Set Map bounds based on multiple marker Lng,Lat
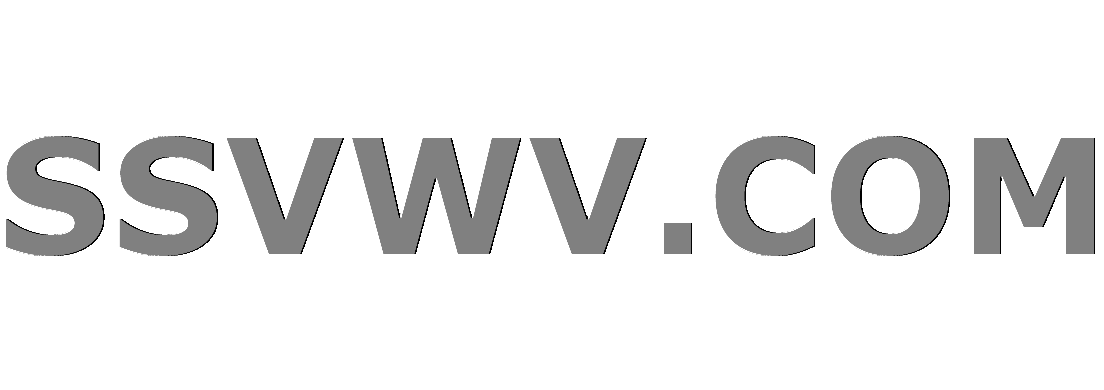
Multi tool use
Am using vue and have installed the vue-mapbox component located here: https://soal.github.io/vue-mapbox/#/quickstart
I have updated the js and css to the latest versions also that gets added to the index.html:
<!-- Mapbox GL CSS -->
<link href="https://api.tiles.mapbox.com/mapbox-gl-js/v0.51.0/mapbox-gl.css" rel="stylesheet" />
<!-- Mapbox GL JS -->
<script src="https://api.tiles.mapbox.com/mapbox-gl-js/v0.51.0/mapbox-gl.js"></script>
I am trying to utilize this component to set the default view of the map bounds using either center
or bounds
or fitBounds
to a list of Lng,Lat coordinates. So, basically, how to plug in lng,lat coordinates and have the map default to centering these coordinates inside of the container?
Here's a Component I created, called Map
in vue to output the mapbox using the component vue-mapbox
listed above:
<template>
<b-row id="map" class="d-flex justify-content-center align-items-center my-2">
<b-col cols="24" id="map-holder" v-bind:class="getMapType">
<mgl-map
id="map-obj"
:accessToken="accessToken"
:mapStyle.sync="mapStyle"
:zoom="zoom"
:center="center"
container="map-holder"
:interactive="interactive"
@load="loadMap"
ref="mapbox" />
</b-col>
</b-row>
</template>
<script>
import MglMap from 'vue-mapbox'
export default
components:
MglMap
,
data ()
return
accessToken: 'pk.eyJ1Ijoic29sb2dob3N0IiwiYSI6ImNqb2htbmpwNjA0aG8zcWxjc3IzOGI1ejcifQ.nGL4NwbJYffJpjOiBL-Zpg',
mapStyle: 'mapbox://styles/mapbox/streets-v9', // options: basic-v9, streets-v9, bright-v9, light-v9, dark-v9, satellite-v9
zoom: 9,
map: , // Holds the Map...
fitBounds: [[-79, 43], [-73, 45]]
,
props:
interactive:
default: true
,
resizeMap:
default: false
,
mapType:
default: ''
,
center:
type: Array,
default: function () return [4.899, 52.372]
,
computed:
getMapType ()
let classes = 'inner-map'
if (this.mapType !== '')
classes += ' map-' + this.mapType
return classes
,
watch:
resizeMap (val)
if (val)
this.$nextTick(() => this.$refs.mapbox.resize())
,
fitBounds (val)
if (this.fitBounds.length)
this.MoveMapCoords()
,
methods:
loadMap ()
if (this.map === null)
this.map = event.map // store the map object in here...
