Fast way find strings within hamming distance x of each other in a large array of random fixed length strings
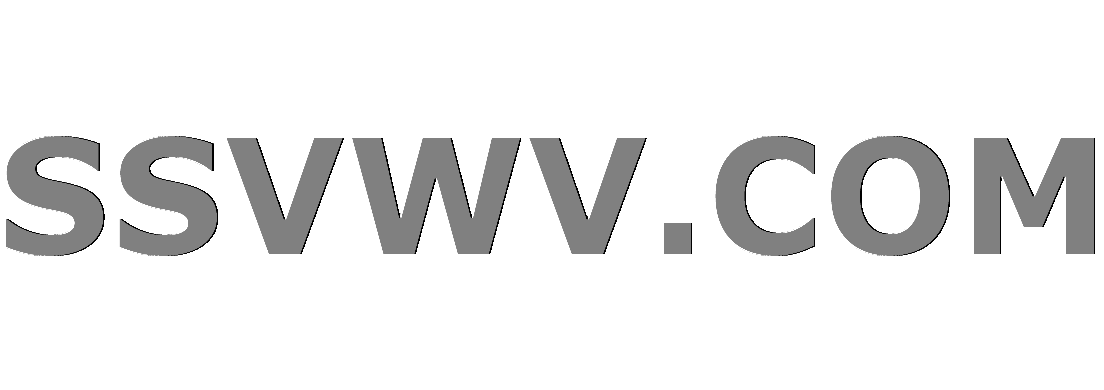
Multi tool use
I have a large array with millions of DNA sequences which are all 24 characters long. The DNA sequences should be random and can only contain A,T,G,C,N. I am trying to find strings that are within a certain hamming distance of each other.
My first approach was calculating the hamming distance between every string but this would take way to long.
My second approach used a masking method to create all possible variations of the strings and store them in a dictionary and then check if this variation was found more then 1 time. This worked pretty fast(20 min) for a hamming distance of 1 but is very memory intensive and would not be viable to use for a hamming distance of 2 or 3.
Python 2.7 implementation of my second approach.
sequences =
masks =
for sequence in sequences:
for i in range(len(sequence)):
try:
masks[sequence[:i] + '?' + sequence[i + 1:]].append(sequence[i])
except KeyError:
masks[sequence[:i] + '?' + sequence[i + 1:]] = [sequence[i], ]
matches =
for mask in masks:
if len(masks[mask]) > 1:
matches[mask] = masks[mask]
I am looking for a more efficient method. I came across Trie-trees, KD-trees, n-grams and indexing but I am lost as to what will be the best approach to this problem.
python algorithm bioinformatics
add a comment |
I have a large array with millions of DNA sequences which are all 24 characters long. The DNA sequences should be random and can only contain A,T,G,C,N. I am trying to find strings that are within a certain hamming distance of each other.
My first approach was calculating the hamming distance between every string but this would take way to long.
My second approach used a masking method to create all possible variations of the strings and store them in a dictionary and then check if this variation was found more then 1 time. This worked pretty fast(20 min) for a hamming distance of 1 but is very memory intensive and would not be viable to use for a hamming distance of 2 or 3.
Python 2.7 implementation of my second approach.
sequences =
masks =
for sequence in sequences:
for i in range(len(sequence)):
try:
masks[sequence[:i] + '?' + sequence[i + 1:]].append(sequence[i])
except KeyError:
masks[sequence[:i] + '?' + sequence[i + 1:]] = [sequence[i], ]
matches =
for mask in masks:
if len(masks[mask]) > 1:
matches[mask] = masks[mask]
I am looking for a more efficient method. I came across Trie-trees, KD-trees, n-grams and indexing but I am lost as to what will be the best approach to this problem.
python algorithm bioinformatics
which is the max hamming distance you want to allow?
– juvian
Nov 12 at 14:48
Without details this is hard to answer. I would compute the hamming weights of all sequences, and create a dictionary, which has the weight as key and as value a list of all matching strings. Then with the weight of your reference value you should be capable of finding all words with given distance easily.
– guidot
Nov 12 at 15:09
Good question, but likely beyond scope of SO as it is: (1) Domain specific to some regard (bioinformatics), so you may want to try bioinformatics.stackexchange.com or biostars.org, and (2) Does not pose precise technical question (bug, specific optimization of code, etc). However, a tip I can give you is to search for software that already does this, as it has been been likely optimized and tested. Also, this may be helpful: en.wikipedia.org/wiki/Alignment-free_sequence_analysis
– Vince
Nov 12 at 17:08
add a comment |
I have a large array with millions of DNA sequences which are all 24 characters long. The DNA sequences should be random and can only contain A,T,G,C,N. I am trying to find strings that are within a certain hamming distance of each other.
