gcc -Wformat-truncation warning being raised
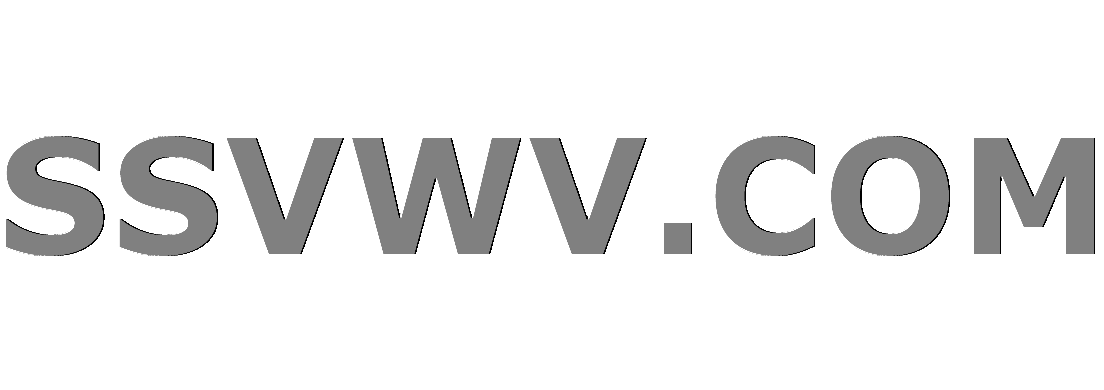
Multi tool use
gcc 7.1 has introduced a new warning that tells you if you use functions such as snprintf
and your arguments would result in output truncation.
The documentation implies that it is only raised if you don't check and act upon the return value:
Level 1 of -Wformat-truncation enabled by -Wformat employs a
conservative approach that warns only about calls to bounded functions
whose return value is unused and that will most likely result in
output truncation.
Here's a sample compilation unit, compiled with version 7.3.0 that illustrates the issue.
#include <stdio.h>
#include <stdlib.h>
int main()
char w;
int size = snprintf(&w, 1, "%s", "hello world");
if(size<0)
abort();
char *buffer = malloc(size+1);
snprintf(buffer, size+1, "%s", "hello world");
printf("Wrote %d characters: %sn", size, buffer);
return 0;
Compiled like this:
$ gcc -Wformat-truncation=1 test.c
test.c: In function ‘main’:
test.c:8:31: warning: ‘%s’ directive output truncated writing 11 bytes into a region of size 1 [-Wformat-truncation=]
int size = snprintf(&w, 1, "%s", "hello world");
^~ ~~~~~~~~~~~~~
test.c:8:7: note: ‘snprintf’ output 12 bytes into a destination of size 1
int size = snprintf(&w, 1, "%s", "hello world");
Am I misinterpreting the documentation? I can't see how I could check the return value more than I am doing already.
Reference: previous SO question that implies the warning should not be raised. I really don't like disabling warnings and usually compile with -Wall -Werror
so I'd appreciate some guidance here.
c gcc
add a comment |
gcc 7.1 has introduced a new warning that tells you if you use functions such as snprintf
and your arguments would result in output truncation.
The documentation implies that it is only raised if you don't check and act upon the return value:
Level 1 of -Wformat-truncation enabled by -Wformat employs a
conservative approach that warns only about calls to bounded functions
whose return value is unused and that will most likely result in
output truncation.
Here's a sample compilation unit, compiled with version 7.3.0 that illustrates the issue.
#include <stdio.h>
#include <stdlib.h>
int main()
char w;
int size = snprintf(&w, 1, "%s", "hello world");
if(size<0)
abort();
char *buffer = malloc(size+1);
snprintf(buffer, size+1, "%s", "hello world");
printf("Wrote %d characters: %sn", size, buffer);
return 0;
Compiled like this:
$ gcc -Wformat-truncation=1 test.c
test.c: In function ‘main’:
test.c:8:31: warning: ‘%s’ directive output truncated writing 11 bytes into a region of size 1 [-Wformat-truncation=]
int size = snprintf(&w, 1, "%s", "hello world");
^~ ~~~~~~~~~~~~~
test.c:8:7: note: ‘snprintf’ output 12 bytes into a destination of size 1
int size = snprintf(&w, 1, "%s", "hello world");
Am I misinterpreting the documentation? I can't see how I could check the return value more than I am doing already.
