get the object as :
var selectedEmp = Items.EmpList[SelectedIndex];
Or you can use a Bindable Picker.
Thank you sir. I need to get uid from the selected text. I separated uid and employee name with "dash" so I can get uid without accessing object.
– Dipak
Aug 4 '16 at 11:07
add a comment |
up vote
1
down vote
Your signature is wrong.
Also the following code is wrong:
var item = sender as EmployeeList;
var selectedItem = item.uid;
Please find the corrected version below :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = PickerEmployee[SelectedIndex];
DisplayAlert (selectedItem, "OK", "OK");
The Xamarin Forms picker will get you only the string which was added to the list and not the object.
If you need the object either you can use the selectedIdex on your orginal lsit to get the object as :
var selectedEmp = Items.EmpList[SelectedIndex];
Or you can use a Bindable Picker.
Thank you sir. I need to get uid from the selected text. I separated uid and employee name with "dash" so I can get uid without accessing object.
– Dipak
Aug 4 '16 at 11:07
add a comment |
up vote
1
down vote
up vote
1
down vote
Your signature is wrong.
Also the following code is wrong:
var item = sender as EmployeeList;
var selectedItem = item.uid;
Please find the corrected version below :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = PickerEmployee[SelectedIndex];
DisplayAlert (selectedItem, "OK", "OK");
The Xamarin Forms picker will get you only the string which was added to the list and not the object.
If you need the object either you can use the selectedIdex on your orginal lsit to get the object as :
var selectedEmp = Items.EmpList[SelectedIndex];
Or you can use a Bindable Picker.
Your signature is wrong.
Also the following code is wrong:
var item = sender as EmployeeList;
var selectedItem = item.uid;
Please find the corrected version below :
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = PickerEmployee[SelectedIndex];
DisplayAlert (selectedItem, "OK", "OK");
The Xamarin Forms picker will get you only the string which was added to the list and not the object.
If you need the object either you can use the selectedIdex on your orginal lsit to get the object as :
var selectedEmp = Items.EmpList[SelectedIndex];
Or you can use a Bindable Picker.
answered Aug 4 '16 at 10:46
Rohit Vipin Mathews
7,755104089
7,755104089
Thank you sir. I need to get uid from the selected text. I separated uid and employee name with "dash" so I can get uid without accessing object.
– Dipak
Aug 4 '16 at 11:07
add a comment |
Thank you sir. I need to get uid from the selected text. I separated uid and employee name with "dash" so I can get uid without accessing object.
– Dipak
Aug 4 '16 at 11:07
Thank you sir. I need to get uid from the selected text. I separated uid and employee name with "dash" so I can get uid without accessing object.
– Dipak
Aug 4 '16 at 11:07
Thank you sir. I need to get uid from the selected text. I separated uid and employee name with "dash" so I can get uid without accessing object.
– Dipak
Aug 4 '16 at 11:07
add a comment |
up vote
1
down vote
You can take sellected value with this:
string selectedEmployee = string.Empty;
selectedEmployee = pickerEmployee.Items[pickerEmployee.SelectedIndex];
add a comment |
up vote
1
down vote
You can take sellected value with this:
string selectedEmployee = string.Empty;
selectedEmployee = pickerEmployee.Items[pickerEmployee.SelectedIndex];
add a comment |
up vote
1
down vote
up vote
1
down vote
You can take sellected value with this:
string selectedEmployee = string.Empty;
selectedEmployee = pickerEmployee.Items[pickerEmployee.SelectedIndex];
You can take sellected value with this:
string selectedEmployee = string.Empty;
selectedEmployee = pickerEmployee.Items[pickerEmployee.SelectedIndex];
edited Jan 6 at 19:41


