Anime.js adding consequential animations using canvas
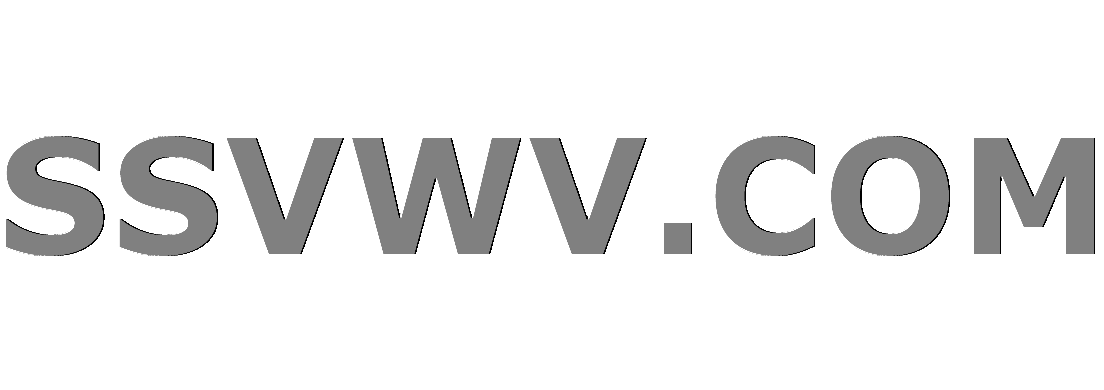
Multi tool use
up vote
1
down vote
favorite
I'm trying to make a simple interaction using canvas and Anime.js, using a modified version of one of Julian's examples. The idea is that a circle would enlarge on screen, then the user clicks anywhere on the screen and the circle would contract. I can't get the second animation to play, not sure if this is because my target is not valid or something to do with the timeline? Here is the example.
//Canvas set up
var canvas = document.querySelector('.gameCanvas');
var ctx = canvas.getContext('2d');
var pointerX = 0;
var pointerY = 0;
var circle;
let basicTimeline = anime.timeline();
function setCanvasSize()
canvas.width = window.innerWidth * 2;
canvas.height = window.innerHeight * 2;
canvas.style.width = window.innerWidth + 'px';
canvas.style.height = window.innerHeight + 'px';
canvas.getContext('2d').scale(2, 2);
//Objects and animation
function createCircle(x,y)
var p = ;
p.x = x;
p.y = y;
p.color = "#fff";
p.radius = anime.random(16, 32);
p.alpha = .5;
p.draw = function()
ctx.globalAlpha = p.alpha;
ctx.beginPath();
ctx.arc(p.x, p.y, p.radius, 0, 2 * Math.PI, true);
ctx.fillStyle = p.color;
ctx.fill();
return p;
function renderParticle(anim)
for (var i = 0; i < anim.animatables.length; i++)
anim.animatables[i].target.draw();
function createExpandCircle(x, y)
circle = createCircle(x, y);
basicTimeline.add(
targets: circle,
radius: anime.random(80, 160),
alpha:
value: 1,
easing: 'linear',
duration: anime.random(600, 800),
,
duration: anime.random(1200, 1800),
easing: 'linear',
update: renderParticle,
offset: 0
);
function contractCircle()
basicTimeline.add(
targets: circle,
radius: 20,
easing: 'easeOutExpo'
update: renderParticle
);
//Mouse Events
var tap = ('ontouchstart' in window || navigator.msMaxTouchPoints) ? 'touchstart' : 'mousedown';
function updateCoords(e) e.touches[0].clientX;
pointerY = e.clientY
document.addEventListener(tap, function(e)
updateCoords(e);
contractCircle();
, false);
var centerX = window.innerWidth / 2;
var centerY = window.innerHeight / 2;
createExpandCircle(centerX, centerY);
setCanvasSize();
window.addEventListener('resize', setCanvasSize, false);
javascript canvas anime.js
add a comment |
up vote
1
down vote
favorite
I'm trying to make a simple interaction using canvas and Anime.js, using a modified version of one of Julian's examples. The idea is that a circle would enlarge on screen, then the user clicks anywhere on the screen and the circle would contract. I can't get the second animation to play, not sure if this is because my target is not valid or something to do with the timeline? Here is the example.