,
MoveMapCoords ()
this.$refs.mapbox.fitBounds(this.fitBounds)
</script>
<style lang="scss" scoped>
@import '../../styles/custom.scss';
#map
#map-obj
text-align: justify;
width: 100%;
#map-holder
&.map-modal
#map-obj
height: 340px;
&.map-large
#map-obj
height: 500px;
.mapboxgl-map
border: 2px solid lightgray;
</style>
So, I'm trying to use fitBounds
method here to get the map to initialize centered over 2 Lng,Lat coordinates here: [[-79, 43], [-73, 45]]
How to do this exactly? Ok, I think I might have an error in my code a bit, so I think the fitBounds
should look something like this instead:
fitBounds: () =>
return bounds: [[-79, 43], [-73, 45]]
In any case, having the most difficult time setting the initial location of the mapbox to be centered over 2 or more coordinates. Anyone do this successfully yet?
Ok, so I wound up creating a filter to add space to the bbox like so:
Vue.filter('addSpaceToBBoxBounds', function (value)
if (value && value.length)
var boxArea =
for (var b = 0, len = value.length; b < len; b++)
boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2)
return boxArea
return value
)
This looks to be good enough for now. Than just use it like so:
let line = turf.lineString(this.markers)
mapOptions['bounds'] = this.$options.filters.addSpaceToBBoxBounds(turf.bbox(line))
return mapOptions
javascript vuejs2 mapbox-gl-js vue-mapbox
add a comment |
Am using vue and have installed the vue-mapbox component located here: https://soal.github.io/vue-mapbox/#/quickstart
I have updated the js and css to the latest versions also that gets added to the index.html:
<!-- Mapbox GL CSS -->
<link href="https://api.tiles.mapbox.com/mapbox-gl-js/v0.51.0/mapbox-gl.css" rel="stylesheet" />
<!-- Mapbox GL JS -->
<script src="https://api.tiles.mapbox.com/mapbox-gl-js/v0.51.0/mapbox-gl.js"></script>
I am trying to utilize this component to set the default view of the map bounds using either center
or bounds
or fitBounds
to a list of Lng,Lat coordinates. So, basically, how to plug in lng,lat coordinates and have the map default to centering these coordinates inside of the container?
Here's a Component I created, called Map
in vue to output the mapbox using the component vue-mapbox
listed above:
<template>
<b-row id="map" class="d-flex justify-content-center align-items-center my-2">
<b-col cols="24" id="map-holder" v-bind:class="getMapType">
<mgl-map
id="map-obj"
:accessToken="accessToken"
:mapStyle.sync="mapStyle"
:zoom="zoom"
:center="center"
container="map-holder"
:interactive="interactive"
@load="loadMap"
ref="mapbox" />
</b-col>
</b-row>
</template>
<script>
import MglMap from 'vue-mapbox'
export default
components:
MglMap
,
data ()
return
accessToken: 'pk.eyJ1Ijoic29sb2dob3N0IiwiYSI6ImNqb2htbmpwNjA0aG8zcWxjc3IzOGI1ejcifQ.nGL4NwbJYffJpjOiBL-Zpg',
mapStyle: 'mapbox://styles/mapbox/streets-v9', // options: basic-v9, streets-v9, bright-v9, light-v9, dark-v9, satellite-v9
zoom: 9,
map: , // Holds the Map...
fitBounds: [[-79, 43], [-73, 45]]
,
props:
interactive:
default: true
,
resizeMap:
default: false
,
mapType:
default: ''
,
center:
type: Array,
default: function () return [4.899, 52.372]
,
computed:
getMapType ()
let classes = 'inner-map'
if (this.mapType !== '')
classes += ' map-' + this.mapType
return classes
,
watch:
resizeMap (val)
if (val)
this.$nextTick(() => this.$refs.mapbox.resize())
,
fitBounds (val)
if (this.fitBounds.length)
this.MoveMapCoords()
,
methods:
loadMap ()
if (this.map === null)
this.map = event.map // store the map object in here...
