Java Tip Calculator for newbie
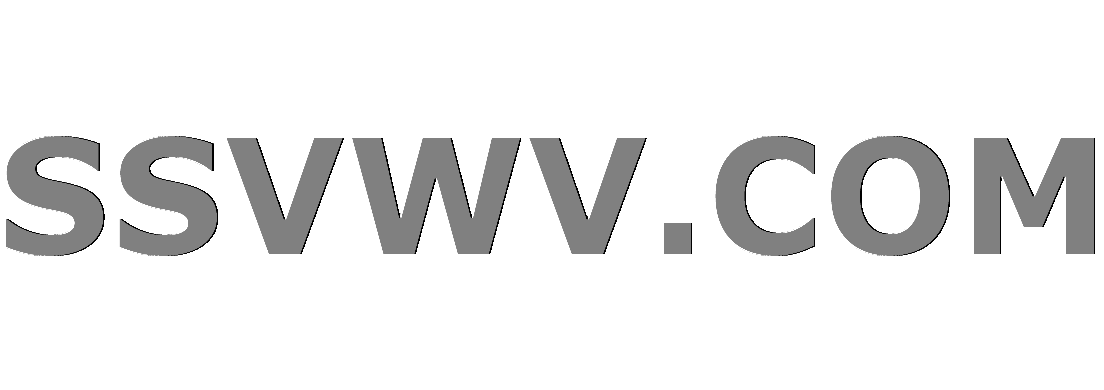
Multi tool use
I hope I'm posting in the right place.
I'm pretty new to Java (meaning this is only my third program besides 'hello world').
I have a tip calculator I'm working on for an assignment. I'm not getting an 'error' as such,
but the method for splitting the bill always seems to think each customer pays 'infinity'.
I have my program set up in two classes: tipCalc1 and tipCalc2 (no points for originality of course).
The program appears to run without issue besides the 'infinity' issue.
Here's what I have so far. Any assistance appreciated, thanks.
***TipCalc1 Class:***
import java.util.Scanner;
public class Tipcalc1
public static void main(String args)
System.out.println("Welcome to Tip Calculator! ");
TipCalc2 Calculator = new TipCalc2();
System.out.println("Please enter the bill amount: ");
TipCalc2.calBill();
System.out.println("What percentage would you like to tip?: ");
Calculator.percTip();
***And the tipCalc2 class which does the dirty work:***
import java.util.Scanner;
public class TipCalc2
static double bill;
double tip;
double total;
double split;
double splitPrompt;
double Y;
double N;
double billPerPerson;
static Scanner scan = new Scanner(System.in);
public static void calBill()
bill = scan.nextDouble();
public void percTip()
tip = scan.nextDouble();
if(tip<1)
total = bill * tip;
else total = bill * (tip/100);
System.out.println("Your total is: " + total);
Split();
public void Split()
System.out.println("Would you like to split the bill? ");
System.out.println("Enter 1 for YES or 0 for NO: ");
splitPrompt = scan.nextDouble();
if(splitPrompt == 0)
System.out.println("Your total is: " + total);
System.out.println("Thankyou. Goodbye.");
System.out.println("End Program");
if(splitPrompt == 1)
System.out.println("How many ways would you like to split the bill? ");
splitPrompt = scan.nextDouble();
billPerPerson = total / split;
System.out.println("Each person pays: " + billPerPerson);
System.out.println("Thankyou. Goodbye.");
System.out.println("End Program.");
else System.out.println("Invalid Entry");
java
add a comment |
I hope I'm posting in the right place.
I'm pretty new to Java (meaning this is only my third program besides 'hello world').
I have a tip calculator I'm working on for an assignment. I'm not getting an 'error' as such,
but the method for splitting the bill always seems to think each customer pays 'infinity'.
I have my program set up in two classes: tipCalc1 and tipCalc2 (no points for originality of course).
The program appears to run without issue besides the 'infinity' issue.
Here's what I have so far. Any assistance appreciated, thanks.
