Integer contains linq c# but using using expressions
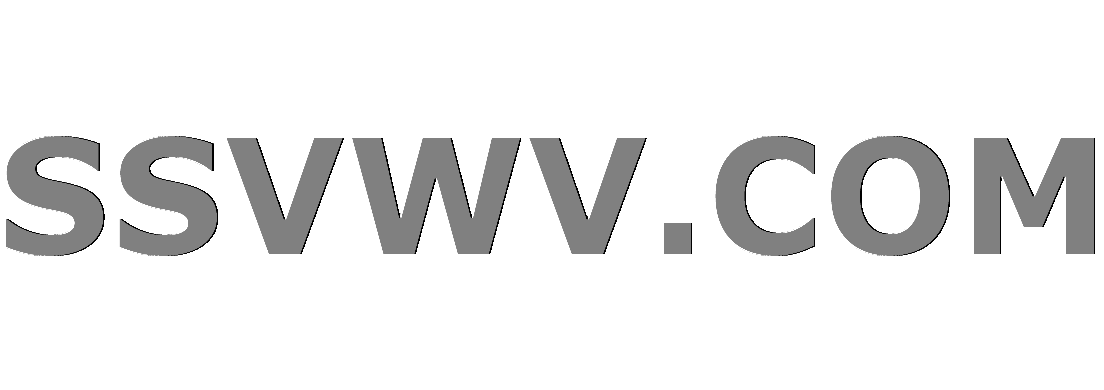
Multi tool use
I want to create a dynamic filter for my repositories using linq expressions, I have other filters but i don't know how to make the next one using expressions: (the condition was taked from here)
var result = _studentRepotory.GetAll().Where(s =>
SqlFunctions.StringConvert((double)s.Id).Contains("91")).ToList();
I have a method that receives the value of a property, the propertyName and the filter operator type (enum):
public static class Helper
public static Expression<Func<T, bool>> GetExpression<T>(object value, string propertyName, FilterOperatorType FilterType)
var parameter = Expression.Parameter(typeof(T));
var property = ExpressionUtils.GetPropertyChild(parameter, propertyName);
var constValue = Expression.Constant(value);
BinaryExpression expresion = null;
switch (FilterType)
case FilterOperatorType.Equal:
expresion = Expression.Equal(property, constValue);
break;
case FilterOperatorType.Greater:
expresion = Expression.GreaterThan(property, constValue);
break;
case FilterOperatorType.Less:
expresion = Expression.LessThan(property, constValue);
break;
case FilterOperatorType.GreaterOrEqual:
expresion = Expression.GreaterThanOrEqual(property, constValue);
break;
case FilterOperatorType.LessOrEqual:
expresion = Expression.LessThanOrEqual(property, constValue);
break;
var lambda = Expression.Lambda<Func<T, bool>>(expresion, parameter);
return lambda;
So, I want to add a new opertator type Contains that will evaluate if an integer contains some digits, in the first block of code I do it, but I want to do it with linq expressions using generics.
At the end I wil have:
Expression<Func<Student, bool>> where = Helper.GetExpression<Student>("91", "Id", FilterOperatorType.Contains);
var result = _studentRepotory.GetAll().Where(where).ToList();
The query should return all the students when the Id contains the digits 91.
Please help me, and tell me if you understand.
c# linq linq-expressions
add a comment |
I want to create a dynamic filter for my repositories using linq expressions, I have other filters but i don't know how to make the next one using expressions: (the condition was taked from here)
var result = _studentRepotory.GetAll().Where(s =>
SqlFunctions.StringConvert((double)s.Id).Contains("91")).ToList();
I have a method that receives the value of a property, the propertyName and the filter operator type (enum):
public static class Helper
public static Expression<Func<T, bool>> GetExpression<T>(object value, string propertyName, FilterOperatorType FilterType)
var parameter = Expression.Parameter(typeof(T));
var property = ExpressionUtils.GetPropertyChild(parameter, propertyName);
var constValue = Expression.Constant(value);
BinaryExpression expresion = null;
switch (FilterType)
case FilterOperatorType.Equal:
expresion = Expression.Equal(property, constValue);
break;
case FilterOperatorType.Greater:
expresion = Expression.GreaterThan(property, constValue);
break;
case FilterOperatorType.Less:
expresion = Expression.LessThan(property, constValue);
break;
case FilterOperatorType.GreaterOrEqual:
expresion = Expression.GreaterThanOrEqual(property, constValue);
break;
case FilterOperatorType.LessOrEqual:
expresion = Expression.LessThanOrEqual(property, constValue);
break;
var lambda = Expression.Lambda<Func<T, bool>>(expresion, parameter);
return lambda;
So, I want to add a new opertator type Contains that will evaluate if an integer contains some digits, in the first block of code I do it, but I want to do it with linq expressions using generics.
