Converting binary to decimal only using basic functions len(), ord(), print(), etc
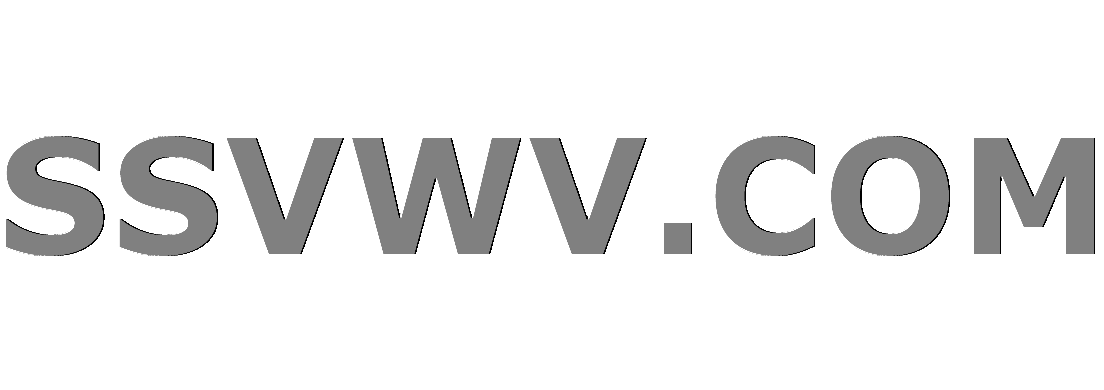
Multi tool use
up vote
0
down vote
favorite
I'm trying to create a scientific calculator for an assignment. I'm looking for a bit of help with python syntax, and I think my design and pseudo code are doing well, but for whatever reason python isn't having any of my syntactical issues. Here is the code I have for converting binary to decimal.
I need the code to reprompt when the input is invalid, but when it does reprompt, it gets stuck in a loop of reprompting and won't give me any way out.
def bintodec(var):
power = (len(var) + 1)
value = ' '
while True:
var = input('Give a number to convert from binary to decimal: ')
for x in range(len(var)):
if (ord(var[x]) == 49):
power -= 1
value += x * (2 ** power)
if (ord(var[x]) == 48):
power -= 1
value += x * (2 ** power)
if power == -1:
break
else:
boo = True
return value
Any help is greatly appreciated!
python binary decimal
add a comment |
up vote
0
down vote
favorite
I'm trying to create a scientific calculator for an assignment. I'm looking for a bit of help with python syntax, and I think my design and pseudo code are doing well, but for whatever reason python isn't having any of my syntactical issues. Here is the code I have for converting binary to decimal.
I need the code to reprompt when the input is invalid, but when it does reprompt, it gets stuck in a loop of reprompting and won't give me any way out.
def bintodec(var):
power = (len(var) + 1)
value = ' '
while True:
var = input('Give a number to convert from binary to decimal: ')
for x in range(len(var)):
if (ord(var[x]) == 49):
power -= 1
value += x * (2 ** power)
if (ord(var[x]) == 48):
power -= 1
value += x * (2 ** power)
if power == -1:
break
else:
boo = True
return value
Any help is greatly appreciated!
python binary decimal
Thebreak
statement only exits the innermost loop. See the duplicate (link above) for ways to refactor your code to break out of the outer loop.
– Jim Stewart
Nov 12 at 1:08
1
Do you actually have to useord
here... The code would be much more readable if you just dofor x in var: if x == '0'
.... etc... Also you're doing the same operations for0
and1
so you might as well put those in the same block
– Jon Clements♦
Nov 12 at 1:09
The answers to the question Asking the user for input until they give a valid response should help with getting input from the user.
– martineau
Nov 12 at 2:32
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to create a scientific calculator for an assignment. I'm looking for a bit of help with python syntax, and I think my design and pseudo code are doing well, but for whatever reason python isn't having any of my syntactical issues. Here is the code I have for converting binary to decimal.
I need the code to reprompt when the input is invalid, but when it does reprompt, it gets stuck in a loop of reprompting and won't give me any way out.
def bintodec(var):
power = (len(var) + 1)
value = ' '
while True:
var = input('Give a number to convert from binary to decimal: ')
for x in range(len(var)):
if (ord(var[x]) == 49):
power -= 1
value += x * (2 ** power)
if (ord(var[x]) == 48):
power -= 1
value += x * (2 ** power)
if power == -1:
break
else:
boo = True
return value
Any help is greatly appreciated!
python binary decimal
I'm trying to create a scientific calculator for an assignment. I'm looking for a bit of help with python syntax, and I think my design and pseudo code are doing well, but for whatever reason python isn't having any of my syntactical issues. Here is the code I have for converting binary to decimal.