Diego Venâncio
1,3351129
1,3351129
answered Aug 4 '16 at 13:02
Burak Tokmak
111
111
add a comment |
add a comment |
up vote
0
down vote
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = (EmployeeList)PickerEmployee.SelectedItem;
DisplayAlert (selectedItem.fname, "OK", "OK");
add a comment |
up vote
0
down vote
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = (EmployeeList)PickerEmployee.SelectedItem;
DisplayAlert (selectedItem.fname, "OK", "OK");
add a comment |
up vote
0
down vote
up vote
0
down vote
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = (EmployeeList)PickerEmployee.SelectedItem;
DisplayAlert (selectedItem.fname, "OK", "OK");
public void PickerEmployee_SelectedIndexChanged(object sender, EventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var selectedItem = (EmployeeList)PickerEmployee.SelectedItem;
DisplayAlert (selectedItem.fname, "OK", "OK");
edited Jul 14 '17 at 22:15
marcadian
2,458817
2,458817
answered Jul 14 '17 at 21:56
José Miguel Sagastume
11
11
add a comment |
add a comment |
up vote
0
down vote
The Items collection is a list of strings so you can get the currently selected value using SelectedIndex
var selectedValue = picker.Items [picker.SelectedIndex];
If you are using binding then yes, the exposed property is the SelectedIndex.
For more info click here
add a comment |
up vote
0
down vote
The Items collection is a list of strings so you can get the currently selected value using SelectedIndex
var selectedValue = picker.Items [picker.SelectedIndex];
If you are using binding then yes, the exposed property is the SelectedIndex.
For more info click here
add a comment |
up vote
0
down vote
up vote
0
down vote
The Items collection is a list of strings so you can get the currently selected value using SelectedIndex
var selectedValue = picker.Items [picker.SelectedIndex];
If you are using binding then yes, the exposed property is the SelectedIndex.
For more info click here
The Items collection is a list of strings so you can get the currently selected value using SelectedIndex
var selectedValue = picker.Items [picker.SelectedIndex];
If you are using binding then yes, the exposed property is the SelectedIndex.
For more info click here
answered Jul 15 '17 at 16:04


Venkata Swamy Balaraju
828311
828311
add a comment |
add a comment |
up vote
0
down vote
public void PickerEmployee_SelectedIndexChanged(object sender, SelectedItemChangedEventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var item = sender as Picker;
var selectedItem = item.SelectedItem as EmployeeList;
var uid =selectedItem.uid;
DisplayAlert (uid .ToString (), "OK", "OK");
New contributor
Mustafa Mohammed Eldkhri is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
public void PickerEmployee_SelectedIndexChanged(object sender, SelectedItemChangedEventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var item = sender as Picker;
var selectedItem = item.SelectedItem as EmployeeList;
var uid =selectedItem.uid;
DisplayAlert (uid .ToString (), "OK", "OK");
New contributor
Mustafa Mohammed Eldkhri is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
public void PickerEmployee_SelectedIndexChanged(object sender, SelectedItemChangedEventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var item = sender as Picker;
var selectedItem = item.SelectedItem as EmployeeList;
var uid =selectedItem.uid;
DisplayAlert (uid .ToString (), "OK", "OK");
New contributor
Mustafa Mohammed Eldkhri is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
public void PickerEmployee_SelectedIndexChanged(object sender, SelectedItemChangedEventArgs e)
if (pickerEmployee.SelectedIndex == -1)
//Message
else
var item = sender as Picker;
var selectedItem = item.SelectedItem as EmployeeList;
var uid =selectedItem.uid;
DisplayAlert (uid .ToString (), "OK", "OK");
New contributor
Mustafa Mohammed Eldkhri is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Mustafa Mohammed Eldkhri is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 2 days ago


Mustafa Mohammed Eldkhri
161
161
New contributor
Mustafa Mohammed Eldkhri is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Mustafa Mohammed Eldkhri is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Mustafa Mohammed Eldkhri is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f38764006%2fgetting-selected-value-from-picker-in-xamarin%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
DR r0P1,eLcqnmyNM0KlgKS7pAdvBJ WvCHIXiQLHv 2wr,i6r fc8DH