how to use an attribute of an entity that has a manytomany relationship with another entity in symfony
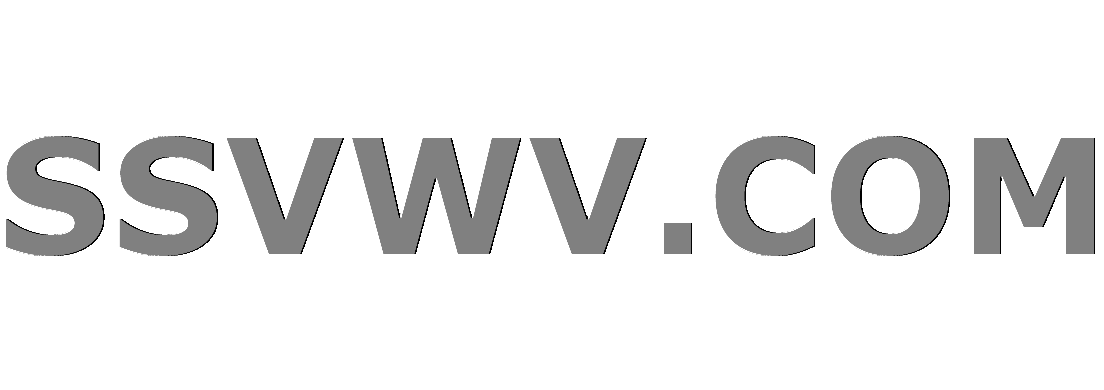
Multi tool use
i have a relation ManyToMany between two entities Doctor and insurance.
I configured my annotation ManyToMany in the entity Doctor, and in my table, Doctrine has created another separate doctor_insurance table.
now I would like to use the image attribute of an insurance to which the doctor is affiliated in my twig, but I do not know how to use this attribute
Entity Doctor
/**
* @ORMManyToMany(targetEntity="DoctixMedecinBundleEntityAssurance", cascade="persist", "remove")
* @ORMJoinColumn(nullable=true)
*/
private $assurance;
public function __construct()
$this->assurance = new ArrayCollection();
/**
* Add assurance
*
* @param DoctixMedecinBundleEntityAssurance $assurance
*
* @return Medecin
*/
public function addAssurance(DoctixMedecinBundleEntityAssurance $assurance)
$this->assurance = $assurance;
return $this;
/**
* Remove assurance
*
* @param DoctixMedecinBundleEntityAssurance $assurance
*/
public function removeAssurance(DoctixMedecinBundleEntityAssurance $assurance)
$this->assurance->removeElement($assurance);
/**
* Get assurance
*
* @return DoctrineCommonCollectionsCollection
*/
public function getAssurance()
return $this->assurance;
Entity Assurance
<?php
namespace DoctixMedecinBundleEntity;
use DoctrineORMMapping as ORM;
/**
* Assurance
*
* @ORMTable(name="assurance")
*
*/
class Assurance
/**
* @var int
*
* @ORMColumn(name="id", type="integer")
* @ORMId
* @ORMGeneratedValue(strategy="AUTO")
*/
private $id;
/**
* @var string
*
* @ORMColumn(name="nom", type="string", length=40)
*/
public $nom;
/**
* @ORMOneToOne(targetEntity="DoctixMedecinBundleEntityLogo", cascade="persist","remove","refresh")
* @ORMJoinColumn(nullable=true)
*/
private $logo;
/**
* Get id
*
* @return integer
*/
public function getId()
return $this->id;
/**
* Set nom
*
* @param string $nom
*
* @return Assurance
*/
public function setNom($nom)
$this->nom = $nom;
return $this;
/**
* Get nom
*
* @return string
*/
public function getNom()
return $this->nom;
/**
* Set logo
*
* @param DoctixMedecinBundleEntityMedia $logo
*
* @return Assurance
*/
public function setLogo(DoctixMedecinBundleEntityMedia $logo = null)
$this->logo = $logo;
return $this;
/**
* Get logo
*
* @return DoctixMedecinBundleEntityMedia
*/
public function getLogo()
return $this->logo;
Controller
public function parametreAction(Request $request)
$em = $this->getDoctrine()->getManager();
$repo = $em->getRepository('DoctixMedecinBundle:Medecin');
$medecin = $repo->findOneBy(array(
'user' => $this->getUser(),
));
$medecin->getAssurance();
return $this->render('DoctixMedecinBundle:Medecin:parametre.html.twig', array(
'medecin' => $medecin
));
Twig
<div class = "col-md-2">
<div class="box_list photo-medecin">
<figure>
<img src=" vich_uploader_asset(medecin.assurance, 'logoFile')
" class="img-fluid" alt="">
</figure>
</div>
Thanks
symfony many-to-many
add a comment |
i have a relation ManyToMany between two entities Doctor and insurance.
