“Private” (implementation) class in Python
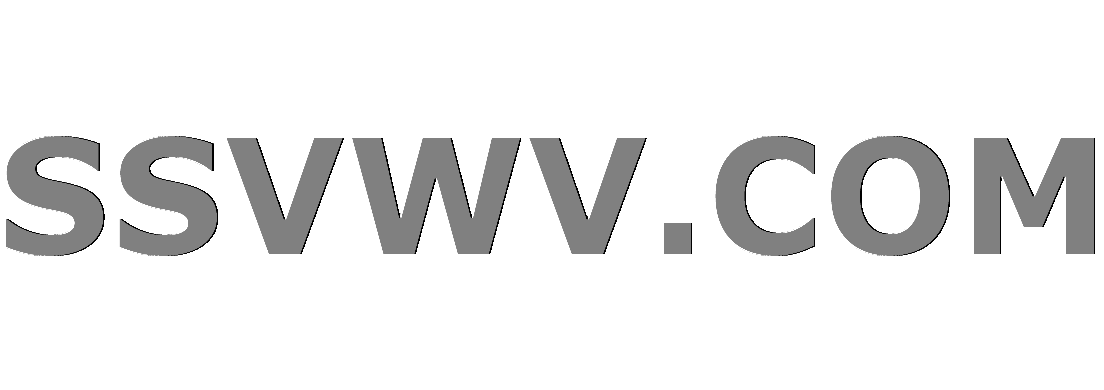
Multi tool use
up vote
82
down vote
favorite
I am coding a small Python module composed of two parts:
- some functions defining a public interface,
- an implementation class used by the above functions, but which is not meaningful outside the module.
At first, I decided to "hide" this implementation class by defining it inside the function using it, but this hampers readability and cannot be used if multiple functions reuse the same class.
So, in addition to comments and docstrings, is there a mechanism to mark a class as "private" or "internal"? I am aware of the underscore mechanism, but as I understand it it only applies to variables, function and methods name.
python access-modifiers
add a comment |
up vote
82
down vote
favorite
I am coding a small Python module composed of two parts:
- some functions defining a public interface,
- an implementation class used by the above functions, but which is not meaningful outside the module.
At first, I decided to "hide" this implementation class by defining it inside the function using it, but this hampers readability and cannot be used if multiple functions reuse the same class.
So, in addition to comments and docstrings, is there a mechanism to mark a class as "private" or "internal"? I am aware of the underscore mechanism, but as I understand it it only applies to variables, function and methods name.
python access-modifiers
add a comment |
up vote
82
down vote
favorite
up vote
82
down vote
favorite
I am coding a small Python module composed of two parts:
- some functions defining a public interface,
- an implementation class used by the above functions, but which is not meaningful outside the module.
At first, I decided to "hide" this implementation class by defining it inside the function using it, but this hampers readability and cannot be used if multiple functions reuse the same class.
So, in addition to comments and docstrings, is there a mechanism to mark a class as "private" or "internal"? I am aware of the underscore mechanism, but as I understand it it only applies to variables, function and methods name.
python access-modifiers
I am coding a small Python module composed of two parts:
- some functions defining a public interface,
- an implementation class used by the above functions, but which is not meaningful outside the module.
At first, I decided to "hide" this implementation class by defining it inside the function using it, but this hampers readability and cannot be used if multiple functions reuse the same class.
So, in addition to comments and docstrings, is there a mechanism to mark a class as "private" or "internal"? I am aware of the underscore mechanism, but as I understand it it only applies to variables, function and methods name.
python access-modifiers
python access-modifiers
edited Nov 11 at 12:28


Stephen Kennedy
6,949134866
6,949134866
asked Feb 15 '09 at 15:29
oparisy
7331816
7331816
add a comment |
add a comment |
7 Answers
7
active
oldest
votes
up vote
135
down vote
accepted
Use a single underscore prefix:
class _Internal:
...
This is the official Python convention for 'internal' symbols; "from module import *" does not import underscore-prefixed objects.
Edit: Reference to the single underscore convention
2
I did not know the underscore rule extended to classes. I do not want to clutter my namespace when importing, so this behavior is what I was looking for. Thanks!
