ReactJS and Express with Axios returning 404
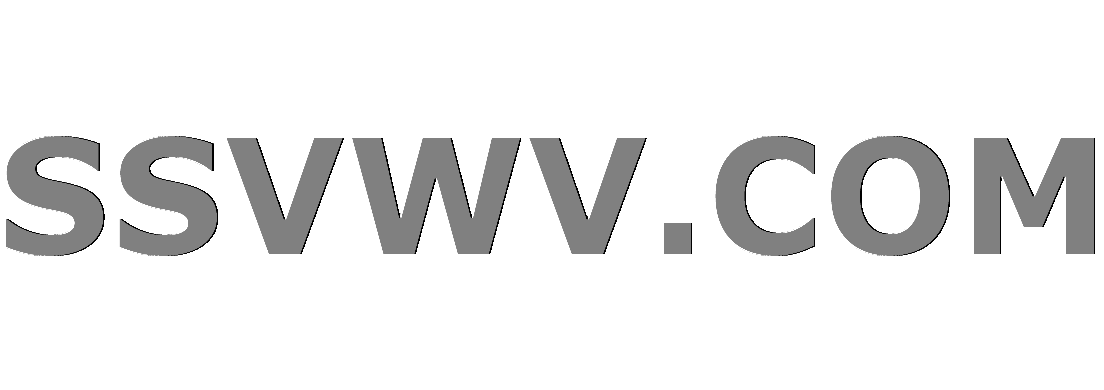
Multi tool use
Not sure what's going on with my code, but I'm trying to just do a test run with axios console logging something out, but I'm getting a 404.
class SubscribeForm extends Component
constructor(props)
super(props);
this.state = value: '';
this.emailChange = this.emailChange.bind(this);
this.emailSubmit = this.emailSubmit.bind(this);
emailChange(e)
this.setState(value: e.target.value);
emailSubmit(e)
e.preventDefault();
axios.post('/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
).then(res =>
if(res.data.success)
this.setState(email: '')
).catch(error =>
if (error.response)
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.log(error.response.data);
console.log(error.response.status);
console.log(error.response.headers);
else if (error.request)
// The request was made but no response was received
// `error.request` is an instance of XMLHttpRequest in the browser and an instance of
// http.ClientRequest in node.js
console.log(error.request);
else
// Something happened in setting up the request that triggered an Error
console.log('Error', error.message);
console.log(error.config);
);
render()
const classes = this.props;
return (
<div className=classes.subFormSection>
<p className=classes.formHelper>
Join the club, enter your email address
</p>
<form method="post" className=classes.subFormWrap onSubmit=this.emailSubmit>
<TextField
value = this.state.value
onChange = this.emailChange
/>
<Button
className=classes.button
type="submit"
>
Send
<Icon className=classes.rightIcon>send</Icon>
</Button>
</form>
</div>
);
I tried adding headers into axios to see if thats the issue, but i don't think it is (or perhaps I'm doing it wrong).
Also, I don't have a /subscribe file, I don't think I need one? Since, I'm capturing it with express here:
const cors = require("cors");
app.use(cors());
app.post("/subscribe", (req, res, next) =>
console.log(req.body.email);
);
This file is within my server files, while the react portion is within client files. I have proxy set up, so when I have run the server, front end and back end is talking to each other.
Updated 404 status turned (pending)
I also have set up proxy middleware in my client folder setupProxy.js
const proxy = require("http-proxy-middleware");
module.exports = function(app)
app.use(proxy("/subscribe",
target: "http://localhost:5000"
));
;
I'm getting in the request header in my network request for /subscrib
:
Provisional headers are shown
Is this causing it to be (pending)?
reactjs forms react-native react-redux axios
add a comment |
Not sure what's going on with my code, but I'm trying to just do a test run with axios console logging something out, but I'm getting a 404.
