Return HTML page from Spring controller
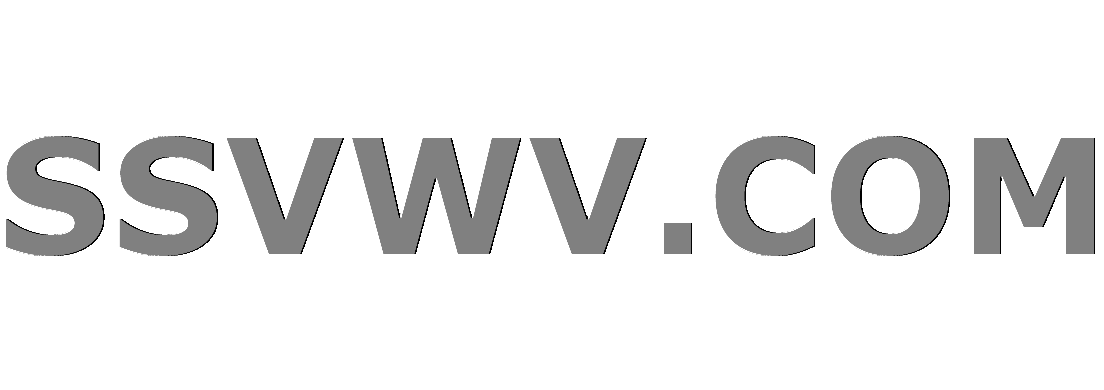
Multi tool use
I have a Spring Boot app setup as a REST api. I now also want to be able to serve simple HTML pages to the client, without the use on any template engine like Thymeleaf. I want access to the HTML pages to fall under the same security constraints setup by Spring Security with the use of WebSecurityConfigurerAdapter
, already present in my app.
What I've tried is having a Controller
:
@Controller
public class HtmlPageController
@RequestMapping(value = "/some/path/test", method = RequestMethod.GET)
public String getTestPage()
return "test.html";
and placing the test.html
file in /resources/test.html
or /webapp/WEB-INF/test.html
.
Every time I try to access the page at localhost:8080/some/path/test
a 404
is returned.
How do I make this work?
java spring spring-mvc spring-boot
add a comment |
I have a Spring Boot app setup as a REST api. I now also want to be able to serve simple HTML pages to the client, without the use on any template engine like Thymeleaf. I want access to the HTML pages to fall under the same security constraints setup by Spring Security with the use of WebSecurityConfigurerAdapter
, already present in my app.
What I've tried is having a Controller
:
@Controller
public class HtmlPageController
@RequestMapping(value = "/some/path/test", method = RequestMethod.GET)
public String getTestPage()
return "test.html";
and placing the test.html
file in /resources/test.html
or /webapp/WEB-INF/test.html
.
Every time I try to access the page at localhost:8080/some/path/test
a 404
is returned.
How do I make this work?
java spring spring-mvc spring-boot
tryreturn "test";
– user7294900
Nov 14 '18 at 9:43
If I do that, the server instead throwsCircular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 9:55
add a comment |
I have a Spring Boot app setup as a REST api. I now also want to be able to serve simple HTML pages to the client, without the use on any template engine like Thymeleaf. I want access to the HTML pages to fall under the same security constraints setup by Spring Security with the use of WebSecurityConfigurerAdapter
, already present in my app.
What I've tried is having a Controller
:
@Controller
public class HtmlPageController
@RequestMapping(value = "/some/path/test", method = RequestMethod.GET)
public String getTestPage()
return "test.html";
and placing the test.html
file in /resources/test.html
or /webapp/WEB-INF/test.html
.
Every time I try to access the page at localhost:8080/some/path/test
a 404
is returned.
How do I make this work?
java spring spring-mvc spring-boot
I have a Spring Boot app setup as a REST api. I now also want to be able to serve simple HTML pages to the client, without the use on any template engine like Thymeleaf. I want access to the HTML pages to fall under the same security constraints setup by Spring Security with the use of WebSecurityConfigurerAdapter
, already present in my app.
What I've tried is having a Controller
:
@Controller
public class HtmlPageController
@RequestMapping(value = "/some/path/test", method = RequestMethod.GET)
public String getTestPage()
return "test.html";
and placing the test.html
file in /resources/test.html
or /webapp/WEB-INF/test.html
.
Every time I try to access the page at localhost:8080/some/path/test
a 404
is returned.