***TipCalc1 Class:***
import java.util.Scanner;
public class Tipcalc1
public static void main(String args)
System.out.println("Welcome to Tip Calculator! ");
TipCalc2 Calculator = new TipCalc2();
System.out.println("Please enter the bill amount: ");
TipCalc2.calBill();
System.out.println("What percentage would you like to tip?: ");
Calculator.percTip();
***And the tipCalc2 class which does the dirty work:***
import java.util.Scanner;
public class TipCalc2
static double bill;
double tip;
double total;
double split;
double splitPrompt;
double Y;
double N;
double billPerPerson;
static Scanner scan = new Scanner(System.in);
public static void calBill()
bill = scan.nextDouble();
public void percTip()
tip = scan.nextDouble();
if(tip<1)
total = bill * tip;
else total = bill * (tip/100);
System.out.println("Your total is: " + total);
Split();
public void Split()
System.out.println("Would you like to split the bill? ");
System.out.println("Enter 1 for YES or 0 for NO: ");
splitPrompt = scan.nextDouble();
if(splitPrompt == 0)
System.out.println("Your total is: " + total);
System.out.println("Thankyou. Goodbye.");
System.out.println("End Program");
if(splitPrompt == 1)
System.out.println("How many ways would you like to split the bill? ");
splitPrompt = scan.nextDouble();
billPerPerson = total / split;
System.out.println("Each person pays: " + billPerPerson);
System.out.println("Thankyou. Goodbye.");
System.out.println("End Program.");
else System.out.println("Invalid Entry");
java
Could you be more specific about your problem? What are expected outputs? What are you getting instead?
– BitNinja
May 18 '14 at 1:37
4
While you are still a beginner, now is the best time to get out of the habit of usingdouble
to store an amount of money. If you usedouble
in this way, you will introduce errors as soon as you start doing arithmetic. I strongly recommend either usingBigDecimal
to store your money, or usingint
to store a number of cents.
– Dawood ibn Kareem
May 18 '14 at 1:41
add a comment |
I hope I'm posting in the right place.
I'm pretty new to Java (meaning this is only my third program besides 'hello world').
I have a tip calculator I'm working on for an assignment. I'm not getting an 'error' as such,
but the method for splitting the bill always seems to think each customer pays 'infinity'.
I have my program set up in two classes: tipCalc1 and tipCalc2 (no points for originality of course).
The program appears to run without issue besides the 'infinity' issue.
Here's what I have so far. Any assistance appreciated, thanks.
***TipCalc1 Class:***
import java.util.Scanner;
public class Tipcalc1
public static void main(String args)
System.out.println("Welcome to Tip Calculator! ");
TipCalc2 Calculator = new TipCalc2();
System.out.println("Please enter the bill amount: ");
TipCalc2.calBill();
System.out.println("What percentage would you like to tip?: ");
Calculator.percTip();
***And the tipCalc2 class which does the dirty work:***
import java.util.Scanner;
public class TipCalc2
static double bill;
double tip;
double total;
double split;
double splitPrompt;
double Y;
double N;
double billPerPerson;
static Scanner scan = new Scanner(System.in);
public static void calBill()
bill = scan.nextDouble();
public void percTip()
tip = scan.nextDouble();
if(tip<1)
total = bill * tip;
else total = bill * (tip/100);
System.out.println("Your total is: " + total);
Split();
public void Split()
System.out.println("Would you like to split the bill? ");
System.out.println("Enter 1 for YES or 0 for NO: ");
splitPrompt = scan.nextDouble();
if(splitPrompt == 0)
System.out.println("Your total is: " + total);
System.out.println("Thankyou. Goodbye.");
System.out.println("End Program");
if(splitPrompt == 1)
System.out.println("How many ways would you like to split the bill? ");
splitPrompt = scan.nextDouble();
billPerPerson = total / split;
System.out.println("Each person pays: " + billPerPerson);
System.out.println("Thankyou. Goodbye.");
System.out.println("End Program.");
else System.out.println("Invalid Entry");
java
I hope I'm posting in the right place.
I'm pretty new to Java (meaning this is only my third program besides 'hello world').
I have a tip calculator I'm working on for an assignment. I'm not getting an 'error' as such,
but the method for splitting the bill always seems to think each customer pays 'infinity'.
I have my program set up in two classes: tipCalc1 and tipCalc2 (no points for originality of course).
The program appears to run without issue besides the 'infinity' issue.
Here's what I have so far. Any assistance appreciated, thanks.