class SubscribeForm extends Component
constructor(props)
super(props);
this.state = value: '';
this.emailChange = this.emailChange.bind(this);
this.emailSubmit = this.emailSubmit.bind(this);
emailChange(e)
this.setState(value: e.target.value);
emailSubmit(e)
e.preventDefault();
axios.post('/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
).then(res =>
if(res.data.success)
this.setState(email: '')
).catch(error =>
if (error.response)
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.log(error.response.data);
console.log(error.response.status);
console.log(error.response.headers);
else if (error.request)
// The request was made but no response was received
// `error.request` is an instance of XMLHttpRequest in the browser and an instance of
// http.ClientRequest in node.js
console.log(error.request);
else
// Something happened in setting up the request that triggered an Error
console.log('Error', error.message);
console.log(error.config);
);
render()
const classes = this.props;
return (
<div className=classes.subFormSection>
<p className=classes.formHelper>
Join the club, enter your email address
</p>
<form method="post" className=classes.subFormWrap onSubmit=this.emailSubmit>
<TextField
value = this.state.value
onChange = this.emailChange
/>
<Button
className=classes.button
type="submit"
>
Send
<Icon className=classes.rightIcon>send</Icon>
</Button>
</form>
</div>
);
I tried adding headers into axios to see if thats the issue, but i don't think it is (or perhaps I'm doing it wrong).
Also, I don't have a /subscribe file, I don't think I need one? Since, I'm capturing it with express here:
const cors = require("cors");
app.use(cors());
app.post("/subscribe", (req, res, next) =>
console.log(req.body.email);
);
This file is within my server files, while the react portion is within client files. I have proxy set up, so when I have run the server, front end and back end is talking to each other.
Updated 404 status turned (pending)
I also have set up proxy middleware in my client folder setupProxy.js
const proxy = require("http-proxy-middleware");
module.exports = function(app)
app.use(proxy("/subscribe",
target: "http://localhost:5000"
));
;
I'm getting in the request header in my network request for /subscrib
:
Provisional headers are shown
Is this causing it to be (pending)?
reactjs forms react-native react-redux axios
add a comment |
Not sure what's going on with my code, but I'm trying to just do a test run with axios console logging something out, but I'm getting a 404.
class SubscribeForm extends Component
constructor(props)
super(props);
this.state = value: '';
this.emailChange = this.emailChange.bind(this);
this.emailSubmit = this.emailSubmit.bind(this);
emailChange(e)
this.setState(value: e.target.value);
emailSubmit(e)
e.preventDefault();
axios.post('/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
).then(res =>
if(res.data.success)
this.setState(email: '')
).catch(error =>
if (error.response)
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.log(error.response.data);
console.log(error.response.status);
console.log(error.response.headers);
else if (error.request)
// The request was made but no response was received
// `error.request` is an instance of XMLHttpRequest in the browser and an instance of
// http.ClientRequest in node.js
console.log(error.request);
else
// Something happened in setting up the request that triggered an Error
console.log('Error', error.message);
console.log(error.config);
);
render()
const classes = this.props;
return (
<div className=classes.subFormSection>
<p className=classes.formHelper>
Join the club, enter your email address
</p>
<form method="post" className=classes.subFormWrap onSubmit=this.emailSubmit>
<TextField
value = this.state.value
onChange = this.emailChange
/>
<Button
className=classes.button
type="submit"
>
Send
<Icon className=classes.rightIcon>send</Icon>
</Button>
</form>
</div>
);
I tried adding headers into axios to see if thats the issue, but i don't think it is (or perhaps I'm doing it wrong).
Also, I don't have a /subscribe file, I don't think I need one? Since, I'm capturing it with express here:
const cors = require("cors");
app.use(cors());
app.post("/subscribe", (req, res, next) =>
console.log(req.body.email);
);
This file is within my server files, while the react portion is within client files. I have proxy set up, so when I have run the server, front end and back end is talking to each other.
Updated 404 status turned (pending)
I also have set up proxy middleware in my client folder setupProxy.js
const proxy = require("http-proxy-middleware");
module.exports = function(app)
app.use(proxy("/subscribe",
target: "http://localhost:5000"
));
;
I'm getting in the request header in my network request for /subscrib
:
Provisional headers are shown
Is this causing it to be (pending)?
reactjs forms react-native react-redux axios
Not sure what's going on with my code, but I'm trying to just do a test run with axios console logging something out, but I'm getting a 404.
