Is it possible to nest JUnit 5 parameterized tests?
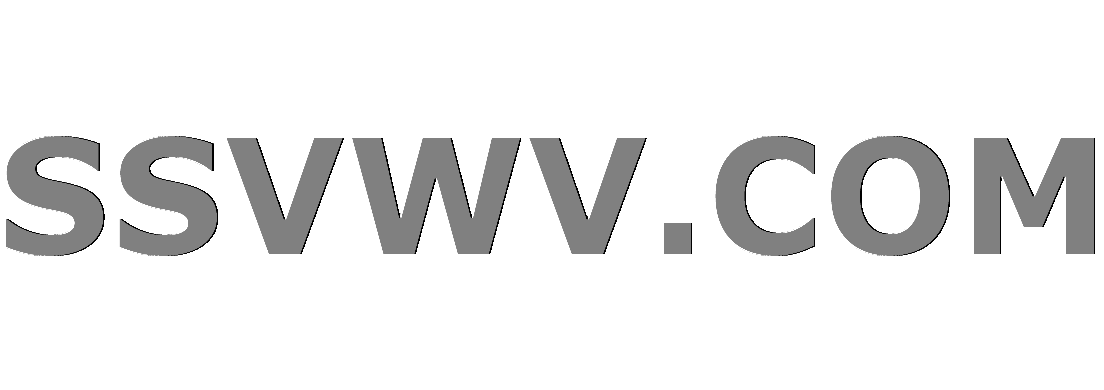
Multi tool use
I am attempting to write a parameterized test for an interface Foo, which declares a method getFooEventInt(int, int). I have written a paramterized test that works for a single instance of Foo (a FooImpl object).
public class FooTest
@ParameterizedTest
@MethodSource("getFooEvenIntProvider")
public void getFooEvenIntTest(int seed, int expectedResult)
Foo foo = new FooImpl();
Assertions.assertEquals(expectedResult, foo.getFooEvenInt(seed));
private static Stream getFooEvenIntProvider()
return Stream.of(
Arguments.of(-2, 0),
Arguments.of(-1, 0),
Arguments.of( 0, 2),
Arguments.of( 1, 2),
);
However, I'd like to be able to have getFooEvenIntTest(int, int) be invoked against a provided list of Foo implementation instances, with each iteration then using the provide list of seed/expectedResult values.
I realize I could do this as...
public class FooTest
@ParameterizedTest
@MethodSource("getFooProvider")
public void getFooImplEvenIntTest(Foo foo)
int expectedResult = 0, 0, 2, 2 ;
int seed = -2, -1, 0, 1 ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFooEvenInt(seed[i]));
private static Stream getFooProvider()
return Stream.of(
Arguments.of(new FooImpl()),
Arguments.of(new FooImpl2())
);
Any ideas? I'll post if I figure it out, but I thought I'd check to see if this is even doable, or if there's a different approach.
nested parameter-passing junit5
add a comment |
I am attempting to write a parameterized test for an interface Foo, which declares a method getFooEventInt(int, int). I have written a paramterized test that works for a single instance of Foo (a FooImpl object).
public class FooTest
@ParameterizedTest
@MethodSource("getFooEvenIntProvider")
public void getFooEvenIntTest(int seed, int expectedResult)
Foo foo = new FooImpl();
Assertions.assertEquals(expectedResult, foo.getFooEvenInt(seed));
private static Stream getFooEvenIntProvider()
return Stream.of(
Arguments.of(-2, 0),
Arguments.of(-1, 0),
Arguments.of( 0, 2),
Arguments.of( 1, 2),
);
However, I'd like to be able to have getFooEvenIntTest(int, int) be invoked against a provided list of Foo implementation instances, with each iteration then using the provide list of seed/expectedResult values.
