AttributeError: module 'asyncio' has no attribute 'create_task'
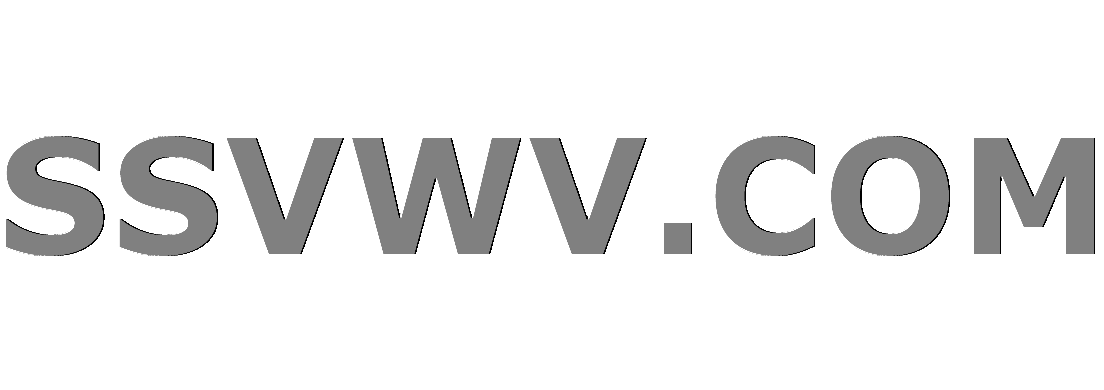
Multi tool use
up vote
4
down vote
favorite
I'm trying to asyncio.create_task()
but I dealing with this error:
Here's an example:
import asyncio
import time
async def async_say(delay, msg):
await asyncio.sleep(delay)
print(msg)
async def main():
task1 = asyncio.create_task(async_say(4, 'hello'))
task2 = asyncio.create_task(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
Out:
AttributeError: module 'asyncio' has no attribute 'create_task'
So I tried with the following snippet code (.ensure_future()
) instead, without any problem:
async def main():
task1 = asyncio.ensure_future(async_say(4, 'hello'))
task2 = asyncio.ensure_future(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
Out:
started at 13:19:44
hello
world
finished at 13:19:50
[UPDATE]:
With borrowing from @user4815162342 answer, I updated my question:
async def main():
loop = asyncio.get_event_loop()
task1 = loop.create_task(async_say(4, 'hello'))
task2 = loop.create_task(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
What's wrong?
[NOTE]:
- Python 3.6
- Ubuntu 16.04
python python-3.x async-await python-3.6 python-asyncio
add a comment |
up vote
4
down vote
favorite
I'm trying to asyncio.create_task()
but I dealing with this error:
Here's an example:
import asyncio
import time
async def async_say(delay, msg):
await asyncio.sleep(delay)
print(msg)
async def main():
task1 = asyncio.create_task(async_say(4, 'hello'))
task2 = asyncio.create_task(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
Out:
AttributeError: module 'asyncio' has no attribute 'create_task'
So I tried with the following snippet code (.ensure_future()
) instead, without any problem:
async def main():
task1 = asyncio.ensure_future(async_say(4, 'hello'))
task2 = asyncio.ensure_future(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
Out:
started at 13:19:44
hello
world
finished at 13:19:50
[UPDATE]:
With borrowing from @user4815162342 answer, I updated my question:
async def main():
loop = asyncio.get_event_loop()
task1 = loop.create_task(async_say(4, 'hello'))
task2 = loop.create_task(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
What's wrong?
[NOTE]:
- Python 3.6
- Ubuntu 16.04
python python-3.x async-await python-3.6 python-asyncio
add a comment |
up vote
4
down vote
favorite
up vote
4
down vote
favorite
I'm trying to asyncio.create_task()
but I dealing with this error:
Here's an example:
import asyncio
import time
async def async_say(delay, msg):
await asyncio.sleep(delay)
print(msg)
async def main():
task1 = asyncio.create_task(async_say(4, 'hello'))
task2 = asyncio.create_task(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
Out:
AttributeError: module 'asyncio' has no attribute 'create_task'
So I tried with the following snippet code (.ensure_future()
) instead, without any problem:
async def main():
task1 = asyncio.ensure_future(async_say(4, 'hello'))
task2 = asyncio.ensure_future(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
Out:
started at 13:19:44
hello
world
finished at 13:19:50
[UPDATE]:
With borrowing from @user4815162342 answer, I updated my question:
async def main():
loop = asyncio.get_event_loop()
task1 = loop.create_task(async_say(4, 'hello'))
task2 = loop.create_task(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
What's wrong?
