Bubble Sort from File in C
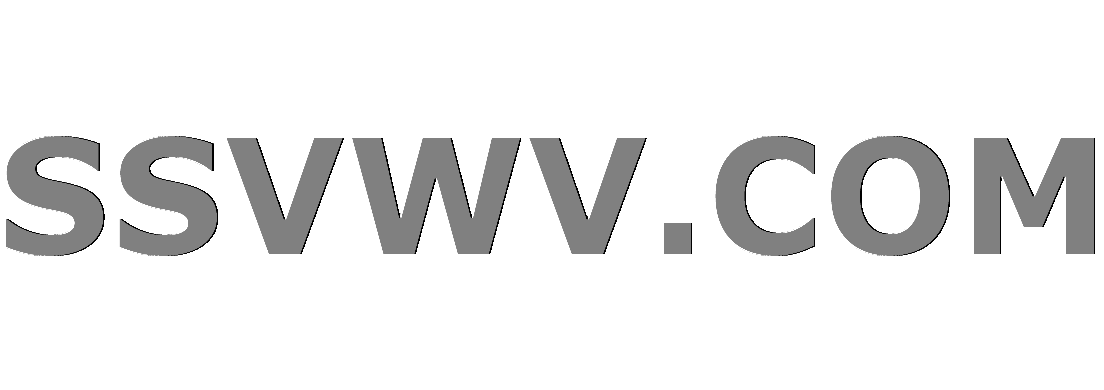
Multi tool use
up vote
1
down vote
favorite
I have try and figure why am I getting error messages to create bubble sort from file. It can be any from file depending on when I do labcheck command. This is over due but I really want to know why. The comments in the C programming are the directions by CS instructor. Any one can help how to fix the C program?
This is when I labcheck:
p8.c: In function ‘main’:
p8.c:63:24: warning: format ‘%s’ expects a matching ‘char *’ argument [-Wformat=]
printf ("Usage: %s <file>n");/*this should be followed as from
~^
p8.c:67:22: warning: implicit declaration of function ‘atof’; did you mean ‘feof’? [-Wimplicit-function-declaration]
char array = atof (argv[2]);
^~~~
feof
p8.c:67:22: error: invalid initializer
p8.c:68:18: warning: passing argument 1 of ‘bubblesort’ from incompatible pointer type [-Wincompatible-pointer-types]
bubblesort(array, 100);
^~~~~
p8.c:25:24: note: expected ‘char **’ but argument is of type ‘char *’
void bubblesort (char *A, int n)
~~~~~~^~~
make: *** [Makefile:37: p8.o] Error 1
-4.0 p8 failed to compile.
This is my code assignment:
/* 4 points */
#include <stdio.h>
#include <string.h>
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void bubblesort (char *A, int n)
int i, j, temp;
int length;
for (i = 0; i < length; i++)
for (j = 0; j < length - 1; j++)
if (*A[j + 1] < *A[j])
temp = *A[j];
*A[j] = *A[j + 1];
*A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int main (int argc, char *argv)
int rc, i;//rc is Read Character
if (argc < 2)
printf ("Usage: %s <file>n");/*this should be followed as from
instruction, nothing can be change due to error message.*/
rc = -1;
else
char array = atof (argv[2]);
bubblesort(array, 100);
rc = 0;
while (rc < i)
printf ("%cn", array[rc]);
rc++;
// prints print new lines from file
return 0;
c file bubble-sort
add a comment |
up vote
1
down vote
favorite
I have try and figure why am I getting error messages to create bubble sort from file. It can be any from file depending on when I do labcheck command. This is over due but I really want to know why. The comments in the C programming are the directions by CS instructor. Any one can help how to fix the C program?
This is when I labcheck:
p8.c: In function ‘main’:
p8.c:63:24: warning: format ‘%s’ expects a matching ‘char *’ argument [-Wformat=]
printf ("Usage: %s <file>n");/*this should be followed as from
~^
p8.c:67:22: warning: implicit declaration of function ‘atof’; did you mean ‘feof’? [-Wimplicit-function-declaration]
char array = atof (argv[2]);
^~~~
feof
p8.c:67:22: error: invalid initializer
p8.c:68:18: warning: passing argument 1 of ‘bubblesort’ from incompatible pointer type [-Wincompatible-pointer-types]
bubblesort(array, 100);
^~~~~
p8.c:25:24: note: expected ‘char **’ but argument is of type ‘char *’
void bubblesort (char *A, int n)
~~~~~~^~~
make: *** [Makefile:37: p8.o] Error 1
-4.0 p8 failed to compile.