– oparisy
Feb 15 '09 at 19:52
1
Since you state that this is the "official" python convention, it would be nice with a link. Another post here says that all is the official way and does link to the documentation.
– flodin
Feb 15 '09 at 20:00
10
python.org/dev/peps/pep-0008 -- _single_leading_underscore: weak "internal use" indicator. E.g. "from M import *" does not import objects whose name starts with an underscore. -- Classes for internal use have a leading underscore
– Miles
Feb 15 '09 at 20:16
2
A leading underscore is the convention for marking things as internal, "don't mess with this," whereas all is more for modules designed to be used with "from M import *", without necessarily implying that the module users shouldn't touch that class.
– Miles
Feb 15 '09 at 20:20
add a comment |
up vote
54
down vote
In short:
You cannot enforce privacy. There are no private classes/methods/functions in Python. At least, not strict privacy as in other languages, such as Java.
You can only indicate/suggest privacy. This follows a convention. The python convention for marking a class/function/method as private is to preface it with an _ (underscore). For example,
def _myfunc()
orclass _MyClass:
. You can also create pseudo-privacy by prefacing the method with two underscores (eg:__foo
). You cannot access the method directly, but you can still call it through a special prefix using the classname (eg:_classname__foo
). So the best you can do is indicate/suggest privacy, not enforce it.
Python is like perl in this respect. To paraphrase a famous line about privacy from the Perl book, the philosophy is that you should stay out of the living room because you weren't invited, not because it is defended with a shotgun.
For more information:
Private variables Python Documentation
Private functions Dive into Python, by Mark Pilgrim
Why are Python’s ‘private’ methods not actually private? StackOverflow question 70528
In response to #1, you sort of can enforce privacy of methods. Using a double underscore like __method(self) will make it inaccessible outside of the class. But there is a way around it by calling it like, Foo()._Foo__method(). I guess it just renames it to something more esoteric.
– Evan Fosmark
Feb 15 '09 at 20:54
1
That quote is accurate.
– Paul Draper
Apr 14 '13 at 7:31
add a comment |
up vote
35
down vote
Define __all__
, a list of names that you want to be exported (see documentation).
__all__ = ['public_class'] # don't add here the 'implementation_class'
add a comment |
up vote
9
down vote
A pattern that I sometimes use is this:
Define a class:
class x(object):
def doThis(self):
...
def doThat(self):
...
Create an instance of the class, overwriting the class name:
x = x()
Define symbols that expose the functionality:
doThis = x.doThis
doThat = x.doThat
Delete the instance itself:
del x
Now you have a module that only exposes your public functions.
that's kinda cool!
– ʞɔıu
Feb 15 '09 at 18:52
1
Took a minute to understand the purpose/function of "overwriting the class name", but I got a big smile when I did. Not sure when I'll use it. :)
– Zachary Young
May 23 '15 at 18:39
add a comment |
up vote
7
down vote
The convention is prepend "_" to internal classes, functions, and variables.
add a comment |
up vote
4
down vote
To address the issue of design conventions, and as Christopher said, there's really no such thing as "private" in Python. This may sound twisted for someone coming from C/C++ background (like me a while back), but eventually, you'll probably realize following conventions is plenty enough.
Seeing something having an underscore in front should be a good enough hint not to use it directly. If you're concerned with cluttering help(MyClass)
output (which is what everyone looks at when searching on how to use a class), the underscored attributes/classes are not included there, so you'll end up just having your "public" interface described.
Plus, having everything public has its own awesome perks, like for instance, you can unit test pretty much anything from outside (which you can't really do with C/C++ private constructs).
add a comment |
up vote
2
down vote
Use two underscores to prefix names of "private" identifiers. For classes in a module, use a single leading underscore and they will not be imported using "from module import *".
class _MyInternalClass:
def __my_private_method:
pass
(There is no such thing as true "private" in Python. For example, Python just automatically mangles the names of class members with double underscores to be __clssname_mymember
. So really, if you know the mangled name you can use the "private" entity anyway. See here. And of course you can choose to manually import "internal" classes if you wanted to).
Why two _'s? One is sufficient.
– S.Lott
Feb 15 '09 at 19:30
A single underscore is sufficient to prevent "import" from importing classes and functions. You need two underscores to induce Python's name mangling feature. Should have been clearer; I edited.