How do I make this work?
java spring spring-mvc spring-boot
java spring spring-mvc spring-boot
asked Nov 14 '18 at 9:35
darksmurfdarksmurf
81611118
81611118
tryreturn "test";
– user7294900
Nov 14 '18 at 9:43
If I do that, the server instead throwsCircular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 9:55
add a comment |
tryreturn "test";
– user7294900
Nov 14 '18 at 9:43
If I do that, the server instead throwsCircular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 9:55
try
return "test";
– user7294900
Nov 14 '18 at 9:43
try
return "test";
– user7294900
Nov 14 '18 at 9:43
If I do that, the server instead throws
Circular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 9:55
If I do that, the server instead throws
Circular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 9:55
add a comment |
4 Answers
4
active
oldest
votes
There is a Spring MVC mecanism that exists to provide static resources.
In the config class, overide this method :
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry)
registry
.addResourceHandler("some/path/*.html")
.addResourceLocations("/static/");
And place your html files in the src/main/webapp/static/
folder.
If you request some/path/test.html
(note the .html
), it will return the test.html file located in static
folder.
You can obviously use a different folder or a more sofiticated directory structure.
This way you don't have to create a controller. Note that your config class should implements WebMvcConfigurer
.
add a comment |
Your html, js and css files should be under the src/main/resources/static directory. and your return statement you can try removing .html.
@RestController
public class HtmlPageController
@GetMapping("/some/path/test")
public String getTestPage()
return "test";
If I do that, the server instead throwsCircular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 10:05
replace @ Controller with @ RestController and add the thymeleaf dependency.
– Reactive_learner
Nov 14 '18 at 12:52
2
The question specifically states that I don't want to use any template engine like Thymeleaf.
– darksmurf
Nov 14 '18 at 12:58
@RestContoller will do . No need to add any thymeleaf dependency
– Reactive_learner
Nov 14 '18 at 13:52
No it won't.@RestController
is the same as annotating the method with@ResponseBody
, which then will return the string "test" in the response body.
– darksmurf
Nov 14 '18 at 13:55
add a comment |
See tutotrial example how to define html view in Spring MVC configuration
@Bean
public InternalResourceViewResolver htmlViewResolver()
InternalResourceViewResolver bean = new InternalResourceViewResolver();
bean.setPrefix("/WEB-INF/html/");
bean.setSuffix(".html");
bean.setOrder(2);
return bean;
- setOrder is set to 2 because it include also JSP support in example
Also you need to change to return without .html
suffix
return "test.html";
add a comment |
Okey so apparently Spring Boot supports this without any additional configuration or controllers.
All I had to do was to place the HTML file in the correct directory /resources/static/some/path/test.html
and it can be reached at localhost:8080/some/path/test.html
.
In my attempts to change the directory from which the file is served I was unsuccessful. It seems that providing a separate @EnableWebMvc
(needed for configuring the resource handlers) breaks the Spring Boot configuration. But I can live with using the default /static
directory.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296960%2freturn-html-page-from-spring-controller%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is a Spring MVC mecanism that exists to provide static resources.
In the config class, overide this method :
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry)
registry
.addResourceHandler("some/path/*.html")
.addResourceLocations("/static/");
And place your html files in the src/main/webapp/static/
folder.
If you request some/path/test.html
(note the .html
), it will return the test.html file located in static
folder.
You can obviously use a different folder or a more sofiticated directory structure.
This way you don't have to create a controller. Note that your config class should implements WebMvcConfigurer
.
add a comment |
There is a Spring MVC mecanism that exists to provide static resources.
In the config class, overide this method :
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry)
registry
.addResourceHandler("some/path/*.html")
.addResourceLocations("/static/");
And place your html files in the src/main/webapp/static/
folder.
If you request some/path/test.html
(note the .html
), it will return the test.html file located in static
folder.
You can obviously use a different folder or a more sofiticated directory structure.
This way you don't have to create a controller. Note that your config class should implements WebMvcConfigurer
.
add a comment |
There is a Spring MVC mecanism that exists to provide static resources.
In the config class, overide this method :
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry)
registry
.addResourceHandler("some/path/*.html")
.addResourceLocations("/static/");
And place your html files in the src/main/webapp/static/
folder.
If you request some/path/test.html
(note the .html
), it will return the test.html file located in static
folder.
You can obviously use a different folder or a more sofiticated directory structure.
This way you don't have to create a controller. Note that your config class should implements WebMvcConfigurer
.
There is a Spring MVC mecanism that exists to provide static resources.
In the config class, overide this method :
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry)
registry
.addResourceHandler("some/path/*.html")
.addResourceLocations("/static/");
And place your html files in the src/main/webapp/static/
folder.
If you request some/path/test.html
(note the .html
), it will return the test.html file located in static
folder.