***TipCalc1 Class:***
import java.util.Scanner;
public class Tipcalc1
public static void main(String args)
System.out.println("Welcome to Tip Calculator! ");
TipCalc2 Calculator = new TipCalc2();
System.out.println("Please enter the bill amount: ");
TipCalc2.calBill();
System.out.println("What percentage would you like to tip?: ");
Calculator.percTip();
***And the tipCalc2 class which does the dirty work:***
import java.util.Scanner;
public class TipCalc2
static double bill;
double tip;
double total;
double split;
double splitPrompt;
double Y;
double N;
double billPerPerson;
static Scanner scan = new Scanner(System.in);
public static void calBill()
bill = scan.nextDouble();
public void percTip()
tip = scan.nextDouble();
if(tip<1)
total = bill * tip;
else total = bill * (tip/100);
System.out.println("Your total is: " + total);
Split();
public void Split()
System.out.println("Would you like to split the bill? ");
System.out.println("Enter 1 for YES or 0 for NO: ");
splitPrompt = scan.nextDouble();
if(splitPrompt == 0)
System.out.println("Your total is: " + total);
System.out.println("Thankyou. Goodbye.");
System.out.println("End Program");
if(splitPrompt == 1)
System.out.println("How many ways would you like to split the bill? ");
splitPrompt = scan.nextDouble();
billPerPerson = total / split;
System.out.println("Each person pays: " + billPerPerson);
System.out.println("Thankyou. Goodbye.");
System.out.println("End Program.");
else System.out.println("Invalid Entry");
java
java
asked May 18 '14 at 1:35
user3648784user3648784
111
111
Could you be more specific about your problem? What are expected outputs? What are you getting instead?
– BitNinja
May 18 '14 at 1:37
4
While you are still a beginner, now is the best time to get out of the habit of usingdouble
to store an amount of money. If you usedouble
in this way, you will introduce errors as soon as you start doing arithmetic. I strongly recommend either usingBigDecimal
to store your money, or usingint
to store a number of cents.
– Dawood ibn Kareem
May 18 '14 at 1:41
add a comment |
Could you be more specific about your problem? What are expected outputs? What are you getting instead?
– BitNinja
May 18 '14 at 1:37
4
While you are still a beginner, now is the best time to get out of the habit of usingdouble
to store an amount of money. If you usedouble
in this way, you will introduce errors as soon as you start doing arithmetic. I strongly recommend either usingBigDecimal
to store your money, or usingint
to store a number of cents.
– Dawood ibn Kareem
May 18 '14 at 1:41
Could you be more specific about your problem? What are expected outputs? What are you getting instead?
– BitNinja
May 18 '14 at 1:37
Could you be more specific about your problem? What are expected outputs? What are you getting instead?
– BitNinja
May 18 '14 at 1:37
4
4
While you are still a beginner, now is the best time to get out of the habit of using
double
to store an amount of money. If you use double
in this way, you will introduce errors as soon as you start doing arithmetic. I strongly recommend either using BigDecimal
to store your money, or using int
to store a number of cents.– Dawood ibn Kareem
May 18 '14 at 1:41
While you are still a beginner, now is the best time to get out of the habit of using
double
to store an amount of money. If you use double
in this way, you will introduce errors as soon as you start doing arithmetic. I strongly recommend either using BigDecimal
to store your money, or using int
to store a number of cents.– Dawood ibn Kareem
May 18 '14 at 1:41
add a comment |
3 Answers
3
active
oldest
votes
The default value for split
(because you have not initialized it with another value) is 0.0
, therefore, when you do
billPerPerson = total / split;
you divide by 0.0
, so you will get Infinity
.
Notes:
- Since your variable
splitPrompt
is double and computers doesn't store real values with a 100% accuracy, you shouldn't compare it with0.0
. Since this variable will store0
or1
for input, you can declare it asint
, which will be accurate. - Try to follow Java naming conventions. Use
mixedCase
for methods/variables and useCamelCase
for classes/interfaces. In the method
split()
, you should use anif-else if-else
structure:if(splitPrompt == 0)
...
else if(splitPrompt == 1)
...
else
...
add a comment |
Silly mistake.
Change
System.out.println("How many ways would you like to split the bill?
splitPrompt = scan.nextDouble();
to
System.out.println("How many ways would you like to split the bill?
split = scan.nextDouble();
since you never change split which, like all double variables, is initialized to 0.0.
Also, you should use ints where appropriate as not all of the numbers should be doubles. Or even better, use 'y' and 'n' chars.
Never mind, saw the second one...
– awksp
May 18 '14 at 1:40
@user3580294: I needed to make it more specific...
