Image Classification Using TensorFlow, Value error
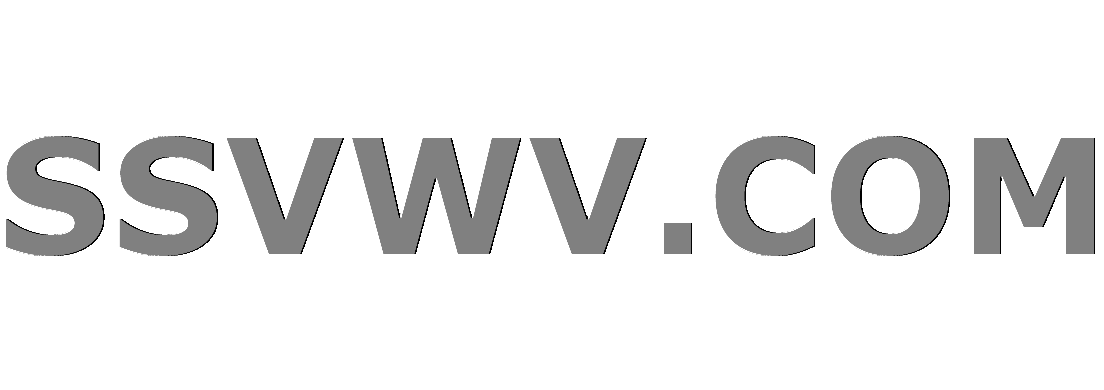
Multi tool use
up vote
0
down vote
favorite
I am new to deep learning and tensorflow and i'm trying to create an image classifier using tensorflow that will classify 5 classes of images.
My training dataset is 25000 images and testing dataset is 5000 images.
below is my code:
import os
from random import shuffle
import cv2
import matplotlib.pyplot as plt
import numpy as np
import tensorflow as tf
import tflearn
from tflearn.layers.conv import conv_2d, max_pool_2d
from tflearn.layers.core import input_data, dropout, fully_connected
from tflearn.layers.estimator import regression
from tqdm import tqdm
import keras
from keras.models import save_model
"""from keras.models import Sequential
from keras.layers import Dense"""
TRAIN_DIR = 'train'
TEST_DIR = 'test'
IMG_SIZE = 16
LR = 1e-3
MODEL_NAME = 'cifar 10 -convnet'
def create_label(image_name):
""" Create an one-hot encoded vector from image name """
word_label = image_name.split('.')[0:2]
if word_label == 'cat':
return np.array([1,0,0,0,0])
elif word_label == 'Dog':
return np.array([0,1,0,0,0])
elif word_label == 'Automobile':
return np.array([0,0,1,0,0])
elif word_label == 'Airplane':
return np.array([0,0,0,1,0])
elif word_label == 'Ship':
return np.array([0,0,0,0,1])
def create_train_data():
training_data =
for img in tqdm(os.listdir(TRAIN_DIR)):
path = os.path.join(TRAIN_DIR, img)
img_data = cv2.imread(path, cv2.IMREAD_GRAYSCALE)
img_data = cv2.resize(img_data, (IMG_SIZE, IMG_SIZE))
training_data.append([np.array(img_data), create_label(img)])
shuffle(training_data)
np.save('train_data.npy', training_data)
return training_data
def create_test_data():
testing_data =
for img in tqdm(os.listdir(TEST_DIR)):
path = os.path.join(TEST_DIR, img)
img_num = img.split('.')[0:2]
img_data = cv2.imread(path, cv2.IMREAD_GRAYSCALE)
img_data = cv2.resize(img_data, (IMG_SIZE, IMG_SIZE))
testing_data.append([np.array(img_data), img_num])
shuffle(testing_data)
np.save('test_data.npy', testing_data)
return testing_data
# If dataset is not created:
train_data = create_train_data()
test_data = create_test_data()
# If you have already created the dataset:
# train_data = np.load('train_data.npy')
# test_data = np.load('test_data.npy')
train = train_data[:25000]
test = train_data[:5000]
X_train = np.array([i[0] for i in train]).reshape(-1, IMG_SIZE, IMG_SIZE, 1)
y_train = [i[1] for i in train]
X_test = np.array([i[0] for i in test]).reshape(-1, IMG_SIZE, IMG_SIZE, 1)
y_test = [i[1] for i in test]
# Building The Model
tf.reset_default_graph()
convnet = input_data(shape=[None, IMG_SIZE, IMG_SIZE, 1], name='input')
convnet = conv_2d(convnet, 32, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 64, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 128, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 64, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 32, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = fully_connected(convnet, 1024, activation='relu')
convnet = dropout(convnet, 0.8)
convnet = fully_connected(convnet, 2, activation='softmax')
convnet = regression(convnet, optimizer='adam', learning_rate=LR, loss='categorical_crossentropy', name='targets')
