lottery iterator running out of bounds in university project
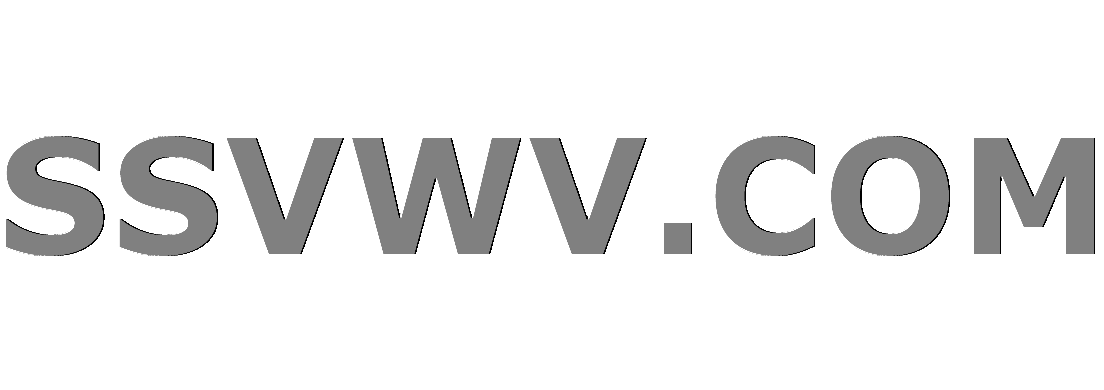
Multi tool use
Currently, for my university project, we have to simulate a lottery system, or "euro millions" for that we are given two default classes: the key class and the iterator class. We cannot change them in any way.
I wanted to compare the values from the key iterator to an array of scanned integers, returning a number of matches found. The problem is that whenever i insert those numbers the iterator goes out of bounds, and as such I cannot compare both.
Here is what I tried to do, with the numbers (there are 5 numbers and 2 stars - like euromillions):
public int compareNums()
int n = 0;
int numberOfMatches = 0;
It2Storage.reinitialize();
while (n < 5)
if (It2Storage.hasNextInt() && It2Storage.nextInt() == numArray[n])
numberOfMatches++;
n++;
It2Storage.reinitialize();
else if (((It2Storage.hasNextInt()) && (It2Storage.nextInt() != numArray[n])))
System.out.print(It2Storage.nextInt());
n++;
It2Storage.reinitialize();
return numberOfMatches;
The It2Storage
is a copy of an Iterator containing the values of the numbers array of the key class.
The NumArray
is the Array containing all scanner input numbers.
The if states that the Iterator should only go to the next Integer if it has a next Integer. However, I do not see that happening.
Here is the default Iterator
Class and Key
class.
Iterator:
public class IteratorInt
private int countNumbers,currNumber;
private int numbers;
public IteratorInt(int numbers)
countNumbers=numbers.length;
currNumber=0;
this.numbers=numbers;
/
public IteratorInt(int numbers, int nElems)
countNumbers=nElems;
currNumber=0;
this.numbers=numbers;
public boolean hasNextInt()
return currNumber < countNumbers;
public int nextInt()
return numbers[currNumber++];
public void reinitialize()
currNumber=0;
And here is the Key
public class Key
private static final int NUMBERGAMES =10;
private static final int NUMBERS = 4,5,28,50,11,4,8,18,44,13,1,2,28,48,49,2,15,22,33,11,10,20,30,50,40,4,15,21,32,11,2,5,18,20,11,6,7,28,50,14,1,5,33,50,11,4,6,28,45,10;
private static final int STARS = 4,5,4,6,4,10,9,10,1,5,6,7,1,11,5,8,9,10,1,12;
private static int CURRENTGAME=0;
private int idGame;
public Key()
idGame=CURRENTGAME;
CURRENTGAME= ++CURRENTGAME % NUMBERGAMES;
public IteratorInt criaIteratorNumbers() //creates numbers iterator
return new IteratorInt(NUMBERS[idGame]);
public IteratorInt criaIteratorStars() //creates stars iterator
return new IteratorInt(STARS[idGame]);
I created a key in my game class and created Iterators for the stars and numbers, both of which I stored in the form of the Iterator Variables It1Storage
and It2Storage
.
In order to compare the inserted numbers to the numbers of the key, should I continue using my method but modify it, or take a different approach?
java arrays iterator compare indexoutofboundsexception
add a comment |
Currently, for my university project, we have to simulate a lottery system, or "euro millions" for that we are given two default classes: the key class and the iterator class. We cannot change them in any way.
