“datalabels” property applied to all “datasets”
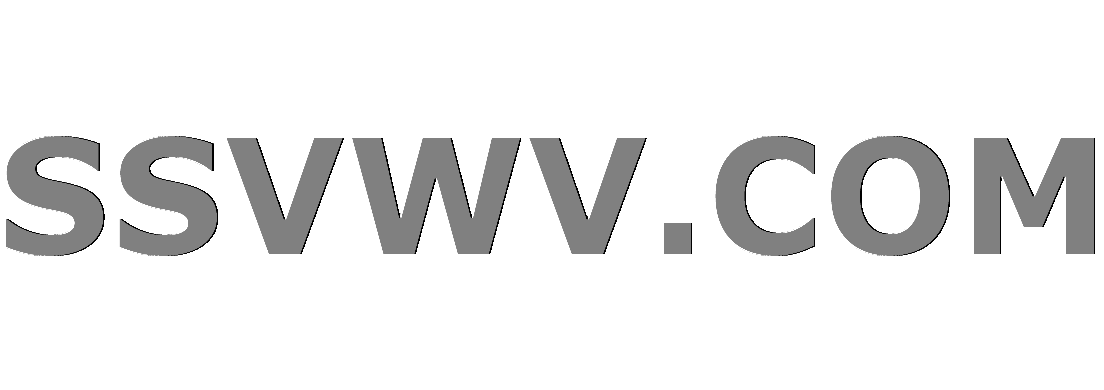
Multi tool use
I've been working on the front end bit recently on one of the projects at work and I got a bit of a problem. We're displaying a graph on the website, which shows some realtime data pulled from the database.
This is the code that has been written to create the graph:
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
],
;
As you can see in the code there, I had to copy the code related to "datalabels" three times, and I want to avoid that. I've read in the Chart.js documentation that you can apply "datalabels" to each dataset, whole chart or globally, and I believe in our situation applying this to a chart would be the best solution. I tried to add "datalabel" code behind the array, but still within datasets
and it didn't work. I also tried to redesign the weeklyBarData
, but I had some errors that wouldn't even load a website correctly, I tried to do something like this:
const weeklyData =
labels: ,
datasets:
data: [
// logic goes here
,
// logic goes here
,
// logic goes here
],
datalabels:
// logic goes here
;
I run out of ideas and I can't find any solution online, hence me question: Is there any way for me to either redesign the weeklyBarData
code or apply the datalabel
property to the whole chart using te current code?
(We use React for our front end).
Secodnly, using the code provided above I am able to create this graph:
As you can see, I get that weird little thing in the top-left corner. I zoomed at it and it seems like some string with "%". Also, it disappears when I remove all datalabel
code. Any thoughts on what that could be and how to get rid of that?
reactjs charts
add a comment |
I've been working on the front end bit recently on one of the projects at work and I got a bit of a problem. We're displaying a graph on the website, which shows some realtime data pulled from the database.
This is the code that has been written to create the graph:
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
],
;
As you can see in the code there, I had to copy the code related to "datalabels" three times, and I want to avoid that. I've read in the Chart.js documentation that you can apply "datalabels" to each dataset, whole chart or globally, and I believe in our situation applying this to a chart would be the best solution. I tried to add "datalabel" code behind the array, but still within datasets
and it didn't work. I also tried to redesign the weeklyBarData
, but I had some errors that wouldn't even load a website correctly, I tried to do something like this:
const weeklyData =
labels: ,
datasets:
data: [
// logic goes here
,
// logic goes here
,
// logic goes here
],
datalabels:
// logic goes here
;
I run out of ideas and I can't find any solution online, hence me question: Is there any way for me to either redesign the weeklyBarData
code or apply the datalabel
property to the whole chart using te current code?
(We use React for our front end).
