How to remake function that group list to simple recursion?
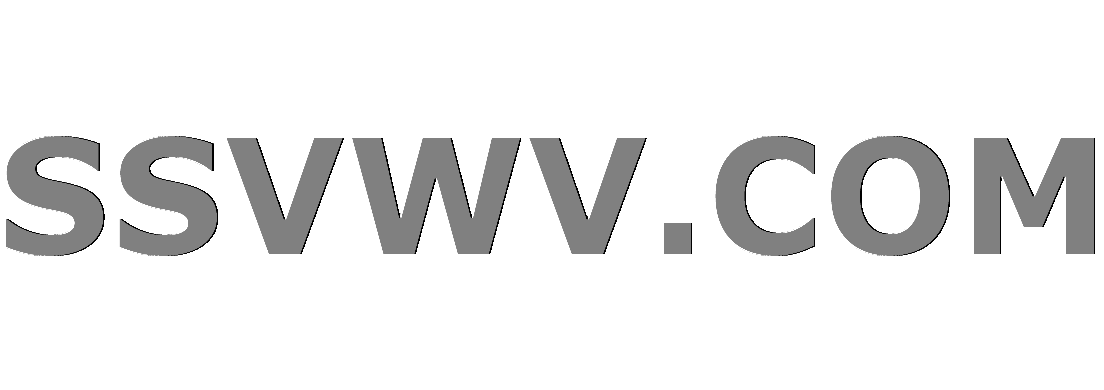
Multi tool use
up vote
0
down vote
favorite
I write function that group list elements by index, with odd index in first list, even in second. But I don not know how to make it with simple recursion and don not get type mismatch.
Here is the code:
// Simple recursion
def group1(list: List[Int]): (List[Int], List[Int]) = list match
case Nil => (Nil, Nil)
case head :: Nil => (List(head), Nil)
case head :: tail => // how can I make this case?
group1(List(2, 6, 7, 9, 0, 4, 1))
// Tail recursion
def group2(list: List[Int]): (List[Int], List[Int]) =
def group2Helper(list: List[Int], listA: List[Int], listB: List[Int]): (List[Int], List[Int]) = list match
case Nil => (listA.reverse, listB.reverse)
case head :: Nil => ((head :: listA).reverse, listB.reverse)
case head :: headNext :: tail => group2Helper(tail, head :: listA, headNext :: listB)
group2Helper(list, Nil, Nil)
group2(List(2, 6, 7, 9, 0, 4, 1))
scala function functional-programming
add a comment |
up vote
0
down vote
favorite
I write function that group list elements by index, with odd index in first list, even in second. But I don not know how to make it with simple recursion and don not get type mismatch.
Here is the code:
// Simple recursion
def group1(list: List[Int]): (List[Int], List[Int]) = list match
case Nil => (Nil, Nil)
case head :: Nil => (List(head), Nil)
case head :: tail => // how can I make this case?
group1(List(2, 6, 7, 9, 0, 4, 1))
// Tail recursion
def group2(list: List[Int]): (List[Int], List[Int]) =
def group2Helper(list: List[Int], listA: List[Int], listB: List[Int]): (List[Int], List[Int]) = list match
case Nil => (listA.reverse, listB.reverse)
case head :: Nil => ((head :: listA).reverse, listB.reverse)
case head :: headNext :: tail => group2Helper(tail, head :: listA, headNext :: listB)
group2Helper(list, Nil, Nil)
group2(List(2, 6, 7, 9, 0, 4, 1))
scala function functional-programming
What did you try that caused a type mismatch?
– Bergi
Nov 12 at 7:38
Don't forget to addheadNext
(as inhead :: next :: tail
) to the simple recursion as well
– Bergi
Nov 12 at 7:38
@Bergi if I trying to do this function like another with simple recursion like this: case head :: headnext :: tail => (head :: group1(List(tail.head)), headnext :: group1(tail.tail)) It gives me mismatch because I can not add head element to (_, _), sorry I don not know how it calls on English. This function is not correct, here it is going about idea
– Ilya
Nov 12 at 7:48
2
You don't want togroup1
the.head
and.tail
oftail
- you just want togroup1(tail)
. And thengroup1(tail)
will return a tuple of two lists. You will want to prependhead
to the first andheadNext
to the second one. Use temporary variables so that you need to callgroup1(tail)
only once and so that you can break up the result into the two lists.