I need the code to reprompt when the input is invalid, but when it does reprompt, it gets stuck in a loop of reprompting and won't give me any way out.
def bintodec(var):
power = (len(var) + 1)
value = ' '
while True:
var = input('Give a number to convert from binary to decimal: ')
for x in range(len(var)):
if (ord(var[x]) == 49):
power -= 1
value += x * (2 ** power)
if (ord(var[x]) == 48):
power -= 1
value += x * (2 ** power)
if power == -1:
break
else:
boo = True
return value
Any help is greatly appreciated!
python binary decimal
python binary decimal
edited Nov 12 at 1:13
cricket_007
78.5k1142109
78.5k1142109
asked Nov 12 at 1:05


Jay F.
31
31
Thebreak
statement only exits the innermost loop. See the duplicate (link above) for ways to refactor your code to break out of the outer loop.
– Jim Stewart
Nov 12 at 1:08
1
Do you actually have to useord
here... The code would be much more readable if you just dofor x in var: if x == '0'
.... etc... Also you're doing the same operations for0
and1
so you might as well put those in the same block
– Jon Clements♦
Nov 12 at 1:09
The answers to the question Asking the user for input until they give a valid response should help with getting input from the user.
– martineau
Nov 12 at 2:32
add a comment |
Thebreak
statement only exits the innermost loop. See the duplicate (link above) for ways to refactor your code to break out of the outer loop.
– Jim Stewart
Nov 12 at 1:08
1
Do you actually have to useord
here... The code would be much more readable if you just dofor x in var: if x == '0'
.... etc... Also you're doing the same operations for0
and1
so you might as well put those in the same block
– Jon Clements♦
Nov 12 at 1:09
The answers to the question Asking the user for input until they give a valid response should help with getting input from the user.
– martineau
Nov 12 at 2:32
The
break
statement only exits the innermost loop. See the duplicate (link above) for ways to refactor your code to break out of the outer loop.– Jim Stewart
Nov 12 at 1:08
The
break
statement only exits the innermost loop. See the duplicate (link above) for ways to refactor your code to break out of the outer loop.– Jim Stewart
Nov 12 at 1:08
1
1
Do you actually have to use
ord
here... The code would be much more readable if you just do for x in var: if x == '0'
.... etc... Also you're doing the same operations for 0
and 1
so you might as well put those in the same block– Jon Clements♦
Nov 12 at 1:09
Do you actually have to use
ord
here... The code would be much more readable if you just do for x in var: if x == '0'
.... etc... Also you're doing the same operations for 0
and 1
so you might as well put those in the same block– Jon Clements♦
Nov 12 at 1:09
The answers to the question Asking the user for input until they give a valid response should help with getting input from the user.
– martineau
Nov 12 at 2:32
The answers to the question Asking the user for input until they give a valid response should help with getting input from the user.
– martineau
Nov 12 at 2:32
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
This is pretty classic. Reading in a base is easier than writing in one.
def bintodec(var):
assert set(var) <= set("01") # Just check that we only have 0s and 1s
assert isinstance(var, str) # Checks that var is a string
result = 0
for character in var: # character will be each character of var, from left to rigth
digitvalue = ord(character) - ord("0")
result *= 2
result += digitvalue
return result
Ok, how does it work ?
Well, it reads the value from left to right. digitvalue
will contain 1
or 0
. For each digit we read, if it is 0
, there is nothing to add the result (so result += digitvalue
adds indeed 0
), but we still need take into account that there is one more 0
at the end of the number.
Now, in base 10, adding a zero to the end makes a number 10 times as big. This is the same in base 2. Adding a zero at the end makes a number twice as big. This is why we multiply it by 2.
Finally, if digitvalue
is 1
instead of 0
, we need to add 1
to the number and result += digitvalue
does it.
Note: Just for things to be clear, the two for loops below are equivalent.
for character in var:
pass # pass does nothing
for i in range(len(var)):
character = var[i]
pass
@JayF.:
Is there any way to reprompt without using assert?
I suppose you want to reprompt if the input is incorrect. You need to use a loop for that:
while True:
var = input()
if set(var) <= set("01"):
print(bintodec(var))
break # Remove this `break` statement if you want to reprompt forever
else:
# print("The input must consist of only 0s and 1s.")
pass # `pass` does nothing.
If you leave the asserts in the bintodec
function, it can be done in a more pythonic way, using exception handling:
while True:
var = input()
try:
print(bintodec(var))
break
except AssertionError:
print("The input must consist of only 0s and 1s.")
Is there any way to reprompt without using assert? Thanks for your help!
– Jay F.
Nov 12 at 21:20
If the answer satisfies you, you can mark it as accepted, or otherwise, append an edit to your question or interact through comments.
