Better way to reorder list of dictionaries?
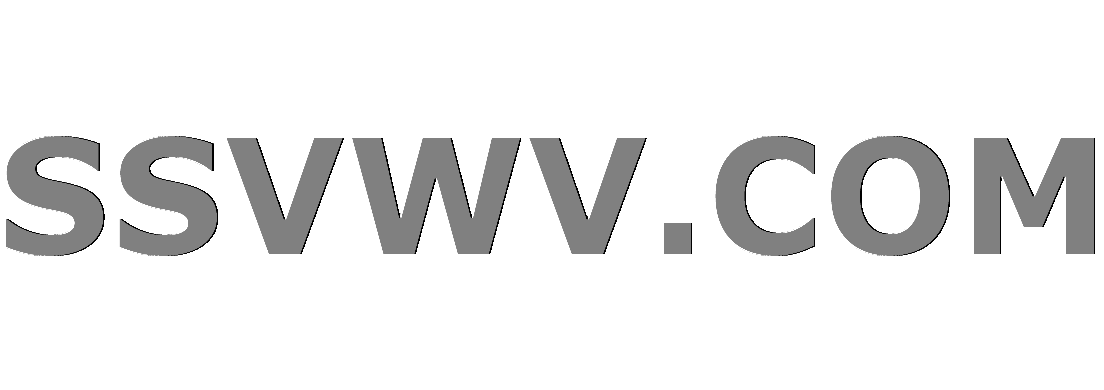
Multi tool use
up vote
3
down vote
favorite
So I have a small data like this:
data = [
"Name":"Arab","Code":"Zl",
"Name":"Korea","Code":"Bl",
"Name":"China","Code":"Bz"
]
I want to find a graph so that the x-axis is: "Bl", "Bz", "Zl" (alphabetic order)
and the y-axis is: "Korea", "China", "Arab" (corresponding to the codenames).
I thought of:
new_data =
for dic in data:
country_data = dic["Name"]
code_data = dic["Code"]
new_data[code_data] = country_data
code_data =
for codes in new_data.keys():
code_data.append(codes)
code_data.sort()
name_data =
for code in code_data:
name_data.append(new_data[code])
Is there a better way to do this?
Perhaps by not creating a new dictionary?
python python-3.x list sorting dictionary
add a comment |
up vote
3
down vote
favorite
So I have a small data like this:
data = [
"Name":"Arab","Code":"Zl",
"Name":"Korea","Code":"Bl",
"Name":"China","Code":"Bz"
]
I want to find a graph so that the x-axis is: "Bl", "Bz", "Zl" (alphabetic order)
and the y-axis is: "Korea", "China", "Arab" (corresponding to the codenames).
I thought of:
new_data =
for dic in data:
country_data = dic["Name"]
code_data = dic["Code"]
new_data[code_data] = country_data
code_data =
for codes in new_data.keys():
code_data.append(codes)
code_data.sort()
name_data =
for code in code_data:
name_data.append(new_data[code])
Is there a better way to do this?
Perhaps by not creating a new dictionary?
python python-3.x list sorting dictionary
pairs = sorted((d['Code'], d['Name']) for d in data)
– FMc
Nov 10 at 8:12
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
So I have a small data like this:
data = [
"Name":"Arab","Code":"Zl",
"Name":"Korea","Code":"Bl",
"Name":"China","Code":"Bz"
]
I want to find a graph so that the x-axis is: "Bl", "Bz", "Zl" (alphabetic order)
and the y-axis is: "Korea", "China", "Arab" (corresponding to the codenames).
I thought of:
new_data =
for dic in data:
country_data = dic["Name"]
code_data = dic["Code"]
new_data[code_data] = country_data
code_data =
for codes in new_data.keys():
code_data.append(codes)
code_data.sort()
name_data =
for code in code_data:
name_data.append(new_data[code])
Is there a better way to do this?
Perhaps by not creating a new dictionary?
python python-3.x list sorting dictionary
So I have a small data like this:
data = [
"Name":"Arab","Code":"Zl",
"Name":"Korea","Code":"Bl",
"Name":"China","Code":"Bz"
]
I want to find a graph so that the x-axis is: "Bl", "Bz", "Zl" (alphabetic order)
and the y-axis is: "Korea", "China", "Arab" (corresponding to the codenames).
