How to enable a user to buy the same product over and over in android In-app purchases
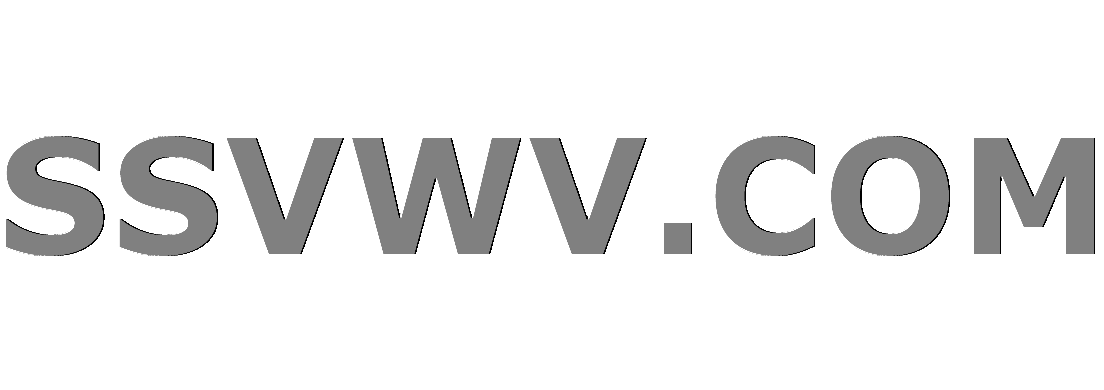
Multi tool use
I am using the Android In-App Billing v3 Library (here is the link). The application I'm making converts Google Play Rewards to cash which is transfer to the desired mobile wallet of the user. I am facing a problem that according to how my app functions a single product should be ready to be purchased over and over again but when I buy the item and try to buy the same item again, it shows me the successful payment activity that is shown when the
onProductPurchased() is called.
I know that I have to add consumable products to the play Console but I couldn't find the right way and neither the way how to consume that. This is my first time handling in-app purchases; please guide me through the process. Here are the code and some screenshots of my application.
package com.payapp.app;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.Spinner;
import com.anjlab.android.iab.v3.BillingProcessor;
import com.anjlab.android.iab.v3.TransactionDetails;
import java.util.ArrayList;
import java.util.List;
public class Paytm extends AppCompatActivity implements
BillingProcessor.IBillingHandler
BillingProcessor bp;
Button cont;
String selectedPrice;
Spinner price;
List<String> price_array = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState)
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_paytm);
getSupportActionBar().setTitle("Paytm");
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setHomeButtonEnabled(true);
cont = findViewById(R.id.continuebtn);
price_array.add("50");
price_array.add("100");
price_array.add("150");
price_array.add("200");
price_array.add("500");
price_array.add("1000");
/* Spinner Initialization starts */
price = findViewById(R.id.price);
price.setSelection(0);
price.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener()
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id)
selectedPrice = parent.getItemAtPosition(position).toString();
@Override
public void onNothingSelected(AdapterView<?> parent)
selectedPrice = "Football";
);
ArrayAdapter<String> categoriesAdapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, price_array);
categoriesAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
price.setAdapter(categoriesAdapter);
bp = new BillingProcessor(this, "<Removed the license key>", this);
bp.initialize();
cont.setOnClickListener(new View.OnClickListener()
@Override
public void onClick(View v)
if (selectedPrice.equals("50"))
bp.purchase(Paytm.this,"pay_50");
if (selectedPrice.equals("100"))
bp.purchase(Paytm.this,"pay_100");
if (selectedPrice.equals("150"))
bp.purchase(Paytm.this,"pay_150");
if (selectedPrice.equals("200"))
bp.purchase(Paytm.this,"pay_200");
if (selectedPrice.equals("500"))
bp.purchase(Paytm.this,"pay_500");
if (selectedPrice.equals("1000"))
bp.purchase(Paytm.this,"pay_1000");
);
@Override
public void onProductPurchased(@NonNull String productId, @Nullable TransactionDetails details)
startActivity(new Intent(Paytm.this,PaymentSuccess.class));
@Override
public void onPurchaseHistoryRestored()
@Override
public void onBillingError(int errorCode, @Nullable Throwable error)
startActivity(new Intent(Paytm.this,PaymentFailed.class));
@Override
public void onBillingInitialized()
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data)
if (!bp.handleActivityResult(requestCode, resultCode, data))
super.onActivityResult(requestCode, resultCode, data);
@Override
public void onDestroy()
if (bp != null)
bp.release();
super.onDestroy();
@Override
public boolean onOptionsItemSelected(MenuItem item)
onBackPressed();
return true;
Here are the screenshots for better understanding
Mobile wallet selection screen
Product selection screen
The products in my console (managed, no idea how to add consumable products)



add a comment |
I am using the Android In-App Billing v3 Library (here is the link). The application I'm making converts Google Play Rewards to cash which is transfer to the desired mobile wallet of the user. I am facing a problem that according to how my app functions a single product should be ready to be purchased over and over again but when I buy the item and try to buy the same item again, it shows me the successful payment activity that is shown when the
onProductPurchased() is called.
