how to query using a specific field instead of id in express js
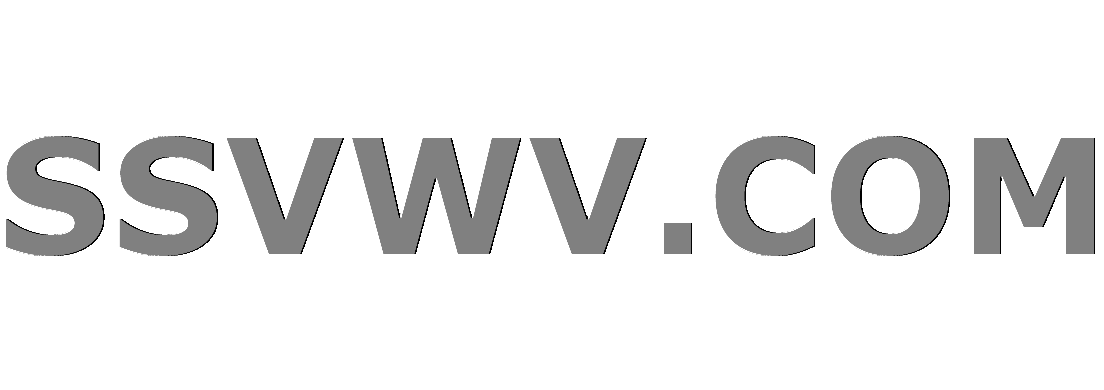
Multi tool use
Below is my code to query search by city. I am getting not found in my post> I need to be able to search for a specific city and get all the records for the field that matches the city. For example, if city is Downtown, I want all the records with the city downtown
router.get("/search", (req, res, next) =>
const city = req.query.city;
Facility.findAll(city)
.select('name type mobile price streetName city state _id')
.exec()
.then(docs =>
console.log("From database", docs);
if (docs)
res.status(200).json(
facility: docs
);
else
res
.status(404)
.json( message: "No valid entry found for provided City" );
)
.catch(err =>
console.log(err);
res.status(500).json( error: err );
);
);
node.js mongodb api express
add a comment |
Below is my code to query search by city. I am getting not found in my post> I need to be able to search for a specific city and get all the records for the field that matches the city. For example, if city is Downtown, I want all the records with the city downtown
router.get("/search", (req, res, next) =>
const city = req.query.city;
Facility.findAll(city)
.select('name type mobile price streetName city state _id')
.exec()
.then(docs =>
console.log("From database", docs);
if (docs)
res.status(200).json(
facility: docs
);
else
res
.status(404)
.json( message: "No valid entry found for provided City" );
)
.catch(err =>
console.log(err);
res.status(500).json( error: err );
);
);
node.js mongodb api express
do you need to add _id to the select feed? just curious 🤔
– Jalasem
Nov 14 '18 at 20:39
Please state the error you're getting as well
– Jalasem
Nov 14 '18 at 20:40
message: 'Cast to ObjectId failed for value "search" at path "_id" for model "Facility"',
– SO Jesse
Nov 14 '18 at 20:43
add a comment |
Below is my code to query search by city. I am getting not found in my post> I need to be able to search for a specific city and get all the records for the field that matches the city. For example, if city is Downtown, I want all the records with the city downtown
router.get("/search", (req, res, next) =>
const city = req.query.city;
Facility.findAll(city)
.select('name type mobile price streetName city state _id')
.exec()
.then(docs =>
console.log("From database", docs);
if (docs)
res.status(200).json(
facility: docs
);
else
res
.status(404)
.json( message: "No valid entry found for provided City" );
)
.catch(err =>
console.log(err);
res.status(500).json( error: err );
);
);
node.js mongodb api express
Below is my code to query search by city. I am getting not found in my post> I need to be able to search for a specific city and get all the records for the field that matches the city. For example, if city is Downtown, I want all the records with the city downtown
router.get("/search", (req, res, next) =>
const city = req.query.city;
Facility.findAll(city)
.select('name type mobile price streetName city state _id')
.exec()
.then(docs =>
console.log("From database", docs);
if (docs)
res.status(200).json(
facility: docs
);
else
res
.status(404)
.json( message: "No valid entry found for provided City" );
)
.catch(err =>
console.log(err);
res.status(500).json( error: err );
);
);
node.js mongodb api express
node.js mongodb api express
asked Nov 14 '18 at 15:59
SO JesseSO Jesse
62
62
do you need to add _id to the select feed? just curious 🤔
– Jalasem
Nov 14 '18 at 20:39
Please state the error you're getting as well
– Jalasem
Nov 14 '18 at 20:40
message: 'Cast to ObjectId failed for value "search" at path "_id" for model "Facility"',
– SO Jesse
Nov 14 '18 at 20:43
add a comment |
do you need to add _id to the select feed? just curious 🤔
– Jalasem
Nov 14 '18 at 20:39
Please state the error you're getting as well
– Jalasem
Nov 14 '18 at 20:40
message: 'Cast to ObjectId failed for value "search" at path "_id" for model "Facility"',
– SO Jesse
Nov 14 '18 at 20:43
do you need to add _id to the select feed? just curious 🤔
– Jalasem
Nov 14 '18 at 20:39
do you need to add _id to the select feed? just curious 🤔
– Jalasem
