Variable number of Oauth Authentication Schemes in .Net Core
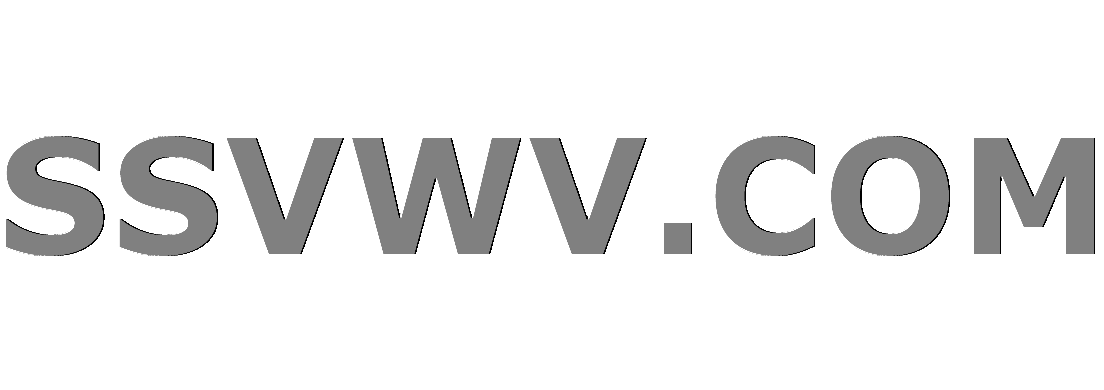
Multi tool use
I'm building a .NET Core service that needs to register an OAuth authentication scheme for each entry in a database. I'm using Autofac in addition to .NET's DI.
The problem is that in order to know how to add the Oauth schemes I need to be able to go to the database. Here's what I tried -
public IServiceProvider ConfigureServices(IServiceCollection services)
var builder = new ContainerBuilder();
var authBuilder = services.AddAuthentication(options =>
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = "OAuth1";
)
.AddCookie();
builder.Populate(services);
var container = builder.Build();
CreateAuths(authBuilder, container).Wait();
return new AutofacServiceProvider(container);
private async Task CreateAuths(AuthenticationBuilder authBuilder, IContainer container)
var dbService = container.Resolve<IDatabaseService>();
var things = await dbService.GetAllThings().ConfigureAwait(false);
foreach (var thing in things)
authBuilder.AddOAuth(thing.Name, CreateOAuthOptionsForThing(thing));
The problem with this is that I'm creating the Oauths to the AuthenticationBuilder AFTER I've called builder.Populate(services); so Autofac doesn't put the OAuths into the object graph.
Any ideas on how to do this correctly?
Thanks!
.net asp.net-core dependency-injection .net-core autofac
add a comment |
I'm building a .NET Core service that needs to register an OAuth authentication scheme for each entry in a database. I'm using Autofac in addition to .NET's DI.
The problem is that in order to know how to add the Oauth schemes I need to be able to go to the database. Here's what I tried -
public IServiceProvider ConfigureServices(IServiceCollection services)
var builder = new ContainerBuilder();
var authBuilder = services.AddAuthentication(options =>
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = "OAuth1";
)
.AddCookie();
builder.Populate(services);
var container = builder.Build();
CreateAuths(authBuilder, container).Wait();
return new AutofacServiceProvider(container);
private async Task CreateAuths(AuthenticationBuilder authBuilder, IContainer container)
var dbService = container.Resolve<IDatabaseService>();
var things = await dbService.GetAllThings().ConfigureAwait(false);
foreach (var thing in things)
authBuilder.AddOAuth(thing.Name, CreateOAuthOptionsForThing(thing));
The problem with this is that I'm creating the Oauths to the AuthenticationBuilder AFTER I've called builder.Populate(services); so Autofac doesn't put the OAuths into the object graph.
Any ideas on how to do this correctly?
Thanks!
.net asp.net-core dependency-injection .net-core autofac
You can resolve the IAuthenticationSchemeProvider (?) and add your schemes directly to that.
– Tratcher
Apr 12 '18 at 5:49
@Tratcher - can you provide any more detail? I'm struggling a bit. I seem to be able to add an auth scheme but I'm not sure how to hook up my OAuthOptions to that scheme. Here's another SO question which seems to be asking the same thing - stackoverflow.com/questions/48910070/…
– Nick
Apr 12 '18 at 17:10
add a comment |
I'm building a .NET Core service that needs to register an OAuth authentication scheme for each entry in a database. I'm using Autofac in addition to .NET's DI.