I know that I have to add consumable products to the play Console but I couldn't find the right way and neither the way how to consume that. This is my first time handling in-app purchases; please guide me through the process. Here are the code and some screenshots of my application.
package com.payapp.app;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.Spinner;
import com.anjlab.android.iab.v3.BillingProcessor;
import com.anjlab.android.iab.v3.TransactionDetails;
import java.util.ArrayList;
import java.util.List;
public class Paytm extends AppCompatActivity implements
BillingProcessor.IBillingHandler
BillingProcessor bp;
Button cont;
String selectedPrice;
Spinner price;
List<String> price_array = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState)
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_paytm);
getSupportActionBar().setTitle("Paytm");
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setHomeButtonEnabled(true);
cont = findViewById(R.id.continuebtn);
price_array.add("50");
price_array.add("100");
price_array.add("150");
price_array.add("200");
price_array.add("500");
price_array.add("1000");
/* Spinner Initialization starts */
price = findViewById(R.id.price);
price.setSelection(0);
price.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener()
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id)
selectedPrice = parent.getItemAtPosition(position).toString();
@Override
public void onNothingSelected(AdapterView<?> parent)
selectedPrice = "Football";
);
ArrayAdapter<String> categoriesAdapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, price_array);
categoriesAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
price.setAdapter(categoriesAdapter);
bp = new BillingProcessor(this, "<Removed the license key>", this);
bp.initialize();
cont.setOnClickListener(new View.OnClickListener()
@Override
public void onClick(View v)
if (selectedPrice.equals("50"))
bp.purchase(Paytm.this,"pay_50");
if (selectedPrice.equals("100"))
bp.purchase(Paytm.this,"pay_100");
if (selectedPrice.equals("150"))
bp.purchase(Paytm.this,"pay_150");
if (selectedPrice.equals("200"))
bp.purchase(Paytm.this,"pay_200");
if (selectedPrice.equals("500"))
bp.purchase(Paytm.this,"pay_500");
if (selectedPrice.equals("1000"))
bp.purchase(Paytm.this,"pay_1000");
);
@Override
public void onProductPurchased(@NonNull String productId, @Nullable TransactionDetails details)
startActivity(new Intent(Paytm.this,PaymentSuccess.class));
@Override
public void onPurchaseHistoryRestored()
@Override
public void onBillingError(int errorCode, @Nullable Throwable error)
startActivity(new Intent(Paytm.this,PaymentFailed.class));
@Override
public void onBillingInitialized()
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data)
if (!bp.handleActivityResult(requestCode, resultCode, data))
super.onActivityResult(requestCode, resultCode, data);
@Override
public void onDestroy()
if (bp != null)
bp.release();
super.onDestroy();
@Override
public boolean onOptionsItemSelected(MenuItem item)
onBackPressed();
return true;
Here are the screenshots for better understanding
Mobile wallet selection screen
Product selection screen
The products in my console (managed, no idea how to add consumable products)



add a comment |
I am using the Android In-App Billing v3 Library (here is the link). The application I'm making converts Google Play Rewards to cash which is transfer to the desired mobile wallet of the user. I am facing a problem that according to how my app functions a single product should be ready to be purchased over and over again but when I buy the item and try to buy the same item again, it shows me the successful payment activity that is shown when the
onProductPurchased() is called.
