Compiling multiple files to exe
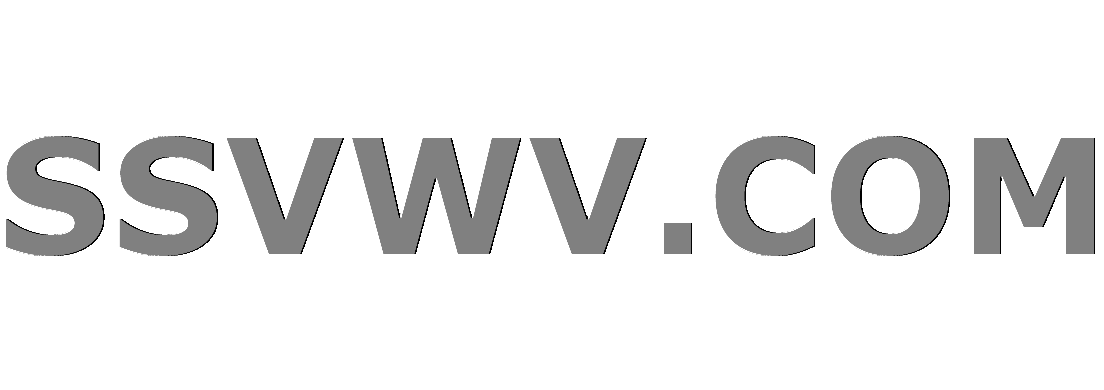
Multi tool use
up vote
1
down vote
favorite
I got multiple files to compile to a standalone exe using cython.
compile.py
from distutils.core import setup
from distutils.extension import Extension
from Cython.Distutils import build_ext
ext_modules = [
Extension("TheMainFile", ["TheMainFile.py"]),
Extension("HelperClass", ["HelperClass.py"]),
Extension("HelperClass2", ["HelperClass2.py"]),
]
setup(
name = 'Main',
cmdclass = 'build_ext': build_ext,
ext_modules = ext_modules
)
The main
file that should be run by the exe is TheMainFile
running the following command
python .compiler.py build_ext --inplace
creates 3 .c
files, in the build dir however is no .exe, just .lib
,.exp
and .obj
files.
Manually adding these files to a VS Projects to build, results in the following error
"Python.h": No such file or directory
Adding the following files to the project from C:Pythoninclude
lastly results in "structmember.h": No such file or directory
My question is:
How do I compile these files to a binary where TheMainFile
is executed.
python-3.x compilation python-3.6 cython
add a comment |
up vote
1
down vote
favorite
I got multiple files to compile to a standalone exe using cython.
compile.py
from distutils.core import setup
from distutils.extension import Extension
from Cython.Distutils import build_ext
ext_modules = [
Extension("TheMainFile", ["TheMainFile.py"]),
Extension("HelperClass", ["HelperClass.py"]),
Extension("HelperClass2", ["HelperClass2.py"]),
]
setup(
name = 'Main',
cmdclass = 'build_ext': build_ext,
ext_modules = ext_modules
)
The main
file that should be run by the exe is TheMainFile
running the following command
python .compiler.py build_ext --inplace
creates 3 .c
files, in the build dir however is no .exe, just .lib
,.exp
and .obj
files.
Manually adding these files to a VS Projects to build, results in the following error
"Python.h": No such file or directory
Adding the following files to the project from C:Pythoninclude
lastly results in "structmember.h": No such file or directory
My question is:
How do I compile these files to a binary where TheMainFile
is executed.
python-3.x compilation python-3.6 cython
Have you read stackoverflow.com/questions/52959902/…? Cython is mostly designed to make compiled modules instead of executables (but it is possible), and it isn't really designed to compile multiple source files to one object (but it is possible with a lot of work). You can do what you want but it isn't well supported.
– DavidW
Nov 10 at 12:45
@DavidW tried that approach with gcc, but then the above errors with no file found appears
– 0x45
Nov 10 at 12:51
You can work out the path to add to find "Python.h" with the commandpython-config --includes
– DavidW
Nov 10 at 13:38
@DavidW if you read the question, you'll see I included it ;)
– 0x45
Nov 10 at 16:30
In the question you say you added some files to your visual studio project, not that you set the include path (which is what Python expects you to do). However I don't really understand visual studio projects well enough to say any more so I'll stop here
– DavidW
Nov 10 at 17:34
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I got multiple files to compile to a standalone exe using cython.
compile.py
from distutils.core import setup
from distutils.extension import Extension
from Cython.Distutils import build_ext
ext_modules = [
Extension("TheMainFile", ["TheMainFile.py"]),
Extension("HelperClass", ["HelperClass.py"]),
Extension("HelperClass2", ["HelperClass2.py"]),
]
setup(
name = 'Main',
cmdclass = 'build_ext': build_ext,
ext_modules = ext_modules
)
The main
file that should be run by the exe is TheMainFile
running the following command
python .compiler.py build_ext --inplace
creates 3 .c
files, in the build dir however is no .exe, just .lib
,.exp
and .obj
files.