//Canvas set up
var canvas = document.querySelector('.gameCanvas');
var ctx = canvas.getContext('2d');
var pointerX = 0;
var pointerY = 0;
var circle;
let basicTimeline = anime.timeline();
function setCanvasSize()
canvas.width = window.innerWidth * 2;
canvas.height = window.innerHeight * 2;
canvas.style.width = window.innerWidth + 'px';
canvas.style.height = window.innerHeight + 'px';
canvas.getContext('2d').scale(2, 2);
//Objects and animation
function createCircle(x,y)
var p = ;
p.x = x;
p.y = y;
p.color = "#fff";
p.radius = anime.random(16, 32);
p.alpha = .5;
p.draw = function()
ctx.globalAlpha = p.alpha;
ctx.beginPath();
ctx.arc(p.x, p.y, p.radius, 0, 2 * Math.PI, true);
ctx.fillStyle = p.color;
ctx.fill();
return p;
function renderParticle(anim)
for (var i = 0; i < anim.animatables.length; i++)
anim.animatables[i].target.draw();
function createExpandCircle(x, y)
circle = createCircle(x, y);
basicTimeline.add(
targets: circle,
radius: anime.random(80, 160),
alpha:
value: 1,
easing: 'linear',
duration: anime.random(600, 800),
,
duration: anime.random(1200, 1800),
easing: 'linear',
update: renderParticle,
offset: 0
);
function contractCircle()
basicTimeline.add(
targets: circle,
radius: 20,
easing: 'easeOutExpo'
update: renderParticle
);
//Mouse Events
var tap = ('ontouchstart' in window || navigator.msMaxTouchPoints) ? 'touchstart' : 'mousedown';
function updateCoords(e) e.touches[0].clientX;
pointerY = e.clientY
document.addEventListener(tap, function(e)
updateCoords(e);
contractCircle();
, false);
var centerX = window.innerWidth / 2;
var centerY = window.innerHeight / 2;
createExpandCircle(centerX, centerY);
setCanvasSize();
window.addEventListener('resize', setCanvasSize, false);
javascript canvas anime.js
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to make a simple interaction using canvas and Anime.js, using a modified version of one of Julian's examples. The idea is that a circle would enlarge on screen, then the user clicks anywhere on the screen and the circle would contract. I can't get the second animation to play, not sure if this is because my target is not valid or something to do with the timeline? Here is the example.
//Canvas set up
var canvas = document.querySelector('.gameCanvas');
var ctx = canvas.getContext('2d');
var pointerX = 0;
var pointerY = 0;
var circle;
let basicTimeline = anime.timeline();
function setCanvasSize()
canvas.width = window.innerWidth * 2;
canvas.height = window.innerHeight * 2;
canvas.style.width = window.innerWidth + 'px';
canvas.style.height = window.innerHeight + 'px';
canvas.getContext('2d').scale(2, 2);
//Objects and animation
function createCircle(x,y)
var p = ;
p.x = x;
p.y = y;
p.color = "#fff";
p.radius = anime.random(16, 32);
p.alpha = .5;
p.draw = function()
ctx.globalAlpha = p.alpha;
ctx.beginPath();
ctx.arc(p.x, p.y, p.radius, 0, 2 * Math.PI, true);
ctx.fillStyle = p.color;
ctx.fill();
return p;
function renderParticle(anim)
for (var i = 0; i < anim.animatables.length; i++)
anim.animatables[i].target.draw();
function createExpandCircle(x, y)
circle = createCircle(x, y);
basicTimeline.add(
targets: circle,
radius: anime.random(80, 160),
alpha:
value: 1,
easing: 'linear',
duration: anime.random(600, 800),
,
duration: anime.random(1200, 1800),
easing: 'linear',
update: renderParticle,
offset: 0
);
function contractCircle()
basicTimeline.add(
targets: circle,
radius: 20,
easing: 'easeOutExpo'
update: renderParticle
);
//Mouse Events
var tap = ('ontouchstart' in window || navigator.msMaxTouchPoints) ? 'touchstart' : 'mousedown';
function updateCoords(e) e.touches[0].clientX;
pointerY = e.clientY
document.addEventListener(tap, function(e)
updateCoords(e);
contractCircle();
, false);
var centerX = window.innerWidth / 2;
var centerY = window.innerHeight / 2;
createExpandCircle(centerX, centerY);
setCanvasSize();
window.addEventListener('resize', setCanvasSize, false);
javascript canvas anime.js
I'm trying to make a simple interaction using canvas and Anime.js, using a modified version of one of Julian's examples. The idea is that a circle would enlarge on screen, then the user clicks anywhere on the screen and the circle would contract. I can't get the second animation to play, not sure if this is because my target is not valid or something to do with the timeline? Here is the example.