My first approach was calculating the hamming distance between every string but this would take way to long.
My second approach used a masking method to create all possible variations of the strings and store them in a dictionary and then check if this variation was found more then 1 time. This worked pretty fast(20 min) for a hamming distance of 1 but is very memory intensive and would not be viable to use for a hamming distance of 2 or 3.
Python 2.7 implementation of my second approach.
sequences =
masks =
for sequence in sequences:
for i in range(len(sequence)):
try:
masks[sequence[:i] + '?' + sequence[i + 1:]].append(sequence[i])
except KeyError:
masks[sequence[:i] + '?' + sequence[i + 1:]] = [sequence[i], ]
matches =
for mask in masks:
if len(masks[mask]) > 1:
matches[mask] = masks[mask]
I am looking for a more efficient method. I came across Trie-trees, KD-trees, n-grams and indexing but I am lost as to what will be the best approach to this problem.
python algorithm bioinformatics
I have a large array with millions of DNA sequences which are all 24 characters long. The DNA sequences should be random and can only contain A,T,G,C,N. I am trying to find strings that are within a certain hamming distance of each other.
My first approach was calculating the hamming distance between every string but this would take way to long.
My second approach used a masking method to create all possible variations of the strings and store them in a dictionary and then check if this variation was found more then 1 time. This worked pretty fast(20 min) for a hamming distance of 1 but is very memory intensive and would not be viable to use for a hamming distance of 2 or 3.
Python 2.7 implementation of my second approach.
sequences =
masks =
for sequence in sequences:
for i in range(len(sequence)):
try:
masks[sequence[:i] + '?' + sequence[i + 1:]].append(sequence[i])
except KeyError:
masks[sequence[:i] + '?' + sequence[i + 1:]] = [sequence[i], ]
matches =
for mask in masks:
if len(masks[mask]) > 1:
matches[mask] = masks[mask]
I am looking for a more efficient method. I came across Trie-trees, KD-trees, n-grams and indexing but I am lost as to what will be the best approach to this problem.
python algorithm bioinformatics
python algorithm bioinformatics
asked Nov 12 at 14:39
Yano
112
112
which is the max hamming distance you want to allow?
– juvian
Nov 12 at 14:48
Without details this is hard to answer. I would compute the hamming weights of all sequences, and create a dictionary, which has the weight as key and as value a list of all matching strings. Then with the weight of your reference value you should be capable of finding all words with given distance easily.
– guidot
Nov 12 at 15:09
Good question, but likely beyond scope of SO as it is: (1) Domain specific to some regard (bioinformatics), so you may want to try bioinformatics.stackexchange.com or biostars.org, and (2) Does not pose precise technical question (bug, specific optimization of code, etc). However, a tip I can give you is to search for software that already does this, as it has been been likely optimized and tested. Also, this may be helpful: en.wikipedia.org/wiki/Alignment-free_sequence_analysis
– Vince
Nov 12 at 17:08
add a comment |
which is the max hamming distance you want to allow?
– juvian
Nov 12 at 14:48
Without details this is hard to answer. I would compute the hamming weights of all sequences, and create a dictionary, which has the weight as key and as value a list of all matching strings. Then with the weight of your reference value you should be capable of finding all words with given distance easily.
– guidot
Nov 12 at 15:09
Good question, but likely beyond scope of SO as it is: (1) Domain specific to some regard (bioinformatics), so you may want to try bioinformatics.stackexchange.com or biostars.org, and (2) Does not pose precise technical question (bug, specific optimization of code, etc). However, a tip I can give you is to search for software that already does this, as it has been been likely optimized and tested. Also, this may be helpful: en.wikipedia.org/wiki/Alignment-free_sequence_analysis
– Vince
Nov 12 at 17:08
which is the max hamming distance you want to allow?
– juvian
Nov 12 at 14:48
which is the max hamming distance you want to allow?
– juvian
Nov 12 at 14:48
Without details this is hard to answer. I would compute the hamming weights of all sequences, and create a dictionary, which has the weight as key and as value a list of all matching strings. Then with the weight of your reference value you should be capable of finding all words with given distance easily.
– guidot
Nov 12 at 15:09
Without details this is hard to answer. I would compute the hamming weights of all sequences, and create a dictionary, which has the weight as key and as value a list of all matching strings. Then with the weight of your reference value you should be capable of finding all words with given distance easily.