Nov 14 '18 at 20:39
Please state the error you're getting as well
– Jalasem
Nov 14 '18 at 20:40
Please state the error you're getting as well
– Jalasem
Nov 14 '18 at 20:40
message: 'Cast to ObjectId failed for value "search" at path "_id" for model "Facility"',
– SO Jesse
Nov 14 '18 at 20:43
message: 'Cast to ObjectId failed for value "search" at path "_id" for model "Facility"',
– SO Jesse
Nov 14 '18 at 20:43
add a comment |
3 Answers
3
active
oldest
votes
Here is a better way to do it;
router.get('/search', (req, res) =>
const city = req.query
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, doc) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
facility: docs
);
)
)
find()
will do the job. I don't see a need for you to select _id
, you only specify with -_id
if you don't want it but _id
is selected by default.
Below is a list of valid queries in Mongoose if that is what you're using
Model.deleteMany()
Model.deleteOne()
Model.find()
Model.findById()
Model.findByIdAndDelete()
Model.findByIdAndRemove()
Model.findByIdAndUpdate()
Model.findOne()
Model.findOneAndDelete()
Model.findOneAndRemove()
Model.findOneAndUpdate()
Model.replaceOne()
Model.updateMany()
Model.updateOne()
Check https://mongoosejs.com/docs/queries.html for more information on queries
I hope this helps
The above didn't give the right output
– SO Jesse
Nov 22 '18 at 9:08
add a comment |
You're almost there. Just specify your query and you should be fine
https://mongoosejs.com/docs/api.html#model_Model.find
router.get("/search", (req, res, next) =>
const city = req.query.city;
Facility.findAll(propertyName: city)
.select('name type mobile price streetName city state _id')
.exec()
.then(docs =>
console.log("From database", docs);
if (docs)
res.status(200).json(
facility: docs
);
else
res
.status(404)
.json( message: "No valid entry found for provided City" );
)
.catch(err =>
console.log(err);
res.status(500).json( error: err );
);
);
it didn't work when I used postman. See error below "error": "message": "Cast to ObjectId failed for value "search" at path "_id" for model "Facility"", "name": "CastError", "stringValue": ""search"", "kind": "ObjectId", "value": "search", "path": "_id" below is the link i used: localhost:9944/facilities/search?Sabo
– SO Jesse
Nov 14 '18 at 16:10
It's still trying to query _id property. can you update your code?
– Manish Gharat
Nov 14 '18 at 16:16
add a comment |
Below is the working code:
router.get('/search', (req, res) =>
const city = req.query.city
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, docs) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
count: docs.length,
facility: docs
);
)
);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53304207%2fhow-to-query-using-a-specific-field-instead-of-id-in-express-js%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here is a better way to do it;
router.get('/search', (req, res) =>
const city = req.query
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, doc) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
facility: docs
);
)
)
find()
will do the job. I don't see a need for you to select _id
, you only specify with -_id
if you don't want it but _id
is selected by default.
Below is a list of valid queries in Mongoose if that is what you're using
Model.deleteMany()
Model.deleteOne()
Model.find()
Model.findById()
Model.findByIdAndDelete()
Model.findByIdAndRemove()
Model.findByIdAndUpdate()
Model.findOne()
Model.findOneAndDelete()
Model.findOneAndRemove()
Model.findOneAndUpdate()
Model.replaceOne()
Model.updateMany()
Model.updateOne()
Check https://mongoosejs.com/docs/queries.html for more information on queries
I hope this helps
The above didn't give the right output
– SO Jesse
Nov 22 '18 at 9:08
add a comment |
Here is a better way to do it;
router.get('/search', (req, res) =>
const city = req.query
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, doc) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
facility: docs
);
)
)
find()
will do the job. I don't see a need for you to select _id
, you only specify with -_id
if you don't want it but _id
is selected by default.
