Keep a table column at the earliest related value of a column in another table
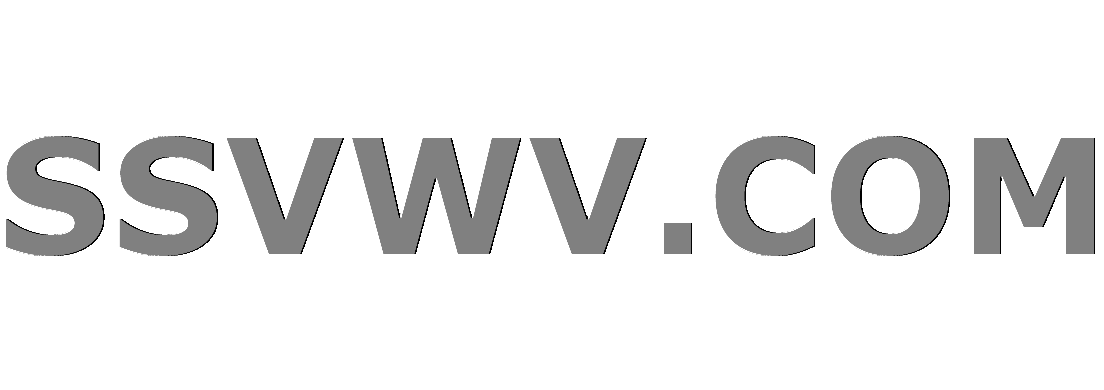
Multi tool use
I have two related tables. Lets call them event_types
and occurrences
with the following columns:
event_types
-------------
id VARCHAR(30)
name VARCHAR(50)
first_event Timestamp
and
occurrences
-------------
id VARCHAR(30)
event_type_id VARCHAR(30)
timestamp Timestamp
postal_number Integer
city VARCHAR(50)
street VARCHAR(50)
I want to add a trigger which updates the first_event
in case a new occurrence
comes up with an earlier timestamp
.
Use case: The reason is that I need to query this event_type
table frequently with conditions on the first_event
and sort it by this field to get the ones inside a time frame.
I tried with something like this:
CREATE OR REPLACE FUNCTION set_to_minimum_time() RETURNS TRIGGER AS
$$
BEGIN
IF NEW.timestamp < SELECT first_event from event_types where id=NEW.event_type_id THEN
UPDATE event_types
SET first_event = NEW.timestamp
WHERE id = NEW.event_type_id
END IF
END;
$$
LANGUAGE PLPGSQL;
CREATE TRIGGER after_trigger_time_updater
AFTER INSERT OR UPDATE OR DELETE
ON occurrences
FOR EACH ROW
EXECUTE PROCEDURE set_to_minimum_time();
This fails already due to the select statement in the if clause with syntax error. So how can I achieve such an update via trigger?
I am using Postgres > 9.5 and 10.
One further note: Such updates on occurrences won't come very often.
postgresql triggers postgresql-triggers
add a comment |
I have two related tables. Lets call them event_types
and occurrences
with the following columns:
event_types
-------------
id VARCHAR(30)
name VARCHAR(50)
first_event Timestamp
and
occurrences
-------------
id VARCHAR(30)
event_type_id VARCHAR(30)
timestamp Timestamp
postal_number Integer
city VARCHAR(50)
street VARCHAR(50)
I want to add a trigger which updates the first_event
in case a new occurrence
comes up with an earlier timestamp
.
Use case: The reason is that I need to query this event_type
table frequently with conditions on the first_event
and sort it by this field to get the ones inside a time frame.
I tried with something like this:
CREATE OR REPLACE FUNCTION set_to_minimum_time() RETURNS TRIGGER AS
$$
BEGIN
IF NEW.timestamp < SELECT first_event from event_types where id=NEW.event_type_id THEN
UPDATE event_types
SET first_event = NEW.timestamp
WHERE id = NEW.event_type_id
END IF
END;
$$
LANGUAGE PLPGSQL;
CREATE TRIGGER after_trigger_time_updater
AFTER INSERT OR UPDATE OR DELETE
ON occurrences
FOR EACH ROW
EXECUTE PROCEDURE set_to_minimum_time();
This fails already due to the select statement in the if clause with syntax error. So how can I achieve such an update via trigger?