– Bergi
Nov 12 at 8:02
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I write function that group list elements by index, with odd index in first list, even in second. But I don not know how to make it with simple recursion and don not get type mismatch.
Here is the code:
// Simple recursion
def group1(list: List[Int]): (List[Int], List[Int]) = list match
case Nil => (Nil, Nil)
case head :: Nil => (List(head), Nil)
case head :: tail => // how can I make this case?
group1(List(2, 6, 7, 9, 0, 4, 1))
// Tail recursion
def group2(list: List[Int]): (List[Int], List[Int]) =
def group2Helper(list: List[Int], listA: List[Int], listB: List[Int]): (List[Int], List[Int]) = list match
case Nil => (listA.reverse, listB.reverse)
case head :: Nil => ((head :: listA).reverse, listB.reverse)
case head :: headNext :: tail => group2Helper(tail, head :: listA, headNext :: listB)
group2Helper(list, Nil, Nil)
group2(List(2, 6, 7, 9, 0, 4, 1))
scala function functional-programming
I write function that group list elements by index, with odd index in first list, even in second. But I don not know how to make it with simple recursion and don not get type mismatch.
Here is the code:
// Simple recursion
def group1(list: List[Int]): (List[Int], List[Int]) = list match
case Nil => (Nil, Nil)
case head :: Nil => (List(head), Nil)
case head :: tail => // how can I make this case?
group1(List(2, 6, 7, 9, 0, 4, 1))
// Tail recursion
def group2(list: List[Int]): (List[Int], List[Int]) =
def group2Helper(list: List[Int], listA: List[Int], listB: List[Int]): (List[Int], List[Int]) = list match
case Nil => (listA.reverse, listB.reverse)
case head :: Nil => ((head :: listA).reverse, listB.reverse)
case head :: headNext :: tail => group2Helper(tail, head :: listA, headNext :: listB)
group2Helper(list, Nil, Nil)
group2(List(2, 6, 7, 9, 0, 4, 1))
scala function functional-programming
scala function functional-programming
asked Nov 12 at 7:31
Ilya
335
335
What did you try that caused a type mismatch?
– Bergi
Nov 12 at 7:38
Don't forget to addheadNext
(as inhead :: next :: tail
) to the simple recursion as well
– Bergi
Nov 12 at 7:38
@Bergi if I trying to do this function like another with simple recursion like this: case head :: headnext :: tail => (head :: group1(List(tail.head)), headnext :: group1(tail.tail)) It gives me mismatch because I can not add head element to (_, _), sorry I don not know how it calls on English. This function is not correct, here it is going about idea
– Ilya
Nov 12 at 7:48
2
You don't want togroup1
the.head
and.tail
oftail
- you just want togroup1(tail)
. And thengroup1(tail)
will return a tuple of two lists. You will want to prependhead
to the first andheadNext
to the second one. Use temporary variables so that you need to callgroup1(tail)
only once and so that you can break up the result into the two lists.
– Bergi
Nov 12 at 8:02
add a comment |
What did you try that caused a type mismatch?
– Bergi
Nov 12 at 7:38
Don't forget to addheadNext
(as inhead :: next :: tail
) to the simple recursion as well
– Bergi
Nov 12 at 7:38
@Bergi if I trying to do this function like another with simple recursion like this: case head :: headnext :: tail => (head :: group1(List(tail.head)), headnext :: group1(tail.tail)) It gives me mismatch because I can not add head element to (_, _), sorry I don not know how it calls on English. This function is not correct, here it is going about idea
– Ilya
Nov 12 at 7:48
2
You don't want togroup1
the.head
and.tail
oftail
- you just want togroup1(tail)
. And thengroup1(tail)
will return a tuple of two lists. You will want to prependhead
to the first andheadNext
to the second one. Use temporary variables so that you need to callgroup1(tail)
only once and so that you can break up the result into the two lists.
– Bergi
Nov 12 at 8:02
What did you try that caused a type mismatch?
– Bergi
Nov 12 at 7:38
What did you try that caused a type mismatch?