[NOTE]:
- Python 3.6
- Ubuntu 16.04
python python-3.x async-await python-3.6 python-asyncio
I'm trying to asyncio.create_task()
but I dealing with this error:
Here's an example:
import asyncio
import time
async def async_say(delay, msg):
await asyncio.sleep(delay)
print(msg)
async def main():
task1 = asyncio.create_task(async_say(4, 'hello'))
task2 = asyncio.create_task(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
Out:
AttributeError: module 'asyncio' has no attribute 'create_task'
So I tried with the following snippet code (.ensure_future()
) instead, without any problem:
async def main():
task1 = asyncio.ensure_future(async_say(4, 'hello'))
task2 = asyncio.ensure_future(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
Out:
started at 13:19:44
hello
world
finished at 13:19:50
[UPDATE]:
With borrowing from @user4815162342 answer, I updated my question:
async def main():
loop = asyncio.get_event_loop()
task1 = loop.create_task(async_say(4, 'hello'))
task2 = loop.create_task(async_say(6, 'world'))
print(f"started at time.strftime('%X')")
await task1
await task2
print(f"finished at time.strftime('%X')")
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
What's wrong?
[NOTE]:
- Python 3.6
- Ubuntu 16.04
python python-3.x async-await python-3.6 python-asyncio
python python-3.x async-await python-3.6 python-asyncio
edited Nov 11 at 15:03
asked Nov 11 at 9:50


Benyamin Jafari
2,43531833
2,43531833
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
3
down vote
accepted
The create_task
top-level function was added in Python 3.7, and you are using Python 3.6. Prior to 3.7, create_task
was only available as a method on the event loop, so you can invoke it like that:
async def main():
loop = asyncio.get_event_loop()
task1 = loop.create_task(async_say(4, 'hello'))
task2 = loop.create_task(async_say(6, 'world'))
That works in both 3.6 and 3.7, as well as in earlier versions. asyncio.ensure_future
will work as well, but when you know you're dealing with a coroutine, create_task
is more explicit and is the preferred option.
1
Thank's for the answer, but I encountered with this error:RuntimeWarning: coroutine 'main' was never awaited
– Benyamin Jafari
Nov 11 at 10:34
1
@BenyaminJafari Please edit the question to include the new code you are testing.
– user4815162342
Nov 11 at 11:26
2
I updated my question, problem solved +1
– Benyamin Jafari
Nov 11 at 12:59
@BenyaminJafari Thanks. Note that updating the question is not needed if the problem is solved.
– user4815162342
Nov 11 at 14:49
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
The create_task
top-level function was added in Python 3.7, and you are using Python 3.6. Prior to 3.7, create_task
was only available as a method on the event loop, so you can invoke it like that:
async def main():
loop = asyncio.get_event_loop()
task1 = loop.create_task(async_say(4, 'hello'))
task2 = loop.create_task(async_say(6, 'world'))
That works in both 3.6 and 3.7, as well as in earlier versions. asyncio.ensure_future
will work as well, but when you know you're dealing with a coroutine, create_task
is more explicit and is the preferred option.
1
Thank's for the answer, but I encountered with this error:RuntimeWarning: coroutine 'main' was never awaited
– Benyamin Jafari
Nov 11 at 10:34
1
@BenyaminJafari Please edit the question to include the new code you are testing.
– user4815162342
Nov 11 at 11:26
2
I updated my question, problem solved +1
– Benyamin Jafari
Nov 11 at 12:59
@BenyaminJafari Thanks. Note that updating the question is not needed if the problem is solved.
– user4815162342
Nov 11 at 14:49
add a comment |
up vote
3
down vote
accepted
The create_task
top-level function was added in Python 3.7, and you are using Python 3.6. Prior to 3.7, create_task
was only available as a method on the event loop, so you can invoke it like that:
async def main():
loop = asyncio.get_event_loop()
task1 = loop.create_task(async_say(4, 'hello'))
task2 = loop.create_task(async_say(6, 'world'))
That works in both 3.6 and 3.7, as well as in earlier versions. asyncio.ensure_future
will work as well, but when you know you're dealing with a coroutine, create_task
is more explicit and is the preferred option.
