Can any one explain how to use ViewModel and LiveData while implementing MVVM architecture in android
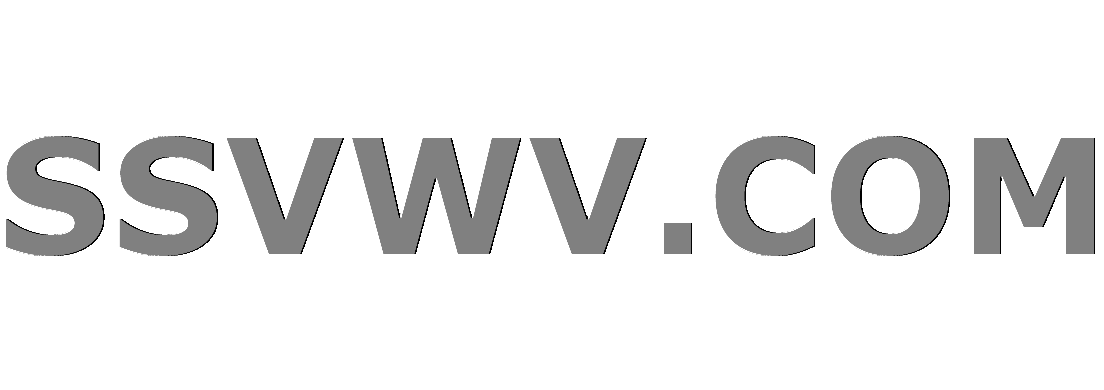
Multi tool use
I am bit stuck at understanding the relation b/w using viewmodel and livedata. I hope that someone can explain it for me. I am new to Android Development.

add a comment |
I am bit stuck at understanding the relation b/w using viewmodel and livedata. I hope that someone can explain it for me. I am new to Android Development.

add a comment |
I am bit stuck at understanding the relation b/w using viewmodel and livedata. I hope that someone can explain it for me. I am new to Android Development.

I am bit stuck at understanding the relation b/w using viewmodel and livedata. I hope that someone can explain it for me. I am new to Android Development.