I am using Postgres > 9.5 and 10.
One further note: Such updates on occurrences won't come very often.
postgresql triggers postgresql-triggers
add a comment |
I have two related tables. Lets call them event_types
and occurrences
with the following columns:
event_types
-------------
id VARCHAR(30)
name VARCHAR(50)
first_event Timestamp
and
occurrences
-------------
id VARCHAR(30)
event_type_id VARCHAR(30)
timestamp Timestamp
postal_number Integer
city VARCHAR(50)
street VARCHAR(50)
I want to add a trigger which updates the first_event
in case a new occurrence
comes up with an earlier timestamp
.
Use case: The reason is that I need to query this event_type
table frequently with conditions on the first_event
and sort it by this field to get the ones inside a time frame.
I tried with something like this:
CREATE OR REPLACE FUNCTION set_to_minimum_time() RETURNS TRIGGER AS
$$
BEGIN
IF NEW.timestamp < SELECT first_event from event_types where id=NEW.event_type_id THEN
UPDATE event_types
SET first_event = NEW.timestamp
WHERE id = NEW.event_type_id
END IF
END;
$$
LANGUAGE PLPGSQL;
CREATE TRIGGER after_trigger_time_updater
AFTER INSERT OR UPDATE OR DELETE
ON occurrences
FOR EACH ROW
EXECUTE PROCEDURE set_to_minimum_time();
This fails already due to the select statement in the if clause with syntax error. So how can I achieve such an update via trigger?
I am using Postgres > 9.5 and 10.
One further note: Such updates on occurrences won't come very often.
postgresql triggers postgresql-triggers
I have two related tables. Lets call them event_types
and occurrences
with the following columns:
event_types
-------------
id VARCHAR(30)
name VARCHAR(50)
first_event Timestamp
and
occurrences
-------------
id VARCHAR(30)
event_type_id VARCHAR(30)
timestamp Timestamp
postal_number Integer
city VARCHAR(50)
street VARCHAR(50)
I want to add a trigger which updates the first_event
in case a new occurrence
comes up with an earlier timestamp
.
Use case: The reason is that I need to query this event_type
table frequently with conditions on the first_event
and sort it by this field to get the ones inside a time frame.
I tried with something like this:
CREATE OR REPLACE FUNCTION set_to_minimum_time() RETURNS TRIGGER AS
$$
BEGIN
IF NEW.timestamp < SELECT first_event from event_types where id=NEW.event_type_id THEN
UPDATE event_types
SET first_event = NEW.timestamp
WHERE id = NEW.event_type_id
END IF
END;
$$
LANGUAGE PLPGSQL;
CREATE TRIGGER after_trigger_time_updater
AFTER INSERT OR UPDATE OR DELETE
ON occurrences
FOR EACH ROW
EXECUTE PROCEDURE set_to_minimum_time();
This fails already due to the select statement in the if clause with syntax error. So how can I achieve such an update via trigger?
I am using Postgres > 9.5 and 10.
One further note: Such updates on occurrences won't come very often.
postgresql triggers postgresql-triggers
postgresql triggers postgresql-triggers
edited Nov 16 '18 at 9:37
Stoecki
asked Nov 15 '18 at 15:46
StoeckiStoecki
15019
15019
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You could avoid the syntax error by putting parentheses around the SELECT
statement.
But you made another mistake: you defined the trigger ON DELETE
, and there is no NEW
record in that case. It doesn't make sense to have this trigger ON DELETE
anyway, does it?
The SQL statement in the trigger could be simpler:
UPDATE event_types
SET first_event = NEW.timestamp
WHERE id = NEW.event_type_id
AND first_event < NEW.timestamp;
That performs the check in the same statement.
That should have come to my mind as well. Maybe it was just a long day. Thank you. Final solution in edit
– Stoecki
Nov 16 '18 at 9:32
It is better to post your final solution as an answer, that is less confusing.
