Modifying PagedList in Android Paging Architecture library
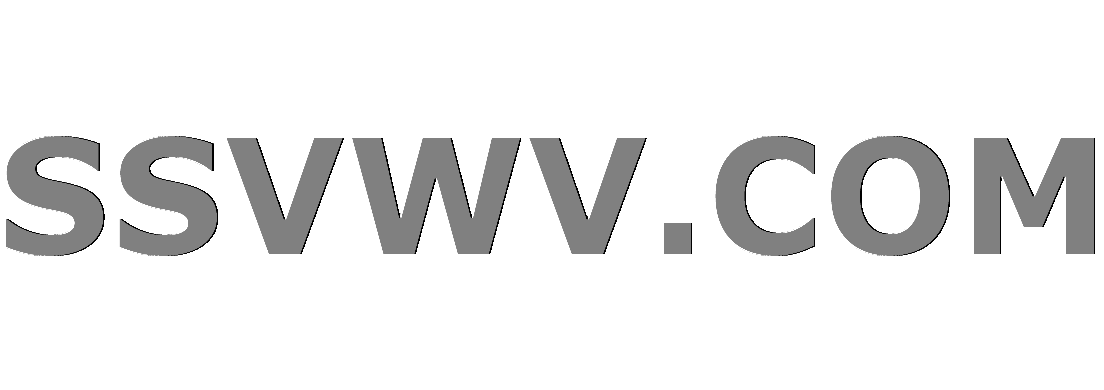
Multi tool use
up vote
5
down vote
favorite
I'm currently looking into incorporating the Paging Architecture library (version 2.1.0-beta01
at the time of writing) into my app. One components is a list which allows the user to delete individual items from it. This list is network-only and caching localy with Room does not make sense.
PagedList
is immutable and does not support modification. I have read that having a copy of the list which is than modified and returned as the new one is the way to go. The documentation states the same:
If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from the network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
I currently have the basic recommended implementation to show a simple list. My DataSource
looks like this:
class MyDataSource<SomeItem> : PageKeyedDataSource<Int, SomeItem>()
override fun loadInitial(params: LoadInitialParams<Int>, callback: LoadInitialCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
override fun loadAfter(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
override fun loadBefore(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
How would a concrete implementation of an in-memory cache (without Room and without invalidating the entire dataset) as referenced in the documentation look like?


add a comment |
up vote
5
down vote
favorite
I'm currently looking into incorporating the Paging Architecture library (version 2.1.0-beta01
at the time of writing) into my app. One components is a list which allows the user to delete individual items from it. This list is network-only and caching localy with Room does not make sense.
PagedList
is immutable and does not support modification. I have read that having a copy of the list which is than modified and returned as the new one is the way to go. The documentation states the same:
If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from the network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
I currently have the basic recommended implementation to show a simple list. My DataSource
looks like this:
class MyDataSource<SomeItem> : PageKeyedDataSource<Int, SomeItem>()
override fun loadInitial(params: LoadInitialParams<Int>, callback: LoadInitialCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
override fun loadAfter(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
override fun loadBefore(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
How would a concrete implementation of an in-memory cache (without Room and without invalidating the entire dataset) as referenced in the documentation look like?


add a comment |
up vote
5
down vote
favorite
up vote
5
down vote
favorite
I'm currently looking into incorporating the Paging Architecture library (version 2.1.0-beta01
at the time of writing) into my app. One components is a list which allows the user to delete individual items from it. This list is network-only and caching localy with Room does not make sense.
PagedList
is immutable and does not support modification. I have read that having a copy of the list which is than modified and returned as the new one is the way to go. The documentation states the same:
If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from the network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
I currently have the basic recommended implementation to show a simple list. My DataSource
looks like this:
class MyDataSource<SomeItem> : PageKeyedDataSource<Int, SomeItem>()
override fun loadInitial(params: LoadInitialParams<Int>, callback: LoadInitialCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
override fun loadAfter(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
override fun loadBefore(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
How would a concrete implementation of an in-memory cache (without Room and without invalidating the entire dataset) as referenced in the documentation look like?


