Sort nested dictionary by value in JS
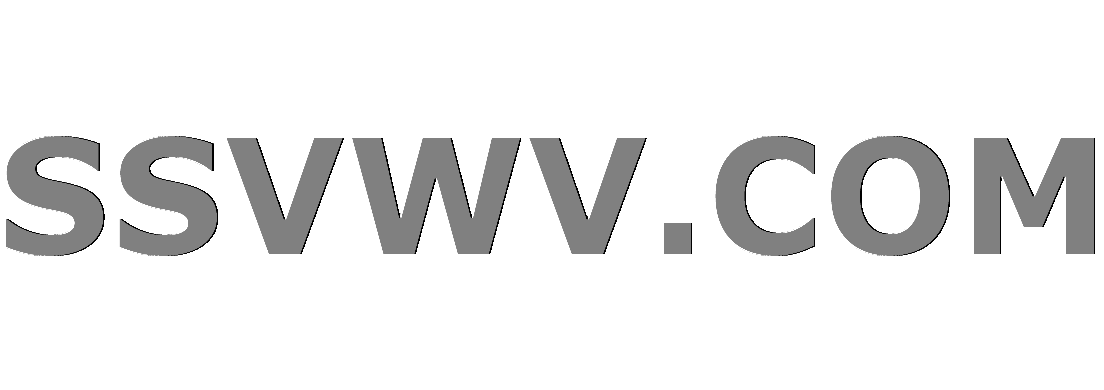
Multi tool use
up vote
-1
down vote
favorite
Can someone please help to figure out the way to sort a dictionary with another dictionary inside in JavaScript?
This is how my dict looks like:
'POS2': 'stegano': 0, 'sum': 200, 'misc': 100, 'web': 0, 'ppc': 0, 'crypto': 0, 'admin': 0, 'vuln': 0, 'forensics': 0, 'hardware': 0, 'reverse': 0, 'recon': 100, ...
I want to sort it by 'sum' key that is stored in nested dict.
I can find some solutions written in python, but the challenge here is to achieve the same functionality in JS.
Would appreciate any help.
javascript dictionary
add a comment |
up vote
-1
down vote
favorite
Can someone please help to figure out the way to sort a dictionary with another dictionary inside in JavaScript?
This is how my dict looks like:
'POS2': 'stegano': 0, 'sum': 200, 'misc': 100, 'web': 0, 'ppc': 0, 'crypto': 0, 'admin': 0, 'vuln': 0, 'forensics': 0, 'hardware': 0, 'reverse': 0, 'recon': 100, ...
I want to sort it by 'sum' key that is stored in nested dict.
I can find some solutions written in python, but the challenge here is to achieve the same functionality in JS.
Would appreciate any help.
javascript dictionary
1
You would then expect an array as output, yes?
– Icepickle
Nov 11 at 12:17
1
Objects are unordered sets of properties. So when you speak ofsort
what output do you expect?
– trincot
Nov 11 at 12:17
Thank you, both , for your interest. At first I thought of having dictionary as an output, but having the elements inside reorganized, so the first element would have the smallest sum number. But now that @trincot has mentioned and I did my checking, I can see why that wouldn’t work. My dictionary is an object in JS, which properties are unordered. So, I suppose having an array as an output is the way to go
– Ilja Leiko
Nov 11 at 12:28
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
Can someone please help to figure out the way to sort a dictionary with another dictionary inside in JavaScript?
This is how my dict looks like:
'POS2': 'stegano': 0, 'sum': 200, 'misc': 100, 'web': 0, 'ppc': 0, 'crypto': 0, 'admin': 0, 'vuln': 0, 'forensics': 0, 'hardware': 0, 'reverse': 0, 'recon': 100, ...
I want to sort it by 'sum' key that is stored in nested dict.
I can find some solutions written in python, but the challenge here is to achieve the same functionality in JS.
Would appreciate any help.
javascript dictionary
Can someone please help to figure out the way to sort a dictionary with another dictionary inside in JavaScript?
This is how my dict looks like:
'POS2': 'stegano': 0, 'sum': 200, 'misc': 100, 'web': 0, 'ppc': 0, 'crypto': 0, 'admin': 0, 'vuln': 0, 'forensics': 0, 'hardware': 0, 'reverse': 0, 'recon': 100, ...
I want to sort it by 'sum' key that is stored in nested dict.
I can find some solutions written in python, but the challenge here is to achieve the same functionality in JS.