At the end I wil have:
Expression<Func<Student, bool>> where = Helper.GetExpression<Student>("91", "Id", FilterOperatorType.Contains);
var result = _studentRepotory.GetAll().Where(where).ToList();
The query should return all the students when the Id contains the digits 91.
Please help me, and tell me if you understand.
c# linq linq-expressions
There's no out of box way, you need to create aMethodCallExpression
– Mrinal Kamboj
Nov 16 '18 at 5:12
do google search for apredicatebuilder
library/class. i've used this one recently: gist.github.com/anomepani/…
– JohnB
Nov 16 '18 at 5:13
@MrinalKamboj I have other filter for strings and I user Expression.Call but I dont know how to do this one.
– allamgr
Nov 16 '18 at 5:17
add a comment |
I want to create a dynamic filter for my repositories using linq expressions, I have other filters but i don't know how to make the next one using expressions: (the condition was taked from here)
var result = _studentRepotory.GetAll().Where(s =>
SqlFunctions.StringConvert((double)s.Id).Contains("91")).ToList();
I have a method that receives the value of a property, the propertyName and the filter operator type (enum):
public static class Helper
public static Expression<Func<T, bool>> GetExpression<T>(object value, string propertyName, FilterOperatorType FilterType)
var parameter = Expression.Parameter(typeof(T));
var property = ExpressionUtils.GetPropertyChild(parameter, propertyName);
var constValue = Expression.Constant(value);
BinaryExpression expresion = null;
switch (FilterType)
case FilterOperatorType.Equal:
expresion = Expression.Equal(property, constValue);
break;
case FilterOperatorType.Greater:
expresion = Expression.GreaterThan(property, constValue);
break;
case FilterOperatorType.Less:
expresion = Expression.LessThan(property, constValue);
break;
case FilterOperatorType.GreaterOrEqual:
expresion = Expression.GreaterThanOrEqual(property, constValue);
break;
case FilterOperatorType.LessOrEqual:
expresion = Expression.LessThanOrEqual(property, constValue);
break;
var lambda = Expression.Lambda<Func<T, bool>>(expresion, parameter);
return lambda;
So, I want to add a new opertator type Contains that will evaluate if an integer contains some digits, in the first block of code I do it, but I want to do it with linq expressions using generics.
At the end I wil have:
Expression<Func<Student, bool>> where = Helper.GetExpression<Student>("91", "Id", FilterOperatorType.Contains);
var result = _studentRepotory.GetAll().Where(where).ToList();
The query should return all the students when the Id contains the digits 91.
Please help me, and tell me if you understand.
c# linq linq-expressions
I want to create a dynamic filter for my repositories using linq expressions, I have other filters but i don't know how to make the next one using expressions: (the condition was taked from here)
var result = _studentRepotory.GetAll().Where(s =>
SqlFunctions.StringConvert((double)s.Id).Contains("91")).ToList();
I have a method that receives the value of a property, the propertyName and the filter operator type (enum):
public static class Helper
public static Expression<Func<T, bool>> GetExpression<T>(object value, string propertyName, FilterOperatorType FilterType)
var parameter = Expression.Parameter(typeof(T));
var property = ExpressionUtils.GetPropertyChild(parameter, propertyName);
var constValue = Expression.Constant(value);
BinaryExpression expresion = null;
switch (FilterType)
case FilterOperatorType.Equal:
expresion = Expression.Equal(property, constValue);
break;
case FilterOperatorType.Greater:
expresion = Expression.GreaterThan(property, constValue);
break;
case FilterOperatorType.Less:
expresion = Expression.LessThan(property, constValue);
break;
case FilterOperatorType.GreaterOrEqual:
expresion = Expression.GreaterThanOrEqual(property, constValue);
break;
case FilterOperatorType.LessOrEqual:
expresion = Expression.LessThanOrEqual(property, constValue);
break;
var lambda = Expression.Lambda<Func<T, bool>>(expresion, parameter);
return lambda;
So, I want to add a new opertator type Contains that will evaluate if an integer contains some digits, in the first block of code I do it, but I want to do it with linq expressions using generics.
