Encrypt character string in AES using OpenSSL in C++
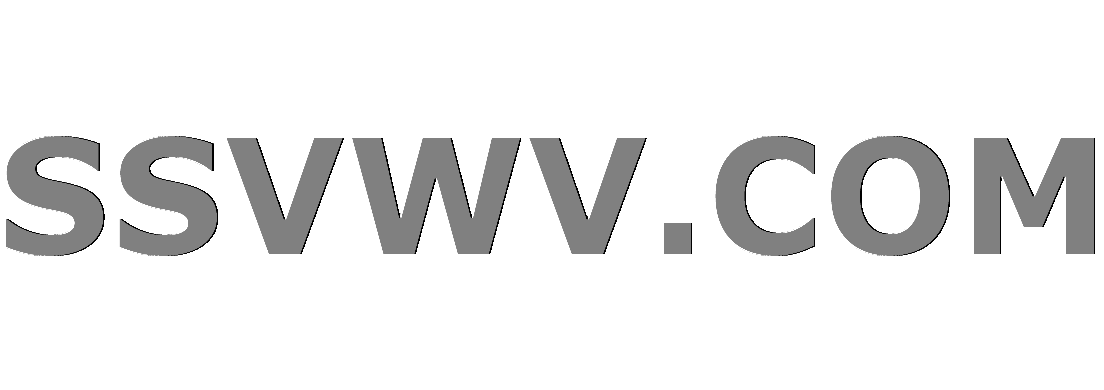
Multi tool use
I have this AES encryption function with the OpenSSL library that receives 3 values as parameters, the first is the key (16 ASCII characters), the second is the message to be encrypted (also in ASCII) and the third is the size of the message, the function returns the encrypted string in string
format.
#include <stdlib.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdarg.h>
#include "openSSL/aes.h"
using namespace std;
string encAES(char* Key, char* Msg, int size)
static char* Res;
string output;
AES_KEY enc_key;
static const char* const lut = "0123456789ABCDEF";
Res = (char *)malloc(size);
AES_set_encrypt_key((unsigned char *)Key, 128, &enc_key);
for(int aux = 0; aux <= size; aux += 16)
AES_ecb_encrypt((unsigned char *)Msg + aux, (unsigned char *)Res + aux, &enc_key, AES_ENCRYPT);
output.reserve(2 * size);
for (size_t i = 0; i < size; ++i)
const unsigned char c = Res[i];
output.push_back(lut[c >> 4]);
output.push_back(lut[c & 15]);
free(Res);
return output;
This is how i call and use the function:
int main(int argc, char const *argv)
char charKey[16 + 1] = 0;
char charMes[800 + 1] = 0;
//16 KEY ASCII
string str_key = "ABCDEFGHIJKLMNOP";
memset(charKey, 0, sizeof(charKey));
strcpy(charKey, str_key.c_str());
//Variable MESSAGE ASCII
string str_mes = "Hello World! This is a test chain.";
memset(charMes, 0, sizeof(charMes));
strcpy(charMes, str_mes.c_str());
string enc_mes = encAES(charKey, charMes, sizeof(charMes));
return 0;
What I want is that the function encAES()
now receive two values: the key and the message in string
, try the following but it generates errors (I do not know since the program closes unexpectedly):
string encAES2(string enc_key, string enc_message)
int enc_message_len = (enc_message.length() / 2);
unsigned char key1[16] = 0;
unsigned char in[800] = 0;
unsigned char out[800] = 0;
AES_KEY aes_key;
memset(key1, 0, sizeof(key1));
memset(in, 0, sizeof(in));
memset(out, 0, sizeof(out));
for(int aux = 0, str_len = enc_key.length(); aux != str_len; aux += 2)
int valor;
stringstream ss;
ss << std::hex << enc_key.substr(aux, 2);
ss >> valor;
key1[aux/2] = valor;
for(int aux = 0, str_len = enc_message.length(); aux != str_len; aux += 2)
int valor;
stringstream ss;
ss << std::hex << enc_message.substr(aux, 2);
ss >> valor;
in[aux/2] = valor;
AES_set_encrypt_key((unsigned char *)key1, 128, &aes_key);
for(int aux = 0; aux <= 320; aux += 16)
AES_ecb_encrypt((unsigned char *)(in + aux), (unsigned char *)(out + aux), &aes_key, AES_ENCRYPT);
string encrypt_str = unsignedcharHex_to_stringHex(out, 320);
return encrypt_str;
c++ encryption openssl
add a comment |
I have this AES encryption function with the OpenSSL library that receives 3 values as parameters, the first is the key (16 ASCII characters), the second is the message to be encrypted (also in ASCII) and the third is the size of the message, the function returns the encrypted string in string
format.