– guidot
Nov 12 at 15:09
Good question, but likely beyond scope of SO as it is: (1) Domain specific to some regard (bioinformatics), so you may want to try bioinformatics.stackexchange.com or biostars.org, and (2) Does not pose precise technical question (bug, specific optimization of code, etc). However, a tip I can give you is to search for software that already does this, as it has been been likely optimized and tested. Also, this may be helpful: en.wikipedia.org/wiki/Alignment-free_sequence_analysis
– Vince
Nov 12 at 17:08
Good question, but likely beyond scope of SO as it is: (1) Domain specific to some regard (bioinformatics), so you may want to try bioinformatics.stackexchange.com or biostars.org, and (2) Does not pose precise technical question (bug, specific optimization of code, etc). However, a tip I can give you is to search for software that already does this, as it has been been likely optimized and tested. Also, this may be helpful: en.wikipedia.org/wiki/Alignment-free_sequence_analysis
– Vince
Nov 12 at 17:08
add a comment |
2 Answers
2
active
oldest
votes
One approach is Locality Sensitive Hashing
First, you should note that this method does not necessarily return all the pairs, it returns all the pairs with a high probability (or most pairs).
Locality Sensitive Hashing can be summarised as: data points that are located close to each other are mapped to similar hashes (in the same bucket with a high probability). Check this link for more details.
Your problem can be recast mathematically as:
Given N
vectors v ∈ R^24, N<<5^24
and a maximum hamming distance d
, return pairs which have a hamming distance atmost d
.
The way you'll solve this is to randomly generates K
planes P_1,P_2,...,P_K
in R^24
; Where K
is a parameter you'll have to experiment with. For every data point v
, you'll define a hash of v
as the tuple Hash(v)=(a_1,a_2,...,a_K)
where a_i∈0,1
denotes if v
is above this plane or below it. You can prove (I'll omit the proof) that if the hamming distance between two vectors is small then the probability that their hash is close is high.
So, for any given data point, rather than checking all the datapoints in the sequences, you only check data points in the bin of "close" hashes.
Note that these are very heuristic based and will need you to experiment with K
and how "close" you want to search from each hash. As K
increases, your number of bins increase exponentially with it, but the likelihood of similarity increases.
Judging by what you said, it looks like you have a gigantic dataset so I thought I would throw this for you to consider.
add a comment |
Found my solution here: http://www.cs.princeton.edu/~rs/strings/
This uses ternary search trees and took only a couple of minutes and ~1GB of ram. I modified the demo.c file to work for my use case.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53264464%2ffast-way-find-strings-within-hamming-distance-x-of-each-other-in-a-large-array-o%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
One approach is Locality Sensitive Hashing
First, you should note that this method does not necessarily return all the pairs, it returns all the pairs with a high probability (or most pairs).
Locality Sensitive Hashing can be summarised as: data points that are located close to each other are mapped to similar hashes (in the same bucket with a high probability). Check this link for more details.
Your problem can be recast mathematically as:
Given N
vectors v ∈ R^24, N<<5^24
and a maximum hamming distance d
, return pairs which have a hamming distance atmost d
.
The way you'll solve this is to randomly generates K
planes P_1,P_2,...,P_K
in R^24
; Where K
is a parameter you'll have to experiment with. For every data point v
, you'll define a hash of v
as the tuple Hash(v)=(a_1,a_2,...,a_K)
where a_i∈0,1
denotes if v
is above this plane or below it. You can prove (I'll omit the proof) that if the hamming distance between two vectors is small then the probability that their hash is close is high.
So, for any given data point, rather than checking all the datapoints in the sequences, you only check data points in the bin of "close" hashes.
Note that these are very heuristic based and will need you to experiment with K
and how "close" you want to search from each hash. As K
increases, your number of bins increase exponentially with it, but the likelihood of similarity increases.
Judging by what you said, it looks like you have a gigantic dataset so I thought I would throw this for you to consider.
add a comment |
One approach is Locality Sensitive Hashing
First, you should note that this method does not necessarily return all the pairs, it returns all the pairs with a high probability (or most pairs).
Locality Sensitive Hashing can be summarised as: data points that are located close to each other are mapped to similar hashes (in the same bucket with a high probability). Check this link for more details.
Your problem can be recast mathematically as:
Given N
vectors v ∈ R^24, N<<5^24
and a maximum hamming distance d
, return pairs which have a hamming distance atmost d
.
The way you'll solve this is to randomly generates K
planes P_1,P_2,...,P_K
in R^24
; Where K
is a parameter you'll have to experiment with. For every data point v
, you'll define a hash of v
as the tuple Hash(v)=(a_1,a_2,...,a_K)
where a_i∈0,1
denotes if v
is above this plane or below it. You can prove (I'll omit the proof) that if the hamming distance between two vectors is small then the probability that their hash is close is high.
So, for any given data point, rather than checking all the datapoints in the sequences, you only check data points in the bin of "close" hashes.
Note that these are very heuristic based and will need you to experiment with K
and how "close" you want to search from each hash. As K
increases, your number of bins increase exponentially with it, but the likelihood of similarity increases.
