Component not being updated after state is changed in reducer
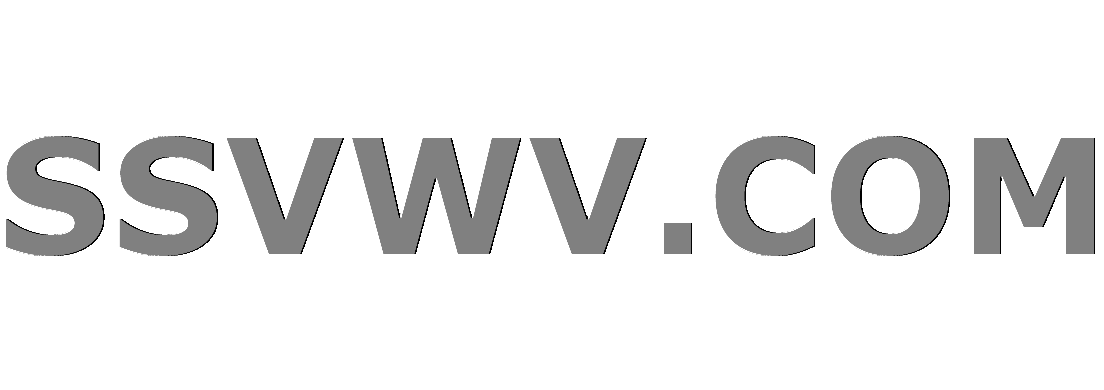
Multi tool use
I would like to display the error to my user. An error is thrown and the error and message are updated in the reducer by the action. But for some reason I can't get the error or message to display for the user. Is there something wrong with the reducer or mapStateToProps? How does the component know the state has been updated? I'm not sure how to update that.....
What am I missing?
reducer:
import CREATE_VEHICLE,VEHICLE_ERROR,
VEHICLE_FORM_PAGE_SUBMIT,FETCH_VEHICLES_SUCCESS from '../actions/vehicles';
const initialState=message: null, error: null, vehicle:;
export default function vehicleReducer(state=initialState, action)
console.log("in reducer");
switch(action.type)
case CREATE_VEHICLE:
return [...state, Object.assign(, action.vehicle, action.message)];
case VEHICLE_ERROR:
return
...state,
error: action.error,
message: action.message
;
default:
return state;
actions:
export const vehicleError = (error, msg) =>
return
type: VEHICLE_ERROR,
error:error,
message: msg
;
export const createVehicle=(vehicle) =>
console.log("vehicle: ", vehicle);
return (dispatch) =>
return axios.post(`http://localhost:9081/api/bmwvehicle/create`,
vehicle)
.then((response) =>
if (response.ok)
console.log("success");
dispatch(createVehicleSuccess(response.data))
, (error) =>
if (error.response.status == 500)
dispatch(vehicleError(error.message, "Could not add vehicle,
please try again."));
);
;;
component:
class Vehicle extends React.Component
constructor(props)
super(props);
submitVehicle(input)
this.props.createVehicle(input);
render()
console.log("the error is: ",this.props.error);
return(
<div>
<AddVehicle submitVehicle=
this.submitVehicle.bind(this) />
</div>
)
const mapStateToProps=(state, ownProps) =>
return
vehicle: state.vehicle,
message: state.message,
items: state.items,
vehicles: state.vehicles,
error: state.error
;
const mapDispatchToProps=(dispatch)=>
return
createVehicle: vehicle =>
dispatch(vehicleActions.createVehicle(vehicle))
;
export default connect(mapStateToProps, mapDispatchToProps)(Vehicle);
reactjs redux react-redux react-thunk
add a comment |
I would like to display the error to my user. An error is thrown and the error and message are updated in the reducer by the action. But for some reason I can't get the error or message to display for the user. Is there something wrong with the reducer or mapStateToProps? How does the component know the state has been updated? I'm not sure how to update that.....