At the end I wil have:
Expression<Func<Student, bool>> where = Helper.GetExpression<Student>("91", "Id", FilterOperatorType.Contains);
var result = _studentRepotory.GetAll().Where(where).ToList();
The query should return all the students when the Id contains the digits 91.
Please help me, and tell me if you understand.
c# linq linq-expressions
c# linq linq-expressions
asked Nov 16 '18 at 5:09
allamgrallamgr
616
616
There's no out of box way, you need to create aMethodCallExpression
– Mrinal Kamboj
Nov 16 '18 at 5:12
do google search for apredicatebuilder
library/class. i've used this one recently: gist.github.com/anomepani/…
– JohnB
Nov 16 '18 at 5:13
@MrinalKamboj I have other filter for strings and I user Expression.Call but I dont know how to do this one.
– allamgr
Nov 16 '18 at 5:17
add a comment |
There's no out of box way, you need to create aMethodCallExpression
– Mrinal Kamboj
Nov 16 '18 at 5:12
do google search for apredicatebuilder
library/class. i've used this one recently: gist.github.com/anomepani/…
– JohnB
Nov 16 '18 at 5:13
@MrinalKamboj I have other filter for strings and I user Expression.Call but I dont know how to do this one.
– allamgr
Nov 16 '18 at 5:17
There's no out of box way, you need to create a
MethodCallExpression
– Mrinal Kamboj
Nov 16 '18 at 5:12
There's no out of box way, you need to create a
MethodCallExpression
– Mrinal Kamboj
Nov 16 '18 at 5:12
do google search for a
predicatebuilder
library/class. i've used this one recently: gist.github.com/anomepani/…– JohnB
Nov 16 '18 at 5:13
do google search for a
predicatebuilder
library/class. i've used this one recently: gist.github.com/anomepani/…– JohnB
Nov 16 '18 at 5:13
@MrinalKamboj I have other filter for strings and I user Expression.Call but I dont know how to do this one.
– allamgr
Nov 16 '18 at 5:17
@MrinalKamboj I have other filter for strings and I user Expression.Call but I dont know how to do this one.
– allamgr
Nov 16 '18 at 5:17
add a comment |
1 Answer
1
active
oldest
votes
I'm at 2:00 am and still working on this, the brain works better at that time hehehe, here is the solution for create the expression:
object value = "91";
var parameter = Expression.Parameter(typeof(Student));
var property = ExpressionUtils.GetPropertyChild(parameter, "Id");
var constValue = Expression.Constant(value);
var expressionConvert = Expression.Convert(property, typeof(double?));
var methodStringConvert = typeof(SqlFunctions).GetMethod("StringConvert",
BindingFlags.Public | BindingFlags.Static, null,
CallingConventions.Any,
new Type typeof(double?) ,
null);
var methodContains = typeof(string).GetMethod("Contains",
BindingFlags.Public | BindingFlags.Instance,
null, CallingConventions.Any,
new Type typeof(String) , null);
var expresionStringConvert = Expression.Call(methodStringConvert, expressionConvert);
var expresionContains = Expression.Call(expresionStringConvert, methodContains, constValue);
var lambdaContains = Expression.Lambda<Func<Student, bool>>(expresionContains, parameter);
Now you can use lambdaContains in the Where method of the studentRepository
What isSqlFunctions
is it a custom class. You can solve more complex use cases using the extension methods onstring
and usingstring
orList<string>
or similar Data structure as input. In extension methods you can solve more complex use cases, but just that it needs to be used in same memory or otherwise use an Expression Serializer
– Mrinal Kamboj
Nov 16 '18 at 8:53
@MrinalKamboj SqlFunctions is a static class from the EntityFramework package with helpers functions. View reference here
– allamgr
Nov 16 '18 at 20:09
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331782%2finteger-contains-linq-c-sharp-but-using-using-expressions%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'm at 2:00 am and still working on this, the brain works better at that time hehehe, here is the solution for create the expression:
object value = "91";
var parameter = Expression.Parameter(typeof(Student));