I realize I could do this as...
public class FooTest
@ParameterizedTest
@MethodSource("getFooProvider")
public void getFooImplEvenIntTest(Foo foo)
int expectedResult = 0, 0, 2, 2 ;
int seed = -2, -1, 0, 1 ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFooEvenInt(seed[i]));
private static Stream getFooProvider()
return Stream.of(
Arguments.of(new FooImpl()),
Arguments.of(new FooImpl2())
);
Any ideas? I'll post if I figure it out, but I thought I'd check to see if this is even doable, or if there's a different approach.
nested parameter-passing junit5
add a comment |
I am attempting to write a parameterized test for an interface Foo, which declares a method getFooEventInt(int, int). I have written a paramterized test that works for a single instance of Foo (a FooImpl object).
public class FooTest
@ParameterizedTest
@MethodSource("getFooEvenIntProvider")
public void getFooEvenIntTest(int seed, int expectedResult)
Foo foo = new FooImpl();
Assertions.assertEquals(expectedResult, foo.getFooEvenInt(seed));
private static Stream getFooEvenIntProvider()
return Stream.of(
Arguments.of(-2, 0),
Arguments.of(-1, 0),
Arguments.of( 0, 2),
Arguments.of( 1, 2),
);
However, I'd like to be able to have getFooEvenIntTest(int, int) be invoked against a provided list of Foo implementation instances, with each iteration then using the provide list of seed/expectedResult values.
I realize I could do this as...
public class FooTest
@ParameterizedTest
@MethodSource("getFooProvider")
public void getFooImplEvenIntTest(Foo foo)
int expectedResult = 0, 0, 2, 2 ;
int seed = -2, -1, 0, 1 ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFooEvenInt(seed[i]));
private static Stream getFooProvider()
return Stream.of(
Arguments.of(new FooImpl()),
Arguments.of(new FooImpl2())
);
Any ideas? I'll post if I figure it out, but I thought I'd check to see if this is even doable, or if there's a different approach.
nested parameter-passing junit5
I am attempting to write a parameterized test for an interface Foo, which declares a method getFooEventInt(int, int). I have written a paramterized test that works for a single instance of Foo (a FooImpl object).
public class FooTest
@ParameterizedTest
@MethodSource("getFooEvenIntProvider")
public void getFooEvenIntTest(int seed, int expectedResult)
Foo foo = new FooImpl();
Assertions.assertEquals(expectedResult, foo.getFooEvenInt(seed));
private static Stream getFooEvenIntProvider()
return Stream.of(
Arguments.of(-2, 0),
Arguments.of(-1, 0),
Arguments.of( 0, 2),
Arguments.of( 1, 2),
);
However, I'd like to be able to have getFooEvenIntTest(int, int) be invoked against a provided list of Foo implementation instances, with each iteration then using the provide list of seed/expectedResult values.
I realize I could do this as...
public class FooTest
@ParameterizedTest
@MethodSource("getFooProvider")
public void getFooImplEvenIntTest(Foo foo)
int expectedResult = 0, 0, 2, 2 ;
int seed = -2, -1, 0, 1 ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFooEvenInt(seed[i]));
private static Stream getFooProvider()
return Stream.of(
Arguments.of(new FooImpl()),
Arguments.of(new FooImpl2())
);
Any ideas? I'll post if I figure it out, but I thought I'd check to see if this is even doable, or if there's a different approach.
nested parameter-passing junit5
nested parameter-passing junit5
edited Nov 15 '18 at 21:14
SoCal
asked Nov 15 '18 at 20:54
SoCalSoCal
859
859
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
BLUF: I will interpret the crickets to mean "even if you could, you shouldn't be nesting parameterized tests", in which case I run with the approach outlined below.
For an interface Foo...
public interface Foo
public char getFirstChar(String strValue);
public int getNextEvenInt(int seed);
The "best" use of parameterized tests for implementations of Foo would be...
public class FooTest
@ParameterizedTest
@MethodSource("getFooProvider")
public void getFirstCharTest(Foo foo)
char expectedResult = 'a', 'b', 'c', 'd' ;
String seed = "alpha", "bravo", "charlie", "delta" ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFirstChar(seed[i]));
@ParameterizedTest
@MethodSource("getFooProvider")
public void getNextEvenIntTest(Foo foo)
int expectedResult = 0, 0, 2, 2 ;
int seed = -2, -1, 0, 1 ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFooEvenInt(seed[i]));
private static Stream getFooProvider()
return Stream.of(
Arguments.of(new FooImplOne()),
Arguments.of(new FooImplTwo())
// extend as need for implementations of Foo
);
While I won't get the "warm fuzzies" of seeing the passing results for each value-pair in the various tests, it will fulfill my goal of having a test at the interface level that I can easily extend to validate/verify the interface's implementations.
add a comment |
I guess you think of combining two arguments streams. You could achieve this by creating the cartesian product of two arguments lists.