Secodnly, using the code provided above I am able to create this graph:
As you can see, I get that weird little thing in the top-left corner. I zoomed at it and it seems like some string with "%". Also, it disappears when I remove all datalabel
code. Any thoughts on what that could be and how to get rid of that?
reactjs charts
add a comment |
I've been working on the front end bit recently on one of the projects at work and I got a bit of a problem. We're displaying a graph on the website, which shows some realtime data pulled from the database.
This is the code that has been written to create the graph:
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
],
;
As you can see in the code there, I had to copy the code related to "datalabels" three times, and I want to avoid that. I've read in the Chart.js documentation that you can apply "datalabels" to each dataset, whole chart or globally, and I believe in our situation applying this to a chart would be the best solution. I tried to add "datalabel" code behind the array, but still within datasets
and it didn't work. I also tried to redesign the weeklyBarData
, but I had some errors that wouldn't even load a website correctly, I tried to do something like this:
const weeklyData =
labels: ,
datasets:
data: [
// logic goes here
,
// logic goes here
,
// logic goes here
],
datalabels:
// logic goes here
;
I run out of ideas and I can't find any solution online, hence me question: Is there any way for me to either redesign the weeklyBarData
code or apply the datalabel
property to the whole chart using te current code?
(We use React for our front end).
Secodnly, using the code provided above I am able to create this graph:
As you can see, I get that weird little thing in the top-left corner. I zoomed at it and it seems like some string with "%". Also, it disappears when I remove all datalabel
code. Any thoughts on what that could be and how to get rid of that?
reactjs charts
I've been working on the front end bit recently on one of the projects at work and I got a bit of a problem. We're displaying a graph on the website, which shows some realtime data pulled from the database.
This is the code that has been written to create the graph:
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
,
],
;
As you can see in the code there, I had to copy the code related to "datalabels" three times, and I want to avoid that. I've read in the Chart.js documentation that you can apply "datalabels" to each dataset, whole chart or globally, and I believe in our situation applying this to a chart would be the best solution. I tried to add "datalabel" code behind the array, but still within datasets
and it didn't work. I also tried to redesign the weeklyBarData
, but I had some errors that wouldn't even load a website correctly, I tried to do something like this:
const weeklyData =
labels: ,
datasets:
data: [
// logic goes here
,
// logic goes here
,
// logic goes here
],
datalabels:
// logic goes here
;
I run out of ideas and I can't find any solution online, hence me question: Is there any way for me to either redesign the weeklyBarData
code or apply the datalabel
property to the whole chart using te current code?
(We use React for our front end).
Secodnly, using the code provided above I am able to create this graph:
As you can see, I get that weird little thing in the top-left corner. I zoomed at it and it seems like some string with "%". Also, it disappears when I remove all datalabel
code. Any thoughts on what that could be and how to get rid of that?
reactjs charts
reactjs charts
asked Nov 14 '18 at 15:45
IrekIrek
307
307
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
to apply the labels to the entire chart,
add datalabels
to the plugins option...
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
see following working snippet...
$(document).ready(function()
var totalPensDaily = [100, 100, 100, 100, 100];
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
],
;
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
);
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.7.2/Chart.bundle.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/chartjs-plugin-datalabels@0.2.0/dist/chartjs-plugin-datalabels.min.js"></script>
<canvas id="data"></canvas>
note: I don't see any weird little thing in the top-left corner here...
Thanks for your answer! It definitely makes things clearer and I will try to deploy them. Our code looks slightly different, and from what I can see we pass the data for the bar withinrender()
and it looks like this:<WeeklyOverview weeklyBarData=weeklyBarData weeklyBarOpts=weeklyBarOpts />
. Inside 'WeeklyOverview.js' we create each chart Bar separate (?), by doing this:<Bar data=this.props.weeklyBarData options=this.props.weeklyBarOpts height=350 />
. I will probably have to edit code a little bit to adapt your suggestion.
– Irek
Nov 15 '18 at 8:28
Also, the little-weird thing is between "500" on y-axis and the textWeekly Overview
- on the photo.