model = tflearn.DNN(convnet, tensorboard_dir='log', tensorboard_verbose=0)
history = model.fit('input': X_train, 'targets': y_train, n_epoch=25,
validation_set=('input': X_test, 'targets': y_test),
snapshot_step=500, show_metric=True, run_id=MODEL_NAME)
#print(history.history.keys())
#plt.figure(1)
# summarize history for accuracy
"""plt.subplot(211)
plt.plot(history.history['acc'])
plt.plot(history.history['val_acc'])
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['train', 'test1'], loc='upper left')
plt.show()"""
fig = plt.figure(figsize=(25, 12))
for num, data in enumerate(test_data[:25]):
img_num = data[1]
img_data = data[0]
y = fig.add_subplot(5, 5, num + 1)
orig = img_data
data = img_data.reshape(IMG_SIZE, IMG_SIZE, 1)
model_out = model.predict([data])[0]
if np.argmax(model_out) == 1:
str_label = 'Dog'
if np.argmax(model_out) == 2:
str_label = 'Automobile'
if np.argmax(model_out) == 3:
str_label = 'Airplane'
if np.argmax(model_out) == 4:
str_label = 'Ship'
else:
str_label = 'Cat'
y.imshow(orig, cmap='gray')
plt.title(str_label)
y.axes.get_xaxis().set_visible(False)
y.axes.get_yaxis().set_visible(False)
plt.show()
i'm getting following error:
ValueError: Cannot feed value of shape (64,) for Tensor 'targets/Y:0', which has shape '(?, 2)'
Can someone help me with this?
thanks in advance.
python tensorflow conv-neural-network tflearn
add a comment |
up vote
0
down vote
favorite
I am new to deep learning and tensorflow and i'm trying to create an image classifier using tensorflow that will classify 5 classes of images.
My training dataset is 25000 images and testing dataset is 5000 images.
below is my code:
import os
from random import shuffle
import cv2
import matplotlib.pyplot as plt
import numpy as np
import tensorflow as tf
import tflearn
from tflearn.layers.conv import conv_2d, max_pool_2d
from tflearn.layers.core import input_data, dropout, fully_connected
from tflearn.layers.estimator import regression
from tqdm import tqdm
import keras
from keras.models import save_model
"""from keras.models import Sequential
from keras.layers import Dense"""
TRAIN_DIR = 'train'
TEST_DIR = 'test'
IMG_SIZE = 16
LR = 1e-3
MODEL_NAME = 'cifar 10 -convnet'
def create_label(image_name):
""" Create an one-hot encoded vector from image name """
word_label = image_name.split('.')[0:2]
if word_label == 'cat':
return np.array([1,0,0,0,0])
elif word_label == 'Dog':
return np.array([0,1,0,0,0])
elif word_label == 'Automobile':
return np.array([0,0,1,0,0])
elif word_label == 'Airplane':
return np.array([0,0,0,1,0])
elif word_label == 'Ship':
return np.array([0,0,0,0,1])
def create_train_data():
training_data =
for img in tqdm(os.listdir(TRAIN_DIR)):
path = os.path.join(TRAIN_DIR, img)
img_data = cv2.imread(path, cv2.IMREAD_GRAYSCALE)
img_data = cv2.resize(img_data, (IMG_SIZE, IMG_SIZE))
training_data.append([np.array(img_data), create_label(img)])
shuffle(training_data)
np.save('train_data.npy', training_data)
return training_data
def create_test_data():
testing_data =
for img in tqdm(os.listdir(TEST_DIR)):
path = os.path.join(TEST_DIR, img)
img_num = img.split('.')[0:2]
img_data = cv2.imread(path, cv2.IMREAD_GRAYSCALE)
img_data = cv2.resize(img_data, (IMG_SIZE, IMG_SIZE))
testing_data.append([np.array(img_data), img_num])