– chroder
Feb 15 '09 at 19:57
Now, the follow-up. Why name mangling? What is the possible benefit of that?
– S.Lott
Feb 15 '09 at 20:01
2
The possible benefit is annoying other developers who need access to those variables :)
– Richard Levasseur
Feb 15 '09 at 20:08
add a comment |
7 Answers
7
active
oldest
votes
7 Answers
7
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
135
down vote
accepted
Use a single underscore prefix:
class _Internal:
...
This is the official Python convention for 'internal' symbols; "from module import *" does not import underscore-prefixed objects.
Edit: Reference to the single underscore convention
2
I did not know the underscore rule extended to classes. I do not want to clutter my namespace when importing, so this behavior is what I was looking for. Thanks!
– oparisy
Feb 15 '09 at 19:52
1
Since you state that this is the "official" python convention, it would be nice with a link. Another post here says that all is the official way and does link to the documentation.
– flodin
Feb 15 '09 at 20:00
10
python.org/dev/peps/pep-0008 -- _single_leading_underscore: weak "internal use" indicator. E.g. "from M import *" does not import objects whose name starts with an underscore. -- Classes for internal use have a leading underscore
– Miles
Feb 15 '09 at 20:16
2
A leading underscore is the convention for marking things as internal, "don't mess with this," whereas all is more for modules designed to be used with "from M import *", without necessarily implying that the module users shouldn't touch that class.
– Miles
Feb 15 '09 at 20:20
add a comment |
up vote
135
down vote
accepted
Use a single underscore prefix:
class _Internal:
...
This is the official Python convention for 'internal' symbols; "from module import *" does not import underscore-prefixed objects.
Edit: Reference to the single underscore convention
2
I did not know the underscore rule extended to classes. I do not want to clutter my namespace when importing, so this behavior is what I was looking for. Thanks!
– oparisy
Feb 15 '09 at 19:52
1
Since you state that this is the "official" python convention, it would be nice with a link. Another post here says that all is the official way and does link to the documentation.
– flodin
Feb 15 '09 at 20:00
10
python.org/dev/peps/pep-0008 -- _single_leading_underscore: weak "internal use" indicator. E.g. "from M import *" does not import objects whose name starts with an underscore. -- Classes for internal use have a leading underscore
– Miles
Feb 15 '09 at 20:16
2
A leading underscore is the convention for marking things as internal, "don't mess with this," whereas all is more for modules designed to be used with "from M import *", without necessarily implying that the module users shouldn't touch that class.
– Miles
Feb 15 '09 at 20:20
add a comment |
up vote
135
down vote
accepted
up vote
135
down vote
accepted
Use a single underscore prefix:
class _Internal:
...
This is the official Python convention for 'internal' symbols; "from module import *" does not import underscore-prefixed objects.
Edit: Reference to the single underscore convention
Use a single underscore prefix:
class _Internal:
...
This is the official Python convention for 'internal' symbols; "from module import *" does not import underscore-prefixed objects.
Edit: Reference to the single underscore convention
edited Nov 11 '16 at 0:06


Kiran Vemuri
6641831
6641831
answered Feb 15 '09 at 15:34
Ferdinand Beyer
47.5k12115124
47.5k12115124
2
I did not know the underscore rule extended to classes. I do not want to clutter my namespace when importing, so this behavior is what I was looking for. Thanks!
– oparisy
Feb 15 '09 at 19:52
1
Since you state that this is the "official" python convention, it would be nice with a link. Another post here says that all is the official way and does link to the documentation.
– flodin
Feb 15 '09 at 20:00
10
python.org/dev/peps/pep-0008 -- _single_leading_underscore: weak "internal use" indicator. E.g. "from M import *" does not import objects whose name starts with an underscore. -- Classes for internal use have a leading underscore
– Miles
Feb 15 '09 at 20:16
2
A leading underscore is the convention for marking things as internal, "don't mess with this," whereas all is more for modules designed to be used with "from M import *", without necessarily implying that the module users shouldn't touch that class.
– Miles
Feb 15 '09 at 20:20
add a comment |
2
I did not know the underscore rule extended to classes. I do not want to clutter my namespace when importing, so this behavior is what I was looking for. Thanks!
