Kivy: Delete Data in JSON using RecycleView
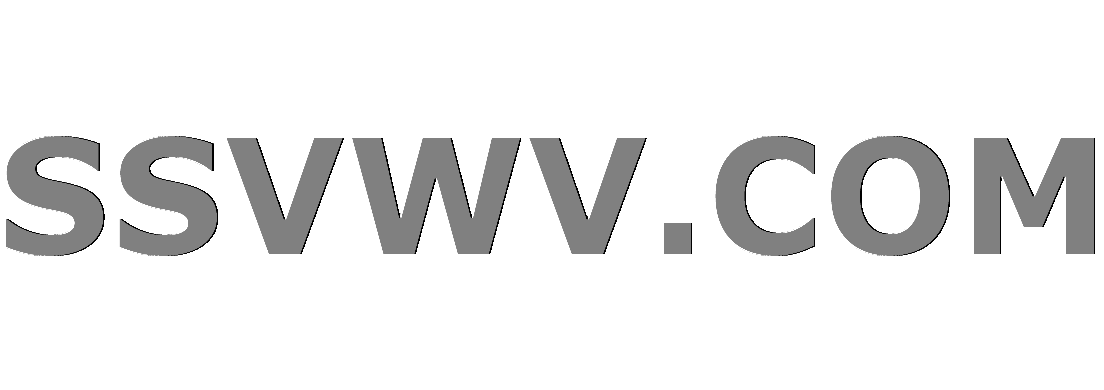
Multi tool use
I want to delete data from a JSON file using store.delete('key_of_dictionary')
by selecting a label in RecycleView, then pressing a 'Delete' button.
I've made my code to select the labels, and get the value, but I cannot pass it to a method/function. The method must be in class SelectableLabel(...)
, as it seems. I'm not sure how to access that class.
I would appreciate any insight. Thank you in advance!
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.label import Label
from kivy.properties import BooleanProperty
from kivy.uix.behaviors import FocusBehavior
from kivy.storage.jsonstore import JsonStore
from kivy.uix.recyclegridlayout import RecycleGridLayout
from kivy.uix.recycleview.views import RecycleDataViewBehavior
from kivy.uix.recycleview.layout import LayoutSelectionBehavior
from operator import itemgetter
import json
kv_string = """
ScreenManager:
id: manager
Screen:
BoxLayout:
orientation: 'vertical'
canvas:
Color:
rgba: .2,.2,.5,1
Rectangle:
pos: self.pos
size: self.size
GridLayout:
size_hint_y: .3
cols:4
MyButton:
text: 'Rank'
size_hint_x: 0.5
MyButton:
text: 'Ratings'
MyButton:
text: 'Name'
size_hint_x: 2
MyButton:
text: 'Score'
on_press:
#arrange the boxing in ascending or descending order
RecycleView:
data: ['text': str(x) for x in app.new_new_sorted_data]
viewclass: 'SelectableLabel'
SelectableRecycleGridLayout:
cols: 4
default_size_hint: 1, None
size_hint_y: None
height: self.minimum_height
orientation: 'vertical'
multiselect: True
touch_multiselect: True
Button:
size_hint_y: .3
text: 'Press to Use Method'
on_press:
SelectableLabel().function()
<SelectableLabel>:
canvas.before:
Color:
rgba: (.0, 0.9, .1, .3) if self.selected else (0, 0, 0, 1)
Rectangle:
pos: self.pos
size: self.size
<MyButton@Button>:
background_color: 0,0,0,1
"""
class SelectableLabel(RecycleDataViewBehavior, Label):
def function(self):
print('Take the value in rv.data[index] if 4 % == 0 then donstore.delete(rv.data[index])')
index = None
selected = BooleanProperty(False)
selectable = BooleanProperty(True)
def refresh_view_layout(self, rv, index, layout, viewport):
mod = index % 4
size_hints = [(0.5, None), (1, None), (2, None), (1, None)]
layout['size_hint'] = size_hints[mod]
colors = (1,1,1,1), (1,1,1,1), (1,1,1,1), (0,1,1,1)
layout['color'] = colors[mod]
super(SelectableLabel, self).refresh_view_layout(rv, index, layout, viewport)
def refresh_view_attrs(self, rv, index, data):
self.index = index
return super(SelectableLabel, self).refresh_view_attrs(
rv, index, data)
def on_touch_down(self, touch):
if super(SelectableLabel, self).on_touch_down(touch):
return True
if self.collide_point(*touch.pos) and self.selectable:
self.parent.select_with_touch(self.index, touch)
if self.index % 4 == 0:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index + 3)
return
elif self.index % 4 == 1:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index - 1)
return
elif self.index % 4 == 2:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 1)
return
elif self.index % 4 == 3:
self.parent.select_with_touch(self.index - 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 3)
return
index_list =
def apply_selection(self, rv, index, is_selected):
self.selected = is_selected
if is_selected:
self.index_list.append(rv.data[index]) if index % 4 == 0 else self.index_list
print(self.index_list) if index % 4 == 0 else ''
#print("selection changed to 0".format(rv.data[index]))
else:
try:
self.index_list.remove(rv.data[index]) if index % 4 == 0 else self.index_list
print(self.index_list) if index % 4 == 0 else ''
#print("selection removed for 0".format(rv.data[index]))
except:
pass
class SelectableRecycleGridLayout(FocusBehavior, LayoutSelectionBehavior, RecycleGridLayout):
pass
class MyApp(App):
store = JsonStore('file.json')
store.put('Example: 1', value_1 = 'Rating: C', value_2 = 10, value_3 = 'Zack')
store.put('Example: 2', value_1 = 'Rating: C', value_2 = 13, value_3 = 'Kate')
store.put('Example: 3', value_1 = 'Rating: A', value_2 = 32, value_3 = 'Pete')
store.put('Example: 4', value_1 = 'Rating: B', value_2 = 24, value_3 = 'Toby')
store.put('Example: 5', value_1 = 'Rating: D', value_2 = 03, value_3 = 'Lars')
# ---------------------- v Sorting Data v ----------------------
json_data = open('./file.json')
score_data = json.load(json_data)
newlist =
newnewlist =
for x in score_data:
newlist.append(x)
for y in newlist:
newnewlist.append(score_data[str(y)])
print(newlist)
print(newnewlist)
sorted_data = sorted(newnewlist,key=itemgetter('value_2'), reverse = True)
new_sorted_data =
for z in sorted_data:
new_sorted_data.append(z.values())
new_new_sorted_data =
for x,y,z in new_sorted_data:
new_new_sorted_data.append(newlist.pop())
new_new_sorted_data.append(x)
new_new_sorted_data.append(y)
new_new_sorted_data.append(z)
# ---------------------- ^ Sorting Data ^ ----------------------
def build(self):
root_widget = Builder.load_string(kv_string)
return root_widget
if __name__ == "__main__":
MyApp().run()
python json kivy
add a comment |
I want to delete data from a JSON file using store.delete('key_of_dictionary')
by selecting a label in RecycleView, then pressing a 'Delete' button.