I thought of:
new_data =
for dic in data:
country_data = dic["Name"]
code_data = dic["Code"]
new_data[code_data] = country_data
code_data =
for codes in new_data.keys():
code_data.append(codes)
code_data.sort()
name_data =
for code in code_data:
name_data.append(new_data[code])
Is there a better way to do this?
Perhaps by not creating a new dictionary?
python python-3.x list sorting dictionary
python python-3.x list sorting dictionary
edited Nov 10 at 18:07


jpp
81.8k194795
81.8k194795
asked Nov 10 at 7:20
Kun Hwi Ko
161
161
pairs = sorted((d['Code'], d['Name']) for d in data)
– FMc
Nov 10 at 8:12
add a comment |
pairs = sorted((d['Code'], d['Name']) for d in data)
– FMc
Nov 10 at 8:12
pairs = sorted((d['Code'], d['Name']) for d in data)
– FMc
Nov 10 at 8:12
pairs = sorted((d['Code'], d['Name']) for d in data)
– FMc
Nov 10 at 8:12
add a comment |
3 Answers
3
active
oldest
votes
up vote
1
down vote
So here's the data:
data = [
"Name":"Arab","Code":"Zl",
"Name":"Korea","Code":"Bl",
"Name":"China","Code":"Bz"
]
To create a new sorted list:
new_list = sorted(data, key=lambda k: k['Code'])
If you don't want to get a new list:
data[:] = sorted(data, key=lambda k: k['Code'])
The result is:
['Code': 'Bl', 'Name': 'Korea', 'Code': 'Bz', 'Name': 'China', 'Code': 'Zl', 'Name': 'Arab']
I hope I could help you!
The second version also creates a new list object. It just binds this new list to the old variable. If you really want to mutate the old list object, use slice assignmentdata[:] = sorted(data, key=lambda k: k['Code'])
.
– schwobaseggl
Nov 10 at 10:07
Yes but it's the same effect
– T.Wimma
Nov 10 at 11:57
Imagine that code in a function with data being passed as an argument. The effect visible to the caller will be vastly different. Also, the "not a new list" statement in itself is just plain wrong.
– schwobaseggl
Nov 10 at 16:41
add a comment |
up vote
0
down vote
Better way to produce same results:
from operator import itemgetter
data = [
"Name": "Arab", "Code": "Zl",
"Name": "Korea", "Code": "Bl",
"Name": "China", "Code": "Bz"
]
sorted_data = ((d["Code"], d["Name"]) for d in sorted(data, key=itemgetter("Code")))
code_data, name_data = (list(item) for item in zip(*sorted_data))
print(code_data) # -> ['Bl', 'Bz', 'Zl']
print(name_data) # -> ['Korea', 'China', 'Arab']
add a comment |
up vote
0
down vote
Here's one way using operator.itemgetter
and unpacking via zip
:
from operator import itemgetter
_, data_sorted = zip(*sorted(enumerate(data), key=lambda x: x[1]['Code']))
codes, names = zip(*map(itemgetter('Code', 'Name'), data_sorted))
print(codes)
# ('Bl', 'Bz', 'Zl')
print(names)
# ('Korea', 'China', 'Arab')
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
So here's the data:
data = [
"Name":"Arab","Code":"Zl",
"Name":"Korea","Code":"Bl",
"Name":"China","Code":"Bz"
]
To create a new sorted list:
new_list = sorted(data, key=lambda k: k['Code'])
If you don't want to get a new list:
data[:] = sorted(data, key=lambda k: k['Code'])
The result is:
['Code': 'Bl', 'Name': 'Korea', 'Code': 'Bz', 'Name': 'China', 'Code': 'Zl', 'Name': 'Arab']
I hope I could help you!
The second version also creates a new list object. It just binds this new list to the old variable. If you really want to mutate the old list object, use slice assignmentdata[:] = sorted(data, key=lambda k: k['Code'])
.
– schwobaseggl
Nov 10 at 10:07
Yes but it's the same effect
– T.Wimma
Nov 10 at 11:57
Imagine that code in a function with data being passed as an argument. The effect visible to the caller will be vastly different. Also, the "not a new list" statement in itself is just plain wrong.