I configured my annotation ManyToMany in the entity Doctor, and in my table, Doctrine has created another separate doctor_insurance table.
now I would like to use the image attribute of an insurance to which the doctor is affiliated in my twig, but I do not know how to use this attribute
Entity Doctor
/**
* @ORMManyToMany(targetEntity="DoctixMedecinBundleEntityAssurance", cascade="persist", "remove")
* @ORMJoinColumn(nullable=true)
*/
private $assurance;
public function __construct()
$this->assurance = new ArrayCollection();
/**
* Add assurance
*
* @param DoctixMedecinBundleEntityAssurance $assurance
*
* @return Medecin
*/
public function addAssurance(DoctixMedecinBundleEntityAssurance $assurance)
$this->assurance = $assurance;
return $this;
/**
* Remove assurance
*
* @param DoctixMedecinBundleEntityAssurance $assurance
*/
public function removeAssurance(DoctixMedecinBundleEntityAssurance $assurance)
$this->assurance->removeElement($assurance);
/**
* Get assurance
*
* @return DoctrineCommonCollectionsCollection
*/
public function getAssurance()
return $this->assurance;
Entity Assurance
<?php
namespace DoctixMedecinBundleEntity;
use DoctrineORMMapping as ORM;
/**
* Assurance
*
* @ORMTable(name="assurance")
*
*/
class Assurance
/**
* @var int
*
* @ORMColumn(name="id", type="integer")
* @ORMId
* @ORMGeneratedValue(strategy="AUTO")
*/
private $id;
/**
* @var string
*
* @ORMColumn(name="nom", type="string", length=40)
*/
public $nom;
/**
* @ORMOneToOne(targetEntity="DoctixMedecinBundleEntityLogo", cascade="persist","remove","refresh")
* @ORMJoinColumn(nullable=true)
*/
private $logo;
/**
* Get id
*
* @return integer
*/
public function getId()
return $this->id;
/**
* Set nom
*
* @param string $nom
*
* @return Assurance
*/
public function setNom($nom)
$this->nom = $nom;
return $this;
/**
* Get nom
*
* @return string
*/
public function getNom()
return $this->nom;
/**
* Set logo
*
* @param DoctixMedecinBundleEntityMedia $logo
*
* @return Assurance
*/
public function setLogo(DoctixMedecinBundleEntityMedia $logo = null)
$this->logo = $logo;
return $this;
/**
* Get logo
*
* @return DoctixMedecinBundleEntityMedia
*/
public function getLogo()
return $this->logo;
Controller
public function parametreAction(Request $request)
$em = $this->getDoctrine()->getManager();
$repo = $em->getRepository('DoctixMedecinBundle:Medecin');
$medecin = $repo->findOneBy(array(
'user' => $this->getUser(),
));
$medecin->getAssurance();
return $this->render('DoctixMedecinBundle:Medecin:parametre.html.twig', array(
'medecin' => $medecin
));
Twig
<div class = "col-md-2">
<div class="box_list photo-medecin">
<figure>
<img src=" vich_uploader_asset(medecin.assurance, 'logoFile')
" class="img-fluid" alt="">
</figure>
</div>
Thanks
symfony many-to-many
add a comment |
i have a relation ManyToMany between two entities Doctor and insurance.