,
MoveMapCoords ()
this.$refs.mapbox.fitBounds(this.fitBounds)
</script>
<style lang="scss" scoped>
@import '../../styles/custom.scss';
#map
#map-obj
text-align: justify;
width: 100%;
#map-holder
&.map-modal
#map-obj
height: 340px;
&.map-large
#map-obj
height: 500px;
.mapboxgl-map
border: 2px solid lightgray;
</style>
So, I'm trying to use fitBounds
method here to get the map to initialize centered over 2 Lng,Lat coordinates here: [[-79, 43], [-73, 45]]
How to do this exactly? Ok, I think I might have an error in my code a bit, so I think the fitBounds
should look something like this instead:
fitBounds: () =>
return bounds: [[-79, 43], [-73, 45]]
In any case, having the most difficult time setting the initial location of the mapbox to be centered over 2 or more coordinates. Anyone do this successfully yet?
Ok, so I wound up creating a filter to add space to the bbox like so:
Vue.filter('addSpaceToBBoxBounds', function (value)
if (value && value.length)
var boxArea =
for (var b = 0, len = value.length; b < len; b++)
boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2)
return boxArea
return value
)
This looks to be good enough for now. Than just use it like so:
let line = turf.lineString(this.markers)
mapOptions['bounds'] = this.$options.filters.addSpaceToBBoxBounds(turf.bbox(line))
return mapOptions
javascript vuejs2 mapbox-gl-js vue-mapbox
add a comment |
Am using vue and have installed the vue-mapbox component located here: https://soal.github.io/vue-mapbox/#/quickstart
I have updated the js and css to the latest versions also that gets added to the index.html:
<!-- Mapbox GL CSS -->
<link href="https://api.tiles.mapbox.com/mapbox-gl-js/v0.51.0/mapbox-gl.css" rel="stylesheet" />
<!-- Mapbox GL JS -->
<script src="https://api.tiles.mapbox.com/mapbox-gl-js/v0.51.0/mapbox-gl.js"></script>
I am trying to utilize this component to set the default view of the map bounds using either center
or bounds
or fitBounds
to a list of Lng,Lat coordinates. So, basically, how to plug in lng,lat coordinates and have the map default to centering these coordinates inside of the container?
Here's a Component I created, called Map
in vue to output the mapbox using the component vue-mapbox
listed above:
<template>
<b-row id="map" class="d-flex justify-content-center align-items-center my-2">
<b-col cols="24" id="map-holder" v-bind:class="getMapType">
<mgl-map
id="map-obj"
:accessToken="accessToken"
:mapStyle.sync="mapStyle"
:zoom="zoom"
:center="center"
container="map-holder"
:interactive="interactive"
@load="loadMap"
ref="mapbox" />
</b-col>
</b-row>
</template>
<script>
import MglMap from 'vue-mapbox'
export default
components:
MglMap
,
data ()
return
accessToken: 'pk.eyJ1Ijoic29sb2dob3N0IiwiYSI6ImNqb2htbmpwNjA0aG8zcWxjc3IzOGI1ejcifQ.nGL4NwbJYffJpjOiBL-Zpg',
mapStyle: 'mapbox://styles/mapbox/streets-v9', // options: basic-v9, streets-v9, bright-v9, light-v9, dark-v9, satellite-v9
zoom: 9,
map: , // Holds the Map...
fitBounds: [[-79, 43], [-73, 45]]
,
props:
interactive:
default: true
,
resizeMap:
default: false
,
mapType:
default: ''
,
center:
type: Array,
default: function () return [4.899, 52.372]
,
computed:
getMapType ()
let classes = 'inner-map'
if (this.mapType !== '')
classes += ' map-' + this.mapType
return classes
,
watch:
resizeMap (val)
if (val)
this.$nextTick(() => this.$refs.mapbox.resize())
,
fitBounds (val)
if (this.fitBounds.length)
this.MoveMapCoords()
,
methods:
loadMap ()
if (this.map === null)
this.map = event.map // store the map object in here...