The problem is that in order to know how to add the Oauth schemes I need to be able to go to the database. Here's what I tried -
public IServiceProvider ConfigureServices(IServiceCollection services)
var builder = new ContainerBuilder();
var authBuilder = services.AddAuthentication(options =>
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = "OAuth1";
)
.AddCookie();
builder.Populate(services);
var container = builder.Build();
CreateAuths(authBuilder, container).Wait();
return new AutofacServiceProvider(container);
private async Task CreateAuths(AuthenticationBuilder authBuilder, IContainer container)
var dbService = container.Resolve<IDatabaseService>();
var things = await dbService.GetAllThings().ConfigureAwait(false);
foreach (var thing in things)
authBuilder.AddOAuth(thing.Name, CreateOAuthOptionsForThing(thing));
The problem with this is that I'm creating the Oauths to the AuthenticationBuilder AFTER I've called builder.Populate(services); so Autofac doesn't put the OAuths into the object graph.
Any ideas on how to do this correctly?
Thanks!
.net asp.net-core dependency-injection .net-core autofac
I'm building a .NET Core service that needs to register an OAuth authentication scheme for each entry in a database. I'm using Autofac in addition to .NET's DI.
The problem is that in order to know how to add the Oauth schemes I need to be able to go to the database. Here's what I tried -
public IServiceProvider ConfigureServices(IServiceCollection services)
var builder = new ContainerBuilder();
var authBuilder = services.AddAuthentication(options =>
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = "OAuth1";
)
.AddCookie();
builder.Populate(services);
var container = builder.Build();
CreateAuths(authBuilder, container).Wait();
return new AutofacServiceProvider(container);
private async Task CreateAuths(AuthenticationBuilder authBuilder, IContainer container)
var dbService = container.Resolve<IDatabaseService>();
var things = await dbService.GetAllThings().ConfigureAwait(false);
foreach (var thing in things)
authBuilder.AddOAuth(thing.Name, CreateOAuthOptionsForThing(thing));
The problem with this is that I'm creating the Oauths to the AuthenticationBuilder AFTER I've called builder.Populate(services); so Autofac doesn't put the OAuths into the object graph.
Any ideas on how to do this correctly?
Thanks!
.net asp.net-core dependency-injection .net-core autofac
.net asp.net-core dependency-injection .net-core autofac
asked Apr 12 '18 at 4:15
NickNick
168315
168315
You can resolve the IAuthenticationSchemeProvider (?) and add your schemes directly to that.
– Tratcher
Apr 12 '18 at 5:49
@Tratcher - can you provide any more detail? I'm struggling a bit. I seem to be able to add an auth scheme but I'm not sure how to hook up my OAuthOptions to that scheme. Here's another SO question which seems to be asking the same thing - stackoverflow.com/questions/48910070/…
– Nick
Apr 12 '18 at 17:10
add a comment |
You can resolve the IAuthenticationSchemeProvider (?) and add your schemes directly to that.
– Tratcher
Apr 12 '18 at 5:49
@Tratcher - can you provide any more detail? I'm struggling a bit. I seem to be able to add an auth scheme but I'm not sure how to hook up my OAuthOptions to that scheme. Here's another SO question which seems to be asking the same thing - stackoverflow.com/questions/48910070/…
– Nick
Apr 12 '18 at 17:10
You can resolve the IAuthenticationSchemeProvider (?) and add your schemes directly to that.
– Tratcher
Apr 12 '18 at 5:49
You can resolve the IAuthenticationSchemeProvider (?) and add your schemes directly to that.