shuffle(testing_data)
np.save('test_data.npy', testing_data)
return testing_data
# If dataset is not created:
train_data = create_train_data()
test_data = create_test_data()
# If you have already created the dataset:
# train_data = np.load('train_data.npy')
# test_data = np.load('test_data.npy')
train = train_data[:25000]
test = train_data[:5000]
X_train = np.array([i[0] for i in train]).reshape(-1, IMG_SIZE, IMG_SIZE, 1)
y_train = [i[1] for i in train]
X_test = np.array([i[0] for i in test]).reshape(-1, IMG_SIZE, IMG_SIZE, 1)
y_test = [i[1] for i in test]
# Building The Model
tf.reset_default_graph()
convnet = input_data(shape=[None, IMG_SIZE, IMG_SIZE, 1], name='input')
convnet = conv_2d(convnet, 32, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 64, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 128, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 64, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 32, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = fully_connected(convnet, 1024, activation='relu')
convnet = dropout(convnet, 0.8)
convnet = fully_connected(convnet, 2, activation='softmax')
convnet = regression(convnet, optimizer='adam', learning_rate=LR, loss='categorical_crossentropy', name='targets')
model = tflearn.DNN(convnet, tensorboard_dir='log', tensorboard_verbose=0)
history = model.fit('input': X_train, 'targets': y_train, n_epoch=25,
validation_set=('input': X_test, 'targets': y_test),
snapshot_step=500, show_metric=True, run_id=MODEL_NAME)
#print(history.history.keys())
#plt.figure(1)
# summarize history for accuracy
"""plt.subplot(211)
plt.plot(history.history['acc'])
plt.plot(history.history['val_acc'])
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['train', 'test1'], loc='upper left')
plt.show()"""
fig = plt.figure(figsize=(25, 12))
for num, data in enumerate(test_data[:25]):
img_num = data[1]
img_data = data[0]
y = fig.add_subplot(5, 5, num + 1)
orig = img_data
data = img_data.reshape(IMG_SIZE, IMG_SIZE, 1)
model_out = model.predict([data])[0]
if np.argmax(model_out) == 1:
str_label = 'Dog'
if np.argmax(model_out) == 2:
str_label = 'Automobile'
if np.argmax(model_out) == 3:
str_label = 'Airplane'
if np.argmax(model_out) == 4:
str_label = 'Ship'
else:
str_label = 'Cat'
y.imshow(orig, cmap='gray')
plt.title(str_label)
y.axes.get_xaxis().set_visible(False)
y.axes.get_yaxis().set_visible(False)
plt.show()
i'm getting following error:
ValueError: Cannot feed value of shape (64,) for Tensor 'targets/Y:0', which has shape '(?, 2)'
Can someone help me with this?
thanks in advance.
python tensorflow conv-neural-network tflearn
Your network is expecting a 2D tensor, and you're giving it a 1D tensor. Does that make sense?
– Josh Friedlander
Nov 10 at 19:06
@JoshFriedlander can you tell me how to do that? can you provide me code?
– Atif Ali
Nov 10 at 19:57
@JoshFriedlander can you please tell which line should be changed to what exactly?
– Atif Ali
Nov 10 at 20:00
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am new to deep learning and tensorflow and i'm trying to create an image classifier using tensorflow that will classify 5 classes of images.
My training dataset is 25000 images and testing dataset is 5000 images.