Would appreciate any help.
javascript dictionary
javascript dictionary
asked Nov 11 at 12:13
Ilja Leiko
6812
6812
1
You would then expect an array as output, yes?
– Icepickle
Nov 11 at 12:17
1
Objects are unordered sets of properties. So when you speak ofsort
what output do you expect?
– trincot
Nov 11 at 12:17
Thank you, both , for your interest. At first I thought of having dictionary as an output, but having the elements inside reorganized, so the first element would have the smallest sum number. But now that @trincot has mentioned and I did my checking, I can see why that wouldn’t work. My dictionary is an object in JS, which properties are unordered. So, I suppose having an array as an output is the way to go
– Ilja Leiko
Nov 11 at 12:28
add a comment |
1
You would then expect an array as output, yes?
– Icepickle
Nov 11 at 12:17
1
Objects are unordered sets of properties. So when you speak ofsort
what output do you expect?
– trincot
Nov 11 at 12:17
Thank you, both , for your interest. At first I thought of having dictionary as an output, but having the elements inside reorganized, so the first element would have the smallest sum number. But now that @trincot has mentioned and I did my checking, I can see why that wouldn’t work. My dictionary is an object in JS, which properties are unordered. So, I suppose having an array as an output is the way to go
– Ilja Leiko
Nov 11 at 12:28
1
1
You would then expect an array as output, yes?
– Icepickle
Nov 11 at 12:17
You would then expect an array as output, yes?
– Icepickle
Nov 11 at 12:17
1
1
Objects are unordered sets of properties. So when you speak of
sort
what output do you expect?– trincot
Nov 11 at 12:17
Objects are unordered sets of properties. So when you speak of
sort
what output do you expect?– trincot
Nov 11 at 12:17
Thank you, both , for your interest. At first I thought of having dictionary as an output, but having the elements inside reorganized, so the first element would have the smallest sum number. But now that @trincot has mentioned and I did my checking, I can see why that wouldn’t work. My dictionary is an object in JS, which properties are unordered. So, I suppose having an array as an output is the way to go
– Ilja Leiko
Nov 11 at 12:28
Thank you, both , for your interest. At first I thought of having dictionary as an output, but having the elements inside reorganized, so the first element would have the smallest sum number. But now that @trincot has mentioned and I did my checking, I can see why that wouldn’t work. My dictionary is an object in JS, which properties are unordered. So, I suppose having an array as an output is the way to go
– Ilja Leiko
Nov 11 at 12:28
add a comment |
3 Answers
3
active
oldest
votes
up vote
0
down vote
Just retrieve the inner object using Object.values
and then use Array.sort
to sort by it's content. Note that you cannot sort an objects properties as they are per default an unsorted set (or at least, it's not properly described how properties should be sorted on an object)
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.values( input ).map( value => value ).sort( (a, b) => a[key] - b[key] );
console.log( orderBySubKey( dict, 'sum' ) );
This sample would remove the outer properties, if you want to keep these, you can change your ordering a bit, for example like
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.keys( input ).map( key => ( key, value: input[key] ) ).sort( (a, b) => a.value[key] - b.value[key] );
console.log( orderBySubKey( dict, 'sum' ) );
Which gives you a different output, but would still allow you to lookup inside the original dictionary
add a comment |
up vote
0
down vote
Since you can't order objects by property you'd need to map
the objects to an array and then sort that. And you'd probably not want to lose the key for each nested object (like POS2), so you'd want to embed that key in the object for safe-keeping.
const obj = "POS2":"stegano":0,"sum":2200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS3":"stegano":0,"sum":1200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS4":"stegano":0,"sum":22,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS5":"stegano":0,"sum":2001,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100
// map over the object keys
const out = Object.keys(obj).map(key =>
// on each iteration return the object with its new key property
return ...obj[key], key
// then sort the array of object by their sums
).sort((a, b) => a.sum - b.sum);
console.log(out);
add a comment |
up vote
0
down vote
For example, you have next array (similar to your array, but has less properties to readable):
let obj =
pos1: sum: 100, name: 'p1' ,
pos2: sum: 1, name: 'p2' ,
pos3: sum: 50, name: 'p3'
;
var result = Object.keys(obj)
.map(key => return key, val: obj[key]) // output: unsorted array
.sort((a, b) => a.val.sum > b.val.sum);
console.log(result);
and as result you will see:
[ key: 'pos2', val: sum: 1, name: 'p2' ,
key: 'pos3', val: sum: 50, name: 'p3' ,
key: 'pos1', val: sum: 100, name: 'p1' ]
And what if the OP wanted to keep the key names likePOS2
?