This is my code assignment:
/* 4 points */
#include <stdio.h>
#include <string.h>
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void bubblesort (char *A, int n)
int i, j, temp;
int length;
for (i = 0; i < length; i++)
for (j = 0; j < length - 1; j++)
if (*A[j + 1] < *A[j])
temp = *A[j];
*A[j] = *A[j + 1];
*A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int main (int argc, char *argv)
int rc, i;//rc is Read Character
if (argc < 2)
printf ("Usage: %s <file>n");/*this should be followed as from
instruction, nothing can be change due to error message.*/
rc = -1;
else
char array = atof (argv[2]);
bubblesort(array, 100);
rc = 0;
while (rc < i)
printf ("%cn", array[rc]);
rc++;
// prints print new lines from file
return 0;
c file bubble-sort
Are you trying to read in the file and convert the values tofloat
or are you trying to read in a list of strings? There are a number of other bugs, but before I can help you, I need to know this.
– Craig Estey
Nov 10 at 21:09
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have try and figure why am I getting error messages to create bubble sort from file. It can be any from file depending on when I do labcheck command. This is over due but I really want to know why. The comments in the C programming are the directions by CS instructor. Any one can help how to fix the C program?
This is when I labcheck:
p8.c: In function ‘main’:
p8.c:63:24: warning: format ‘%s’ expects a matching ‘char *’ argument [-Wformat=]
printf ("Usage: %s <file>n");/*this should be followed as from
~^
p8.c:67:22: warning: implicit declaration of function ‘atof’; did you mean ‘feof’? [-Wimplicit-function-declaration]
char array = atof (argv[2]);
^~~~
feof
p8.c:67:22: error: invalid initializer
p8.c:68:18: warning: passing argument 1 of ‘bubblesort’ from incompatible pointer type [-Wincompatible-pointer-types]
bubblesort(array, 100);
^~~~~
p8.c:25:24: note: expected ‘char **’ but argument is of type ‘char *’
void bubblesort (char *A, int n)
~~~~~~^~~
make: *** [Makefile:37: p8.o] Error 1
-4.0 p8 failed to compile.
This is my code assignment:
/* 4 points */
#include <stdio.h>
#include <string.h>
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void bubblesort (char *A, int n)
int i, j, temp;
int length;
for (i = 0; i < length; i++)
for (j = 0; j < length - 1; j++)
if (*A[j + 1] < *A[j])
temp = *A[j];
*A[j] = *A[j + 1];
*A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int main (int argc, char *argv)
int rc, i;//rc is Read Character
if (argc < 2)
printf ("Usage: %s <file>n");/*this should be followed as from
instruction, nothing can be change due to error message.*/
rc = -1;
else
char array = atof (argv[2]);
bubblesort(array, 100);
rc = 0;
while (rc < i)
printf ("%cn", array[rc]);
rc++;
// prints print new lines from file
return 0;
c file bubble-sort
I have try and figure why am I getting error messages to create bubble sort from file. It can be any from file depending on when I do labcheck command. This is over due but I really want to know why. The comments in the C programming are the directions by CS instructor. Any one can help how to fix the C program?
This is when I labcheck:
p8.c: In function ‘main’:
p8.c:63:24: warning: format ‘%s’ expects a matching ‘char *’ argument [-Wformat=]
printf ("Usage: %s <file>n");/*this should be followed as from
~^
p8.c:67:22: warning: implicit declaration of function ‘atof’; did you mean ‘feof’? [-Wimplicit-function-declaration]
char array = atof (argv[2]);
^~~~
feof
p8.c:67:22: error: invalid initializer
p8.c:68:18: warning: passing argument 1 of ‘bubblesort’ from incompatible pointer type [-Wincompatible-pointer-types]
bubblesort(array, 100);
^~~~~
p8.c:25:24: note: expected ‘char **’ but argument is of type ‘char *’
void bubblesort (char *A, int n)
~~~~~~^~~
make: *** [Makefile:37: p8.o] Error 1
-4.0 p8 failed to compile.
