Updating a User's skills using ManyToManyField in django
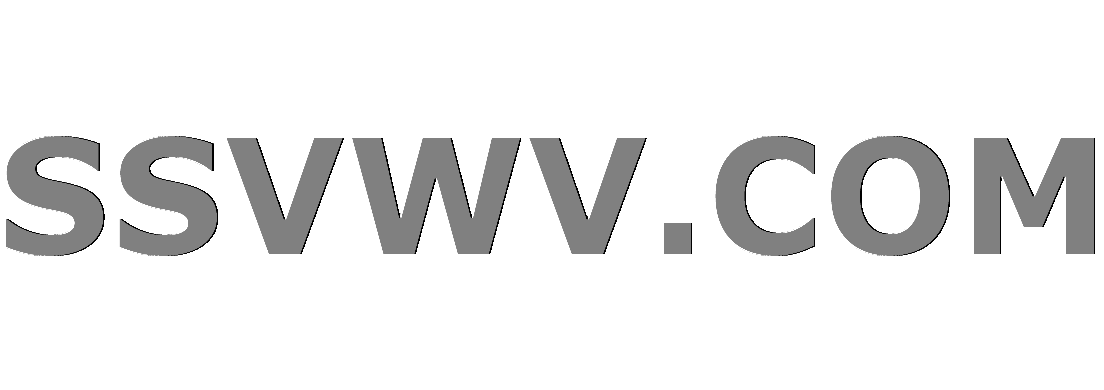
Multi tool use
I've been stuck on this for a few days (new to django) and can't figure out how to update skills for a specific user model using a ManyToManyField, while simultaneously updating a skill model containing a list of skills. Currently when I enter a value in my SkillForm, it updates the skill model properly and creates a dropdown list of skills for a given CustomUser in the admin. However, I can't figure out how to assign a SPECIFIC skill to a particular user. Any help is appreciated.
models.py:
class Skill(models.Model):
name = models.CharField(max_length =50, null=True, default='')
def __str__(self):
return self.name
class CustomUserManager(UserManager):
pass
class CustomUser(AbstractUser):
objects = CustomUserManager()
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
admin.py:
class SkillsInline(admin.StackedInline):
model = CustomUser.skills.through
class SkillAdmin(admin.ModelAdmin):
inlines = [SkillsInline ,]
UserAdmin.fieldsets += ('Custom fields set', 'fields': ('position', 'bio', )),
class CustomUserAdmin(UserAdmin):
model = CustomUser
add_form = CustomUserCreationForm
form = EditProfile
inlines = [SkillsInline ,]
admin.site.register(CustomUser, CustomUserAdmin)
forms.py:
class SkillForm(forms.ModelForm):
name = forms.CharField()
class Meta:
model = Skill
fields =('name' ,)
def clean(self):
cleaned_data = super().clean()
name = cleaned_data.get('name')
python django
add a comment |
I've been stuck on this for a few days (new to django) and can't figure out how to update skills for a specific user model using a ManyToManyField, while simultaneously updating a skill model containing a list of skills. Currently when I enter a value in my SkillForm, it updates the skill model properly and creates a dropdown list of skills for a given CustomUser in the admin. However, I can't figure out how to assign a SPECIFIC skill to a particular user. Any help is appreciated.
models.py:
class Skill(models.Model):
name = models.CharField(max_length =50, null=True, default='')
def __str__(self):
return self.name
class CustomUserManager(UserManager):
pass
class CustomUser(AbstractUser):
objects = CustomUserManager()
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
admin.py:
class SkillsInline(admin.StackedInline):
model = CustomUser.skills.through
class SkillAdmin(admin.ModelAdmin):
inlines = [SkillsInline ,]
UserAdmin.fieldsets += ('Custom fields set', 'fields': ('position', 'bio', )),
class CustomUserAdmin(UserAdmin):
model = CustomUser
add_form = CustomUserCreationForm
form = EditProfile
inlines = [SkillsInline ,]
admin.site.register(CustomUser, CustomUserAdmin)
forms.py:
class SkillForm(forms.ModelForm):
name = forms.CharField()
class Meta:
model = Skill
fields =('name' ,)
def clean(self):
cleaned_data = super().clean()
name = cleaned_data.get('name')
python django
I don't understand what you mean by "how to assign a SPECIFIC skill to a particular user". You go to the admin page for the user and select the skill from the inline form, surely? Where exactly are you having trouble?