– oparisy
Feb 15 '09 at 19:52
1
Since you state that this is the "official" python convention, it would be nice with a link. Another post here says that all is the official way and does link to the documentation.
– flodin
Feb 15 '09 at 20:00
10
python.org/dev/peps/pep-0008 -- _single_leading_underscore: weak "internal use" indicator. E.g. "from M import *" does not import objects whose name starts with an underscore. -- Classes for internal use have a leading underscore
– Miles
Feb 15 '09 at 20:16
2
A leading underscore is the convention for marking things as internal, "don't mess with this," whereas all is more for modules designed to be used with "from M import *", without necessarily implying that the module users shouldn't touch that class.
– Miles
Feb 15 '09 at 20:20
2
2
I did not know the underscore rule extended to classes. I do not want to clutter my namespace when importing, so this behavior is what I was looking for. Thanks!
– oparisy
Feb 15 '09 at 19:52
I did not know the underscore rule extended to classes. I do not want to clutter my namespace when importing, so this behavior is what I was looking for. Thanks!
– oparisy
Feb 15 '09 at 19:52
1
1
Since you state that this is the "official" python convention, it would be nice with a link. Another post here says that all is the official way and does link to the documentation.
– flodin
Feb 15 '09 at 20:00
Since you state that this is the "official" python convention, it would be nice with a link. Another post here says that all is the official way and does link to the documentation.
– flodin
Feb 15 '09 at 20:00
10
10
python.org/dev/peps/pep-0008 -- _single_leading_underscore: weak "internal use" indicator. E.g. "from M import *" does not import objects whose name starts with an underscore. -- Classes for internal use have a leading underscore
– Miles
Feb 15 '09 at 20:16
python.org/dev/peps/pep-0008 -- _single_leading_underscore: weak "internal use" indicator. E.g. "from M import *" does not import objects whose name starts with an underscore. -- Classes for internal use have a leading underscore
– Miles
Feb 15 '09 at 20:16
2
2
A leading underscore is the convention for marking things as internal, "don't mess with this," whereas all is more for modules designed to be used with "from M import *", without necessarily implying that the module users shouldn't touch that class.
– Miles
Feb 15 '09 at 20:20
A leading underscore is the convention for marking things as internal, "don't mess with this," whereas all is more for modules designed to be used with "from M import *", without necessarily implying that the module users shouldn't touch that class.
– Miles
Feb 15 '09 at 20:20
add a comment |
up vote
54
down vote
In short:
You cannot enforce privacy. There are no private classes/methods/functions in Python. At least, not strict privacy as in other languages, such as Java.
You can only indicate/suggest privacy. This follows a convention. The python convention for marking a class/function/method as private is to preface it with an _ (underscore). For example,
def _myfunc()
orclass _MyClass:
. You can also create pseudo-privacy by prefacing the method with two underscores (eg:__foo
). You cannot access the method directly, but you can still call it through a special prefix using the classname (eg:_classname__foo
). So the best you can do is indicate/suggest privacy, not enforce it.
Python is like perl in this respect. To paraphrase a famous line about privacy from the Perl book, the philosophy is that you should stay out of the living room because you weren't invited, not because it is defended with a shotgun.
For more information:
Private variables Python Documentation
Private functions Dive into Python, by Mark Pilgrim
Why are Python’s ‘private’ methods not actually private? StackOverflow question 70528
In response to #1, you sort of can enforce privacy of methods. Using a double underscore like __method(self) will make it inaccessible outside of the class. But there is a way around it by calling it like, Foo()._Foo__method(). I guess it just renames it to something more esoteric.
– Evan Fosmark
Feb 15 '09 at 20:54
1
That quote is accurate.
– Paul Draper
Apr 14 '13 at 7:31
add a comment |
up vote
54
down vote
In short:
You cannot enforce privacy. There are no private classes/methods/functions in Python. At least, not strict privacy as in other languages, such as Java.
You can only indicate/suggest privacy. This follows a convention. The python convention for marking a class/function/method as private is to preface it with an _ (underscore). For example,
def _myfunc()
orclass _MyClass:
. You can also create pseudo-privacy by prefacing the method with two underscores (eg:__foo
). You cannot access the method directly, but you can still call it through a special prefix using the classname (eg:_classname__foo
). So the best you can do is indicate/suggest privacy, not enforce it.