below is my code:
import os
from random import shuffle
import cv2
import matplotlib.pyplot as plt
import numpy as np
import tensorflow as tf
import tflearn
from tflearn.layers.conv import conv_2d, max_pool_2d
from tflearn.layers.core import input_data, dropout, fully_connected
from tflearn.layers.estimator import regression
from tqdm import tqdm
import keras
from keras.models import save_model
"""from keras.models import Sequential
from keras.layers import Dense"""
TRAIN_DIR = 'train'
TEST_DIR = 'test'
IMG_SIZE = 16
LR = 1e-3
MODEL_NAME = 'cifar 10 -convnet'
def create_label(image_name):
""" Create an one-hot encoded vector from image name """
word_label = image_name.split('.')[0:2]
if word_label == 'cat':
return np.array([1,0,0,0,0])
elif word_label == 'Dog':
return np.array([0,1,0,0,0])
elif word_label == 'Automobile':
return np.array([0,0,1,0,0])
elif word_label == 'Airplane':
return np.array([0,0,0,1,0])
elif word_label == 'Ship':
return np.array([0,0,0,0,1])
def create_train_data():
training_data =
for img in tqdm(os.listdir(TRAIN_DIR)):
path = os.path.join(TRAIN_DIR, img)
img_data = cv2.imread(path, cv2.IMREAD_GRAYSCALE)
img_data = cv2.resize(img_data, (IMG_SIZE, IMG_SIZE))
training_data.append([np.array(img_data), create_label(img)])
shuffle(training_data)
np.save('train_data.npy', training_data)
return training_data
def create_test_data():
testing_data =
for img in tqdm(os.listdir(TEST_DIR)):
path = os.path.join(TEST_DIR, img)
img_num = img.split('.')[0:2]
img_data = cv2.imread(path, cv2.IMREAD_GRAYSCALE)
img_data = cv2.resize(img_data, (IMG_SIZE, IMG_SIZE))
testing_data.append([np.array(img_data), img_num])
shuffle(testing_data)
np.save('test_data.npy', testing_data)
return testing_data
# If dataset is not created:
train_data = create_train_data()
test_data = create_test_data()
# If you have already created the dataset:
# train_data = np.load('train_data.npy')
# test_data = np.load('test_data.npy')
train = train_data[:25000]
test = train_data[:5000]
X_train = np.array([i[0] for i in train]).reshape(-1, IMG_SIZE, IMG_SIZE, 1)
y_train = [i[1] for i in train]
X_test = np.array([i[0] for i in test]).reshape(-1, IMG_SIZE, IMG_SIZE, 1)
y_test = [i[1] for i in test]
# Building The Model
tf.reset_default_graph()
convnet = input_data(shape=[None, IMG_SIZE, IMG_SIZE, 1], name='input')
convnet = conv_2d(convnet, 32, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 64, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 128, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 64, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 32, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = fully_connected(convnet, 1024, activation='relu')
convnet = dropout(convnet, 0.8)
convnet = fully_connected(convnet, 2, activation='softmax')
convnet = regression(convnet, optimizer='adam', learning_rate=LR, loss='categorical_crossentropy', name='targets')
model = tflearn.DNN(convnet, tensorboard_dir='log', tensorboard_verbose=0)
history = model.fit('input': X_train, 'targets': y_train, n_epoch=25,
validation_set=('input': X_test, 'targets': y_test),
snapshot_step=500, show_metric=True, run_id=MODEL_NAME)
#print(history.history.keys())
#plt.figure(1)
# summarize history for accuracy
"""plt.subplot(211)
plt.plot(history.history['acc'])
plt.plot(history.history['val_acc'])
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['train', 'test1'], loc='upper left')
plt.show()"""
fig = plt.figure(figsize=(25, 12))
for num, data in enumerate(test_data[:25]):
img_num = data[1]
img_data = data[0]
y = fig.add_subplot(5, 5, num + 1)
orig = img_data
data = img_data.reshape(IMG_SIZE, IMG_SIZE, 1)
model_out = model.predict([data])[0]
if np.argmax(model_out) == 1:
str_label = 'Dog'
if np.argmax(model_out) == 2:
str_label = 'Automobile'
if np.argmax(model_out) == 3:
str_label = 'Airplane'
if np.argmax(model_out) == 4:
str_label = 'Ship'
else:
str_label = 'Cat'
y.imshow(orig, cmap='gray')
plt.title(str_label)
y.axes.get_xaxis().set_visible(False)
y.axes.get_yaxis().set_visible(False)
plt.show()
i'm getting following error:
ValueError: Cannot feed value of shape (64,) for Tensor 'targets/Y:0', which has shape '(?, 2)'
Can someone help me with this?
thanks in advance.
python tensorflow conv-neural-network tflearn
I am new to deep learning and tensorflow and i'm trying to create an image classifier using tensorflow that will classify 5 classes of images.
My training dataset is 25000 images and testing dataset is 5000 images.