– Bergi
Nov 12 at 7:38
Don't forget to add
headNext
(as in head :: next :: tail
) to the simple recursion as well– Bergi
Nov 12 at 7:38
Don't forget to add
headNext
(as in head :: next :: tail
) to the simple recursion as well– Bergi
Nov 12 at 7:38
@Bergi if I trying to do this function like another with simple recursion like this: case head :: headnext :: tail => (head :: group1(List(tail.head)), headnext :: group1(tail.tail)) It gives me mismatch because I can not add head element to (_, _), sorry I don not know how it calls on English. This function is not correct, here it is going about idea
– Ilya
Nov 12 at 7:48
@Bergi if I trying to do this function like another with simple recursion like this: case head :: headnext :: tail => (head :: group1(List(tail.head)), headnext :: group1(tail.tail)) It gives me mismatch because I can not add head element to (_, _), sorry I don not know how it calls on English. This function is not correct, here it is going about idea
– Ilya
Nov 12 at 7:48
2
2
You don't want to
group1
the .head
and .tail
of tail
- you just want to group1(tail)
. And then group1(tail)
will return a tuple of two lists. You will want to prepend head
to the first and headNext
to the second one. Use temporary variables so that you need to call group1(tail)
only once and so that you can break up the result into the two lists.– Bergi
Nov 12 at 8:02
You don't want to
group1
the .head
and .tail
of tail
- you just want to group1(tail)
. And then group1(tail)
will return a tuple of two lists. You will want to prepend head
to the first and headNext
to the second one. Use temporary variables so that you need to call group1(tail)
only once and so that you can break up the result into the two lists.– Bergi
Nov 12 at 8:02
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
You have to invoke the next recursion, unpack the result tuple, pre-pend each head element to the proper List
, and repackage the new result tuple.
def group1(list: List[Int]) :(List[Int], List[Int]) = list match
case Nil => (Nil, Nil)
case head :: Nil => (List(head), Nil)
case hdA :: hdB :: tail => val (lstA, lstB) = group1(tail)
(hdA :: lstA, hdB :: lstB)
Thanks, now I understand how to use variable in case. But I don not know is it still functional way programming? Or this variable does not like a statement or imperative style?
– Ilya
Nov 12 at 8:43
1
Thisgroup1()
method creates 7 variables. They are (in order)list
,head
,hdA
,hdB
,tail
,lstA
, andlstB
. Every one of them is aval
(i.e. immutable) so it doesn't conflict with the tenets of Functional Programming.
– jwvh
Nov 12 at 9:46
Thanks a lot for explaining
– Ilya
Nov 12 at 11:10
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257596%2fhow-to-remake-function-that-group-list-to-simple-recursion%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You have to invoke the next recursion, unpack the result tuple, pre-pend each head element to the proper List
, and repackage the new result tuple.
def group1(list: List[Int]) :(List[Int], List[Int]) = list match
case Nil => (Nil, Nil)
case head :: Nil => (List(head), Nil)
case hdA :: hdB :: tail => val (lstA, lstB) = group1(tail)
(hdA :: lstA, hdB :: lstB)
Thanks, now I understand how to use variable in case. But I don not know is it still functional way programming? Or this variable does not like a statement or imperative style?
– Ilya
Nov 12 at 8:43
1
Thisgroup1()
method creates 7 variables. They are (in order)list
,head
,hdA
,hdB
,tail
,lstA
, andlstB
. Every one of them is aval
(i.e. immutable) so it doesn't conflict with the tenets of Functional Programming.
– jwvh
Nov 12 at 9:46
Thanks a lot for explaining
– Ilya
Nov 12 at 11:10
add a comment |
up vote
2
down vote
accepted
You have to invoke the next recursion, unpack the result tuple, pre-pend each head element to the proper List
, and repackage the new result tuple.
def group1(list: List[Int]) :(List[Int], List[Int]) = list match
case Nil => (Nil, Nil)
case head :: Nil => (List(head), Nil)
case hdA :: hdB :: tail => val (lstA, lstB) = group1(tail)
(hdA :: lstA, hdB :: lstB)
Thanks, now I understand how to use variable in case. But I don not know is it still functional way programming? Or this variable does not like a statement or imperative style?
– Ilya
Nov 12 at 8:43
1
Thisgroup1()
method creates 7 variables. They are (in order)list
,head
,hdA
,hdB
,tail
,lstA
, andlstB
. Every one of them is aval
(i.e. immutable) so it doesn't conflict with the tenets of Functional Programming.