I configured my annotation ManyToMany in the entity Doctor, and in my table, Doctrine has created another separate doctor_insurance table.
now I would like to use the image attribute of an insurance to which the doctor is affiliated in my twig, but I do not know how to use this attribute
Entity Doctor
/**
* @ORMManyToMany(targetEntity="DoctixMedecinBundleEntityAssurance", cascade="persist", "remove")
* @ORMJoinColumn(nullable=true)
*/
private $assurance;
public function __construct()
$this->assurance = new ArrayCollection();
/**
* Add assurance
*
* @param DoctixMedecinBundleEntityAssurance $assurance
*
* @return Medecin
*/
public function addAssurance(DoctixMedecinBundleEntityAssurance $assurance)
$this->assurance = $assurance;
return $this;
/**
* Remove assurance
*
* @param DoctixMedecinBundleEntityAssurance $assurance
*/
public function removeAssurance(DoctixMedecinBundleEntityAssurance $assurance)
$this->assurance->removeElement($assurance);
/**
* Get assurance
*
* @return DoctrineCommonCollectionsCollection
*/
public function getAssurance()
return $this->assurance;
Entity Assurance
<?php
namespace DoctixMedecinBundleEntity;
use DoctrineORMMapping as ORM;
/**
* Assurance
*
* @ORMTable(name="assurance")
*
*/
class Assurance
/**
* @var int
*
* @ORMColumn(name="id", type="integer")
* @ORMId
* @ORMGeneratedValue(strategy="AUTO")
*/
private $id;
/**
* @var string
*
* @ORMColumn(name="nom", type="string", length=40)
*/
public $nom;
/**
* @ORMOneToOne(targetEntity="DoctixMedecinBundleEntityLogo", cascade="persist","remove","refresh")
* @ORMJoinColumn(nullable=true)
*/
private $logo;
/**
* Get id
*
* @return integer
*/
public function getId()
return $this->id;
/**
* Set nom
*
* @param string $nom
*
* @return Assurance
*/
public function setNom($nom)
$this->nom = $nom;
return $this;
/**
* Get nom
*
* @return string
*/
public function getNom()
return $this->nom;
/**
* Set logo
*
* @param DoctixMedecinBundleEntityMedia $logo
*
* @return Assurance
*/
public function setLogo(DoctixMedecinBundleEntityMedia $logo = null)
$this->logo = $logo;
return $this;
/**
* Get logo
*
* @return DoctixMedecinBundleEntityMedia
*/
public function getLogo()
return $this->logo;
Controller
public function parametreAction(Request $request)
$em = $this->getDoctrine()->getManager();
$repo = $em->getRepository('DoctixMedecinBundle:Medecin');
$medecin = $repo->findOneBy(array(
'user' => $this->getUser(),
));
$medecin->getAssurance();
return $this->render('DoctixMedecinBundle:Medecin:parametre.html.twig', array(
'medecin' => $medecin
));
Twig
<div class = "col-md-2">
<div class="box_list photo-medecin">
<figure>
<img src=" vich_uploader_asset(medecin.assurance, 'logoFile')
" class="img-fluid" alt="">
</figure>
</div>
Thanks
symfony many-to-many
i have a relation ManyToMany between two entities Doctor and insurance.
I configured my annotation ManyToMany in the entity Doctor, and in my table, Doctrine has created another separate doctor_insurance table.