I have implemented that on https://github.com/joerg-pfruender/junit-goodies/blob/master/src/main/java/com/github/joergpfruender/junitgoodies/ParameterizedTestHelper.java
public static Stream<Arguments> cartesian(Stream a, Stream b)
List argumentsA = (List) a.collect(Collectors.toList());
List argumentsB = (List) b.collect(Collectors.toList());
List<Arguments> result = new ArrayList();
for (Object o : argumentsA)
Object objects = asArray(o);
for (Object o1 : argumentsB)
Object objects1 = asArray(o1);
Object arguments = ArrayUtils.addAll(objects, objects1);
result.add(Arguments.of(arguments));
return result.stream();
private static Object asArray(Object o)
Object objects;
if (o instanceof Arguments)
objects = ((Arguments) o).get();
else
objects = new Objecto;
return objects;
Then your test code will be:
public static Stream<Arguments> fooIntsAndFooProvider()
return ParameterizedTestHelper.cartesian(getFooEvenIntProvider(), getFooProvider());
@ParameterizedTest
@MethodSource("fooIntsAndFooProvider")
public void getFooImplEvenIntTest(Integer seed, Integer expectedResult, Foo foo)
Assertions.assertEquals(expectedResult,
foo.getFooEvenInt(seed));
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53327761%2fis-it-possible-to-nest-junit-5-parameterized-tests%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
BLUF: I will interpret the crickets to mean "even if you could, you shouldn't be nesting parameterized tests", in which case I run with the approach outlined below.
For an interface Foo...
public interface Foo
public char getFirstChar(String strValue);
public int getNextEvenInt(int seed);
The "best" use of parameterized tests for implementations of Foo would be...
public class FooTest
@ParameterizedTest
@MethodSource("getFooProvider")
public void getFirstCharTest(Foo foo)
char expectedResult = 'a', 'b', 'c', 'd' ;
String seed = "alpha", "bravo", "charlie", "delta" ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFirstChar(seed[i]));
@ParameterizedTest
@MethodSource("getFooProvider")
public void getNextEvenIntTest(Foo foo)
int expectedResult = 0, 0, 2, 2 ;
int seed = -2, -1, 0, 1 ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFooEvenInt(seed[i]));
private static Stream getFooProvider()
return Stream.of(
Arguments.of(new FooImplOne()),
Arguments.of(new FooImplTwo())
// extend as need for implementations of Foo
);
While I won't get the "warm fuzzies" of seeing the passing results for each value-pair in the various tests, it will fulfill my goal of having a test at the interface level that I can easily extend to validate/verify the interface's implementations.
add a comment |
BLUF: I will interpret the crickets to mean "even if you could, you shouldn't be nesting parameterized tests", in which case I run with the approach outlined below.
For an interface Foo...
public interface Foo
public char getFirstChar(String strValue);
public int getNextEvenInt(int seed);
The "best" use of parameterized tests for implementations of Foo would be...
public class FooTest
@ParameterizedTest
@MethodSource("getFooProvider")
public void getFirstCharTest(Foo foo)
char expectedResult = 'a', 'b', 'c', 'd' ;
String seed = "alpha", "bravo", "charlie", "delta" ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFirstChar(seed[i]));
@ParameterizedTest
@MethodSource("getFooProvider")
public void getNextEvenIntTest(Foo foo)
int expectedResult = 0, 0, 2, 2 ;
int seed = -2, -1, 0, 1 ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFooEvenInt(seed[i]));
private static Stream getFooProvider()
return Stream.of(
Arguments.of(new FooImplOne()),
Arguments.of(new FooImplTwo())
// extend as need for implementations of Foo
);
While I won't get the "warm fuzzies" of seeing the passing results for each value-pair in the various tests, it will fulfill my goal of having a test at the interface level that I can easily extend to validate/verify the interface's implementations.
add a comment |
BLUF: I will interpret the crickets to mean "even if you could, you shouldn't be nesting parameterized tests", in which case I run with the approach outlined below.
