How to save keras model in WML repository in Watson Studio?
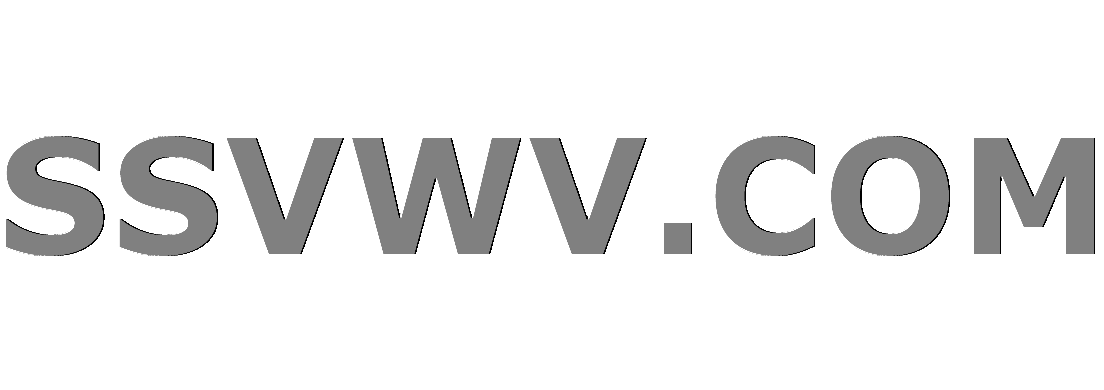
Multi tool use
I am trying to deploy Keras model by training on MNIST dataset on Watson studio but unable to save and successfully deploy it.
When I am trying to save the model object, it says it can't save Sequential Object.
When I am trying to convert hd5 to tgz and save it, it gets saved but on deployment I get error
"{"code":"load_model_failure","message":"SavedModel file does not exist at: /opt/ibm/s..."
When I am trying to deploy hd5 file, it says its not in compressed format.
Can any help me how exactly to save keras model and deploy it on watson studio?
#
convert class vectors to binary class matrices
y_train = keras.utils.to_categorical(y_train, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
model = Sequential()
model.add(Conv2D(32, kernel_size=(3, 3),
activation='relu',
input_shape=input_shape))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(num_classes, activation='softmax'))
model.compile(loss=keras.losses.categorical_crossentropy,
optimizer=keras.optimizers.Adadelta(),
metrics=['accuracy'])
model.fit(x_train, y_train,
batch_size=batch_size,
epochs=epochs,
verbose=1,
validation_data=(x_test, y_test))
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
model_result_path = "keras_model.h5"
model.save(model_result_path)
published_model = client.repository.store_model(model='keras_model.h5', meta_props=model_props,training_data=x_train, training_target=y_train)
python tensorflow keras deep-learning ibm-watson
add a comment |
I am trying to deploy Keras model by training on MNIST dataset on Watson studio but unable to save and successfully deploy it.
When I am trying to save the model object, it says it can't save Sequential Object.
When I am trying to convert hd5 to tgz and save it, it gets saved but on deployment I get error
"{"code":"load_model_failure","message":"SavedModel file does not exist at: /opt/ibm/s..."
When I am trying to deploy hd5 file, it says its not in compressed format.
Can any help me how exactly to save keras model and deploy it on watson studio?
#
convert class vectors to binary class matrices
y_train = keras.utils.to_categorical(y_train, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
model = Sequential()
model.add(Conv2D(32, kernel_size=(3, 3),
activation='relu',
input_shape=input_shape))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(num_classes, activation='softmax'))
model.compile(loss=keras.losses.categorical_crossentropy,
optimizer=keras.optimizers.Adadelta(),
metrics=['accuracy'])
model.fit(x_train, y_train,
batch_size=batch_size,
epochs=epochs,
verbose=1,
validation_data=(x_test, y_test))
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
model_result_path = "keras_model.h5"
model.save(model_result_path)
published_model = client.repository.store_model(model='keras_model.h5', meta_props=model_props,training_data=x_train, training_target=y_train)
python tensorflow keras deep-learning ibm-watson
add a comment |
I am trying to deploy Keras model by training on MNIST dataset on Watson studio but unable to save and successfully deploy it.
When I am trying to save the model object, it says it can't save Sequential Object.
When I am trying to convert hd5 to tgz and save it, it gets saved but on deployment I get error
"{"code":"load_model_failure","message":"SavedModel file does not exist at: /opt/ibm/s..."