//Canvas set up
var canvas = document.querySelector('.gameCanvas');
var ctx = canvas.getContext('2d');
var pointerX = 0;
var pointerY = 0;
var circle;
let basicTimeline = anime.timeline();
function setCanvasSize()
canvas.width = window.innerWidth * 2;
canvas.height = window.innerHeight * 2;
canvas.style.width = window.innerWidth + 'px';
canvas.style.height = window.innerHeight + 'px';
canvas.getContext('2d').scale(2, 2);
//Objects and animation
function createCircle(x,y)
var p = ;
p.x = x;
p.y = y;
p.color = "#fff";
p.radius = anime.random(16, 32);
p.alpha = .5;
p.draw = function()
ctx.globalAlpha = p.alpha;
ctx.beginPath();
ctx.arc(p.x, p.y, p.radius, 0, 2 * Math.PI, true);
ctx.fillStyle = p.color;
ctx.fill();
return p;
function renderParticle(anim)
for (var i = 0; i < anim.animatables.length; i++)
anim.animatables[i].target.draw();
function createExpandCircle(x, y)
circle = createCircle(x, y);
basicTimeline.add(
targets: circle,
radius: anime.random(80, 160),
alpha:
value: 1,
easing: 'linear',
duration: anime.random(600, 800),
,
duration: anime.random(1200, 1800),
easing: 'linear',
update: renderParticle,
offset: 0
);
function contractCircle()
basicTimeline.add(
targets: circle,
radius: 20,
easing: 'easeOutExpo'
update: renderParticle
);
//Mouse Events
var tap = ('ontouchstart' in window || navigator.msMaxTouchPoints) ? 'touchstart' : 'mousedown';
function updateCoords(e) e.touches[0].clientX;
pointerY = e.clientY
document.addEventListener(tap, function(e)
updateCoords(e);
contractCircle();
, false);
var centerX = window.innerWidth / 2;
var centerY = window.innerHeight / 2;
createExpandCircle(centerX, centerY);
setCanvasSize();
window.addEventListener('resize', setCanvasSize, false);
javascript canvas anime.js
javascript canvas anime.js
asked Nov 10 at 15:41
xsk12
61
61
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
So I was able to solve this by adding the following:
ctx.clearRect(0, 0, canvas.width, canvas.height);
To p.draw, and
basicTimeline = anime.timeline();
To contractCircle.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
So I was able to solve this by adding the following:
ctx.clearRect(0, 0, canvas.width, canvas.height);
To p.draw, and
basicTimeline = anime.timeline();
To contractCircle.
add a comment |
up vote
0
down vote
So I was able to solve this by adding the following:
ctx.clearRect(0, 0, canvas.width, canvas.height);
To p.draw, and
basicTimeline = anime.timeline();
To contractCircle.
add a comment |
up vote
0
down vote
up vote
0
down vote
So I was able to solve this by adding the following:
ctx.clearRect(0, 0, canvas.width, canvas.height);
To p.draw, and
basicTimeline = anime.timeline();
To contractCircle.
So I was able to solve this by adding the following:
ctx.clearRect(0, 0, canvas.width, canvas.height);
To p.draw, and
basicTimeline = anime.timeline();
To contractCircle.
answered Nov 10 at 18:35
xsk12
61
61
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240541%2fanime-js-adding-consequential-animations-using-canvas%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6VJiZN1Kfxpr 1kCgEip2c0TkXrAjMDKE2rqtyR 3YK Pd42PufYX,CS5B VtCvRwZKQh