– Hovercraft Full Of Eels
May 18 '14 at 1:40
Yep, my apologies for jumping to conclusions a bit early
– awksp
May 18 '14 at 1:41
add a comment |
Class TipCalc2
//Total = **bill** * (gets percentage in decimal 15 = 0.15) + **bill**
Line 18 needs to be:
total = bill * (tip / 100) + bill;
Line 36/37 needs to be:
split = splitPrompt = scan.nextInt();
billPerPerson = total / split;
//You're dividing billPerPerson = total by ZERO (split);
Line 36/37 original:
billPerPerson = total / split;
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f23717749%2fjava-tip-calculator-for-newbie%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
The default value for split
(because you have not initialized it with another value) is 0.0
, therefore, when you do
billPerPerson = total / split;
you divide by 0.0
, so you will get Infinity
.
Notes:
- Since your variable
splitPrompt
is double and computers doesn't store real values with a 100% accuracy, you shouldn't compare it with0.0
. Since this variable will store0
or1
for input, you can declare it asint
, which will be accurate. - Try to follow Java naming conventions. Use
mixedCase
for methods/variables and useCamelCase
for classes/interfaces. In the method
split()
, you should use anif-else if-else
structure:if(splitPrompt == 0)
...
else if(splitPrompt == 1)
...
else
...
add a comment |
The default value for split
(because you have not initialized it with another value) is 0.0
, therefore, when you do
billPerPerson = total / split;
you divide by 0.0
, so you will get Infinity
.
Notes:
- Since your variable
splitPrompt
is double and computers doesn't store real values with a 100% accuracy, you shouldn't compare it with0.0
. Since this variable will store0
or1
for input, you can declare it asint
, which will be accurate. - Try to follow Java naming conventions. Use
mixedCase
for methods/variables and useCamelCase
for classes/interfaces. In the method
split()
, you should use anif-else if-else
structure:if(splitPrompt == 0)
...
else if(splitPrompt == 1)
...
else
...
add a comment |
The default value for split
(because you have not initialized it with another value) is 0.0
, therefore, when you do
billPerPerson = total / split;
you divide by 0.0
, so you will get Infinity
.
Notes:
- Since your variable
splitPrompt
is double and computers doesn't store real values with a 100% accuracy, you shouldn't compare it with0.0
. Since this variable will store0
or1
for input, you can declare it asint
, which will be accurate. - Try to follow Java naming conventions. Use
mixedCase
for methods/variables and useCamelCase
for classes/interfaces. In the method
split()
, you should use anif-else if-else
structure:if(splitPrompt == 0)
...
else if(splitPrompt == 1)
...
else
...
The default value for split
(because you have not initialized it with another value) is 0.0
, therefore, when you do
billPerPerson = total / split;
you divide by 0.0
, so you will get Infinity
.
Notes:
- Since your variable
splitPrompt
is double and computers doesn't store real values with a 100% accuracy, you shouldn't compare it with0.0
. Since this variable will store0
or1
for input, you can declare it asint
, which will be accurate. - Try to follow Java naming conventions. Use
mixedCase
for methods/variables and useCamelCase
for classes/interfaces. In the method
split()
, you should use anif-else if-else
structure:if(splitPrompt == 0)
...
else if(splitPrompt == 1)
...
else
...
answered May 18 '14 at 1:38


ChristianChristian
27.5k43361
27.5k43361
add a comment |
add a comment |
Silly mistake.
Change
System.out.println("How many ways would you like to split the bill?
splitPrompt = scan.nextDouble();
to
System.out.println("How many ways would you like to split the bill?
split = scan.nextDouble();
since you never change split which, like all double variables, is initialized to 0.0.
Also, you should use ints where appropriate as not all of the numbers should be doubles. Or even better, use 'y' and 'n' chars.
Never mind, saw the second one...
– awksp
May 18 '14 at 1:40
@user3580294: I needed to make it more specific...
– Hovercraft Full Of Eels
May 18 '14 at 1:40
Yep, my apologies for jumping to conclusions a bit early
– awksp
May 18 '14 at 1:41
add a comment |
Silly mistake.
Change
System.out.println("How many ways would you like to split the bill?
splitPrompt = scan.nextDouble();
to
System.out.println("How many ways would you like to split the bill?
split = scan.nextDouble();
since you never change split which, like all double variables, is initialized to 0.0.
Also, you should use ints where appropriate as not all of the numbers should be doubles. Or even better, use 'y' and 'n' chars.
Never mind, saw the second one...
– awksp
May 18 '14 at 1:40
@user3580294: I needed to make it more specific...
– Hovercraft Full Of Eels
May 18 '14 at 1:40
Yep, my apologies for jumping to conclusions a bit early
– awksp
May 18 '14 at 1:41
add a comment |
Silly mistake.