asked Nov 14 '18 at 6:29


Abdul Rahman ShamairAbdul Rahman Shamair
3327
3327
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
For MVVM architecture it looks like this: you create model with your data, you access and change it in view model(in instances of LiveData). And observe it in views(activities/fragments).
MainViewModel extends ViewModel
MutableLiveData<String> someStringObject = new MutableLiveData<>;
private void someMethod
someStringObject.setValue("For main thread");
someStringObject.postValue("For back thread");
public MutableLiveData<String> getSomeStringObject()
return someStringObject;
FragmentA extends Fragment
@BindView(R.id.tv) //ButterKnife
TextView someTV;
private MainViewModel mainViewModel;
@Override
public View onCreateView(@NonNull LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState)
//getting viewModel
mainViewModel = ViewModelProviders.of(getActivity()).get(MainViewModel.class);
//registering observer
mainViewModel.getSomeStringObject.observe(this, value ->
someTV.setText(value);
);
This way you can react to changes of ViewModel in your View. Now if getSomeStringObject is changed in mainViewModel it will automatically change in FragmentA.
If there are multiple TextViews then there will that many observers,right? Also in "someMethod" , I dont understand the use of different threads,if you can then kindly explain. Thanks
– Abdul Rahman Shamair
Nov 20 '18 at 13:08
On main thread(default UI thread) use setValue, for non-main thread use postValue. About observers: if values of some textView depends on one source of data(can be array like MutableLiveData<ArrayList<Object>>) then you can create one observer. Otherwise make more. In my real project atm i have 5 observers in one fragment, one of them is observing some position(int value) from viewModel and changes 5 views(textViews, imageViews etc.).
– Andriy Tereshko
Nov 21 '18 at 15:06
add a comment |
It's all well explained here. The ViewModel purpose is to manipulate the data so to provide the data needed to the view, like in any normal mvp pattern. LiveData instead is the (lifecycle aware) callback for the view model, so that any time the data set is updated (so the model has a potential change in its state), the execution flow is brought back to the model, so that the model can update itself, for instance manipulate the new data set before to provide it to the view. I hope it's clear
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294303%2fcan-any-one-explain-how-to-use-viewmodel-and-livedata-while-implementing-mvvm-ar%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
For MVVM architecture it looks like this: you create model with your data, you access and change it in view model(in instances of LiveData). And observe it in views(activities/fragments).
MainViewModel extends ViewModel
MutableLiveData<String> someStringObject = new MutableLiveData<>;
private void someMethod
someStringObject.setValue("For main thread");
someStringObject.postValue("For back thread");
public MutableLiveData<String> getSomeStringObject()
return someStringObject;
FragmentA extends Fragment
@BindView(R.id.tv) //ButterKnife
TextView someTV;
private MainViewModel mainViewModel;
@Override
public View onCreateView(@NonNull LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState)
//getting viewModel
mainViewModel = ViewModelProviders.of(getActivity()).get(MainViewModel.class);
//registering observer
mainViewModel.getSomeStringObject.observe(this, value ->
someTV.setText(value);
);
This way you can react to changes of ViewModel in your View. Now if getSomeStringObject is changed in mainViewModel it will automatically change in FragmentA.
If there are multiple TextViews then there will that many observers,right? Also in "someMethod" , I dont understand the use of different threads,if you can then kindly explain. Thanks
– Abdul Rahman Shamair
Nov 20 '18 at 13:08
On main thread(default UI thread) use setValue, for non-main thread use postValue. About observers: if values of some textView depends on one source of data(can be array like MutableLiveData<ArrayList<Object>>) then you can create one observer. Otherwise make more. In my real project atm i have 5 observers in one fragment, one of them is observing some position(int value) from viewModel and changes 5 views(textViews, imageViews etc.).
– Andriy Tereshko
Nov 21 '18 at 15:06
add a comment |
For MVVM architecture it looks like this: you create model with your data, you access and change it in view model(in instances of LiveData). And observe it in views(activities/fragments).
MainViewModel extends ViewModel
MutableLiveData<String> someStringObject = new MutableLiveData<>;
private void someMethod
someStringObject.setValue("For main thread");
someStringObject.postValue("For back thread");
public MutableLiveData<String> getSomeStringObject()
return someStringObject;
FragmentA extends Fragment
@BindView(R.id.tv) //ButterKnife
TextView someTV;
private MainViewModel mainViewModel;
@Override
public View onCreateView(@NonNull LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState)
//getting viewModel
mainViewModel = ViewModelProviders.of(getActivity()).get(MainViewModel.class);
//registering observer
mainViewModel.getSomeStringObject.observe(this, value ->
someTV.setText(value);
);
This way you can react to changes of ViewModel in your View. Now if getSomeStringObject is changed in mainViewModel it will automatically change in FragmentA.
If there are multiple TextViews then there will that many observers,right? Also in "someMethod" , I dont understand the use of different threads,if you can then kindly explain. Thanks
– Abdul Rahman Shamair
Nov 20 '18 at 13:08
On main thread(default UI thread) use setValue, for non-main thread use postValue. About observers: if values of some textView depends on one source of data(can be array like MutableLiveData<ArrayList<Object>>) then you can create one observer. Otherwise make more. In my real project atm i have 5 observers in one fragment, one of them is observing some position(int value) from viewModel and changes 5 views(textViews, imageViews etc.).
– Andriy Tereshko
Nov 21 '18 at 15:06
add a comment |
For MVVM architecture it looks like this: you create model with your data, you access and change it in view model(in instances of LiveData). And observe it in views(activities/fragments).
MainViewModel extends ViewModel
MutableLiveData<String> someStringObject = new MutableLiveData<>;
private void someMethod
someStringObject.setValue("For main thread");
someStringObject.postValue("For back thread");
public MutableLiveData<String> getSomeStringObject()
return someStringObject;
FragmentA extends Fragment
@BindView(R.id.tv) //ButterKnife
TextView someTV;
private MainViewModel mainViewModel;
@Override
public View onCreateView(@NonNull LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState)
//getting viewModel
mainViewModel = ViewModelProviders.of(getActivity()).get(MainViewModel.class);
//registering observer
mainViewModel.getSomeStringObject.observe(this, value ->
someTV.setText(value);
);
This way you can react to changes of ViewModel in your View. Now if getSomeStringObject is changed in mainViewModel it will automatically change in FragmentA.
For MVVM architecture it looks like this: you create model with your data, you access and change it in view model(in instances of LiveData). And observe it in views(activities/fragments).
MainViewModel extends ViewModel
MutableLiveData<String> someStringObject = new MutableLiveData<>;
private void someMethod
someStringObject.setValue("For main thread");
someStringObject.postValue("For back thread");
public MutableLiveData<String> getSomeStringObject()
return someStringObject;
FragmentA extends Fragment
@BindView(R.id.tv) //ButterKnife
TextView someTV;
private MainViewModel mainViewModel;
@Override
public View onCreateView(@NonNull LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState)
//getting viewModel
mainViewModel = ViewModelProviders.of(getActivity()).get(MainViewModel.class);
//registering observer
mainViewModel.getSomeStringObject.observe(this, value ->
someTV.setText(value);
);
This way you can react to changes of ViewModel in your View. Now if getSomeStringObject is changed in mainViewModel it will automatically change in FragmentA.
answered Nov 16 '18 at 14:31