I wanted to compare the values from the key iterator to an array of scanned integers, returning a number of matches found. The problem is that whenever i insert those numbers the iterator goes out of bounds, and as such I cannot compare both.
Here is what I tried to do, with the numbers (there are 5 numbers and 2 stars - like euromillions):
public int compareNums()
int n = 0;
int numberOfMatches = 0;
It2Storage.reinitialize();
while (n < 5)
if (It2Storage.hasNextInt() && It2Storage.nextInt() == numArray[n])
numberOfMatches++;
n++;
It2Storage.reinitialize();
else if (((It2Storage.hasNextInt()) && (It2Storage.nextInt() != numArray[n])))
System.out.print(It2Storage.nextInt());
n++;
It2Storage.reinitialize();
return numberOfMatches;
The It2Storage
is a copy of an Iterator containing the values of the numbers array of the key class.
The NumArray
is the Array containing all scanner input numbers.
The if states that the Iterator should only go to the next Integer if it has a next Integer. However, I do not see that happening.
Here is the default Iterator
Class and Key
class.
Iterator:
public class IteratorInt
private int countNumbers,currNumber;
private int numbers;
public IteratorInt(int numbers)
countNumbers=numbers.length;
currNumber=0;
this.numbers=numbers;
/
public IteratorInt(int numbers, int nElems)
countNumbers=nElems;
currNumber=0;
this.numbers=numbers;
public boolean hasNextInt()
return currNumber < countNumbers;
public int nextInt()
return numbers[currNumber++];
public void reinitialize()
currNumber=0;
And here is the Key
public class Key
private static final int NUMBERGAMES =10;
private static final int NUMBERS = 4,5,28,50,11,4,8,18,44,13,1,2,28,48,49,2,15,22,33,11,10,20,30,50,40,4,15,21,32,11,2,5,18,20,11,6,7,28,50,14,1,5,33,50,11,4,6,28,45,10;
private static final int STARS = 4,5,4,6,4,10,9,10,1,5,6,7,1,11,5,8,9,10,1,12;
private static int CURRENTGAME=0;
private int idGame;
public Key()
idGame=CURRENTGAME;
CURRENTGAME= ++CURRENTGAME % NUMBERGAMES;
public IteratorInt criaIteratorNumbers() //creates numbers iterator
return new IteratorInt(NUMBERS[idGame]);
public IteratorInt criaIteratorStars() //creates stars iterator
return new IteratorInt(STARS[idGame]);
I created a key in my game class and created Iterators for the stars and numbers, both of which I stored in the form of the Iterator Variables It1Storage
and It2Storage
.
In order to compare the inserted numbers to the numbers of the key, should I continue using my method but modify it, or take a different approach?
java arrays iterator compare indexoutofboundsexception
add a comment |
Currently, for my university project, we have to simulate a lottery system, or "euro millions" for that we are given two default classes: the key class and the iterator class. We cannot change them in any way.
I wanted to compare the values from the key iterator to an array of scanned integers, returning a number of matches found. The problem is that whenever i insert those numbers the iterator goes out of bounds, and as such I cannot compare both.
Here is what I tried to do, with the numbers (there are 5 numbers and 2 stars - like euromillions):
public int compareNums()
int n = 0;
int numberOfMatches = 0;
It2Storage.reinitialize();
while (n < 5)
if (It2Storage.hasNextInt() && It2Storage.nextInt() == numArray[n])
numberOfMatches++;
n++;
It2Storage.reinitialize();
else if (((It2Storage.hasNextInt()) && (It2Storage.nextInt() != numArray[n])))
System.out.print(It2Storage.nextInt());
n++;
It2Storage.reinitialize();
return numberOfMatches;
The It2Storage
is a copy of an Iterator containing the values of the numbers array of the key class.
The NumArray
is the Array containing all scanner input numbers.
The if states that the Iterator should only go to the next Integer if it has a next Integer. However, I do not see that happening.
Here is the default Iterator
Class and Key
class.