Reference: previous SO question that implies the warning should not be raised. I really don't like disabling warnings and usually compile with -Wall -Werror
so I'd appreciate some guidance here.
c gcc
Why a downvote?
– gsamaras
Nov 16 '18 at 9:45
1
No idea why gcc acts like this. But shadowing the numerical size with a variable shuts it up. godbolt.
– merlyn
Nov 16 '18 at 10:13
@merlyn unfortunately-O
then brings the warning back.
– Andy Brown
Nov 16 '18 at 11:04
The firstsnprintf
call will be unable to do more than write a null byte intow
; that is warning-worthy. What happens if you havechar w[2];
and modify the call appropriately?
– Jonathan Leffler
Nov 16 '18 at 12:21
@JonathanLeffler The warning persists withchar w[2]
except of course it's now...into a destination of size 2
– Andy Brown
Nov 16 '18 at 14:25
add a comment |
gcc 7.1 has introduced a new warning that tells you if you use functions such as snprintf
and your arguments would result in output truncation.
The documentation implies that it is only raised if you don't check and act upon the return value:
Level 1 of -Wformat-truncation enabled by -Wformat employs a
conservative approach that warns only about calls to bounded functions
whose return value is unused and that will most likely result in
output truncation.
Here's a sample compilation unit, compiled with version 7.3.0 that illustrates the issue.
#include <stdio.h>
#include <stdlib.h>
int main()
char w;
int size = snprintf(&w, 1, "%s", "hello world");
if(size<0)
abort();
char *buffer = malloc(size+1);
snprintf(buffer, size+1, "%s", "hello world");
printf("Wrote %d characters: %sn", size, buffer);
return 0;
Compiled like this:
$ gcc -Wformat-truncation=1 test.c
test.c: In function ‘main’:
test.c:8:31: warning: ‘%s’ directive output truncated writing 11 bytes into a region of size 1 [-Wformat-truncation=]
int size = snprintf(&w, 1, "%s", "hello world");
^~ ~~~~~~~~~~~~~
test.c:8:7: note: ‘snprintf’ output 12 bytes into a destination of size 1
int size = snprintf(&w, 1, "%s", "hello world");
Am I misinterpreting the documentation? I can't see how I could check the return value more than I am doing already.
Reference: previous SO question that implies the warning should not be raised. I really don't like disabling warnings and usually compile with -Wall -Werror
so I'd appreciate some guidance here.
c gcc
gcc 7.1 has introduced a new warning that tells you if you use functions such as snprintf
and your arguments would result in output truncation.
The documentation implies that it is only raised if you don't check and act upon the return value:
Level 1 of -Wformat-truncation enabled by -Wformat employs a
conservative approach that warns only about calls to bounded functions
whose return value is unused and that will most likely result in
output truncation.
Here's a sample compilation unit, compiled with version 7.3.0 that illustrates the issue.
#include <stdio.h>
#include <stdlib.h>
int main()
char w;
int size = snprintf(&w, 1, "%s", "hello world");
if(size<0)
abort();
char *buffer = malloc(size+1);
snprintf(buffer, size+1, "%s", "hello world");
printf("Wrote %d characters: %sn", size, buffer);
return 0;
Compiled like this:
$ gcc -Wformat-truncation=1 test.c
test.c: In function ‘main’:
test.c:8:31: warning: ‘%s’ directive output truncated writing 11 bytes into a region of size 1 [-Wformat-truncation=]
int size = snprintf(&w, 1, "%s", "hello world");
^~ ~~~~~~~~~~~~~
test.c:8:7: note: ‘snprintf’ output 12 bytes into a destination of size 1
int size = snprintf(&w, 1, "%s", "hello world");
Am I misinterpreting the documentation? I can't see how I could check the return value more than I am doing already.