below is my code:
import os
from random import shuffle
import cv2
import matplotlib.pyplot as plt
import numpy as np
import tensorflow as tf
import tflearn
from tflearn.layers.conv import conv_2d, max_pool_2d
from tflearn.layers.core import input_data, dropout, fully_connected
from tflearn.layers.estimator import regression
from tqdm import tqdm
import keras
from keras.models import save_model
"""from keras.models import Sequential
from keras.layers import Dense"""
TRAIN_DIR = 'train'
TEST_DIR = 'test'
IMG_SIZE = 16
LR = 1e-3
MODEL_NAME = 'cifar 10 -convnet'
def create_label(image_name):
""" Create an one-hot encoded vector from image name """
word_label = image_name.split('.')[0:2]
if word_label == 'cat':
return np.array([1,0,0,0,0])
elif word_label == 'Dog':
return np.array([0,1,0,0,0])
elif word_label == 'Automobile':
return np.array([0,0,1,0,0])
elif word_label == 'Airplane':
return np.array([0,0,0,1,0])
elif word_label == 'Ship':
return np.array([0,0,0,0,1])
def create_train_data():
training_data =
for img in tqdm(os.listdir(TRAIN_DIR)):
path = os.path.join(TRAIN_DIR, img)
img_data = cv2.imread(path, cv2.IMREAD_GRAYSCALE)
img_data = cv2.resize(img_data, (IMG_SIZE, IMG_SIZE))
training_data.append([np.array(img_data), create_label(img)])
shuffle(training_data)
np.save('train_data.npy', training_data)
return training_data
def create_test_data():
testing_data =
for img in tqdm(os.listdir(TEST_DIR)):
path = os.path.join(TEST_DIR, img)
img_num = img.split('.')[0:2]
img_data = cv2.imread(path, cv2.IMREAD_GRAYSCALE)
img_data = cv2.resize(img_data, (IMG_SIZE, IMG_SIZE))
testing_data.append([np.array(img_data), img_num])
shuffle(testing_data)
np.save('test_data.npy', testing_data)
return testing_data
# If dataset is not created:
train_data = create_train_data()
test_data = create_test_data()
# If you have already created the dataset:
# train_data = np.load('train_data.npy')
# test_data = np.load('test_data.npy')
train = train_data[:25000]
test = train_data[:5000]
X_train = np.array([i[0] for i in train]).reshape(-1, IMG_SIZE, IMG_SIZE, 1)
y_train = [i[1] for i in train]
X_test = np.array([i[0] for i in test]).reshape(-1, IMG_SIZE, IMG_SIZE, 1)
y_test = [i[1] for i in test]
# Building The Model
tf.reset_default_graph()
convnet = input_data(shape=[None, IMG_SIZE, IMG_SIZE, 1], name='input')
convnet = conv_2d(convnet, 32, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 64, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 128, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 64, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = conv_2d(convnet, 32, 5, activation='relu')
convnet = max_pool_2d(convnet, 5)
convnet = fully_connected(convnet, 1024, activation='relu')
convnet = dropout(convnet, 0.8)
convnet = fully_connected(convnet, 2, activation='softmax')
convnet = regression(convnet, optimizer='adam', learning_rate=LR, loss='categorical_crossentropy', name='targets')
model = tflearn.DNN(convnet, tensorboard_dir='log', tensorboard_verbose=0)
history = model.fit('input': X_train, 'targets': y_train, n_epoch=25,
validation_set=('input': X_test, 'targets': y_test),
snapshot_step=500, show_metric=True, run_id=MODEL_NAME)
#print(history.history.keys())
#plt.figure(1)
# summarize history for accuracy
"""plt.subplot(211)
plt.plot(history.history['acc'])
plt.plot(history.history['val_acc'])
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['train', 'test1'], loc='upper left')
plt.show()"""
fig = plt.figure(figsize=(25, 12))
for num, data in enumerate(test_data[:25]):
img_num = data[1]
img_data = data[0]
y = fig.add_subplot(5, 5, num + 1)
orig = img_data
data = img_data.reshape(IMG_SIZE, IMG_SIZE, 1)
model_out = model.predict([data])[0]
if np.argmax(model_out) == 1:
str_label = 'Dog'
if np.argmax(model_out) == 2:
str_label = 'Automobile'
if np.argmax(model_out) == 3:
str_label = 'Airplane'
if np.argmax(model_out) == 4:
str_label = 'Ship'
else:
str_label = 'Cat'
y.imshow(orig, cmap='gray')
plt.title(str_label)
y.axes.get_xaxis().set_visible(False)
y.axes.get_yaxis().set_visible(False)
plt.show()
i'm getting following error:
ValueError: Cannot feed value of shape (64,) for Tensor 'targets/Y:0', which has shape '(?, 2)'
Can someone help me with this?
thanks in advance.
python tensorflow conv-neural-network tflearn
python tensorflow conv-neural-network tflearn
asked Nov 10 at 18:49
Atif Ali
184
184
Your network is expecting a 2D tensor, and you're giving it a 1D tensor. Does that make sense?