This is my code assignment:
/* 4 points */
#include <stdio.h>
#include <string.h>
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void bubblesort (char *A, int n)
int i, j, temp;
int length;
for (i = 0; i < length; i++)
for (j = 0; j < length - 1; j++)
if (*A[j + 1] < *A[j])
temp = *A[j];
*A[j] = *A[j + 1];
*A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int main (int argc, char *argv)
int rc, i;//rc is Read Character
if (argc < 2)
printf ("Usage: %s <file>n");/*this should be followed as from
instruction, nothing can be change due to error message.*/
rc = -1;
else
char array = atof (argv[2]);
bubblesort(array, 100);
rc = 0;
while (rc < i)
printf ("%cn", array[rc]);
rc++;
// prints print new lines from file
return 0;
c file bubble-sort
c file bubble-sort
asked Nov 10 at 20:18
Devin Morlan
226
226
Are you trying to read in the file and convert the values tofloat
or are you trying to read in a list of strings? There are a number of other bugs, but before I can help you, I need to know this.
– Craig Estey
Nov 10 at 21:09
add a comment |
Are you trying to read in the file and convert the values tofloat
or are you trying to read in a list of strings? There are a number of other bugs, but before I can help you, I need to know this.
– Craig Estey
Nov 10 at 21:09
Are you trying to read in the file and convert the values to
float
or are you trying to read in a list of strings? There are a number of other bugs, but before I can help you, I need to know this.– Craig Estey
Nov 10 at 21:09
Are you trying to read in the file and convert the values to
float
or are you trying to read in a list of strings? There are a number of other bugs, but before I can help you, I need to know this.– Craig Estey
Nov 10 at 21:09
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
There are a number of bugs. I created two versions of your code. One with the bugs annotated. And another cleaned up and working version.
Here's the annotated version:
/* 4 points */
#include <stdio.h>
#include <string.h>
// NOTE/BUG: we need this for atof
#if 1
#include <stdlib.h>
#include <errno.h>
#endif
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i,
j,
temp;
int length;
// NOTE/BUG: temp needs to be "char *temp"
// NOTE/BUG: "length" should be "n" here
// NOTE/BUG: replace all "*A[whatever]" with "A[whatever]"
for (i = 0; i < length; i++)
for (j = 0; j < length - 1; j++)
// NOTE/BUG: we need strcmp here
if (*A[j + 1] < *A[j])
temp = *A[j];
*A[j] = *A[j + 1];
*A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc,
i; // rc is Read Character
if (argc < 2)
/* this should be followed as from instruction, nothing can be change due to
error message. */
// NOTE/BUG: this is missing the argv[0] argument
#if 0
printf("Usage: %s <file>n");
#else
printf("Usage: %s <file>n",argv[0]);
#endif
rc = -1;
else
// NOTE/BUG: this is too short (i.e. it needs to be 100)
// NOTE/BUG: we need to read in the values
#if 0
char array = atof(argv[2]);
bubblesort(array, 100);
#else
char *array[100];
// NOTE/BUG: this should be argv[1]
FILE *fi = fopen(argv[2],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[2],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[1000];
char *cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(cp);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
#endif
rc = 0;
while (rc < i)
// NOTE/BUG: this should be "%s"
printf("%cn", array[rc]);
rc++;
// prints print new lines from file
return 0;
Here's the cleaned up and working version:
/* 4 points */
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <errno.h>
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i, j;
char *temp;
for (i = 0; i < n; i++)
for (j = 0; j < n - 1; j++)
if (strcmp(A[j + 1],A[j]) < 0)
temp = A[j];
A[j] = A[j + 1];
A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc = 0;
if (argc < 2)
/* this should be followed as from instruction, nothing can be change
due to error message. */
printf("Usage: %s <file>n",argv[0]);
rc = 1;
else
char *array[100];
int arrmax = sizeof(array) / sizeof(array[0]);
FILE *fi = fopen(argv[1],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[1],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[MAXLEN];
char *cp;
cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
if (arrcnt >= arrmax)
printf("too many input linesn");
exit(1);
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(buf);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
for (int arridx = 0; arridx < arrcnt; ++arridx)
printf("%sn", array[arridx]);
// prints print new lines from file
return rc;
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
There are a number of bugs. I created two versions of your code. One with the bugs annotated. And another cleaned up and working version.