,
MoveMapCoords ()
this.$refs.mapbox.fitBounds(this.fitBounds)
</script>
<style lang="scss" scoped>
@import '../../styles/custom.scss';
#map
#map-obj
text-align: justify;
width: 100%;
#map-holder
&.map-modal
#map-obj
height: 340px;
&.map-large
#map-obj
height: 500px;
.mapboxgl-map
border: 2px solid lightgray;
</style>
So, I'm trying to use fitBounds
method here to get the map to initialize centered over 2 Lng,Lat coordinates here: [[-79, 43], [-73, 45]]
How to do this exactly? Ok, I think I might have an error in my code a bit, so I think the fitBounds
should look something like this instead:
fitBounds: () =>
return bounds: [[-79, 43], [-73, 45]]
In any case, having the most difficult time setting the initial location of the mapbox to be centered over 2 or more coordinates. Anyone do this successfully yet?
Ok, so I wound up creating a filter to add space to the bbox like so:
Vue.filter('addSpaceToBBoxBounds', function (value)
if (value && value.length)
var boxArea =
for (var b = 0, len = value.length; b < len; b++)
boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2)
return boxArea
return value
)
This looks to be good enough for now. Than just use it like so:
let line = turf.lineString(this.markers)
mapOptions['bounds'] = this.$options.filters.addSpaceToBBoxBounds(turf.bbox(line))
return mapOptions
javascript vuejs2 mapbox-gl-js vue-mapbox
Am using vue and have installed the vue-mapbox component located here: https://soal.github.io/vue-mapbox/#/quickstart
I have updated the js and css to the latest versions also that gets added to the index.html:
<!-- Mapbox GL CSS -->
<link href="https://api.tiles.mapbox.com/mapbox-gl-js/v0.51.0/mapbox-gl.css" rel="stylesheet" />
<!-- Mapbox GL JS -->
<script src="https://api.tiles.mapbox.com/mapbox-gl-js/v0.51.0/mapbox-gl.js"></script>
I am trying to utilize this component to set the default view of the map bounds using either center
or bounds
or fitBounds
to a list of Lng,Lat coordinates. So, basically, how to plug in lng,lat coordinates and have the map default to centering these coordinates inside of the container?
Here's a Component I created, called Map
in vue to output the mapbox using the component vue-mapbox
listed above:
<template>
<b-row id="map" class="d-flex justify-content-center align-items-center my-2">
<b-col cols="24" id="map-holder" v-bind:class="getMapType">
<mgl-map
id="map-obj"
:accessToken="accessToken"
:mapStyle.sync="mapStyle"
:zoom="zoom"
:center="center"
container="map-holder"
:interactive="interactive"
@load="loadMap"
ref="mapbox" />
</b-col>
</b-row>
</template>
<script>
import MglMap from 'vue-mapbox'
export default
components:
MglMap
,
data ()
return
accessToken: 'pk.eyJ1Ijoic29sb2dob3N0IiwiYSI6ImNqb2htbmpwNjA0aG8zcWxjc3IzOGI1ejcifQ.nGL4NwbJYffJpjOiBL-Zpg',
mapStyle: 'mapbox://styles/mapbox/streets-v9', // options: basic-v9, streets-v9, bright-v9, light-v9, dark-v9, satellite-v9
zoom: 9,
map: , // Holds the Map...
fitBounds: [[-79, 43], [-73, 45]]
,
props:
interactive:
default: true
,
resizeMap:
default: false
,
mapType:
default: ''
,
center:
type: Array,
default: function () return [4.899, 52.372]
,
computed:
getMapType ()
let classes = 'inner-map'
if (this.mapType !== '')
classes += ' map-' + this.mapType
return classes
,
watch:
resizeMap (val)
if (val)
this.$nextTick(() => this.$refs.mapbox.resize())
,
fitBounds (val)
if (this.fitBounds.length)
this.MoveMapCoords()
,
methods:
loadMap ()
if (this.map === null)
this.map = event.map // store the map object in here...