now I would like to use the image attribute of an insurance to which the doctor is affiliated in my twig, but I do not know how to use this attribute
Entity Doctor
/**
* @ORMManyToMany(targetEntity="DoctixMedecinBundleEntityAssurance", cascade="persist", "remove")
* @ORMJoinColumn(nullable=true)
*/
private $assurance;
public function __construct()
$this->assurance = new ArrayCollection();
/**
* Add assurance
*
* @param DoctixMedecinBundleEntityAssurance $assurance
*
* @return Medecin
*/
public function addAssurance(DoctixMedecinBundleEntityAssurance $assurance)
$this->assurance = $assurance;
return $this;
/**
* Remove assurance
*
* @param DoctixMedecinBundleEntityAssurance $assurance
*/
public function removeAssurance(DoctixMedecinBundleEntityAssurance $assurance)
$this->assurance->removeElement($assurance);
/**
* Get assurance
*
* @return DoctrineCommonCollectionsCollection
*/
public function getAssurance()
return $this->assurance;
Entity Assurance
<?php
namespace DoctixMedecinBundleEntity;
use DoctrineORMMapping as ORM;
/**
* Assurance
*
* @ORMTable(name="assurance")
*
*/
class Assurance
/**
* @var int
*
* @ORMColumn(name="id", type="integer")
* @ORMId
* @ORMGeneratedValue(strategy="AUTO")
*/
private $id;
/**
* @var string
*
* @ORMColumn(name="nom", type="string", length=40)
*/
public $nom;
/**
* @ORMOneToOne(targetEntity="DoctixMedecinBundleEntityLogo", cascade="persist","remove","refresh")
* @ORMJoinColumn(nullable=true)
*/
private $logo;
/**
* Get id
*
* @return integer
*/
public function getId()
return $this->id;
/**
* Set nom
*
* @param string $nom
*
* @return Assurance
*/
public function setNom($nom)
$this->nom = $nom;
return $this;
/**
* Get nom
*
* @return string
*/
public function getNom()
return $this->nom;
/**
* Set logo
*
* @param DoctixMedecinBundleEntityMedia $logo
*
* @return Assurance
*/
public function setLogo(DoctixMedecinBundleEntityMedia $logo = null)
$this->logo = $logo;
return $this;
/**
* Get logo
*
* @return DoctixMedecinBundleEntityMedia
*/
public function getLogo()
return $this->logo;
Controller
public function parametreAction(Request $request)
$em = $this->getDoctrine()->getManager();
$repo = $em->getRepository('DoctixMedecinBundle:Medecin');
$medecin = $repo->findOneBy(array(
'user' => $this->getUser(),
));
$medecin->getAssurance();
return $this->render('DoctixMedecinBundle:Medecin:parametre.html.twig', array(
'medecin' => $medecin
));
Twig
<div class = "col-md-2">
<div class="box_list photo-medecin">
<figure>
<img src=" vich_uploader_asset(medecin.assurance, 'logoFile')
" class="img-fluid" alt="">
</figure>
</div>
Thanks
symfony many-to-many
symfony many-to-many
asked Nov 13 '18 at 16:30


Mohamed SackoMohamed Sacko
499
499
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Assurance means Guarantee / To be sure, So It is more likely you are after Insurance which is protection against a possible eventuality.
Also you called the first entity Doctor, but used it as Medicine, so please change this if doctor is medicine.
You need to revise your entities as they are completely wrong:
Doctor
use DoctixMedecinBundleEntityInsurance;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Doctor
// ...
/**
* @ORMManyToMany(targetEntity="Insurance", mappedBy="doctors" cascade="persist", "remove")
* @ORMJoinColumn(nullable=true)
*/
private $insurances;
public function __construct()
$this->insurances = new ArrayCollection();
public function addInsurance(Insurance $insurance)
if (!$this->insurances->contains($insurance))
$this->insurances->add($insurance);
$insurance->addDoctor($this);
public function removeInsurance(Insurance $insurance)
if ($this->insurances->contains($insurance))
$this->insurances->removeElement($insurance);
$insurance->removeDoctor($this);
/**
* @return Collection
*/
public function getInsurances()
return $this->insurances;
// ...
Insurance
use DoctixMedecinBundleEntityDoctor;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Insurance
// ...
/**
* @ORMManyToMany(targetEntity="Doctor", inversedBy="insurances")
* @ORMJoinColumn(nullable=true)
*/
private $doctors;
public function __construct()
$this->doctors = new ArrayCollection();
public function addDoctor(Doctor $doctor)
if (!$this->doctors->contains($doctor))
$this->doctors->add($doctor);
$doctor->addInsurance($this);
public function removeDoctor(Doctor $doctor)
if ($this->doctors->contains($doctor))
$this->doctors->removeElement($doctor);
$doctor->removeInsurance($this);
/**
* @return Collection
*/
public function getDoctors()
return $this->doctors;
// ...