For an interface Foo...
public interface Foo
public char getFirstChar(String strValue);
public int getNextEvenInt(int seed);
The "best" use of parameterized tests for implementations of Foo would be...
public class FooTest
@ParameterizedTest
@MethodSource("getFooProvider")
public void getFirstCharTest(Foo foo)
char expectedResult = 'a', 'b', 'c', 'd' ;
String seed = "alpha", "bravo", "charlie", "delta" ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFirstChar(seed[i]));
@ParameterizedTest
@MethodSource("getFooProvider")
public void getNextEvenIntTest(Foo foo)
int expectedResult = 0, 0, 2, 2 ;
int seed = -2, -1, 0, 1 ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFooEvenInt(seed[i]));
private static Stream getFooProvider()
return Stream.of(
Arguments.of(new FooImplOne()),
Arguments.of(new FooImplTwo())
// extend as need for implementations of Foo
);
While I won't get the "warm fuzzies" of seeing the passing results for each value-pair in the various tests, it will fulfill my goal of having a test at the interface level that I can easily extend to validate/verify the interface's implementations.
BLUF: I will interpret the crickets to mean "even if you could, you shouldn't be nesting parameterized tests", in which case I run with the approach outlined below.
For an interface Foo...
public interface Foo
public char getFirstChar(String strValue);
public int getNextEvenInt(int seed);
The "best" use of parameterized tests for implementations of Foo would be...
public class FooTest
@ParameterizedTest
@MethodSource("getFooProvider")
public void getFirstCharTest(Foo foo)
char expectedResult = 'a', 'b', 'c', 'd' ;
String seed = "alpha", "bravo", "charlie", "delta" ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFirstChar(seed[i]));
@ParameterizedTest
@MethodSource("getFooProvider")
public void getNextEvenIntTest(Foo foo)
int expectedResult = 0, 0, 2, 2 ;
int seed = -2, -1, 0, 1 ;
for(int i=0; i<seed.length; i++)
Assertions.assertEquals(expectedResult[i],
foo.getFooEvenInt(seed[i]));
private static Stream getFooProvider()
return Stream.of(
Arguments.of(new FooImplOne()),
Arguments.of(new FooImplTwo())
// extend as need for implementations of Foo
);
While I won't get the "warm fuzzies" of seeing the passing results for each value-pair in the various tests, it will fulfill my goal of having a test at the interface level that I can easily extend to validate/verify the interface's implementations.
edited Nov 21 '18 at 18:53
answered Nov 21 '18 at 16:25
SoCalSoCal
859
859
add a comment |
add a comment |
I guess you think of combining two arguments streams. You could achieve this by creating the cartesian product of two arguments lists.
I have implemented that on https://github.com/joerg-pfruender/junit-goodies/blob/master/src/main/java/com/github/joergpfruender/junitgoodies/ParameterizedTestHelper.java
public static Stream<Arguments> cartesian(Stream a, Stream b)
List argumentsA = (List) a.collect(Collectors.toList());
List argumentsB = (List) b.collect(Collectors.toList());
List<Arguments> result = new ArrayList();
for (Object o : argumentsA)
Object objects = asArray(o);
for (Object o1 : argumentsB)
Object objects1 = asArray(o1);
Object arguments = ArrayUtils.addAll(objects, objects1);
result.add(Arguments.of(arguments));
return result.stream();
private static Object asArray(Object o)
Object objects;
if (o instanceof Arguments)
objects = ((Arguments) o).get();
else
objects = new Objecto;
return objects;
Then your test code will be:
public static Stream<Arguments> fooIntsAndFooProvider()
return ParameterizedTestHelper.cartesian(getFooEvenIntProvider(), getFooProvider());
@ParameterizedTest
@MethodSource("fooIntsAndFooProvider")
public void getFooImplEvenIntTest(Integer seed, Integer expectedResult, Foo foo)
Assertions.assertEquals(expectedResult,
foo.getFooEvenInt(seed));
add a comment |
I guess you think of combining two arguments streams. You could achieve this by creating the cartesian product of two arguments lists.