– jwvh
Nov 12 at 9:46
Thanks a lot for explaining
– Ilya
Nov 12 at 11:10
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You have to invoke the next recursion, unpack the result tuple, pre-pend each head element to the proper List
, and repackage the new result tuple.
def group1(list: List[Int]) :(List[Int], List[Int]) = list match
case Nil => (Nil, Nil)
case head :: Nil => (List(head), Nil)
case hdA :: hdB :: tail => val (lstA, lstB) = group1(tail)
(hdA :: lstA, hdB :: lstB)
You have to invoke the next recursion, unpack the result tuple, pre-pend each head element to the proper List
, and repackage the new result tuple.
def group1(list: List[Int]) :(List[Int], List[Int]) = list match
case Nil => (Nil, Nil)
case head :: Nil => (List(head), Nil)
case hdA :: hdB :: tail => val (lstA, lstB) = group1(tail)
(hdA :: lstA, hdB :: lstB)
answered Nov 12 at 8:16
jwvh
25.3k52038
25.3k52038
Thanks, now I understand how to use variable in case. But I don not know is it still functional way programming? Or this variable does not like a statement or imperative style?
– Ilya
Nov 12 at 8:43
1
Thisgroup1()
method creates 7 variables. They are (in order)list
,head
,hdA
,hdB
,tail
,lstA
, andlstB
. Every one of them is aval
(i.e. immutable) so it doesn't conflict with the tenets of Functional Programming.
– jwvh
Nov 12 at 9:46
Thanks a lot for explaining
– Ilya
Nov 12 at 11:10
add a comment |
Thanks, now I understand how to use variable in case. But I don not know is it still functional way programming? Or this variable does not like a statement or imperative style?
– Ilya
Nov 12 at 8:43
1
Thisgroup1()
method creates 7 variables. They are (in order)list
,head
,hdA
,hdB
,tail
,lstA
, andlstB
. Every one of them is aval
(i.e. immutable) so it doesn't conflict with the tenets of Functional Programming.
– jwvh
Nov 12 at 9:46
Thanks a lot for explaining
– Ilya
Nov 12 at 11:10
Thanks, now I understand how to use variable in case. But I don not know is it still functional way programming? Or this variable does not like a statement or imperative style?
– Ilya
Nov 12 at 8:43
Thanks, now I understand how to use variable in case. But I don not know is it still functional way programming? Or this variable does not like a statement or imperative style?
– Ilya
Nov 12 at 8:43
1
1
This
group1()
method creates 7 variables. They are (in order) list
, head
, hdA
, hdB
, tail
, lstA
, and lstB
. Every one of them is a val
(i.e. immutable) so it doesn't conflict with the tenets of Functional Programming.– jwvh
Nov 12 at 9:46
This
group1()
method creates 7 variables. They are (in order) list
, head
, hdA
, hdB
, tail
, lstA
, and lstB
. Every one of them is a val
(i.e. immutable) so it doesn't conflict with the tenets of Functional Programming.– jwvh
Nov 12 at 9:46
Thanks a lot for explaining
– Ilya
Nov 12 at 11:10
Thanks a lot for explaining
– Ilya
Nov 12 at 11:10
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257596%2fhow-to-remake-function-that-group-list-to-simple-recursion%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4b0UmrT U2m3qBC9E6 R494zyushNrl,Rlgb,Yx8NGbK yfavmaB 67Nxj16dQ
What did you try that caused a type mismatch?
– Bergi
Nov 12 at 7:38
Don't forget to add
headNext
(as inhead :: next :: tail
) to the simple recursion as well– Bergi
Nov 12 at 7:38
@Bergi if I trying to do this function like another with simple recursion like this: case head :: headnext :: tail => (head :: group1(List(tail.head)), headnext :: group1(tail.tail)) It gives me mismatch because I can not add head element to (_, _), sorry I don not know how it calls on English. This function is not correct, here it is going about idea
– Ilya
Nov 12 at 7:48
2
You don't want to
group1
the.head
and.tail
oftail
- you just want togroup1(tail)
. And thengroup1(tail)
will return a tuple of two lists. You will want to prependhead
to the first andheadNext
to the second one. Use temporary variables so that you need to callgroup1(tail)
only once and so that you can break up the result into the two lists.– Bergi
Nov 12 at 8:02