Update
Controller
public function parametreAction(Request $request)
$em = $this->getDoctrine()->getManager();
$doctor = $em->getRepository('DoctixMedecinBundle:Medecin')->findOneBy(array(
'user' => $this->getUser(),
));
return $this->render('DoctixMedecinBundle:Medecin:parametre.html.twig', array(
'doctor' => $doctor
));
Twig
<div class="col-md-2">
<div class="box_list photo-medecin">
% for insurance in doctor.insurances %
<figure>
<img src=" vich_uploader_asset(insurance.logo, 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
</div>
Hi @Trix, I started from the premise that a doctor can be subscribed to 1 or more insurances for his clinic.And a given insurance ensures 1 or more doctors.So if your entity is correct how can I access the image of an insurance in my twig file?
– Mohamed Sacko
Nov 14 '18 at 11:00
so what do you mean by that medecin?
– Trix
Nov 14 '18 at 11:07
excuse me for my english. I speak french. In french medecin is a doctor in english.
– Mohamed Sacko
Nov 14 '18 at 11:46
Check the Update, and please consider accepting & up-voting, if it was helpful
– Trix
Nov 14 '18 at 11:59
add a comment |
<div class = "col-md-2">
% for item in medecin.assurance %
<div class="box_list photo-medecin">
<figure>
<img src=" vich_uploader_asset(item.logo , 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53285470%2fhow-to-use-an-attribute-of-an-entity-that-has-a-manytomany-relationship-with-ano%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Assurance means Guarantee / To be sure, So It is more likely you are after Insurance which is protection against a possible eventuality.
Also you called the first entity Doctor, but used it as Medicine, so please change this if doctor is medicine.
You need to revise your entities as they are completely wrong:
Doctor
use DoctixMedecinBundleEntityInsurance;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Doctor
// ...
/**
* @ORMManyToMany(targetEntity="Insurance", mappedBy="doctors" cascade="persist", "remove")
* @ORMJoinColumn(nullable=true)
*/
private $insurances;
public function __construct()
$this->insurances = new ArrayCollection();
public function addInsurance(Insurance $insurance)
if (!$this->insurances->contains($insurance))
$this->insurances->add($insurance);
$insurance->addDoctor($this);
public function removeInsurance(Insurance $insurance)
if ($this->insurances->contains($insurance))
$this->insurances->removeElement($insurance);
$insurance->removeDoctor($this);
/**
* @return Collection
*/
public function getInsurances()
return $this->insurances;
// ...
Insurance
use DoctixMedecinBundleEntityDoctor;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Insurance
// ...
/**
* @ORMManyToMany(targetEntity="Doctor", inversedBy="insurances")
* @ORMJoinColumn(nullable=true)
*/
private $doctors;
public function __construct()
$this->doctors = new ArrayCollection();
public function addDoctor(Doctor $doctor)
if (!$this->doctors->contains($doctor))
$this->doctors->add($doctor);
$doctor->addInsurance($this);
public function removeDoctor(Doctor $doctor)
if ($this->doctors->contains($doctor))
$this->doctors->removeElement($doctor);
$doctor->removeInsurance($this);
/**
* @return Collection
*/
public function getDoctors()
return $this->doctors;
// ...
Update
Controller
public function parametreAction(Request $request)
$em = $this->getDoctrine()->getManager();
$doctor = $em->getRepository('DoctixMedecinBundle:Medecin')->findOneBy(array(
'user' => $this->getUser(),
));
return $this->render('DoctixMedecinBundle:Medecin:parametre.html.twig', array(
'doctor' => $doctor
));
Twig
<div class="col-md-2">
<div class="box_list photo-medecin">
% for insurance in doctor.insurances %
<figure>
<img src=" vich_uploader_asset(insurance.logo, 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
</div>
Hi @Trix, I started from the premise that a doctor can be subscribed to 1 or more insurances for his clinic.And a given insurance ensures 1 or more doctors.So if your entity is correct how can I access the image of an insurance in my twig file?