I have implemented that on https://github.com/joerg-pfruender/junit-goodies/blob/master/src/main/java/com/github/joergpfruender/junitgoodies/ParameterizedTestHelper.java
public static Stream<Arguments> cartesian(Stream a, Stream b)
List argumentsA = (List) a.collect(Collectors.toList());
List argumentsB = (List) b.collect(Collectors.toList());
List<Arguments> result = new ArrayList();
for (Object o : argumentsA)
Object objects = asArray(o);
for (Object o1 : argumentsB)
Object objects1 = asArray(o1);
Object arguments = ArrayUtils.addAll(objects, objects1);
result.add(Arguments.of(arguments));
return result.stream();
private static Object asArray(Object o)
Object objects;
if (o instanceof Arguments)
objects = ((Arguments) o).get();
else
objects = new Objecto;
return objects;
Then your test code will be:
public static Stream<Arguments> fooIntsAndFooProvider()
return ParameterizedTestHelper.cartesian(getFooEvenIntProvider(), getFooProvider());
@ParameterizedTest
@MethodSource("fooIntsAndFooProvider")
public void getFooImplEvenIntTest(Integer seed, Integer expectedResult, Foo foo)
Assertions.assertEquals(expectedResult,
foo.getFooEvenInt(seed));
add a comment |
I guess you think of combining two arguments streams. You could achieve this by creating the cartesian product of two arguments lists.
I have implemented that on https://github.com/joerg-pfruender/junit-goodies/blob/master/src/main/java/com/github/joergpfruender/junitgoodies/ParameterizedTestHelper.java
public static Stream<Arguments> cartesian(Stream a, Stream b)
List argumentsA = (List) a.collect(Collectors.toList());
List argumentsB = (List) b.collect(Collectors.toList());
List<Arguments> result = new ArrayList();
for (Object o : argumentsA)
Object objects = asArray(o);
for (Object o1 : argumentsB)
Object objects1 = asArray(o1);
Object arguments = ArrayUtils.addAll(objects, objects1);
result.add(Arguments.of(arguments));
return result.stream();
private static Object asArray(Object o)
Object objects;
if (o instanceof Arguments)
objects = ((Arguments) o).get();
else
objects = new Objecto;
return objects;
Then your test code will be:
public static Stream<Arguments> fooIntsAndFooProvider()
return ParameterizedTestHelper.cartesian(getFooEvenIntProvider(), getFooProvider());
@ParameterizedTest
@MethodSource("fooIntsAndFooProvider")
public void getFooImplEvenIntTest(Integer seed, Integer expectedResult, Foo foo)
Assertions.assertEquals(expectedResult,
foo.getFooEvenInt(seed));
I guess you think of combining two arguments streams. You could achieve this by creating the cartesian product of two arguments lists.
I have implemented that on https://github.com/joerg-pfruender/junit-goodies/blob/master/src/main/java/com/github/joergpfruender/junitgoodies/ParameterizedTestHelper.java
public static Stream<Arguments> cartesian(Stream a, Stream b)
List argumentsA = (List) a.collect(Collectors.toList());
List argumentsB = (List) b.collect(Collectors.toList());
List<Arguments> result = new ArrayList();
for (Object o : argumentsA)
Object objects = asArray(o);
for (Object o1 : argumentsB)
Object objects1 = asArray(o1);
Object arguments = ArrayUtils.addAll(objects, objects1);
result.add(Arguments.of(arguments));
return result.stream();
private static Object asArray(Object o)
Object objects;
if (o instanceof Arguments)
objects = ((Arguments) o).get();
else
objects = new Objecto;
return objects;
Then your test code will be:
public static Stream<Arguments> fooIntsAndFooProvider()
return ParameterizedTestHelper.cartesian(getFooEvenIntProvider(), getFooProvider());
@ParameterizedTest
@MethodSource("fooIntsAndFooProvider")
public void getFooImplEvenIntTest(Integer seed, Integer expectedResult, Foo foo)
Assertions.assertEquals(expectedResult,
foo.getFooEvenInt(seed));
answered Feb 27 at 22:47


Jörg PfründerJörg Pfründer
14124
14124
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53327761%2fis-it-possible-to-nest-junit-5-parameterized-tests%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uMAsWGdYA2r9pDCqdx92yB57mTBG,yzNZmbGDFRbF 0