AVOID DUPLICATES in sequelize query
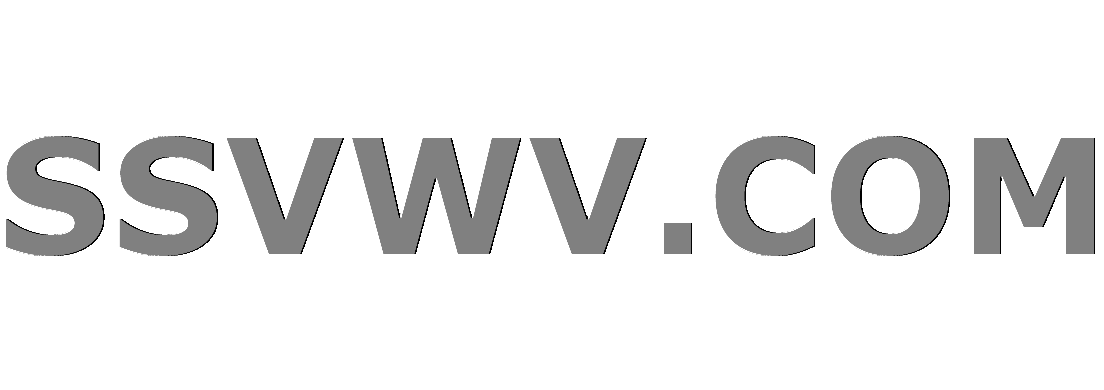
Multi tool use
i'm implementing a like system for a project. And I need some help with a query.
Basically i have 2 buttons (upvote and downvote) that call my function and give the id of a thread, the username voting, and the vote ( 1 or -1).
addPositiveorNegativeLikes = function(thread_id, username, vote)
sequelize.query('INSERT INTO Likes (thread_id, userId, vote, createdAt, updatedAt)
VALUES((?), (SELECT id FROM Users WHERE username=(?)), (?), (?), (?))
ON DUPLICATE KEY UPDATE thread_id=(?), userId=(SELECT id FROM Users WHERE username=(?))',
replacements: [thread_id, username, vote, new Date(), new Date(), thread_id, username]
)
But now in my Likes table althought thread_id and userId ara both primary keys, inserts multiple repeated "Likes".
How I can modify my query so it deletes an existing vote and replaces it for a new one??
Here is my Like model:
'use strict';
module.exports = (sequelize, DataTypes) =>
const Like = sequelize.define('Like',
id:
allowNull: false,
autoIncrement: true,
primaryKey: true,
type: DataTypes.INTEGER
,
userId:
allowNull: false,
primaryKey: true,
type: DataTypes.INTEGER
,
thread_id:
allowNull: false,
primaryKey: true,
type: DataTypes.INTEGER
,
createdAt:
allowNull: false,
type: DataTypes.DATE
,
updatedAt:
allowNull: false,
type: DataTypes.DATE
,
vote:
type: DataTypes.INTEGER
, );
Like.associate = function(models)
// associations can be defined here
;
return Like;
;
mysql database express sequelize.js
add a comment |
i'm implementing a like system for a project. And I need some help with a query.
Basically i have 2 buttons (upvote and downvote) that call my function and give the id of a thread, the username voting, and the vote ( 1 or -1).
addPositiveorNegativeLikes = function(thread_id, username, vote)
sequelize.query('INSERT INTO Likes (thread_id, userId, vote, createdAt, updatedAt)
VALUES((?), (SELECT id FROM Users WHERE username=(?)), (?), (?), (?))
ON DUPLICATE KEY UPDATE thread_id=(?), userId=(SELECT id FROM Users WHERE username=(?))',
replacements: [thread_id, username, vote, new Date(), new Date(), thread_id, username]
)
But now in my Likes table althought thread_id and userId ara both primary keys, inserts multiple repeated "Likes".
How I can modify my query so it deletes an existing vote and replaces it for a new one??