– Mohamed Sacko
Nov 14 '18 at 11:00
so what do you mean by that medecin?
– Trix
Nov 14 '18 at 11:07
excuse me for my english. I speak french. In french medecin is a doctor in english.
– Mohamed Sacko
Nov 14 '18 at 11:46
Check the Update, and please consider accepting & up-voting, if it was helpful
– Trix
Nov 14 '18 at 11:59
add a comment |
Assurance means Guarantee / To be sure, So It is more likely you are after Insurance which is protection against a possible eventuality.
Also you called the first entity Doctor, but used it as Medicine, so please change this if doctor is medicine.
You need to revise your entities as they are completely wrong:
Doctor
use DoctixMedecinBundleEntityInsurance;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Doctor
// ...
/**
* @ORMManyToMany(targetEntity="Insurance", mappedBy="doctors" cascade="persist", "remove")
* @ORMJoinColumn(nullable=true)
*/
private $insurances;
public function __construct()
$this->insurances = new ArrayCollection();
public function addInsurance(Insurance $insurance)
if (!$this->insurances->contains($insurance))
$this->insurances->add($insurance);
$insurance->addDoctor($this);
public function removeInsurance(Insurance $insurance)
if ($this->insurances->contains($insurance))
$this->insurances->removeElement($insurance);
$insurance->removeDoctor($this);
/**
* @return Collection
*/
public function getInsurances()
return $this->insurances;
// ...
Insurance
use DoctixMedecinBundleEntityDoctor;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Insurance
// ...
/**
* @ORMManyToMany(targetEntity="Doctor", inversedBy="insurances")
* @ORMJoinColumn(nullable=true)
*/
private $doctors;
public function __construct()
$this->doctors = new ArrayCollection();
public function addDoctor(Doctor $doctor)
if (!$this->doctors->contains($doctor))
$this->doctors->add($doctor);
$doctor->addInsurance($this);
public function removeDoctor(Doctor $doctor)
if ($this->doctors->contains($doctor))
$this->doctors->removeElement($doctor);
$doctor->removeInsurance($this);
/**
* @return Collection
*/
public function getDoctors()
return $this->doctors;
// ...
Update
Controller
public function parametreAction(Request $request)
$em = $this->getDoctrine()->getManager();
$doctor = $em->getRepository('DoctixMedecinBundle:Medecin')->findOneBy(array(
'user' => $this->getUser(),
));
return $this->render('DoctixMedecinBundle:Medecin:parametre.html.twig', array(
'doctor' => $doctor
));
Twig
<div class="col-md-2">
<div class="box_list photo-medecin">
% for insurance in doctor.insurances %
<figure>
<img src=" vich_uploader_asset(insurance.logo, 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
</div>
Hi @Trix, I started from the premise that a doctor can be subscribed to 1 or more insurances for his clinic.And a given insurance ensures 1 or more doctors.So if your entity is correct how can I access the image of an insurance in my twig file?
– Mohamed Sacko
Nov 14 '18 at 11:00
so what do you mean by that medecin?
– Trix
Nov 14 '18 at 11:07
excuse me for my english. I speak french. In french medecin is a doctor in english.
– Mohamed Sacko
Nov 14 '18 at 11:46
Check the Update, and please consider accepting & up-voting, if it was helpful
– Trix
Nov 14 '18 at 11:59
add a comment |
Assurance means Guarantee / To be sure, So It is more likely you are after Insurance which is protection against a possible eventuality.
Also you called the first entity Doctor, but used it as Medicine, so please change this if doctor is medicine.
You need to revise your entities as they are completely wrong:
Doctor
use DoctixMedecinBundleEntityInsurance;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Doctor
// ...