Judging by what you said, it looks like you have a gigantic dataset so I thought I would throw this for you to consider.
add a comment |
One approach is Locality Sensitive Hashing
First, you should note that this method does not necessarily return all the pairs, it returns all the pairs with a high probability (or most pairs).
Locality Sensitive Hashing can be summarised as: data points that are located close to each other are mapped to similar hashes (in the same bucket with a high probability). Check this link for more details.
Your problem can be recast mathematically as:
Given N
vectors v ∈ R^24, N<<5^24
and a maximum hamming distance d
, return pairs which have a hamming distance atmost d
.
The way you'll solve this is to randomly generates K
planes P_1,P_2,...,P_K
in R^24
; Where K
is a parameter you'll have to experiment with. For every data point v
, you'll define a hash of v
as the tuple Hash(v)=(a_1,a_2,...,a_K)
where a_i∈0,1
denotes if v
is above this plane or below it. You can prove (I'll omit the proof) that if the hamming distance between two vectors is small then the probability that their hash is close is high.
So, for any given data point, rather than checking all the datapoints in the sequences, you only check data points in the bin of "close" hashes.
Note that these are very heuristic based and will need you to experiment with K
and how "close" you want to search from each hash. As K
increases, your number of bins increase exponentially with it, but the likelihood of similarity increases.
Judging by what you said, it looks like you have a gigantic dataset so I thought I would throw this for you to consider.
One approach is Locality Sensitive Hashing
First, you should note that this method does not necessarily return all the pairs, it returns all the pairs with a high probability (or most pairs).
Locality Sensitive Hashing can be summarised as: data points that are located close to each other are mapped to similar hashes (in the same bucket with a high probability). Check this link for more details.
Your problem can be recast mathematically as:
Given N
vectors v ∈ R^24, N<<5^24
and a maximum hamming distance d
, return pairs which have a hamming distance atmost d
.
The way you'll solve this is to randomly generates K
planes P_1,P_2,...,P_K
in R^24
; Where K
is a parameter you'll have to experiment with. For every data point v
, you'll define a hash of v
as the tuple Hash(v)=(a_1,a_2,...,a_K)
where a_i∈0,1
denotes if v
is above this plane or below it. You can prove (I'll omit the proof) that if the hamming distance between two vectors is small then the probability that their hash is close is high.
So, for any given data point, rather than checking all the datapoints in the sequences, you only check data points in the bin of "close" hashes.
Note that these are very heuristic based and will need you to experiment with K
and how "close" you want to search from each hash. As K
increases, your number of bins increase exponentially with it, but the likelihood of similarity increases.
Judging by what you said, it looks like you have a gigantic dataset so I thought I would throw this for you to consider.
answered Nov 12 at 17:01
AspiringMat
471418
471418
add a comment |
add a comment |
Found my solution here: http://www.cs.princeton.edu/~rs/strings/
This uses ternary search trees and took only a couple of minutes and ~1GB of ram. I modified the demo.c file to work for my use case.
add a comment |
Found my solution here: http://www.cs.princeton.edu/~rs/strings/
This uses ternary search trees and took only a couple of minutes and ~1GB of ram. I modified the demo.c file to work for my use case.
add a comment |
Found my solution here: http://www.cs.princeton.edu/~rs/strings/
This uses ternary search trees and took only a couple of minutes and ~1GB of ram. I modified the demo.c file to work for my use case.
Found my solution here: http://www.cs.princeton.edu/~rs/strings/
This uses ternary search trees and took only a couple of minutes and ~1GB of ram. I modified the demo.c file to work for my use case.
answered Nov 29 at 12:49
Yano
112
112
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53264464%2ffast-way-find-strings-within-hamming-distance-x-of-each-other-in-a-large-array-o%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
A YMPztMoVDIQNODEn94dYjC5MGd8,fkkIdGt0VV5HtQdRpv Z87kcB NrrJ0JmpI3 Vi080SOlE4Pau C3FkFx5ysHLOg3ygHS
which is the max hamming distance you want to allow?
– juvian
Nov 12 at 14:48
Without details this is hard to answer. I would compute the hamming weights of all sequences, and create a dictionary, which has the weight as key and as value a list of all matching strings. Then with the weight of your reference value you should be capable of finding all words with given distance easily.
– guidot
Nov 12 at 15:09
Good question, but likely beyond scope of SO as it is: (1) Domain specific to some regard (bioinformatics), so you may want to try bioinformatics.stackexchange.com or biostars.org, and (2) Does not pose precise technical question (bug, specific optimization of code, etc). However, a tip I can give you is to search for software that already does this, as it has been been likely optimized and tested. Also, this may be helpful: en.wikipedia.org/wiki/Alignment-free_sequence_analysis
– Vince
Nov 12 at 17:08