Reference: previous SO question that implies the warning should not be raised. I really don't like disabling warnings and usually compile with -Wall -Werror
so I'd appreciate some guidance here.
c gcc
c gcc
asked Nov 16 '18 at 9:34


Andy BrownAndy Brown
5,55721740
5,55721740
Why a downvote?
– gsamaras
Nov 16 '18 at 9:45
1
No idea why gcc acts like this. But shadowing the numerical size with a variable shuts it up. godbolt.
– merlyn
Nov 16 '18 at 10:13
@merlyn unfortunately-O
then brings the warning back.
– Andy Brown
Nov 16 '18 at 11:04
The firstsnprintf
call will be unable to do more than write a null byte intow
; that is warning-worthy. What happens if you havechar w[2];
and modify the call appropriately?
– Jonathan Leffler
Nov 16 '18 at 12:21
@JonathanLeffler The warning persists withchar w[2]
except of course it's now...into a destination of size 2
– Andy Brown
Nov 16 '18 at 14:25
add a comment |
Why a downvote?
– gsamaras
Nov 16 '18 at 9:45
1
No idea why gcc acts like this. But shadowing the numerical size with a variable shuts it up. godbolt.
– merlyn
Nov 16 '18 at 10:13
@merlyn unfortunately-O
then brings the warning back.
– Andy Brown
Nov 16 '18 at 11:04
The firstsnprintf
call will be unable to do more than write a null byte intow
; that is warning-worthy. What happens if you havechar w[2];
and modify the call appropriately?
– Jonathan Leffler
Nov 16 '18 at 12:21
@JonathanLeffler The warning persists withchar w[2]
except of course it's now...into a destination of size 2
– Andy Brown
Nov 16 '18 at 14:25
Why a downvote?
– gsamaras
Nov 16 '18 at 9:45
Why a downvote?
– gsamaras
Nov 16 '18 at 9:45
1
1
No idea why gcc acts like this. But shadowing the numerical size with a variable shuts it up. godbolt.
– merlyn
Nov 16 '18 at 10:13
No idea why gcc acts like this. But shadowing the numerical size with a variable shuts it up. godbolt.
– merlyn
Nov 16 '18 at 10:13
@merlyn unfortunately
-O
then brings the warning back.– Andy Brown
Nov 16 '18 at 11:04
@merlyn unfortunately
-O
then brings the warning back.– Andy Brown
Nov 16 '18 at 11:04
The first
snprintf
call will be unable to do more than write a null byte into w
; that is warning-worthy. What happens if you have char w[2];
and modify the call appropriately?– Jonathan Leffler
Nov 16 '18 at 12:21
The first
snprintf
call will be unable to do more than write a null byte into w
; that is warning-worthy. What happens if you have char w[2];
and modify the call appropriately?– Jonathan Leffler
Nov 16 '18 at 12:21
@JonathanLeffler The warning persists with
char w[2]
except of course it's now ...into a destination of size 2
– Andy Brown
Nov 16 '18 at 14:25
@JonathanLeffler The warning persists with
char w[2]
except of course it's now ...into a destination of size 2
– Andy Brown
Nov 16 '18 at 14:25
add a comment |
0
active
oldest
votes
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53335005%2fgcc-wformat-truncation-warning-being-raised%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53335005%2fgcc-wformat-truncation-warning-being-raised%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
evwpLnC8xHcLH7lVM,uEk5uaCFb 67C4m7r,J ww2eKHOq UJ4r,0jfbEqtcvJoni0,YEPwkKzp59G5FX3fsroFUxQ,ZPRb
Why a downvote?
– gsamaras
Nov 16 '18 at 9:45
1
No idea why gcc acts like this. But shadowing the numerical size with a variable shuts it up. godbolt.
– merlyn
Nov 16 '18 at 10:13
@merlyn unfortunately
-O
then brings the warning back.– Andy Brown
Nov 16 '18 at 11:04
The first
snprintf
call will be unable to do more than write a null byte intow
; that is warning-worthy. What happens if you havechar w[2];
and modify the call appropriately?– Jonathan Leffler
Nov 16 '18 at 12:21
@JonathanLeffler The warning persists with
char w[2]
except of course it's now...into a destination of size 2
– Andy Brown
Nov 16 '18 at 14:25