– Mathieu CAROFF
Nov 16 at 20:06
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254810%2fconverting-binary-to-decimal-only-using-basic-functions-len-ord-print-e%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
This is pretty classic. Reading in a base is easier than writing in one.
def bintodec(var):
assert set(var) <= set("01") # Just check that we only have 0s and 1s
assert isinstance(var, str) # Checks that var is a string
result = 0
for character in var: # character will be each character of var, from left to rigth
digitvalue = ord(character) - ord("0")
result *= 2
result += digitvalue
return result
Ok, how does it work ?
Well, it reads the value from left to right. digitvalue
will contain 1
or 0
. For each digit we read, if it is 0
, there is nothing to add the result (so result += digitvalue
adds indeed 0
), but we still need take into account that there is one more 0
at the end of the number.
Now, in base 10, adding a zero to the end makes a number 10 times as big. This is the same in base 2. Adding a zero at the end makes a number twice as big. This is why we multiply it by 2.
Finally, if digitvalue
is 1
instead of 0
, we need to add 1
to the number and result += digitvalue
does it.
Note: Just for things to be clear, the two for loops below are equivalent.
for character in var:
pass # pass does nothing
for i in range(len(var)):
character = var[i]
pass
@JayF.:
Is there any way to reprompt without using assert?
I suppose you want to reprompt if the input is incorrect. You need to use a loop for that:
while True:
var = input()
if set(var) <= set("01"):
print(bintodec(var))
break # Remove this `break` statement if you want to reprompt forever
else:
# print("The input must consist of only 0s and 1s.")
pass # `pass` does nothing.
If you leave the asserts in the bintodec
function, it can be done in a more pythonic way, using exception handling:
while True:
var = input()
try:
print(bintodec(var))
break
except AssertionError:
print("The input must consist of only 0s and 1s.")
Is there any way to reprompt without using assert? Thanks for your help!
– Jay F.
Nov 12 at 21:20
If the answer satisfies you, you can mark it as accepted, or otherwise, append an edit to your question or interact through comments.
– Mathieu CAROFF
Nov 16 at 20:06
add a comment |
up vote
0
down vote
accepted
This is pretty classic. Reading in a base is easier than writing in one.
def bintodec(var):
assert set(var) <= set("01") # Just check that we only have 0s and 1s
assert isinstance(var, str) # Checks that var is a string
result = 0
for character in var: # character will be each character of var, from left to rigth
digitvalue = ord(character) - ord("0")
result *= 2
result += digitvalue
return result
Ok, how does it work ?
Well, it reads the value from left to right. digitvalue
will contain 1
or 0
. For each digit we read, if it is 0
, there is nothing to add the result (so result += digitvalue
adds indeed 0
), but we still need take into account that there is one more 0
at the end of the number.
Now, in base 10, adding a zero to the end makes a number 10 times as big. This is the same in base 2. Adding a zero at the end makes a number twice as big. This is why we multiply it by 2.
Finally, if digitvalue
is 1
instead of 0
, we need to add 1
to the number and result += digitvalue
does it.
Note: Just for things to be clear, the two for loops below are equivalent.
for character in var:
pass # pass does nothing
for i in range(len(var)):
character = var[i]
pass
@JayF.:
Is there any way to reprompt without using assert?
I suppose you want to reprompt if the input is incorrect. You need to use a loop for that:
while True:
var = input()
if set(var) <= set("01"):
print(bintodec(var))
break # Remove this `break` statement if you want to reprompt forever
else:
# print("The input must consist of only 0s and 1s.")
pass # `pass` does nothing.
If you leave the asserts in the bintodec
function, it can be done in a more pythonic way, using exception handling:
while True:
var = input()
try:
print(bintodec(var))
break
except AssertionError:
print("The input must consist of only 0s and 1s.")
Is there any way to reprompt without using assert? Thanks for your help!
– Jay F.
Nov 12 at 21:20
If the answer satisfies you, you can mark it as accepted, or otherwise, append an edit to your question or interact through comments.
– Mathieu CAROFF
Nov 16 at 20:06
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
This is pretty classic. Reading in a base is easier than writing in one.
def bintodec(var):
assert set(var) <= set("01") # Just check that we only have 0s and 1s
assert isinstance(var, str) # Checks that var is a string
result = 0
for character in var: # character will be each character of var, from left to rigth
digitvalue = ord(character) - ord("0")
result *= 2
result += digitvalue
return result
Ok, how does it work ?
Well, it reads the value from left to right. digitvalue
will contain 1
or 0
. For each digit we read, if it is 0
, there is nothing to add the result (so result += digitvalue
adds indeed 0
), but we still need take into account that there is one more 0
at the end of the number.