Here's the annotated version:
/* 4 points */
#include <stdio.h>
#include <string.h>
// NOTE/BUG: we need this for atof
#if 1
#include <stdlib.h>
#include <errno.h>
#endif
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i,
j,
temp;
int length;
// NOTE/BUG: temp needs to be "char *temp"
// NOTE/BUG: "length" should be "n" here
// NOTE/BUG: replace all "*A[whatever]" with "A[whatever]"
for (i = 0; i < length; i++)
for (j = 0; j < length - 1; j++)
// NOTE/BUG: we need strcmp here
if (*A[j + 1] < *A[j])
temp = *A[j];
*A[j] = *A[j + 1];
*A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc,
i; // rc is Read Character
if (argc < 2)
/* this should be followed as from instruction, nothing can be change due to
error message. */
// NOTE/BUG: this is missing the argv[0] argument
#if 0
printf("Usage: %s <file>n");
#else
printf("Usage: %s <file>n",argv[0]);
#endif
rc = -1;
else
// NOTE/BUG: this is too short (i.e. it needs to be 100)
// NOTE/BUG: we need to read in the values
#if 0
char array = atof(argv[2]);
bubblesort(array, 100);
#else
char *array[100];
// NOTE/BUG: this should be argv[1]
FILE *fi = fopen(argv[2],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[2],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[1000];
char *cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(cp);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
#endif
rc = 0;
while (rc < i)
// NOTE/BUG: this should be "%s"
printf("%cn", array[rc]);
rc++;
// prints print new lines from file
return 0;
Here's the cleaned up and working version:
/* 4 points */
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <errno.h>
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i, j;
char *temp;
for (i = 0; i < n; i++)
for (j = 0; j < n - 1; j++)
if (strcmp(A[j + 1],A[j]) < 0)
temp = A[j];
A[j] = A[j + 1];
A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc = 0;
if (argc < 2)
/* this should be followed as from instruction, nothing can be change
due to error message. */
printf("Usage: %s <file>n",argv[0]);
rc = 1;
else
char *array[100];
int arrmax = sizeof(array) / sizeof(array[0]);
FILE *fi = fopen(argv[1],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[1],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[MAXLEN];
char *cp;
cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
if (arrcnt >= arrmax)
printf("too many input linesn");
exit(1);
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(buf);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
for (int arridx = 0; arridx < arrcnt; ++arridx)
printf("%sn", array[arridx]);
// prints print new lines from file
return rc;
add a comment |
up vote
2
down vote
There are a number of bugs. I created two versions of your code. One with the bugs annotated. And another cleaned up and working version.