I'm currently looking into incorporating the Paging Architecture library (version 2.1.0-beta01
at the time of writing) into my app. One components is a list which allows the user to delete individual items from it. This list is network-only and caching localy with Room does not make sense.
PagedList
is immutable and does not support modification. I have read that having a copy of the list which is than modified and returned as the new one is the way to go. The documentation states the same:
If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from the network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
I currently have the basic recommended implementation to show a simple list. My DataSource
looks like this:
class MyDataSource<SomeItem> : PageKeyedDataSource<Int, SomeItem>()
override fun loadInitial(params: LoadInitialParams<Int>, callback: LoadInitialCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
override fun loadAfter(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
override fun loadBefore(params: LoadParams<Int>, callback: LoadCallback<Int, SomeItem>)
// Simple load from API and notification of `callback`.
How would a concrete implementation of an in-memory cache (without Room and without invalidating the entire dataset) as referenced in the documentation look like?




edited Nov 17 at 22:44
Martin Zeitler
12.2k33560
12.2k33560
asked Nov 10 at 21:43


rubengees
1,26911021
1,26911021
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You are correct in that a DataSource
is meant to hold immutable data.
I believe this is because Room and Paging Library is trying to have more opinionated design decisions and advocate for immutable data.
This is why in the official docs, they have a section for updating or mutating your dataset should invalidate the datasource when such a change occurs.
Updating Paged Data: If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
With that in mind, I believe it's possible to solve the problem you described using a couple of steps.
This may not be the cleanest way, as it involves 2 steps.
You can get a reference the the snapshot that the PagedList is holding, which is a type MutableList
. Then, you can just remove or update the item inside that snapshot, without invalidating the data source.
Then step two would be to calling something like notifyItemRemoved(index)
or notifyItemChanged(index)
.
Since you can't force the DataSource to notify the observers of the change, you'll have to do that manually.
pagedList.snapshot().remove(index) // Removes item from the pagedList
adapter.notifyItemRemoved(index) // Triggers recyclerview to redraw/rebind to account for the deleted item.
There maybe a better solution found in your DataSource.Factory
.
According to the official docs, your DataSource.Factory
should be the one to emit a new PagedList
once the data is updated.
Updating Paged Data: To page in data from a source that does provide updates, you can create a DataSource.Factory, where each DataSource created is invalidated when an update to the data set occurs that makes the current snapshot invalid. For example, when paging a query from the Database, and the table being queried inserts or removes items. You can also use a DataSource.Factory to provide multiple versions of network-paged lists. If reloading all content (e.g. in response to an action like swipe-to-refresh) is required to get a new version of data, you can connect an explicit refresh signal to call invalidate() on the current DataSource.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
I haven't found a good solution for this second approach however.
Upvoted for the detailed answer and for the effort
– Ümañg ßürmån
Nov 23 at 7:56
Thank you for this detailed answer, but this approach does not work. The snapshot returned may be of typeMutableList
, but does not actually implement the interface. Calling for exampleremoveAt
yields anUnsupportedOperationException
. Also, the documentation states that snapshots are not meant for reflecting modification and are in fact also immutable. So thanks for the answer, but downvoting, because it's incorrect and may mislead other people.
– rubengees
Nov 23 at 20:57
Ah, I see. Then I the only other way I can think of is the second option mentioned above, and utilizing theDataSource.Factory
.
– Felipe Roriz
Nov 24 at 2:49
Yes, that seems the way to go. I have not yet found a way of implementing that and tried to get help on that with this question.
– rubengees
Nov 25 at 0:21
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You are correct in that a DataSource
is meant to hold immutable data.
I believe this is because Room and Paging Library is trying to have more opinionated design decisions and advocate for immutable data.
This is why in the official docs, they have a section for updating or mutating your dataset should invalidate the datasource when such a change occurs.