– Tratcher
Apr 12 '18 at 5:49
@Tratcher - can you provide any more detail? I'm struggling a bit. I seem to be able to add an auth scheme but I'm not sure how to hook up my OAuthOptions to that scheme. Here's another SO question which seems to be asking the same thing - stackoverflow.com/questions/48910070/…
– Nick
Apr 12 '18 at 17:10
@Tratcher - can you provide any more detail? I'm struggling a bit. I seem to be able to add an auth scheme but I'm not sure how to hook up my OAuthOptions to that scheme. Here's another SO question which seems to be asking the same thing - stackoverflow.com/questions/48910070/…
– Nick
Apr 12 '18 at 17:10
add a comment |
1 Answer
1
active
oldest
votes
There's an example of how to do this here - https://github.com/aspnet/AuthSamples/tree/master/samples/DynamicSchemes
Keep in mind that for OAuth schemes, you'll have to do more then just calling schemeProvider.AddScheme and optionsCache.TryAdd - there's also a "postconfigure" step when adding options via the normal method. Here's the class - https://github.com/aspnet/Security/blob/master/src/Microsoft.AspNetCore.Authentication.OAuth/OAuthPostConfigureOptions.cs
So you can register the type "OAuthPostConfigureOptions<OAuthOptions, OAuthHandler<OAuthOptions>>" into your DI container then grab it via a constructor and call OAuthPostConfigureOptions.PostConfigure on your options before adding the options to the optionsCache.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49787907%2fvariable-number-of-oauth-authentication-schemes-in-net-core%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There's an example of how to do this here - https://github.com/aspnet/AuthSamples/tree/master/samples/DynamicSchemes
Keep in mind that for OAuth schemes, you'll have to do more then just calling schemeProvider.AddScheme and optionsCache.TryAdd - there's also a "postconfigure" step when adding options via the normal method. Here's the class - https://github.com/aspnet/Security/blob/master/src/Microsoft.AspNetCore.Authentication.OAuth/OAuthPostConfigureOptions.cs
So you can register the type "OAuthPostConfigureOptions<OAuthOptions, OAuthHandler<OAuthOptions>>" into your DI container then grab it via a constructor and call OAuthPostConfigureOptions.PostConfigure on your options before adding the options to the optionsCache.
add a comment |
There's an example of how to do this here - https://github.com/aspnet/AuthSamples/tree/master/samples/DynamicSchemes
Keep in mind that for OAuth schemes, you'll have to do more then just calling schemeProvider.AddScheme and optionsCache.TryAdd - there's also a "postconfigure" step when adding options via the normal method. Here's the class - https://github.com/aspnet/Security/blob/master/src/Microsoft.AspNetCore.Authentication.OAuth/OAuthPostConfigureOptions.cs
So you can register the type "OAuthPostConfigureOptions<OAuthOptions, OAuthHandler<OAuthOptions>>" into your DI container then grab it via a constructor and call OAuthPostConfigureOptions.PostConfigure on your options before adding the options to the optionsCache.
add a comment |
There's an example of how to do this here - https://github.com/aspnet/AuthSamples/tree/master/samples/DynamicSchemes
Keep in mind that for OAuth schemes, you'll have to do more then just calling schemeProvider.AddScheme and optionsCache.TryAdd - there's also a "postconfigure" step when adding options via the normal method. Here's the class - https://github.com/aspnet/Security/blob/master/src/Microsoft.AspNetCore.Authentication.OAuth/OAuthPostConfigureOptions.cs
So you can register the type "OAuthPostConfigureOptions<OAuthOptions, OAuthHandler<OAuthOptions>>" into your DI container then grab it via a constructor and call OAuthPostConfigureOptions.PostConfigure on your options before adding the options to the optionsCache.
There's an example of how to do this here - https://github.com/aspnet/AuthSamples/tree/master/samples/DynamicSchemes
Keep in mind that for OAuth schemes, you'll have to do more then just calling schemeProvider.AddScheme and optionsCache.TryAdd - there's also a "postconfigure" step when adding options via the normal method. Here's the class - https://github.com/aspnet/Security/blob/master/src/Microsoft.AspNetCore.Authentication.OAuth/OAuthPostConfigureOptions.cs
So you can register the type "OAuthPostConfigureOptions<OAuthOptions, OAuthHandler<OAuthOptions>>" into your DI container then grab it via a constructor and call OAuthPostConfigureOptions.PostConfigure on your options before adding the options to the optionsCache.
edited Nov 14 '18 at 22:35
answered Apr 13 '18 at 21:03
NickNick
168315
168315
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49787907%2fvariable-number-of-oauth-authentication-schemes-in-net-core%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0O0szQXZPj5n6YX sZp RLVA9J4d1fhu PHfg Ge,8C g3eG66iD47,YluKby39289yR,9
You can resolve the IAuthenticationSchemeProvider (?) and add your schemes directly to that.
– Tratcher
Apr 12 '18 at 5:49
@Tratcher - can you provide any more detail? I'm struggling a bit. I seem to be able to add an auth scheme but I'm not sure how to hook up my OAuthOptions to that scheme. Here's another SO question which seems to be asking the same thing - stackoverflow.com/questions/48910070/…
– Nick
Apr 12 '18 at 17:10