Now, in base 10, adding a zero to the end makes a number 10 times as big. This is the same in base 2. Adding a zero at the end makes a number twice as big. This is why we multiply it by 2.
Finally, if digitvalue
is 1
instead of 0
, we need to add 1
to the number and result += digitvalue
does it.
Note: Just for things to be clear, the two for loops below are equivalent.
for character in var:
pass # pass does nothing
for i in range(len(var)):
character = var[i]
pass
@JayF.:
Is there any way to reprompt without using assert?
I suppose you want to reprompt if the input is incorrect. You need to use a loop for that:
while True:
var = input()
if set(var) <= set("01"):
print(bintodec(var))
break # Remove this `break` statement if you want to reprompt forever
else:
# print("The input must consist of only 0s and 1s.")
pass # `pass` does nothing.
If you leave the asserts in the bintodec
function, it can be done in a more pythonic way, using exception handling:
while True:
var = input()
try:
print(bintodec(var))
break
except AssertionError:
print("The input must consist of only 0s and 1s.")
This is pretty classic. Reading in a base is easier than writing in one.
def bintodec(var):
assert set(var) <= set("01") # Just check that we only have 0s and 1s
assert isinstance(var, str) # Checks that var is a string
result = 0
for character in var: # character will be each character of var, from left to rigth
digitvalue = ord(character) - ord("0")
result *= 2
result += digitvalue
return result
Ok, how does it work ?
Well, it reads the value from left to right. digitvalue
will contain 1
or 0
. For each digit we read, if it is 0
, there is nothing to add the result (so result += digitvalue
adds indeed 0
), but we still need take into account that there is one more 0
at the end of the number.
Now, in base 10, adding a zero to the end makes a number 10 times as big. This is the same in base 2. Adding a zero at the end makes a number twice as big. This is why we multiply it by 2.
Finally, if digitvalue
is 1
instead of 0
, we need to add 1
to the number and result += digitvalue
does it.
Note: Just for things to be clear, the two for loops below are equivalent.
for character in var:
pass # pass does nothing
for i in range(len(var)):
character = var[i]
pass
@JayF.:
Is there any way to reprompt without using assert?
I suppose you want to reprompt if the input is incorrect. You need to use a loop for that:
while True:
var = input()
if set(var) <= set("01"):
print(bintodec(var))
break # Remove this `break` statement if you want to reprompt forever
else:
# print("The input must consist of only 0s and 1s.")
pass # `pass` does nothing.
If you leave the asserts in the bintodec
function, it can be done in a more pythonic way, using exception handling:
while True:
var = input()
try:
print(bintodec(var))
break
except AssertionError:
print("The input must consist of only 0s and 1s.")
edited Nov 13 at 13:29


loxaxs
401517
401517
answered Nov 12 at 2:48
Mathieu CAROFF
3467
3467
Is there any way to reprompt without using assert? Thanks for your help!
– Jay F.
Nov 12 at 21:20
If the answer satisfies you, you can mark it as accepted, or otherwise, append an edit to your question or interact through comments.
– Mathieu CAROFF
Nov 16 at 20:06
add a comment |
Is there any way to reprompt without using assert? Thanks for your help!
– Jay F.
Nov 12 at 21:20
If the answer satisfies you, you can mark it as accepted, or otherwise, append an edit to your question or interact through comments.
– Mathieu CAROFF
Nov 16 at 20:06
Is there any way to reprompt without using assert? Thanks for your help!
– Jay F.
Nov 12 at 21:20
Is there any way to reprompt without using assert? Thanks for your help!
– Jay F.
Nov 12 at 21:20
If the answer satisfies you, you can mark it as accepted, or otherwise, append an edit to your question or interact through comments.
– Mathieu CAROFF
Nov 16 at 20:06
If the answer satisfies you, you can mark it as accepted, or otherwise, append an edit to your question or interact through comments.
– Mathieu CAROFF
Nov 16 at 20:06
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254810%2fconverting-binary-to-decimal-only-using-basic-functions-len-ord-print-e%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ExN0N CSPaSQhK ZwI VrWO86hVkNOa,GLmAy,cZRYS3A6VPV,ecbzy1wz,j9YLSYE02nsaHUB0FYF ue6Uta,7bc5 6LIKd sd,C
The
break
statement only exits the innermost loop. See the duplicate (link above) for ways to refactor your code to break out of the outer loop.– Jim Stewart
Nov 12 at 1:08
1
Do you actually have to use
ord
here... The code would be much more readable if you just dofor x in var: if x == '0'
.... etc... Also you're doing the same operations for0
and1
so you might as well put those in the same block– Jon Clements♦
Nov 12 at 1:09
The answers to the question Asking the user for input until they give a valid response should help with getting input from the user.
– martineau
Nov 12 at 2:32