Python is like perl in this respect. To paraphrase a famous line about privacy from the Perl book, the philosophy is that you should stay out of the living room because you weren't invited, not because it is defended with a shotgun.
For more information:
Private variables Python Documentation
Private functions Dive into Python, by Mark Pilgrim
Why are Python’s ‘private’ methods not actually private? StackOverflow question 70528
In response to #1, you sort of can enforce privacy of methods. Using a double underscore like __method(self) will make it inaccessible outside of the class. But there is a way around it by calling it like, Foo()._Foo__method(). I guess it just renames it to something more esoteric.
– Evan Fosmark
Feb 15 '09 at 20:54
1
That quote is accurate.
– Paul Draper
Apr 14 '13 at 7:31
add a comment |
up vote
54
down vote
up vote
54
down vote
In short:
You cannot enforce privacy. There are no private classes/methods/functions in Python. At least, not strict privacy as in other languages, such as Java.
You can only indicate/suggest privacy. This follows a convention. The python convention for marking a class/function/method as private is to preface it with an _ (underscore). For example,
def _myfunc()
orclass _MyClass:
. You can also create pseudo-privacy by prefacing the method with two underscores (eg:__foo
). You cannot access the method directly, but you can still call it through a special prefix using the classname (eg:_classname__foo
). So the best you can do is indicate/suggest privacy, not enforce it.
Python is like perl in this respect. To paraphrase a famous line about privacy from the Perl book, the philosophy is that you should stay out of the living room because you weren't invited, not because it is defended with a shotgun.
For more information:
Private variables Python Documentation
Private functions Dive into Python, by Mark Pilgrim
Why are Python’s ‘private’ methods not actually private? StackOverflow question 70528
In short:
You cannot enforce privacy. There are no private classes/methods/functions in Python. At least, not strict privacy as in other languages, such as Java.
You can only indicate/suggest privacy. This follows a convention. The python convention for marking a class/function/method as private is to preface it with an _ (underscore). For example,
def _myfunc()
orclass _MyClass:
. You can also create pseudo-privacy by prefacing the method with two underscores (eg:__foo
). You cannot access the method directly, but you can still call it through a special prefix using the classname (eg:_classname__foo
). So the best you can do is indicate/suggest privacy, not enforce it.
Python is like perl in this respect. To paraphrase a famous line about privacy from the Perl book, the philosophy is that you should stay out of the living room because you weren't invited, not because it is defended with a shotgun.
For more information:
Private variables Python Documentation
Private functions Dive into Python, by Mark Pilgrim
Why are Python’s ‘private’ methods not actually private? StackOverflow question 70528
edited Jun 21 at 13:49
BlackVegetable
5,60033562
5,60033562
answered Feb 15 '09 at 18:31
Karl Fast
3,4163188
3,4163188
In response to #1, you sort of can enforce privacy of methods. Using a double underscore like __method(self) will make it inaccessible outside of the class. But there is a way around it by calling it like, Foo()._Foo__method(). I guess it just renames it to something more esoteric.
– Evan Fosmark
Feb 15 '09 at 20:54
1
That quote is accurate.
– Paul Draper
Apr 14 '13 at 7:31
add a comment |
In response to #1, you sort of can enforce privacy of methods. Using a double underscore like __method(self) will make it inaccessible outside of the class. But there is a way around it by calling it like, Foo()._Foo__method(). I guess it just renames it to something more esoteric.
– Evan Fosmark
Feb 15 '09 at 20:54
1
That quote is accurate.
– Paul Draper
Apr 14 '13 at 7:31
In response to #1, you sort of can enforce privacy of methods. Using a double underscore like __method(self) will make it inaccessible outside of the class. But there is a way around it by calling it like, Foo()._Foo__method(). I guess it just renames it to something more esoteric.
– Evan Fosmark
Feb 15 '09 at 20:54
In response to #1, you sort of can enforce privacy of methods. Using a double underscore like __method(self) will make it inaccessible outside of the class. But there is a way around it by calling it like, Foo()._Foo__method(). I guess it just renames it to something more esoteric.
– Evan Fosmark
Feb 15 '09 at 20:54
1
1
That quote is accurate.
– Paul Draper
Apr 14 '13 at 7:31
That quote is accurate.