I've made my code to select the labels, and get the value, but I cannot pass it to a method/function. The method must be in class SelectableLabel(...)
, as it seems. I'm not sure how to access that class.
I would appreciate any insight. Thank you in advance!
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.label import Label
from kivy.properties import BooleanProperty
from kivy.uix.behaviors import FocusBehavior
from kivy.storage.jsonstore import JsonStore
from kivy.uix.recyclegridlayout import RecycleGridLayout
from kivy.uix.recycleview.views import RecycleDataViewBehavior
from kivy.uix.recycleview.layout import LayoutSelectionBehavior
from operator import itemgetter
import json
kv_string = """
ScreenManager:
id: manager
Screen:
BoxLayout:
orientation: 'vertical'
canvas:
Color:
rgba: .2,.2,.5,1
Rectangle:
pos: self.pos
size: self.size
GridLayout:
size_hint_y: .3
cols:4
MyButton:
text: 'Rank'
size_hint_x: 0.5
MyButton:
text: 'Ratings'
MyButton:
text: 'Name'
size_hint_x: 2
MyButton:
text: 'Score'
on_press:
#arrange the boxing in ascending or descending order
RecycleView:
data: ['text': str(x) for x in app.new_new_sorted_data]
viewclass: 'SelectableLabel'
SelectableRecycleGridLayout:
cols: 4
default_size_hint: 1, None
size_hint_y: None
height: self.minimum_height
orientation: 'vertical'
multiselect: True
touch_multiselect: True
Button:
size_hint_y: .3
text: 'Press to Use Method'
on_press:
SelectableLabel().function()
<SelectableLabel>:
canvas.before:
Color:
rgba: (.0, 0.9, .1, .3) if self.selected else (0, 0, 0, 1)
Rectangle:
pos: self.pos
size: self.size
<MyButton@Button>:
background_color: 0,0,0,1
"""
class SelectableLabel(RecycleDataViewBehavior, Label):
def function(self):
print('Take the value in rv.data[index] if 4 % == 0 then donstore.delete(rv.data[index])')
index = None
selected = BooleanProperty(False)
selectable = BooleanProperty(True)
def refresh_view_layout(self, rv, index, layout, viewport):
mod = index % 4
size_hints = [(0.5, None), (1, None), (2, None), (1, None)]
layout['size_hint'] = size_hints[mod]
colors = (1,1,1,1), (1,1,1,1), (1,1,1,1), (0,1,1,1)
layout['color'] = colors[mod]
super(SelectableLabel, self).refresh_view_layout(rv, index, layout, viewport)
def refresh_view_attrs(self, rv, index, data):
self.index = index
return super(SelectableLabel, self).refresh_view_attrs(
rv, index, data)
def on_touch_down(self, touch):
if super(SelectableLabel, self).on_touch_down(touch):
return True
if self.collide_point(*touch.pos) and self.selectable:
self.parent.select_with_touch(self.index, touch)
if self.index % 4 == 0:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index + 3)
return
elif self.index % 4 == 1:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index - 1)
return
elif self.index % 4 == 2:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 1)
return
elif self.index % 4 == 3:
self.parent.select_with_touch(self.index - 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 3)
return
index_list =
def apply_selection(self, rv, index, is_selected):
self.selected = is_selected
if is_selected:
self.index_list.append(rv.data[index]) if index % 4 == 0 else self.index_list
print(self.index_list) if index % 4 == 0 else ''
#print("selection changed to 0".format(rv.data[index]))
else:
try:
self.index_list.remove(rv.data[index]) if index % 4 == 0 else self.index_list
print(self.index_list) if index % 4 == 0 else ''
#print("selection removed for 0".format(rv.data[index]))
except:
pass
class SelectableRecycleGridLayout(FocusBehavior, LayoutSelectionBehavior, RecycleGridLayout):
pass
class MyApp(App):
store = JsonStore('file.json')
store.put('Example: 1', value_1 = 'Rating: C', value_2 = 10, value_3 = 'Zack')
store.put('Example: 2', value_1 = 'Rating: C', value_2 = 13, value_3 = 'Kate')
store.put('Example: 3', value_1 = 'Rating: A', value_2 = 32, value_3 = 'Pete')
store.put('Example: 4', value_1 = 'Rating: B', value_2 = 24, value_3 = 'Toby')
store.put('Example: 5', value_1 = 'Rating: D', value_2 = 03, value_3 = 'Lars')
# ---------------------- v Sorting Data v ----------------------
json_data = open('./file.json')
score_data = json.load(json_data)
newlist =
newnewlist =
for x in score_data:
newlist.append(x)
for y in newlist:
newnewlist.append(score_data[str(y)])
print(newlist)
print(newnewlist)
sorted_data = sorted(newnewlist,key=itemgetter('value_2'), reverse = True)
new_sorted_data =
for z in sorted_data:
new_sorted_data.append(z.values())
new_new_sorted_data =
for x,y,z in new_sorted_data:
new_new_sorted_data.append(newlist.pop())
new_new_sorted_data.append(x)
new_new_sorted_data.append(y)
new_new_sorted_data.append(z)
# ---------------------- ^ Sorting Data ^ ----------------------
def build(self):
root_widget = Builder.load_string(kv_string)
return root_widget
if __name__ == "__main__":
MyApp().run()
python json kivy
Do you want to select n items and after pressing the button should you delete those items?
– eyllanesc
Nov 14 '18 at 2:13
Hey @eyllanesc , yes, I want to first select them then if I press the bottom button, they will be deleted. It seems like the method/function will have to be inclass SelectableLabel(RecycleDataViewBehavior, Label):
but I'm not sure how to access it, nor how to pass theindex_list
that is filled with the keys.
– Petar Luketina
Nov 14 '18 at 19:12
that you must explain in your question, so I recommend you edit your question and add that information.
– eyllanesc
Nov 14 '18 at 19:14
Okay, I edited the description to make it clear. Thank you for letting me know!!!
– Petar Luketina
Nov 14 '18 at 23:59
add a comment |
I want to delete data from a JSON file using store.delete('key_of_dictionary')
by selecting a label in RecycleView, then pressing a 'Delete' button.
I've made my code to select the labels, and get the value, but I cannot pass it to a method/function. The method must be in class SelectableLabel(...)
, as it seems. I'm not sure how to access that class.
I would appreciate any insight. Thank you in advance!