Here is my Like model:
'use strict';
module.exports = (sequelize, DataTypes) =>
const Like = sequelize.define('Like',
id:
allowNull: false,
autoIncrement: true,
primaryKey: true,
type: DataTypes.INTEGER
,
userId:
allowNull: false,
primaryKey: true,
type: DataTypes.INTEGER
,
thread_id:
allowNull: false,
primaryKey: true,
type: DataTypes.INTEGER
,
createdAt:
allowNull: false,
type: DataTypes.DATE
,
updatedAt:
allowNull: false,
type: DataTypes.DATE
,
vote:
type: DataTypes.INTEGER
, );
Like.associate = function(models)
// associations can be defined here
;
return Like;
;
mysql database express sequelize.js
add a comment |
i'm implementing a like system for a project. And I need some help with a query.
Basically i have 2 buttons (upvote and downvote) that call my function and give the id of a thread, the username voting, and the vote ( 1 or -1).
addPositiveorNegativeLikes = function(thread_id, username, vote)
sequelize.query('INSERT INTO Likes (thread_id, userId, vote, createdAt, updatedAt)
VALUES((?), (SELECT id FROM Users WHERE username=(?)), (?), (?), (?))
ON DUPLICATE KEY UPDATE thread_id=(?), userId=(SELECT id FROM Users WHERE username=(?))',
replacements: [thread_id, username, vote, new Date(), new Date(), thread_id, username]
)
But now in my Likes table althought thread_id and userId ara both primary keys, inserts multiple repeated "Likes".
How I can modify my query so it deletes an existing vote and replaces it for a new one??
Here is my Like model:
'use strict';
module.exports = (sequelize, DataTypes) =>
const Like = sequelize.define('Like',
id:
allowNull: false,
autoIncrement: true,
primaryKey: true,
type: DataTypes.INTEGER
,
userId:
allowNull: false,
primaryKey: true,
type: DataTypes.INTEGER
,
thread_id:
allowNull: false,
primaryKey: true,
type: DataTypes.INTEGER
,
createdAt:
allowNull: false,
type: DataTypes.DATE
,
updatedAt:
allowNull: false,
type: DataTypes.DATE
,
vote:
type: DataTypes.INTEGER
, );
Like.associate = function(models)
// associations can be defined here
;
return Like;
;
mysql database express sequelize.js
i'm implementing a like system for a project. And I need some help with a query.
Basically i have 2 buttons (upvote and downvote) that call my function and give the id of a thread, the username voting, and the vote ( 1 or -1).
addPositiveorNegativeLikes = function(thread_id, username, vote)
sequelize.query('INSERT INTO Likes (thread_id, userId, vote, createdAt, updatedAt)
VALUES((?), (SELECT id FROM Users WHERE username=(?)), (?), (?), (?))
ON DUPLICATE KEY UPDATE thread_id=(?), userId=(SELECT id FROM Users WHERE username=(?))',
replacements: [thread_id, username, vote, new Date(), new Date(), thread_id, username]
)
But now in my Likes table althought thread_id and userId ara both primary keys, inserts multiple repeated "Likes".
How I can modify my query so it deletes an existing vote and replaces it for a new one??
Here is my Like model:
'use strict';
module.exports = (sequelize, DataTypes) =>
const Like = sequelize.define('Like',
id:
allowNull: false,
autoIncrement: true,
primaryKey: true,
type: DataTypes.INTEGER
,
userId:
allowNull: false,
primaryKey: true,
type: DataTypes.INTEGER
,
thread_id:
allowNull: false,
primaryKey: true,
type: DataTypes.INTEGER
,
createdAt:
allowNull: false,
type: DataTypes.DATE
,
updatedAt:
allowNull: false,
type: DataTypes.DATE
,
vote:
type: DataTypes.INTEGER
, );
Like.associate = function(models)
// associations can be defined here
;
return Like;
;
mysql database express sequelize.js
mysql database express sequelize.js
asked Nov 13 '18 at 13:13


Arnau MartínezArnau Martínez
82
82
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Here , what you can do is , create a composite key , with this
userId:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
thread_id:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
NOTE :
// Creating two objects with the same value will throw an error. The unique property can be either a
// boolean, or a string. If you provide the same string for multiple columns, they will form a
// composite unique key.