– Paul Draper
Apr 14 '13 at 7:31
add a comment |
up vote
35
down vote
Define __all__
, a list of names that you want to be exported (see documentation).
__all__ = ['public_class'] # don't add here the 'implementation_class'
add a comment |
up vote
35
down vote
Define __all__
, a list of names that you want to be exported (see documentation).
__all__ = ['public_class'] # don't add here the 'implementation_class'
add a comment |
up vote
35
down vote
up vote
35
down vote
Define __all__
, a list of names that you want to be exported (see documentation).
__all__ = ['public_class'] # don't add here the 'implementation_class'
Define __all__
, a list of names that you want to be exported (see documentation).
__all__ = ['public_class'] # don't add here the 'implementation_class'
answered Feb 15 '09 at 15:36
UncleZeiv
14.2k64168
14.2k64168
add a comment |
add a comment |
up vote
9
down vote
A pattern that I sometimes use is this:
Define a class:
class x(object):
def doThis(self):
...
def doThat(self):
...
Create an instance of the class, overwriting the class name:
x = x()
Define symbols that expose the functionality:
doThis = x.doThis
doThat = x.doThat
Delete the instance itself:
del x
Now you have a module that only exposes your public functions.
that's kinda cool!
– ʞɔıu
Feb 15 '09 at 18:52
1
Took a minute to understand the purpose/function of "overwriting the class name", but I got a big smile when I did. Not sure when I'll use it. :)
– Zachary Young
May 23 '15 at 18:39
add a comment |
up vote
9
down vote
A pattern that I sometimes use is this:
Define a class:
class x(object):
def doThis(self):
...
def doThat(self):
...
Create an instance of the class, overwriting the class name:
x = x()
Define symbols that expose the functionality:
doThis = x.doThis
doThat = x.doThat
Delete the instance itself:
del x
Now you have a module that only exposes your public functions.
that's kinda cool!
– ʞɔıu
Feb 15 '09 at 18:52
1
Took a minute to understand the purpose/function of "overwriting the class name", but I got a big smile when I did. Not sure when I'll use it. :)
– Zachary Young
May 23 '15 at 18:39
add a comment |
up vote
9
down vote
up vote
9
down vote
A pattern that I sometimes use is this:
Define a class:
class x(object):
def doThis(self):
...
def doThat(self):
...
Create an instance of the class, overwriting the class name:
x = x()
Define symbols that expose the functionality:
doThis = x.doThis
doThat = x.doThat
Delete the instance itself:
del x
Now you have a module that only exposes your public functions.
A pattern that I sometimes use is this:
Define a class:
class x(object):
def doThis(self):
...
def doThat(self):
...
Create an instance of the class, overwriting the class name:
x = x()
Define symbols that expose the functionality:
doThis = x.doThis
doThat = x.doThat
Delete the instance itself:
del x
Now you have a module that only exposes your public functions.
answered Feb 15 '09 at 15:58
theller
2,2661115
2,2661115
that's kinda cool!
– ʞɔıu
Feb 15 '09 at 18:52
1
Took a minute to understand the purpose/function of "overwriting the class name", but I got a big smile when I did. Not sure when I'll use it. :)
– Zachary Young
May 23 '15 at 18:39
add a comment |
that's kinda cool!
– ʞɔıu
Feb 15 '09 at 18:52
1
Took a minute to understand the purpose/function of "overwriting the class name", but I got a big smile when I did. Not sure when I'll use it. :)
– Zachary Young
May 23 '15 at 18:39
that's kinda cool!
– ʞɔıu
Feb 15 '09 at 18:52
that's kinda cool!
– ʞɔıu
Feb 15 '09 at 18:52
1
1
Took a minute to understand the purpose/function of "overwriting the class name", but I got a big smile when I did. Not sure when I'll use it. :)
– Zachary Young
May 23 '15 at 18:39
Took a minute to understand the purpose/function of "overwriting the class name", but I got a big smile when I did. Not sure when I'll use it. :)
– Zachary Young
May 23 '15 at 18:39
add a comment |
up vote
7
down vote
The convention is prepend "_" to internal classes, functions, and variables.
add a comment |
up vote
7
down vote
The convention is prepend "_" to internal classes, functions, and variables.
add a comment |
up vote
7
down vote
up vote
7
down vote
The convention is prepend "_" to internal classes, functions, and variables.