I know that I have to add consumable products to the play Console but I couldn't find the right way and neither the way how to consume that. This is my first time handling in-app purchases; please guide me through the process. Here are the code and some screenshots of my application.
package com.payapp.app;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.Spinner;
import com.anjlab.android.iab.v3.BillingProcessor;
import com.anjlab.android.iab.v3.TransactionDetails;
import java.util.ArrayList;
import java.util.List;
public class Paytm extends AppCompatActivity implements
BillingProcessor.IBillingHandler
BillingProcessor bp;
Button cont;
String selectedPrice;
Spinner price;
List<String> price_array = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState)
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_paytm);
getSupportActionBar().setTitle("Paytm");
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setHomeButtonEnabled(true);
cont = findViewById(R.id.continuebtn);
price_array.add("50");
price_array.add("100");
price_array.add("150");
price_array.add("200");
price_array.add("500");
price_array.add("1000");
/* Spinner Initialization starts */
price = findViewById(R.id.price);
price.setSelection(0);
price.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener()
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id)
selectedPrice = parent.getItemAtPosition(position).toString();
@Override
public void onNothingSelected(AdapterView<?> parent)
selectedPrice = "Football";
);
ArrayAdapter<String> categoriesAdapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, price_array);
categoriesAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
price.setAdapter(categoriesAdapter);
bp = new BillingProcessor(this, "<Removed the license key>", this);
bp.initialize();
cont.setOnClickListener(new View.OnClickListener()
@Override
public void onClick(View v)
if (selectedPrice.equals("50"))
bp.purchase(Paytm.this,"pay_50");
if (selectedPrice.equals("100"))
bp.purchase(Paytm.this,"pay_100");
if (selectedPrice.equals("150"))
bp.purchase(Paytm.this,"pay_150");
if (selectedPrice.equals("200"))
bp.purchase(Paytm.this,"pay_200");
if (selectedPrice.equals("500"))
bp.purchase(Paytm.this,"pay_500");
if (selectedPrice.equals("1000"))
bp.purchase(Paytm.this,"pay_1000");
);
@Override
public void onProductPurchased(@NonNull String productId, @Nullable TransactionDetails details)
startActivity(new Intent(Paytm.this,PaymentSuccess.class));
@Override
public void onPurchaseHistoryRestored()
@Override
public void onBillingError(int errorCode, @Nullable Throwable error)
startActivity(new Intent(Paytm.this,PaymentFailed.class));
@Override
public void onBillingInitialized()
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data)
if (!bp.handleActivityResult(requestCode, resultCode, data))
super.onActivityResult(requestCode, resultCode, data);
@Override
public void onDestroy()
if (bp != null)
bp.release();
super.onDestroy();
@Override
public boolean onOptionsItemSelected(MenuItem item)
onBackPressed();
return true;
Here are the screenshots for better understanding
Mobile wallet selection screen
Product selection screen
The products in my console (managed, no idea how to add consumable products)



I am using the Android In-App Billing v3 Library (here is the link). The application I'm making converts Google Play Rewards to cash which is transfer to the desired mobile wallet of the user. I am facing a problem that according to how my app functions a single product should be ready to be purchased over and over again but when I buy the item and try to buy the same item again, it shows me the successful payment activity that is shown when the
onProductPurchased() is called.