– Daniel Roseman
Nov 15 '18 at 19:38
That method works, however, I want the user to be able to enter their own skill or list of skills in a form, and then have that entry update 1) their user skills field and 2) the skill model which contains all possible skills in the database. I've tried several form variations with no luck
– user6210879
Nov 15 '18 at 20:23
add a comment |
I've been stuck on this for a few days (new to django) and can't figure out how to update skills for a specific user model using a ManyToManyField, while simultaneously updating a skill model containing a list of skills. Currently when I enter a value in my SkillForm, it updates the skill model properly and creates a dropdown list of skills for a given CustomUser in the admin. However, I can't figure out how to assign a SPECIFIC skill to a particular user. Any help is appreciated.
models.py:
class Skill(models.Model):
name = models.CharField(max_length =50, null=True, default='')
def __str__(self):
return self.name
class CustomUserManager(UserManager):
pass
class CustomUser(AbstractUser):
objects = CustomUserManager()
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
admin.py:
class SkillsInline(admin.StackedInline):
model = CustomUser.skills.through
class SkillAdmin(admin.ModelAdmin):
inlines = [SkillsInline ,]
UserAdmin.fieldsets += ('Custom fields set', 'fields': ('position', 'bio', )),
class CustomUserAdmin(UserAdmin):
model = CustomUser
add_form = CustomUserCreationForm
form = EditProfile
inlines = [SkillsInline ,]
admin.site.register(CustomUser, CustomUserAdmin)
forms.py:
class SkillForm(forms.ModelForm):
name = forms.CharField()
class Meta:
model = Skill
fields =('name' ,)
def clean(self):
cleaned_data = super().clean()
name = cleaned_data.get('name')
python django
I've been stuck on this for a few days (new to django) and can't figure out how to update skills for a specific user model using a ManyToManyField, while simultaneously updating a skill model containing a list of skills. Currently when I enter a value in my SkillForm, it updates the skill model properly and creates a dropdown list of skills for a given CustomUser in the admin. However, I can't figure out how to assign a SPECIFIC skill to a particular user. Any help is appreciated.
models.py:
class Skill(models.Model):
name = models.CharField(max_length =50, null=True, default='')
def __str__(self):
return self.name
class CustomUserManager(UserManager):
pass
class CustomUser(AbstractUser):
objects = CustomUserManager()
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
admin.py:
class SkillsInline(admin.StackedInline):
model = CustomUser.skills.through
class SkillAdmin(admin.ModelAdmin):
inlines = [SkillsInline ,]
UserAdmin.fieldsets += ('Custom fields set', 'fields': ('position', 'bio', )),
class CustomUserAdmin(UserAdmin):
model = CustomUser
add_form = CustomUserCreationForm
form = EditProfile
inlines = [SkillsInline ,]
admin.site.register(CustomUser, CustomUserAdmin)
forms.py:
class SkillForm(forms.ModelForm):
name = forms.CharField()
class Meta:
model = Skill
fields =('name' ,)
def clean(self):
cleaned_data = super().clean()
name = cleaned_data.get('name')
python django
python django
edited Nov 15 '18 at 17:50
user6210879
asked Nov 15 '18 at 17:29
user6210879user6210879
226
226
I don't understand what you mean by "how to assign a SPECIFIC skill to a particular user". You go to the admin page for the user and select the skill from the inline form, surely? Where exactly are you having trouble?
– Daniel Roseman
Nov 15 '18 at 19:38
That method works, however, I want the user to be able to enter their own skill or list of skills in a form, and then have that entry update 1) their user skills field and 2) the skill model which contains all possible skills in the database. I've tried several form variations with no luck
– user6210879
Nov 15 '18 at 20:23
add a comment |
I don't understand what you mean by "how to assign a SPECIFIC skill to a particular user". You go to the admin page for the user and select the skill from the inline form, surely? Where exactly are you having trouble?
– Daniel Roseman
Nov 15 '18 at 19:38
That method works, however, I want the user to be able to enter their own skill or list of skills in a form, and then have that entry update 1) their user skills field and 2) the skill model which contains all possible skills in the database. I've tried several form variations with no luck
– user6210879
Nov 15 '18 at 20:23
I don't understand what you mean by "how to assign a SPECIFIC skill to a particular user". You go to the admin page for the user and select the skill from the inline form, surely? Where exactly are you having trouble?