Andriy TereshkoAndriy Tereshko
562
562
If there are multiple TextViews then there will that many observers,right? Also in "someMethod" , I dont understand the use of different threads,if you can then kindly explain. Thanks
– Abdul Rahman Shamair
Nov 20 '18 at 13:08
On main thread(default UI thread) use setValue, for non-main thread use postValue. About observers: if values of some textView depends on one source of data(can be array like MutableLiveData<ArrayList<Object>>) then you can create one observer. Otherwise make more. In my real project atm i have 5 observers in one fragment, one of them is observing some position(int value) from viewModel and changes 5 views(textViews, imageViews etc.).
– Andriy Tereshko
Nov 21 '18 at 15:06
add a comment |
If there are multiple TextViews then there will that many observers,right? Also in "someMethod" , I dont understand the use of different threads,if you can then kindly explain. Thanks
– Abdul Rahman Shamair
Nov 20 '18 at 13:08
On main thread(default UI thread) use setValue, for non-main thread use postValue. About observers: if values of some textView depends on one source of data(can be array like MutableLiveData<ArrayList<Object>>) then you can create one observer. Otherwise make more. In my real project atm i have 5 observers in one fragment, one of them is observing some position(int value) from viewModel and changes 5 views(textViews, imageViews etc.).
– Andriy Tereshko
Nov 21 '18 at 15:06
If there are multiple TextViews then there will that many observers,right? Also in "someMethod" , I dont understand the use of different threads,if you can then kindly explain. Thanks
– Abdul Rahman Shamair
Nov 20 '18 at 13:08
If there are multiple TextViews then there will that many observers,right? Also in "someMethod" , I dont understand the use of different threads,if you can then kindly explain. Thanks
– Abdul Rahman Shamair
Nov 20 '18 at 13:08
On main thread(default UI thread) use setValue, for non-main thread use postValue. About observers: if values of some textView depends on one source of data(can be array like MutableLiveData<ArrayList<Object>>) then you can create one observer. Otherwise make more. In my real project atm i have 5 observers in one fragment, one of them is observing some position(int value) from viewModel and changes 5 views(textViews, imageViews etc.).
– Andriy Tereshko
Nov 21 '18 at 15:06
On main thread(default UI thread) use setValue, for non-main thread use postValue. About observers: if values of some textView depends on one source of data(can be array like MutableLiveData<ArrayList<Object>>) then you can create one observer. Otherwise make more. In my real project atm i have 5 observers in one fragment, one of them is observing some position(int value) from viewModel and changes 5 views(textViews, imageViews etc.).
– Andriy Tereshko
Nov 21 '18 at 15:06
add a comment |
It's all well explained here. The ViewModel purpose is to manipulate the data so to provide the data needed to the view, like in any normal mvp pattern. LiveData instead is the (lifecycle aware) callback for the view model, so that any time the data set is updated (so the model has a potential change in its state), the execution flow is brought back to the model, so that the model can update itself, for instance manipulate the new data set before to provide it to the view. I hope it's clear
add a comment |
It's all well explained here. The ViewModel purpose is to manipulate the data so to provide the data needed to the view, like in any normal mvp pattern. LiveData instead is the (lifecycle aware) callback for the view model, so that any time the data set is updated (so the model has a potential change in its state), the execution flow is brought back to the model, so that the model can update itself, for instance manipulate the new data set before to provide it to the view. I hope it's clear
add a comment |
It's all well explained here. The ViewModel purpose is to manipulate the data so to provide the data needed to the view, like in any normal mvp pattern. LiveData instead is the (lifecycle aware) callback for the view model, so that any time the data set is updated (so the model has a potential change in its state), the execution flow is brought back to the model, so that the model can update itself, for instance manipulate the new data set before to provide it to the view. I hope it's clear
It's all well explained here. The ViewModel purpose is to manipulate the data so to provide the data needed to the view, like in any normal mvp pattern. LiveData instead is the (lifecycle aware) callback for the view model, so that any time the data set is updated (so the model has a potential change in its state), the execution flow is brought back to the model, so that the model can update itself, for instance manipulate the new data set before to provide it to the view. I hope it's clear
answered Nov 14 '18 at 6:34


AlessioAlessio
1,981913
1,981913
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294303%2fcan-any-one-explain-how-to-use-viewmodel-and-livedata-while-implementing-mvvm-ar%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gdQ,VpYbw4I,pBQ