– Irek
Nov 15 '18 at 8:31
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53303917%2fdatalabels-property-applied-to-all-datasets%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
to apply the labels to the entire chart,
add datalabels
to the plugins option...
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
see following working snippet...
$(document).ready(function()
var totalPensDaily = [100, 100, 100, 100, 100];
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
],
;
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
);
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.7.2/Chart.bundle.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/chartjs-plugin-datalabels@0.2.0/dist/chartjs-plugin-datalabels.min.js"></script>
<canvas id="data"></canvas>
note: I don't see any weird little thing in the top-left corner here...
Thanks for your answer! It definitely makes things clearer and I will try to deploy them. Our code looks slightly different, and from what I can see we pass the data for the bar withinrender()
and it looks like this:<WeeklyOverview weeklyBarData=weeklyBarData weeklyBarOpts=weeklyBarOpts />
. Inside 'WeeklyOverview.js' we create each chart Bar separate (?), by doing this:<Bar data=this.props.weeklyBarData options=this.props.weeklyBarOpts height=350 />
. I will probably have to edit code a little bit to adapt your suggestion.
– Irek
Nov 15 '18 at 8:28
Also, the little-weird thing is between "500" on y-axis and the textWeekly Overview
- on the photo.
– Irek
Nov 15 '18 at 8:31
add a comment |
to apply the labels to the entire chart,
add datalabels
to the plugins option...
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
see following working snippet...
$(document).ready(function()
var totalPensDaily = [100, 100, 100, 100, 100];
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
],
;
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
);
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.7.2/Chart.bundle.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/chartjs-plugin-datalabels@0.2.0/dist/chartjs-plugin-datalabels.min.js"></script>
<canvas id="data"></canvas>
note: I don't see any weird little thing in the top-left corner here...
Thanks for your answer! It definitely makes things clearer and I will try to deploy them. Our code looks slightly different, and from what I can see we pass the data for the bar withinrender()
and it looks like this:<WeeklyOverview weeklyBarData=weeklyBarData weeklyBarOpts=weeklyBarOpts />
. Inside 'WeeklyOverview.js' we create each chart Bar separate (?), by doing this:<Bar data=this.props.weeklyBarData options=this.props.weeklyBarOpts height=350 />
. I will probably have to edit code a little bit to adapt your suggestion.
– Irek
Nov 15 '18 at 8:28
Also, the little-weird thing is between "500" on y-axis and the textWeekly Overview
- on the photo.
– Irek
Nov 15 '18 at 8:31
add a comment |
to apply the labels to the entire chart,
add datalabels
to the plugins option...
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
see following working snippet...
$(document).ready(function()
var totalPensDaily = [100, 100, 100, 100, 100];
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
],
;
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
);
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.7.2/Chart.bundle.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/chartjs-plugin-datalabels@0.2.0/dist/chartjs-plugin-datalabels.min.js"></script>
<canvas id="data"></canvas>
note: I don't see any weird little thing in the top-left corner here...
to apply the labels to the entire chart,
add datalabels
to the plugins option...
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
see following working snippet...
$(document).ready(function()
var totalPensDaily = [100, 100, 100, 100, 100];
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
],
;
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
);
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.7.2/Chart.bundle.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/chartjs-plugin-datalabels@0.2.0/dist/chartjs-plugin-datalabels.min.js"></script>
<canvas id="data"></canvas>
note: I don't see any weird little thing in the top-left corner here...