– Andy
Nov 11 at 12:22
@Andy yes, you right. i missed that input parameter is object, not array. I fixed my answer
– Sergey Shulik
Nov 11 at 12:41
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Just retrieve the inner object using Object.values
and then use Array.sort
to sort by it's content. Note that you cannot sort an objects properties as they are per default an unsorted set (or at least, it's not properly described how properties should be sorted on an object)
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.values( input ).map( value => value ).sort( (a, b) => a[key] - b[key] );
console.log( orderBySubKey( dict, 'sum' ) );
This sample would remove the outer properties, if you want to keep these, you can change your ordering a bit, for example like
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.keys( input ).map( key => ( key, value: input[key] ) ).sort( (a, b) => a.value[key] - b.value[key] );
console.log( orderBySubKey( dict, 'sum' ) );
Which gives you a different output, but would still allow you to lookup inside the original dictionary
add a comment |
up vote
0
down vote
Just retrieve the inner object using Object.values
and then use Array.sort
to sort by it's content. Note that you cannot sort an objects properties as they are per default an unsorted set (or at least, it's not properly described how properties should be sorted on an object)
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.values( input ).map( value => value ).sort( (a, b) => a[key] - b[key] );
console.log( orderBySubKey( dict, 'sum' ) );
This sample would remove the outer properties, if you want to keep these, you can change your ordering a bit, for example like
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.keys( input ).map( key => ( key, value: input[key] ) ).sort( (a, b) => a.value[key] - b.value[key] );
console.log( orderBySubKey( dict, 'sum' ) );
Which gives you a different output, but would still allow you to lookup inside the original dictionary
add a comment |
up vote
0
down vote
up vote
0
down vote
Just retrieve the inner object using Object.values
and then use Array.sort
to sort by it's content. Note that you cannot sort an objects properties as they are per default an unsorted set (or at least, it's not properly described how properties should be sorted on an object)
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.values( input ).map( value => value ).sort( (a, b) => a[key] - b[key] );
console.log( orderBySubKey( dict, 'sum' ) );
This sample would remove the outer properties, if you want to keep these, you can change your ordering a bit, for example like
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.keys( input ).map( key => ( key, value: input[key] ) ).sort( (a, b) => a.value[key] - b.value[key] );
console.log( orderBySubKey( dict, 'sum' ) );
Which gives you a different output, but would still allow you to lookup inside the original dictionary
Just retrieve the inner object using Object.values
and then use Array.sort
to sort by it's content. Note that you cannot sort an objects properties as they are per default an unsorted set (or at least, it's not properly described how properties should be sorted on an object)
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.values( input ).map( value => value ).sort( (a, b) => a[key] - b[key] );
console.log( orderBySubKey( dict, 'sum' ) );
This sample would remove the outer properties, if you want to keep these, you can change your ordering a bit, for example like
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.keys( input ).map( key => ( key, value: input[key] ) ).sort( (a, b) => a.value[key] - b.value[key] );
console.log( orderBySubKey( dict, 'sum' ) );
Which gives you a different output, but would still allow you to lookup inside the original dictionary
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.values( input ).map( value => value ).sort( (a, b) => a[key] - b[key] );
console.log( orderBySubKey( dict, 'sum' ) );
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.values( input ).map( value => value ).sort( (a, b) => a[key] - b[key] );
console.log( orderBySubKey( dict, 'sum' ) );
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.keys( input ).map( key => ( key, value: input[key] ) ).sort( (a, b) => a.value[key] - b.value[key] );
console.log( orderBySubKey( dict, 'sum' ) );
const dict =
'POS2':
'stegano': 0,
'sum': 200,
'misc': 100,
'web': 0,
'ppc': 0,
'crypto': 0,
'admin': 0,
'vuln': 0,
'forensics': 0,
'hardware': 0,
'reverse': 0,
'recon': 100
,
'POS1':
'sum': 100
,
'POS3':
'sum': 250
;
function orderBySubKey( input, key )
return Object.keys( input ).map( key => ( key, value: input[key] ) ).sort( (a, b) => a.value[key] - b.value[key] );
console.log( orderBySubKey( dict, 'sum' ) );
answered Nov 11 at 12:24


Icepickle
8,43232034
8,43232034
add a comment |
add a comment |
up vote
0
down vote
Since you can't order objects by property you'd need to map
the objects to an array and then sort that. And you'd probably not want to lose the key for each nested object (like POS2), so you'd want to embed that key in the object for safe-keeping.