The convention is prepend "_" to internal classes, functions, and variables.
answered Feb 15 '09 at 15:33
Benjamin Peterson
10.4k62532
10.4k62532
add a comment |
add a comment |
up vote
4
down vote
To address the issue of design conventions, and as Christopher said, there's really no such thing as "private" in Python. This may sound twisted for someone coming from C/C++ background (like me a while back), but eventually, you'll probably realize following conventions is plenty enough.
Seeing something having an underscore in front should be a good enough hint not to use it directly. If you're concerned with cluttering help(MyClass)
output (which is what everyone looks at when searching on how to use a class), the underscored attributes/classes are not included there, so you'll end up just having your "public" interface described.
Plus, having everything public has its own awesome perks, like for instance, you can unit test pretty much anything from outside (which you can't really do with C/C++ private constructs).
add a comment |
up vote
4
down vote
To address the issue of design conventions, and as Christopher said, there's really no such thing as "private" in Python. This may sound twisted for someone coming from C/C++ background (like me a while back), but eventually, you'll probably realize following conventions is plenty enough.
Seeing something having an underscore in front should be a good enough hint not to use it directly. If you're concerned with cluttering help(MyClass)
output (which is what everyone looks at when searching on how to use a class), the underscored attributes/classes are not included there, so you'll end up just having your "public" interface described.
Plus, having everything public has its own awesome perks, like for instance, you can unit test pretty much anything from outside (which you can't really do with C/C++ private constructs).
add a comment |
up vote
4
down vote
up vote
4
down vote
To address the issue of design conventions, and as Christopher said, there's really no such thing as "private" in Python. This may sound twisted for someone coming from C/C++ background (like me a while back), but eventually, you'll probably realize following conventions is plenty enough.
Seeing something having an underscore in front should be a good enough hint not to use it directly. If you're concerned with cluttering help(MyClass)
output (which is what everyone looks at when searching on how to use a class), the underscored attributes/classes are not included there, so you'll end up just having your "public" interface described.
Plus, having everything public has its own awesome perks, like for instance, you can unit test pretty much anything from outside (which you can't really do with C/C++ private constructs).
To address the issue of design conventions, and as Christopher said, there's really no such thing as "private" in Python. This may sound twisted for someone coming from C/C++ background (like me a while back), but eventually, you'll probably realize following conventions is plenty enough.
Seeing something having an underscore in front should be a good enough hint not to use it directly. If you're concerned with cluttering help(MyClass)
output (which is what everyone looks at when searching on how to use a class), the underscored attributes/classes are not included there, so you'll end up just having your "public" interface described.
Plus, having everything public has its own awesome perks, like for instance, you can unit test pretty much anything from outside (which you can't really do with C/C++ private constructs).
answered Feb 15 '09 at 18:09
Dimitri Tcaciuc
1,71321420
1,71321420
add a comment |
add a comment |
up vote
2
down vote
Use two underscores to prefix names of "private" identifiers. For classes in a module, use a single leading underscore and they will not be imported using "from module import *".
class _MyInternalClass:
def __my_private_method:
pass
(There is no such thing as true "private" in Python. For example, Python just automatically mangles the names of class members with double underscores to be __clssname_mymember
. So really, if you know the mangled name you can use the "private" entity anyway. See here. And of course you can choose to manually import "internal" classes if you wanted to).
Why two _'s? One is sufficient.
– S.Lott
Feb 15 '09 at 19:30
A single underscore is sufficient to prevent "import" from importing classes and functions. You need two underscores to induce Python's name mangling feature. Should have been clearer; I edited.
– chroder
Feb 15 '09 at 19:57
Now, the follow-up. Why name mangling? What is the possible benefit of that?
– S.Lott
Feb 15 '09 at 20:01
2
The possible benefit is annoying other developers who need access to those variables :)
– Richard Levasseur
Feb 15 '09 at 20:08
add a comment |
up vote
2
down vote
Use two underscores to prefix names of "private" identifiers. For classes in a module, use a single leading underscore and they will not be imported using "from module import *".
class _MyInternalClass:
def __my_private_method:
pass
(There is no such thing as true "private" in Python. For example, Python just automatically mangles the names of class members with double underscores to be __clssname_mymember
. So really, if you know the mangled name you can use the "private" entity anyway. See here. And of course you can choose to manually import "internal" classes if you wanted to).