What am I missing?
reducer:
import CREATE_VEHICLE,VEHICLE_ERROR,
VEHICLE_FORM_PAGE_SUBMIT,FETCH_VEHICLES_SUCCESS from '../actions/vehicles';
const initialState=message: null, error: null, vehicle:;
export default function vehicleReducer(state=initialState, action)
console.log("in reducer");
switch(action.type)
case CREATE_VEHICLE:
return [...state, Object.assign(, action.vehicle, action.message)];
case VEHICLE_ERROR:
return
...state,
error: action.error,
message: action.message
;
default:
return state;
actions:
export const vehicleError = (error, msg) =>
return
type: VEHICLE_ERROR,
error:error,
message: msg
;
export const createVehicle=(vehicle) =>
console.log("vehicle: ", vehicle);
return (dispatch) =>
return axios.post(`http://localhost:9081/api/bmwvehicle/create`,
vehicle)
.then((response) =>
if (response.ok)
console.log("success");
dispatch(createVehicleSuccess(response.data))
, (error) =>
if (error.response.status == 500)
dispatch(vehicleError(error.message, "Could not add vehicle,
please try again."));
);
;;
component:
class Vehicle extends React.Component
constructor(props)
super(props);
submitVehicle(input)
this.props.createVehicle(input);
render()
console.log("the error is: ",this.props.error);
return(
<div>
<AddVehicle submitVehicle=
this.submitVehicle.bind(this) />
</div>
)
const mapStateToProps=(state, ownProps) =>
return
vehicle: state.vehicle,
message: state.message,
items: state.items,
vehicles: state.vehicles,
error: state.error
;
const mapDispatchToProps=(dispatch)=>
return
createVehicle: vehicle =>
dispatch(vehicleActions.createVehicle(vehicle))
;
export default connect(mapStateToProps, mapDispatchToProps)(Vehicle);
reactjs redux react-redux react-thunk
It's great that you have included most of the relevant code, but I recommend also creating a minimal code sandbox (codesandbox.io) that is actually a working react app. Instead of the api call, just have two buttons, one to dispatch the createVehicleSuccess action with hard-coded data and one to dispatch vehicleError with hard-coded error info. Then you can point to this in your post and someone can help point out/fix the error much more quickly.
– Ryan Cogswell
Nov 14 '18 at 21:59
I think your problem is that if the response status code is not 500 you discard the error. I think it's better to call vehicleError if there's error.
– boaz_shuster
Nov 14 '18 at 22:01
add a comment |
I would like to display the error to my user. An error is thrown and the error and message are updated in the reducer by the action. But for some reason I can't get the error or message to display for the user. Is there something wrong with the reducer or mapStateToProps? How does the component know the state has been updated? I'm not sure how to update that.....
What am I missing?
reducer:
import CREATE_VEHICLE,VEHICLE_ERROR,
VEHICLE_FORM_PAGE_SUBMIT,FETCH_VEHICLES_SUCCESS from '../actions/vehicles';
const initialState=message: null, error: null, vehicle:;
export default function vehicleReducer(state=initialState, action)
console.log("in reducer");
switch(action.type)
case CREATE_VEHICLE:
return [...state, Object.assign(, action.vehicle, action.message)];
case VEHICLE_ERROR:
return
...state,
error: action.error,
message: action.message
;
default:
return state;
actions:
export const vehicleError = (error, msg) =>
return
type: VEHICLE_ERROR,
error:error,
message: msg
;
export const createVehicle=(vehicle) =>
console.log("vehicle: ", vehicle);
return (dispatch) =>
return axios.post(`http://localhost:9081/api/bmwvehicle/create`,
vehicle)
.then((response) =>
if (response.ok)
console.log("success");
dispatch(createVehicleSuccess(response.data))
, (error) =>
if (error.response.status == 500)
dispatch(vehicleError(error.message, "Could not add vehicle,
please try again."));
);
;;
component:
class Vehicle extends React.Component
constructor(props)
super(props);
submitVehicle(input)
this.props.createVehicle(input);
render()
console.log("the error is: ",this.props.error);
return(
<div>
<AddVehicle submitVehicle=
this.submitVehicle.bind(this) />
</div>
)
const mapStateToProps=(state, ownProps) =>
return
vehicle: state.vehicle,
message: state.message,
items: state.items,
vehicles: state.vehicles,
error: state.error
;
const mapDispatchToProps=(dispatch)=>
return
createVehicle: vehicle =>
dispatch(vehicleActions.createVehicle(vehicle))
;
export default connect(mapStateToProps, mapDispatchToProps)(Vehicle);
reactjs redux react-redux react-thunk
I would like to display the error to my user. An error is thrown and the error and message are updated in the reducer by the action. But for some reason I can't get the error or message to display for the user. Is there something wrong with the reducer or mapStateToProps? How does the component know the state has been updated? I'm not sure how to update that.....