const obj = "POS2":"stegano":0,"sum":2200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS3":"stegano":0,"sum":1200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS4":"stegano":0,"sum":22,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS5":"stegano":0,"sum":2001,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100
// map over the object keys
const out = Object.keys(obj).map(key =>
// on each iteration return the object with its new key property
return ...obj[key], key
// then sort the array of object by their sums
).sort((a, b) => a.sum - b.sum);
console.log(out);
add a comment |
up vote
0
down vote
Since you can't order objects by property you'd need to map
the objects to an array and then sort that. And you'd probably not want to lose the key for each nested object (like POS2), so you'd want to embed that key in the object for safe-keeping.
const obj = "POS2":"stegano":0,"sum":2200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS3":"stegano":0,"sum":1200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS4":"stegano":0,"sum":22,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS5":"stegano":0,"sum":2001,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100
// map over the object keys
const out = Object.keys(obj).map(key =>
// on each iteration return the object with its new key property
return ...obj[key], key
// then sort the array of object by their sums
).sort((a, b) => a.sum - b.sum);
console.log(out);
add a comment |
up vote
0
down vote
up vote
0
down vote
Since you can't order objects by property you'd need to map
the objects to an array and then sort that. And you'd probably not want to lose the key for each nested object (like POS2), so you'd want to embed that key in the object for safe-keeping.
const obj = "POS2":"stegano":0,"sum":2200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS3":"stegano":0,"sum":1200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS4":"stegano":0,"sum":22,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS5":"stegano":0,"sum":2001,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100
// map over the object keys
const out = Object.keys(obj).map(key =>
// on each iteration return the object with its new key property
return ...obj[key], key
// then sort the array of object by their sums
).sort((a, b) => a.sum - b.sum);
console.log(out);
Since you can't order objects by property you'd need to map
the objects to an array and then sort that. And you'd probably not want to lose the key for each nested object (like POS2), so you'd want to embed that key in the object for safe-keeping.
const obj = "POS2":"stegano":0,"sum":2200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS3":"stegano":0,"sum":1200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS4":"stegano":0,"sum":22,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS5":"stegano":0,"sum":2001,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100
// map over the object keys
const out = Object.keys(obj).map(key =>
// on each iteration return the object with its new key property
return ...obj[key], key
// then sort the array of object by their sums
).sort((a, b) => a.sum - b.sum);
console.log(out);
const obj = "POS2":"stegano":0,"sum":2200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS3":"stegano":0,"sum":1200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS4":"stegano":0,"sum":22,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS5":"stegano":0,"sum":2001,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100
// map over the object keys
const out = Object.keys(obj).map(key =>
// on each iteration return the object with its new key property
return ...obj[key], key
// then sort the array of object by their sums
).sort((a, b) => a.sum - b.sum);
console.log(out);
const obj = "POS2":"stegano":0,"sum":2200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS3":"stegano":0,"sum":1200,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS4":"stegano":0,"sum":22,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100,"POS5":"stegano":0,"sum":2001,"misc":100,"web":0,"ppc":0,"crypto":0,"admin":0,"vuln":0,"forensics":0,"hardware":0,"reverse":0,"recon":100
// map over the object keys
const out = Object.keys(obj).map(key =>
// on each iteration return the object with its new key property
return ...obj[key], key
// then sort the array of object by their sums
).sort((a, b) => a.sum - b.sum);
console.log(out);
answered Nov 11 at 12:36


Andy
27.8k63160
27.8k63160
add a comment |
add a comment |
up vote
0
down vote
For example, you have next array (similar to your array, but has less properties to readable):
let obj =
pos1: sum: 100, name: 'p1' ,
pos2: sum: 1, name: 'p2' ,
pos3: sum: 50, name: 'p3'
;
var result = Object.keys(obj)
.map(key => return key, val: obj[key]) // output: unsorted array
.sort((a, b) => a.val.sum > b.val.sum);
console.log(result);
and as result you will see:
[ key: 'pos2', val: sum: 1, name: 'p2' ,
key: 'pos3', val: sum: 50, name: 'p3' ,
key: 'pos1', val: sum: 100, name: 'p1' ]
And what if the OP wanted to keep the key names likePOS2
?