– Daniel Roseman
Nov 15 '18 at 19:38
I don't understand what you mean by "how to assign a SPECIFIC skill to a particular user". You go to the admin page for the user and select the skill from the inline form, surely? Where exactly are you having trouble?
– Daniel Roseman
Nov 15 '18 at 19:38
That method works, however, I want the user to be able to enter their own skill or list of skills in a form, and then have that entry update 1) their user skills field and 2) the skill model which contains all possible skills in the database. I've tried several form variations with no luck
– user6210879
Nov 15 '18 at 20:23
That method works, however, I want the user to be able to enter their own skill or list of skills in a form, and then have that entry update 1) their user skills field and 2) the skill model which contains all possible skills in the database. I've tried several form variations with no luck
– user6210879
Nov 15 '18 at 20:23
add a comment |
1 Answer
1
active
oldest
votes
I see somes problem here :
class CustomUser(AbstractUser):
objects = CustomUserManager()
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
When you define an AbstractUser` class, it's mean for Django Authentification purpose, see the doc here., dont do that because it's better to have an unique entry (each user have an unique entry, so you can login each one).
In your system you dont have unique CustomUser
, here is a solution IMO:
class User(AbstractUser):
email = models.EmailField(_('email'), unique=True)
class UserAction(models.Model):
objects = CustomUserManager()
user = models.ForeignKey(User, verbose_name="User")
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
class Skill(models.Model):
name = models.CharField(max_length =50, null=True, default='')
def __str__(self):
return self.name
You now have an unique User
, a list of UserAction
that have a related Skill
user = User.objects.create(email='my@email.com')
skill = Skill.objects.create(name="shadow step")
user_action = UserAction.objects.create(user=user, skill=skill, position='HERE', bio='10')
Is that what you needed ?
Thanks I will try this. However, I was following the steps on this blog: wsvincent.com/django-allauth-tutorial-custom-user-model which suggests using a CustomUser model. I believe the problem is: when a skill is entered in a form, I need it to update the User skills or vice versa but can't achieve this with my current set-up.
– user6210879
Nov 15 '18 at 18:53
I should also mention that all of my other fields update appropriately for a given user with a "CustomUserChangeForm"
– user6210879
Nov 15 '18 at 18:57
Your justification for not using a custom user class doesn't make much sense here; why would a user need multiple positions and bios? OP was right originally, they belong on the CustomUser class.
– Daniel Roseman
Nov 15 '18 at 19:33
Hi, i dont have the purpose of this code, in my head its just like an RPG game where the user move, use skill and loose HP, maybe i'm in the wrong
– MaximeK
Nov 16 '18 at 9:28
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324944%2fupdating-a-users-skills-using-manytomanyfield-in-django%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I see somes problem here :
class CustomUser(AbstractUser):
objects = CustomUserManager()
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
When you define an AbstractUser` class, it's mean for Django Authentification purpose, see the doc here., dont do that because it's better to have an unique entry (each user have an unique entry, so you can login each one).
In your system you dont have unique CustomUser
, here is a solution IMO:
class User(AbstractUser):
email = models.EmailField(_('email'), unique=True)
class UserAction(models.Model):
objects = CustomUserManager()
user = models.ForeignKey(User, verbose_name="User")
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
class Skill(models.Model):
name = models.CharField(max_length =50, null=True, default='')
def __str__(self):
return self.name
You now have an unique User
, a list of UserAction
that have a related Skill
user = User.objects.create(email='my@email.com')
skill = Skill.objects.create(name="shadow step")
user_action = UserAction.objects.create(user=user, skill=skill, position='HERE', bio='10')
Is that what you needed ?
Thanks I will try this. However, I was following the steps on this blog: wsvincent.com/django-allauth-tutorial-custom-user-model which suggests using a CustomUser model. I believe the problem is: when a skill is entered in a form, I need it to update the User skills or vice versa but can't achieve this with my current set-up.