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.label import Label
from kivy.properties import BooleanProperty
from kivy.uix.behaviors import FocusBehavior
from kivy.storage.jsonstore import JsonStore
from kivy.uix.recyclegridlayout import RecycleGridLayout
from kivy.uix.recycleview.views import RecycleDataViewBehavior
from kivy.uix.recycleview.layout import LayoutSelectionBehavior
from operator import itemgetter
import json
kv_string = """
ScreenManager:
id: manager
Screen:
BoxLayout:
orientation: 'vertical'
canvas:
Color:
rgba: .2,.2,.5,1
Rectangle:
pos: self.pos
size: self.size
GridLayout:
size_hint_y: .3
cols:4
MyButton:
text: 'Rank'
size_hint_x: 0.5
MyButton:
text: 'Ratings'
MyButton:
text: 'Name'
size_hint_x: 2
MyButton:
text: 'Score'
on_press:
#arrange the boxing in ascending or descending order
RecycleView:
data: ['text': str(x) for x in app.new_new_sorted_data]
viewclass: 'SelectableLabel'
SelectableRecycleGridLayout:
cols: 4
default_size_hint: 1, None
size_hint_y: None
height: self.minimum_height
orientation: 'vertical'
multiselect: True
touch_multiselect: True
Button:
size_hint_y: .3
text: 'Press to Use Method'
on_press:
SelectableLabel().function()
<SelectableLabel>:
canvas.before:
Color:
rgba: (.0, 0.9, .1, .3) if self.selected else (0, 0, 0, 1)
Rectangle:
pos: self.pos
size: self.size
<MyButton@Button>:
background_color: 0,0,0,1
"""
class SelectableLabel(RecycleDataViewBehavior, Label):
def function(self):
print('Take the value in rv.data[index] if 4 % == 0 then donstore.delete(rv.data[index])')
index = None
selected = BooleanProperty(False)
selectable = BooleanProperty(True)
def refresh_view_layout(self, rv, index, layout, viewport):
mod = index % 4
size_hints = [(0.5, None), (1, None), (2, None), (1, None)]
layout['size_hint'] = size_hints[mod]
colors = (1,1,1,1), (1,1,1,1), (1,1,1,1), (0,1,1,1)
layout['color'] = colors[mod]
super(SelectableLabel, self).refresh_view_layout(rv, index, layout, viewport)
def refresh_view_attrs(self, rv, index, data):
self.index = index
return super(SelectableLabel, self).refresh_view_attrs(
rv, index, data)
def on_touch_down(self, touch):
if super(SelectableLabel, self).on_touch_down(touch):
return True
if self.collide_point(*touch.pos) and self.selectable:
self.parent.select_with_touch(self.index, touch)
if self.index % 4 == 0:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index + 3)
return
elif self.index % 4 == 1:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index - 1)
return
elif self.index % 4 == 2:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 1)
return
elif self.index % 4 == 3:
self.parent.select_with_touch(self.index - 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 3)
return
index_list =
def apply_selection(self, rv, index, is_selected):
self.selected = is_selected
if is_selected:
self.index_list.append(rv.data[index]) if index % 4 == 0 else self.index_list
print(self.index_list) if index % 4 == 0 else ''
#print("selection changed to 0".format(rv.data[index]))
else:
try:
self.index_list.remove(rv.data[index]) if index % 4 == 0 else self.index_list
print(self.index_list) if index % 4 == 0 else ''
#print("selection removed for 0".format(rv.data[index]))
except:
pass
class SelectableRecycleGridLayout(FocusBehavior, LayoutSelectionBehavior, RecycleGridLayout):
pass
class MyApp(App):
store = JsonStore('file.json')
store.put('Example: 1', value_1 = 'Rating: C', value_2 = 10, value_3 = 'Zack')
store.put('Example: 2', value_1 = 'Rating: C', value_2 = 13, value_3 = 'Kate')
store.put('Example: 3', value_1 = 'Rating: A', value_2 = 32, value_3 = 'Pete')
store.put('Example: 4', value_1 = 'Rating: B', value_2 = 24, value_3 = 'Toby')
store.put('Example: 5', value_1 = 'Rating: D', value_2 = 03, value_3 = 'Lars')
# ---------------------- v Sorting Data v ----------------------
json_data = open('./file.json')
score_data = json.load(json_data)
newlist =
newnewlist =
for x in score_data:
newlist.append(x)
for y in newlist:
newnewlist.append(score_data[str(y)])
print(newlist)
print(newnewlist)
sorted_data = sorted(newnewlist,key=itemgetter('value_2'), reverse = True)
new_sorted_data =
for z in sorted_data:
new_sorted_data.append(z.values())
new_new_sorted_data =
for x,y,z in new_sorted_data:
new_new_sorted_data.append(newlist.pop())
new_new_sorted_data.append(x)
new_new_sorted_data.append(y)
new_new_sorted_data.append(z)
# ---------------------- ^ Sorting Data ^ ----------------------
def build(self):
root_widget = Builder.load_string(kv_string)
return root_widget
if __name__ == "__main__":
MyApp().run()
python json kivy
I want to delete data from a JSON file using store.delete('key_of_dictionary')
by selecting a label in RecycleView, then pressing a 'Delete' button.
I've made my code to select the labels, and get the value, but I cannot pass it to a method/function. The method must be in class SelectableLabel(...)
, as it seems. I'm not sure how to access that class.
I would appreciate any insight. Thank you in advance!
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.label import Label
from kivy.properties import BooleanProperty
from kivy.uix.behaviors import FocusBehavior
from kivy.storage.jsonstore import JsonStore
from kivy.uix.recyclegridlayout import RecycleGridLayout
from kivy.uix.recycleview.views import RecycleDataViewBehavior
from kivy.uix.recycleview.layout import LayoutSelectionBehavior
from operator import itemgetter
import json
kv_string = """
ScreenManager:
id: manager
Screen:
BoxLayout:
orientation: 'vertical'
canvas:
Color:
rgba: .2,.2,.5,1
Rectangle:
pos: self.pos
size: self.size
GridLayout:
size_hint_y: .3
cols:4
MyButton:
text: 'Rank'
size_hint_x: 0.5
MyButton:
text: 'Ratings'
MyButton:
text: 'Name'
size_hint_x: 2
MyButton:
text: 'Score'
on_press:
#arrange the boxing in ascending or descending order
RecycleView:
data: ['text': str(x) for x in app.new_new_sorted_data]
viewclass: 'SelectableLabel'
SelectableRecycleGridLayout:
cols: 4
default_size_hint: 1, None
size_hint_y: None
height: self.minimum_height
orientation: 'vertical'
multiselect: True
touch_multiselect: True
Button:
size_hint_y: .3
text: 'Press to Use Method'
on_press:
SelectableLabel().function()
<SelectableLabel>:
canvas.before:
Color:
rgba: (.0, 0.9, .1, .3) if self.selected else (0, 0, 0, 1)
Rectangle:
pos: self.pos
size: self.size
<MyButton@Button>:
background_color: 0,0,0,1
"""
class SelectableLabel(RecycleDataViewBehavior, Label):
def function(self):