class SubscribeForm extends Component
constructor(props)
super(props);
this.state = value: '';
this.emailChange = this.emailChange.bind(this);
this.emailSubmit = this.emailSubmit.bind(this);
emailChange(e)
this.setState(value: e.target.value);
emailSubmit(e)
e.preventDefault();
axios.post('/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
).then(res =>
if(res.data.success)
this.setState(email: '')
).catch(error =>
if (error.response)
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.log(error.response.data);
console.log(error.response.status);
console.log(error.response.headers);
else if (error.request)
// The request was made but no response was received
// `error.request` is an instance of XMLHttpRequest in the browser and an instance of
// http.ClientRequest in node.js
console.log(error.request);
else
// Something happened in setting up the request that triggered an Error
console.log('Error', error.message);
console.log(error.config);
);
render()
const classes = this.props;
return (
<div className=classes.subFormSection>
<p className=classes.formHelper>
Join the club, enter your email address
</p>
<form method="post" className=classes.subFormWrap onSubmit=this.emailSubmit>
<TextField
value = this.state.value
onChange = this.emailChange
/>
<Button
className=classes.button
type="submit"
>
Send
<Icon className=classes.rightIcon>send</Icon>
</Button>
</form>
</div>
);
I tried adding headers into axios to see if thats the issue, but i don't think it is (or perhaps I'm doing it wrong).
Also, I don't have a /subscribe file, I don't think I need one? Since, I'm capturing it with express here:
const cors = require("cors");
app.use(cors());
app.post("/subscribe", (req, res, next) =>
console.log(req.body.email);
);
This file is within my server files, while the react portion is within client files. I have proxy set up, so when I have run the server, front end and back end is talking to each other.
Updated 404 status turned (pending)
I also have set up proxy middleware in my client folder setupProxy.js
const proxy = require("http-proxy-middleware");
module.exports = function(app)
app.use(proxy("/subscribe",
target: "http://localhost:5000"
));
;
I'm getting in the request header in my network request for /subscrib
:
Provisional headers are shown
Is this causing it to be (pending)?
reactjs forms react-native react-redux axios
reactjs forms react-native react-redux axios
edited Nov 14 '18 at 15:33
hellomello
asked Nov 14 '18 at 6:31


hellomellohellomello
3,10327102226
3,10327102226
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
From what I can see, it seem your server and client app run on different ports. If that is the case you cannot do this:
axios.post('/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
You are using the path /subscribe
without a host. Axios tries to use the host from which your client app is running and it doesn't find that path hence the 404
status code.
If this is the case, you can fix it by using the host and port of your server before the /subscribe
path. See below:
axios.post('localhost:4000/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
Kindly change the port here localhost:4000
to your server port.
Note: This is for your local development only.
If you plan on deploying the app, you might want to use process.env.PORT
instead.
Thanks, I set up an http-proxy-middleware, and I connected the route to my server's localhost port.
– hellomello
Nov 14 '18 at 7:07
Hey, I'm getting a status "pending" now. I know this isn't part of the question, but do you have any idea why its not giving me a correct status?
– hellomello
Nov 14 '18 at 7:16
Can you paste the code on the question?
– John Kennedy
Nov 14 '18 at 7:27
Hi, I updated my question with the proxy code. This helped me connect the server and client side, but now I'm getting an pending status on my /subscribe. I also haveProvisional headers are shown
in network request. could this be an issue?
– hellomello
Nov 14 '18 at 15:34
It seem more like you are not sending a response back to the client in that case it pends. You have justconsole.log(req.body.email)
in your/subscribe
route. Useres.send(...)
to send back a response.
– John Kennedy
Nov 15 '18 at 3:13
|
show 1 more comment
You can use browser's inspect element to check if request is made properly(Inspect Element > Network).
By looking at code I think you are missing base url.
it should be like
axios.post(baseul + port + request url)
Example:
axios.post('http://localhost:3000/subscribe')
Unfortunately, that's not the case. my error is calling that out:POST http://localhost:3000/subscribe 404 (Not Found)
– hellomello
Nov 14 '18 at 6:54
1
@hellomello error says localhost:3000 which means it is trying to connect with port 3000. If you're trying to connect with express on a different port, then make sure it's calling the correct port as well.
– Fawaz
Nov 14 '18 at 6:58
@Fawaz this comment made me realize that I had to setup a proxy especially for/subscribe
. I had already done so with other routes, but forgot I needed to set it up with this one! Thanks!
– hellomello
Nov 14 '18 at 7:05
@hellomello Happy it helped!