– user6210879
Nov 15 '18 at 18:53
I should also mention that all of my other fields update appropriately for a given user with a "CustomUserChangeForm"
– user6210879
Nov 15 '18 at 18:57
Your justification for not using a custom user class doesn't make much sense here; why would a user need multiple positions and bios? OP was right originally, they belong on the CustomUser class.
– Daniel Roseman
Nov 15 '18 at 19:33
Hi, i dont have the purpose of this code, in my head its just like an RPG game where the user move, use skill and loose HP, maybe i'm in the wrong
– MaximeK
Nov 16 '18 at 9:28
add a comment |
I see somes problem here :
class CustomUser(AbstractUser):
objects = CustomUserManager()
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
When you define an AbstractUser` class, it's mean for Django Authentification purpose, see the doc here., dont do that because it's better to have an unique entry (each user have an unique entry, so you can login each one).
In your system you dont have unique CustomUser
, here is a solution IMO:
class User(AbstractUser):
email = models.EmailField(_('email'), unique=True)
class UserAction(models.Model):
objects = CustomUserManager()
user = models.ForeignKey(User, verbose_name="User")
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
class Skill(models.Model):
name = models.CharField(max_length =50, null=True, default='')
def __str__(self):
return self.name
You now have an unique User
, a list of UserAction
that have a related Skill
user = User.objects.create(email='my@email.com')
skill = Skill.objects.create(name="shadow step")
user_action = UserAction.objects.create(user=user, skill=skill, position='HERE', bio='10')
Is that what you needed ?
Thanks I will try this. However, I was following the steps on this blog: wsvincent.com/django-allauth-tutorial-custom-user-model which suggests using a CustomUser model. I believe the problem is: when a skill is entered in a form, I need it to update the User skills or vice versa but can't achieve this with my current set-up.
– user6210879
Nov 15 '18 at 18:53
I should also mention that all of my other fields update appropriately for a given user with a "CustomUserChangeForm"
– user6210879
Nov 15 '18 at 18:57
Your justification for not using a custom user class doesn't make much sense here; why would a user need multiple positions and bios? OP was right originally, they belong on the CustomUser class.
– Daniel Roseman
Nov 15 '18 at 19:33
Hi, i dont have the purpose of this code, in my head its just like an RPG game where the user move, use skill and loose HP, maybe i'm in the wrong
– MaximeK
Nov 16 '18 at 9:28
add a comment |
I see somes problem here :
class CustomUser(AbstractUser):
objects = CustomUserManager()
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
When you define an AbstractUser` class, it's mean for Django Authentification purpose, see the doc here., dont do that because it's better to have an unique entry (each user have an unique entry, so you can login each one).
In your system you dont have unique CustomUser
, here is a solution IMO:
class User(AbstractUser):
email = models.EmailField(_('email'), unique=True)
class UserAction(models.Model):
objects = CustomUserManager()
user = models.ForeignKey(User, verbose_name="User")
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
class Skill(models.Model):
name = models.CharField(max_length =50, null=True, default='')
def __str__(self):
return self.name
You now have an unique User
, a list of UserAction
that have a related Skill
user = User.objects.create(email='my@email.com')
skill = Skill.objects.create(name="shadow step")
user_action = UserAction.objects.create(user=user, skill=skill, position='HERE', bio='10')
Is that what you needed ?
I see somes problem here :
class CustomUser(AbstractUser):
objects = CustomUserManager()
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
When you define an AbstractUser` class, it's mean for Django Authentification purpose, see the doc here., dont do that because it's better to have an unique entry (each user have an unique entry, so you can login each one).
In your system you dont have unique CustomUser
, here is a solution IMO:
class User(AbstractUser):
email = models.EmailField(_('email'), unique=True)
class UserAction(models.Model):
objects = CustomUserManager()
user = models.ForeignKey(User, verbose_name="User")
skills = models.ManyToManyField(Skill, null=True, blank=True)
position = models.CharField(max_length =50, null=True, default='')
bio = models.CharField(max_length=300, null=True, default='')
class Skill(models.Model):
name = models.CharField(max_length =50, null=True, default='')
def __str__(self):
return self.name
You now have an unique User
, a list of UserAction
that have a related Skill
user = User.objects.create(email='my@email.com')
skill = Skill.objects.create(name="shadow step")
user_action = UserAction.objects.create(user=user, skill=skill, position='HERE', bio='10')
Is that what you needed ?
answered Nov 15 '18 at 18:15
MaximeKMaximeK
1,0261510
1,0261510
Thanks I will try this. However, I was following the steps on this blog: wsvincent.com/django-allauth-tutorial-custom-user-model which suggests using a CustomUser model. I believe the problem is: when a skill is entered in a form, I need it to update the User skills or vice versa but can't achieve this with my current set-up.
