HashMap/Hashtable not returning int as key value in for loop
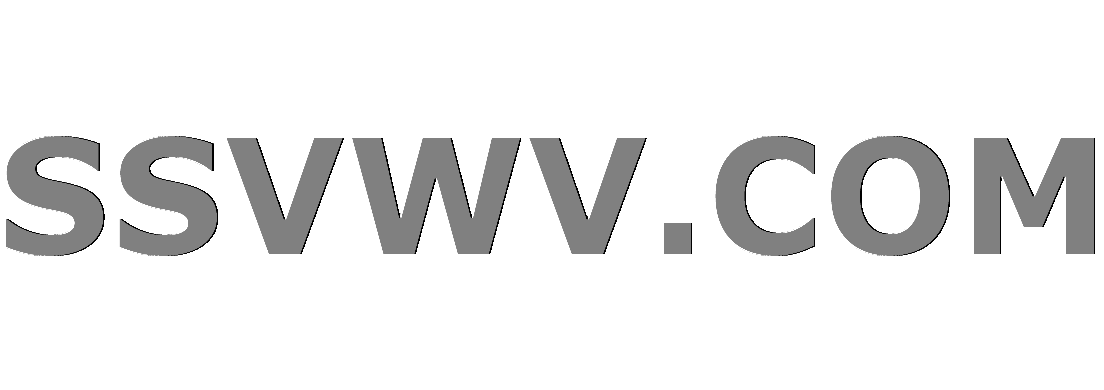
Multi tool use
When I am trying to access map.get(c) in for loop (as shown in version 2), it returns null value and sets upper bound to be null, resulting in null-pointer exception. On the other hand, if I create end variable and assign it map.get(c) value (as shown in version 1), it works fine. So, would you please explain me why?
version 1: Works perfectly fine
int count=0;
int st=0;
string s = "abcabcbb";
Hashtable<Character, Integer> map = new Hashtable<Character, Integer>();
char str = s.toCharArray();
for(int i=0; i<str.length; i++)
char c = str[i];
if(map.get(c)==null)
map.put(c, i);
if(count < map.get(c) - st + 1)
count = map.get(c) - st + 1;
;
else
int end = map.get(c); // End variable --> returns int value as expected
for(int j=st; j<=end; j++)
map.remove(str[j]);
st = j+1;
map.put(c,i);
System.out.println(count);
Version 2: Gives null-pointer exception
int count=0;
int st=0;
string s = "abcabcbb";
Hashtable<Character, Integer> map = new Hashtable<Character, Integer>();
char str = s.toCharArray();
for(int i=0; i<str.length; i++)
char c = str[i];
if(map.get(c)==null)
map.put(c, i);
if(count < map.get(c) - st + 1)
count = map.get(c) - st + 1;
;
else
//int end = map.get(c); // End variable commented
for(int j=st; j<=map.get(c); j++) // replaced end w map.get(c) --> returns null instead of int
map.remove(str[j]);
st = j+1;
map.put(c,i);
System.out.println(count);
Thanks for your help in advance!
Rohan.
java hashmap hashtable
add a comment |Â
When I am trying to access map.get(c) in for loop (as shown in version 2), it returns null value and sets upper bound to be null, resulting in null-pointer exception. On the other hand, if I create end variable and assign it map.get(c) value (as shown in version 1), it works fine. So, would you please explain me why?
version 1: Works perfectly fine
int count=0;
int st=0;
string s = "abcabcbb";
Hashtable<Character, Integer> map = new Hashtable<Character, Integer>();
char str = s.toCharArray();
for(int i=0; i<str.length; i++)
char c = str[i];
if(map.get(c)==null)
map.put(c, i);
if(count < map.get(c) - st + 1)
count = map.get(c) - st + 1;
;
else
int end = map.get(c); // End variable --> returns int value as expected
for(int j=st; j<=end; j++)
map.remove(str[j]);
st = j+1;
map.put(c,i);
System.out.println(count);
Version 2: Gives null-pointer exception
int count=0;
int st=0;
string s = "abcabcbb";
Hashtable<Character, Integer> map = new Hashtable<Character, Integer>();
char str = s.toCharArray();
for(int i=0; i<str.length; i++)
char c = str[i];
if(map.get(c)==null)
map.put(c, i);
if(count < map.get(c) - st + 1)
count = map.get(c) - st + 1;
;
else
//int end = map.get(c); // End variable commented
for(int j=st; j<=map.get(c); j++) // replaced end w map.get(c) --> returns null instead of int
map.remove(str[j]);
st = j+1;
map.put(c,i);
System.out.println(count);
Thanks for your help in advance!
Rohan.
java hashmap hashtable
Also stackoverflow.com/questions/40471/…
– azro
Nov 11 at 0:25
add a comment |Â
When I am trying to access map.get(c) in for loop (as shown in version 2), it returns null value and sets upper bound to be null, resulting in null-pointer exception. On the other hand, if I create end variable and assign it map.get(c) value (as shown in version 1), it works fine. So, would you please explain me why?
version 1: Works perfectly fine
int count=0;
int st=0;
string s = "abcabcbb";
Hashtable<Character, Integer> map = new Hashtable<Character, Integer>();
char str = s.toCharArray();
for(int i=0; i<str.length; i++)
char c = str[i];
if(map.get(c)==null)
map.put(c, i);
if(count < map.get(c) - st + 1)
count = map.get(c) - st + 1;
;
else
int end = map.get(c); // End variable --> returns int value as expected
for(int j=st; j<=end; j++)
map.remove(str[j]);
st = j+1;
map.put(c,i);
System.out.println(count);
Version 2: Gives null-pointer exception
int count=0;
int st=0;
string s = "abcabcbb";
Hashtable<Character, Integer> map = new Hashtable<Character, Integer>();
char str = s.toCharArray();
for(int i=0; i<str.length; i++)
char c = str[i];
if(map.get(c)==null)
map.put(c, i);
if(count < map.get(c) - st + 1)
count = map.get(c) - st + 1;
;
else
//int end = map.get(c); // End variable commented
for(int j=st; j<=map.get(c); j++) // replaced end w map.get(c) --> returns null instead of int
map.remove(str[j]);
st = j+1;
map.put(c,i);
System.out.println(count);
Thanks for your help in advance!