/**
* @ORMManyToMany(targetEntity="Insurance", mappedBy="doctors" cascade="persist", "remove")
* @ORMJoinColumn(nullable=true)
*/
private $insurances;
public function __construct()
$this->insurances = new ArrayCollection();
public function addInsurance(Insurance $insurance)
if (!$this->insurances->contains($insurance))
$this->insurances->add($insurance);
$insurance->addDoctor($this);
public function removeInsurance(Insurance $insurance)
if ($this->insurances->contains($insurance))
$this->insurances->removeElement($insurance);
$insurance->removeDoctor($this);
/**
* @return Collection
*/
public function getInsurances()
return $this->insurances;
// ...
Insurance
use DoctixMedecinBundleEntityDoctor;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Insurance
// ...
/**
* @ORMManyToMany(targetEntity="Doctor", inversedBy="insurances")
* @ORMJoinColumn(nullable=true)
*/
private $doctors;
public function __construct()
$this->doctors = new ArrayCollection();
public function addDoctor(Doctor $doctor)
if (!$this->doctors->contains($doctor))
$this->doctors->add($doctor);
$doctor->addInsurance($this);
public function removeDoctor(Doctor $doctor)
if ($this->doctors->contains($doctor))
$this->doctors->removeElement($doctor);
$doctor->removeInsurance($this);
/**
* @return Collection
*/
public function getDoctors()
return $this->doctors;
// ...
Update
Controller
public function parametreAction(Request $request)
$em = $this->getDoctrine()->getManager();
$doctor = $em->getRepository('DoctixMedecinBundle:Medecin')->findOneBy(array(
'user' => $this->getUser(),
));
return $this->render('DoctixMedecinBundle:Medecin:parametre.html.twig', array(
'doctor' => $doctor
));
Twig
<div class="col-md-2">
<div class="box_list photo-medecin">
% for insurance in doctor.insurances %
<figure>
<img src=" vich_uploader_asset(insurance.logo, 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
</div>
Assurance means Guarantee / To be sure, So It is more likely you are after Insurance which is protection against a possible eventuality.
Also you called the first entity Doctor, but used it as Medicine, so please change this if doctor is medicine.
You need to revise your entities as they are completely wrong:
Doctor
use DoctixMedecinBundleEntityInsurance;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Doctor
// ...
/**
* @ORMManyToMany(targetEntity="Insurance", mappedBy="doctors" cascade="persist", "remove")
* @ORMJoinColumn(nullable=true)
*/
private $insurances;
public function __construct()
$this->insurances = new ArrayCollection();
public function addInsurance(Insurance $insurance)
if (!$this->insurances->contains($insurance))
$this->insurances->add($insurance);
$insurance->addDoctor($this);
public function removeInsurance(Insurance $insurance)
if ($this->insurances->contains($insurance))
$this->insurances->removeElement($insurance);
$insurance->removeDoctor($this);
/**
* @return Collection
*/
public function getInsurances()
return $this->insurances;
// ...
Insurance
use DoctixMedecinBundleEntityDoctor;
use DoctrineCommonCollectionsArrayCollection;
use DoctrineCommonCollectionsCollection;
use DoctrineORMMapping as ORM;
class Insurance
// ...
/**
* @ORMManyToMany(targetEntity="Doctor", inversedBy="insurances")
* @ORMJoinColumn(nullable=true)
*/
private $doctors;
public function __construct()
$this->doctors = new ArrayCollection();
public function addDoctor(Doctor $doctor)
if (!$this->doctors->contains($doctor))
$this->doctors->add($doctor);
$doctor->addInsurance($this);
public function removeDoctor(Doctor $doctor)
if ($this->doctors->contains($doctor))
$this->doctors->removeElement($doctor);
$doctor->removeInsurance($this);
/**
* @return Collection
*/
public function getDoctors()
return $this->doctors;
// ...