,
MoveMapCoords ()
this.$refs.mapbox.fitBounds(this.fitBounds)
</script>
<style lang="scss" scoped>
@import '../../styles/custom.scss';
#map
#map-obj
text-align: justify;
width: 100%;
#map-holder
&.map-modal
#map-obj
height: 340px;
&.map-large
#map-obj
height: 500px;
.mapboxgl-map
border: 2px solid lightgray;
</style>
So, I'm trying to use fitBounds
method here to get the map to initialize centered over 2 Lng,Lat coordinates here: [[-79, 43], [-73, 45]]
How to do this exactly? Ok, I think I might have an error in my code a bit, so I think the fitBounds
should look something like this instead:
fitBounds: () =>
return bounds: [[-79, 43], [-73, 45]]
In any case, having the most difficult time setting the initial location of the mapbox to be centered over 2 or more coordinates. Anyone do this successfully yet?
Ok, so I wound up creating a filter to add space to the bbox like so:
Vue.filter('addSpaceToBBoxBounds', function (value)
if (value && value.length)
var boxArea =
for (var b = 0, len = value.length; b < len; b++)
boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2)
return boxArea
return value
)
This looks to be good enough for now. Than just use it like so:
let line = turf.lineString(this.markers)
mapOptions['bounds'] = this.$options.filters.addSpaceToBBoxBounds(turf.bbox(line))
return mapOptions
javascript vuejs2 mapbox-gl-js vue-mapbox
javascript vuejs2 mapbox-gl-js vue-mapbox
edited Nov 19 '18 at 0:17
Solomon Closson
asked Nov 16 '18 at 1:31
Solomon ClossonSolomon Closson
2,573104279
2,573104279
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
setting the initial location of the map to be centered over 2 or
more coordinates
You could use Turf.js to calculate the bounding box of all point features and initialize the map with this bbox using the bounds
map option:
http://turfjs.org/docs#bbox
https://www.mapbox.com/mapbox-gl-js/api/#map
Thanks, I took your advice and got it working, just need to figure out how to add some padding to the bounds here, cause some of the markers are on the line of the bounds and doesn't have space to show them all.
– Solomon Closson
Nov 16 '18 at 19:20
There is an open GL JS issue regarding adding padding (among other options) to thebounds
map option. Workaround described there: ... set the bounds without additional fitBounds options as a Map option to get it close enough at initialisation, and then immediately call map.fitBounds with the same bounds but with additional options. mapbox.com/mapbox-gl-js/api/#map#fitbounds
– pathmapper
Nov 17 '18 at 0:39
2
map.fitBounds(bbox, padding: top: 20, bottom: 20, left: 20, right: 20 );
– pathmapper
Nov 17 '18 at 0:50
2
or for shorthandmap.fitBounds(bbox, padding: 20 );
– AndrewHarvey
Nov 17 '18 at 5:03
I actually winded up creating a filter that added +2 and -2 zoom level to the bounds like so:Vue.filter('addSpaceToBBoxBounds', function (value) if (value && value.length) var boxArea = for (var b = 0, len = value.length; b < len; b++) boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2) return boxArea return value )
– Solomon Closson
Nov 19 '18 at 0:08
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53330202%2fset-map-bounds-based-on-multiple-marker-lng-lat%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
setting the initial location of the map to be centered over 2 or
more coordinates
You could use Turf.js to calculate the bounding box of all point features and initialize the map with this bbox using the bounds
map option:
http://turfjs.org/docs#bbox
https://www.mapbox.com/mapbox-gl-js/api/#map
Thanks, I took your advice and got it working, just need to figure out how to add some padding to the bounds here, cause some of the markers are on the line of the bounds and doesn't have space to show them all.