Why two _'s? One is sufficient.
– S.Lott
Feb 15 '09 at 19:30
A single underscore is sufficient to prevent "import" from importing classes and functions. You need two underscores to induce Python's name mangling feature. Should have been clearer; I edited.
– chroder
Feb 15 '09 at 19:57
Now, the follow-up. Why name mangling? What is the possible benefit of that?
– S.Lott
Feb 15 '09 at 20:01
2
The possible benefit is annoying other developers who need access to those variables :)
– Richard Levasseur
Feb 15 '09 at 20:08
add a comment |
up vote
2
down vote
up vote
2
down vote
Use two underscores to prefix names of "private" identifiers. For classes in a module, use a single leading underscore and they will not be imported using "from module import *".
class _MyInternalClass:
def __my_private_method:
pass
(There is no such thing as true "private" in Python. For example, Python just automatically mangles the names of class members with double underscores to be __clssname_mymember
. So really, if you know the mangled name you can use the "private" entity anyway. See here. And of course you can choose to manually import "internal" classes if you wanted to).
Use two underscores to prefix names of "private" identifiers. For classes in a module, use a single leading underscore and they will not be imported using "from module import *".
class _MyInternalClass:
def __my_private_method:
pass
(There is no such thing as true "private" in Python. For example, Python just automatically mangles the names of class members with double underscores to be __clssname_mymember
. So really, if you know the mangled name you can use the "private" entity anyway. See here. And of course you can choose to manually import "internal" classes if you wanted to).
edited Feb 15 '09 at 19:59
answered Feb 15 '09 at 16:37


chroder
3,28712139
3,28712139
Why two _'s? One is sufficient.
– S.Lott
Feb 15 '09 at 19:30
A single underscore is sufficient to prevent "import" from importing classes and functions. You need two underscores to induce Python's name mangling feature. Should have been clearer; I edited.
– chroder
Feb 15 '09 at 19:57
Now, the follow-up. Why name mangling? What is the possible benefit of that?
– S.Lott
Feb 15 '09 at 20:01
2
The possible benefit is annoying other developers who need access to those variables :)
– Richard Levasseur
Feb 15 '09 at 20:08
add a comment |
Why two _'s? One is sufficient.
– S.Lott
Feb 15 '09 at 19:30
A single underscore is sufficient to prevent "import" from importing classes and functions. You need two underscores to induce Python's name mangling feature. Should have been clearer; I edited.
– chroder
Feb 15 '09 at 19:57
Now, the follow-up. Why name mangling? What is the possible benefit of that?
– S.Lott
Feb 15 '09 at 20:01
2
The possible benefit is annoying other developers who need access to those variables :)
– Richard Levasseur
Feb 15 '09 at 20:08
Why two _'s? One is sufficient.
– S.Lott
Feb 15 '09 at 19:30
Why two _'s? One is sufficient.
– S.Lott
Feb 15 '09 at 19:30
A single underscore is sufficient to prevent "import" from importing classes and functions. You need two underscores to induce Python's name mangling feature. Should have been clearer; I edited.
– chroder
Feb 15 '09 at 19:57
A single underscore is sufficient to prevent "import" from importing classes and functions. You need two underscores to induce Python's name mangling feature. Should have been clearer; I edited.
– chroder
Feb 15 '09 at 19:57
Now, the follow-up. Why name mangling? What is the possible benefit of that?
– S.Lott
Feb 15 '09 at 20:01
Now, the follow-up. Why name mangling? What is the possible benefit of that?
– S.Lott
Feb 15 '09 at 20:01
2
2
The possible benefit is annoying other developers who need access to those variables :)
– Richard Levasseur
Feb 15 '09 at 20:08
The possible benefit is annoying other developers who need access to those variables :)
– Richard Levasseur
Feb 15 '09 at 20:08
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f551038%2fprivate-implementation-class-in-python%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ElN6eyV5pjn1 ORI XTrJq h yanjghnv21t,MaaJOgS BNI7tczJJ3gz0 dIfKbjCnbq6r1qNLt9P1Pw,GK,TNDV89e0dIyiAHdc,v ahi