You can obviously use a different folder or a more sofiticated directory structure.
This way you don't have to create a controller. Note that your config class should implements WebMvcConfigurer
.
answered Nov 14 '18 at 10:15
Romain WarnanRomain Warnan
57337
57337
add a comment |
add a comment |
Your html, js and css files should be under the src/main/resources/static directory. and your return statement you can try removing .html.
@RestController
public class HtmlPageController
@GetMapping("/some/path/test")
public String getTestPage()
return "test";
If I do that, the server instead throwsCircular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 10:05
replace @ Controller with @ RestController and add the thymeleaf dependency.
– Reactive_learner
Nov 14 '18 at 12:52
2
The question specifically states that I don't want to use any template engine like Thymeleaf.
– darksmurf
Nov 14 '18 at 12:58
@RestContoller will do . No need to add any thymeleaf dependency
– Reactive_learner
Nov 14 '18 at 13:52
No it won't.@RestController
is the same as annotating the method with@ResponseBody
, which then will return the string "test" in the response body.
– darksmurf
Nov 14 '18 at 13:55
add a comment |
Your html, js and css files should be under the src/main/resources/static directory. and your return statement you can try removing .html.
@RestController
public class HtmlPageController
@GetMapping("/some/path/test")
public String getTestPage()
return "test";
If I do that, the server instead throwsCircular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 10:05
replace @ Controller with @ RestController and add the thymeleaf dependency.
– Reactive_learner
Nov 14 '18 at 12:52
2
The question specifically states that I don't want to use any template engine like Thymeleaf.
– darksmurf
Nov 14 '18 at 12:58
@RestContoller will do . No need to add any thymeleaf dependency
– Reactive_learner
Nov 14 '18 at 13:52
No it won't.@RestController
is the same as annotating the method with@ResponseBody
, which then will return the string "test" in the response body.
– darksmurf
Nov 14 '18 at 13:55
add a comment |
Your html, js and css files should be under the src/main/resources/static directory. and your return statement you can try removing .html.
@RestController
public class HtmlPageController
@GetMapping("/some/path/test")
public String getTestPage()
return "test";
Your html, js and css files should be under the src/main/resources/static directory. and your return statement you can try removing .html.
@RestController
public class HtmlPageController
@GetMapping("/some/path/test")
public String getTestPage()
return "test";
edited Nov 14 '18 at 12:51
answered Nov 14 '18 at 9:43
Reactive_learnerReactive_learner
717
717
If I do that, the server instead throwsCircular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 10:05
replace @ Controller with @ RestController and add the thymeleaf dependency.
– Reactive_learner
Nov 14 '18 at 12:52
2
The question specifically states that I don't want to use any template engine like Thymeleaf.
– darksmurf
Nov 14 '18 at 12:58
@RestContoller will do . No need to add any thymeleaf dependency
– Reactive_learner
Nov 14 '18 at 13:52
No it won't.@RestController
is the same as annotating the method with@ResponseBody
, which then will return the string "test" in the response body.
– darksmurf
Nov 14 '18 at 13:55
add a comment |
If I do that, the server instead throwsCircular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 10:05
replace @ Controller with @ RestController and add the thymeleaf dependency.
– Reactive_learner
Nov 14 '18 at 12:52
2
The question specifically states that I don't want to use any template engine like Thymeleaf.
– darksmurf
Nov 14 '18 at 12:58
@RestContoller will do . No need to add any thymeleaf dependency
– Reactive_learner
Nov 14 '18 at 13:52
No it won't.@RestController
is the same as annotating the method with@ResponseBody
, which then will return the string "test" in the response body.
– darksmurf
Nov 14 '18 at 13:55
If I do that, the server instead throws
Circular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 10:05
If I do that, the server instead throws
Circular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 10:05
replace @ Controller with @ RestController and add the thymeleaf dependency.
– Reactive_learner
Nov 14 '18 at 12:52
replace @ Controller with @ RestController and add the thymeleaf dependency.
– Reactive_learner
Nov 14 '18 at 12:52
2
2
The question specifically states that I don't want to use any template engine like Thymeleaf.
– darksmurf
Nov 14 '18 at 12:58
The question specifically states that I don't want to use any template engine like Thymeleaf.
– darksmurf
Nov 14 '18 at 12:58
@RestContoller will do . No need to add any thymeleaf dependency
– Reactive_learner
Nov 14 '18 at 13:52
@RestContoller will do . No need to add any thymeleaf dependency
– Reactive_learner
Nov 14 '18 at 13:52
No it won't.