– Josh Friedlander
Nov 10 at 19:06
@JoshFriedlander can you tell me how to do that? can you provide me code?
– Atif Ali
Nov 10 at 19:57
@JoshFriedlander can you please tell which line should be changed to what exactly?
– Atif Ali
Nov 10 at 20:00
add a comment |
Your network is expecting a 2D tensor, and you're giving it a 1D tensor. Does that make sense?
– Josh Friedlander
Nov 10 at 19:06
@JoshFriedlander can you tell me how to do that? can you provide me code?
– Atif Ali
Nov 10 at 19:57
@JoshFriedlander can you please tell which line should be changed to what exactly?
– Atif Ali
Nov 10 at 20:00
Your network is expecting a 2D tensor, and you're giving it a 1D tensor. Does that make sense?
– Josh Friedlander
Nov 10 at 19:06
Your network is expecting a 2D tensor, and you're giving it a 1D tensor. Does that make sense?
– Josh Friedlander
Nov 10 at 19:06
@JoshFriedlander can you tell me how to do that? can you provide me code?
– Atif Ali
Nov 10 at 19:57
@JoshFriedlander can you tell me how to do that? can you provide me code?
– Atif Ali
Nov 10 at 19:57
@JoshFriedlander can you please tell which line should be changed to what exactly?
– Atif Ali
Nov 10 at 20:00
@JoshFriedlander can you please tell which line should be changed to what exactly?
– Atif Ali
Nov 10 at 20:00
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
The problem is with the shape of your labels, so check the shape for y_train
and y_test
. Both of them should have to have a shape similar to the out put of your model, which is shown at the error ...which has shape '(?, 2)'
and defined at the line where you create the last fully connected layer convnet = fully_connected(convnet, 2, activation='softmax')
.
so what should i do? can you help? i cant understand it clearly...
– Atif Ali
Nov 15 at 13:56
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
The problem is with the shape of your labels, so check the shape for y_train
and y_test
. Both of them should have to have a shape similar to the out put of your model, which is shown at the error ...which has shape '(?, 2)'
and defined at the line where you create the last fully connected layer convnet = fully_connected(convnet, 2, activation='softmax')
.
so what should i do? can you help? i cant understand it clearly...
– Atif Ali
Nov 15 at 13:56
add a comment |
up vote
0
down vote
The problem is with the shape of your labels, so check the shape for y_train
and y_test
. Both of them should have to have a shape similar to the out put of your model, which is shown at the error ...which has shape '(?, 2)'
and defined at the line where you create the last fully connected layer convnet = fully_connected(convnet, 2, activation='softmax')
.
so what should i do? can you help? i cant understand it clearly...
– Atif Ali
Nov 15 at 13:56
add a comment |
up vote
0
down vote
up vote
0
down vote
The problem is with the shape of your labels, so check the shape for y_train
and y_test
. Both of them should have to have a shape similar to the out put of your model, which is shown at the error ...which has shape '(?, 2)'
and defined at the line where you create the last fully connected layer convnet = fully_connected(convnet, 2, activation='softmax')
.
The problem is with the shape of your labels, so check the shape for y_train
and y_test
. Both of them should have to have a shape similar to the out put of your model, which is shown at the error ...which has shape '(?, 2)'
and defined at the line where you create the last fully connected layer convnet = fully_connected(convnet, 2, activation='softmax')
.
answered Nov 13 at 11:20
D.negn
262
262
so what should i do? can you help? i cant understand it clearly...
– Atif Ali
Nov 15 at 13:56
add a comment |
so what should i do? can you help? i cant understand it clearly...
– Atif Ali
Nov 15 at 13:56
so what should i do? can you help? i cant understand it clearly...
– Atif Ali
Nov 15 at 13:56
so what should i do? can you help? i cant understand it clearly...
– Atif Ali
Nov 15 at 13:56
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242299%2fimage-classification-using-tensorflow-value-error%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NH3nfnayXuILpbVldRJyt VHmXlcpZRwHd2GdIKaVBB9,VA68y,b2sLJzf
Your network is expecting a 2D tensor, and you're giving it a 1D tensor. Does that make sense?
– Josh Friedlander
Nov 10 at 19:06
@JoshFriedlander can you tell me how to do that? can you provide me code?
– Atif Ali
Nov 10 at 19:57
@JoshFriedlander can you please tell which line should be changed to what exactly?
– Atif Ali
Nov 10 at 20:00