print('Take the value in rv.data[index] if 4 % == 0 then donstore.delete(rv.data[index])')
index = None
selected = BooleanProperty(False)
selectable = BooleanProperty(True)
def refresh_view_layout(self, rv, index, layout, viewport):
mod = index % 4
size_hints = [(0.5, None), (1, None), (2, None), (1, None)]
layout['size_hint'] = size_hints[mod]
colors = (1,1,1,1), (1,1,1,1), (1,1,1,1), (0,1,1,1)
layout['color'] = colors[mod]
super(SelectableLabel, self).refresh_view_layout(rv, index, layout, viewport)
def refresh_view_attrs(self, rv, index, data):
self.index = index
return super(SelectableLabel, self).refresh_view_attrs(
rv, index, data)
def on_touch_down(self, touch):
if super(SelectableLabel, self).on_touch_down(touch):
return True
if self.collide_point(*touch.pos) and self.selectable:
self.parent.select_with_touch(self.index, touch)
if self.index % 4 == 0:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index + 3)
return
elif self.index % 4 == 1:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index - 1)
return
elif self.index % 4 == 2:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 1)
return
elif self.index % 4 == 3:
self.parent.select_with_touch(self.index - 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 3)
return
index_list =
def apply_selection(self, rv, index, is_selected):
self.selected = is_selected
if is_selected:
self.index_list.append(rv.data[index]) if index % 4 == 0 else self.index_list
print(self.index_list) if index % 4 == 0 else ''
#print("selection changed to 0".format(rv.data[index]))
else:
try:
self.index_list.remove(rv.data[index]) if index % 4 == 0 else self.index_list
print(self.index_list) if index % 4 == 0 else ''
#print("selection removed for 0".format(rv.data[index]))
except:
pass
class SelectableRecycleGridLayout(FocusBehavior, LayoutSelectionBehavior, RecycleGridLayout):
pass
class MyApp(App):
store = JsonStore('file.json')
store.put('Example: 1', value_1 = 'Rating: C', value_2 = 10, value_3 = 'Zack')
store.put('Example: 2', value_1 = 'Rating: C', value_2 = 13, value_3 = 'Kate')
store.put('Example: 3', value_1 = 'Rating: A', value_2 = 32, value_3 = 'Pete')
store.put('Example: 4', value_1 = 'Rating: B', value_2 = 24, value_3 = 'Toby')
store.put('Example: 5', value_1 = 'Rating: D', value_2 = 03, value_3 = 'Lars')
# ---------------------- v Sorting Data v ----------------------
json_data = open('./file.json')
score_data = json.load(json_data)
newlist =
newnewlist =
for x in score_data:
newlist.append(x)
for y in newlist:
newnewlist.append(score_data[str(y)])
print(newlist)
print(newnewlist)
sorted_data = sorted(newnewlist,key=itemgetter('value_2'), reverse = True)
new_sorted_data =
for z in sorted_data:
new_sorted_data.append(z.values())
new_new_sorted_data =
for x,y,z in new_sorted_data:
new_new_sorted_data.append(newlist.pop())
new_new_sorted_data.append(x)
new_new_sorted_data.append(y)
new_new_sorted_data.append(z)
# ---------------------- ^ Sorting Data ^ ----------------------
def build(self):
root_widget = Builder.load_string(kv_string)
return root_widget
if __name__ == "__main__":
MyApp().run()
python json kivy
python json kivy
edited Nov 14 '18 at 23:57
asked Nov 13 '18 at 1:44
Petar Luketina
447
447
Do you want to select n items and after pressing the button should you delete those items?
– eyllanesc
Nov 14 '18 at 2:13
Hey @eyllanesc , yes, I want to first select them then if I press the bottom button, they will be deleted. It seems like the method/function will have to be inclass SelectableLabel(RecycleDataViewBehavior, Label):
but I'm not sure how to access it, nor how to pass theindex_list
that is filled with the keys.
– Petar Luketina
Nov 14 '18 at 19:12
that you must explain in your question, so I recommend you edit your question and add that information.
– eyllanesc
Nov 14 '18 at 19:14
Okay, I edited the description to make it clear. Thank you for letting me know!!!
– Petar Luketina
Nov 14 '18 at 23:59
add a comment |
Do you want to select n items and after pressing the button should you delete those items?
– eyllanesc
Nov 14 '18 at 2:13
Hey @eyllanesc , yes, I want to first select them then if I press the bottom button, they will be deleted. It seems like the method/function will have to be inclass SelectableLabel(RecycleDataViewBehavior, Label):
but I'm not sure how to access it, nor how to pass theindex_list
that is filled with the keys.
– Petar Luketina
Nov 14 '18 at 19:12
that you must explain in your question, so I recommend you edit your question and add that information.
– eyllanesc
Nov 14 '18 at 19:14
Okay, I edited the description to make it clear. Thank you for letting me know!!!
– Petar Luketina
Nov 14 '18 at 23:59
Do you want to select n items and after pressing the button should you delete those items?
– eyllanesc
Nov 14 '18 at 2:13
Do you want to select n items and after pressing the button should you delete those items?
– eyllanesc
Nov 14 '18 at 2:13
Hey @eyllanesc , yes, I want to first select them then if I press the bottom button, they will be deleted. It seems like the method/function will have to be in
class SelectableLabel(RecycleDataViewBehavior, Label):
but I'm not sure how to access it, nor how to pass the index_list
that is filled with the keys.– Petar Luketina
Nov 14 '18 at 19:12
Hey @eyllanesc , yes, I want to first select them then if I press the bottom button, they will be deleted. It seems like the method/function will have to be in
class SelectableLabel(RecycleDataViewBehavior, Label):
but I'm not sure how to access it, nor how to pass the index_list
that is filled with the keys.– Petar Luketina
Nov 14 '18 at 19:12
that you must explain in your question, so I recommend you edit your question and add that information.
– eyllanesc
Nov 14 '18 at 19:14
that you must explain in your question, so I recommend you edit your question and add that information.
– eyllanesc
Nov 14 '18 at 19:14
Okay, I edited the description to make it clear. Thank you for letting me know!!!
– Petar Luketina
Nov 14 '18 at 23:59
Okay, I edited the description to make it clear. Thank you for letting me know!!!
– Petar Luketina
Nov 14 '18 at 23:59
add a comment |
1 Answer
1
active
oldest
votes
I finally got my sample code to do what I wanted. I went through the MyApp
class to get to the SelectLabel
class. I also found a way to delete specific JSON keys through RecycleView
. I didn't comment this piece of code because it's just a sample, but if someone is using this as a reference and needs assistance, feel free to message me.