Updating Paged Data: If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
With that in mind, I believe it's possible to solve the problem you described using a couple of steps.
This may not be the cleanest way, as it involves 2 steps.
You can get a reference the the snapshot that the PagedList is holding, which is a type MutableList
. Then, you can just remove or update the item inside that snapshot, without invalidating the data source.
Then step two would be to calling something like notifyItemRemoved(index)
or notifyItemChanged(index)
.
Since you can't force the DataSource to notify the observers of the change, you'll have to do that manually.
pagedList.snapshot().remove(index) // Removes item from the pagedList
adapter.notifyItemRemoved(index) // Triggers recyclerview to redraw/rebind to account for the deleted item.
There maybe a better solution found in your DataSource.Factory
.
According to the official docs, your DataSource.Factory
should be the one to emit a new PagedList
once the data is updated.
Updating Paged Data: To page in data from a source that does provide updates, you can create a DataSource.Factory, where each DataSource created is invalidated when an update to the data set occurs that makes the current snapshot invalid. For example, when paging a query from the Database, and the table being queried inserts or removes items. You can also use a DataSource.Factory to provide multiple versions of network-paged lists. If reloading all content (e.g. in response to an action like swipe-to-refresh) is required to get a new version of data, you can connect an explicit refresh signal to call invalidate() on the current DataSource.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
I haven't found a good solution for this second approach however.
Upvoted for the detailed answer and for the effort
– Ümañg ßürmån
Nov 23 at 7:56
Thank you for this detailed answer, but this approach does not work. The snapshot returned may be of typeMutableList
, but does not actually implement the interface. Calling for exampleremoveAt
yields anUnsupportedOperationException
. Also, the documentation states that snapshots are not meant for reflecting modification and are in fact also immutable. So thanks for the answer, but downvoting, because it's incorrect and may mislead other people.
– rubengees
Nov 23 at 20:57
Ah, I see. Then I the only other way I can think of is the second option mentioned above, and utilizing theDataSource.Factory
.
– Felipe Roriz
Nov 24 at 2:49
Yes, that seems the way to go. I have not yet found a way of implementing that and tried to get help on that with this question.
– rubengees
Nov 25 at 0:21
add a comment |
up vote
0
down vote
You are correct in that a DataSource
is meant to hold immutable data.
I believe this is because Room and Paging Library is trying to have more opinionated design decisions and advocate for immutable data.
This is why in the official docs, they have a section for updating or mutating your dataset should invalidate the datasource when such a change occurs.
Updating Paged Data: If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
With that in mind, I believe it's possible to solve the problem you described using a couple of steps.
This may not be the cleanest way, as it involves 2 steps.
You can get a reference the the snapshot that the PagedList is holding, which is a type MutableList
. Then, you can just remove or update the item inside that snapshot, without invalidating the data source.
Then step two would be to calling something like notifyItemRemoved(index)
or notifyItemChanged(index)
.
Since you can't force the DataSource to notify the observers of the change, you'll have to do that manually.
pagedList.snapshot().remove(index) // Removes item from the pagedList
adapter.notifyItemRemoved(index) // Triggers recyclerview to redraw/rebind to account for the deleted item.
There maybe a better solution found in your DataSource.Factory
.
According to the official docs, your DataSource.Factory
should be the one to emit a new PagedList
once the data is updated.
Updating Paged Data: To page in data from a source that does provide updates, you can create a DataSource.Factory, where each DataSource created is invalidated when an update to the data set occurs that makes the current snapshot invalid. For example, when paging a query from the Database, and the table being queried inserts or removes items. You can also use a DataSource.Factory to provide multiple versions of network-paged lists. If reloading all content (e.g. in response to an action like swipe-to-refresh) is required to get a new version of data, you can connect an explicit refresh signal to call invalidate() on the current DataSource.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
I haven't found a good solution for this second approach however.