Here's the annotated version:
/* 4 points */
#include <stdio.h>
#include <string.h>
// NOTE/BUG: we need this for atof
#if 1
#include <stdlib.h>
#include <errno.h>
#endif
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i,
j,
temp;
int length;
// NOTE/BUG: temp needs to be "char *temp"
// NOTE/BUG: "length" should be "n" here
// NOTE/BUG: replace all "*A[whatever]" with "A[whatever]"
for (i = 0; i < length; i++)
for (j = 0; j < length - 1; j++)
// NOTE/BUG: we need strcmp here
if (*A[j + 1] < *A[j])
temp = *A[j];
*A[j] = *A[j + 1];
*A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc,
i; // rc is Read Character
if (argc < 2)
/* this should be followed as from instruction, nothing can be change due to
error message. */
// NOTE/BUG: this is missing the argv[0] argument
#if 0
printf("Usage: %s <file>n");
#else
printf("Usage: %s <file>n",argv[0]);
#endif
rc = -1;
else
// NOTE/BUG: this is too short (i.e. it needs to be 100)
// NOTE/BUG: we need to read in the values
#if 0
char array = atof(argv[2]);
bubblesort(array, 100);
#else
char *array[100];
// NOTE/BUG: this should be argv[1]
FILE *fi = fopen(argv[2],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[2],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[1000];
char *cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(cp);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
#endif
rc = 0;
while (rc < i)
// NOTE/BUG: this should be "%s"
printf("%cn", array[rc]);
rc++;
// prints print new lines from file
return 0;
Here's the cleaned up and working version:
/* 4 points */
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <errno.h>
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i, j;
char *temp;
for (i = 0; i < n; i++)
for (j = 0; j < n - 1; j++)
if (strcmp(A[j + 1],A[j]) < 0)
temp = A[j];
A[j] = A[j + 1];
A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc = 0;
if (argc < 2)
/* this should be followed as from instruction, nothing can be change
due to error message. */
printf("Usage: %s <file>n",argv[0]);
rc = 1;
else
char *array[100];
int arrmax = sizeof(array) / sizeof(array[0]);
FILE *fi = fopen(argv[1],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[1],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[MAXLEN];
char *cp;
cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
if (arrcnt >= arrmax)
printf("too many input linesn");
exit(1);
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(buf);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
for (int arridx = 0; arridx < arrcnt; ++arridx)
printf("%sn", array[arridx]);
// prints print new lines from file
return rc;
add a comment |
up vote
2
down vote
up vote
2
down vote
There are a number of bugs. I created two versions of your code. One with the bugs annotated. And another cleaned up and working version.
Here's the annotated version:
/* 4 points */
#include <stdio.h>
#include <string.h>
// NOTE/BUG: we need this for atof
#if 1
#include <stdlib.h>
#include <errno.h>
#endif
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i,
j,
temp;
int length;
// NOTE/BUG: temp needs to be "char *temp"
// NOTE/BUG: "length" should be "n" here
// NOTE/BUG: replace all "*A[whatever]" with "A[whatever]"
for (i = 0; i < length; i++)
for (j = 0; j < length - 1; j++)
// NOTE/BUG: we need strcmp here
if (*A[j + 1] < *A[j])
temp = *A[j];
*A[j] = *A[j + 1];
*A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc,
i; // rc is Read Character
if (argc < 2)
/* this should be followed as from instruction, nothing can be change due to
error message. */
// NOTE/BUG: this is missing the argv[0] argument
#if 0
printf("Usage: %s <file>n");
#else
printf("Usage: %s <file>n",argv[0]);
#endif
rc = -1;
else
// NOTE/BUG: this is too short (i.e. it needs to be 100)
// NOTE/BUG: we need to read in the values
#if 0
char array = atof(argv[2]);
bubblesort(array, 100);
#else
char *array[100];
// NOTE/BUG: this should be argv[1]
FILE *fi = fopen(argv[2],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[2],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[1000];
char *cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(cp);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
#endif
rc = 0;
while (rc < i)
// NOTE/BUG: this should be "%s"
printf("%cn", array[rc]);
rc++;
// prints print new lines from file
return 0;
Here's the cleaned up and working version:
/* 4 points */
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <errno.h>
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i, j;
char *temp;
for (i = 0; i < n; i++)
for (j = 0; j < n - 1; j++)
if (strcmp(A[j + 1],A[j]) < 0)
temp = A[j];
A[j] = A[j + 1];
A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc = 0;
if (argc < 2)
/* this should be followed as from instruction, nothing can be change
due to error message. */
printf("Usage: %s <file>n",argv[0]);
rc = 1;
else
char *array[100];
int arrmax = sizeof(array) / sizeof(array[0]);
FILE *fi = fopen(argv[1],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[1],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[MAXLEN];
char *cp;
cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
if (arrcnt >= arrmax)
printf("too many input linesn");
exit(1);
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(buf);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
for (int arridx = 0; arridx < arrcnt; ++arridx)
printf("%sn", array[arridx]);
// prints print new lines from file
return rc;
There are a number of bugs. I created two versions of your code. One with the bugs annotated. And another cleaned up and working version.