– Fawaz
Nov 14 '18 at 8:09
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294316%2freactjs-and-express-with-axios-returning-404%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
From what I can see, it seem your server and client app run on different ports. If that is the case you cannot do this:
axios.post('/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
You are using the path /subscribe
without a host. Axios tries to use the host from which your client app is running and it doesn't find that path hence the 404
status code.
If this is the case, you can fix it by using the host and port of your server before the /subscribe
path. See below:
axios.post('localhost:4000/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
Kindly change the port here localhost:4000
to your server port.
Note: This is for your local development only.
If you plan on deploying the app, you might want to use process.env.PORT
instead.
Thanks, I set up an http-proxy-middleware, and I connected the route to my server's localhost port.
– hellomello
Nov 14 '18 at 7:07
Hey, I'm getting a status "pending" now. I know this isn't part of the question, but do you have any idea why its not giving me a correct status?
– hellomello
Nov 14 '18 at 7:16
Can you paste the code on the question?
– John Kennedy
Nov 14 '18 at 7:27
Hi, I updated my question with the proxy code. This helped me connect the server and client side, but now I'm getting an pending status on my /subscribe. I also haveProvisional headers are shown
in network request. could this be an issue?
– hellomello
Nov 14 '18 at 15:34
It seem more like you are not sending a response back to the client in that case it pends. You have justconsole.log(req.body.email)
in your/subscribe
route. Useres.send(...)
to send back a response.
– John Kennedy
Nov 15 '18 at 3:13
|
show 1 more comment
From what I can see, it seem your server and client app run on different ports. If that is the case you cannot do this:
axios.post('/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
You are using the path /subscribe
without a host. Axios tries to use the host from which your client app is running and it doesn't find that path hence the 404
status code.
If this is the case, you can fix it by using the host and port of your server before the /subscribe
path. See below:
axios.post('localhost:4000/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
Kindly change the port here localhost:4000
to your server port.
Note: This is for your local development only.
If you plan on deploying the app, you might want to use process.env.PORT
instead.
Thanks, I set up an http-proxy-middleware, and I connected the route to my server's localhost port.
– hellomello
Nov 14 '18 at 7:07
Hey, I'm getting a status "pending" now. I know this isn't part of the question, but do you have any idea why its not giving me a correct status?
– hellomello
Nov 14 '18 at 7:16
Can you paste the code on the question?
– John Kennedy
Nov 14 '18 at 7:27
Hi, I updated my question with the proxy code. This helped me connect the server and client side, but now I'm getting an pending status on my /subscribe. I also haveProvisional headers are shown
in network request. could this be an issue?
– hellomello
Nov 14 '18 at 15:34
It seem more like you are not sending a response back to the client in that case it pends. You have justconsole.log(req.body.email)
in your/subscribe
route. Useres.send(...)
to send back a response.
– John Kennedy
Nov 15 '18 at 3:13
|
show 1 more comment
From what I can see, it seem your server and client app run on different ports. If that is the case you cannot do this:
axios.post('/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
You are using the path /subscribe
without a host. Axios tries to use the host from which your client app is running and it doesn't find that path hence the 404
status code.
If this is the case, you can fix it by using the host and port of your server before the /subscribe
path. See below:
axios.post('localhost:4000/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
Kindly change the port here localhost:4000
to your server port.
Note: This is for your local development only.
If you plan on deploying the app, you might want to use process.env.PORT
instead.
From what I can see, it seem your server and client app run on different ports. If that is the case you cannot do this:
axios.post('/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
You are using the path /subscribe
without a host. Axios tries to use the host from which your client app is running and it doesn't find that path hence the 404
status code.
If this is the case, you can fix it by using the host and port of your server before the /subscribe
path. See below:
axios.post('localhost:4000/subscribe',
email: 'someemail@email.com',
headers:
'Content-Type': 'application/json;charset=UTF-8',
"Access-Control-Allow-Origin": "*",
)
Kindly change the port here localhost:4000
to your server port.
Note: This is for your local development only.
If you plan on deploying the app, you might want to use process.env.PORT
instead.
answered Nov 14 '18 at 7:02


John KennedyJohn Kennedy
2,61321026
2,61321026
Thanks, I set up an http-proxy-middleware, and I connected the route to my server's localhost port.
– hellomello
Nov 14 '18 at 7:07
Hey, I'm getting a status "pending" now. I know this isn't part of the question, but do you have any idea why its not giving me a correct status?