#include <stdlib.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdarg.h>
#include "openSSL/aes.h"
using namespace std;
string encAES(char* Key, char* Msg, int size)
static char* Res;
string output;
AES_KEY enc_key;
static const char* const lut = "0123456789ABCDEF";
Res = (char *)malloc(size);
AES_set_encrypt_key((unsigned char *)Key, 128, &enc_key);
for(int aux = 0; aux <= size; aux += 16)
AES_ecb_encrypt((unsigned char *)Msg + aux, (unsigned char *)Res + aux, &enc_key, AES_ENCRYPT);
output.reserve(2 * size);
for (size_t i = 0; i < size; ++i)
const unsigned char c = Res[i];
output.push_back(lut[c >> 4]);
output.push_back(lut[c & 15]);
free(Res);
return output;
This is how i call and use the function:
int main(int argc, char const *argv)
char charKey[16 + 1] = 0;
char charMes[800 + 1] = 0;
//16 KEY ASCII
string str_key = "ABCDEFGHIJKLMNOP";
memset(charKey, 0, sizeof(charKey));
strcpy(charKey, str_key.c_str());
//Variable MESSAGE ASCII
string str_mes = "Hello World! This is a test chain.";
memset(charMes, 0, sizeof(charMes));
strcpy(charMes, str_mes.c_str());
string enc_mes = encAES(charKey, charMes, sizeof(charMes));
return 0;
What I want is that the function encAES()
now receive two values: the key and the message in string
, try the following but it generates errors (I do not know since the program closes unexpectedly):
string encAES2(string enc_key, string enc_message)
int enc_message_len = (enc_message.length() / 2);
unsigned char key1[16] = 0;
unsigned char in[800] = 0;
unsigned char out[800] = 0;
AES_KEY aes_key;
memset(key1, 0, sizeof(key1));
memset(in, 0, sizeof(in));
memset(out, 0, sizeof(out));
for(int aux = 0, str_len = enc_key.length(); aux != str_len; aux += 2)
int valor;
stringstream ss;
ss << std::hex << enc_key.substr(aux, 2);
ss >> valor;
key1[aux/2] = valor;
for(int aux = 0, str_len = enc_message.length(); aux != str_len; aux += 2)
int valor;
stringstream ss;
ss << std::hex << enc_message.substr(aux, 2);
ss >> valor;
in[aux/2] = valor;
AES_set_encrypt_key((unsigned char *)key1, 128, &aes_key);
for(int aux = 0; aux <= 320; aux += 16)
AES_ecb_encrypt((unsigned char *)(in + aux), (unsigned char *)(out + aux), &aes_key, AES_ENCRYPT);
string encrypt_str = unsignedcharHex_to_stringHex(out, 320);
return encrypt_str;
c++ encryption openssl
2
1. You are working too hard. OpenSSL does not need to be called per-block, it will handle the entire message in one call and add padding as needed. 2. Note that AES is byte array based, not character sostrcpy
will not work, since the output is random bytes including 0x00. 3. ECB mode is not secure, at a ,minimum use CBC mode with a random IV or GCM mode is available and it fits your needs.
– zaph
Nov 15 '18 at 17:44
add a comment |
I have this AES encryption function with the OpenSSL library that receives 3 values as parameters, the first is the key (16 ASCII characters), the second is the message to be encrypted (also in ASCII) and the third is the size of the message, the function returns the encrypted string in string
format.