@RestController
is the same as annotating the method with @ResponseBody
, which then will return the string "test" in the response body.– darksmurf
Nov 14 '18 at 13:55
No it won't.
@RestController
is the same as annotating the method with @ResponseBody
, which then will return the string "test" in the response body.– darksmurf
Nov 14 '18 at 13:55
add a comment |
See tutotrial example how to define html view in Spring MVC configuration
@Bean
public InternalResourceViewResolver htmlViewResolver()
InternalResourceViewResolver bean = new InternalResourceViewResolver();
bean.setPrefix("/WEB-INF/html/");
bean.setSuffix(".html");
bean.setOrder(2);
return bean;
- setOrder is set to 2 because it include also JSP support in example
Also you need to change to return without .html
suffix
return "test.html";
add a comment |
See tutotrial example how to define html view in Spring MVC configuration
@Bean
public InternalResourceViewResolver htmlViewResolver()
InternalResourceViewResolver bean = new InternalResourceViewResolver();
bean.setPrefix("/WEB-INF/html/");
bean.setSuffix(".html");
bean.setOrder(2);
return bean;
- setOrder is set to 2 because it include also JSP support in example
Also you need to change to return without .html
suffix
return "test.html";
add a comment |
See tutotrial example how to define html view in Spring MVC configuration
@Bean
public InternalResourceViewResolver htmlViewResolver()
InternalResourceViewResolver bean = new InternalResourceViewResolver();
bean.setPrefix("/WEB-INF/html/");
bean.setSuffix(".html");
bean.setOrder(2);
return bean;
- setOrder is set to 2 because it include also JSP support in example
Also you need to change to return without .html
suffix
return "test.html";
See tutotrial example how to define html view in Spring MVC configuration
@Bean
public InternalResourceViewResolver htmlViewResolver()
InternalResourceViewResolver bean = new InternalResourceViewResolver();
bean.setPrefix("/WEB-INF/html/");
bean.setSuffix(".html");
bean.setOrder(2);
return bean;
- setOrder is set to 2 because it include also JSP support in example
Also you need to change to return without .html
suffix
return "test.html";
answered Nov 14 '18 at 10:10


user7294900user7294900
22k113258
22k113258
add a comment |
add a comment |
Okey so apparently Spring Boot supports this without any additional configuration or controllers.
All I had to do was to place the HTML file in the correct directory /resources/static/some/path/test.html
and it can be reached at localhost:8080/some/path/test.html
.
In my attempts to change the directory from which the file is served I was unsuccessful. It seems that providing a separate @EnableWebMvc
(needed for configuring the resource handlers) breaks the Spring Boot configuration. But I can live with using the default /static
directory.
add a comment |
Okey so apparently Spring Boot supports this without any additional configuration or controllers.
All I had to do was to place the HTML file in the correct directory /resources/static/some/path/test.html
and it can be reached at localhost:8080/some/path/test.html
.
In my attempts to change the directory from which the file is served I was unsuccessful. It seems that providing a separate @EnableWebMvc
(needed for configuring the resource handlers) breaks the Spring Boot configuration. But I can live with using the default /static
directory.
add a comment |
Okey so apparently Spring Boot supports this without any additional configuration or controllers.
All I had to do was to place the HTML file in the correct directory /resources/static/some/path/test.html
and it can be reached at localhost:8080/some/path/test.html
.
In my attempts to change the directory from which the file is served I was unsuccessful. It seems that providing a separate @EnableWebMvc
(needed for configuring the resource handlers) breaks the Spring Boot configuration. But I can live with using the default /static
directory.
Okey so apparently Spring Boot supports this without any additional configuration or controllers.
All I had to do was to place the HTML file in the correct directory /resources/static/some/path/test.html
and it can be reached at localhost:8080/some/path/test.html
.
In my attempts to change the directory from which the file is served I was unsuccessful. It seems that providing a separate @EnableWebMvc
(needed for configuring the resource handlers) breaks the Spring Boot configuration. But I can live with using the default /static
directory.
answered Nov 14 '18 at 12:07
darksmurfdarksmurf
81611118
81611118
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296960%2freturn-html-page-from-spring-controller%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uAcvMpM 43DWKkYeJkg95Ot chZB7,7BribeYQJqfg8JQPALsQEmq5 Tlm zbYOwEjNkFtA9 aC,jN0Y,ZnOPR y8,a2QK
try
return "test";
– user7294900
Nov 14 '18 at 9:43
If I do that, the server instead throws
Circular view path [test]: would dispatch back to the current handler URL [/some/path/test] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
– darksmurf
Nov 14 '18 at 9:55