– Solomon Closson
Nov 16 '18 at 19:20
There is an open GL JS issue regarding adding padding (among other options) to thebounds
map option. Workaround described there: ... set the bounds without additional fitBounds options as a Map option to get it close enough at initialisation, and then immediately call map.fitBounds with the same bounds but with additional options. mapbox.com/mapbox-gl-js/api/#map#fitbounds
– pathmapper
Nov 17 '18 at 0:39
2
map.fitBounds(bbox, padding: top: 20, bottom: 20, left: 20, right: 20 );
– pathmapper
Nov 17 '18 at 0:50
2
or for shorthandmap.fitBounds(bbox, padding: 20 );
– AndrewHarvey
Nov 17 '18 at 5:03
I actually winded up creating a filter that added +2 and -2 zoom level to the bounds like so:Vue.filter('addSpaceToBBoxBounds', function (value) if (value && value.length) var boxArea = for (var b = 0, len = value.length; b < len; b++) boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2) return boxArea return value )
– Solomon Closson
Nov 19 '18 at 0:08
add a comment |
setting the initial location of the map to be centered over 2 or
more coordinates
You could use Turf.js to calculate the bounding box of all point features and initialize the map with this bbox using the bounds
map option:
http://turfjs.org/docs#bbox
https://www.mapbox.com/mapbox-gl-js/api/#map
Thanks, I took your advice and got it working, just need to figure out how to add some padding to the bounds here, cause some of the markers are on the line of the bounds and doesn't have space to show them all.
– Solomon Closson
Nov 16 '18 at 19:20
There is an open GL JS issue regarding adding padding (among other options) to thebounds
map option. Workaround described there: ... set the bounds without additional fitBounds options as a Map option to get it close enough at initialisation, and then immediately call map.fitBounds with the same bounds but with additional options. mapbox.com/mapbox-gl-js/api/#map#fitbounds
– pathmapper
Nov 17 '18 at 0:39
2
map.fitBounds(bbox, padding: top: 20, bottom: 20, left: 20, right: 20 );
– pathmapper
Nov 17 '18 at 0:50
2
or for shorthandmap.fitBounds(bbox, padding: 20 );
– AndrewHarvey
Nov 17 '18 at 5:03
I actually winded up creating a filter that added +2 and -2 zoom level to the bounds like so:Vue.filter('addSpaceToBBoxBounds', function (value) if (value && value.length) var boxArea = for (var b = 0, len = value.length; b < len; b++) boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2) return boxArea return value )
– Solomon Closson
Nov 19 '18 at 0:08
add a comment |
setting the initial location of the map to be centered over 2 or
more coordinates
You could use Turf.js to calculate the bounding box of all point features and initialize the map with this bbox using the bounds
map option:
http://turfjs.org/docs#bbox
https://www.mapbox.com/mapbox-gl-js/api/#map
setting the initial location of the map to be centered over 2 or
more coordinates
You could use Turf.js to calculate the bounding box of all point features and initialize the map with this bbox using the bounds
map option:
http://turfjs.org/docs#bbox
https://www.mapbox.com/mapbox-gl-js/api/#map
edited Nov 17 '18 at 0:45
answered Nov 16 '18 at 7:04
pathmapperpathmapper
500310
500310
Thanks, I took your advice and got it working, just need to figure out how to add some padding to the bounds here, cause some of the markers are on the line of the bounds and doesn't have space to show them all.
– Solomon Closson
Nov 16 '18 at 19:20
There is an open GL JS issue regarding adding padding (among other options) to thebounds
map option. Workaround described there: ... set the bounds without additional fitBounds options as a Map option to get it close enough at initialisation, and then immediately call map.fitBounds with the same bounds but with additional options. mapbox.com/mapbox-gl-js/api/#map#fitbounds
– pathmapper
Nov 17 '18 at 0:39
2
map.fitBounds(bbox, padding: top: 20, bottom: 20, left: 20, right: 20 );
– pathmapper
Nov 17 '18 at 0:50
2
or for shorthandmap.fitBounds(bbox, padding: 20 );
– AndrewHarvey
Nov 17 '18 at 5:03
I actually winded up creating a filter that added +2 and -2 zoom level to the bounds like so:Vue.filter('addSpaceToBBoxBounds', function (value) if (value && value.length) var boxArea = for (var b = 0, len = value.length; b < len; b++) boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2) return boxArea return value )
– Solomon Closson
Nov 19 '18 at 0:08
add a comment |
Thanks, I took your advice and got it working, just need to figure out how to add some padding to the bounds here, cause some of the markers are on the line of the bounds and doesn't have space to show them all.