var property = ExpressionUtils.GetPropertyChild(parameter, "Id");
var constValue = Expression.Constant(value);
var expressionConvert = Expression.Convert(property, typeof(double?));
var methodStringConvert = typeof(SqlFunctions).GetMethod("StringConvert",
BindingFlags.Public | BindingFlags.Static, null,
CallingConventions.Any,
new Type typeof(double?) ,
null);
var methodContains = typeof(string).GetMethod("Contains",
BindingFlags.Public | BindingFlags.Instance,
null, CallingConventions.Any,
new Type typeof(String) , null);
var expresionStringConvert = Expression.Call(methodStringConvert, expressionConvert);
var expresionContains = Expression.Call(expresionStringConvert, methodContains, constValue);
var lambdaContains = Expression.Lambda<Func<Student, bool>>(expresionContains, parameter);
Now you can use lambdaContains in the Where method of the studentRepository
What isSqlFunctions
is it a custom class. You can solve more complex use cases using the extension methods onstring
and usingstring
orList<string>
or similar Data structure as input. In extension methods you can solve more complex use cases, but just that it needs to be used in same memory or otherwise use an Expression Serializer
– Mrinal Kamboj
Nov 16 '18 at 8:53
@MrinalKamboj SqlFunctions is a static class from the EntityFramework package with helpers functions. View reference here
– allamgr
Nov 16 '18 at 20:09
add a comment |
I'm at 2:00 am and still working on this, the brain works better at that time hehehe, here is the solution for create the expression:
object value = "91";
var parameter = Expression.Parameter(typeof(Student));
var property = ExpressionUtils.GetPropertyChild(parameter, "Id");
var constValue = Expression.Constant(value);
var expressionConvert = Expression.Convert(property, typeof(double?));
var methodStringConvert = typeof(SqlFunctions).GetMethod("StringConvert",
BindingFlags.Public | BindingFlags.Static, null,
CallingConventions.Any,
new Type typeof(double?) ,
null);
var methodContains = typeof(string).GetMethod("Contains",
BindingFlags.Public | BindingFlags.Instance,
null, CallingConventions.Any,
new Type typeof(String) , null);
var expresionStringConvert = Expression.Call(methodStringConvert, expressionConvert);
var expresionContains = Expression.Call(expresionStringConvert, methodContains, constValue);
var lambdaContains = Expression.Lambda<Func<Student, bool>>(expresionContains, parameter);
Now you can use lambdaContains in the Where method of the studentRepository
What isSqlFunctions
is it a custom class. You can solve more complex use cases using the extension methods onstring
and usingstring
orList<string>
or similar Data structure as input. In extension methods you can solve more complex use cases, but just that it needs to be used in same memory or otherwise use an Expression Serializer
– Mrinal Kamboj
Nov 16 '18 at 8:53
@MrinalKamboj SqlFunctions is a static class from the EntityFramework package with helpers functions. View reference here
– allamgr
Nov 16 '18 at 20:09
add a comment |
I'm at 2:00 am and still working on this, the brain works better at that time hehehe, here is the solution for create the expression:
object value = "91";
var parameter = Expression.Parameter(typeof(Student));
var property = ExpressionUtils.GetPropertyChild(parameter, "Id");
var constValue = Expression.Constant(value);
var expressionConvert = Expression.Convert(property, typeof(double?));
var methodStringConvert = typeof(SqlFunctions).GetMethod("StringConvert",
BindingFlags.Public | BindingFlags.Static, null,
CallingConventions.Any,
new Type typeof(double?) ,
null);
var methodContains = typeof(string).GetMethod("Contains",
BindingFlags.Public | BindingFlags.Instance,
null, CallingConventions.Any,
new Type typeof(String) , null);
var expresionStringConvert = Expression.Call(methodStringConvert, expressionConvert);
var expresionContains = Expression.Call(expresionStringConvert, methodContains, constValue);