Manually adding these files to a VS Projects to build, results in the following error
"Python.h": No such file or directory
Adding the following files to the project from C:Pythoninclude
lastly results in "structmember.h": No such file or directory
My question is:
How do I compile these files to a binary where TheMainFile
is executed.
python-3.x compilation python-3.6 cython
I got multiple files to compile to a standalone exe using cython.
compile.py
from distutils.core import setup
from distutils.extension import Extension
from Cython.Distutils import build_ext
ext_modules = [
Extension("TheMainFile", ["TheMainFile.py"]),
Extension("HelperClass", ["HelperClass.py"]),
Extension("HelperClass2", ["HelperClass2.py"]),
]
setup(
name = 'Main',
cmdclass = 'build_ext': build_ext,
ext_modules = ext_modules
)
The main
file that should be run by the exe is TheMainFile
running the following command
python .compiler.py build_ext --inplace
creates 3 .c
files, in the build dir however is no .exe, just .lib
,.exp
and .obj
files.
Manually adding these files to a VS Projects to build, results in the following error
"Python.h": No such file or directory
Adding the following files to the project from C:Pythoninclude
lastly results in "structmember.h": No such file or directory
My question is:
How do I compile these files to a binary where TheMainFile
is executed.
python-3.x compilation python-3.6 cython
python-3.x compilation python-3.6 cython
asked Nov 10 at 12:37


0x45
284314
284314
Have you read stackoverflow.com/questions/52959902/…? Cython is mostly designed to make compiled modules instead of executables (but it is possible), and it isn't really designed to compile multiple source files to one object (but it is possible with a lot of work). You can do what you want but it isn't well supported.
– DavidW
Nov 10 at 12:45
@DavidW tried that approach with gcc, but then the above errors with no file found appears
– 0x45
Nov 10 at 12:51
You can work out the path to add to find "Python.h" with the commandpython-config --includes
– DavidW
Nov 10 at 13:38
@DavidW if you read the question, you'll see I included it ;)
– 0x45
Nov 10 at 16:30
In the question you say you added some files to your visual studio project, not that you set the include path (which is what Python expects you to do). However I don't really understand visual studio projects well enough to say any more so I'll stop here
– DavidW
Nov 10 at 17:34
add a comment |
Have you read stackoverflow.com/questions/52959902/…? Cython is mostly designed to make compiled modules instead of executables (but it is possible), and it isn't really designed to compile multiple source files to one object (but it is possible with a lot of work). You can do what you want but it isn't well supported.
– DavidW
Nov 10 at 12:45
@DavidW tried that approach with gcc, but then the above errors with no file found appears
– 0x45
Nov 10 at 12:51
You can work out the path to add to find "Python.h" with the commandpython-config --includes
– DavidW
Nov 10 at 13:38
@DavidW if you read the question, you'll see I included it ;)
– 0x45
Nov 10 at 16:30
In the question you say you added some files to your visual studio project, not that you set the include path (which is what Python expects you to do). However I don't really understand visual studio projects well enough to say any more so I'll stop here
– DavidW
Nov 10 at 17:34
Have you read stackoverflow.com/questions/52959902/…? Cython is mostly designed to make compiled modules instead of executables (but it is possible), and it isn't really designed to compile multiple source files to one object (but it is possible with a lot of work). You can do what you want but it isn't well supported.
– DavidW
Nov 10 at 12:45
Have you read stackoverflow.com/questions/52959902/…? Cython is mostly designed to make compiled modules instead of executables (but it is possible), and it isn't really designed to compile multiple source files to one object (but it is possible with a lot of work). You can do what you want but it isn't well supported.
– DavidW
Nov 10 at 12:45
@DavidW tried that approach with gcc, but then the above errors with no file found appears
– 0x45
Nov 10 at 12:51
@DavidW tried that approach with gcc, but then the above errors with no file found appears
– 0x45
Nov 10 at 12:51
You can work out the path to add to find "Python.h" with the command
python-config --includes
– DavidW
Nov 10 at 13:38
You can work out the path to add to find "Python.h" with the command
python-config --includes
– DavidW
Nov 10 at 13:38
@DavidW if you read the question, you'll see I included it ;)
– 0x45
Nov 10 at 16:30
@DavidW if you read the question, you'll see I included it ;)
– 0x45
Nov 10 at 16:30
In the question you say you added some files to your visual studio project, not that you set the include path (which is what Python expects you to do). However I don't really understand visual studio projects well enough to say any more so I'll stop here
– DavidW
Nov 10 at 17:34
In the question you say you added some files to your visual studio project, not that you set the include path (which is what Python expects you to do). However I don't really understand visual studio projects well enough to say any more so I'll stop here
– DavidW
Nov 10 at 17:34
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53239025%2fcompiling-multiple-files-to-exe%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hJnme29ODwaS1Q,Wk7Wp6F
Have you read stackoverflow.com/questions/52959902/…? Cython is mostly designed to make compiled modules instead of executables (but it is possible), and it isn't really designed to compile multiple source files to one object (but it is possible with a lot of work). You can do what you want but it isn't well supported.
– DavidW
Nov 10 at 12:45
@DavidW tried that approach with gcc, but then the above errors with no file found appears
– 0x45
Nov 10 at 12:51
You can work out the path to add to find "Python.h" with the command
python-config --includes
– DavidW
Nov 10 at 13:38
@DavidW if you read the question, you'll see I included it ;)
– 0x45
Nov 10 at 16:30
In the question you say you added some files to your visual studio project, not that you set the include path (which is what Python expects you to do). However I don't really understand visual studio projects well enough to say any more so I'll stop here
– DavidW
Nov 10 at 17:34