– schwobaseggl
Nov 10 at 16:41
add a comment |
up vote
1
down vote
So here's the data:
data = [
"Name":"Arab","Code":"Zl",
"Name":"Korea","Code":"Bl",
"Name":"China","Code":"Bz"
]
To create a new sorted list:
new_list = sorted(data, key=lambda k: k['Code'])
If you don't want to get a new list:
data[:] = sorted(data, key=lambda k: k['Code'])
The result is:
['Code': 'Bl', 'Name': 'Korea', 'Code': 'Bz', 'Name': 'China', 'Code': 'Zl', 'Name': 'Arab']
I hope I could help you!
The second version also creates a new list object. It just binds this new list to the old variable. If you really want to mutate the old list object, use slice assignmentdata[:] = sorted(data, key=lambda k: k['Code'])
.
– schwobaseggl
Nov 10 at 10:07
Yes but it's the same effect
– T.Wimma
Nov 10 at 11:57
Imagine that code in a function with data being passed as an argument. The effect visible to the caller will be vastly different. Also, the "not a new list" statement in itself is just plain wrong.
– schwobaseggl
Nov 10 at 16:41
add a comment |
up vote
1
down vote
up vote
1
down vote
So here's the data:
data = [
"Name":"Arab","Code":"Zl",
"Name":"Korea","Code":"Bl",
"Name":"China","Code":"Bz"
]
To create a new sorted list:
new_list = sorted(data, key=lambda k: k['Code'])
If you don't want to get a new list:
data[:] = sorted(data, key=lambda k: k['Code'])
The result is:
['Code': 'Bl', 'Name': 'Korea', 'Code': 'Bz', 'Name': 'China', 'Code': 'Zl', 'Name': 'Arab']
I hope I could help you!
So here's the data:
data = [
"Name":"Arab","Code":"Zl",
"Name":"Korea","Code":"Bl",
"Name":"China","Code":"Bz"
]
To create a new sorted list:
new_list = sorted(data, key=lambda k: k['Code'])
If you don't want to get a new list:
data[:] = sorted(data, key=lambda k: k['Code'])
The result is:
['Code': 'Bl', 'Name': 'Korea', 'Code': 'Bz', 'Name': 'China', 'Code': 'Zl', 'Name': 'Arab']
I hope I could help you!
edited Nov 10 at 17:45
answered Nov 10 at 8:01
T.Wimma
916
916
The second version also creates a new list object. It just binds this new list to the old variable. If you really want to mutate the old list object, use slice assignmentdata[:] = sorted(data, key=lambda k: k['Code'])
.
– schwobaseggl
Nov 10 at 10:07
Yes but it's the same effect
– T.Wimma
Nov 10 at 11:57
Imagine that code in a function with data being passed as an argument. The effect visible to the caller will be vastly different. Also, the "not a new list" statement in itself is just plain wrong.
– schwobaseggl
Nov 10 at 16:41
add a comment |
The second version also creates a new list object. It just binds this new list to the old variable. If you really want to mutate the old list object, use slice assignmentdata[:] = sorted(data, key=lambda k: k['Code'])
.
– schwobaseggl
Nov 10 at 10:07
Yes but it's the same effect
– T.Wimma
Nov 10 at 11:57
Imagine that code in a function with data being passed as an argument. The effect visible to the caller will be vastly different. Also, the "not a new list" statement in itself is just plain wrong.
– schwobaseggl
Nov 10 at 16:41
The second version also creates a new list object. It just binds this new list to the old variable. If you really want to mutate the old list object, use slice assignment
data[:] = sorted(data, key=lambda k: k['Code'])
.– schwobaseggl
Nov 10 at 10:07
The second version also creates a new list object. It just binds this new list to the old variable. If you really want to mutate the old list object, use slice assignment
data[:] = sorted(data, key=lambda k: k['Code'])
.– schwobaseggl
Nov 10 at 10:07
Yes but it's the same effect
– T.Wimma
Nov 10 at 11:57
Yes but it's the same effect
– T.Wimma
Nov 10 at 11:57
Imagine that code in a function with data being passed as an argument. The effect visible to the caller will be vastly different. Also, the "not a new list" statement in itself is just plain wrong.
– schwobaseggl
Nov 10 at 16:41
Imagine that code in a function with data being passed as an argument. The effect visible to the caller will be vastly different. Also, the "not a new list" statement in itself is just plain wrong.