– Andy
Nov 11 at 12:22
@Andy yes, you right. i missed that input parameter is object, not array. I fixed my answer
– Sergey Shulik
Nov 11 at 12:41
add a comment |
up vote
0
down vote
For example, you have next array (similar to your array, but has less properties to readable):
let obj =
pos1: sum: 100, name: 'p1' ,
pos2: sum: 1, name: 'p2' ,
pos3: sum: 50, name: 'p3'
;
var result = Object.keys(obj)
.map(key => return key, val: obj[key]) // output: unsorted array
.sort((a, b) => a.val.sum > b.val.sum);
console.log(result);
and as result you will see:
[ key: 'pos2', val: sum: 1, name: 'p2' ,
key: 'pos3', val: sum: 50, name: 'p3' ,
key: 'pos1', val: sum: 100, name: 'p1' ]
And what if the OP wanted to keep the key names likePOS2
?
– Andy
Nov 11 at 12:22
@Andy yes, you right. i missed that input parameter is object, not array. I fixed my answer
– Sergey Shulik
Nov 11 at 12:41
add a comment |
up vote
0
down vote
up vote
0
down vote
For example, you have next array (similar to your array, but has less properties to readable):
let obj =
pos1: sum: 100, name: 'p1' ,
pos2: sum: 1, name: 'p2' ,
pos3: sum: 50, name: 'p3'
;
var result = Object.keys(obj)
.map(key => return key, val: obj[key]) // output: unsorted array
.sort((a, b) => a.val.sum > b.val.sum);
console.log(result);
and as result you will see:
[ key: 'pos2', val: sum: 1, name: 'p2' ,
key: 'pos3', val: sum: 50, name: 'p3' ,
key: 'pos1', val: sum: 100, name: 'p1' ]
For example, you have next array (similar to your array, but has less properties to readable):
let obj =
pos1: sum: 100, name: 'p1' ,
pos2: sum: 1, name: 'p2' ,
pos3: sum: 50, name: 'p3'
;
var result = Object.keys(obj)
.map(key => return key, val: obj[key]) // output: unsorted array
.sort((a, b) => a.val.sum > b.val.sum);
console.log(result);
and as result you will see:
[ key: 'pos2', val: sum: 1, name: 'p2' ,
key: 'pos3', val: sum: 50, name: 'p3' ,
key: 'pos1', val: sum: 100, name: 'p1' ]
edited Nov 11 at 12:41
answered Nov 11 at 12:20


Sergey Shulik
678824
678824
And what if the OP wanted to keep the key names likePOS2
?
– Andy
Nov 11 at 12:22
@Andy yes, you right. i missed that input parameter is object, not array. I fixed my answer
– Sergey Shulik
Nov 11 at 12:41
add a comment |
And what if the OP wanted to keep the key names likePOS2
?
– Andy
Nov 11 at 12:22
@Andy yes, you right. i missed that input parameter is object, not array. I fixed my answer
– Sergey Shulik
Nov 11 at 12:41
And what if the OP wanted to keep the key names like
POS2
?– Andy
Nov 11 at 12:22
And what if the OP wanted to keep the key names like
POS2
?– Andy
Nov 11 at 12:22
@Andy yes, you right. i missed that input parameter is object, not array. I fixed my answer
– Sergey Shulik
Nov 11 at 12:41
@Andy yes, you right. i missed that input parameter is object, not array. I fixed my answer
– Sergey Shulik
Nov 11 at 12:41
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53248638%2fsort-nested-dictionary-by-value-in-js%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Kq7dp3UnWOutAoIRUB BcYRObj 0PYP i7mZBCo SVJbHXATCmks9cTSclBQ7PbpR6PqayzEo,GietCLiQ 5U,1LnPAIsZl,84
1
You would then expect an array as output, yes?
– Icepickle
Nov 11 at 12:17
1
Objects are unordered sets of properties. So when you speak of
sort
what output do you expect?– trincot
Nov 11 at 12:17
Thank you, both , for your interest. At first I thought of having dictionary as an output, but having the elements inside reorganized, so the first element would have the smallest sum number. But now that @trincot has mentioned and I did my checking, I can see why that wouldn’t work. My dictionary is an object in JS, which properties are unordered. So, I suppose having an array as an output is the way to go
– Ilja Leiko
Nov 11 at 12:28