– Laurenz Albe
Nov 16 '18 at 9:36
Yes, you are right
– Stoecki
Nov 16 '18 at 9:39
add a comment |
My final solution after Laurenz Albe's help:
CREATE OR REPLACE FUNCTION maybe_update_event_type_time() RETURNS TRIGGER AS $maybe_update_event_type_time$
BEGIN
UPDATE event_types t
SET t.first_event = NEW.timestamp
WHERE t.id = NEW.event_type_id
AND t.first_event > NEW.timestamp;
RETURN NULL;
END;
$maybe_update_event_type_time$ LANGUAGE PLPGSQL;
CREATE TRIGGER after_trigger_anomaly_time_updater
AFTER INSERT OR UPDATE
ON occurrences
FOR EACH ROW
EXECUTE PROCEDURE maybe_update_event_type_time();
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323057%2fkeep-a-table-column-at-the-earliest-related-value-of-a-column-in-another-table%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could avoid the syntax error by putting parentheses around the SELECT
statement.
But you made another mistake: you defined the trigger ON DELETE
, and there is no NEW
record in that case. It doesn't make sense to have this trigger ON DELETE
anyway, does it?
The SQL statement in the trigger could be simpler:
UPDATE event_types
SET first_event = NEW.timestamp
WHERE id = NEW.event_type_id
AND first_event < NEW.timestamp;
That performs the check in the same statement.
That should have come to my mind as well. Maybe it was just a long day. Thank you. Final solution in edit
– Stoecki
Nov 16 '18 at 9:32
It is better to post your final solution as an answer, that is less confusing.
– Laurenz Albe
Nov 16 '18 at 9:36
Yes, you are right
– Stoecki
Nov 16 '18 at 9:39
add a comment |
You could avoid the syntax error by putting parentheses around the SELECT
statement.
But you made another mistake: you defined the trigger ON DELETE
, and there is no NEW
record in that case. It doesn't make sense to have this trigger ON DELETE
anyway, does it?
The SQL statement in the trigger could be simpler:
UPDATE event_types
SET first_event = NEW.timestamp
WHERE id = NEW.event_type_id
AND first_event < NEW.timestamp;
That performs the check in the same statement.
That should have come to my mind as well. Maybe it was just a long day. Thank you. Final solution in edit
– Stoecki
Nov 16 '18 at 9:32
It is better to post your final solution as an answer, that is less confusing.
– Laurenz Albe
Nov 16 '18 at 9:36
Yes, you are right
– Stoecki
Nov 16 '18 at 9:39
add a comment |
You could avoid the syntax error by putting parentheses around the SELECT
statement.
But you made another mistake: you defined the trigger ON DELETE
, and there is no NEW
record in that case. It doesn't make sense to have this trigger ON DELETE
anyway, does it?
The SQL statement in the trigger could be simpler:
UPDATE event_types
SET first_event = NEW.timestamp
WHERE id = NEW.event_type_id
AND first_event < NEW.timestamp;
That performs the check in the same statement.
You could avoid the syntax error by putting parentheses around the SELECT
statement.
But you made another mistake: you defined the trigger ON DELETE
, and there is no NEW
record in that case. It doesn't make sense to have this trigger ON DELETE
anyway, does it?
The SQL statement in the trigger could be simpler:
UPDATE event_types
SET first_event = NEW.timestamp
WHERE id = NEW.event_type_id
AND first_event < NEW.timestamp;
That performs the check in the same statement.
answered Nov 15 '18 at 16:14


Laurenz AlbeLaurenz Albe
48.9k102848
48.9k102848
That should have come to my mind as well. Maybe it was just a long day. Thank you. Final solution in edit
– Stoecki
Nov 16 '18 at 9:32
It is better to post your final solution as an answer, that is less confusing.