$(document).ready(function()
var totalPensDaily = [100, 100, 100, 100, 100];
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
],
;
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
);
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.7.2/Chart.bundle.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/chartjs-plugin-datalabels@0.2.0/dist/chartjs-plugin-datalabels.min.js"></script>
<canvas id="data"></canvas>
$(document).ready(function()
var totalPensDaily = [100, 100, 100, 100, 100];
const weeklyBarData =
labels: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
datasets: [
label: 'Pens Assembled',
backgroundColor: 'rgba(200,10,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Disassembled',
backgroundColor: 'rgba(10,200,10,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
label: 'Pens Claimed',
backgroundColor: 'rgba(10,10,200,0.8)',
borderColor: 'transparent',
data: [100, 200, 300, 400, 500],
,
],
;
var ctx = document.getElementById("data");
var myChart = new Chart(ctx,
type: 'bar',
data: weeklyBarData,
options:
plugins:
datalabels:
align: 'end',
anchor: 'end',
formatter: function(value, context)
let percent = (totalPensDaily[context.dataIndex] !== 0 ? (value / totalPensDaily[context.dataIndex])*100 : "");
percent = Math.round(percent);
percent = (percent !== 0) ? percent.toString() + '%' : "";
return percent;
);
);
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.7.2/Chart.bundle.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/chartjs-plugin-datalabels@0.2.0/dist/chartjs-plugin-datalabels.min.js"></script>
<canvas id="data"></canvas>
answered Nov 14 '18 at 17:18


WhiteHatWhiteHat
36.8k61576
36.8k61576
Thanks for your answer! It definitely makes things clearer and I will try to deploy them. Our code looks slightly different, and from what I can see we pass the data for the bar withinrender()
and it looks like this:<WeeklyOverview weeklyBarData=weeklyBarData weeklyBarOpts=weeklyBarOpts />
. Inside 'WeeklyOverview.js' we create each chart Bar separate (?), by doing this:<Bar data=this.props.weeklyBarData options=this.props.weeklyBarOpts height=350 />
. I will probably have to edit code a little bit to adapt your suggestion.
– Irek
Nov 15 '18 at 8:28
Also, the little-weird thing is between "500" on y-axis and the textWeekly Overview
- on the photo.
– Irek
Nov 15 '18 at 8:31
add a comment |
Thanks for your answer! It definitely makes things clearer and I will try to deploy them. Our code looks slightly different, and from what I can see we pass the data for the bar withinrender()
and it looks like this:<WeeklyOverview weeklyBarData=weeklyBarData weeklyBarOpts=weeklyBarOpts />
. Inside 'WeeklyOverview.js' we create each chart Bar separate (?), by doing this:<Bar data=this.props.weeklyBarData options=this.props.weeklyBarOpts height=350 />
. I will probably have to edit code a little bit to adapt your suggestion.
– Irek
Nov 15 '18 at 8:28
Also, the little-weird thing is between "500" on y-axis and the textWeekly Overview
- on the photo.
– Irek
Nov 15 '18 at 8:31
Thanks for your answer! It definitely makes things clearer and I will try to deploy them. Our code looks slightly different, and from what I can see we pass the data for the bar within
render()
and it looks like this: <WeeklyOverview weeklyBarData=weeklyBarData weeklyBarOpts=weeklyBarOpts />
. Inside 'WeeklyOverview.js' we create each chart Bar separate (?), by doing this: <Bar data=this.props.weeklyBarData options=this.props.weeklyBarOpts height=350 />
. I will probably have to edit code a little bit to adapt your suggestion.– Irek
Nov 15 '18 at 8:28
Thanks for your answer! It definitely makes things clearer and I will try to deploy them. Our code looks slightly different, and from what I can see we pass the data for the bar within
render()
and it looks like this: <WeeklyOverview weeklyBarData=weeklyBarData weeklyBarOpts=weeklyBarOpts />
. Inside 'WeeklyOverview.js' we create each chart Bar separate (?), by doing this: <Bar data=this.props.weeklyBarData options=this.props.weeklyBarOpts height=350 />
. I will probably have to edit code a little bit to adapt your suggestion.– Irek
Nov 15 '18 at 8:28
Also, the little-weird thing is between "500" on y-axis and the text
Weekly Overview
- on the photo.– Irek
Nov 15 '18 at 8:31
Also, the little-weird thing is between "500" on y-axis and the text
Weekly Overview
- on the photo.– Irek
Nov 15 '18 at 8:31
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53303917%2fdatalabels-property-applied-to-all-datasets%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
z5sQTwnaMwtU UuZDv9