– hellomello
Nov 14 '18 at 7:16
Can you paste the code on the question?
– John Kennedy
Nov 14 '18 at 7:27
Hi, I updated my question with the proxy code. This helped me connect the server and client side, but now I'm getting an pending status on my /subscribe. I also haveProvisional headers are shown
in network request. could this be an issue?
– hellomello
Nov 14 '18 at 15:34
It seem more like you are not sending a response back to the client in that case it pends. You have justconsole.log(req.body.email)
in your/subscribe
route. Useres.send(...)
to send back a response.
– John Kennedy
Nov 15 '18 at 3:13
|
show 1 more comment
Thanks, I set up an http-proxy-middleware, and I connected the route to my server's localhost port.
– hellomello
Nov 14 '18 at 7:07
Hey, I'm getting a status "pending" now. I know this isn't part of the question, but do you have any idea why its not giving me a correct status?
– hellomello
Nov 14 '18 at 7:16
Can you paste the code on the question?
– John Kennedy
Nov 14 '18 at 7:27
Hi, I updated my question with the proxy code. This helped me connect the server and client side, but now I'm getting an pending status on my /subscribe. I also haveProvisional headers are shown
in network request. could this be an issue?
– hellomello
Nov 14 '18 at 15:34
It seem more like you are not sending a response back to the client in that case it pends. You have justconsole.log(req.body.email)
in your/subscribe
route. Useres.send(...)
to send back a response.
– John Kennedy
Nov 15 '18 at 3:13
Thanks, I set up an http-proxy-middleware, and I connected the route to my server's localhost port.
– hellomello
Nov 14 '18 at 7:07
Thanks, I set up an http-proxy-middleware, and I connected the route to my server's localhost port.
– hellomello
Nov 14 '18 at 7:07
Hey, I'm getting a status "pending" now. I know this isn't part of the question, but do you have any idea why its not giving me a correct status?
– hellomello
Nov 14 '18 at 7:16
Hey, I'm getting a status "pending" now. I know this isn't part of the question, but do you have any idea why its not giving me a correct status?
– hellomello
Nov 14 '18 at 7:16
Can you paste the code on the question?
– John Kennedy
Nov 14 '18 at 7:27
Can you paste the code on the question?
– John Kennedy
Nov 14 '18 at 7:27
Hi, I updated my question with the proxy code. This helped me connect the server and client side, but now I'm getting an pending status on my /subscribe. I also have
Provisional headers are shown
in network request. could this be an issue?– hellomello
Nov 14 '18 at 15:34
Hi, I updated my question with the proxy code. This helped me connect the server and client side, but now I'm getting an pending status on my /subscribe. I also have
Provisional headers are shown
in network request. could this be an issue?– hellomello
Nov 14 '18 at 15:34
It seem more like you are not sending a response back to the client in that case it pends. You have just
console.log(req.body.email)
in your /subscribe
route. Use res.send(...)
to send back a response.– John Kennedy
Nov 15 '18 at 3:13
It seem more like you are not sending a response back to the client in that case it pends. You have just
console.log(req.body.email)
in your /subscribe
route. Use res.send(...)
to send back a response.– John Kennedy
Nov 15 '18 at 3:13
|
show 1 more comment
You can use browser's inspect element to check if request is made properly(Inspect Element > Network).
By looking at code I think you are missing base url.
it should be like
axios.post(baseul + port + request url)
Example:
axios.post('http://localhost:3000/subscribe')
Unfortunately, that's not the case. my error is calling that out:POST http://localhost:3000/subscribe 404 (Not Found)
– hellomello
Nov 14 '18 at 6:54
1
@hellomello error says localhost:3000 which means it is trying to connect with port 3000. If you're trying to connect with express on a different port, then make sure it's calling the correct port as well.
– Fawaz
Nov 14 '18 at 6:58
@Fawaz this comment made me realize that I had to setup a proxy especially for/subscribe
. I had already done so with other routes, but forgot I needed to set it up with this one! Thanks!
– hellomello
Nov 14 '18 at 7:05
@hellomello Happy it helped!
– Fawaz
Nov 14 '18 at 8:09
add a comment |
You can use browser's inspect element to check if request is made properly(Inspect Element > Network).