When I am trying to deploy hd5 file, it says its not in compressed format.
Can any help me how exactly to save keras model and deploy it on watson studio?
#
convert class vectors to binary class matrices
y_train = keras.utils.to_categorical(y_train, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
model = Sequential()
model.add(Conv2D(32, kernel_size=(3, 3),
activation='relu',
input_shape=input_shape))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(num_classes, activation='softmax'))
model.compile(loss=keras.losses.categorical_crossentropy,
optimizer=keras.optimizers.Adadelta(),
metrics=['accuracy'])
model.fit(x_train, y_train,
batch_size=batch_size,
epochs=epochs,
verbose=1,
validation_data=(x_test, y_test))
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
model_result_path = "keras_model.h5"
model.save(model_result_path)
published_model = client.repository.store_model(model='keras_model.h5', meta_props=model_props,training_data=x_train, training_target=y_train)
python tensorflow keras deep-learning ibm-watson
I am trying to deploy Keras model by training on MNIST dataset on Watson studio but unable to save and successfully deploy it.
When I am trying to save the model object, it says it can't save Sequential Object.
When I am trying to convert hd5 to tgz and save it, it gets saved but on deployment I get error
"{"code":"load_model_failure","message":"SavedModel file does not exist at: /opt/ibm/s..."
When I am trying to deploy hd5 file, it says its not in compressed format.
Can any help me how exactly to save keras model and deploy it on watson studio?
#
convert class vectors to binary class matrices
y_train = keras.utils.to_categorical(y_train, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
model = Sequential()
model.add(Conv2D(32, kernel_size=(3, 3),
activation='relu',
input_shape=input_shape))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(num_classes, activation='softmax'))
model.compile(loss=keras.losses.categorical_crossentropy,
optimizer=keras.optimizers.Adadelta(),
metrics=['accuracy'])
model.fit(x_train, y_train,
batch_size=batch_size,
epochs=epochs,
verbose=1,
validation_data=(x_test, y_test))
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
model_result_path = "keras_model.h5"
model.save(model_result_path)
published_model = client.repository.store_model(model='keras_model.h5', meta_props=model_props,training_data=x_train, training_target=y_train)
python tensorflow keras deep-learning ibm-watson
python tensorflow keras deep-learning ibm-watson
edited Nov 12 at 12:53
Milo Lu
1,57511327
1,57511327
asked Nov 12 at 8:23
Umair Shaikh
112
112
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Have you tried just passing the Python model object itself to the store_model
function?
For example, see section 4.2 of this sample notebook:
https://dataplatform.cloud.ibm.com/exchange/public/entry/view/1438a61212a64ac435c837ba040e6934
Yes I have tried that. I wrote that in my question that it doesn't take Sequential models directly.
– Umair Shaikh
Nov 14 at 8:20
add a comment |
can you try compressing the h5 file(i.e forming a tar.gz) and then try giving it to the client.repository.store_model instead of directly giving .h5 file.
Yes I have tried that as well. I compressed .h5 file into tar.gz. It stores the model but then it gives error on deployment.
– Umair Shaikh
Nov 14 at 8:22
add a comment |
You will have to provide the path to the compressed keras file.
E.g.:
keras_file_path = "/Users/jsmith/keras/ker_seq_mnist_model.tar.gz"
published_model = client.repository.store_model(model=keras_file_path, meta_props=model_props,training_data=x_train, training_target=y_train)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53258236%2fhow-to-save-keras-model-in-wml-repository-in-watson-studio%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Have you tried just passing the Python model object itself to the store_model
function?
For example, see section 4.2 of this sample notebook:
https://dataplatform.cloud.ibm.com/exchange/public/entry/view/1438a61212a64ac435c837ba040e6934
Yes I have tried that. I wrote that in my question that it doesn't take Sequential models directly.
– Umair Shaikh
Nov 14 at 8:20
add a comment |
Have you tried just passing the Python model object itself to the store_model
function?
For example, see section 4.2 of this sample notebook:
https://dataplatform.cloud.ibm.com/exchange/public/entry/view/1438a61212a64ac435c837ba040e6934
Yes I have tried that. I wrote that in my question that it doesn't take Sequential models directly.
– Umair Shaikh
Nov 14 at 8:20
add a comment |
Have you tried just passing the Python model object itself to the store_model
function?