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.label import Label
from kivy.properties import BooleanProperty, ListProperty
from kivy.uix.behaviors import FocusBehavior
from kivy.storage.jsonstore import JsonStore
from kivy.uix.recyclegridlayout import RecycleGridLayout
from kivy.uix.recycleview.views import RecycleDataViewBehavior
from kivy.uix.recycleview.layout import LayoutSelectionBehavior
from operator import itemgetter
import json
kv_string = """
ScreenManager:
id: manager
Screen:
BoxLayout:
orientation: 'vertical'
canvas:
Color:
rgba: .2,.2,.5,1
Rectangle:
pos: self.pos
size: self.size
GridLayout:
size_hint_y: .3
cols:4
MyButton:
text: 'Rank'
size_hint_x: 0.5
MyButton:
text: 'Ratings'
MyButton:
text: 'Name'
size_hint_x: 2
MyButton:
text: 'Score'
on_press:
#arrange the boxing in ascending or descending order
RecycleView:
data: ['text': str(x) for x in app.new_new_sorted_data]
viewclass: 'SelectableLabel'
SelectableRecycleGridLayout:
cols: 4
default_size_hint: 1, None
size_hint_y: None
height: self.minimum_height
orientation: 'vertical'
multiselect: True
touch_multiselect: True
Button:
size_hint_y: .3
text: 'Press to Use Method'
on_press:
app.pre_function()
<SelectableLabel>:
canvas.before:
Color:
rgba: (.0, 0.9, .1, .3) if self.selected else (0, 0, 0, 1)
Rectangle:
pos: self.pos
size: self.size
<MyButton@Button>:
background_color: 0,0,0,1
"""
class SelectableLabel(RecycleDataViewBehavior, Label):
index = None
selected = BooleanProperty(False)
selectable = BooleanProperty(True)
def refresh_view_layout(self, rv, index, layout, viewport):
mod = index % 4
size_hints = [(0.5, None), (1, None), (2, None), (1, None)]
layout['size_hint'] = size_hints[mod]
colors = (1,1,1,1), (1,1,1,1), (1,1,1,1), (0,1,1,1)
layout['color'] = colors[mod]
super(SelectableLabel, self).refresh_view_layout(rv, index, layout, viewport)
def refresh_view_attrs(self, rv, index, data):
self.index = index
return super(SelectableLabel, self).refresh_view_attrs(
rv, index, data)
def on_touch_down(self, touch):
if super(SelectableLabel, self).on_touch_down(touch):
return True
if self.collide_point(*touch.pos) and self.selectable:
self.parent.select_with_touch(self.index, touch)
if self.index % 4 == 0:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index + 3)
return
elif self.index % 4 == 1:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 2:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 3:
self.parent.select_with_touch(self.index - 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 3)
return
index_list =
def apply_selection(self, rv, index, is_selected):
self.selected = is_selected
if is_selected:
self.index_list.append(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection changed to 0".format(rv.data[index]))
else:
try:
self.index_list.remove(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection removed for 0".format(rv.data[index]))
except:
pass
class SelectableRecycleGridLayout(FocusBehavior, LayoutSelectionBehavior, RecycleGridLayout):
pass
class MyApp(App):
store = JsonStore('file.json')
store.put('Example: 1', value_1 = 'Rating: C', value_2 = 10, value_3 = 'Zack')
store.put('Example: 2', value_1 = 'Rating: C', value_2 = 13, value_3 = 'Kate')
store.put('Example: 3', value_1 = 'Rating: A', value_2 = 32, value_3 = 'Pete')
store.put('Example: 4', value_1 = 'Rating: B', value_2 = 24, value_3 = 'Toby')
store.put('Example: 5', value_1 = 'Rating: D', value_2 = 03, value_3 = 'Lars')
# ---------------------- v Sorting Data v ----------------------
new_new_sorted_data = ListProperty()
def sort_data(self):
try:
self.store = JsonStore('file.json')
self.new_new_sorted_data =
json_data = open('./file.json')
score_data = json.load(json_data)
newlist =
newnewlist =
for x in score_data:
newlist.append(x)
for y in newlist:
newnewlist.append(score_data[str(y)])
print(newlist)
print(newnewlist)
sorted_data = sorted(newnewlist,key=itemgetter('value_2'), reverse = True)
new_sorted_data =
for z in sorted_data:
new_sorted_data.append(z.values())
for x,y,z in new_sorted_data:
self.new_new_sorted_data.append(newlist.pop())
self.new_new_sorted_data.append(x)
self.new_new_sorted_data.append(y)
self.new_new_sorted_data.append(z)
except:
pass
# ---------------------- ^ Sorting Data ^ ----------------------
# --------------------- v Deleting Data v ----------------------
only_key_values_list =
only_key_values =
def pre_function(self):
self.only_key_values_list =
self.only_key_values =
for value in SelectableLabel().index_list:
self.only_key_values_list.append(value.values())
for keys in self.only_key_values_list:
for key in keys:
try:
self.store.delete(str(key))
except:
pass
self.sort_data()
# --------------------- ^ Deleting Data ^ ----------------------
def __init__(self, **kwargs):
super(MyApp, self).__init__(**kwargs)
self.sort_data()
def build(self):
root_widget = Builder.load_string(kv_string)
return root_widget
if __name__ == "__main__":
MyApp().run()
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272586%2fkivy-delete-data-in-json-using-recycleview%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I finally got my sample code to do what I wanted. I went through the MyApp
class to get to the SelectLabel
class. I also found a way to delete specific JSON keys through RecycleView
. I didn't comment this piece of code because it's just a sample, but if someone is using this as a reference and needs assistance, feel free to message me.
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.label import Label
from kivy.properties import BooleanProperty, ListProperty
from kivy.uix.behaviors import FocusBehavior
from kivy.storage.jsonstore import JsonStore
from kivy.uix.recyclegridlayout import RecycleGridLayout
from kivy.uix.recycleview.views import RecycleDataViewBehavior
from kivy.uix.recycleview.layout import LayoutSelectionBehavior
from operator import itemgetter
import json
kv_string = """
ScreenManager:
id: manager
Screen:
BoxLayout:
orientation: 'vertical'
canvas:
Color:
rgba: .2,.2,.5,1
Rectangle:
pos: self.pos
size: self.size
GridLayout:
size_hint_y: .3
cols:4
MyButton:
text: 'Rank'
size_hint_x: 0.5
MyButton:
text: 'Ratings'
MyButton:
text: 'Name'
size_hint_x: 2
MyButton:
text: 'Score'
on_press:
#arrange the boxing in ascending or descending order
RecycleView:
data: ['text': str(x) for x in app.new_new_sorted_data]
viewclass: 'SelectableLabel'
SelectableRecycleGridLayout:
cols: 4
default_size_hint: 1, None
size_hint_y: None
height: self.minimum_height
orientation: 'vertical'
multiselect: True
touch_multiselect: True
Button:
size_hint_y: .3
text: 'Press to Use Method'
on_press:
app.pre_function()
<SelectableLabel>:
canvas.before:
Color:
rgba: (.0, 0.9, .1, .3) if self.selected else (0, 0, 0, 1)
Rectangle:
pos: self.pos
size: self.size
<MyButton@Button>:
background_color: 0,0,0,1
"""
class SelectableLabel(RecycleDataViewBehavior, Label):
index = None
selected = BooleanProperty(False)
selectable = BooleanProperty(True)
def refresh_view_layout(self, rv, index, layout, viewport):
mod = index % 4
size_hints = [(0.5, None), (1, None), (2, None), (1, None)]
layout['size_hint'] = size_hints[mod]
colors = (1,1,1,1), (1,1,1,1), (1,1,1,1), (0,1,1,1)
layout['color'] = colors[mod]
super(SelectableLabel, self).refresh_view_layout(rv, index, layout, viewport)
def refresh_view_attrs(self, rv, index, data):
self.index = index
return super(SelectableLabel, self).refresh_view_attrs(
rv, index, data)
def on_touch_down(self, touch):
if super(SelectableLabel, self).on_touch_down(touch):
return True
if self.collide_point(*touch.pos) and self.selectable:
self.parent.select_with_touch(self.index, touch)
if self.index % 4 == 0:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index + 3)
return
elif self.index % 4 == 1:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 2:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 3:
self.parent.select_with_touch(self.index - 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 3)
return
index_list =
def apply_selection(self, rv, index, is_selected):