Upvoted for the detailed answer and for the effort
– Ümañg ßürmån
Nov 23 at 7:56
Thank you for this detailed answer, but this approach does not work. The snapshot returned may be of typeMutableList
, but does not actually implement the interface. Calling for exampleremoveAt
yields anUnsupportedOperationException
. Also, the documentation states that snapshots are not meant for reflecting modification and are in fact also immutable. So thanks for the answer, but downvoting, because it's incorrect and may mislead other people.
– rubengees
Nov 23 at 20:57
Ah, I see. Then I the only other way I can think of is the second option mentioned above, and utilizing theDataSource.Factory
.
– Felipe Roriz
Nov 24 at 2:49
Yes, that seems the way to go. I have not yet found a way of implementing that and tried to get help on that with this question.
– rubengees
Nov 25 at 0:21
add a comment |
up vote
0
down vote
up vote
0
down vote
You are correct in that a DataSource
is meant to hold immutable data.
I believe this is because Room and Paging Library is trying to have more opinionated design decisions and advocate for immutable data.
This is why in the official docs, they have a section for updating or mutating your dataset should invalidate the datasource when such a change occurs.
Updating Paged Data: If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
With that in mind, I believe it's possible to solve the problem you described using a couple of steps.
This may not be the cleanest way, as it involves 2 steps.
You can get a reference the the snapshot that the PagedList is holding, which is a type MutableList
. Then, you can just remove or update the item inside that snapshot, without invalidating the data source.
Then step two would be to calling something like notifyItemRemoved(index)
or notifyItemChanged(index)
.
Since you can't force the DataSource to notify the observers of the change, you'll have to do that manually.
pagedList.snapshot().remove(index) // Removes item from the pagedList
adapter.notifyItemRemoved(index) // Triggers recyclerview to redraw/rebind to account for the deleted item.
There maybe a better solution found in your DataSource.Factory
.
According to the official docs, your DataSource.Factory
should be the one to emit a new PagedList
once the data is updated.
Updating Paged Data: To page in data from a source that does provide updates, you can create a DataSource.Factory, where each DataSource created is invalidated when an update to the data set occurs that makes the current snapshot invalid. For example, when paging a query from the Database, and the table being queried inserts or removes items. You can also use a DataSource.Factory to provide multiple versions of network-paged lists. If reloading all content (e.g. in response to an action like swipe-to-refresh) is required to get a new version of data, you can connect an explicit refresh signal to call invalidate() on the current DataSource.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
I haven't found a good solution for this second approach however.
You are correct in that a DataSource
is meant to hold immutable data.
I believe this is because Room and Paging Library is trying to have more opinionated design decisions and advocate for immutable data.
This is why in the official docs, they have a section for updating or mutating your dataset should invalidate the datasource when such a change occurs.
Updating Paged Data: If you have more granular update signals, such as a network API signaling an update to a single item in the list, it's recommended to load data from network into memory. Then present that data to the PagedList via a DataSource that wraps an in-memory snapshot. Each time the in-memory copy changes, invalidate the previous DataSource, and a new one wrapping the new state of the snapshot can be created.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
With that in mind, I believe it's possible to solve the problem you described using a couple of steps.
This may not be the cleanest way, as it involves 2 steps.
You can get a reference the the snapshot that the PagedList is holding, which is a type MutableList
. Then, you can just remove or update the item inside that snapshot, without invalidating the data source.
Then step two would be to calling something like notifyItemRemoved(index)
or notifyItemChanged(index)
.
Since you can't force the DataSource to notify the observers of the change, you'll have to do that manually.
pagedList.snapshot().remove(index) // Removes item from the pagedList
adapter.notifyItemRemoved(index) // Triggers recyclerview to redraw/rebind to account for the deleted item.
There maybe a better solution found in your DataSource.Factory
.
According to the official docs, your DataSource.Factory
should be the one to emit a new PagedList
once the data is updated.
Updating Paged Data: To page in data from a source that does provide updates, you can create a DataSource.Factory, where each DataSource created is invalidated when an update to the data set occurs that makes the current snapshot invalid. For example, when paging a query from the Database, and the table being queried inserts or removes items. You can also use a DataSource.Factory to provide multiple versions of network-paged lists. If reloading all content (e.g. in response to an action like swipe-to-refresh) is required to get a new version of data, you can connect an explicit refresh signal to call invalidate() on the current DataSource.