– Laurenz Albe
Nov 16 '18 at 9:36
Yes, you are right
– Stoecki
Nov 16 '18 at 9:39
add a comment |
That should have come to my mind as well. Maybe it was just a long day. Thank you. Final solution in edit
– Stoecki
Nov 16 '18 at 9:32
It is better to post your final solution as an answer, that is less confusing.
– Laurenz Albe
Nov 16 '18 at 9:36
Yes, you are right
– Stoecki
Nov 16 '18 at 9:39
That should have come to my mind as well. Maybe it was just a long day. Thank you. Final solution in edit
– Stoecki
Nov 16 '18 at 9:32
That should have come to my mind as well. Maybe it was just a long day. Thank you. Final solution in edit
– Stoecki
Nov 16 '18 at 9:32
It is better to post your final solution as an answer, that is less confusing.
– Laurenz Albe
Nov 16 '18 at 9:36
It is better to post your final solution as an answer, that is less confusing.
– Laurenz Albe
Nov 16 '18 at 9:36
Yes, you are right
– Stoecki
Nov 16 '18 at 9:39
Yes, you are right
– Stoecki
Nov 16 '18 at 9:39
add a comment |
My final solution after Laurenz Albe's help:
CREATE OR REPLACE FUNCTION maybe_update_event_type_time() RETURNS TRIGGER AS $maybe_update_event_type_time$
BEGIN
UPDATE event_types t
SET t.first_event = NEW.timestamp
WHERE t.id = NEW.event_type_id
AND t.first_event > NEW.timestamp;
RETURN NULL;
END;
$maybe_update_event_type_time$ LANGUAGE PLPGSQL;
CREATE TRIGGER after_trigger_anomaly_time_updater
AFTER INSERT OR UPDATE
ON occurrences
FOR EACH ROW
EXECUTE PROCEDURE maybe_update_event_type_time();
add a comment |
My final solution after Laurenz Albe's help:
CREATE OR REPLACE FUNCTION maybe_update_event_type_time() RETURNS TRIGGER AS $maybe_update_event_type_time$
BEGIN
UPDATE event_types t
SET t.first_event = NEW.timestamp
WHERE t.id = NEW.event_type_id
AND t.first_event > NEW.timestamp;
RETURN NULL;
END;
$maybe_update_event_type_time$ LANGUAGE PLPGSQL;
CREATE TRIGGER after_trigger_anomaly_time_updater
AFTER INSERT OR UPDATE
ON occurrences
FOR EACH ROW
EXECUTE PROCEDURE maybe_update_event_type_time();
add a comment |
My final solution after Laurenz Albe's help:
CREATE OR REPLACE FUNCTION maybe_update_event_type_time() RETURNS TRIGGER AS $maybe_update_event_type_time$
BEGIN
UPDATE event_types t
SET t.first_event = NEW.timestamp
WHERE t.id = NEW.event_type_id
AND t.first_event > NEW.timestamp;
RETURN NULL;
END;
$maybe_update_event_type_time$ LANGUAGE PLPGSQL;
CREATE TRIGGER after_trigger_anomaly_time_updater
AFTER INSERT OR UPDATE
ON occurrences
FOR EACH ROW
EXECUTE PROCEDURE maybe_update_event_type_time();
My final solution after Laurenz Albe's help:
CREATE OR REPLACE FUNCTION maybe_update_event_type_time() RETURNS TRIGGER AS $maybe_update_event_type_time$
BEGIN
UPDATE event_types t
SET t.first_event = NEW.timestamp
WHERE t.id = NEW.event_type_id
AND t.first_event > NEW.timestamp;
RETURN NULL;
END;
$maybe_update_event_type_time$ LANGUAGE PLPGSQL;
CREATE TRIGGER after_trigger_anomaly_time_updater
AFTER INSERT OR UPDATE
ON occurrences
FOR EACH ROW
EXECUTE PROCEDURE maybe_update_event_type_time();
answered Nov 16 '18 at 9:39
StoeckiStoecki
15019
15019
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53323057%2fkeep-a-table-column-at-the-earliest-related-value-of-a-column-in-another-table%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DPf9ZcHh1eRMUs I wo8y,qjUxvZrzHkeKGWc85