Change
System.out.println("How many ways would you like to split the bill?
splitPrompt = scan.nextDouble();
to
System.out.println("How many ways would you like to split the bill?
split = scan.nextDouble();
since you never change split which, like all double variables, is initialized to 0.0.
Also, you should use ints where appropriate as not all of the numbers should be doubles. Or even better, use 'y' and 'n' chars.
Silly mistake.
Change
System.out.println("How many ways would you like to split the bill?
splitPrompt = scan.nextDouble();
to
System.out.println("How many ways would you like to split the bill?
split = scan.nextDouble();
since you never change split which, like all double variables, is initialized to 0.0.
Also, you should use ints where appropriate as not all of the numbers should be doubles. Or even better, use 'y' and 'n' chars.
answered May 18 '14 at 1:38
Hovercraft Full Of EelsHovercraft Full Of Eels
262k20213319
262k20213319
Never mind, saw the second one...
– awksp
May 18 '14 at 1:40
@user3580294: I needed to make it more specific...
– Hovercraft Full Of Eels
May 18 '14 at 1:40
Yep, my apologies for jumping to conclusions a bit early
– awksp
May 18 '14 at 1:41
add a comment |
Never mind, saw the second one...
– awksp
May 18 '14 at 1:40
@user3580294: I needed to make it more specific...
– Hovercraft Full Of Eels
May 18 '14 at 1:40
Yep, my apologies for jumping to conclusions a bit early
– awksp
May 18 '14 at 1:41
Never mind, saw the second one...
– awksp
May 18 '14 at 1:40
Never mind, saw the second one...
– awksp
May 18 '14 at 1:40
@user3580294: I needed to make it more specific...
– Hovercraft Full Of Eels
May 18 '14 at 1:40
@user3580294: I needed to make it more specific...
– Hovercraft Full Of Eels
May 18 '14 at 1:40
Yep, my apologies for jumping to conclusions a bit early
– awksp
May 18 '14 at 1:41
Yep, my apologies for jumping to conclusions a bit early
– awksp
May 18 '14 at 1:41
add a comment |
Class TipCalc2
//Total = **bill** * (gets percentage in decimal 15 = 0.15) + **bill**
Line 18 needs to be:
total = bill * (tip / 100) + bill;
Line 36/37 needs to be:
split = splitPrompt = scan.nextInt();
billPerPerson = total / split;
//You're dividing billPerPerson = total by ZERO (split);
Line 36/37 original:
billPerPerson = total / split;
add a comment |
Class TipCalc2
//Total = **bill** * (gets percentage in decimal 15 = 0.15) + **bill**
Line 18 needs to be:
total = bill * (tip / 100) + bill;
Line 36/37 needs to be:
split = splitPrompt = scan.nextInt();
billPerPerson = total / split;
//You're dividing billPerPerson = total by ZERO (split);
Line 36/37 original:
billPerPerson = total / split;
add a comment |
Class TipCalc2
//Total = **bill** * (gets percentage in decimal 15 = 0.15) + **bill**
Line 18 needs to be:
total = bill * (tip / 100) + bill;
Line 36/37 needs to be:
split = splitPrompt = scan.nextInt();
billPerPerson = total / split;
//You're dividing billPerPerson = total by ZERO (split);
Line 36/37 original:
billPerPerson = total / split;
Class TipCalc2
//Total = **bill** * (gets percentage in decimal 15 = 0.15) + **bill**
Line 18 needs to be:
total = bill * (tip / 100) + bill;
Line 36/37 needs to be:
split = splitPrompt = scan.nextInt();
billPerPerson = total / split;
//You're dividing billPerPerson = total by ZERO (split);
Line 36/37 original:
billPerPerson = total / split;
edited Nov 16 '18 at 1:02


Stephen Rauch
30k153758
30k153758
answered Nov 16 '18 at 0:41
ReyRey
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f23717749%2fjava-tip-calculator-for-newbie%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
z6vg,GLt3s1CWS24jZ,NT
Could you be more specific about your problem? What are expected outputs? What are you getting instead?
– BitNinja
May 18 '14 at 1:37
4
While you are still a beginner, now is the best time to get out of the habit of using
double
to store an amount of money. If you usedouble
in this way, you will introduce errors as soon as you start doing arithmetic. I strongly recommend either usingBigDecimal
to store your money, or usingint
to store a number of cents.– Dawood ibn Kareem
May 18 '14 at 1:41