#include <stdlib.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdarg.h>
#include "openSSL/aes.h"
using namespace std;
string encAES(char* Key, char* Msg, int size)
static char* Res;
string output;
AES_KEY enc_key;
static const char* const lut = "0123456789ABCDEF";
Res = (char *)malloc(size);
AES_set_encrypt_key((unsigned char *)Key, 128, &enc_key);
for(int aux = 0; aux <= size; aux += 16)
AES_ecb_encrypt((unsigned char *)Msg + aux, (unsigned char *)Res + aux, &enc_key, AES_ENCRYPT);
output.reserve(2 * size);
for (size_t i = 0; i < size; ++i)
const unsigned char c = Res[i];
output.push_back(lut[c >> 4]);
output.push_back(lut[c & 15]);
free(Res);
return output;
This is how i call and use the function:
int main(int argc, char const *argv)
char charKey[16 + 1] = 0;
char charMes[800 + 1] = 0;
//16 KEY ASCII
string str_key = "ABCDEFGHIJKLMNOP";
memset(charKey, 0, sizeof(charKey));
strcpy(charKey, str_key.c_str());
//Variable MESSAGE ASCII
string str_mes = "Hello World! This is a test chain.";
memset(charMes, 0, sizeof(charMes));
strcpy(charMes, str_mes.c_str());
string enc_mes = encAES(charKey, charMes, sizeof(charMes));
return 0;
What I want is that the function encAES()
now receive two values: the key and the message in string
, try the following but it generates errors (I do not know since the program closes unexpectedly):
string encAES2(string enc_key, string enc_message)
int enc_message_len = (enc_message.length() / 2);
unsigned char key1[16] = 0;
unsigned char in[800] = 0;
unsigned char out[800] = 0;
AES_KEY aes_key;
memset(key1, 0, sizeof(key1));
memset(in, 0, sizeof(in));
memset(out, 0, sizeof(out));
for(int aux = 0, str_len = enc_key.length(); aux != str_len; aux += 2)
int valor;
stringstream ss;
ss << std::hex << enc_key.substr(aux, 2);
ss >> valor;
key1[aux/2] = valor;
for(int aux = 0, str_len = enc_message.length(); aux != str_len; aux += 2)
int valor;
stringstream ss;
ss << std::hex << enc_message.substr(aux, 2);
ss >> valor;
in[aux/2] = valor;
AES_set_encrypt_key((unsigned char *)key1, 128, &aes_key);
for(int aux = 0; aux <= 320; aux += 16)
AES_ecb_encrypt((unsigned char *)(in + aux), (unsigned char *)(out + aux), &aes_key, AES_ENCRYPT);
string encrypt_str = unsignedcharHex_to_stringHex(out, 320);
return encrypt_str;
c++ encryption openssl
I have this AES encryption function with the OpenSSL library that receives 3 values as parameters, the first is the key (16 ASCII characters), the second is the message to be encrypted (also in ASCII) and the third is the size of the message, the function returns the encrypted string in string
format.