self.selected = is_selected
if is_selected:
self.index_list.append(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection changed to 0".format(rv.data[index]))
else:
try:
self.index_list.remove(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection removed for 0".format(rv.data[index]))
except:
pass
class SelectableRecycleGridLayout(FocusBehavior, LayoutSelectionBehavior, RecycleGridLayout):
pass
class MyApp(App):
store = JsonStore('file.json')
store.put('Example: 1', value_1 = 'Rating: C', value_2 = 10, value_3 = 'Zack')
store.put('Example: 2', value_1 = 'Rating: C', value_2 = 13, value_3 = 'Kate')
store.put('Example: 3', value_1 = 'Rating: A', value_2 = 32, value_3 = 'Pete')
store.put('Example: 4', value_1 = 'Rating: B', value_2 = 24, value_3 = 'Toby')
store.put('Example: 5', value_1 = 'Rating: D', value_2 = 03, value_3 = 'Lars')
# ---------------------- v Sorting Data v ----------------------
new_new_sorted_data = ListProperty()
def sort_data(self):
try:
self.store = JsonStore('file.json')
self.new_new_sorted_data =
json_data = open('./file.json')
score_data = json.load(json_data)
newlist =
newnewlist =
for x in score_data:
newlist.append(x)
for y in newlist:
newnewlist.append(score_data[str(y)])
print(newlist)
print(newnewlist)
sorted_data = sorted(newnewlist,key=itemgetter('value_2'), reverse = True)
new_sorted_data =
for z in sorted_data:
new_sorted_data.append(z.values())
for x,y,z in new_sorted_data:
self.new_new_sorted_data.append(newlist.pop())
self.new_new_sorted_data.append(x)
self.new_new_sorted_data.append(y)
self.new_new_sorted_data.append(z)
except:
pass
# ---------------------- ^ Sorting Data ^ ----------------------
# --------------------- v Deleting Data v ----------------------
only_key_values_list =
only_key_values =
def pre_function(self):
self.only_key_values_list =
self.only_key_values =
for value in SelectableLabel().index_list:
self.only_key_values_list.append(value.values())
for keys in self.only_key_values_list:
for key in keys:
try:
self.store.delete(str(key))
except:
pass
self.sort_data()
# --------------------- ^ Deleting Data ^ ----------------------
def __init__(self, **kwargs):
super(MyApp, self).__init__(**kwargs)
self.sort_data()
def build(self):
root_widget = Builder.load_string(kv_string)
return root_widget
if __name__ == "__main__":
MyApp().run()
add a comment |
I finally got my sample code to do what I wanted. I went through the MyApp
class to get to the SelectLabel
class. I also found a way to delete specific JSON keys through RecycleView
. I didn't comment this piece of code because it's just a sample, but if someone is using this as a reference and needs assistance, feel free to message me.
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.label import Label
from kivy.properties import BooleanProperty, ListProperty
from kivy.uix.behaviors import FocusBehavior
from kivy.storage.jsonstore import JsonStore
from kivy.uix.recyclegridlayout import RecycleGridLayout
from kivy.uix.recycleview.views import RecycleDataViewBehavior
from kivy.uix.recycleview.layout import LayoutSelectionBehavior
from operator import itemgetter
import json
kv_string = """
ScreenManager:
id: manager
Screen:
BoxLayout:
orientation: 'vertical'
canvas:
Color:
rgba: .2,.2,.5,1
Rectangle:
pos: self.pos
size: self.size
GridLayout:
size_hint_y: .3
cols:4
MyButton:
text: 'Rank'
size_hint_x: 0.5
MyButton:
text: 'Ratings'
MyButton:
text: 'Name'
size_hint_x: 2
MyButton:
text: 'Score'
on_press:
#arrange the boxing in ascending or descending order
RecycleView:
data: ['text': str(x) for x in app.new_new_sorted_data]
viewclass: 'SelectableLabel'
SelectableRecycleGridLayout:
cols: 4
default_size_hint: 1, None
size_hint_y: None
height: self.minimum_height
orientation: 'vertical'
multiselect: True
touch_multiselect: True
Button:
size_hint_y: .3
text: 'Press to Use Method'
on_press:
app.pre_function()
<SelectableLabel>:
canvas.before:
Color:
rgba: (.0, 0.9, .1, .3) if self.selected else (0, 0, 0, 1)
Rectangle:
pos: self.pos
size: self.size
<MyButton@Button>:
background_color: 0,0,0,1
"""
class SelectableLabel(RecycleDataViewBehavior, Label):
index = None
selected = BooleanProperty(False)
selectable = BooleanProperty(True)
def refresh_view_layout(self, rv, index, layout, viewport):
mod = index % 4
size_hints = [(0.5, None), (1, None), (2, None), (1, None)]
layout['size_hint'] = size_hints[mod]
colors = (1,1,1,1), (1,1,1,1), (1,1,1,1), (0,1,1,1)
layout['color'] = colors[mod]
super(SelectableLabel, self).refresh_view_layout(rv, index, layout, viewport)
def refresh_view_attrs(self, rv, index, data):
self.index = index
return super(SelectableLabel, self).refresh_view_attrs(
rv, index, data)
def on_touch_down(self, touch):
if super(SelectableLabel, self).on_touch_down(touch):
return True
if self.collide_point(*touch.pos) and self.selectable:
self.parent.select_with_touch(self.index, touch)
if self.index % 4 == 0:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index + 3)
return
elif self.index % 4 == 1:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 2:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 3:
self.parent.select_with_touch(self.index - 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 3)
return
index_list =
def apply_selection(self, rv, index, is_selected):
self.selected = is_selected
if is_selected:
self.index_list.append(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection changed to 0".format(rv.data[index]))
else:
try:
self.index_list.remove(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection removed for 0".format(rv.data[index]))
except:
pass
class SelectableRecycleGridLayout(FocusBehavior, LayoutSelectionBehavior, RecycleGridLayout):
pass
class MyApp(App):
store = JsonStore('file.json')
store.put('Example: 1', value_1 = 'Rating: C', value_2 = 10, value_3 = 'Zack')
store.put('Example: 2', value_1 = 'Rating: C', value_2 = 13, value_3 = 'Kate')
store.put('Example: 3', value_1 = 'Rating: A', value_2 = 32, value_3 = 'Pete')
store.put('Example: 4', value_1 = 'Rating: B', value_2 = 24, value_3 = 'Toby')
store.put('Example: 5', value_1 = 'Rating: D', value_2 = 03, value_3 = 'Lars')
# ---------------------- v Sorting Data v ----------------------
new_new_sorted_data = ListProperty()
def sort_data(self):
try:
self.store = JsonStore('file.json')
self.new_new_sorted_data =
json_data = open('./file.json')
score_data = json.load(json_data)
newlist =
newnewlist =
for x in score_data:
newlist.append(x)
for y in newlist:
newnewlist.append(score_data[str(y)])
print(newlist)
print(newnewlist)
sorted_data = sorted(newnewlist,key=itemgetter('value_2'), reverse = True)
new_sorted_data =
for z in sorted_data:
new_sorted_data.append(z.values())
for x,y,z in new_sorted_data:
self.new_new_sorted_data.append(newlist.pop())
self.new_new_sorted_data.append(x)
self.new_new_sorted_data.append(y)
self.new_new_sorted_data.append(z)
except:
pass
# ---------------------- ^ Sorting Data ^ ----------------------
# --------------------- v Deleting Data v ----------------------
only_key_values_list =
only_key_values =
def pre_function(self):
self.only_key_values_list =
self.only_key_values =
for value in SelectableLabel().index_list:
self.only_key_values_list.append(value.values())
for keys in self.only_key_values_list:
for key in keys:
try:
self.store.delete(str(key))
except:
pass
self.sort_data()
# --------------------- ^ Deleting Data ^ ----------------------
def __init__(self, **kwargs):
super(MyApp, self).__init__(**kwargs)
self.sort_data()
def build(self):
root_widget = Builder.load_string(kv_string)
return root_widget
if __name__ == "__main__":
MyApp().run()
add a comment |
I finally got my sample code to do what I wanted. I went through the MyApp
class to get to the SelectLabel
class. I also found a way to delete specific JSON keys through RecycleView
. I didn't comment this piece of code because it's just a sample, but if someone is using this as a reference and needs assistance, feel free to message me.