Below is a list of valid queries in Mongoose if that is what you're using
Model.deleteMany()
Model.deleteOne()
Model.find()
Model.findById()
Model.findByIdAndDelete()
Model.findByIdAndRemove()
Model.findByIdAndUpdate()
Model.findOne()
Model.findOneAndDelete()
Model.findOneAndRemove()
Model.findOneAndUpdate()
Model.replaceOne()
Model.updateMany()
Model.updateOne()
Check https://mongoosejs.com/docs/queries.html for more information on queries
I hope this helps
The above didn't give the right output
– SO Jesse
Nov 22 '18 at 9:08
add a comment |
Here is a better way to do it;
router.get('/search', (req, res) =>
const city = req.query
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, doc) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
facility: docs
);
)
)
find()
will do the job. I don't see a need for you to select _id
, you only specify with -_id
if you don't want it but _id
is selected by default.
Below is a list of valid queries in Mongoose if that is what you're using
Model.deleteMany()
Model.deleteOne()
Model.find()
Model.findById()
Model.findByIdAndDelete()
Model.findByIdAndRemove()
Model.findByIdAndUpdate()
Model.findOne()
Model.findOneAndDelete()
Model.findOneAndRemove()
Model.findOneAndUpdate()
Model.replaceOne()
Model.updateMany()
Model.updateOne()
Check https://mongoosejs.com/docs/queries.html for more information on queries
I hope this helps
Here is a better way to do it;
router.get('/search', (req, res) =>
const city = req.query
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, doc) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
facility: docs
);
)
)
find()
will do the job. I don't see a need for you to select _id
, you only specify with -_id
if you don't want it but _id
is selected by default.
Below is a list of valid queries in Mongoose if that is what you're using
Model.deleteMany()
Model.deleteOne()
Model.find()
Model.findById()
Model.findByIdAndDelete()
Model.findByIdAndRemove()
Model.findByIdAndUpdate()
Model.findOne()
Model.findOneAndDelete()
Model.findOneAndRemove()
Model.findOneAndUpdate()
Model.replaceOne()
Model.updateMany()
Model.updateOne()
Check https://mongoosejs.com/docs/queries.html for more information on queries
I hope this helps
edited Nov 23 '18 at 23:36
answered Nov 14 '18 at 21:14
JalasemJalasem
6,85031117
6,85031117
The above didn't give the right output
– SO Jesse
Nov 22 '18 at 9:08
add a comment |
The above didn't give the right output
– SO Jesse
Nov 22 '18 at 9:08
The above didn't give the right output
– SO Jesse
Nov 22 '18 at 9:08
The above didn't give the right output
– SO Jesse
Nov 22 '18 at 9:08
add a comment |
You're almost there. Just specify your query and you should be fine
https://mongoosejs.com/docs/api.html#model_Model.find
router.get("/search", (req, res, next) =>
const city = req.query.city;
Facility.findAll(propertyName: city)
.select('name type mobile price streetName city state _id')
.exec()
.then(docs =>
console.log("From database", docs);
if (docs)
res.status(200).json(
facility: docs
);
else
res
.status(404)
.json( message: "No valid entry found for provided City" );
)
.catch(err =>
console.log(err);
res.status(500).json( error: err );
);
);
it didn't work when I used postman. See error below "error": "message": "Cast to ObjectId failed for value "search" at path "_id" for model "Facility"", "name": "CastError", "stringValue": ""search"", "kind": "ObjectId", "value": "search", "path": "_id" below is the link i used: localhost:9944/facilities/search?Sabo
– SO Jesse
Nov 14 '18 at 16:10
It's still trying to query _id property. can you update your code?
– Manish Gharat
Nov 14 '18 at 16:16
add a comment |
You're almost there. Just specify your query and you should be fine
https://mongoosejs.com/docs/api.html#model_Model.find
router.get("/search", (req, res, next) =>
const city = req.query.city;
Facility.findAll(propertyName: city)
.select('name type mobile price streetName city state _id')
.exec()
.then(docs =>
console.log("From database", docs);
if (docs)
res.status(200).json(
facility: docs
);
else
res
.status(404)
.json( message: "No valid entry found for provided City" );
)
.catch(err =>
console.log(err);
res.status(500).json( error: err );
);
);
it didn't work when I used postman. See error below "error": "message": "Cast to ObjectId failed for value "search" at path "_id" for model "Facility"", "name": "CastError", "stringValue": ""search"", "kind": "ObjectId", "value": "search", "path": "_id" below is the link i used: localhost:9944/facilities/search?Sabo
– SO Jesse
Nov 14 '18 at 16:10
It's still trying to query _id property. can you update your code?