What am I missing?
reducer:
import CREATE_VEHICLE,VEHICLE_ERROR,
VEHICLE_FORM_PAGE_SUBMIT,FETCH_VEHICLES_SUCCESS from '../actions/vehicles';
const initialState=message: null, error: null, vehicle:;
export default function vehicleReducer(state=initialState, action)
console.log("in reducer");
switch(action.type)
case CREATE_VEHICLE:
return [...state, Object.assign(, action.vehicle, action.message)];
case VEHICLE_ERROR:
return
...state,
error: action.error,
message: action.message
;
default:
return state;
actions:
export const vehicleError = (error, msg) =>
return
type: VEHICLE_ERROR,
error:error,
message: msg
;
export const createVehicle=(vehicle) =>
console.log("vehicle: ", vehicle);
return (dispatch) =>
return axios.post(`http://localhost:9081/api/bmwvehicle/create`,
vehicle)
.then((response) =>
if (response.ok)
console.log("success");
dispatch(createVehicleSuccess(response.data))
, (error) =>
if (error.response.status == 500)
dispatch(vehicleError(error.message, "Could not add vehicle,
please try again."));
);
;;
component:
class Vehicle extends React.Component
constructor(props)
super(props);
submitVehicle(input)
this.props.createVehicle(input);
render()
console.log("the error is: ",this.props.error);
return(
<div>
<AddVehicle submitVehicle=
this.submitVehicle.bind(this) />
</div>
)
const mapStateToProps=(state, ownProps) =>
return
vehicle: state.vehicle,
message: state.message,
items: state.items,
vehicles: state.vehicles,
error: state.error
;
const mapDispatchToProps=(dispatch)=>
return
createVehicle: vehicle =>
dispatch(vehicleActions.createVehicle(vehicle))
;
export default connect(mapStateToProps, mapDispatchToProps)(Vehicle);
reactjs redux react-redux react-thunk
reactjs redux react-redux react-thunk
asked Nov 14 '18 at 17:52
RoroRoro
557
557
It's great that you have included most of the relevant code, but I recommend also creating a minimal code sandbox (codesandbox.io) that is actually a working react app. Instead of the api call, just have two buttons, one to dispatch the createVehicleSuccess action with hard-coded data and one to dispatch vehicleError with hard-coded error info. Then you can point to this in your post and someone can help point out/fix the error much more quickly.
– Ryan Cogswell
Nov 14 '18 at 21:59
I think your problem is that if the response status code is not 500 you discard the error. I think it's better to call vehicleError if there's error.
– boaz_shuster
Nov 14 '18 at 22:01
add a comment |
It's great that you have included most of the relevant code, but I recommend also creating a minimal code sandbox (codesandbox.io) that is actually a working react app. Instead of the api call, just have two buttons, one to dispatch the createVehicleSuccess action with hard-coded data and one to dispatch vehicleError with hard-coded error info. Then you can point to this in your post and someone can help point out/fix the error much more quickly.
– Ryan Cogswell
Nov 14 '18 at 21:59
I think your problem is that if the response status code is not 500 you discard the error. I think it's better to call vehicleError if there's error.
– boaz_shuster
Nov 14 '18 at 22:01
It's great that you have included most of the relevant code, but I recommend also creating a minimal code sandbox (codesandbox.io) that is actually a working react app. Instead of the api call, just have two buttons, one to dispatch the createVehicleSuccess action with hard-coded data and one to dispatch vehicleError with hard-coded error info. Then you can point to this in your post and someone can help point out/fix the error much more quickly.
– Ryan Cogswell
Nov 14 '18 at 21:59
It's great that you have included most of the relevant code, but I recommend also creating a minimal code sandbox (codesandbox.io) that is actually a working react app. Instead of the api call, just have two buttons, one to dispatch the createVehicleSuccess action with hard-coded data and one to dispatch vehicleError with hard-coded error info. Then you can point to this in your post and someone can help point out/fix the error much more quickly.
– Ryan Cogswell
Nov 14 '18 at 21:59
I think your problem is that if the response status code is not 500 you discard the error. I think it's better to call vehicleError if there's error.