Rohan.
java hashmap hashtable
When I am trying to access map.get(c) in for loop (as shown in version 2), it returns null value and sets upper bound to be null, resulting in null-pointer exception. On the other hand, if I create end variable and assign it map.get(c) value (as shown in version 1), it works fine. So, would you please explain me why?
version 1: Works perfectly fine
int count=0;
int st=0;
string s = "abcabcbb";
Hashtable<Character, Integer> map = new Hashtable<Character, Integer>();
char str = s.toCharArray();
for(int i=0; i<str.length; i++)
char c = str[i];
if(map.get(c)==null)
map.put(c, i);
if(count < map.get(c) - st + 1)
count = map.get(c) - st + 1;
;
else
int end = map.get(c); // End variable --> returns int value as expected
for(int j=st; j<=end; j++)
map.remove(str[j]);
st = j+1;
map.put(c,i);
System.out.println(count);
Version 2: Gives null-pointer exception
int count=0;
int st=0;
string s = "abcabcbb";
Hashtable<Character, Integer> map = new Hashtable<Character, Integer>();
char str = s.toCharArray();
for(int i=0; i<str.length; i++)
char c = str[i];
if(map.get(c)==null)
map.put(c, i);
if(count < map.get(c) - st + 1)
count = map.get(c) - st + 1;
;
else
//int end = map.get(c); // End variable commented
for(int j=st; j<=map.get(c); j++) // replaced end w map.get(c) --> returns null instead of int
map.remove(str[j]);
st = j+1;
map.put(c,i);
System.out.println(count);
Thanks for your help in advance!
Rohan.
java hashmap hashtable
java hashmap hashtable
asked Nov 11 at 0:14
Rohan
111
111
Also stackoverflow.com/questions/40471/…
– azro
Nov 11 at 0:25
add a comment |Â
Also stackoverflow.com/questions/40471/…
– azro
Nov 11 at 0:25
Also stackoverflow.com/questions/40471/…
– azro
Nov 11 at 0:25
Also stackoverflow.com/questions/40471/…
– azro
Nov 11 at 0:25
add a comment |Â
1 Answer
1
active
oldest
votes
A for-loop runs until its condition is not met (in your case, until j <= map.get(c)
is false
). This condition is also not cached, as shown by the output of the code below:
public static void main(String args)
for (int i = 0; i < getCondition(); i++)
private static int getCondition()
System.out.println("Test");
return 3;
Output:
Test
Test
Test
Test
For that reason, map.get(c)
will be called for each iteration of the for-loop. If you happen to remove the entry with key c
from map
, then the value returned from Map#get
is null
, which is what causes the NullPointerException
.
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
A for-loop runs until its condition is not met (in your case, until j <= map.get(c)
is false
). This condition is also not cached, as shown by the output of the code below:
public static void main(String args)
for (int i = 0; i < getCondition(); i++)
private static int getCondition()
System.out.println("Test");
return 3;
Output:
Test
Test
Test
Test
For that reason, map.get(c)
will be called for each iteration of the for-loop. If you happen to remove the entry with key c
from map
, then the value returned from Map#get
is null
, which is what causes the NullPointerException
.
add a comment |Â
A for-loop runs until its condition is not met (in your case, until j <= map.get(c)
is false
). This condition is also not cached, as shown by the output of the code below:
public static void main(String args)
for (int i = 0; i < getCondition(); i++)
private static int getCondition()
System.out.println("Test");
return 3;
Output:
Test
Test
Test
Test
For that reason, map.get(c)
will be called for each iteration of the for-loop. If you happen to remove the entry with key c
from map
, then the value returned from Map#get
is null
, which is what causes the NullPointerException
.
add a comment |Â
A for-loop runs until its condition is not met (in your case, until j <= map.get(c)
is false
). This condition is also not cached, as shown by the output of the code below:
public static void main(String args)
for (int i = 0; i < getCondition(); i++)
private static int getCondition()
System.out.println("Test");
return 3;
Output:
Test
Test
Test
Test
For that reason, map.get(c)
will be called for each iteration of the for-loop. If you happen to remove the entry with key c
from map
, then the value returned from Map#get
is null
, which is what causes the NullPointerException
.
A for-loop runs until its condition is not met (in your case, until j <= map.get(c)
is false
). This condition is also not cached, as shown by the output of the code below:
public static void main(String args)
for (int i = 0; i < getCondition(); i++)
private static int getCondition()
System.out.println("Test");
return 3;
Output:
Test
Test
Test
Test
For that reason, map.get(c)
will be called for each iteration of the for-loop. If you happen to remove the entry with key c
from map
, then the value returned from Map#get
is null
, which is what causes the NullPointerException
.
answered Nov 11 at 0:24


Jacob G.
15.2k52162
15.2k52162
add a comment |Â
add a comment |Â
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244688%2fhashmap-hashtable-not-returning-int-as-key-value-in-for-loop%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
e,57pDEQpQwP,cHdS,Gnx,6hda2nAL0Um7UBNYE83j CX
Also stackoverflow.com/questions/40471/…
– azro
Nov 11 at 0:25