#include <stdlib.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdarg.h>
#include "openSSL/aes.h"
using namespace std;
string encAES(char* Key, char* Msg, int size)
static char* Res;
string output;
AES_KEY enc_key;
static const char* const lut = "0123456789ABCDEF";
Res = (char *)malloc(size);
AES_set_encrypt_key((unsigned char *)Key, 128, &enc_key);
for(int aux = 0; aux <= size; aux += 16)
AES_ecb_encrypt((unsigned char *)Msg + aux, (unsigned char *)Res + aux, &enc_key, AES_ENCRYPT);
output.reserve(2 * size);
for (size_t i = 0; i < size; ++i)
const unsigned char c = Res[i];
output.push_back(lut[c >> 4]);
output.push_back(lut[c & 15]);
free(Res);
return output;
This is how i call and use the function:
int main(int argc, char const *argv)
char charKey[16 + 1] = 0;
char charMes[800 + 1] = 0;
//16 KEY ASCII
string str_key = "ABCDEFGHIJKLMNOP";
memset(charKey, 0, sizeof(charKey));
strcpy(charKey, str_key.c_str());
//Variable MESSAGE ASCII
string str_mes = "Hello World! This is a test chain.";
memset(charMes, 0, sizeof(charMes));
strcpy(charMes, str_mes.c_str());
string enc_mes = encAES(charKey, charMes, sizeof(charMes));
return 0;
What I want is that the function encAES()
now receive two values: the key and the message in string
, try the following but it generates errors (I do not know since the program closes unexpectedly):
string encAES2(string enc_key, string enc_message)
int enc_message_len = (enc_message.length() / 2);
unsigned char key1[16] = 0;
unsigned char in[800] = 0;
unsigned char out[800] = 0;
AES_KEY aes_key;
memset(key1, 0, sizeof(key1));
memset(in, 0, sizeof(in));
memset(out, 0, sizeof(out));
for(int aux = 0, str_len = enc_key.length(); aux != str_len; aux += 2)
int valor;
stringstream ss;
ss << std::hex << enc_key.substr(aux, 2);
ss >> valor;
key1[aux/2] = valor;
for(int aux = 0, str_len = enc_message.length(); aux != str_len; aux += 2)
int valor;
stringstream ss;
ss << std::hex << enc_message.substr(aux, 2);
ss >> valor;
in[aux/2] = valor;
AES_set_encrypt_key((unsigned char *)key1, 128, &aes_key);
for(int aux = 0; aux <= 320; aux += 16)
AES_ecb_encrypt((unsigned char *)(in + aux), (unsigned char *)(out + aux), &aes_key, AES_ENCRYPT);
string encrypt_str = unsignedcharHex_to_stringHex(out, 320);
return encrypt_str;
c++ encryption openssl
c++ encryption openssl
asked Nov 15 '18 at 16:44
Noe CanoNoe Cano
154215
154215
2
1. You are working too hard. OpenSSL does not need to be called per-block, it will handle the entire message in one call and add padding as needed. 2. Note that AES is byte array based, not character sostrcpy
will not work, since the output is random bytes including 0x00. 3. ECB mode is not secure, at a ,minimum use CBC mode with a random IV or GCM mode is available and it fits your needs.
– zaph
Nov 15 '18 at 17:44
add a comment |
2
1. You are working too hard. OpenSSL does not need to be called per-block, it will handle the entire message in one call and add padding as needed. 2. Note that AES is byte array based, not character sostrcpy
will not work, since the output is random bytes including 0x00. 3. ECB mode is not secure, at a ,minimum use CBC mode with a random IV or GCM mode is available and it fits your needs.
– zaph
Nov 15 '18 at 17:44
2
2
1. You are working too hard. OpenSSL does not need to be called per-block, it will handle the entire message in one call and add padding as needed. 2. Note that AES is byte array based, not character so
strcpy
will not work, since the output is random bytes including 0x00. 3. ECB mode is not secure, at a ,minimum use CBC mode with a random IV or GCM mode is available and it fits your needs.– zaph
Nov 15 '18 at 17:44
1. You are working too hard. OpenSSL does not need to be called per-block, it will handle the entire message in one call and add padding as needed. 2. Note that AES is byte array based, not character so
strcpy
will not work, since the output is random bytes including 0x00. 3. ECB mode is not secure, at a ,minimum use CBC mode with a random IV or GCM mode is available and it fits your needs.– zaph
Nov 15 '18 at 17:44
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324151%2fencrypt-character-string-in-aes-using-openssl-in-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324151%2fencrypt-character-string-in-aes-using-openssl-in-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
e,oa1BOu4kSL4gf07joLbNB,s rQHO,Nz Dn bqqMCSwtgVtI9gpG
2
1. You are working too hard. OpenSSL does not need to be called per-block, it will handle the entire message in one call and add padding as needed. 2. Note that AES is byte array based, not character so
strcpy
will not work, since the output is random bytes including 0x00. 3. ECB mode is not secure, at a ,minimum use CBC mode with a random IV or GCM mode is available and it fits your needs.– zaph
Nov 15 '18 at 17:44