Checking if a document exists in a Firestore collection
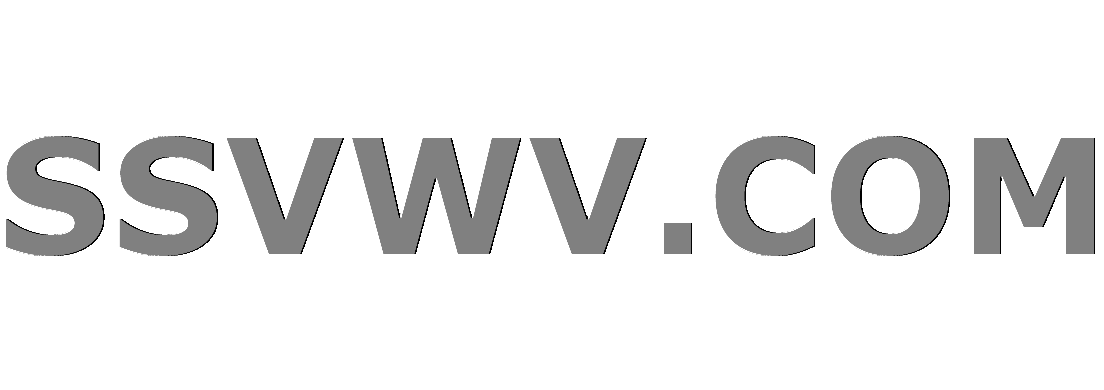
Multi tool use
I have a Firestore collection tree in which I plan to store only one document. I want to check if that collection contains that document and if so, retrieve the id of the document! Any ideas/thoughts?
Thanks :)
java

add a comment |
I have a Firestore collection tree in which I plan to store only one document. I want to check if that collection contains that document and if so, retrieve the id of the document! Any ideas/thoughts?
Thanks :)
java

add a comment |
I have a Firestore collection tree in which I plan to store only one document. I want to check if that collection contains that document and if so, retrieve the id of the document! Any ideas/thoughts?
Thanks :)
java

I have a Firestore collection tree in which I plan to store only one document. I want to check if that collection contains that document and if so, retrieve the id of the document! Any ideas/thoughts?
Thanks :)
java