I know that I have to add consumable products to the play Console but I couldn't find the right way and neither the way how to consume that. This is my first time handling in-app purchases; please guide me through the process. Here are the code and some screenshots of my application.
package com.payapp.app;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.Spinner;
import com.anjlab.android.iab.v3.BillingProcessor;
import com.anjlab.android.iab.v3.TransactionDetails;
import java.util.ArrayList;
import java.util.List;
public class Paytm extends AppCompatActivity implements
BillingProcessor.IBillingHandler
BillingProcessor bp;
Button cont;
String selectedPrice;
Spinner price;
List<String> price_array = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState)
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_paytm);
getSupportActionBar().setTitle("Paytm");
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setHomeButtonEnabled(true);
cont = findViewById(R.id.continuebtn);
price_array.add("50");
price_array.add("100");
price_array.add("150");
price_array.add("200");
price_array.add("500");
price_array.add("1000");
/* Spinner Initialization starts */
price = findViewById(R.id.price);
price.setSelection(0);
price.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener()
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id)
selectedPrice = parent.getItemAtPosition(position).toString();
@Override
public void onNothingSelected(AdapterView<?> parent)
selectedPrice = "Football";
);
ArrayAdapter<String> categoriesAdapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, price_array);
categoriesAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
price.setAdapter(categoriesAdapter);
bp = new BillingProcessor(this, "<Removed the license key>", this);
bp.initialize();
cont.setOnClickListener(new View.OnClickListener()
@Override
public void onClick(View v)
if (selectedPrice.equals("50"))
bp.purchase(Paytm.this,"pay_50");
if (selectedPrice.equals("100"))
bp.purchase(Paytm.this,"pay_100");
if (selectedPrice.equals("150"))
bp.purchase(Paytm.this,"pay_150");
if (selectedPrice.equals("200"))
bp.purchase(Paytm.this,"pay_200");
if (selectedPrice.equals("500"))
bp.purchase(Paytm.this,"pay_500");
if (selectedPrice.equals("1000"))
bp.purchase(Paytm.this,"pay_1000");
);
@Override
public void onProductPurchased(@NonNull String productId, @Nullable TransactionDetails details)
startActivity(new Intent(Paytm.this,PaymentSuccess.class));
@Override
public void onPurchaseHistoryRestored()
@Override
public void onBillingError(int errorCode, @Nullable Throwable error)
startActivity(new Intent(Paytm.this,PaymentFailed.class));
@Override
public void onBillingInitialized()
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data)
if (!bp.handleActivityResult(requestCode, resultCode, data))
super.onActivityResult(requestCode, resultCode, data);
@Override
public void onDestroy()
if (bp != null)
bp.release();
super.onDestroy();
@Override
public boolean onOptionsItemSelected(MenuItem item)
onBackPressed();
return true;
Here are the screenshots for better understanding
Mobile wallet selection screen
Product selection screen
The products in my console (managed, no idea how to add consumable products)






asked Nov 16 '18 at 5:20


Prakhar ShuklaPrakhar Shukla
82
82
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Any added product can be used as consumable product. However to achieve this you need to consume the purchased product.
If you read the documentation in the Android In-App Billing v3 Library you'll find your answer.
So where do I exactly need to add it? I think adding it to the onProductPurchase() method should do good.
– Prakhar Shukla
Nov 16 '18 at 8:35
You can add it after user purchased it and used the feature that you are offering
– ASLOUN
Nov 16 '18 at 8:47
Thank you so much, it's working!
– Prakhar Shukla
Nov 16 '18 at 8:54
How can I get the transaction details from the onProductPurchased() the lib readme doesn't do a very good job of explaining especially to a beginner.
– Prakhar Shukla
Nov 16 '18 at 9:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331874%2fhow-to-enable-a-user-to-buy-the-same-product-over-and-over-in-android-in-app-pur%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Any added product can be used as consumable product. However to achieve this you need to consume the purchased product.
If you read the documentation in the Android In-App Billing v3 Library you'll find your answer.
So where do I exactly need to add it? I think adding it to the onProductPurchase() method should do good.