– schwobaseggl
Nov 10 at 16:41
add a comment |
up vote
0
down vote
Better way to produce same results:
from operator import itemgetter
data = [
"Name": "Arab", "Code": "Zl",
"Name": "Korea", "Code": "Bl",
"Name": "China", "Code": "Bz"
]
sorted_data = ((d["Code"], d["Name"]) for d in sorted(data, key=itemgetter("Code")))
code_data, name_data = (list(item) for item in zip(*sorted_data))
print(code_data) # -> ['Bl', 'Bz', 'Zl']
print(name_data) # -> ['Korea', 'China', 'Arab']
add a comment |
up vote
0
down vote
Better way to produce same results:
from operator import itemgetter
data = [
"Name": "Arab", "Code": "Zl",
"Name": "Korea", "Code": "Bl",
"Name": "China", "Code": "Bz"
]
sorted_data = ((d["Code"], d["Name"]) for d in sorted(data, key=itemgetter("Code")))
code_data, name_data = (list(item) for item in zip(*sorted_data))
print(code_data) # -> ['Bl', 'Bz', 'Zl']
print(name_data) # -> ['Korea', 'China', 'Arab']
add a comment |
up vote
0
down vote
up vote
0
down vote
Better way to produce same results:
from operator import itemgetter
data = [
"Name": "Arab", "Code": "Zl",
"Name": "Korea", "Code": "Bl",
"Name": "China", "Code": "Bz"
]
sorted_data = ((d["Code"], d["Name"]) for d in sorted(data, key=itemgetter("Code")))
code_data, name_data = (list(item) for item in zip(*sorted_data))
print(code_data) # -> ['Bl', 'Bz', 'Zl']
print(name_data) # -> ['Korea', 'China', 'Arab']
Better way to produce same results:
from operator import itemgetter
data = [
"Name": "Arab", "Code": "Zl",
"Name": "Korea", "Code": "Bl",
"Name": "China", "Code": "Bz"
]
sorted_data = ((d["Code"], d["Name"]) for d in sorted(data, key=itemgetter("Code")))
code_data, name_data = (list(item) for item in zip(*sorted_data))
print(code_data) # -> ['Bl', 'Bz', 'Zl']
print(name_data) # -> ['Korea', 'China', 'Arab']
answered Nov 10 at 10:22


martineau
64.4k887170
64.4k887170
add a comment |
add a comment |
up vote
0
down vote
Here's one way using operator.itemgetter
and unpacking via zip
:
from operator import itemgetter
_, data_sorted = zip(*sorted(enumerate(data), key=lambda x: x[1]['Code']))
codes, names = zip(*map(itemgetter('Code', 'Name'), data_sorted))
print(codes)
# ('Bl', 'Bz', 'Zl')
print(names)
# ('Korea', 'China', 'Arab')
add a comment |
up vote
0
down vote
Here's one way using operator.itemgetter
and unpacking via zip
:
from operator import itemgetter
_, data_sorted = zip(*sorted(enumerate(data), key=lambda x: x[1]['Code']))
codes, names = zip(*map(itemgetter('Code', 'Name'), data_sorted))
print(codes)
# ('Bl', 'Bz', 'Zl')
print(names)
# ('Korea', 'China', 'Arab')
add a comment |
up vote
0
down vote
up vote
0
down vote
Here's one way using operator.itemgetter
and unpacking via zip
:
from operator import itemgetter
_, data_sorted = zip(*sorted(enumerate(data), key=lambda x: x[1]['Code']))
codes, names = zip(*map(itemgetter('Code', 'Name'), data_sorted))
print(codes)
# ('Bl', 'Bz', 'Zl')
print(names)
# ('Korea', 'China', 'Arab')
Here's one way using operator.itemgetter
and unpacking via zip
:
from operator import itemgetter
_, data_sorted = zip(*sorted(enumerate(data), key=lambda x: x[1]['Code']))
codes, names = zip(*map(itemgetter('Code', 'Name'), data_sorted))
print(codes)
# ('Bl', 'Bz', 'Zl')
print(names)
# ('Korea', 'China', 'Arab')
answered Nov 10 at 18:07


jpp
81.8k194795
81.8k194795
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53236853%2fbetter-way-to-reorder-list-of-dictionaries%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XajKqmP
pairs = sorted((d['Code'], d['Name']) for d in data)
– FMc
Nov 10 at 8:12