java

edited Nov 16 '18 at 10:20


Alex Mamo
46.6k82965
46.6k82965
asked Nov 16 '18 at 6:20


Emil SharierEmil Sharier
165
165
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Try this one.
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
firestore.collection("users").whereEqualTo("user_uid", user.getUid())
.limit(1).get()
.addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
boolean isEmpty = task.getResult().isEmpty();
);
add a comment |
Make the document structure like this one:
collectionName(Collection) -> documentId(Document) -> id = documentId(and other fields)
Now make a query:
FirebaseFirestore firestoreDatabase= FirebaseFirestore.getInstance();
firestoreDatabase.collection(COLLECTION_NAME)
.whereEqualTo(DOCUMENT_ID, documentId)
.get()
.addOnCompleteListener(task ->
if (task.isSuccessful())
if (task.getResult().getDocuments().size() > 0)
// Here is your document with id
);
add a comment |
If you want to check a particular document for existence based on its document id
, please use the following lines of code:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
DocumentReference docIdRef = rootRef.collection("yourCollection").document(docId);
docIdRef.get().addOnCompleteListener(new OnCompleteListener<DocumentSnapshot>()
@Override
public void onComplete(@NonNull Task<DocumentSnapshot> task)
if (task.isSuccessful())
DocumentSnapshot document = task.getResult();
if (document.exists())
Log.d(TAG, "Document exists!");
else
Log.d(TAG, "Document does not exist!");
else
Log.d(TAG, "Failed with: ", task.getException());
);
If you don't have the document id, you need to use a query and find that document according to a value of a specific property like this:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
CollectionReference yourCollRef = rootRef.collection("yourCollection");
Query query = yourCollRef.whereEqualTo("yourPropery", "yourValue");
query.get().addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
for (QueryDocumentSnapshot document : task.getResult())
Log.d(TAG, document.getId() + " => " + document.getData());
else
Log.d(TAG, "Error getting documents: ", task.getException());
);
Hi Emil! Have you tried my solutions above, does it work?
– Alex Mamo
Nov 17 '18 at 8:57
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53332471%2fchecking-if-a-document-exists-in-a-firestore-collection%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try this one.
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
firestore.collection("users").whereEqualTo("user_uid", user.getUid())
.limit(1).get()
.addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
boolean isEmpty = task.getResult().isEmpty();
);
add a comment |
Try this one.
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
firestore.collection("users").whereEqualTo("user_uid", user.getUid())
.limit(1).get()
.addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
boolean isEmpty = task.getResult().isEmpty();
);
add a comment |
Try this one.
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
firestore.collection("users").whereEqualTo("user_uid", user.getUid())
.limit(1).get()
.addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
boolean isEmpty = task.getResult().isEmpty();
);
Try this one.
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
firestore.collection("users").whereEqualTo("user_uid", user.getUid())
.limit(1).get()
.addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
boolean isEmpty = task.getResult().isEmpty();
);
answered Nov 16 '18 at 6:31
HussainAbbasHussainAbbas
799
799
add a comment |
add a comment |
Make the document structure like this one:
collectionName(Collection) -> documentId(Document) -> id = documentId(and other fields)
Now make a query:
FirebaseFirestore firestoreDatabase= FirebaseFirestore.getInstance();
firestoreDatabase.collection(COLLECTION_NAME)
.whereEqualTo(DOCUMENT_ID, documentId)
.get()
.addOnCompleteListener(task ->
if (task.isSuccessful())
if (task.getResult().getDocuments().size() > 0)
// Here is your document with id
);
add a comment |
Make the document structure like this one:
collectionName(Collection) -> documentId(Document) -> id = documentId(and other fields)
Now make a query:
FirebaseFirestore firestoreDatabase= FirebaseFirestore.getInstance();
firestoreDatabase.collection(COLLECTION_NAME)
.whereEqualTo(DOCUMENT_ID, documentId)
.get()
.addOnCompleteListener(task ->
if (task.isSuccessful())
if (task.getResult().getDocuments().size() > 0)
// Here is your document with id
);
add a comment |
Make the document structure like this one:
collectionName(Collection) -> documentId(Document) -> id = documentId(and other fields)
Now make a query:
FirebaseFirestore firestoreDatabase= FirebaseFirestore.getInstance();
firestoreDatabase.collection(COLLECTION_NAME)
.whereEqualTo(DOCUMENT_ID, documentId)
.get()
.addOnCompleteListener(task ->
if (task.isSuccessful())
if (task.getResult().getDocuments().size() > 0)
// Here is your document with id
);
Make the document structure like this one:
collectionName(Collection) -> documentId(Document) -> id = documentId(and other fields)
Now make a query:
FirebaseFirestore firestoreDatabase= FirebaseFirestore.getInstance();
firestoreDatabase.collection(COLLECTION_NAME)
.whereEqualTo(DOCUMENT_ID, documentId)
.get()
.addOnCompleteListener(task ->
if (task.isSuccessful())
if (task.getResult().getDocuments().size() > 0)
// Here is your document with id
);
answered Nov 16 '18 at 6:34
Rehman Murad AliRehman Murad Ali
274
274
add a comment |
add a comment |
If you want to check a particular document for existence based on its document id
, please use the following lines of code:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