Here's the annotated version:
/* 4 points */
#include <stdio.h>
#include <string.h>
// NOTE/BUG: we need this for atof
#if 1
#include <stdlib.h>
#include <errno.h>
#endif
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i,
j,
temp;
int length;
// NOTE/BUG: temp needs to be "char *temp"
// NOTE/BUG: "length" should be "n" here
// NOTE/BUG: replace all "*A[whatever]" with "A[whatever]"
for (i = 0; i < length; i++)
for (j = 0; j < length - 1; j++)
// NOTE/BUG: we need strcmp here
if (*A[j + 1] < *A[j])
temp = *A[j];
*A[j] = *A[j + 1];
*A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc,
i; // rc is Read Character
if (argc < 2)
/* this should be followed as from instruction, nothing can be change due to
error message. */
// NOTE/BUG: this is missing the argv[0] argument
#if 0
printf("Usage: %s <file>n");
#else
printf("Usage: %s <file>n",argv[0]);
#endif
rc = -1;
else
// NOTE/BUG: this is too short (i.e. it needs to be 100)
// NOTE/BUG: we need to read in the values
#if 0
char array = atof(argv[2]);
bubblesort(array, 100);
#else
char *array[100];
// NOTE/BUG: this should be argv[1]
FILE *fi = fopen(argv[2],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[2],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[1000];
char *cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(cp);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
#endif
rc = 0;
while (rc < i)
// NOTE/BUG: this should be "%s"
printf("%cn", array[rc]);
rc++;
// prints print new lines from file
return 0;
Here's the cleaned up and working version:
/* 4 points */
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <errno.h>
#define MAXLEN 1000
/**
* Fill in the bubblesort function: https://en.wikipedia.org/wiki/Bubble_sort
* Using this pseudo-code, convert to C code:
* procedure bubbleSort( A : list of sortable items, n : length(A) )
* repeat
* newn = 0
* for i = 1 to n-1 inclusive do
* if A[i-1] > A[i] then
*
swap(A[i-1], A[i])
* newn = i
* end if
* end for
* n = newn
* until n <= 1
* end procedure
*/
void
bubblesort(char *A, int n)
int i, j;
char *temp;
for (i = 0; i < n; i++)
for (j = 0; j < n - 1; j++)
if (strcmp(A[j + 1],A[j]) < 0)
temp = A[j];
A[j] = A[j + 1];
A[j + 1] = temp;
/**
* Create a main function that opens a file composed of words, one per line
* and sorts them using the bubble-sort algorithm.
* Usage: "Usage: %s <file>n"
* Example input/output:
* ./p8 testfile
* Bob
* Bubbles
* Butters
* Dave
* ...
*/
int
main(int argc, char *argv)
int rc = 0;
if (argc < 2)
/* this should be followed as from instruction, nothing can be change
due to error message. */
printf("Usage: %s <file>n",argv[0]);
rc = 1;
else
char *array[100];
int arrmax = sizeof(array) / sizeof(array[0]);
FILE *fi = fopen(argv[1],"r");
if (fi == NULL)
printf("unable to open '%s' -- %sn",argv[1],strerror(errno));
exit(1);
int arrcnt = 0;
while (1)
char buf[MAXLEN];
char *cp;
cp = fgets(buf,sizeof(buf),fi);
if (cp == NULL)
break;
if (arrcnt >= arrmax)
printf("too many input linesn");
exit(1);
cp = strchr(buf,'n');
if (cp != NULL)
*cp = 0;
cp = strdup(buf);
array[arrcnt++] = cp;
fclose(fi);
bubblesort(array,arrcnt);
for (int arridx = 0; arridx < arrcnt; ++arridx)
printf("%sn", array[arridx]);
// prints print new lines from file
return rc;
edited Nov 10 at 21:57
answered Nov 10 at 21:37
Craig Estey
13.5k21029
13.5k21029
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243048%2fbubble-sort-from-file-in-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wfzlU4EMBWBJqPk
Are you trying to read in the file and convert the values to
float
or are you trying to read in a list of strings? There are a number of other bugs, but before I can help you, I need to know this.– Craig Estey
Nov 10 at 21:09