uniqueOne: type: Sequelize.STRING, unique: 'compositeIndex' ,
uniqueTwo: type: Sequelize.INTEGER, unique: 'compositeIndex' ,
And then create it like :
Like.create( userId : 1 , thread_id : 1 ).then(data =>
// success
).catch(err =>
// error if same data exists
)
// <--- this will check that if there any entry with userId 1 and thread_id 1 ,
// if yes , then this will throw error
// if no then will create an entry for that
Note :
Never never run raw query , like you have did in your code sample , always use the model to perform CRUD , this way you can utilize all
the features of sequelizejs
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53281798%2favoid-duplicates-in-sequelize-query%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here , what you can do is , create a composite key , with this
userId:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
thread_id:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
NOTE :
// Creating two objects with the same value will throw an error. The unique property can be either a
// boolean, or a string. If you provide the same string for multiple columns, they will form a
// composite unique key.
uniqueOne: type: Sequelize.STRING, unique: 'compositeIndex' ,
uniqueTwo: type: Sequelize.INTEGER, unique: 'compositeIndex' ,
And then create it like :
Like.create( userId : 1 , thread_id : 1 ).then(data =>
// success
).catch(err =>
// error if same data exists
)
// <--- this will check that if there any entry with userId 1 and thread_id 1 ,
// if yes , then this will throw error
// if no then will create an entry for that
Note :
Never never run raw query , like you have did in your code sample , always use the model to perform CRUD , this way you can utilize all
the features of sequelizejs
add a comment |
Here , what you can do is , create a composite key , with this
userId:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
thread_id:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
NOTE :
// Creating two objects with the same value will throw an error. The unique property can be either a
// boolean, or a string. If you provide the same string for multiple columns, they will form a
// composite unique key.
uniqueOne: type: Sequelize.STRING, unique: 'compositeIndex' ,
uniqueTwo: type: Sequelize.INTEGER, unique: 'compositeIndex' ,
And then create it like :
Like.create( userId : 1 , thread_id : 1 ).then(data =>
// success
).catch(err =>
// error if same data exists
)
// <--- this will check that if there any entry with userId 1 and thread_id 1 ,
// if yes , then this will throw error
// if no then will create an entry for that
Note :
Never never run raw query , like you have did in your code sample , always use the model to perform CRUD , this way you can utilize all
the features of sequelizejs
add a comment |
Here , what you can do is , create a composite key , with this
userId:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
thread_id:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
NOTE :
// Creating two objects with the same value will throw an error. The unique property can be either a
// boolean, or a string. If you provide the same string for multiple columns, they will form a
// composite unique key.
uniqueOne: type: Sequelize.STRING, unique: 'compositeIndex' ,
uniqueTwo: type: Sequelize.INTEGER, unique: 'compositeIndex' ,
And then create it like :
Like.create( userId : 1 , thread_id : 1 ).then(data =>
// success
).catch(err =>
// error if same data exists
)
// <--- this will check that if there any entry with userId 1 and thread_id 1 ,
// if yes , then this will throw error
// if no then will create an entry for that
Note :
Never never run raw query , like you have did in your code sample , always use the model to perform CRUD , this way you can utilize all
the features of sequelizejs
Here , what you can do is , create a composite key , with this
userId:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
thread_id:
allowNull: false,
unique:"vote_user" // <------ HERE
type: DataTypes.INTEGER
,
NOTE :
// Creating two objects with the same value will throw an error. The unique property can be either a
// boolean, or a string. If you provide the same string for multiple columns, they will form a
// composite unique key.
uniqueOne: type: Sequelize.STRING, unique: 'compositeIndex' ,
uniqueTwo: type: Sequelize.INTEGER, unique: 'compositeIndex' ,
And then create it like :
Like.create( userId : 1 , thread_id : 1 ).then(data =>
// success
).catch(err =>
// error if same data exists
)
// <--- this will check that if there any entry with userId 1 and thread_id 1 ,
// if yes , then this will throw error
// if no then will create an entry for that
Note :
Never never run raw query , like you have did in your code sample , always use the model to perform CRUD , this way you can utilize all
the features of sequelizejs
edited Nov 13 '18 at 14:57
answered Nov 13 '18 at 14:51


Vivek DoshiVivek Doshi
20.6k22951
20.6k22951
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53281798%2favoid-duplicates-in-sequelize-query%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XftFL,0RSEK 51C,J0EiuwfWbTsrvxv9S U79 C8T BF6hL7k,l99faTg9