DocumentReference docIdRef = rootRef.collection("yourCollection").document(docId);
docIdRef.get().addOnCompleteListener(new OnCompleteListener<DocumentSnapshot>()
@Override
public void onComplete(@NonNull Task<DocumentSnapshot> task)
if (task.isSuccessful())
DocumentSnapshot document = task.getResult();
if (document.exists())
Log.d(TAG, "Document exists!");
else
Log.d(TAG, "Document does not exist!");
else
Log.d(TAG, "Failed with: ", task.getException());
);
If you don't have the document id, you need to use a query and find that document according to a value of a specific property like this:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
CollectionReference yourCollRef = rootRef.collection("yourCollection");
Query query = yourCollRef.whereEqualTo("yourPropery", "yourValue");
query.get().addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
for (QueryDocumentSnapshot document : task.getResult())
Log.d(TAG, document.getId() + " => " + document.getData());
else
Log.d(TAG, "Error getting documents: ", task.getException());
);
Hi Emil! Have you tried my solutions above, does it work?
– Alex Mamo
Nov 17 '18 at 8:57
add a comment |
If you want to check a particular document for existence based on its document id
, please use the following lines of code:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
DocumentReference docIdRef = rootRef.collection("yourCollection").document(docId);
docIdRef.get().addOnCompleteListener(new OnCompleteListener<DocumentSnapshot>()
@Override
public void onComplete(@NonNull Task<DocumentSnapshot> task)
if (task.isSuccessful())
DocumentSnapshot document = task.getResult();
if (document.exists())
Log.d(TAG, "Document exists!");
else
Log.d(TAG, "Document does not exist!");
else
Log.d(TAG, "Failed with: ", task.getException());
);
If you don't have the document id, you need to use a query and find that document according to a value of a specific property like this:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
CollectionReference yourCollRef = rootRef.collection("yourCollection");
Query query = yourCollRef.whereEqualTo("yourPropery", "yourValue");
query.get().addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
for (QueryDocumentSnapshot document : task.getResult())
Log.d(TAG, document.getId() + " => " + document.getData());
else
Log.d(TAG, "Error getting documents: ", task.getException());
);
Hi Emil! Have you tried my solutions above, does it work?
– Alex Mamo
Nov 17 '18 at 8:57
add a comment |
If you want to check a particular document for existence based on its document id
, please use the following lines of code:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
DocumentReference docIdRef = rootRef.collection("yourCollection").document(docId);
docIdRef.get().addOnCompleteListener(new OnCompleteListener<DocumentSnapshot>()
@Override
public void onComplete(@NonNull Task<DocumentSnapshot> task)
if (task.isSuccessful())
DocumentSnapshot document = task.getResult();
if (document.exists())
Log.d(TAG, "Document exists!");
else
Log.d(TAG, "Document does not exist!");
else
Log.d(TAG, "Failed with: ", task.getException());
);
If you don't have the document id, you need to use a query and find that document according to a value of a specific property like this:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
CollectionReference yourCollRef = rootRef.collection("yourCollection");
Query query = yourCollRef.whereEqualTo("yourPropery", "yourValue");
query.get().addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
for (QueryDocumentSnapshot document : task.getResult())
Log.d(TAG, document.getId() + " => " + document.getData());
else
Log.d(TAG, "Error getting documents: ", task.getException());
);
If you want to check a particular document for existence based on its document id
, please use the following lines of code:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
DocumentReference docIdRef = rootRef.collection("yourCollection").document(docId);
docIdRef.get().addOnCompleteListener(new OnCompleteListener<DocumentSnapshot>()
@Override
public void onComplete(@NonNull Task<DocumentSnapshot> task)
if (task.isSuccessful())
DocumentSnapshot document = task.getResult();
if (document.exists())
Log.d(TAG, "Document exists!");
else
Log.d(TAG, "Document does not exist!");
else
Log.d(TAG, "Failed with: ", task.getException());
);
If you don't have the document id, you need to use a query and find that document according to a value of a specific property like this:
FirebaseFirestore rootRef = FirebaseFirestore.getInstance();
CollectionReference yourCollRef = rootRef.collection("yourCollection");
Query query = yourCollRef.whereEqualTo("yourPropery", "yourValue");
query.get().addOnCompleteListener(new OnCompleteListener<QuerySnapshot>()
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task)
if (task.isSuccessful())
for (QueryDocumentSnapshot document : task.getResult())
Log.d(TAG, document.getId() + " => " + document.getData());
else
Log.d(TAG, "Error getting documents: ", task.getException());
);
edited Nov 16 '18 at 12:42
answered Nov 16 '18 at 10:16


Alex MamoAlex Mamo
46.6k82965
46.6k82965
Hi Emil! Have you tried my solutions above, does it work?
– Alex Mamo
Nov 17 '18 at 8:57
add a comment |
Hi Emil! Have you tried my solutions above, does it work?
– Alex Mamo
Nov 17 '18 at 8:57
Hi Emil! Have you tried my solutions above, does it work?
– Alex Mamo
Nov 17 '18 at 8:57
Hi Emil! Have you tried my solutions above, does it work?
– Alex Mamo
Nov 17 '18 at 8:57
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53332471%2fchecking-if-a-document-exists-in-a-firestore-collection%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qxbnVFIL 9ls7T6g0AHNiV,l4I f9b51beV,1 m