Iterator:
public class IteratorInt
private int countNumbers,currNumber;
private int numbers;
public IteratorInt(int numbers)
countNumbers=numbers.length;
currNumber=0;
this.numbers=numbers;
/
public IteratorInt(int numbers, int nElems)
countNumbers=nElems;
currNumber=0;
this.numbers=numbers;
public boolean hasNextInt()
return currNumber < countNumbers;
public int nextInt()
return numbers[currNumber++];
public void reinitialize()
currNumber=0;
And here is the Key
public class Key
private static final int NUMBERGAMES =10;
private static final int NUMBERS = 4,5,28,50,11,4,8,18,44,13,1,2,28,48,49,2,15,22,33,11,10,20,30,50,40,4,15,21,32,11,2,5,18,20,11,6,7,28,50,14,1,5,33,50,11,4,6,28,45,10;
private static final int STARS = 4,5,4,6,4,10,9,10,1,5,6,7,1,11,5,8,9,10,1,12;
private static int CURRENTGAME=0;
private int idGame;
public Key()
idGame=CURRENTGAME;
CURRENTGAME= ++CURRENTGAME % NUMBERGAMES;
public IteratorInt criaIteratorNumbers() //creates numbers iterator
return new IteratorInt(NUMBERS[idGame]);
public IteratorInt criaIteratorStars() //creates stars iterator
return new IteratorInt(STARS[idGame]);
I created a key in my game class and created Iterators for the stars and numbers, both of which I stored in the form of the Iterator Variables It1Storage
and It2Storage
.
In order to compare the inserted numbers to the numbers of the key, should I continue using my method but modify it, or take a different approach?
java arrays iterator compare indexoutofboundsexception
Currently, for my university project, we have to simulate a lottery system, or "euro millions" for that we are given two default classes: the key class and the iterator class. We cannot change them in any way.
I wanted to compare the values from the key iterator to an array of scanned integers, returning a number of matches found. The problem is that whenever i insert those numbers the iterator goes out of bounds, and as such I cannot compare both.
Here is what I tried to do, with the numbers (there are 5 numbers and 2 stars - like euromillions):
public int compareNums()
int n = 0;
int numberOfMatches = 0;
It2Storage.reinitialize();
while (n < 5)
if (It2Storage.hasNextInt() && It2Storage.nextInt() == numArray[n])
numberOfMatches++;
n++;
It2Storage.reinitialize();
else if (((It2Storage.hasNextInt()) && (It2Storage.nextInt() != numArray[n])))
System.out.print(It2Storage.nextInt());
n++;
It2Storage.reinitialize();
return numberOfMatches;
The It2Storage
is a copy of an Iterator containing the values of the numbers array of the key class.
The NumArray
is the Array containing all scanner input numbers.
The if states that the Iterator should only go to the next Integer if it has a next Integer. However, I do not see that happening.
Here is the default Iterator
Class and Key
class.
Iterator:
public class IteratorInt
private int countNumbers,currNumber;
private int numbers;
public IteratorInt(int numbers)
countNumbers=numbers.length;
currNumber=0;
this.numbers=numbers;
/
public IteratorInt(int numbers, int nElems)
countNumbers=nElems;
currNumber=0;
this.numbers=numbers;
public boolean hasNextInt()
return currNumber < countNumbers;
public int nextInt()
return numbers[currNumber++];
public void reinitialize()
currNumber=0;
And here is the Key
public class Key
private static final int NUMBERGAMES =10;
private static final int NUMBERS = 4,5,28,50,11,4,8,18,44,13,1,2,28,48,49,2,15,22,33,11,10,20,30,50,40,4,15,21,32,11,2,5,18,20,11,6,7,28,50,14,1,5,33,50,11,4,6,28,45,10;
private static final int STARS = 4,5,4,6,4,10,9,10,1,5,6,7,1,11,5,8,9,10,1,12;
private static int CURRENTGAME=0;
private int idGame;
public Key()
idGame=CURRENTGAME;
CURRENTGAME= ++CURRENTGAME % NUMBERGAMES;
public IteratorInt criaIteratorNumbers() //creates numbers iterator
return new IteratorInt(NUMBERS[idGame]);
public IteratorInt criaIteratorStars() //creates stars iterator
return new IteratorInt(STARS[idGame]);
I created a key in my game class and created Iterators for the stars and numbers, both of which I stored in the form of the Iterator Variables It1Storage
and It2Storage
.
In order to compare the inserted numbers to the numbers of the key, should I continue using my method but modify it, or take a different approach?
java arrays iterator compare indexoutofboundsexception
java arrays iterator compare indexoutofboundsexception
edited Nov 15 '18 at 11:02


Rai
8981721
8981721
asked Nov 15 '18 at 10:24


CJPortugalCJPortugal
42
42
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317275%2flottery-iterator-running-out-of-bounds-in-university-project%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317275%2flottery-iterator-running-out-of-bounds-in-university-project%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZVDOHoHxnNAqfMA,tatxg4HbegZBiN4Qs8r6uMRBT0Bo5ve,OQx4Om