– Manish Gharat
Nov 14 '18 at 16:16
add a comment |
You're almost there. Just specify your query and you should be fine
https://mongoosejs.com/docs/api.html#model_Model.find
router.get("/search", (req, res, next) =>
const city = req.query.city;
Facility.findAll(propertyName: city)
.select('name type mobile price streetName city state _id')
.exec()
.then(docs =>
console.log("From database", docs);
if (docs)
res.status(200).json(
facility: docs
);
else
res
.status(404)
.json( message: "No valid entry found for provided City" );
)
.catch(err =>
console.log(err);
res.status(500).json( error: err );
);
);
You're almost there. Just specify your query and you should be fine
https://mongoosejs.com/docs/api.html#model_Model.find
router.get("/search", (req, res, next) =>
const city = req.query.city;
Facility.findAll(propertyName: city)
.select('name type mobile price streetName city state _id')
.exec()
.then(docs =>
console.log("From database", docs);
if (docs)
res.status(200).json(
facility: docs
);
else
res
.status(404)
.json( message: "No valid entry found for provided City" );
)
.catch(err =>
console.log(err);
res.status(500).json( error: err );
);
);
answered Nov 14 '18 at 16:01


Manish GharatManish Gharat
31618
31618
it didn't work when I used postman. See error below "error": "message": "Cast to ObjectId failed for value "search" at path "_id" for model "Facility"", "name": "CastError", "stringValue": ""search"", "kind": "ObjectId", "value": "search", "path": "_id" below is the link i used: localhost:9944/facilities/search?Sabo
– SO Jesse
Nov 14 '18 at 16:10
It's still trying to query _id property. can you update your code?
– Manish Gharat
Nov 14 '18 at 16:16
add a comment |
it didn't work when I used postman. See error below "error": "message": "Cast to ObjectId failed for value "search" at path "_id" for model "Facility"", "name": "CastError", "stringValue": ""search"", "kind": "ObjectId", "value": "search", "path": "_id" below is the link i used: localhost:9944/facilities/search?Sabo
– SO Jesse
Nov 14 '18 at 16:10
It's still trying to query _id property. can you update your code?
– Manish Gharat
Nov 14 '18 at 16:16
it didn't work when I used postman. See error below "error": "message": "Cast to ObjectId failed for value "search" at path "_id" for model "Facility"", "name": "CastError", "stringValue": ""search"", "kind": "ObjectId", "value": "search", "path": "_id" below is the link i used: localhost:9944/facilities/search?Sabo
– SO Jesse
Nov 14 '18 at 16:10
it didn't work when I used postman. See error below "error": "message": "Cast to ObjectId failed for value "search" at path "_id" for model "Facility"", "name": "CastError", "stringValue": ""search"", "kind": "ObjectId", "value": "search", "path": "_id" below is the link i used: localhost:9944/facilities/search?Sabo
– SO Jesse
Nov 14 '18 at 16:10
It's still trying to query _id property. can you update your code?
– Manish Gharat
Nov 14 '18 at 16:16
It's still trying to query _id property. can you update your code?
– Manish Gharat
Nov 14 '18 at 16:16
add a comment |
Below is the working code:
router.get('/search', (req, res) =>
const city = req.query.city
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, docs) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
count: docs.length,
facility: docs
);
)
);
add a comment |
Below is the working code:
router.get('/search', (req, res) =>
const city = req.query.city
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, docs) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
count: docs.length,
facility: docs
);
)
);
add a comment |
Below is the working code:
router.get('/search', (req, res) =>
const city = req.query.city
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, docs) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
count: docs.length,
facility: docs
);
)
);
Below is the working code:
router.get('/search', (req, res) =>
const city = req.query.city
Facility.find(city)
.select('name type mobile price streetName city state')
.exec((err, docs) =>
if (err)
return res.status(500)
.json( message: 'error querying cities', error: err );
if (!docs)
return res.status(404)
.json( message: 'No valid entry found for provided City' );
return res.status(200)
.json(
count: docs.length,
facility: docs
);
)
);
answered Nov 22 '18 at 9:26
SO JesseSO Jesse
62
62
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53304207%2fhow-to-query-using-a-specific-field-instead-of-id-in-express-js%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
kXA6NkM4sbhs8a4B1k mge,B40AhL,9UJB73aG,Jtl9FkvWm,TbcWCm
do you need to add _id to the select feed? just curious 🤔
– Jalasem
Nov 14 '18 at 20:39
Please state the error you're getting as well
– Jalasem
Nov 14 '18 at 20:40
message: 'Cast to ObjectId failed for value "search" at path "_id" for model "Facility"',
– SO Jesse
Nov 14 '18 at 20:43