– Solomon Closson
Nov 16 '18 at 19:20
There is an open GL JS issue regarding adding padding (among other options) to thebounds
map option. Workaround described there: ... set the bounds without additional fitBounds options as a Map option to get it close enough at initialisation, and then immediately call map.fitBounds with the same bounds but with additional options. mapbox.com/mapbox-gl-js/api/#map#fitbounds
– pathmapper
Nov 17 '18 at 0:39
2
map.fitBounds(bbox, padding: top: 20, bottom: 20, left: 20, right: 20 );
– pathmapper
Nov 17 '18 at 0:50
2
or for shorthandmap.fitBounds(bbox, padding: 20 );
– AndrewHarvey
Nov 17 '18 at 5:03
I actually winded up creating a filter that added +2 and -2 zoom level to the bounds like so:Vue.filter('addSpaceToBBoxBounds', function (value) if (value && value.length) var boxArea = for (var b = 0, len = value.length; b < len; b++) boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2) return boxArea return value )
– Solomon Closson
Nov 19 '18 at 0:08
Thanks, I took your advice and got it working, just need to figure out how to add some padding to the bounds here, cause some of the markers are on the line of the bounds and doesn't have space to show them all.
– Solomon Closson
Nov 16 '18 at 19:20
Thanks, I took your advice and got it working, just need to figure out how to add some padding to the bounds here, cause some of the markers are on the line of the bounds and doesn't have space to show them all.
– Solomon Closson
Nov 16 '18 at 19:20
There is an open GL JS issue regarding adding padding (among other options) to the
bounds
map option. Workaround described there: ... set the bounds without additional fitBounds options as a Map option to get it close enough at initialisation, and then immediately call map.fitBounds with the same bounds but with additional options. mapbox.com/mapbox-gl-js/api/#map#fitbounds– pathmapper
Nov 17 '18 at 0:39
There is an open GL JS issue regarding adding padding (among other options) to the
bounds
map option. Workaround described there: ... set the bounds without additional fitBounds options as a Map option to get it close enough at initialisation, and then immediately call map.fitBounds with the same bounds but with additional options. mapbox.com/mapbox-gl-js/api/#map#fitbounds– pathmapper
Nov 17 '18 at 0:39
2
2
map.fitBounds(bbox, padding: top: 20, bottom: 20, left: 20, right: 20 );
– pathmapper
Nov 17 '18 at 0:50
map.fitBounds(bbox, padding: top: 20, bottom: 20, left: 20, right: 20 );
– pathmapper
Nov 17 '18 at 0:50
2
2
or for shorthand
map.fitBounds(bbox, padding: 20 );
– AndrewHarvey
Nov 17 '18 at 5:03
or for shorthand
map.fitBounds(bbox, padding: 20 );
– AndrewHarvey
Nov 17 '18 at 5:03
I actually winded up creating a filter that added +2 and -2 zoom level to the bounds like so:
Vue.filter('addSpaceToBBoxBounds', function (value) if (value && value.length) var boxArea = for (var b = 0, len = value.length; b < len; b++) boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2) return boxArea return value )
– Solomon Closson
Nov 19 '18 at 0:08
I actually winded up creating a filter that added +2 and -2 zoom level to the bounds like so:
Vue.filter('addSpaceToBBoxBounds', function (value) if (value && value.length) var boxArea = for (var b = 0, len = value.length; b < len; b++) boxArea.push(b > 1 ? value[b] + 2 : value[b] - 2) return boxArea return value )
– Solomon Closson
Nov 19 '18 at 0:08
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53330202%2fset-map-bounds-based-on-multiple-marker-lng-lat%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NtXdP6MH,BAeqLK2A0PCYOZ4hj6rQ1A,8EMAGome,bB2caSpfNXv,j IglytvBtxqXrGJhPHV2b U,W MGivuY3W1NcFocFv,bj8AZ t,C