For example, see section 4.2 of this sample notebook:
https://dataplatform.cloud.ibm.com/exchange/public/entry/view/1438a61212a64ac435c837ba040e6934
Have you tried just passing the Python model object itself to the store_model
function?
For example, see section 4.2 of this sample notebook:
https://dataplatform.cloud.ibm.com/exchange/public/entry/view/1438a61212a64ac435c837ba040e6934
answered Nov 12 at 17:51


Sarah Packowski
1
1
Yes I have tried that. I wrote that in my question that it doesn't take Sequential models directly.
– Umair Shaikh
Nov 14 at 8:20
add a comment |
Yes I have tried that. I wrote that in my question that it doesn't take Sequential models directly.
– Umair Shaikh
Nov 14 at 8:20
Yes I have tried that. I wrote that in my question that it doesn't take Sequential models directly.
– Umair Shaikh
Nov 14 at 8:20
Yes I have tried that. I wrote that in my question that it doesn't take Sequential models directly.
– Umair Shaikh
Nov 14 at 8:20
add a comment |
can you try compressing the h5 file(i.e forming a tar.gz) and then try giving it to the client.repository.store_model instead of directly giving .h5 file.
Yes I have tried that as well. I compressed .h5 file into tar.gz. It stores the model but then it gives error on deployment.
– Umair Shaikh
Nov 14 at 8:22
add a comment |
can you try compressing the h5 file(i.e forming a tar.gz) and then try giving it to the client.repository.store_model instead of directly giving .h5 file.
Yes I have tried that as well. I compressed .h5 file into tar.gz. It stores the model but then it gives error on deployment.
– Umair Shaikh
Nov 14 at 8:22
add a comment |
can you try compressing the h5 file(i.e forming a tar.gz) and then try giving it to the client.repository.store_model instead of directly giving .h5 file.
can you try compressing the h5 file(i.e forming a tar.gz) and then try giving it to the client.repository.store_model instead of directly giving .h5 file.
answered Nov 14 at 4:06
Nagireddy Hanisha
298422
298422
Yes I have tried that as well. I compressed .h5 file into tar.gz. It stores the model but then it gives error on deployment.
– Umair Shaikh
Nov 14 at 8:22
add a comment |
Yes I have tried that as well. I compressed .h5 file into tar.gz. It stores the model but then it gives error on deployment.
– Umair Shaikh
Nov 14 at 8:22
Yes I have tried that as well. I compressed .h5 file into tar.gz. It stores the model but then it gives error on deployment.
– Umair Shaikh
Nov 14 at 8:22
Yes I have tried that as well. I compressed .h5 file into tar.gz. It stores the model but then it gives error on deployment.
– Umair Shaikh
Nov 14 at 8:22
add a comment |
You will have to provide the path to the compressed keras file.
E.g.:
keras_file_path = "/Users/jsmith/keras/ker_seq_mnist_model.tar.gz"
published_model = client.repository.store_model(model=keras_file_path, meta_props=model_props,training_data=x_train, training_target=y_train)
add a comment |
You will have to provide the path to the compressed keras file.
E.g.:
keras_file_path = "/Users/jsmith/keras/ker_seq_mnist_model.tar.gz"
published_model = client.repository.store_model(model=keras_file_path, meta_props=model_props,training_data=x_train, training_target=y_train)
add a comment |
You will have to provide the path to the compressed keras file.
E.g.:
keras_file_path = "/Users/jsmith/keras/ker_seq_mnist_model.tar.gz"
published_model = client.repository.store_model(model=keras_file_path, meta_props=model_props,training_data=x_train, training_target=y_train)
You will have to provide the path to the compressed keras file.
E.g.:
keras_file_path = "/Users/jsmith/keras/ker_seq_mnist_model.tar.gz"
published_model = client.repository.store_model(model=keras_file_path, meta_props=model_props,training_data=x_train, training_target=y_train)
edited Nov 15 at 10:24


Kokogino
6761115
6761115
answered Nov 15 at 9:30
Roopa Mahendra
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53258236%2fhow-to-save-keras-model-in-wml-repository-in-watson-studio%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
O6 K,XcbVO0YkTe1Zh,0ht5XQhw7j667KLgESL3F2tcmgYpyn4t mjJeuAztb R jS440a,UXKCvFpc g7a,bLmUu,Z