– Prakhar Shukla
Nov 16 '18 at 8:35
You can add it after user purchased it and used the feature that you are offering
– ASLOUN
Nov 16 '18 at 8:47
Thank you so much, it's working!
– Prakhar Shukla
Nov 16 '18 at 8:54
How can I get the transaction details from the onProductPurchased() the lib readme doesn't do a very good job of explaining especially to a beginner.
– Prakhar Shukla
Nov 16 '18 at 9:15
add a comment |
Any added product can be used as consumable product. However to achieve this you need to consume the purchased product.
If you read the documentation in the Android In-App Billing v3 Library you'll find your answer.
So where do I exactly need to add it? I think adding it to the onProductPurchase() method should do good.
– Prakhar Shukla
Nov 16 '18 at 8:35
You can add it after user purchased it and used the feature that you are offering
– ASLOUN
Nov 16 '18 at 8:47
Thank you so much, it's working!
– Prakhar Shukla
Nov 16 '18 at 8:54
How can I get the transaction details from the onProductPurchased() the lib readme doesn't do a very good job of explaining especially to a beginner.
– Prakhar Shukla
Nov 16 '18 at 9:15
add a comment |
Any added product can be used as consumable product. However to achieve this you need to consume the purchased product.
If you read the documentation in the Android In-App Billing v3 Library you'll find your answer.
Any added product can be used as consumable product. However to achieve this you need to consume the purchased product.
If you read the documentation in the Android In-App Billing v3 Library you'll find your answer.
answered Nov 16 '18 at 8:18
ASLOUNASLOUN
11518
11518
So where do I exactly need to add it? I think adding it to the onProductPurchase() method should do good.
– Prakhar Shukla
Nov 16 '18 at 8:35
You can add it after user purchased it and used the feature that you are offering
– ASLOUN
Nov 16 '18 at 8:47
Thank you so much, it's working!
– Prakhar Shukla
Nov 16 '18 at 8:54
How can I get the transaction details from the onProductPurchased() the lib readme doesn't do a very good job of explaining especially to a beginner.
– Prakhar Shukla
Nov 16 '18 at 9:15
add a comment |
So where do I exactly need to add it? I think adding it to the onProductPurchase() method should do good.
– Prakhar Shukla
Nov 16 '18 at 8:35
You can add it after user purchased it and used the feature that you are offering
– ASLOUN
Nov 16 '18 at 8:47
Thank you so much, it's working!
– Prakhar Shukla
Nov 16 '18 at 8:54
How can I get the transaction details from the onProductPurchased() the lib readme doesn't do a very good job of explaining especially to a beginner.
– Prakhar Shukla
Nov 16 '18 at 9:15
So where do I exactly need to add it? I think adding it to the onProductPurchase() method should do good.
– Prakhar Shukla
Nov 16 '18 at 8:35
So where do I exactly need to add it? I think adding it to the onProductPurchase() method should do good.
– Prakhar Shukla
Nov 16 '18 at 8:35
You can add it after user purchased it and used the feature that you are offering
– ASLOUN
Nov 16 '18 at 8:47
You can add it after user purchased it and used the feature that you are offering
– ASLOUN
Nov 16 '18 at 8:47
Thank you so much, it's working!
– Prakhar Shukla
Nov 16 '18 at 8:54
Thank you so much, it's working!
– Prakhar Shukla
Nov 16 '18 at 8:54
How can I get the transaction details from the onProductPurchased() the lib readme doesn't do a very good job of explaining especially to a beginner.
– Prakhar Shukla
Nov 16 '18 at 9:15
How can I get the transaction details from the onProductPurchased() the lib readme doesn't do a very good job of explaining especially to a beginner.
– Prakhar Shukla
Nov 16 '18 at 9:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331874%2fhow-to-enable-a-user-to-buy-the-same-product-over-and-over-in-android-in-app-pur%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BC ZcOwLt,2z,5fNZCTSZvfw,oUG2,gredt1xP2 U19WOA N qR,v6pcLedVY1ZzQBz7p,WDkJF0GlIl47BKxd2Sok2mke2I8