1
Thank's for the answer, but I encountered with this error:RuntimeWarning: coroutine 'main' was never awaited
– Benyamin Jafari
Nov 11 at 10:34
1
@BenyaminJafari Please edit the question to include the new code you are testing.
– user4815162342
Nov 11 at 11:26
2
I updated my question, problem solved +1
– Benyamin Jafari
Nov 11 at 12:59
@BenyaminJafari Thanks. Note that updating the question is not needed if the problem is solved.
– user4815162342
Nov 11 at 14:49
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
The create_task
top-level function was added in Python 3.7, and you are using Python 3.6. Prior to 3.7, create_task
was only available as a method on the event loop, so you can invoke it like that:
async def main():
loop = asyncio.get_event_loop()
task1 = loop.create_task(async_say(4, 'hello'))
task2 = loop.create_task(async_say(6, 'world'))
That works in both 3.6 and 3.7, as well as in earlier versions. asyncio.ensure_future
will work as well, but when you know you're dealing with a coroutine, create_task
is more explicit and is the preferred option.
The create_task
top-level function was added in Python 3.7, and you are using Python 3.6. Prior to 3.7, create_task
was only available as a method on the event loop, so you can invoke it like that:
async def main():
loop = asyncio.get_event_loop()
task1 = loop.create_task(async_say(4, 'hello'))
task2 = loop.create_task(async_say(6, 'world'))
That works in both 3.6 and 3.7, as well as in earlier versions. asyncio.ensure_future
will work as well, but when you know you're dealing with a coroutine, create_task
is more explicit and is the preferred option.
edited Nov 11 at 19:25
answered Nov 11 at 10:05
user4815162342
59.2k488138
59.2k488138
1
Thank's for the answer, but I encountered with this error:RuntimeWarning: coroutine 'main' was never awaited
– Benyamin Jafari
Nov 11 at 10:34
1
@BenyaminJafari Please edit the question to include the new code you are testing.
– user4815162342
Nov 11 at 11:26
2
I updated my question, problem solved +1
– Benyamin Jafari
Nov 11 at 12:59
@BenyaminJafari Thanks. Note that updating the question is not needed if the problem is solved.
– user4815162342
Nov 11 at 14:49
add a comment |
1
Thank's for the answer, but I encountered with this error:RuntimeWarning: coroutine 'main' was never awaited
– Benyamin Jafari
Nov 11 at 10:34
1
@BenyaminJafari Please edit the question to include the new code you are testing.
– user4815162342
Nov 11 at 11:26
2
I updated my question, problem solved +1
– Benyamin Jafari
Nov 11 at 12:59
@BenyaminJafari Thanks. Note that updating the question is not needed if the problem is solved.
– user4815162342
Nov 11 at 14:49
1
1
Thank's for the answer, but I encountered with this error:
RuntimeWarning: coroutine 'main' was never awaited
– Benyamin Jafari
Nov 11 at 10:34
Thank's for the answer, but I encountered with this error:
RuntimeWarning: coroutine 'main' was never awaited
– Benyamin Jafari
Nov 11 at 10:34
1
1
@BenyaminJafari Please edit the question to include the new code you are testing.
– user4815162342
Nov 11 at 11:26
@BenyaminJafari Please edit the question to include the new code you are testing.
– user4815162342
Nov 11 at 11:26
2
2
I updated my question, problem solved +1
– Benyamin Jafari
Nov 11 at 12:59
I updated my question, problem solved +1
– Benyamin Jafari
Nov 11 at 12:59
@BenyaminJafari Thanks. Note that updating the question is not needed if the problem is solved.
– user4815162342
Nov 11 at 14:49
@BenyaminJafari Thanks. Note that updating the question is not needed if the problem is solved.
– user4815162342
Nov 11 at 14:49
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53247533%2fattributeerror-module-asyncio-has-no-attribute-create-task%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
s NOwt9N eD,B6UPWE7nrgj31I hSAnZRGrIlt DOWk X,WyysBpA1