– user6210879
Nov 15 '18 at 18:53
I should also mention that all of my other fields update appropriately for a given user with a "CustomUserChangeForm"
– user6210879
Nov 15 '18 at 18:57
Your justification for not using a custom user class doesn't make much sense here; why would a user need multiple positions and bios? OP was right originally, they belong on the CustomUser class.
– Daniel Roseman
Nov 15 '18 at 19:33
Hi, i dont have the purpose of this code, in my head its just like an RPG game where the user move, use skill and loose HP, maybe i'm in the wrong
– MaximeK
Nov 16 '18 at 9:28
add a comment |
Thanks I will try this. However, I was following the steps on this blog: wsvincent.com/django-allauth-tutorial-custom-user-model which suggests using a CustomUser model. I believe the problem is: when a skill is entered in a form, I need it to update the User skills or vice versa but can't achieve this with my current set-up.
– user6210879
Nov 15 '18 at 18:53
I should also mention that all of my other fields update appropriately for a given user with a "CustomUserChangeForm"
– user6210879
Nov 15 '18 at 18:57
Your justification for not using a custom user class doesn't make much sense here; why would a user need multiple positions and bios? OP was right originally, they belong on the CustomUser class.
– Daniel Roseman
Nov 15 '18 at 19:33
Hi, i dont have the purpose of this code, in my head its just like an RPG game where the user move, use skill and loose HP, maybe i'm in the wrong
– MaximeK
Nov 16 '18 at 9:28
Thanks I will try this. However, I was following the steps on this blog: wsvincent.com/django-allauth-tutorial-custom-user-model which suggests using a CustomUser model. I believe the problem is: when a skill is entered in a form, I need it to update the User skills or vice versa but can't achieve this with my current set-up.
– user6210879
Nov 15 '18 at 18:53
Thanks I will try this. However, I was following the steps on this blog: wsvincent.com/django-allauth-tutorial-custom-user-model which suggests using a CustomUser model. I believe the problem is: when a skill is entered in a form, I need it to update the User skills or vice versa but can't achieve this with my current set-up.
– user6210879
Nov 15 '18 at 18:53
I should also mention that all of my other fields update appropriately for a given user with a "CustomUserChangeForm"
– user6210879
Nov 15 '18 at 18:57
I should also mention that all of my other fields update appropriately for a given user with a "CustomUserChangeForm"
– user6210879
Nov 15 '18 at 18:57
Your justification for not using a custom user class doesn't make much sense here; why would a user need multiple positions and bios? OP was right originally, they belong on the CustomUser class.
– Daniel Roseman
Nov 15 '18 at 19:33
Your justification for not using a custom user class doesn't make much sense here; why would a user need multiple positions and bios? OP was right originally, they belong on the CustomUser class.
– Daniel Roseman
Nov 15 '18 at 19:33
Hi, i dont have the purpose of this code, in my head its just like an RPG game where the user move, use skill and loose HP, maybe i'm in the wrong
– MaximeK
Nov 16 '18 at 9:28
Hi, i dont have the purpose of this code, in my head its just like an RPG game where the user move, use skill and loose HP, maybe i'm in the wrong
– MaximeK
Nov 16 '18 at 9:28
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324944%2fupdating-a-users-skills-using-manytomanyfield-in-django%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hcw2AqOfU gpJSRXXjrafSfBKe4AwavE2jaB0QfF2YC20,3l tDDa5aG00V41IWH3s,L
I don't understand what you mean by "how to assign a SPECIFIC skill to a particular user". You go to the admin page for the user and select the skill from the inline form, surely? Where exactly are you having trouble?
– Daniel Roseman
Nov 15 '18 at 19:38
That method works, however, I want the user to be able to enter their own skill or list of skills in a form, and then have that entry update 1) their user skills field and 2) the skill model which contains all possible skills in the database. I've tried several form variations with no luck
– user6210879
Nov 15 '18 at 20:23