Source: https://developer.android.com/reference/android/arch/paging/DataSource
I haven't found a good solution for this second approach however.
edited Nov 22 at 17:15
answered Nov 22 at 16:26


Felipe Roriz
1244
1244
Upvoted for the detailed answer and for the effort
– Ümañg ßürmån
Nov 23 at 7:56
Thank you for this detailed answer, but this approach does not work. The snapshot returned may be of typeMutableList
, but does not actually implement the interface. Calling for exampleremoveAt
yields anUnsupportedOperationException
. Also, the documentation states that snapshots are not meant for reflecting modification and are in fact also immutable. So thanks for the answer, but downvoting, because it's incorrect and may mislead other people.
– rubengees
Nov 23 at 20:57
Ah, I see. Then I the only other way I can think of is the second option mentioned above, and utilizing theDataSource.Factory
.
– Felipe Roriz
Nov 24 at 2:49
Yes, that seems the way to go. I have not yet found a way of implementing that and tried to get help on that with this question.
– rubengees
Nov 25 at 0:21
add a comment |
Upvoted for the detailed answer and for the effort
– Ümañg ßürmån
Nov 23 at 7:56
Thank you for this detailed answer, but this approach does not work. The snapshot returned may be of typeMutableList
, but does not actually implement the interface. Calling for exampleremoveAt
yields anUnsupportedOperationException
. Also, the documentation states that snapshots are not meant for reflecting modification and are in fact also immutable. So thanks for the answer, but downvoting, because it's incorrect and may mislead other people.
– rubengees
Nov 23 at 20:57
Ah, I see. Then I the only other way I can think of is the second option mentioned above, and utilizing theDataSource.Factory
.
– Felipe Roriz
Nov 24 at 2:49
Yes, that seems the way to go. I have not yet found a way of implementing that and tried to get help on that with this question.
– rubengees
Nov 25 at 0:21
Upvoted for the detailed answer and for the effort
– Ümañg ßürmån
Nov 23 at 7:56
Upvoted for the detailed answer and for the effort
– Ümañg ßürmån
Nov 23 at 7:56
Thank you for this detailed answer, but this approach does not work. The snapshot returned may be of type
MutableList
, but does not actually implement the interface. Calling for example removeAt
yields an UnsupportedOperationException
. Also, the documentation states that snapshots are not meant for reflecting modification and are in fact also immutable. So thanks for the answer, but downvoting, because it's incorrect and may mislead other people.– rubengees
Nov 23 at 20:57
Thank you for this detailed answer, but this approach does not work. The snapshot returned may be of type
MutableList
, but does not actually implement the interface. Calling for example removeAt
yields an UnsupportedOperationException
. Also, the documentation states that snapshots are not meant for reflecting modification and are in fact also immutable. So thanks for the answer, but downvoting, because it's incorrect and may mislead other people.– rubengees
Nov 23 at 20:57
Ah, I see. Then I the only other way I can think of is the second option mentioned above, and utilizing the
DataSource.Factory
.– Felipe Roriz
Nov 24 at 2:49
Ah, I see. Then I the only other way I can think of is the second option mentioned above, and utilizing the
DataSource.Factory
.– Felipe Roriz
Nov 24 at 2:49
Yes, that seems the way to go. I have not yet found a way of implementing that and tried to get help on that with this question.
– rubengees
Nov 25 at 0:21
Yes, that seems the way to go. I have not yet found a way of implementing that and tried to get help on that with this question.
– rubengees
Nov 25 at 0:21
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243704%2fmodifying-pagedlist-in-android-paging-architecture-library%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
j8QF,Ghh,Dq7Qv,OJ2 CI54to6xdPGph1T7PMTD0 YGHe7Uke,lsjpWgE2lIofT14jjW Z C0T5oCnCQ