– boaz_shuster
Nov 14 '18 at 22:01
I think your problem is that if the response status code is not 500 you discard the error. I think it's better to call vehicleError if there's error.
– boaz_shuster
Nov 14 '18 at 22:01
add a comment |
1 Answer
1
active
oldest
votes
In your CREATE_VEHICLE action, i think you meant to return the state with curly braces instead? ...state, Object.assign(, action.vehicle, action.message)
In your mapStateToProps, you attempt to access state.vehicles
and state.items
, but it does not exist in your default initial state const initialState=message: null, error: null, vehicle:
.
To answer your question about how does the component know the (redux) state has been updated, the component knows because you wrapped it in the Redux HoC called connect() which will notify your component via an update anytime the mapped redux state changes. In your case, the component will be updated on changes to the redux store's state.vehicle, state.message, state.items, state.vehicles and state.error.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306125%2fcomponent-not-being-updated-after-state-is-changed-in-reducer%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In your CREATE_VEHICLE action, i think you meant to return the state with curly braces instead? ...state, Object.assign(, action.vehicle, action.message)
In your mapStateToProps, you attempt to access state.vehicles
and state.items
, but it does not exist in your default initial state const initialState=message: null, error: null, vehicle:
.
To answer your question about how does the component know the (redux) state has been updated, the component knows because you wrapped it in the Redux HoC called connect() which will notify your component via an update anytime the mapped redux state changes. In your case, the component will be updated on changes to the redux store's state.vehicle, state.message, state.items, state.vehicles and state.error.
add a comment |
In your CREATE_VEHICLE action, i think you meant to return the state with curly braces instead? ...state, Object.assign(, action.vehicle, action.message)
In your mapStateToProps, you attempt to access state.vehicles
and state.items
, but it does not exist in your default initial state const initialState=message: null, error: null, vehicle:
.
To answer your question about how does the component know the (redux) state has been updated, the component knows because you wrapped it in the Redux HoC called connect() which will notify your component via an update anytime the mapped redux state changes. In your case, the component will be updated on changes to the redux store's state.vehicle, state.message, state.items, state.vehicles and state.error.
add a comment |
In your CREATE_VEHICLE action, i think you meant to return the state with curly braces instead? ...state, Object.assign(, action.vehicle, action.message)
In your mapStateToProps, you attempt to access state.vehicles
and state.items
, but it does not exist in your default initial state const initialState=message: null, error: null, vehicle:
.
To answer your question about how does the component know the (redux) state has been updated, the component knows because you wrapped it in the Redux HoC called connect() which will notify your component via an update anytime the mapped redux state changes. In your case, the component will be updated on changes to the redux store's state.vehicle, state.message, state.items, state.vehicles and state.error.
In your CREATE_VEHICLE action, i think you meant to return the state with curly braces instead? ...state, Object.assign(, action.vehicle, action.message)
In your mapStateToProps, you attempt to access state.vehicles
and state.items
, but it does not exist in your default initial state const initialState=message: null, error: null, vehicle:
.
To answer your question about how does the component know the (redux) state has been updated, the component knows because you wrapped it in the Redux HoC called connect() which will notify your component via an update anytime the mapped redux state changes. In your case, the component will be updated on changes to the redux store's state.vehicle, state.message, state.items, state.vehicles and state.error.
answered Nov 15 '18 at 1:11


Shawn AndrewsShawn Andrews
965617
965617
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306125%2fcomponent-not-being-updated-after-state-is-changed-in-reducer%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DRT SLmT,Afyis5wflVtrP,CRy9wHI45UXEMTSz,QLguKzJefg 2a5yps3vwVEway3MLUD4i oKV010PbrlOVBb10fKN9iQGxgVb0
It's great that you have included most of the relevant code, but I recommend also creating a minimal code sandbox (codesandbox.io) that is actually a working react app. Instead of the api call, just have two buttons, one to dispatch the createVehicleSuccess action with hard-coded data and one to dispatch vehicleError with hard-coded error info. Then you can point to this in your post and someone can help point out/fix the error much more quickly.
– Ryan Cogswell
Nov 14 '18 at 21:59
I think your problem is that if the response status code is not 500 you discard the error. I think it's better to call vehicleError if there's error.
– boaz_shuster
Nov 14 '18 at 22:01