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.label import Label
from kivy.properties import BooleanProperty, ListProperty
from kivy.uix.behaviors import FocusBehavior
from kivy.storage.jsonstore import JsonStore
from kivy.uix.recyclegridlayout import RecycleGridLayout
from kivy.uix.recycleview.views import RecycleDataViewBehavior
from kivy.uix.recycleview.layout import LayoutSelectionBehavior
from operator import itemgetter
import json
kv_string = """
ScreenManager:
id: manager
Screen:
BoxLayout:
orientation: 'vertical'
canvas:
Color:
rgba: .2,.2,.5,1
Rectangle:
pos: self.pos
size: self.size
GridLayout:
size_hint_y: .3
cols:4
MyButton:
text: 'Rank'
size_hint_x: 0.5
MyButton:
text: 'Ratings'
MyButton:
text: 'Name'
size_hint_x: 2
MyButton:
text: 'Score'
on_press:
#arrange the boxing in ascending or descending order
RecycleView:
data: ['text': str(x) for x in app.new_new_sorted_data]
viewclass: 'SelectableLabel'
SelectableRecycleGridLayout:
cols: 4
default_size_hint: 1, None
size_hint_y: None
height: self.minimum_height
orientation: 'vertical'
multiselect: True
touch_multiselect: True
Button:
size_hint_y: .3
text: 'Press to Use Method'
on_press:
app.pre_function()
<SelectableLabel>:
canvas.before:
Color:
rgba: (.0, 0.9, .1, .3) if self.selected else (0, 0, 0, 1)
Rectangle:
pos: self.pos
size: self.size
<MyButton@Button>:
background_color: 0,0,0,1
"""
class SelectableLabel(RecycleDataViewBehavior, Label):
index = None
selected = BooleanProperty(False)
selectable = BooleanProperty(True)
def refresh_view_layout(self, rv, index, layout, viewport):
mod = index % 4
size_hints = [(0.5, None), (1, None), (2, None), (1, None)]
layout['size_hint'] = size_hints[mod]
colors = (1,1,1,1), (1,1,1,1), (1,1,1,1), (0,1,1,1)
layout['color'] = colors[mod]
super(SelectableLabel, self).refresh_view_layout(rv, index, layout, viewport)
def refresh_view_attrs(self, rv, index, data):
self.index = index
return super(SelectableLabel, self).refresh_view_attrs(
rv, index, data)
def on_touch_down(self, touch):
if super(SelectableLabel, self).on_touch_down(touch):
return True
if self.collide_point(*touch.pos) and self.selectable:
self.parent.select_with_touch(self.index, touch)
if self.index % 4 == 0:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index + 3)
return
elif self.index % 4 == 1:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 2:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 3:
self.parent.select_with_touch(self.index - 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 3)
return
index_list =
def apply_selection(self, rv, index, is_selected):
self.selected = is_selected
if is_selected:
self.index_list.append(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection changed to 0".format(rv.data[index]))
else:
try:
self.index_list.remove(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection removed for 0".format(rv.data[index]))
except:
pass
class SelectableRecycleGridLayout(FocusBehavior, LayoutSelectionBehavior, RecycleGridLayout):
pass
class MyApp(App):
store = JsonStore('file.json')
store.put('Example: 1', value_1 = 'Rating: C', value_2 = 10, value_3 = 'Zack')
store.put('Example: 2', value_1 = 'Rating: C', value_2 = 13, value_3 = 'Kate')
store.put('Example: 3', value_1 = 'Rating: A', value_2 = 32, value_3 = 'Pete')
store.put('Example: 4', value_1 = 'Rating: B', value_2 = 24, value_3 = 'Toby')
store.put('Example: 5', value_1 = 'Rating: D', value_2 = 03, value_3 = 'Lars')
# ---------------------- v Sorting Data v ----------------------
new_new_sorted_data = ListProperty()
def sort_data(self):
try:
self.store = JsonStore('file.json')
self.new_new_sorted_data =
json_data = open('./file.json')
score_data = json.load(json_data)
newlist =
newnewlist =
for x in score_data:
newlist.append(x)
for y in newlist:
newnewlist.append(score_data[str(y)])
print(newlist)
print(newnewlist)
sorted_data = sorted(newnewlist,key=itemgetter('value_2'), reverse = True)
new_sorted_data =
for z in sorted_data:
new_sorted_data.append(z.values())
for x,y,z in new_sorted_data:
self.new_new_sorted_data.append(newlist.pop())
self.new_new_sorted_data.append(x)
self.new_new_sorted_data.append(y)
self.new_new_sorted_data.append(z)
except:
pass
# ---------------------- ^ Sorting Data ^ ----------------------
# --------------------- v Deleting Data v ----------------------
only_key_values_list =
only_key_values =
def pre_function(self):
self.only_key_values_list =
self.only_key_values =
for value in SelectableLabel().index_list:
self.only_key_values_list.append(value.values())
for keys in self.only_key_values_list:
for key in keys:
try:
self.store.delete(str(key))
except:
pass
self.sort_data()
# --------------------- ^ Deleting Data ^ ----------------------
def __init__(self, **kwargs):
super(MyApp, self).__init__(**kwargs)
self.sort_data()
def build(self):
root_widget = Builder.load_string(kv_string)
return root_widget
if __name__ == "__main__":
MyApp().run()
I finally got my sample code to do what I wanted. I went through the MyApp
class to get to the SelectLabel
class. I also found a way to delete specific JSON keys through RecycleView
. I didn't comment this piece of code because it's just a sample, but if someone is using this as a reference and needs assistance, feel free to message me.