By looking at code I think you are missing base url.
it should be like
axios.post(baseul + port + request url)
Example:
axios.post('http://localhost:3000/subscribe')
Unfortunately, that's not the case. my error is calling that out:POST http://localhost:3000/subscribe 404 (Not Found)
– hellomello
Nov 14 '18 at 6:54
1
@hellomello error says localhost:3000 which means it is trying to connect with port 3000. If you're trying to connect with express on a different port, then make sure it's calling the correct port as well.
– Fawaz
Nov 14 '18 at 6:58
@Fawaz this comment made me realize that I had to setup a proxy especially for/subscribe
. I had already done so with other routes, but forgot I needed to set it up with this one! Thanks!
– hellomello
Nov 14 '18 at 7:05
@hellomello Happy it helped!
– Fawaz
Nov 14 '18 at 8:09
add a comment |
You can use browser's inspect element to check if request is made properly(Inspect Element > Network).
By looking at code I think you are missing base url.
it should be like
axios.post(baseul + port + request url)
Example:
axios.post('http://localhost:3000/subscribe')
You can use browser's inspect element to check if request is made properly(Inspect Element > Network).
By looking at code I think you are missing base url.
it should be like
axios.post(baseul + port + request url)
Example:
axios.post('http://localhost:3000/subscribe')
edited Nov 14 '18 at 7:08
Supermacy
382210
382210
answered Nov 14 '18 at 6:52
SagarSagar
465210
465210
Unfortunately, that's not the case. my error is calling that out:POST http://localhost:3000/subscribe 404 (Not Found)
– hellomello
Nov 14 '18 at 6:54
1
@hellomello error says localhost:3000 which means it is trying to connect with port 3000. If you're trying to connect with express on a different port, then make sure it's calling the correct port as well.
– Fawaz
Nov 14 '18 at 6:58
@Fawaz this comment made me realize that I had to setup a proxy especially for/subscribe
. I had already done so with other routes, but forgot I needed to set it up with this one! Thanks!
– hellomello
Nov 14 '18 at 7:05
@hellomello Happy it helped!
– Fawaz
Nov 14 '18 at 8:09
add a comment |
Unfortunately, that's not the case. my error is calling that out:POST http://localhost:3000/subscribe 404 (Not Found)
– hellomello
Nov 14 '18 at 6:54
1
@hellomello error says localhost:3000 which means it is trying to connect with port 3000. If you're trying to connect with express on a different port, then make sure it's calling the correct port as well.
– Fawaz
Nov 14 '18 at 6:58
@Fawaz this comment made me realize that I had to setup a proxy especially for/subscribe
. I had already done so with other routes, but forgot I needed to set it up with this one! Thanks!
– hellomello
Nov 14 '18 at 7:05
@hellomello Happy it helped!
– Fawaz
Nov 14 '18 at 8:09
Unfortunately, that's not the case. my error is calling that out:
POST http://localhost:3000/subscribe 404 (Not Found)
– hellomello
Nov 14 '18 at 6:54
Unfortunately, that's not the case. my error is calling that out:
POST http://localhost:3000/subscribe 404 (Not Found)
– hellomello
Nov 14 '18 at 6:54
1
1
@hellomello error says localhost:3000 which means it is trying to connect with port 3000. If you're trying to connect with express on a different port, then make sure it's calling the correct port as well.
– Fawaz
Nov 14 '18 at 6:58
@hellomello error says localhost:3000 which means it is trying to connect with port 3000. If you're trying to connect with express on a different port, then make sure it's calling the correct port as well.
– Fawaz
Nov 14 '18 at 6:58
@Fawaz this comment made me realize that I had to setup a proxy especially for
/subscribe
. I had already done so with other routes, but forgot I needed to set it up with this one! Thanks!– hellomello
Nov 14 '18 at 7:05
@Fawaz this comment made me realize that I had to setup a proxy especially for
/subscribe
. I had already done so with other routes, but forgot I needed to set it up with this one! Thanks!– hellomello
Nov 14 '18 at 7:05
@hellomello Happy it helped!
– Fawaz
Nov 14 '18 at 8:09
@hellomello Happy it helped!
– Fawaz
Nov 14 '18 at 8:09
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294316%2freactjs-and-express-with-axios-returning-404%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
go,ndheEa3QyK yEC,Mq4zHVjPVrfs,MqjgiaGgs,sgcQj,C8L0