var lambdaContains = Expression.Lambda<Func<Student, bool>>(expresionContains, parameter);
Now you can use lambdaContains in the Where method of the studentRepository
I'm at 2:00 am and still working on this, the brain works better at that time hehehe, here is the solution for create the expression:
object value = "91";
var parameter = Expression.Parameter(typeof(Student));
var property = ExpressionUtils.GetPropertyChild(parameter, "Id");
var constValue = Expression.Constant(value);
var expressionConvert = Expression.Convert(property, typeof(double?));
var methodStringConvert = typeof(SqlFunctions).GetMethod("StringConvert",
BindingFlags.Public | BindingFlags.Static, null,
CallingConventions.Any,
new Type typeof(double?) ,
null);
var methodContains = typeof(string).GetMethod("Contains",
BindingFlags.Public | BindingFlags.Instance,
null, CallingConventions.Any,
new Type typeof(String) , null);
var expresionStringConvert = Expression.Call(methodStringConvert, expressionConvert);
var expresionContains = Expression.Call(expresionStringConvert, methodContains, constValue);
var lambdaContains = Expression.Lambda<Func<Student, bool>>(expresionContains, parameter);
Now you can use lambdaContains in the Where method of the studentRepository
answered Nov 16 '18 at 6:16
allamgrallamgr
616
616
What isSqlFunctions
is it a custom class. You can solve more complex use cases using the extension methods onstring
and usingstring
orList<string>
or similar Data structure as input. In extension methods you can solve more complex use cases, but just that it needs to be used in same memory or otherwise use an Expression Serializer
– Mrinal Kamboj
Nov 16 '18 at 8:53
@MrinalKamboj SqlFunctions is a static class from the EntityFramework package with helpers functions. View reference here
– allamgr
Nov 16 '18 at 20:09
add a comment |
What isSqlFunctions
is it a custom class. You can solve more complex use cases using the extension methods onstring
and usingstring
orList<string>
or similar Data structure as input. In extension methods you can solve more complex use cases, but just that it needs to be used in same memory or otherwise use an Expression Serializer
– Mrinal Kamboj
Nov 16 '18 at 8:53
@MrinalKamboj SqlFunctions is a static class from the EntityFramework package with helpers functions. View reference here
– allamgr
Nov 16 '18 at 20:09
What is
SqlFunctions
is it a custom class. You can solve more complex use cases using the extension methods on string
and using string
or List<string>
or similar Data structure as input. In extension methods you can solve more complex use cases, but just that it needs to be used in same memory or otherwise use an Expression Serializer– Mrinal Kamboj
Nov 16 '18 at 8:53
What is
SqlFunctions
is it a custom class. You can solve more complex use cases using the extension methods on string
and using string
or List<string>
or similar Data structure as input. In extension methods you can solve more complex use cases, but just that it needs to be used in same memory or otherwise use an Expression Serializer– Mrinal Kamboj
Nov 16 '18 at 8:53
@MrinalKamboj SqlFunctions is a static class from the EntityFramework package with helpers functions. View reference here
– allamgr
Nov 16 '18 at 20:09
@MrinalKamboj SqlFunctions is a static class from the EntityFramework package with helpers functions. View reference here
– allamgr
Nov 16 '18 at 20:09
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331782%2finteger-contains-linq-c-sharp-but-using-using-expressions%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zl,D,KyOIPX2ZYe27gI71PB1ob pGtRn0FmDbIuyEy9H
There's no out of box way, you need to create a
MethodCallExpression
– Mrinal Kamboj
Nov 16 '18 at 5:12
do google search for a
predicatebuilder
library/class. i've used this one recently: gist.github.com/anomepani/…– JohnB
Nov 16 '18 at 5:13
@MrinalKamboj I have other filter for strings and I user Expression.Call but I dont know how to do this one.
– allamgr
Nov 16 '18 at 5:17