Update
Controller
public function parametreAction(Request $request)
$em = $this->getDoctrine()->getManager();
$doctor = $em->getRepository('DoctixMedecinBundle:Medecin')->findOneBy(array(
'user' => $this->getUser(),
));
return $this->render('DoctixMedecinBundle:Medecin:parametre.html.twig', array(
'doctor' => $doctor
));
Twig
<div class="col-md-2">
<div class="box_list photo-medecin">
% for insurance in doctor.insurances %
<figure>
<img src=" vich_uploader_asset(insurance.logo, 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
</div>
edited Nov 14 '18 at 11:58
answered Nov 14 '18 at 9:52


TrixTrix
9,80285174
9,80285174
Hi @Trix, I started from the premise that a doctor can be subscribed to 1 or more insurances for his clinic.And a given insurance ensures 1 or more doctors.So if your entity is correct how can I access the image of an insurance in my twig file?
– Mohamed Sacko
Nov 14 '18 at 11:00
so what do you mean by that medecin?
– Trix
Nov 14 '18 at 11:07
excuse me for my english. I speak french. In french medecin is a doctor in english.
– Mohamed Sacko
Nov 14 '18 at 11:46
Check the Update, and please consider accepting & up-voting, if it was helpful
– Trix
Nov 14 '18 at 11:59
add a comment |
Hi @Trix, I started from the premise that a doctor can be subscribed to 1 or more insurances for his clinic.And a given insurance ensures 1 or more doctors.So if your entity is correct how can I access the image of an insurance in my twig file?
– Mohamed Sacko
Nov 14 '18 at 11:00
so what do you mean by that medecin?
– Trix
Nov 14 '18 at 11:07
excuse me for my english. I speak french. In french medecin is a doctor in english.
– Mohamed Sacko
Nov 14 '18 at 11:46
Check the Update, and please consider accepting & up-voting, if it was helpful
– Trix
Nov 14 '18 at 11:59
Hi @Trix, I started from the premise that a doctor can be subscribed to 1 or more insurances for his clinic.And a given insurance ensures 1 or more doctors.So if your entity is correct how can I access the image of an insurance in my twig file?
– Mohamed Sacko
Nov 14 '18 at 11:00
Hi @Trix, I started from the premise that a doctor can be subscribed to 1 or more insurances for his clinic.And a given insurance ensures 1 or more doctors.So if your entity is correct how can I access the image of an insurance in my twig file?
– Mohamed Sacko
Nov 14 '18 at 11:00
so what do you mean by that medecin?
– Trix
Nov 14 '18 at 11:07
so what do you mean by that medecin?
– Trix
Nov 14 '18 at 11:07
excuse me for my english. I speak french. In french medecin is a doctor in english.
– Mohamed Sacko
Nov 14 '18 at 11:46
excuse me for my english. I speak french. In french medecin is a doctor in english.
– Mohamed Sacko
Nov 14 '18 at 11:46
Check the Update, and please consider accepting & up-voting, if it was helpful
– Trix
Nov 14 '18 at 11:59
Check the Update, and please consider accepting & up-voting, if it was helpful
– Trix
Nov 14 '18 at 11:59
add a comment |
<div class = "col-md-2">
% for item in medecin.assurance %
<div class="box_list photo-medecin">
<figure>
<img src=" vich_uploader_asset(item.logo , 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
add a comment |
<div class = "col-md-2">
% for item in medecin.assurance %
<div class="box_list photo-medecin">
<figure>
<img src=" vich_uploader_asset(item.logo , 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
add a comment |
<div class = "col-md-2">
% for item in medecin.assurance %
<div class="box_list photo-medecin">
<figure>
<img src=" vich_uploader_asset(item.logo , 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
<div class = "col-md-2">
% for item in medecin.assurance %
<div class="box_list photo-medecin">
<figure>
<img src=" vich_uploader_asset(item.logo , 'logoFile')
" class="img-fluid" alt="">
</figure>
% endfor %
</div>
edited Nov 14 '18 at 16:40
answered Nov 14 '18 at 8:44


mohamed jebrimohamed jebri
1079
1079
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53285470%2fhow-to-use-an-attribute-of-an-entity-that-has-a-manytomany-relationship-with-ano%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
TdwVwsobeIaCRbrNxjG4NabdqGvgcf,3sc,u5r eBuxE2lSZ,ZqgpC,yamnaBf16HFEx,rDXJYl5CI2