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.label import Label
from kivy.properties import BooleanProperty, ListProperty
from kivy.uix.behaviors import FocusBehavior
from kivy.storage.jsonstore import JsonStore
from kivy.uix.recyclegridlayout import RecycleGridLayout
from kivy.uix.recycleview.views import RecycleDataViewBehavior
from kivy.uix.recycleview.layout import LayoutSelectionBehavior
from operator import itemgetter
import json
kv_string = """
ScreenManager:
id: manager
Screen:
BoxLayout:
orientation: 'vertical'
canvas:
Color:
rgba: .2,.2,.5,1
Rectangle:
pos: self.pos
size: self.size
GridLayout:
size_hint_y: .3
cols:4
MyButton:
text: 'Rank'
size_hint_x: 0.5
MyButton:
text: 'Ratings'
MyButton:
text: 'Name'
size_hint_x: 2
MyButton:
text: 'Score'
on_press:
#arrange the boxing in ascending or descending order
RecycleView:
data: ['text': str(x) for x in app.new_new_sorted_data]
viewclass: 'SelectableLabel'
SelectableRecycleGridLayout:
cols: 4
default_size_hint: 1, None
size_hint_y: None
height: self.minimum_height
orientation: 'vertical'
multiselect: True
touch_multiselect: True
Button:
size_hint_y: .3
text: 'Press to Use Method'
on_press:
app.pre_function()
<SelectableLabel>:
canvas.before:
Color:
rgba: (.0, 0.9, .1, .3) if self.selected else (0, 0, 0, 1)
Rectangle:
pos: self.pos
size: self.size
<MyButton@Button>:
background_color: 0,0,0,1
"""
class SelectableLabel(RecycleDataViewBehavior, Label):
index = None
selected = BooleanProperty(False)
selectable = BooleanProperty(True)
def refresh_view_layout(self, rv, index, layout, viewport):
mod = index % 4
size_hints = [(0.5, None), (1, None), (2, None), (1, None)]
layout['size_hint'] = size_hints[mod]
colors = (1,1,1,1), (1,1,1,1), (1,1,1,1), (0,1,1,1)
layout['color'] = colors[mod]
super(SelectableLabel, self).refresh_view_layout(rv, index, layout, viewport)
def refresh_view_attrs(self, rv, index, data):
self.index = index
return super(SelectableLabel, self).refresh_view_attrs(
rv, index, data)
def on_touch_down(self, touch):
if super(SelectableLabel, self).on_touch_down(touch):
return True
if self.collide_point(*touch.pos) and self.selectable:
self.parent.select_with_touch(self.index, touch)
if self.index % 4 == 0:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index + 3)
return
elif self.index % 4 == 1:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index + 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 2:
self.parent.select_with_touch(self.index + 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index -1)
return
elif self.index % 4 == 3:
self.parent.select_with_touch(self.index - 1)
self.parent.select_with_touch(self.index - 2)
self.parent.select_with_touch(self.index - 3)
return
index_list =
def apply_selection(self, rv, index, is_selected):
self.selected = is_selected
if is_selected:
self.index_list.append(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection changed to 0".format(rv.data[index]))
else:
try:
self.index_list.remove(rv.data[index]) if index % 4 == 0 else self.index_list
#print("selection removed for 0".format(rv.data[index]))
except:
pass
class SelectableRecycleGridLayout(FocusBehavior, LayoutSelectionBehavior, RecycleGridLayout):
pass
class MyApp(App):
store = JsonStore('file.json')
store.put('Example: 1', value_1 = 'Rating: C', value_2 = 10, value_3 = 'Zack')
store.put('Example: 2', value_1 = 'Rating: C', value_2 = 13, value_3 = 'Kate')
store.put('Example: 3', value_1 = 'Rating: A', value_2 = 32, value_3 = 'Pete')
store.put('Example: 4', value_1 = 'Rating: B', value_2 = 24, value_3 = 'Toby')
store.put('Example: 5', value_1 = 'Rating: D', value_2 = 03, value_3 = 'Lars')
# ---------------------- v Sorting Data v ----------------------
new_new_sorted_data = ListProperty()
def sort_data(self):
try:
self.store = JsonStore('file.json')
self.new_new_sorted_data =
json_data = open('./file.json')
score_data = json.load(json_data)
newlist =
newnewlist =
for x in score_data:
newlist.append(x)
for y in newlist:
newnewlist.append(score_data[str(y)])
print(newlist)
print(newnewlist)
sorted_data = sorted(newnewlist,key=itemgetter('value_2'), reverse = True)
new_sorted_data =
for z in sorted_data:
new_sorted_data.append(z.values())
for x,y,z in new_sorted_data:
self.new_new_sorted_data.append(newlist.pop())
self.new_new_sorted_data.append(x)
self.new_new_sorted_data.append(y)
self.new_new_sorted_data.append(z)
except:
pass
# ---------------------- ^ Sorting Data ^ ----------------------
# --------------------- v Deleting Data v ----------------------
only_key_values_list =
only_key_values =
def pre_function(self):
self.only_key_values_list =
self.only_key_values =
for value in SelectableLabel().index_list:
self.only_key_values_list.append(value.values())
for keys in self.only_key_values_list:
for key in keys:
try:
self.store.delete(str(key))
except:
pass
self.sort_data()
# --------------------- ^ Deleting Data ^ ----------------------
def __init__(self, **kwargs):
super(MyApp, self).__init__(**kwargs)
self.sort_data()
def build(self):
root_widget = Builder.load_string(kv_string)
return root_widget
if __name__ == "__main__":
MyApp().run()
answered Nov 18 '18 at 1:43
Petar Luketina
447
447
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272586%2fkivy-delete-data-in-json-using-recycleview%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
x3OEoEKUfJP6evM,zslzgW6Q0XA,M HYRooEUz27pJ,JOkqb,Y6iMZ,vHwr1,3DJB05spALon,XtFaCyQrzC,6O6
Do you want to select n items and after pressing the button should you delete those items?
– eyllanesc
Nov 14 '18 at 2:13
Hey @eyllanesc , yes, I want to first select them then if I press the bottom button, they will be deleted. It seems like the method/function will have to be in
class SelectableLabel(RecycleDataViewBehavior, Label):
but I'm not sure how to access it, nor how to pass theindex_list
that is filled with the keys.– Petar Luketina
Nov 14 '18 at 19:12
that you must explain in your question, so I recommend you edit your question and add that information.
– eyllanesc
Nov 14 '18 at 19:14
Okay, I edited the description to make it clear. Thank you for letting me know!!!
– Petar Luketina
Nov 14 '18 at 23:59